/* Extended regular expression matching and search library.
Copyright (C) 2002, 2003, 2004, 2005, 2006 Free Software Foundation, Inc.
This file is part of the GNU C Library.
Contributed by Isamu Hasegawa <isamu@yamato.ibm.com>.
This program is free software; you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation; either version 2, or (at your option)
any later version.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License along
with this program; if not, write to the Free Software Foundation,
Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. */
static reg_errcode_t match_ctx_init (re_match_context_t *cache, int eflags,
Idx n) internal_function;
static void match_ctx_clean (re_match_context_t *mctx) internal_function;
static void match_ctx_free (re_match_context_t *cache) internal_function;
static reg_errcode_t match_ctx_add_entry (re_match_context_t *cache, Idx node,
Idx str_idx, Idx from, Idx to)
internal_function;
static Idx search_cur_bkref_entry (const re_match_context_t *mctx, Idx str_idx)
internal_function;
static reg_errcode_t match_ctx_add_subtop (re_match_context_t *mctx, Idx node,
Idx str_idx) internal_function;
static re_sub_match_last_t * match_ctx_add_sublast (re_sub_match_top_t *subtop,
Idx node, Idx str_idx)
internal_function;
static void sift_ctx_init (re_sift_context_t *sctx, re_dfastate_t **sifted_sts,
re_dfastate_t **limited_sts, Idx last_node,
Idx last_str_idx)
internal_function;
static reg_errcode_t re_search_internal (const regex_t *preg,
const char *string, Idx length,
Idx start, Idx last_start, Idx stop,
size_t nmatch, regmatch_t pmatch[],
int eflags) internal_function;
