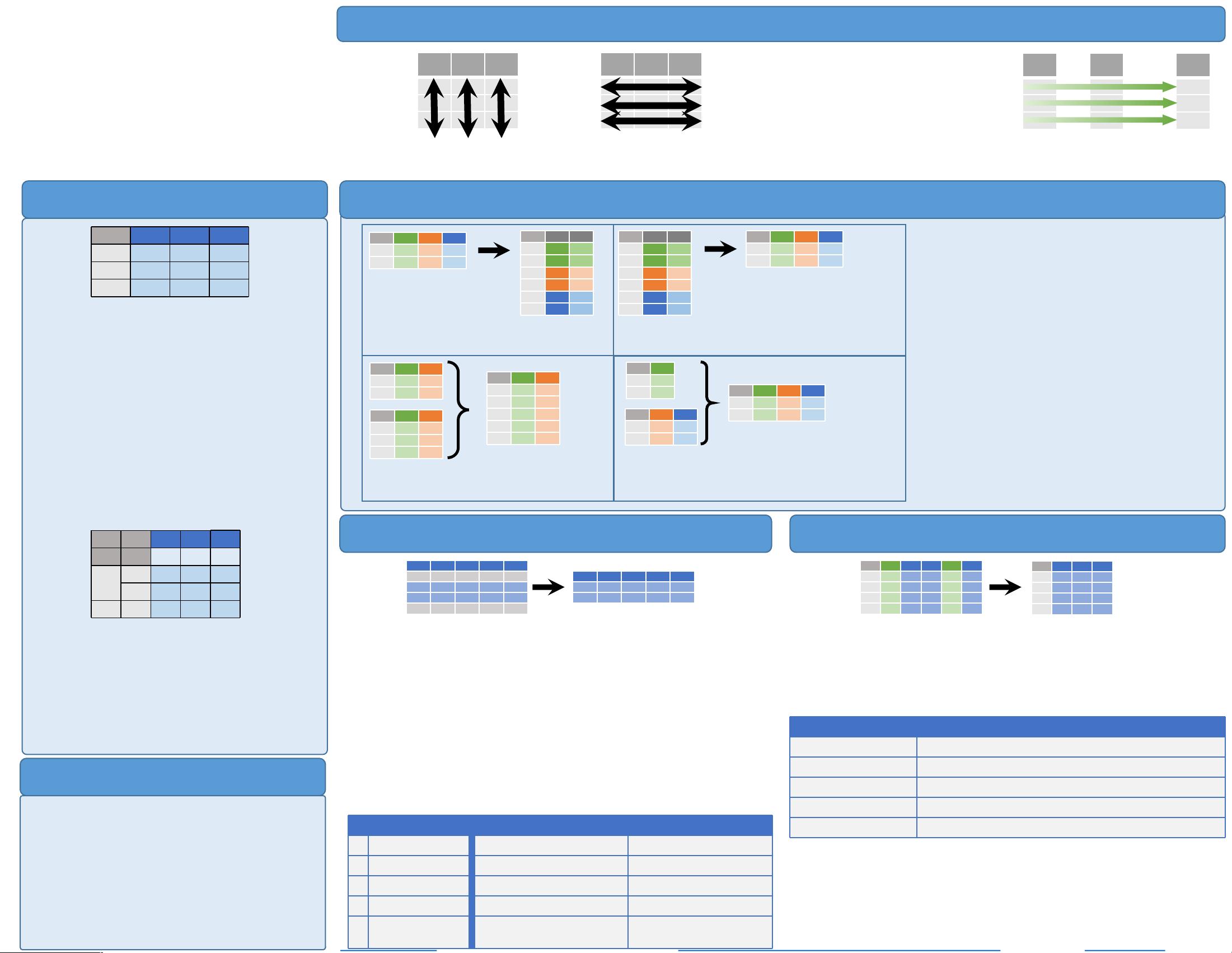
pandas-0.23.2.tar.gz
《Pandas 0.23.2:Python数据分析的核心库》 Pandas是Python编程语言中的一个开源数据处理和分析库,它为Python提供了一种高效、灵活且易于使用的数据结构,使得数据清洗、转换、整合以及分析变得更加简单。Pandas 0.23.2是该库的一个版本,它在前一版本的基础上进行了多项改进和优化,以更好地服务于数据科学家和数据工程师。 在Pandas 0.23.2中,我们主要关注以下几个关键知识点: 1. **DataFrame和Series**:DataFrame是Pandas的核心数据结构,它类似于二维表格,可以理解为列式数据库。Series则是一维数据结构,类似于带索引的数组。这两种结构支持大量的内建函数和操作,如筛选、排序、聚合等,极大地提高了数据处理效率。 2. **数据导入与导出**:Pandas提供了丰富的数据导入功能,可以方便地读取CSV、Excel、SQL数据库等多种格式的数据。同时,它也能将处理后的数据导出为各种格式,方便分享和存储。 3. **缺失数据处理**:Pandas对缺失数据有完善的处理机制,可以便捷地进行缺失值的识别、填充或删除,确保数据的完整性。 4. **时间序列分析**:Pandas内置了对时间序列数据的支持,包括日期范围生成、时间间隔操作、时间戳处理等,适合金融、气象等领域的时间序列数据分析。 5. **数据融合与合并**:通过`merge`和`concat`函数,Pandas可以轻松实现不同数据集之间的连接和拼接,处理复杂的数据整合问题。 6. **数据清洗**:Pandas提供了一系列函数用于处理数据清洗,如去除重复值、替换异常值、数据类型转换等,使得数据预处理更为便捷。 7. **数据统计与可视化**:Pandas内置了一些基本的统计方法,如描述性统计、分组计算、频率统计等。结合Matplotlib或Seaborn等可视化库,可以快速生成统计图表,帮助用户理解数据分布和趋势。 