/*
* Mach Operating System
* Copyright (c) 1993-1989 Carnegie Mellon University
* All Rights Reserved.
*
* Permission to use, copy, modify and distribute this software and its
* documentation is hereby granted, provided that both the copyright
* notice and this permission notice appear in all copies of the
* software, derivative works or modified versions, and any portions
* thereof, and that both notices appear in supporting documentation.
*
* CARNEGIE MELLON ALLOWS FREE USE OF THIS SOFTWARE IN ITS "AS IS"
* CONDITION. CARNEGIE MELLON DISCLAIMS ANY LIABILITY OF ANY KIND FOR
* ANY DAMAGES WHATSOEVER RESULTING FROM THE USE OF THIS SOFTWARE.
*
* Carnegie Mellon requests users of this software to return to
*
* Software Distribution Coordinator or Software.Distribution@CS.CMU.EDU
* School of Computer Science
* Carnegie Mellon University
* Pittsburgh PA 15213-3890
*
* any improvements or extensions that they make and grant Carnegie Mellon
* the rights to redistribute these changes.
*/
/*
* Default pager. Pages to paging partition.
*
* MUST BE ABLE TO ALLOCATE WIRED-DOWN MEMORY!!!
*/
#include <mach.h>
#include <mach/message.h>
#include <mach/notify.h>
#include <mach/mig_errors.h>
#include <mach/thread_switch.h>
#include <mach/task_info.h>
#include <mach/default_pager_types.h>
#include <pthread.h>
#include <stddef.h>
#include <device/device_types.h>
#include <device/device.h>
#include <hurd/ihash.h>
#include "queue.h"
#include "wiring.h"
#include "kalloc.h"
#include "default_pager.h"
#include <assert.h>
#include <errno.h>
#include <stdio.h>
#include <string.h>
#include <stdarg.h>
#include <file_io.h>
#include "memory_object_S.h"
#include "memory_object_default_S.h"
#include "default_pager_S.h"
#include "exc_S.h"
#include "priv.h"
#define debug 0
static char my_name[] = "(default pager):";
static void __attribute__ ((format (printf, 1, 2), unused))
synchronized_printf (const char *fmt, ...)
{
static pthread_mutex_t printf_lock = PTHREAD_MUTEX_INITIALIZER;
va_list ap;
va_start (ap, fmt);
pthread_mutex_lock (&printf_lock);
vprintf (fmt, ap);
fflush (stdout);
pthread_mutex_unlock (&printf_lock);
va_end (ap);
}
#if 0
#define dprintf(f, x...) synchronized_printf (f, ##x)
#else
#define dprintf(f, x...) (void) 0
#endif
#if 0
#define ddprintf(f, x...) synchronized_printf (f, ##x)
#else
#define ddprintf(f, x...) (void) 0
#endif
/*
* parallel vs serial switch
*/
#define PARALLEL 1
#if 0
#define CHECKSUM 1
#endif
#define USE_PRECIOUS 1
#define ptoa(p) ((p)*vm_page_size)
#define atop(a) ((a)/vm_page_size)
partition_t partition_of(x)
int x;
{
if (x >= all_partitions.n_partitions || x < 0)
panic("partition_of x%x", x);
return all_partitions.partition_list[x];
}
void set_partition_of(x, p)
int x;
partition_t p;
{
if (x >= all_partitions.n_partitions || x < 0)
panic("set_partition_of x%x", x);
all_partitions.partition_list[x] = p;
}
/*
* Simple mapping from (file)NAME to id
* Saves space, filenames can be long.