static regoff_t re_search_2_stub (struct re_pattern_buffer *bufp,
const char *string1, Idx length1,
const char *string2, Idx length2,
Idx start, regoff_t range,
struct re_registers *regs,
Idx stop, bool ret_len) internal_function;
static regoff_t re_search_stub (struct re_pattern_buffer *bufp,
const char *string, Idx length, Idx start,
regoff_t range, Idx stop,
struct re_registers *regs,
bool ret_len) internal_function;
static unsigned int re_copy_regs (struct re_registers *regs, regmatch_t *pmatch,
Idx nregs, int regs_allocated)
internal_function;
static reg_errcode_t prune_impossible_nodes (re_match_context_t *mctx)
internal_function;
static Idx check_matching (re_match_context_t *mctx, bool fl_longest_match,
Idx *p_match_first) internal_function;
static Idx check_halt_state_context (const re_match_context_t *mctx,
const re_dfastate_t *state, Idx idx)
internal_function;
static void update_regs (const re_dfa_t *dfa, regmatch_t *pmatch,
regmatch_t *prev_idx_match, Idx cur_node,
Idx cur_idx, Idx nmatch) internal_function;
static reg_errcode_t push_fail_stack (struct re_fail_stack_t *fs,
Idx str_idx, Idx dest_node, Idx nregs,
regmatch_t *regs,
re_node_set *eps_via_nodes)
internal_function;
static reg_errcode_t set_regs (const regex_t *preg,
const re_match_context_t *mctx,
size_t nmatch, regmatch_t *pmatch,
bool fl_backtrack) internal_function;
static reg_errcode_t free_fail_stack_return (struct re_fail_stack_t *fs)
internal_function;
#ifdef RE_ENABLE_I18N
static int sift_states_iter_mb (const re_match_context_t *mctx,
re_sift_context_t *sctx,
Idx node_idx, Idx str_idx, Idx max_str_idx)
internal_function;