8. **性能优化**:Pandas 0.23.2版本在性能上进行了优化,包括更快的运算速度和更小的内存占用,使得大规模数据处理成为可能。 9. **API改进**:此版本可能包含对API的调整和增强,以提高用户的使用体验和代码的可读性,但具体改动需参考官方文档。 10. **错误修复**:每个新版本都会修复一些已知的问题,确保软件的稳定性和可靠性。 Pandas 0.23.2作为Python数据分析的重要工具,无论对于初学者还是经验丰富的数据专业人士,都是一个不可或缺的库。通过掌握其核心功能和特性,我们可以更高效地进行数据探索、模型构建和业务分析。对于想要深入学习Python数据科学的人来说,Pandas的每一个版本都值得我们去了解和研究。
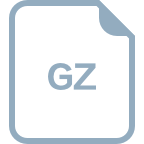
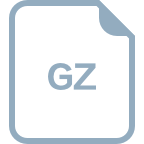
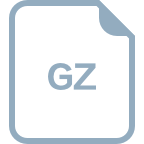
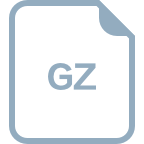
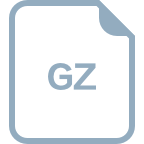
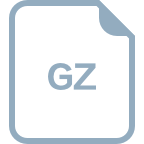
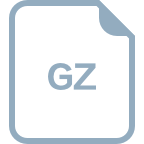
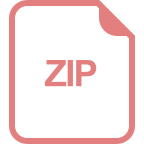
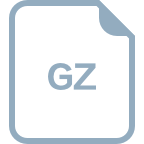
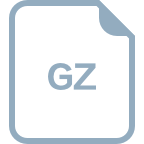
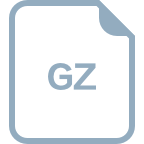
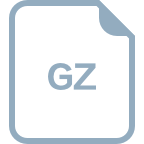
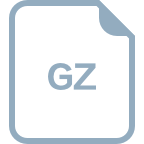
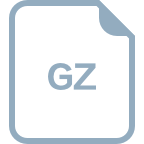
