# Pandas_Alive
Animated plotting extension for Pandas with Matplotlib
**Pandas_Alive** is intended to provide a plotting backend for animated [matplotlib](https://matplotlib.org/) charts for [Pandas](https://pandas.pydata.org/) DataFrames, similar to the already [existing Visualization feature of Pandas](https://pandas.pydata.org/pandas-docs/stable/visualization.html).
With **Pandas_Alive**, creating stunning, animated visualisations is as easy as calling:
`df.plot_animated()`

## Installation
Install with `pip install pandas_alive`
## Usage
As this package builds upon [`bar_chart_race`](https://github.com/dexplo/bar_chart_race), the example data set is sourced from there.
Must begin with a pandas DataFrame containing 'wide' data where:
- Every row represents a single period of time
- Each column holds the value for a particular category
- The index contains the time component (optional)
The data below is an example of properly formatted data. It shows total deaths from COVID-19 for the highest 20 countries by date.

[Example Table](examples/example_dataset_table.md)
To produce the above visualisation:
- Check [Requirements](#requirements) first to ensure you have the tooling installed!
- Call `plot_animated()` on the DataFrame
- Either specify a file name to write to with `df.plot_animated(filename='example.mp4')` or use `df.plot_animated().get_html5_video` to return a HTML5 video
- Done!
``` python
import pandas_alive
covid_df = pandas_alive.load_dataset()
covid_df.plot_animated(filename='examples/example-barh-chart.gif')
```
### Currently Supported Chart Types
`pandas_alive` current supports:
- [Horizontal Bar Charts](#horizontal-bar-charts)
- [Vertical Bar Charts](#vertical-bar-charts)
- [Line Charts](#line-charts)
- [Scatter Charts](#scatter-charts)
#### Horizontal Bar Charts
``` python
import pandas_alive
covid_df = pandas_alive.load_dataset()
covid_df.plot_animated(filename='example-barh-chart.gif')
```

``` python
import pandas as pd
import pandas_alive
elec_df = pd.read_csv("data/Aus_Elec_Gen_1980_2018.csv",index_col=0,parse_dates=[0],thousands=',')
elec_df.fillna(0).plot_animated('examples/example-electricity-generated-australia.gif',period_fmt="%Y",title='Australian Electricity Generation Sources 1980-2018')
```

#### Vertical Bar Charts
``` python
import pandas_alive
covid_df = pandas_alive.load_dataset()
covid_df.plot_animated(filename='examples/example-barv-chart.gif',orientation='v')
```

#### Line Charts
With as many lines as data columns in the DataFrame.
``` python
import pandas_alive
covid_df = pandas_alive.load_dataset()
covid_df.diff().fillna(0).plot_animated(filename='examples/example-line-chart.gif',kind='line',period_label={'x':0.1,'y':0.9})
```

#### Scatter Charts
``` python
import pandas as pd
import pandas_alive
max_temp_df = pd.read_csv(
"data/Newcastle_Australia_Max_Temps.csv",
parse_dates={"Timestamp": ["Year", "Month", "Day"]},
)
min_temp_df = pd.read_csv(
"data/Newcastle_Australia_Min_Temps.csv",
parse_dates={"Timestamp": ["Year", "Month", "Day"]},
)
merged_temp_df = pd.merge_asof(max_temp_df, min_temp_df, on="Timestamp")
merged_temp_df.index = pd.to_datetime(merged_temp_df["Timestamp"].dt.strftime('%Y/%m/%d'))
keep_columns = ["Minimum temperature (Degree C)", "Maximum temperature (Degree C)"]
merged_temp_df[keep_columns].resample("Y").mean().plot_animated(filename='examples/example-scatter-chart.gif',kind="scatter",title='Max & Min Temperature Newcastle, Australia')
```

### Multiple Charts
`pandas_alive` supports multiple animated charts in a single visualisation.
- Create a list of all charts to include in animation
- Use `animate_multiple_plots` with a `filename` and the list of charts (this will use `matplotlib.subplots`)
- Done!
``` python
import pandas_alive
covid_df = pandas_alive.load_dataset()
animated_line_chart = covid_df.diff().fillna(0).plot_animated(kind='line',period_label=False)
animated_bar_chart = covid_df.plot_animated(kind='barh',n_visible=10)
pandas_alive.animate_multiple_plots('examples/example-bar-and-line-chart.gif',[animated_bar_chart,animated_line_chart])
```

``` python
import pandas_alive
urban_df = pandas_alive.load_dataset("urban_pop")
animated_line_chart = (
urban_df.sum(axis=1)
.pct_change()
.dropna()
.mul(100)
.plot_animated(kind="line", title="Total % Change in Population",period_label=False)
)
animated_bar_chart = urban_df.plot_animated(kind='barh',n_visible=10,title='Top 10 Populous Countries',period_fmt="%Y")
pandas_alive.animate_multiple_plots('examples/example-bar-and-line-urban-chart.gif',[animated_bar_chart,animated_line_chart],title='Urban Population 1977 - 2018',adjust_subplot_top=0.85)
```