#endif /* RE_ENABLE_I18N */
static reg_errcode_t sift_states_backward (const re_match_context_t *mctx,
re_sift_context_t *sctx)
internal_function;
static reg_errcode_t build_sifted_states (const re_match_context_t *mctx,
re_sift_context_t *sctx, Idx str_idx,
re_node_set *cur_dest)
internal_function;
static reg_errcode_t update_cur_sifted_state (const re_match_context_t *mctx,
re_sift_context_t *sctx,
Idx str_idx,
re_node_set *dest_nodes)
internal_function;
static reg_errcode_t add_epsilon_src_nodes (const re_dfa_t *dfa,
re_node_set *dest_nodes,
const re_node_set *candidates)
internal_function;
static bool check_dst_limits (const re_match_context_t *mctx,
const re_node_set *limits,
Idx dst_node, Idx dst_idx, Idx src_node,
Idx src_idx) internal_function;
static int check_dst_limits_calc_pos_1 (const re_match_context_t *mctx,
int boundaries, Idx subexp_idx,
Idx from_node, Idx bkref_idx)
internal_function;
static int check_dst_limits_calc_pos (const re_match_context_t *mctx,
Idx limit, Idx subexp_idx,
Idx node, Idx str_idx,
Idx bkref_idx) internal_function;
static reg_errcode_t check_subexp_limits (const re_dfa_t *dfa,
re_node_set *dest_nodes,
const re_node_set *candidates,
re_node_set *limits,
struct re_backref_cache_entry *bkref_ents,
Idx str_idx) internal_function;
static reg_errcode_t sift_states_bkref (const re_match_context_t *mctx,
re_sift_context_t *sctx,
Idx str_idx, const re_node_set *candidates)
internal_function;
static reg_errcode_t merge_state_array (const re_dfa_t *dfa,
re_dfastate_t **dst,
re_dfastate_t **src, Idx num)
internal_function;
static re_dfastate_t *find_recover_state (reg_errcode_t *err,