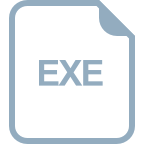
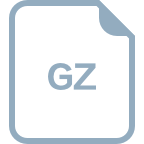
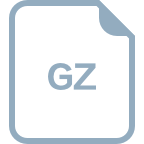
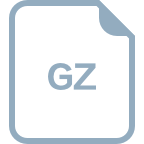
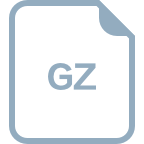
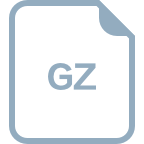
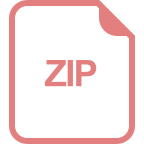
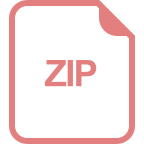
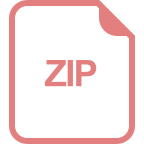
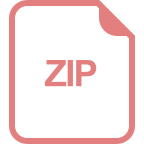
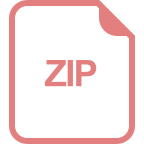
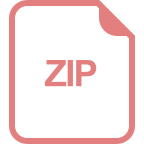
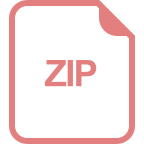
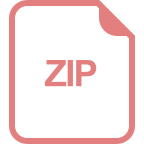

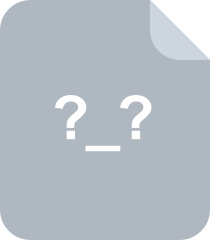
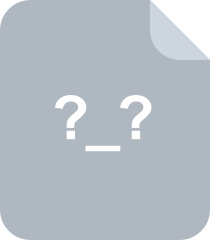
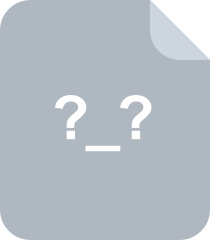
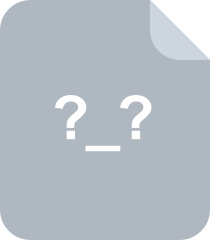
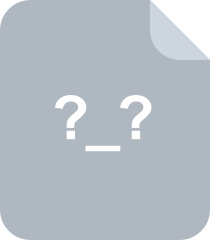
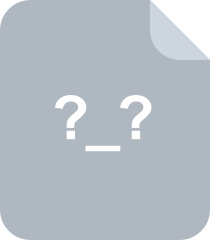
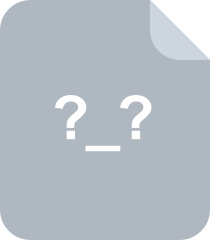