*/
unsigned int
part_id(const char *name)
{
unsigned int id, xorid;
size_t len;
len = strlen(name);
id = xorid = 0;
while (len--) {
xorid ^= *name;
id += *name++;
}
return (id << 8) | xorid;
}
void
partition_init()
{
pthread_mutex_init(&all_partitions.lock, NULL);
all_partitions.n_partitions = 0;
}
static partition_t
new_partition (const char *name, struct file_direct *fdp,
int check_linux_signature)
{
partition_t part;
vm_size_t size, bmsize;
vm_offset_t raddr;
mach_msg_type_number_t rsize;
int rc;
unsigned int id = part_id(name);
unsigned int n = strlen(name);
pthread_mutex_lock(&all_partitions.lock);
{
unsigned int i;
for (i = 0; i < all_partitions.n_partitions; i++)
{
part = partition_of(i);
if (part && part->id == id)
{
pthread_mutex_unlock(&all_partitions.lock);
return 0;
}
}
}
pthread_mutex_unlock(&all_partitions.lock);
size = atop(fdp->fd_size * fdp->fd_bsize);
bmsize = howmany(size, NB_BM) * sizeof(bm_entry_t);
part = (partition_t) kalloc(sizeof(struct part));
pthread_mutex_init(&part->p_lock, NULL);
part->name = (char*) kalloc(n + 1);
strcpy(part->name, name);
part->total_size = size;
part->free = size;
part->id = id;
part->bitmap = (bm_entry_t *)kalloc(bmsize);
part->going_away= FALSE;
part->file = fdp;
memset ((char *)part->bitmap, 0, bmsize);
if (check_linux_signature < 0)
{
if (check_linux_signature != -3)
printf("(default pager): "
"Paging to raw partition %s (%uk paging space)\n",