re_match_context_t *mctx) internal_function;
static re_dfastate_t *transit_state (reg_errcode_t *err,
re_match_context_t *mctx,
re_dfastate_t *state) internal_function;
static re_dfastate_t *merge_state_with_log (reg_errcode_t *err,
re_match_context_t *mctx,
re_dfastate_t *next_state)
internal_function;
static reg_errcode_t check_subexp_matching_top (re_match_context_t *mctx,
re_node_set *cur_nodes,
Idx str_idx) internal_function;
#if 0
static re_dfastate_t *transit_state_sb (reg_errcode_t *err,
re_match_context_t *mctx,
re_dfastate_t *pstate)
internal_function;
#endif
#ifdef RE_ENABLE_I18N
static reg_errcode_t transit_state_mb (re_match_context_t *mctx,
re_dfastate_t *pstate)
internal_function;
#endif /* RE_ENABLE_I18N */
static reg_errcode_t transit_state_bkref (re_match_context_t *mctx,
const re_node_set *nodes)
internal_function;
static reg_errcode_t get_subexp (re_match_context_t *mctx,
Idx bkref_node, Idx bkref_str_idx)
internal_function;
static reg_errcode_t get_subexp_sub (re_match_context_t *mctx,
const re_sub_match_top_t *sub_top,
re_sub_match_last_t *sub_last,
Idx bkref_node, Idx bkref_str)
internal_function;
static Idx find_subexp_node (const re_dfa_t *dfa, const re_node_set *nodes,
Idx subexp_idx, int type) internal_function;
static reg_errcode_t check_arrival (re_match_context_t *mctx,
state_array_t *path, Idx top_node,
Idx top_str, Idx last_node, Idx last_str,
int type) internal_function;
static reg_errcode_t check_arrival_add_next_nodes (re_match_context_t *mctx,
Idx str_idx,
re_node_set *cur_nodes,
re_node_set *next_nodes)
internal_function;