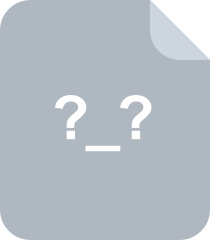
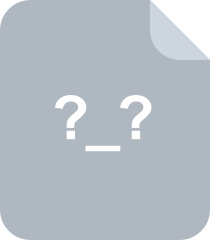
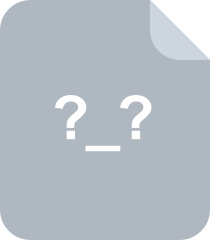
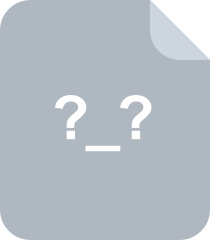
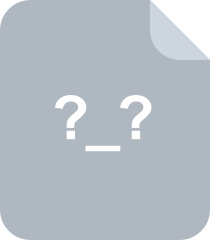
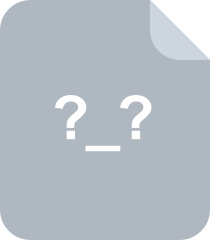
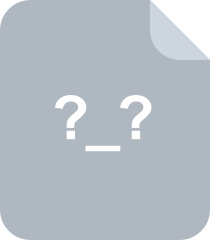
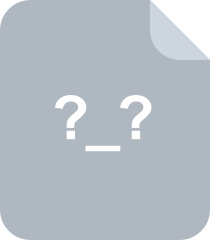
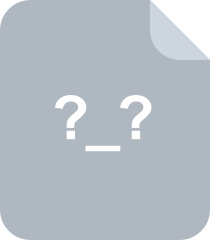
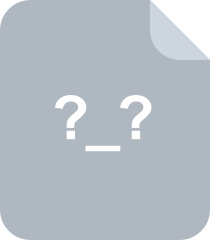
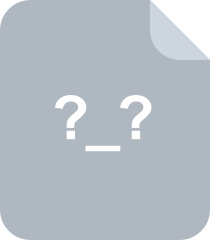
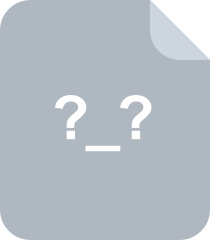
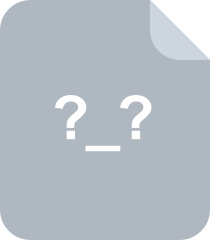
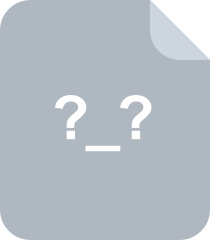
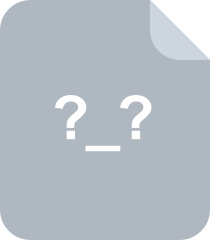
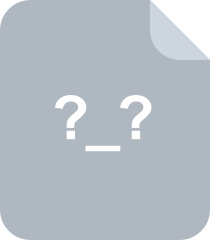
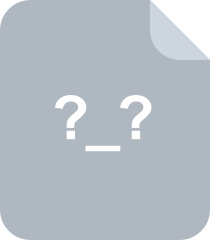
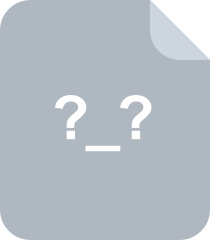
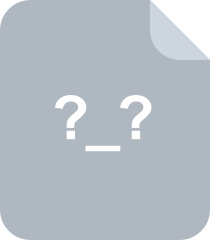
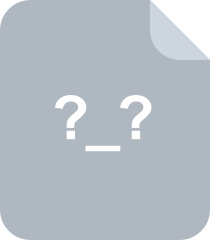
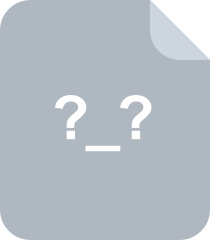
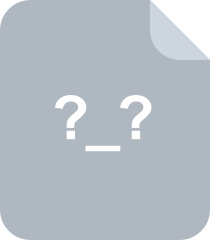