name, part->total_size * (vm_page_size / 1024));
return part;
}
#define LINUX_PAGE_SIZE 4096 /* size of pages in Linux swap partitions */
rc = page_read_file_direct(part->file,
0, LINUX_PAGE_SIZE,
&raddr,
&rsize);
if (rc)
panic("(default pager): cannot read first page of %s! rc=%#x\n",
name, rc);
while (rsize < LINUX_PAGE_SIZE)
{
/* Filesystem block size is smaller than page size,
so we must do several reads to get the whole page. */
vm_address_t baddr;
vm_size_t bsize;
rc = page_read_file_direct(part->file,
rsize, LINUX_PAGE_SIZE-rsize,
&baddr,
&bsize);
if (rc)
panic("(default pager): "
"cannot read first page of %s! rc=%#x at %#x\n",
name, rc, rsize);
memcpy ((char *) raddr + rsize, (void *) baddr, bsize);
rsize += bsize;
vm_deallocate (mach_task_self (), baddr, bsize);
}
if (!memcmp("SWAP-SPACE", (char *) raddr + LINUX_PAGE_SIZE-10, 10))
{
/* The partition's first page has a Linux swap signature.
This means the beginning of the page contains a bitmap
of good pages, and all others are bad. */
unsigned int i, j, bad, max;
int waste;
printf("(default pager): Found Linux 2.0 swap signature in %s\n",
name);
/* The first page, and the pages corresponding to the bits
occupied by the signature in the final 10 bytes of the page,
are always unavailable ("bad"). */
*(u_int32_t *)raddr &= ~(u_int32_t) 1;
memset((char *) raddr + LINUX_PAGE_SIZE-10, 0, 10);
max = LINUX_PAGE_SIZE / sizeof(u_int32_t);
if (max > (part->total_size + 31) / 32)