## Inspiration
The inspiration for this project comes from:
- [bar_chart_race](https://github.com/dexplo/bar_chart_race) by [Ted Petrou](https://github.com/tdpetrou)
- [Pandas-Bokeh](https://github.com/PatrikHlobil/Pandas-Bokeh) by [Patrik Hlobil](https://github.com/PatrikHlobil)
## Requirements
If you get an error such as `TypeError: 'MovieWriterRegistry' object is not an iterator`, this signals there isn't a writer library installed on your machine.
This package utilises the [matplotlib.animation function](https://matplotlib.org/3.2.1/api/animation_api.html), thus requiring a writer library.
Ensure to have one of the supported tooling software installed prior to use!
- [ffmpeg](https://ffmpeg.org/)
- [ImageMagick](https://imagemagick.org/index.php)
- [Pillow](https://pillow.readthedocs.io/en/stable/)
- See more at <https://matplotlib.org/3.2.1/api/animation_api.html#writer-classes>
## Documentation
Documentation is provided at <https://jackmckew.github.io/pandas_alive/>
## Contributing
Pull requests are welcome! Please help to cover more and more chart types!
``` python
```
没有合适的资源?快使用搜索试试~ 我知道了~
pandas_alive-0.1.11.tar.gz
需积分: 1 0 下载量 39 浏览量
2024-03-11
16:21:56
上传
评论
收藏 18KB GZ 举报
温馨提示
Python库是一组预先编写的代码模块,旨在帮助开发者实现特定的编程任务,无需从零开始编写代码。这些库可以包括各种功能,如数学运算、文件操作、数据分析和网络编程等。Python社区提供了大量的第三方库,如NumPy、Pandas和Requests,极大地丰富了Python的应用领域,从数据科学到Web开发。Python库的丰富性是Python成为最受欢迎的编程语言之一的关键原因之一。这些库不仅为初学者提供了快速入门的途径,而且为经验丰富的开发者提供了强大的工具,以高效率、高质量地完成复杂任务。例如,Matplotlib和Seaborn库在数据可视化领域内非常受欢迎,它们提供了广泛的工具和技术,可以创建高度定制化的图表和图形,帮助数据科学家和分析师在数据探索和结果展示中更有效地传达信息。
资源推荐
资源详情
资源评论
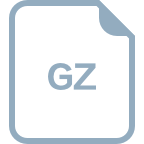
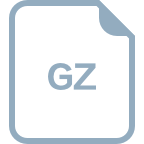
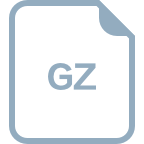
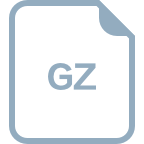
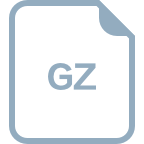
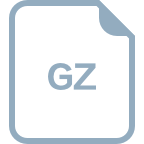
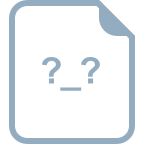
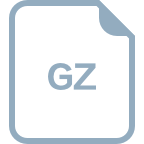
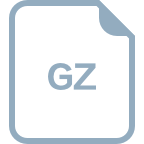
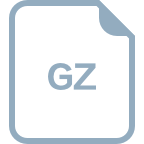
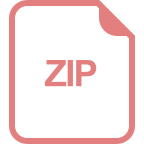
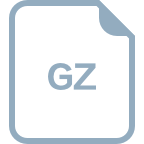
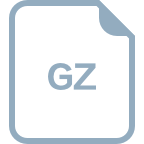
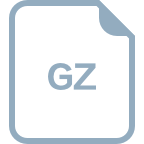
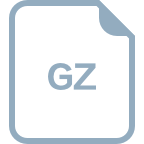
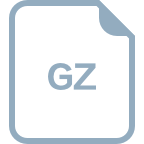
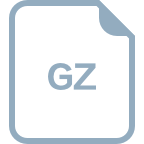
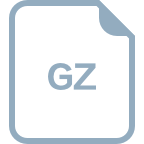
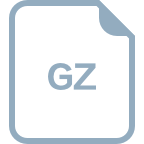
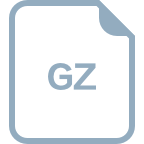
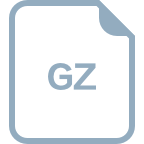
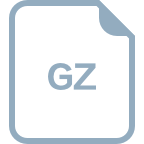
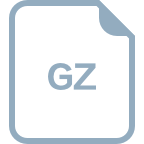
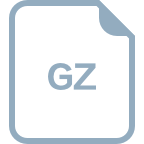
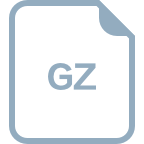
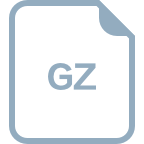
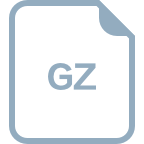
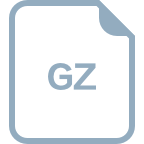
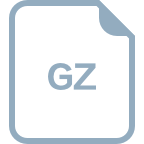
收起资源包目录


