/* $Id: winio.c,v 1.576 2006/11/10 20:13:38 dolorous Exp $ */
/**************************************************************************
* winio.c *
* *
* Copyright (C) 1999, 2000, 2001, 2002, 2003, 2004 Chris Allegretta *
* Copyright (C) 2005, 2006 David Lawrence Ramsey *
* This program is free software; you can redistribute it and/or modify *
* it under the terms of the GNU General Public License as published by *
* the Free Software Foundation; either version 2, or (at your option) *
* any later version. *
* *
* This program is distributed in the hope that it will be useful, but *
* WITHOUT ANY WARRANTY; without even the implied warranty of *
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU *
* General Public License for more details. *
* *
* You should have received a copy of the GNU General Public License *
* along with this program; if not, write to the Free Software *
* Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA *
* 02110-1301, USA. *
* *
**************************************************************************/
#include "proto.h"
#include <stdio.h>
#include <stdarg.h>
#include <string.h>
#include <unistd.h>
#include <ctype.h>
static int *key_buffer = NULL;
/* The keystroke buffer, containing all the keystrokes we have
* at a given point. */
static size_t key_buffer_len = 0;
/* The length of the keystroke buffer. */
static int statusblank = 0;
/* The number of keystrokes left after we call statusbar(),
* before we actually blank the statusbar. */
static bool disable_cursorpos = FALSE;
/* Should we temporarily disable constant cursor position
* display? */
/* Control character compatibility:
*
* - NANO_BACKSPACE_KEY is Ctrl-H, which is Backspace under ASCII, ANSI,
* VT100, and VT220.
* - NANO_TAB_KEY is Ctrl-I, which is Tab under ASCII, ANSI, VT100,
* VT220, and VT320.
* - NANO_ENTER_KEY is Ctrl-M, which is Enter under ASCII, ANSI, VT100,
* VT220, and VT320.
* - NANO_XON_KEY is Ctrl-Q, which is XON under ASCII, ANSI, VT100,
* VT220, and VT320.
* - NANO_XOFF_KEY is Ctrl-S, which is XOFF under ASCII, ANSI, VT100,
* VT220, and VT320.
* - NANO_CONTROL_8 is Ctrl-8 (Ctrl-?), which is Delete under ASCII,
* ANSI, VT100, and VT220, and which is Backspace under VT320.
*
* Note: VT220 and VT320 also generate Esc [ 3 ~ for Delete. By
* default, xterm assumes it's running on a VT320 and generates Ctrl-8
* (Ctrl-?) for Backspace and Esc [ 3 ~ for Delete. This causes
* problems for VT100-derived terminals such as the FreeBSD console,
* which expect Ctrl-H for Backspace and Ctrl-8 (Ctrl-?) for Delete, and
* on which the VT320 sequences are translated by the keypad to KEY_DC
* and [nothing]. We work around this conflict via the REBIND_DELETE
* flag: if it's not set, we assume VT320 compatibility, and if it is,
* we assume VT100 compatibility. Thanks to Lee Nelson and Wouter van
* Hemel for helping work this conflict out.
*
* Escape sequence compatibility:
*
* We support escape sequences for ANSI, VT100, VT220, VT320, the Linux
* console, the FreeBSD console, the Mach console, xterm, rxvt, and
* Eterm. Among these, there are several conflicts and omissions,
* outlined as follows:
*
* - Tab on ANSI == PageUp on FreeBSD console; the former is omitted.
* (Ctrl-I is also Tab on ANSI, which we already support.)
* - PageDown on FreeBSD console == Center (5) on numeric keypad with
* NumLock off on Linux console; the latter is omitted. (The editing
* keypad key is more important to have working than the numeric
* keypad key, because the latter has no value when NumLock is off.)
* - F1 on FreeBSD console == the mouse key on xterm/rxvt/Eterm; the
* latter is omitted. (Mouse input will only work properly if the
* extended keypad value KEY_MOUSE is generated on mouse events
* instead of the escape sequence.)
* - F9 on FreeBSD console == PageDown on Mach console; the former is
* omitted. (The editing keypad is more important to have working
* than the function keys, because the functions of the former are not
* arbitrary and the functions of the latter are.)
* - F10 on FreeBSD console == PageUp on Mach console; the former is
* omitted. (Same as above.)
* - F13 on FreeBSD console == End on Mach console; the former is
* omitted. (Same as above.)
* - F15 on FreeBSD console == Shift-Up on rxvt/Eterm; the former is
* omitted. (The arrow keys, with or without modifiers, are more
* important to have working than the function keys, because the
* functions of the former are not arbitrary and the functions of the
* latter are.)