max = (part->total_size + 31) / 32;
bad = 0;
for (i = 0; i < max; ++i)
{
u_int32_t bm = ((u_int32_t *) raddr)[i];
if (bm == ~(u_int32_t) 0)
continue;
/* There are some zero bits in this word. */
for (j = 0; j < 32; ++j)
if ((bm & (1 << j)) == 0)
{
unsigned int p = i*32 + j;
if (p >= part->total_size)
break;
++bad;
part->bitmap[p / NB_BM] |= 1 << (p % NB_BM);
}
}
part->free -= bad;
--bad; /* Don't complain about first page. */
waste = part->total_size - (8 * (LINUX_PAGE_SIZE-10));
if (waste > 0)
{
/* The wasted pages were already marked "bad". */
bad -= waste;
if (bad > 0)
printf("\
(default pager): Paging to %s, %dk swap-space (%dk bad, %dk wasted at end)\n",
name,
part->free * (LINUX_PAGE_SIZE / 1024),
bad * (LINUX_PAGE_SIZE / 1024),
waste * (LINUX_PAGE_SIZE / 1024));
else
printf("\
(default pager): Paging to %s, %dk swap-space (%dk wasted at end)\n",
name,
part->free * (LINUX_PAGE_SIZE / 1024),
waste * (LINUX_PAGE_SIZE / 1024));
}
else if (bad > 0)
printf("\
(default pager): Paging to %s, %dk swap-space (excludes %dk marked bad)\n",
name,
part->free * (LINUX_PAGE_SIZE / 1024),
bad * (LINUX_PAGE_SIZE / 1024));
else
printf("\
(default pager): Paging to %s, %dk swap-space\n",
name,
part->free * (LINUX_PAGE_SIZE / 1024));
}
else if (!memcmp("SWAPSPACE2",
(char *) raddr + LINUX_PAGE_SIZE-10, 10))
{
struct
{
u_int8_t bootbits[1024];
u_int32_t version;
u_int32_t last_page;
u_int32_t nr_badpages;
没有合适的资源?快使用搜索试试~ 我知道了~
hurd-0.8.tar.gz