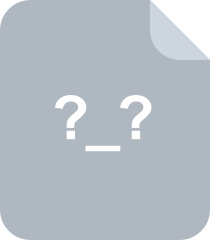
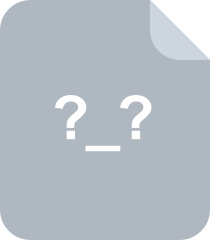
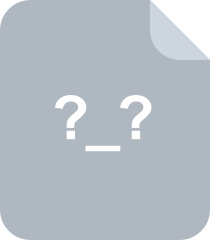

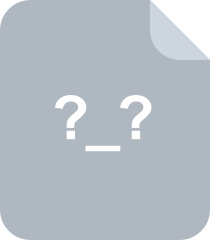
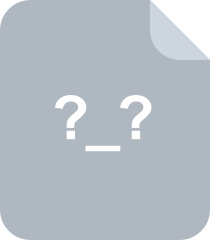
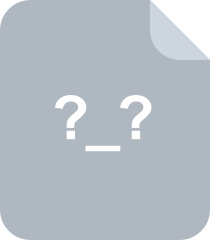
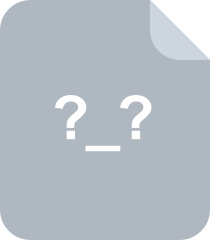
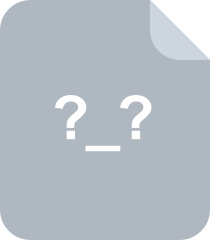
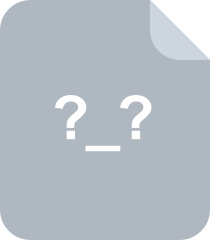
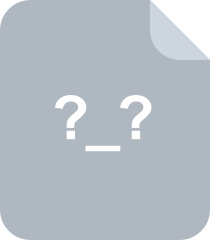
共 10 条
- 1
资源评论
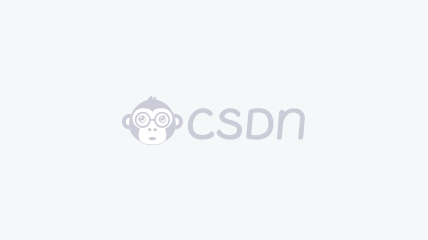

程序员Chino的日记
- 粉丝: 3688
- 资源: 5万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

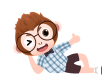
最新资源
- 数据分析案例-车辆燃油经济性数据可视化分析(数据集+代码).rar
- 一个vue全家桶入门演示!.zip
- Python MC 小游戏
- 一个前苏联分离的简单博客案例,适合刚入门vue,学前分离的童鞋!.zip
- 一个基于 SpringBooot 结合 vueJS2.x + webpack2.x 的 Java 全栈 Web 实践示例.zip
- Python FPS 小游戏
- 单片机原理与接口技术 51单片机RAM 数据存储区学习笔记.pdf
- 单片机原理与接口技术 51单片机的学习经验(附学习总结).docx
- 单片机原理与接口技术 51单片机的学习经验(附学习总结).pdf
- 单片机原理与接口技术 单片机作业整理.docx
- 防脉冲干扰移动平均值法数字滤波器的C语言算法及其实现.pdf
- 单片机原理与接口技术 51单片机开发总结(串口篇).docx
- 单片机原理与接口技术 汇编语言入门教程.docx
- 单片机原理与接口技术 汇编语言入门教程.pdf
- 单片机原理与接口技术 例说8051汇编版输入输出口应用 共77页.pptx
- 单片机原理与接口技术 51单片机RAM 数据存储区学习笔记.docx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


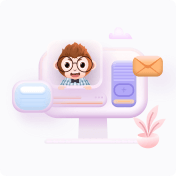
安全验证
文档复制为VIP权益,开通VIP直接复制