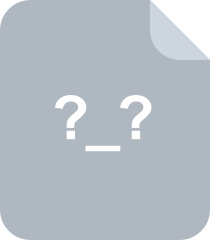
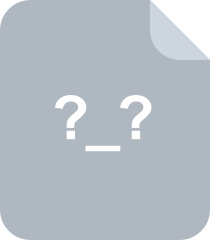
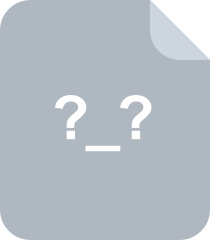
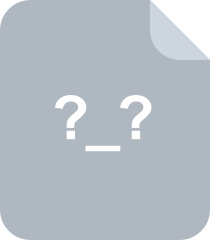
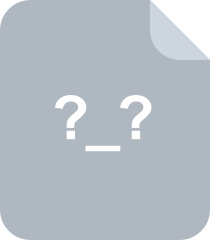
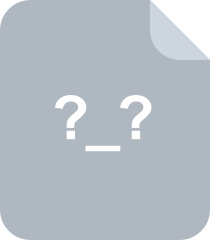
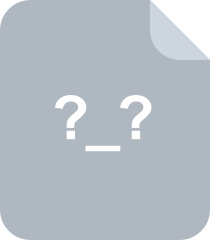
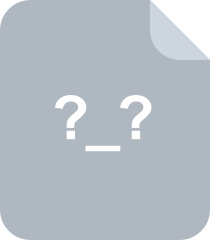
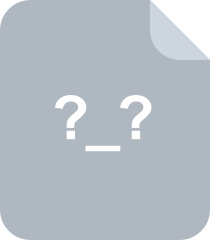
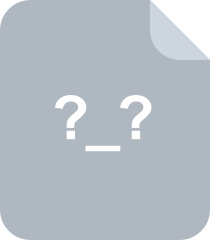
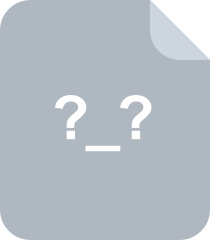
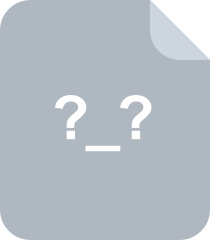
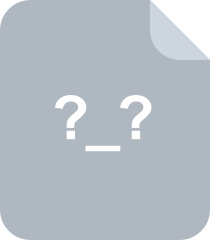
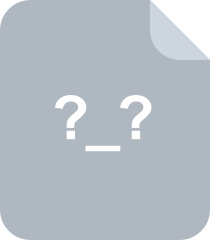
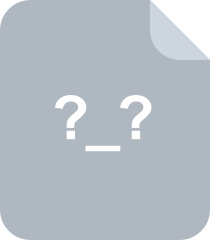
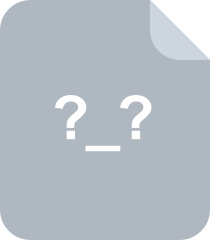
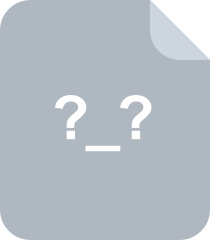
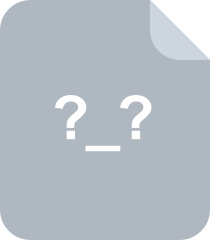
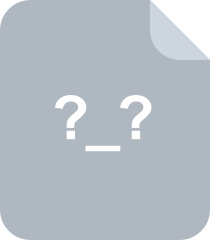
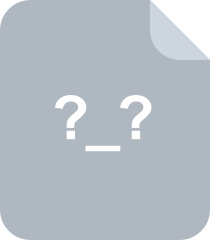
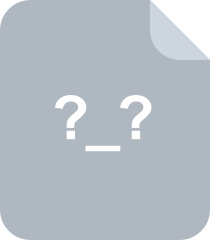
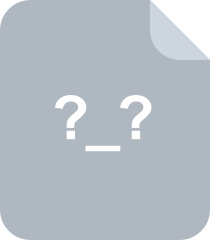