* - F16 on FreeBSD console == Shift-Down on rxvt/Eterm; the former is
* omitted. (Same as above.) */
/* Read in a sequence of keystrokes from win and save them in the
* keystroke buffer. This should only be called when the keystroke
* buffer is empty. */
void get_key_buffer(WINDOW *win)
{
int input;
size_t errcount;
/* If the keystroke buffer isn't empty, get out. */
if (key_buffer != NULL)
return;
/* Read in the first character using blocking input. */
#ifndef NANO_TINY
allow_pending_sigwinch(TRUE);
#endif
/* Just before reading in the first character, display any pending
* screen updates. */
doupdate();
errcount = 0;
while ((input = wgetch(win)) == ERR) {
errcount++;
/* If we've failed to get a character MAX_BUF_SIZE times in a
* row, assume that the input source we were using is gone and
* die gracefully. We could check if errno is set to EIO
* ("Input/output error") and die gracefully in that case, but
* it's not always set properly. Argh. */
if (errcount == MAX_BUF_SIZE)
handle_hupterm(0);
}
#ifndef NANO_TINY
allow_pending_sigwinch(FALSE);
#endif
/* Increment the length of the keystroke buffer, save the value of
* the keystroke in key, and set key_code to TRUE if the keystroke
* is an extended keypad value or FALSE if it isn't. */
key_buffer_len++;
key_buffer = (int *)nmalloc(sizeof(int));
key_buffer[0] = input;
/* Read in the remaining characters using non-blocking input. */
nodelay(win, TRUE);
while (TRUE) {
#ifndef NANO_TINY
allow_pending_sigwinch(TRUE);
#endif
input = wgetch(win);
/* If there aren't any more characters, stop reading. */
if (input == ERR)
break;
/* Otherwise, increment the length of the keystroke buffer, save
* the value of the keystroke in key, and set key_code to TRUE
* if the keystroke is an extended keypad value or FALSE if it
* isn't. */
key_buffer_len++;
key_buffer = (int *)nrealloc(key_buffer, key_buffer_len *
sizeof(int));
key_buffer[key_buffer_len - 1] = input;
#ifndef NANO_TINY
allow_pending_sigwinch(FALSE);
#endif
}
/* Switch back to non-blocking input. */
nodelay(win, FALSE);
#ifdef DEBUG
fprintf(stderr, "get_key_buffer(): key_buffer_len = %lu\n", (unsigned long)key_buffer_len);
#endif
}
/* Return the length of the keystroke buffer. */
size_t get_key_buffer_len(void)
{
return key_buffer_len;
}
/* Add the keystrokes in input to the keystroke buffer. */
void unget_input(int *input, size_t input_len)
{
#ifndef NANO_TINY
allow_pending_sigwinch(TRUE);
allow_pending_sigwinch(FALSE);
#endif
/* If input is empty, get out. */
if (input_len == 0)
return;
/* If adding input would put the keystroke buffer

程序员Chino的日记
- 粉丝: 3754
- 资源: 5万+
最新资源
- 学生宿舍管理系统(毕业设计).zip
- 跨年烟花代码(python)
- springboot-二手物品交易系统的设计与实现-h7ow81fl.zip
- 鲸鱼算法(WOA)优化混合核极限学习机(HKELM)分类预测,多特征输入模型,WOA-HKELM分类预测 多特征输入单输出的
- Android学习笔记
- B站视频助手,视频下载助理
- 双碳目标下综合能源系统低碳运行优化调度Matlab程序,包含光伏、风电、热电联产、燃气锅炉、电锅炉、电储能、碳捕集设备,考虑碳交易 以系统运行成本最小为目标进行调度 没有具体参考文献,这个只是一个授之
- springboot-企业员工薪酬管理系统-n4s02htu.zip
- 基于格雷码的结构光三维重建源码,MATLAB可以跑通
- springboot-人才公寓管理系统-897cjl4r.zip
- 灰狼优化算法GWO算法具有结构简单、需要调节的参数少,容易实现等特点,其中存在能够自适应调整的收敛因子以及信息反馈机制,能够在局部寻优与全局搜索之间实现平衡,因此在对问题的求解精度和收敛速度方面都有良
- 新版Android开发教程与学习笔记
- 差分进化算法DE(Differential Evolution)算法是在遗传算法等进化思想的基础上提出的,本质是一种多目标(连续变量)优化算法,用于求解多维空间中整体最优解 Matlab 程序
- 半桥 全桥LLC电路 谐振变器仿真,采用频率控制(PFM变频控制)输出电压闭环,软开关 原边半桥+副边半波整流 原边半桥+副边全桥整流 原边全桥+副边半波整流 原边全桥+副边全桥整流都有 matlab
- 适合练手、课程设计、毕业设计的Java项目源码:进销存管理系统(jsp+mssql).rar
- video_250112_204741.mp4
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


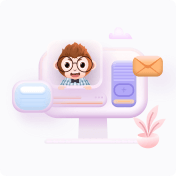