static reg_errcode_t check_arrival_expand_ecl (const re_dfa_t *dfa,
re_node_set *cur_nodes,
Idx ex_subexp, int type)
internal_function;
static reg_errcode_t check_arrival_expand_ecl_sub (const re_dfa_t *dfa,
re_node_set *dst_nodes,
Idx target, Idx ex_subexp,
int type) internal_function;
static reg_errcode_t expand_bkref_cache (re_match_context_t *mctx,
re_node_set *cur_n
没有合适的资源?快使用搜索试试~ 我知道了~
m4-1.4.6.tar.gz
需积分: 1 0 下载量 48 浏览量
2024-03-04
14:46:50
上传
评论
收藏 621KB GZ 举报
温馨提示
GNU项目是一个自由软件集体协作项目,由理查德·斯托曼于1983年发起,旨在创建一个完全自由的操作系统,称为GNU操作系统。该项目强调软件的自由,即用户能够自由地运行、复制、分发、学习、改变和改进软件。GNU项目产生了许多重要的软件,例如GNU编译器集合(GCC)和GNU通用公共许可证(GPL),后者为开源软件提供了法律基础。 Python库是一组预先编写的代码模块,旨在帮助开发者实现特定的编程任务,无需从零开始编写代码。这些库可以包括各种功能,如数学运算、文件操作、数据分析和网络编程等。Python社区提供了大量的第三方库,如NumPy、Pandas和Requests,极大地丰富了Python的应用领域,从数据科学到Web开发。
资源推荐
资源详情
资源评论
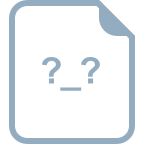
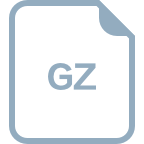
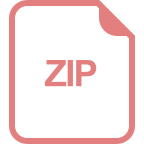
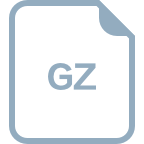
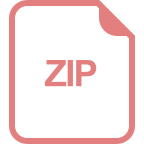
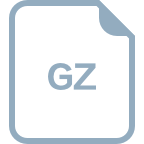
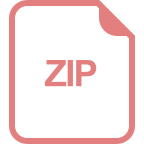
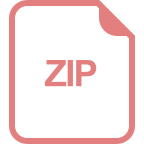
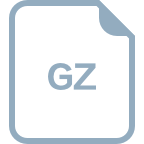
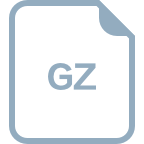
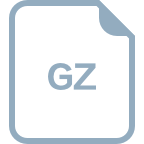
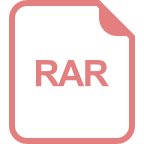
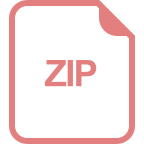
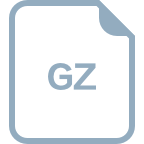
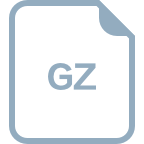
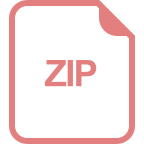
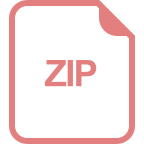
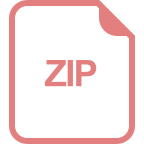
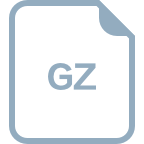
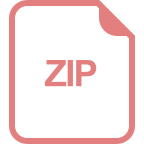
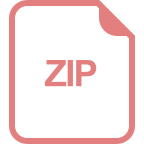