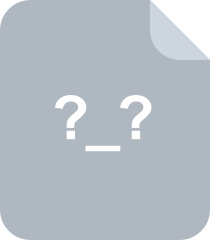
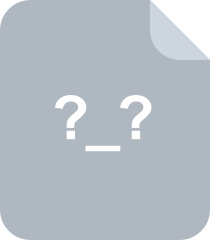
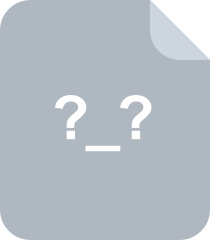
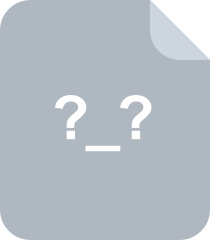
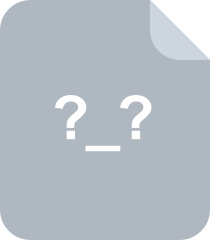
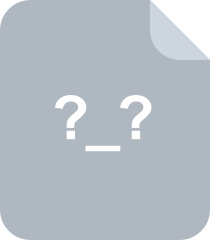
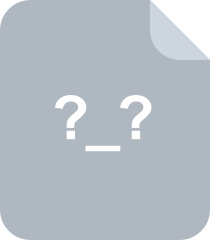
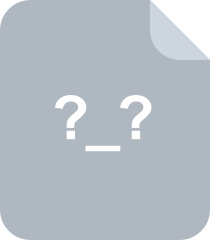
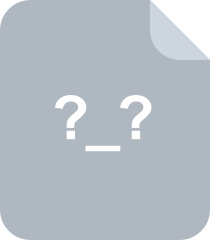
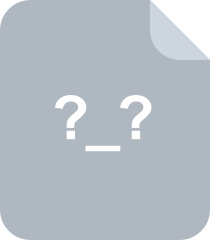
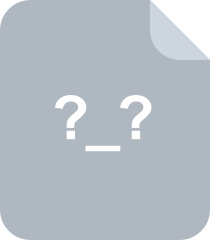
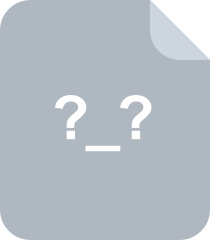
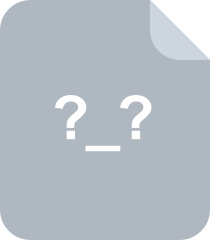
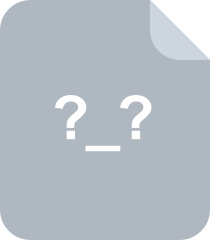
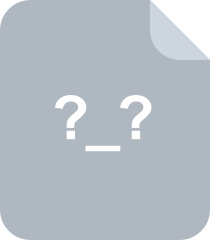
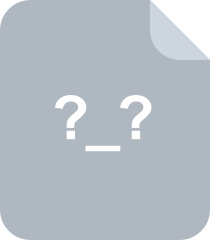
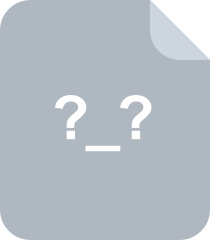
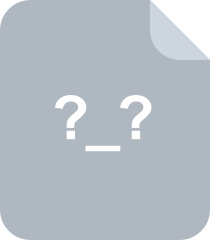
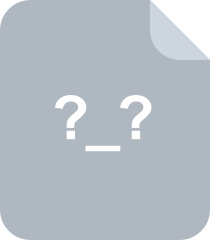
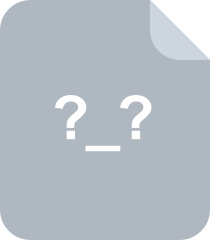
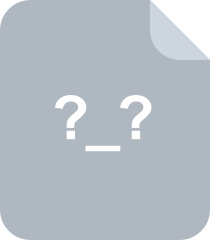