0 下载量 36 浏览量
2024-03-04
14:44:32
上传
评论
收藏 2.96MB GZ 举报
温馨提示
GNU项目是一个自由软件集体协作项目,由理查德·斯托曼于1983年发起,旨在创建一个完全自由的操作系统,称为GNU操作系统。该项目强调软件的自由,即用户能够自由地运行、复制、分发、学习、改变和改进软件。GNU项目产生了许多重要的软件,例如GNU编译器集合(GCC)和GNU通用公共许可证(GPL),后者为开源软件提供了法律基础。 Python库是一组预先编写的代码模块,旨在帮助开发者实现特定的编程任务,无需从零开始编写代码。这些库可以包括各种功能,如数学运算、文件操作、数据分析和网络编程等。Python社区提供了大量的第三方库,如NumPy、Pandas和Requests,极大地丰富了Python的应用领域,从数据科学到Web开发。
资源推荐
资源详情
资源评论
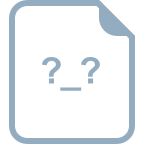
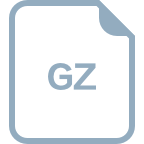
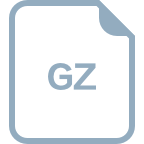
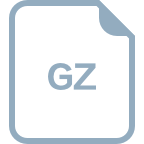
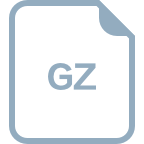
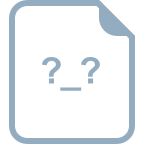
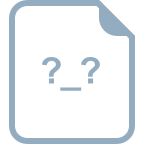
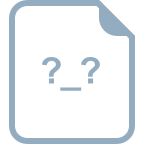
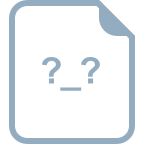
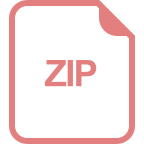
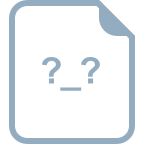
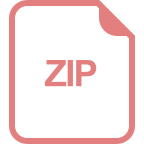
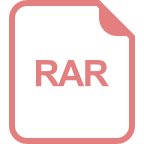
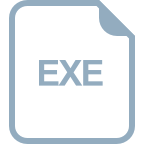
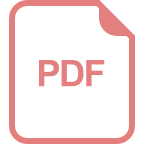
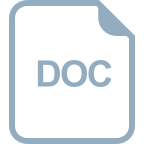
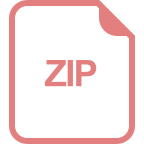
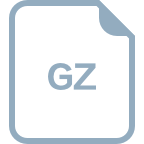
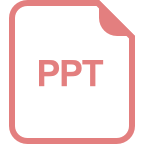
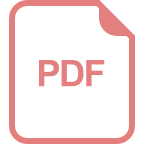
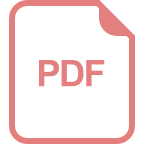
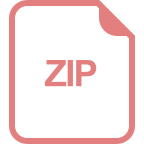
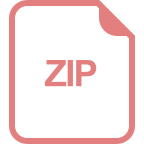
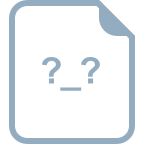
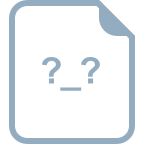
收起资源包目录