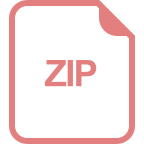
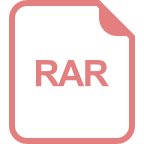
收起资源包目录

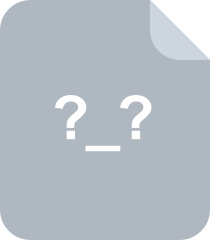
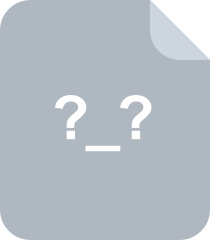
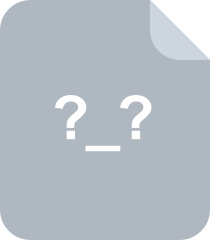
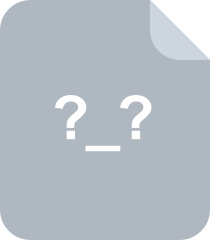
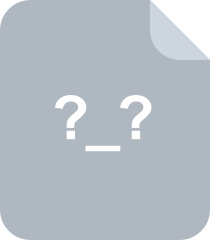
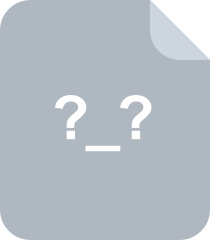
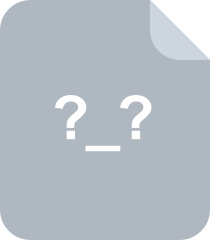
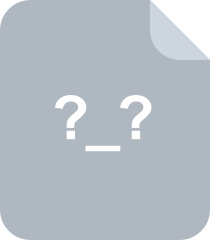
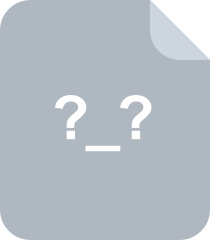
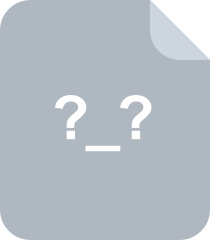
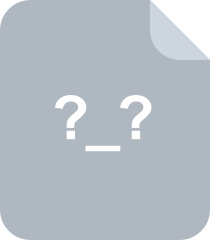
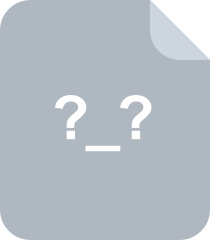
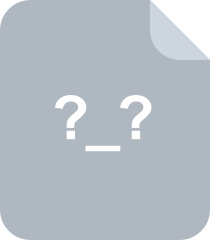
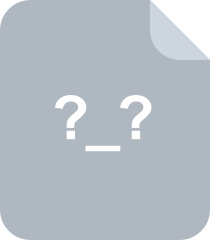
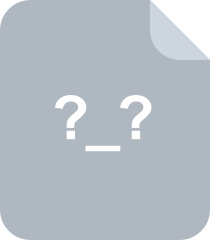
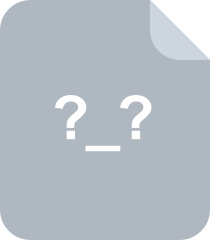
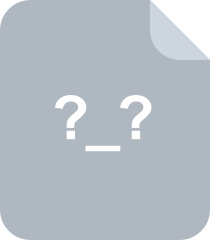
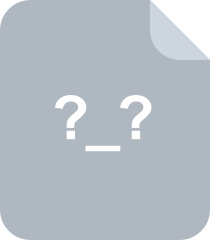
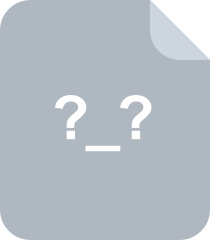
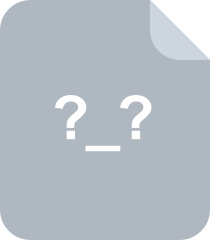
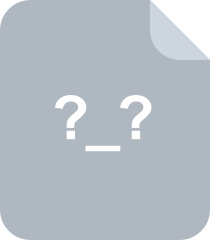
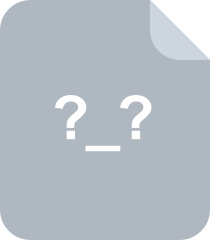
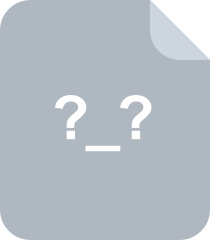
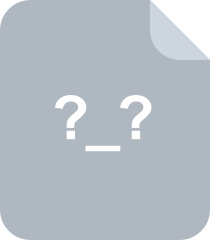
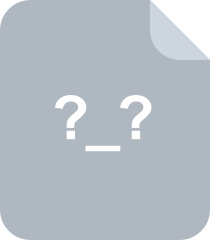
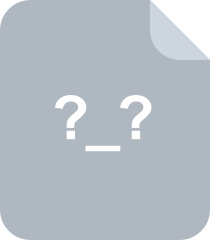
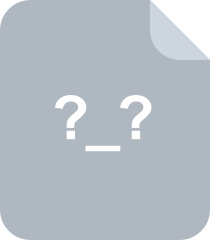
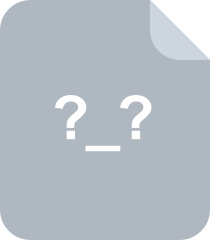
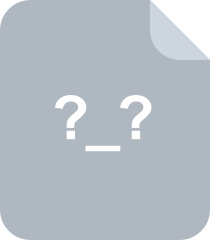
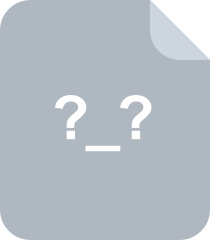
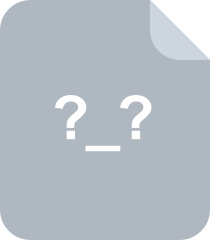
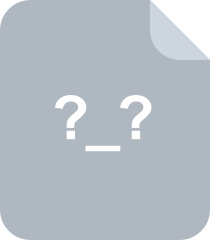
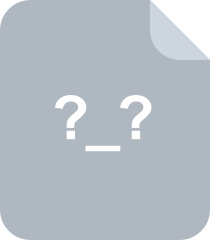
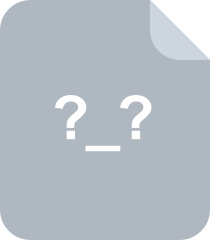
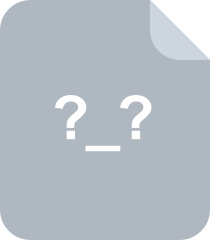
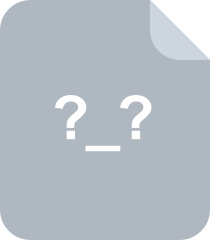
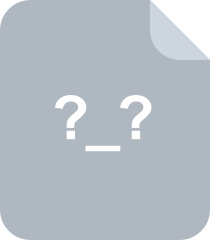
