Pandas Downcast
===============
[](https://pypi.python.org/pypi/pandas-downcast)
[](https://pypi.python.org/pypi/pandas-downcast/)
[](https://travis-ci.com/domvwt/pandas-downcast)
[](https://codecov.io/gh/domvwt/pandas-downcast)
Shrink [Pandas](https://pandas.pydata.org/) DataFrames with intelligent schema inference.
`pandas-downcast` finds the minimum viable type for each column, ensuring that resulting values
are within tolerance of original values.
## Installation
```bash
pip install pandas-downcast
```
## Dependencies
* python >= 3.6
* pandas
* numpy
## License
[MIT](https://opensource.org/licenses/MIT)
## Usage
```python
import pdcast as pdc
import numpy as np
import pandas as pd
data = {
"integers": np.linspace(1, 100, 100),
"floats": np.linspace(1, 1000, 100).round(2),
"booleans": np.random.choice([1, 0], 100),
"categories": np.random.choice(["foo", "bar", "baz"], 100),
}
df = pd.DataFrame(data)
# Downcast DataFrame to minimum viable schema.
df_downcast = pdc.downcast(df)
# Infer minimum schema from DataFrame.
schema = pdc.infer_schema(df)
# Coerce DataFrame to schema - required if converting float to Pandas Integer.
df_new = pdc.coerce_df(df, schema)
```
Smaller data types == smaller memory footprint.
```python
df.info()
# <class 'pandas.core.frame.DataFrame'>
# RangeIndex: 100 entries, 0 to 99
# Data columns (total 4 columns):
# # Column Non-Null Count Dtype
# --- ------ -------------- -----
# 0 integers 100 non-null float64
# 1 floats 100 non-null float64
# 2 booleans 100 non-null int64
# 3 categories 100 non-null object
# dtypes: float64(2), int64(1), object(1)
# memory usage: 3.2+ KB
df_downcast.info()
# <class 'pandas.core.frame.DataFrame'>
# RangeIndex: 100 entries, 0 to 99
# Data columns (total 4 columns):
# # Column Non-Null Count Dtype
# --- ------ -------------- -----
# 0 integers 100 non-null uint8
# 1 floats 100 non-null float32
# 2 booleans 100 non-null bool
# 3 categories 100 non-null category
# dtypes: bool(1), category(1), float32(1), uint8(1)
# memory usage: 932.0 bytes
```
Numerical data types will be downcast if the resulting values are within tolerance of the original values.
For details on tolerance for numeric comparison, see the notes on [`np.allclose`](https://numpy.org/doc/stable/reference/generated/numpy.allclose.html).
```python
print(df.head())
# integers floats booleans categories
# 0 1.0 1.00 1 foo
# 1 2.0 11.09 0 baz
# 2 3.0 21.18 1 bar
# 3 4.0 31.27 0 bar
# 4 5.0 41.36 0 foo
print(df_downcast.head())
# integers floats booleans categories
# 0 1 1.000000 True foo
# 1 2 11.090000 False baz
# 2 3 21.180000 True bar
# 3 4 31.270000 False bar
# 4 5 41.360001 False foo
print(pdc.options.ATOL)
# >>> 1e-08
print(pdc.options.RTOL)
# >>> 1e-05
```
Tolerance can be set at module level or passed in function arguments:
```python
pdc.options.ATOL = 1e-10
pdc.options.RTOL = 1e-10
df_downcast_new = pdc.downcast(df)
```
Or
```python
infer_dtype_kws = {
"ATOL": 1e-10,
"RTOL": 1e-10
}
df_downcast_new = pdc.downcast(df, infer_dtype_kws=infer_dtype_kws)
```
The `floats` column is now kept as `float64` to meet the tolerance requirement.
Values in the `integers` column are still safely cast to `uint8`.
```python
df_downcast_new.info()
# <class 'pandas.core.frame.DataFrame'>
# RangeIndex: 100 entries, 0 to 99
# Data columns (total 4 columns):
# # Column Non-Null Count Dtype
# --- ------ -------------- -----
# 0 integers 100 non-null uint8
# 1 floats 100 non-null float64
# 2 booleans 100 non-null bool
# 3 categories 100 non-null category
# dtypes: bool(1), category(1), float64(1), uint8(1)
# memory usage: 1.3 KB
```
## Example
The following example shows how downcasting data often leads to size reductions of **greater than 70%**, depending on the original types.