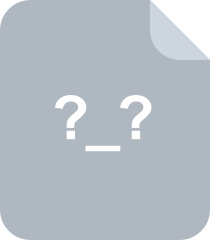
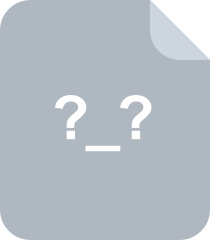
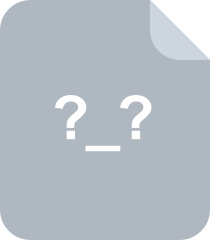
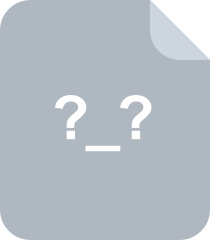
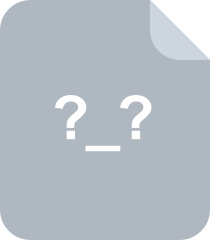
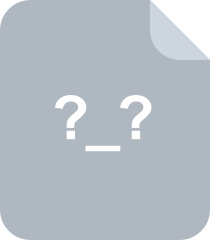
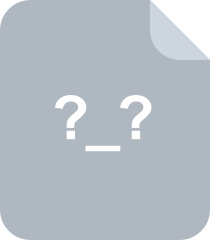
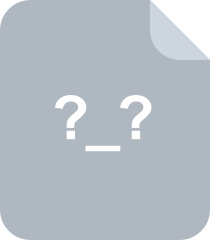
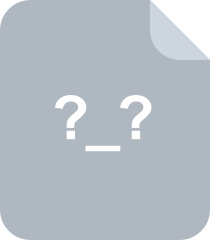
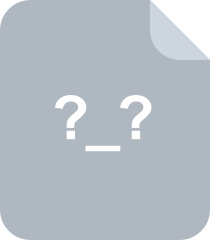
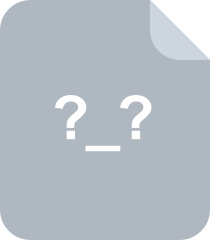
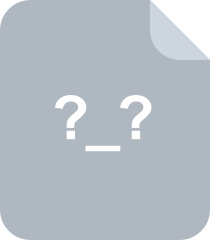
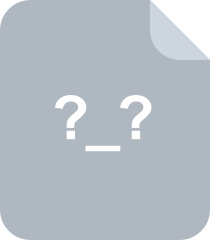
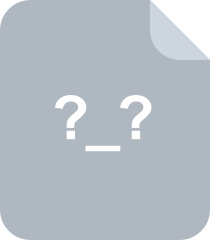
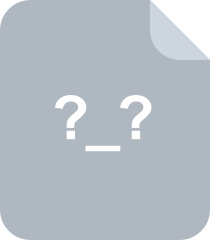
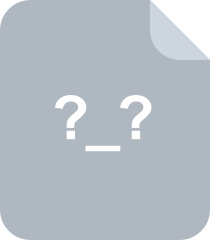
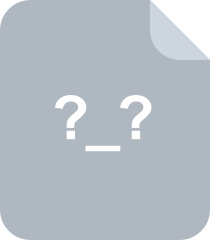
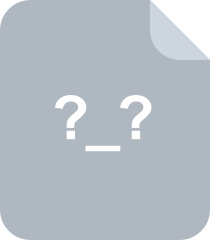
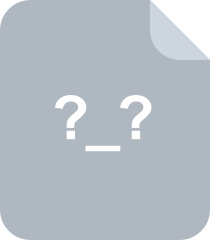
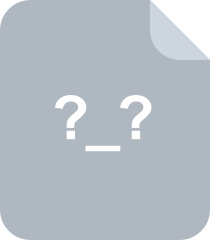
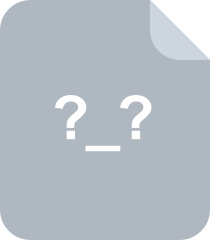
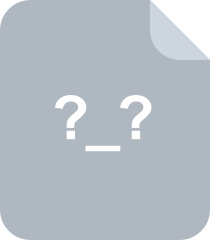
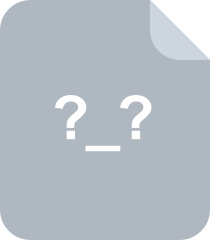
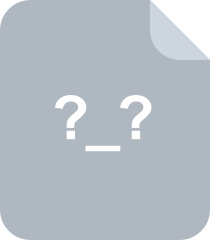
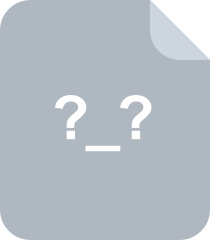
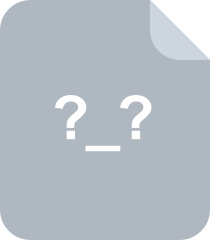
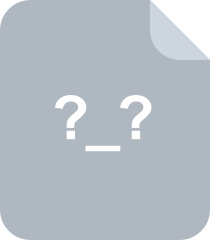
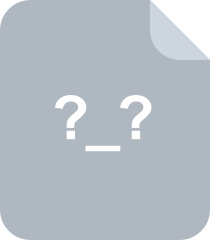
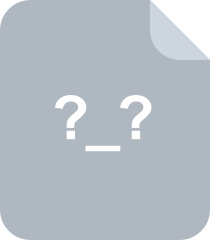
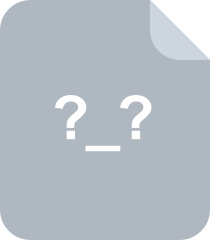
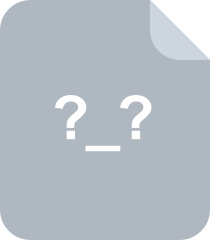
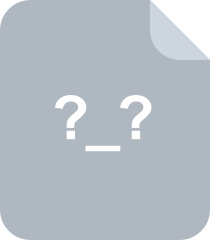
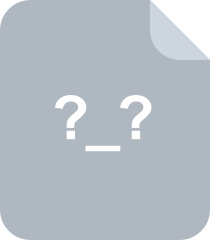
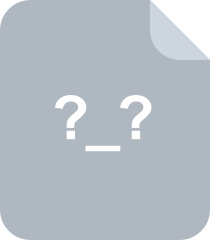
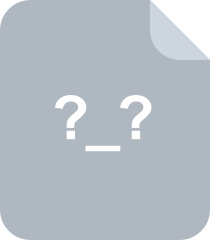