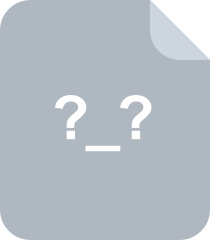
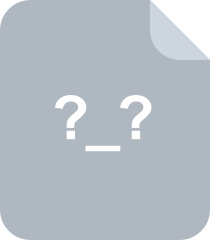
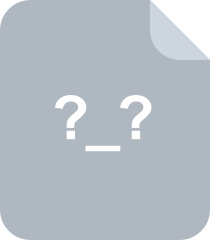
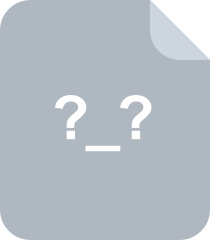
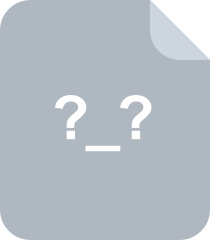
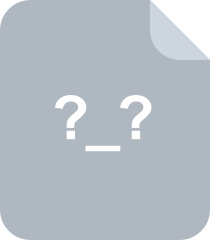
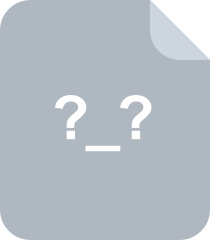
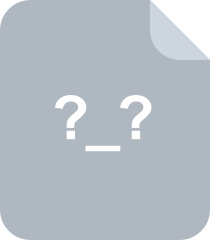
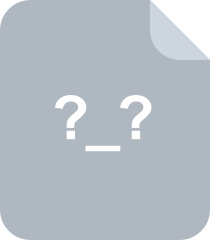
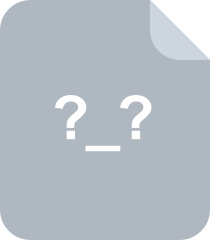
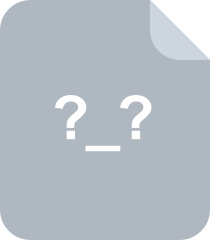
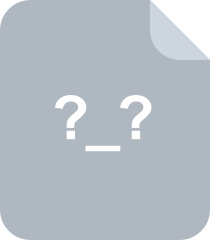
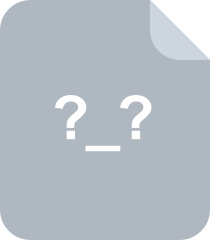
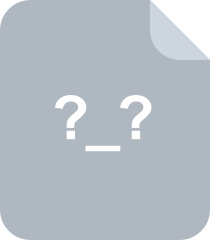
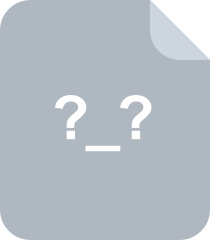
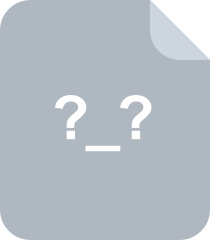
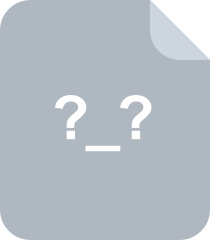
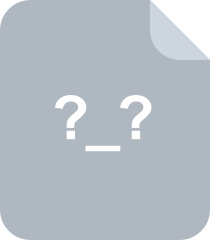
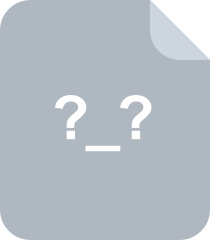
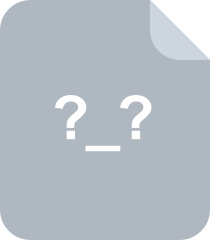
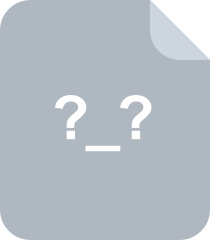
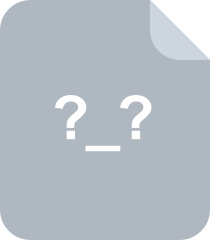
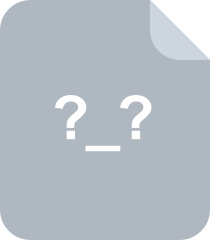
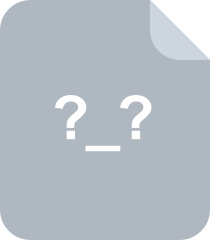
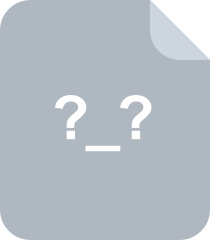
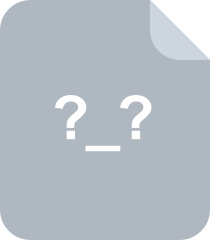
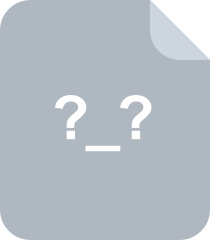
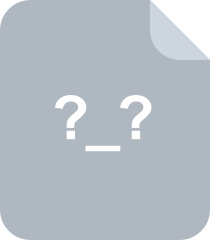
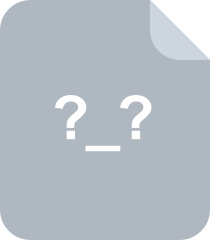
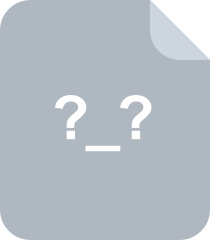
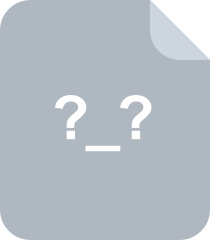
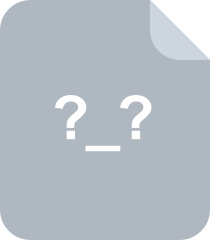
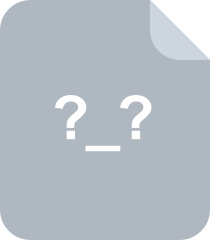
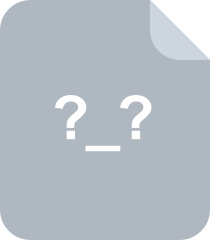
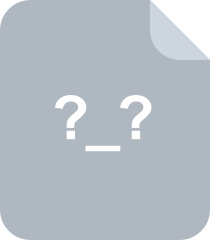
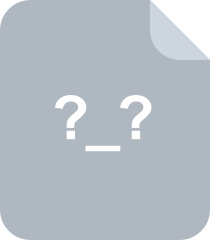
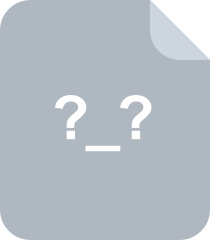
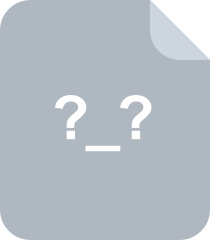
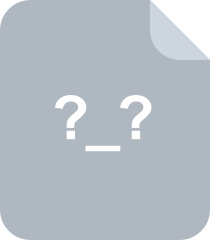
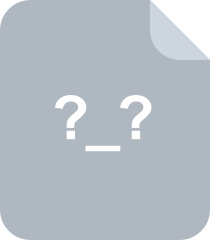
