"""SDMX Information Model (SDMX-IM).
This module implements many of the classes described in the SDMX-IM specification
('spec'), which is available from:
- https://sdmx.org/?page_id=5008
- https://sdmx.org/wp-content/uploads/
SDMX_2-1-1_SECTION_2_InformationModel_201108.pdf
Details of the implementation:
- Python typing and pydantic are used to enforce the types of attributes that reference
instances of other classes.
- Some classes have convenience attributes not mentioned in the spec, to ease navigation
between related objects. These are marked “:mod:`sdmx` extension not in the IM.”
- Class definitions are grouped by section of the spec, but these sections appear out
of order so that dependent classes are defined first.
"""
# TODO for complete implementation of the IM, enforce TimeKeyValue (instead of KeyValue)
# for {Generic,StructureSpecific} TimeSeriesDataSet.
import logging
from collections import ChainMap
from collections.abc import Collection
from collections.abc import Iterable as IterableABC
from copy import copy
from datetime import date, datetime, timedelta
from enum import Enum
from functools import lru_cache
from inspect import isclass
from itertools import product
from operator import attrgetter, itemgetter
from typing import (
Any,
Dict,
Generator,
Generic,
Iterable,
List,
Mapping,
Optional,
Sequence,
Set,
Tuple,
Type,
TypeVar,
Union,
)
from warnings import warn
from pandasdmx.util import (
BaseModel,
DictLike,
compare,
dictlike_field,
only,
Resource,
validate_dictlike,
validator,
)
log = logging.getLogger(__name__)
# TODO read this from the environment, or use any value set in the SDMX-ML spec.
# Currently set to 'en' because test_dsd.py expects it.
DEFAULT_LOCALE = "en"
# Configure validation level (new in v1.8.0)
# This is currently used only to prevent URN matching
ValidationLevels = Enum("ValidationLevels", "strict sloppy")
DEFAULT_VAL_LEVEL = ValidationLevels.sloppy
# §3.2: Base structures
class InternationalString:
"""SDMX-IM InternationalString.
SDMX-IM LocalisedString is not implemented. Instead, the 'localizations' is a
mapping where:
- keys correspond to the 'locale' property of LocalisedString.
- values correspond to the 'label' property of LocalisedString.
When used as a type hint with pydantic, InternationalString fields can be assigned
to in one of four ways::
class Foo(BaseModel):
name: InternationalString = InternationalString()
# Equivalent: no localizations
f = Foo()
f = Foo(name={})
# Using an explicit locale
f.name['en'] = "Foo's name in English"
# Using a (locale, label) tuple
f.name = ('fr', "Foo's name in French")
# Using a dict
f.name = {'en': "Replacement English name",
'fr': "Replacement French name"}
# Using a bare string, implicitly for the DEFAULT_LOCALE
f.name = "Name in DEFAULT_LOCALE language"
Only the first method preserves existing localizations; the latter three replace
them.
"""
localizations: Dict[str, str] = {}
def __init__(self, value=None, **kwargs):
super().__init__()
# Handle initial values according to type
if isinstance(value, str):
# Bare string
value = {DEFAULT_LOCALE: value}
elif (
isinstance(value, Collection)
and len(value) == 2
and isinstance(value[0], str)
):
# 2-tuple of str is (locale, label)
value = {value[0]: value[1]}
elif isinstance(value, dict):
# dict; use directly
pass
elif isinstance(value, IterableABC):
# Iterable of 2-tuples
value = {locale: label for (locale, label) in value}
elif value is None:
# Keyword arguments → dict, possibly empty
value = dict(kwargs)
else:
raise ValueError(value, kwargs)
self.localizations = value
# Convenience access
def __getitem__(self, locale):
return self.localizations[locale]
def __setitem__(self, locale, label):
self.localizations[locale] = label
# Duplicate of __getitem__, to pass existing tests in test_dsd.py
def __getattr__(self, name):
try:
return self.__dict__["localizations"][name]
except KeyError:
raise AttributeError(name) from None
def __add__(self, other):
result = copy(self)
result.localizations.update(other.localizations)
return result
def localized_default(self, locale=None):
"""Return the string in *locale* if not empty, or else the first defined."""
if locale and (locale in self.localizations) and self.localizations[locale]:
return self.localizations[locale]
if len(self.localizations):
# No label in the default locale; use the first stored non-empty str value
return next(filter(None, self.localizations.values()))
else:
return ""
def __str__(self):
return self.localized_default(DEFAULT_LOCALE)
def __repr__(self):
return "\n".join(
["{}: {}".format(*kv) for kv in sorted(self.localizations.items())]
)
def __eq__(self, other):
try:
return self.localizations == other.localizations
except AttributeError:
return NotImplemented
@classmethod
def __get_validators__(cls):
yield cls.__validate
@classmethod
def __validate(cls, value, values, config, field):
# Any value that the constructor can handle can be assigned
if not isinstance(value, InternationalString):
value = InternationalString(value)
try:
# Update existing value
existing = values[field.name]
existing.localizations.update(value.localizations)
return existing
except KeyError:
# No existing value/None; return the assigned value
return value
class Annotation(BaseModel):
#: Can be used to disambiguate multiple annotations for one AnnotableArtefact.