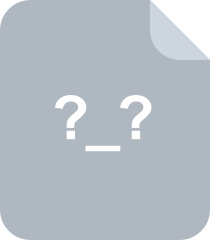
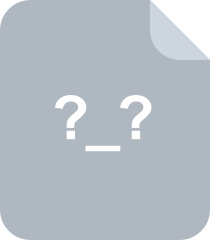
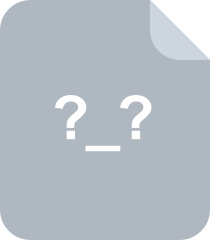
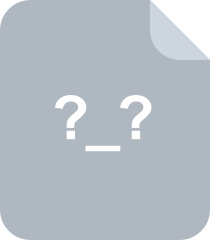
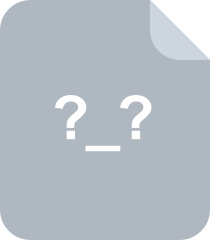
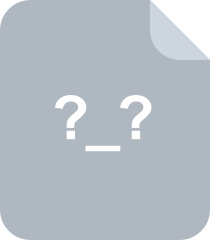
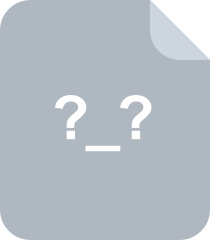
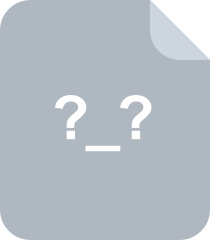
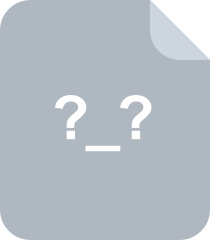
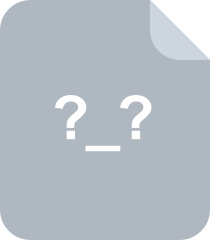
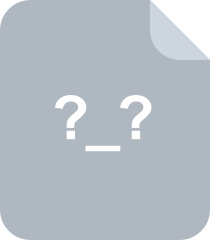
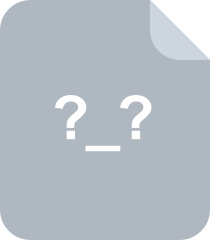
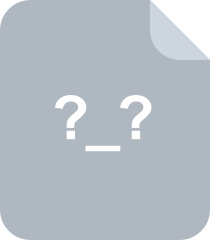
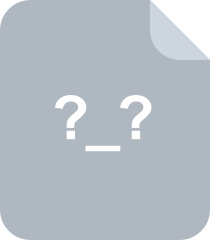
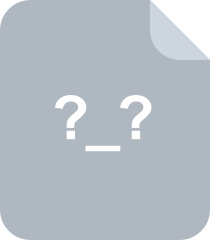
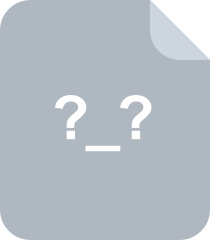
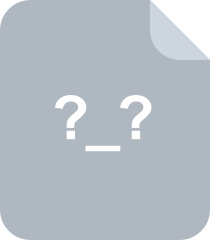
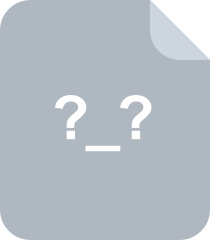
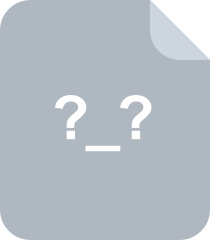
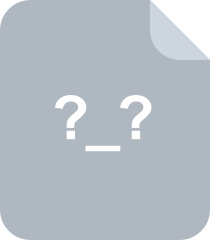
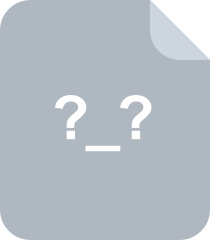
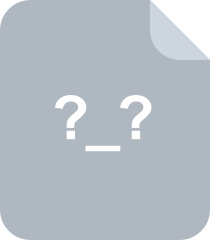
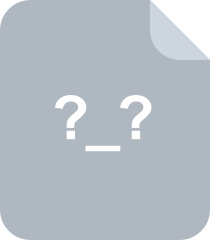
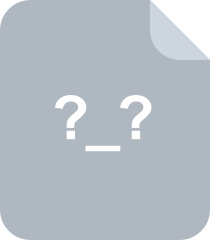
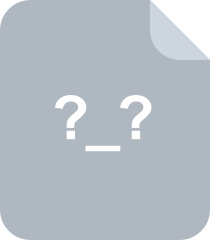
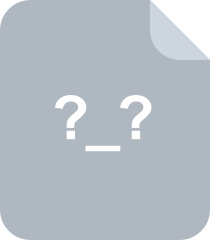
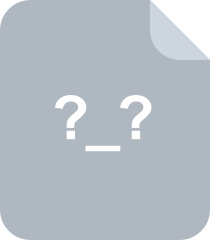
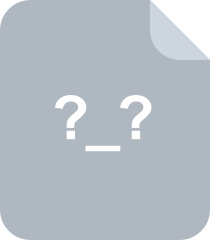
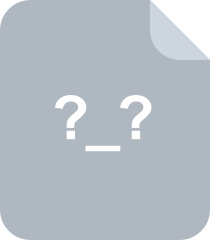
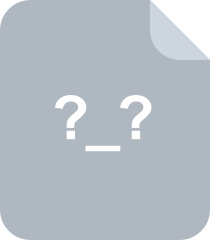
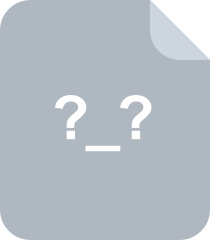
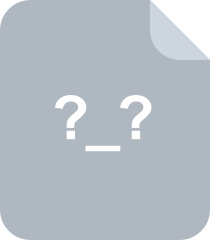
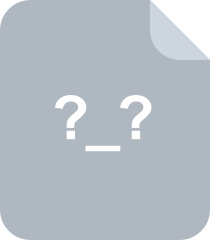
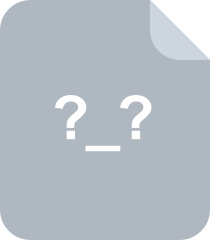
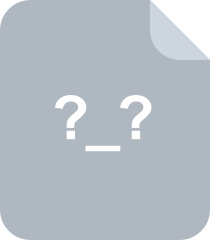
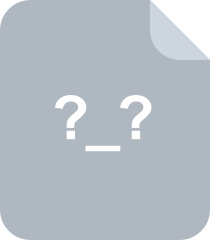