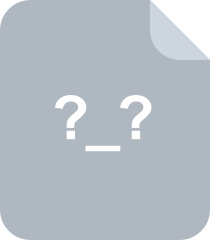
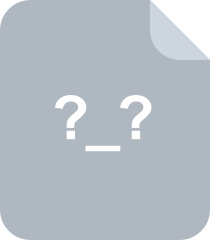
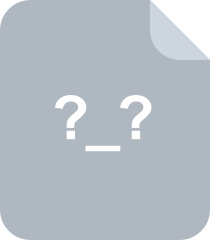
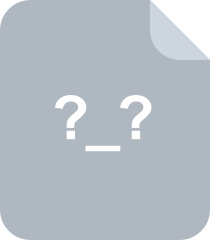
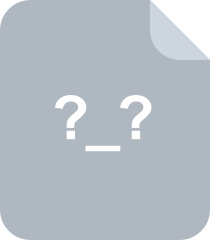
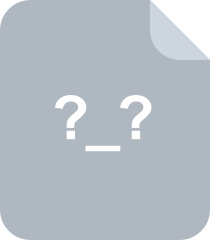
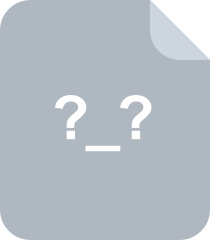
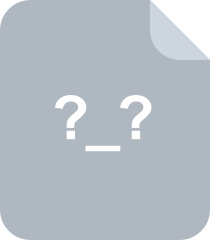
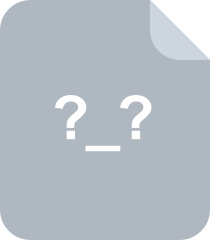
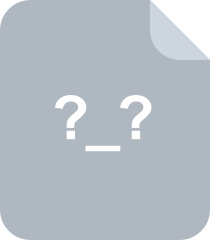
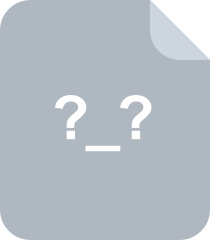
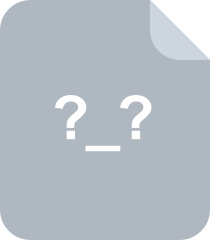
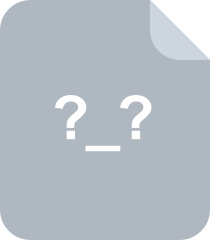
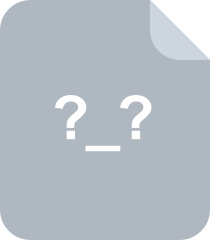
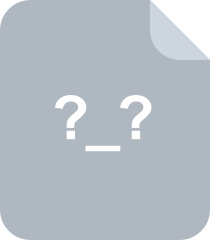
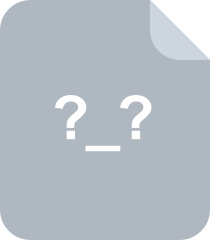
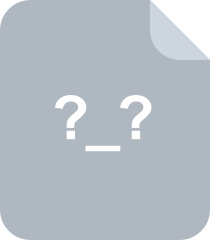
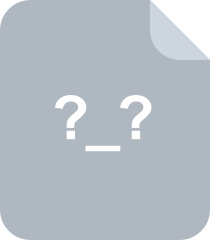
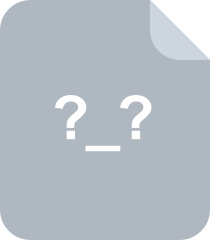
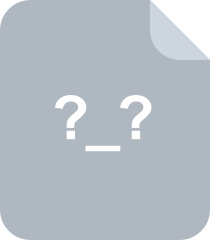
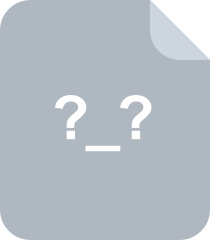
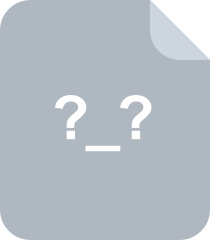