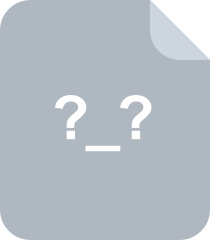
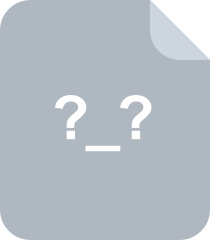
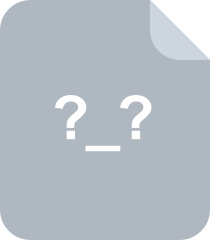
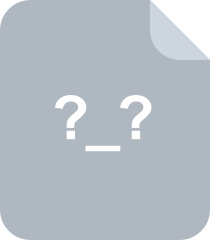
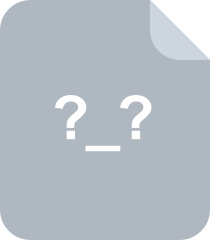
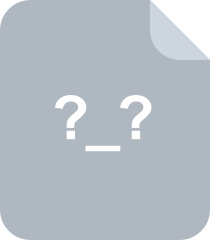
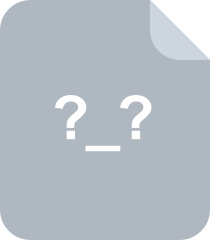
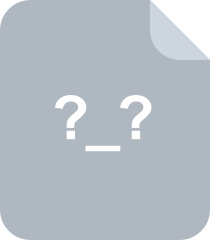
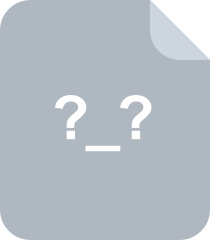
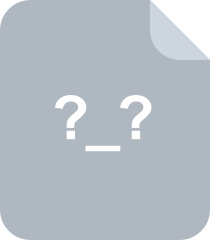
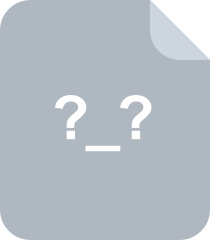
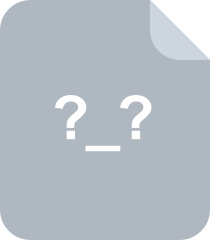
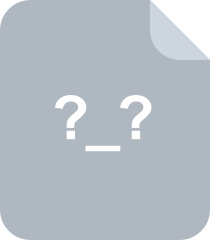
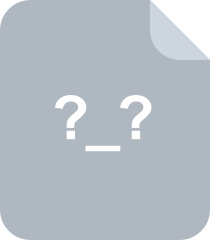
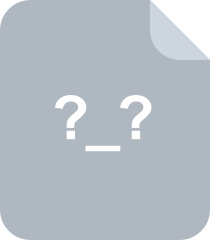
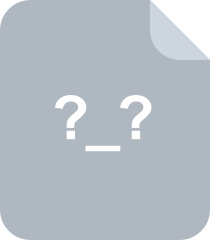
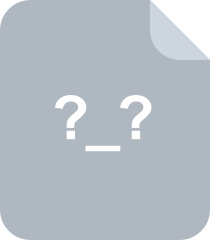
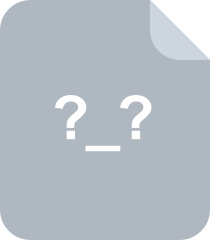
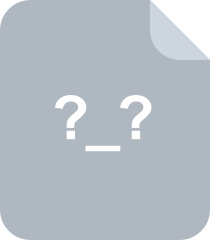
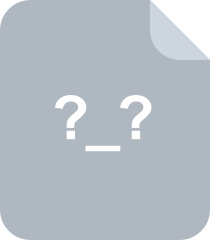
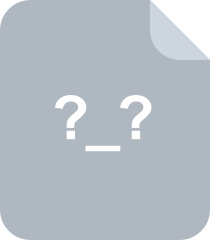
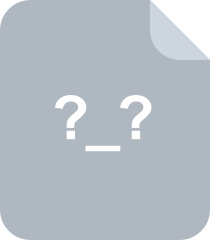
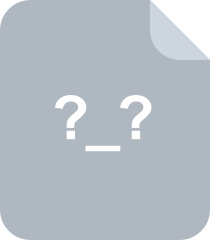
共 343 条
- 1
- 2
- 3
- 4
资源评论
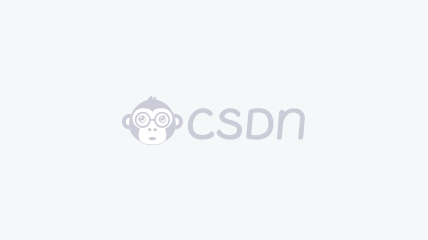

程序员Chino的日记
- 粉丝: 3664
- 资源: 5万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

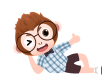
最新资源
- JavaScript函数
- java-leetcode题解之Range Sum Query 2D - Mutable.java
- java-leetcode题解之Random Pick Index.java
- java-leetcode题解之Race Car.java
- java-leetcode题解之Profitable Schemes.java
- java-leetcode题解之Product of Array Exclude Itself.java
- java-leetcode题解之Prime Arrangements.java
- MCU51-51单片机
- java-leetcode题解之Power of Two.java
- java-leetcode题解之Power of Three.java
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


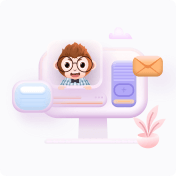
安全验证
文档复制为VIP权益,开通VIP直接复制