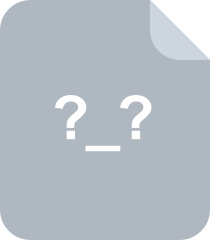
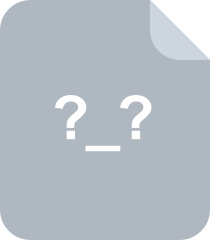
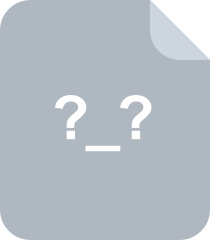
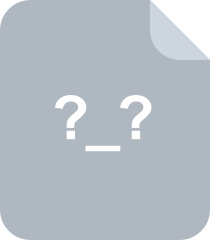
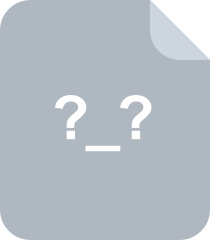
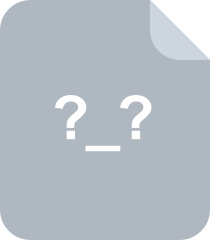
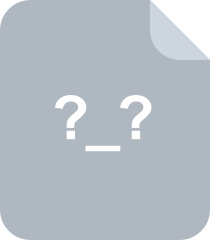
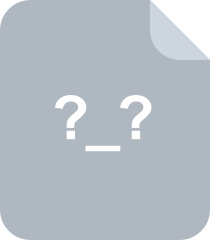
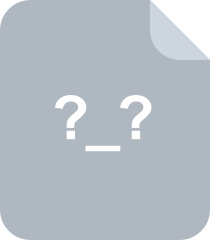
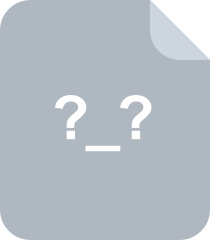
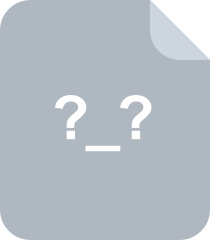
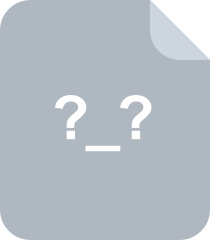
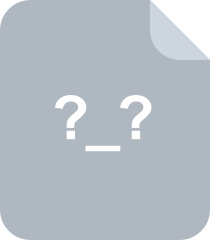
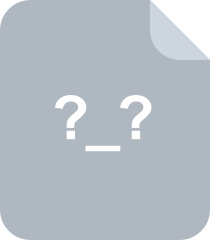
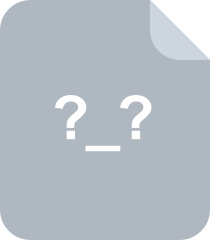
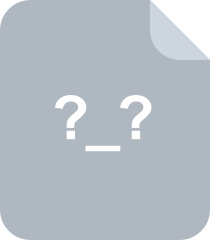
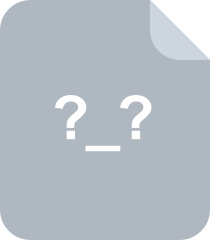
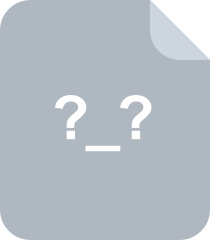
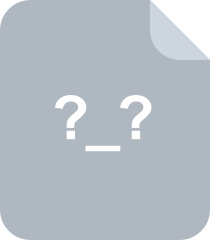
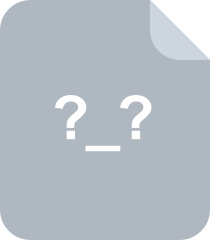
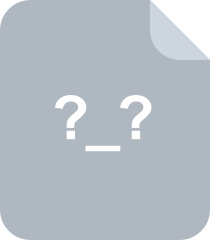
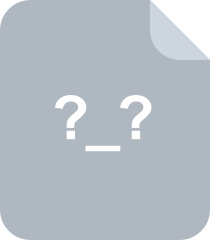
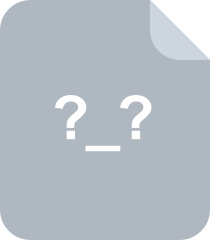
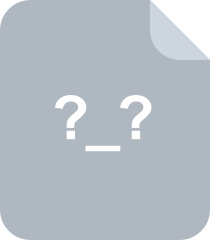
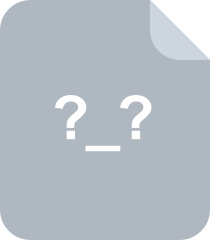
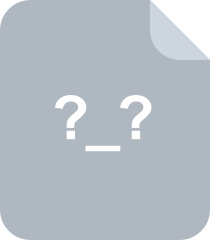
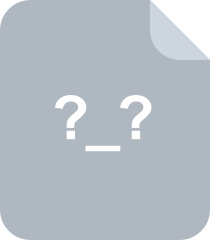
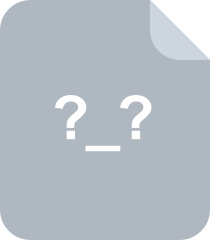
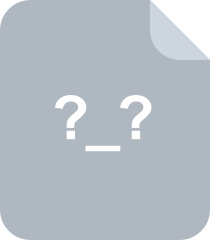
共 1844 条
- 1
- 2
- 3
- 4
- 5
- 6
- 19
资源评论
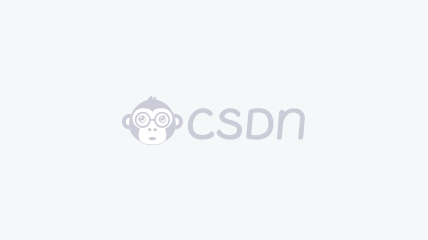

程序员Chino的日记
- 粉丝: 3658
- 资源: 5万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

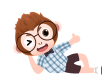
安全验证
文档复制为VIP权益,开通VIP直接复制