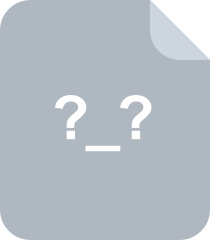
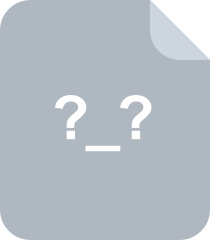
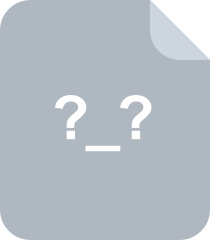
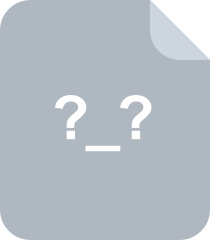
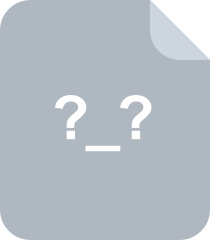
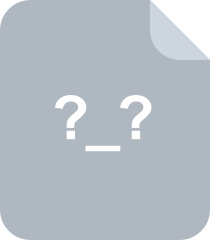
- 1
- 2
- 3
- 4
- 5
- 6
- 10
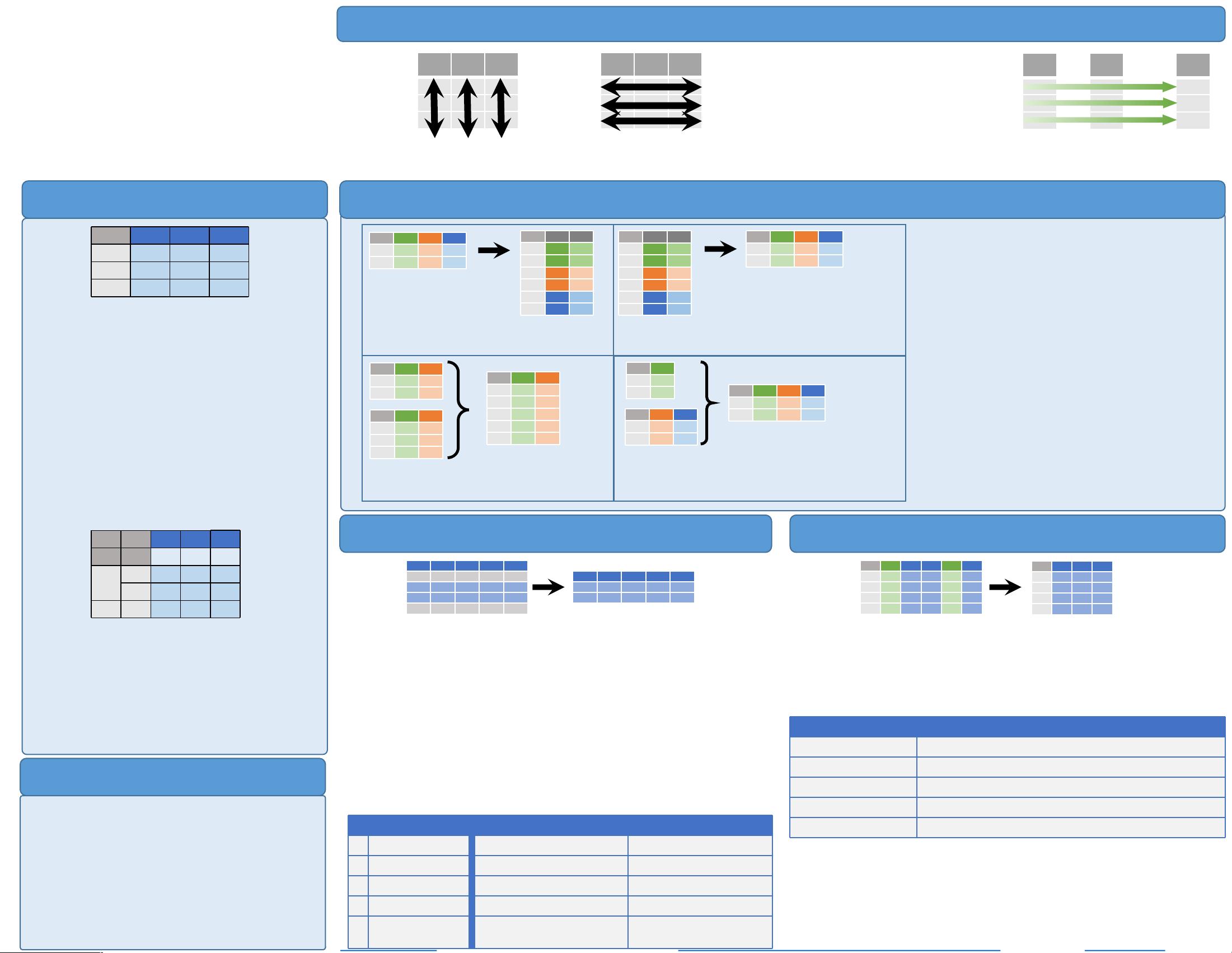
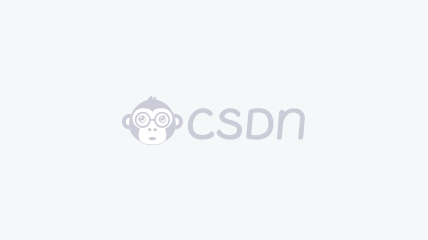

- 粉丝: 3581
- 资源: 5万+
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

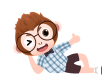
最新资源
- Postman 10.10.9版本安装包
- 2023移动应用开发课表.xls
- NTD5413NT4G-VB一款N-Channel沟道TO252的MOSFET晶体管参数介绍与应用说明
- 实验1HTML题目2文档.doc
- NTD32N06T4G-VB一款N-Channel沟道TO252的MOSFET晶体管参数介绍与应用说明
- NTD32N06LT4G-VB一款N-Channel沟道TO252的MOSFET晶体管参数介绍与应用说明
- 毕业设计 基于python实现网络长度字段的提取算法系统源码+说明文档+数据.tar
- 远程PE,批量安装系统 PXE服务软件
- 高级系统架构师考试全套资料
- NTD32N06LG-VB一款N-Channel沟道TO252的MOSFET晶体管参数介绍与应用说明

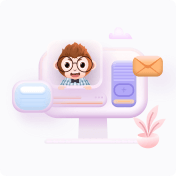