```python
import pdcast as pdc
import pandas as pd
import seaborn as sns
df_dict = {df: sns.load_dataset(df) for df in sns.get_dataset_names()}
results = []
for name, df in df_dict.items():
mem_usage_pre = df.memory_usage(deep=True).sum()
df_post = pdc.downcast(df)
mem_usage_post = df_post.memory_usage(deep=True).sum()
shrinkage = int((1 - (mem_usage_post / mem_usage_pre)) * 100)
results.append(
{"dataset": name, "size_pre": mem_usage_pre, "size_post": mem_usage_post, "shrink_pct": shrinkage}
)
results_df = pd.DataFrame(results).sort_values("shrink_pct", ascending=False).reset_index(drop=True)
print(results_df)
```
```
dataset size_pre size_post shrink_pct
0 fmri 213232 14776 93
1 titanic 321240 28162 91
2 attention 5888 696 88
3 penguins 75711 9131 87
4 dots 122240 17488 85
5 geyser 21172 3051 85
6 gammas 500128 108386 78
7 anagrams 2048 456 77
8 planets 112663 30168 73
9 anscombe 3428 964 71
10 iris 14728 5354 63
11 exercise 3302 1412 57
12 flights 3616 1888 47
13 mpg 75756 43842 42
14 tips 7969 6261 21
15 diamonds 3184588 2860948 10
16 brain_networks 4330642 4330642 0
17 car_crashes 5993 5993 0
```

程序员Chino的日记
- 粉丝: 3743
- 资源: 5万+
最新资源
- 基于粒子群算法的电动汽车充电站和光伏最优选址和定容 关键词:选址定容 电动汽车 充电站位置 仿真平台:MATLAB 主要内容:代码主要做的是一个电动汽车充电站和分布式光伏的选址定容问题,提出了
- 伺服送料机,步进电机,伺服电机,程序,三菱,台达,中达一体机,送料机程序,PLC多段数据不同,可任意调节A段B段c段长度,并定长切断 程序能存储5段工件数据,使用调出非常方便 PLC程序有台达ES
- 考虑安全约束及热备用的电力系统机组组合研究 关键词:机组组合 直流潮流 优化调度 参考文档:店主自编文档,模型数据清晰明了 仿真平台:MATLAB+CPLEX gurobi平台 优势:代码具有一定
- 计及源-荷双重不确定性的电厂 微网日前随机优化调度系统 关键词:电厂 微网 随机优化 随机调度 源-荷双重不确定性 电厂调度 参考文档:Virtual power plant mid-ter
- 基于mpc模型预测轨迹跟踪控制,总共包含两套仿真,一套是不加入四轮侧偏角软约束,一套是加入四轮侧偏角的软约束控制,通过carsim与simulink联合仿真发现加入侧偏角软约束在进行轨迹跟踪时,能够通
- 采用下垂控制的孤岛逆变器仿真 名称:droop-controlled-converter-island 软件:Matlab R2016a 控制:下垂控制,闭环电流反馈控制,解耦电压电流环控制,见图1
- 直驱式波浪发电最大功率捕获matlab仿真 电机:直线电机 控制器:PID控制器 策略:基于RLC等效电路模型的最大功率输出 含:使用说明书+教学视频
- 西门子200smart标准程序,西门子程序模板参考,3轴控制程序,含西门子触摸屏程序,详细注释,IO表,电气原理图
- 基于西门子PLC200自动保暖供水系统,系统用于厂区饮用水,区域热水保暖,系统中大多数用于时间进行各个季节,各个时间的控制 供水区域时间的设定 可以实现在每一个阶段按照每一个流程进行不同的运行
- 西门子S7-1200四层电梯模拟程序 电梯WinCC动画程序 西门子参考学习程序 博图15或者以上可以打开 PLC:西门子S7-1200 触摸屏:KTP900 有人会问:为什么是四层电梯参考学习程序
- 整车电子电气正向开发网络架构 , 倘若您是产品经理或者项目经理又或者是技术leader,这个将帮助您梳理在整车电子电气正向开发过程中不同系统的内部架构设计及相互间的关联,涵盖整车控制系统、网联系统、驾
- dsp28335三相逆变程序,可以开环测试
- 含分布式电源的无功补偿(Matlab程序): 1.以无功补偿调节代价为目标函数,不同风光电源渗透率下,优化确定无功补偿装置出力情况(改进灰狼优化算法IGWO) 2.以网损和电压偏差为目标函数,才用分
- 整车控制器 基于MPC和滑模控制算法实现的车辆稳定性控制,建立了横摆角速度、侧向速度、前后质心侧偏角动力学模型作为预测模型,同时考虑车辆的稳定性可通过控制车辆的侧向速度维持在一定范围内保证车辆的稳定性
- COMSOL MATLAB 代码 二维随机裂隙 2维随机裂隙生成 功能:可以实现多组不同方向,不同分布规律的裂隙生成(任意组数都可以) 需要输入的参数有:每组裂隙的迹长范围、分布规律(正态分布o
- 全阶滑模无位置传感器控制仿真模型,有基本的开关函数,有饱和函数,sigmod函数等多种滑模 还有全阶滑模观测器仿真,相比传统滑模观测器消除了额外的低通滤波器,误差更小,效果堪称完美 不仅误差小
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


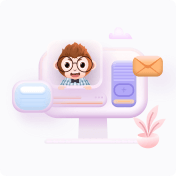