id: Optional[str] = None
#: Title, used to identify an annotation.
title: Optional[str] = None
#: Specifies how the annotation is processed.
type: Optional[str] = None
#: A link to external descriptive text.
url: Optional[str] = None
#: Content of the annotation.
text: InternationalString = InternationalString()
class AnnotableArtefact(BaseModel):
#: :class:`Annotations <.Annotation>` of the object.
#:
#: :mod:`pandaSDMX` implementation: The IM does not specify the name of this
#: feature.
annotations: List[Annotation] = []
def get_annotation(self, **attrib):
"""Return a :class:`Annotation` with given `attrib`, e.g. 'id'.
If more than one `attrib` is given, all must match a particular annotation.
Raises
------
KeyError
If there is no matching annotation.
"""
for anno in self.annotations:
if all(getattr(anno, key, None) == value for key, value in attrib.items()):
return anno
raise KeyError(attrib)
def pop_annotation(self, **attrib):
"""Remove and return a :class:`Annotation` with given `attrib`, e.g. 'id'.
If more than one `attrib` is given, all must match a particular annotation.
Raises
------
KeyError
If there is no matching annotation.
"""
for i, anno in enum

程序员Chino的日记
- 粉丝: 3739
- 资源: 5万+
最新资源
- 三相桥式全控整流电路simulink仿真(阻性 阻感性负载) 2021版本
- 基于二阶锥规划的主动配电网最优潮流求解 参考文献:主动配电网多源协同运行优化研究-乔珊 摘要:最优潮流研究在配 电网规划运行 中不可或缺 , 且在大量分布式能源接入 的主动配 电网环境下尤 为重要
- 混合动力汽车性能分析与simulink建模,采用成熟软件架构,利用桌面应用程序接收用户配置,并自动控制MATLAB运行,并将结果返回桌面应用程序进行显示 以每个部件的模型、控制模型、初始化、前 后处
- 基于卷积神经网络CNN的数据回归预测 多输入单输出预测 代码含详细注释,不负责 数据存入Excel,替方便,指标计算有决定系数R2,平均绝对误差MAE,平均相对误差MBE
- 混合储能,simulink模型储能并网,混合储能能量管理 电池与超级电容混合储能并网matlab simulink仿真模型 (1)混合储能采用低通滤波器进行功率分配,可有效抑制系统功率波动,实现母
- STM32低成本MD500E永磁同步,单电阻采样,无感算法方案,高性价比变频器方案 md500e单电阻采样:精简移植了md500e的无感svc部分到f103中,值得研究学习,电子资料,出不 包括
- 多孔集流体模型模拟锌枝晶生长过程,仿真锌离子在电极表面吸附沉积的过程,通过三次电流分布接口,相场接口进行仿真,对比锌枝晶文献可以肉眼可见的清晰模拟出锌表面沉积过程
- 光伏板向蓄电池充电,恒流恒压法 根据网上频搭建的,可以跟着学,内有一些自己的理解注释 2018b
- 企业展示型百度小程序 智能小程序 小程序 模板 源代码下载
- 无电网电压传感器三相PWM整流器,采用磁链方法估算电网相位角度 模型控制器部分全部采用离散化处理,设置成单采样单更新模式,SVPWM调制模式,开关频率固定,使用的是矢量控制技术 该模型SVPWM模
- Matlab仿真:转速闭环转差频率控制异步电动机的矢量控制(付设计说明) 2021b及以上版本
- 永磁同步电机多物理场仿真案例,电磁-谐响应-噪声分析(NVH分析),该案例可以用于学习,具体参数见第一张图
- 自立袋产品品自动装盒并装箱sw22全套技术资料100%好用.zip
- 制作abaqus隧道CD法开挖,CD法开挖讲 解,CD法开挖模型,step by step,CRD法开挖模型,台阶法开挖,环形开挖预留核心土法开挖,模型,讲 解详细
- 考虑充电需求差异性的电动汽车协同充放电调度方法 关键词:充电需求差异性 电动汽车协同充放电 调度 仿真软件: matlab + yalmip +cplex 研究内容:代码提出了一种微电网中电动汽车的协
- FactoryIO自动分拣+堆垛机+入库仿真,PLC学习最佳模型 使用简单的梯形图与SCL语言编写,通俗易懂,写有详细注释,起到抛砖引玉的作用,比较适合有动手能力的入门初学者,和入门学习,程序可以无限
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


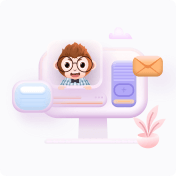