/* Job execution and handling for GNU Make.
Copyright (C) 1988,89,90,91,92,93,94,95,96,97,99 Free Software Foundation, Inc.
This file is part of GNU Make.
GNU Make is free software; you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation; either version 2, or (at your option)
any later version.
GNU Make is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with GNU Make; see the file COPYING. If not, write to
the Free Software Foundation, Inc., 59 Temple Place - Suite 330,
Boston, MA 02111-1307, USA. */
#include <assert.h>
#include "make.h"
#include "job.h"
#include "debug.h"
#include "filedef.h"
#include "commands.h"
#include "variable.h"
#include "debug.h"
#include <string.h>
/* Default shell to use. */
#ifdef WINDOWS32
char *default_shell = "sh.exe";
int no_default_sh_exe = 1;
int batch_mode_shell = 1;
#else /* WINDOWS32 */
# ifdef _AMIGA
char default_shell[] = "";
extern int MyExecute (char **);
# else /* _AMIGA */
# ifdef __MSDOS__
/* The default shell is a pointer so we can change it if Makefile
says so. It is without an explicit path so we get a chance
to search the $PATH for it (since MSDOS doesn't have standard
directories we could trust). */
char *default_shell = "command.com";
# else /* __MSDOS__ */
# ifdef VMS
# include <descrip.h>
char default_shell[] = "";
# else
char default_shell[] = "/bin/sh";
# endif /* VMS */
# endif /* __MSDOS__ */
int batch_mode_shell = 0;
# endif /* _AMIGA */
#endif /* WINDOWS32 */
#ifdef __MSDOS__
# include <process.h>
static int execute_by_shell;
static int dos_pid = 123;
int dos_status;
int dos_command_running;
#endif /* __MSDOS__ */
#ifdef _AMIGA
# include <proto/dos.h>
static int amiga_pid = 123;
static int amiga_status;
static char amiga_bname[32];
static int amiga_batch_file;
#endif /* Amiga. */
#ifdef VMS
# include <time.h>
# ifndef __GNUC__
# include <processes.h>
# endif
# include <starlet.h>
# include <lib$routines.h>
#endif
#ifdef WINDOWS32
# include <windows.h>
# include <io.h>
# include <process.h>
# include "sub_proc.h"
# include "w32err.h"
# include "pathstuff.h"
#endif /* WINDOWS32 */
#ifdef HAVE_FCNTL_H
# include <fcntl.h>
#else
# include <sys/file.h>
#endif
#if defined (HAVE_SYS_WAIT_H) || defined (HAVE_UNION_WAIT)
# include <sys/wait.h>
#endif
#ifdef HAVE_WAITPID
# define WAIT_NOHANG(status) waitpid (-1, (status), WNOHANG)
#else /* Don't have waitpid. */
# ifdef HAVE_WAIT3
# ifndef wait3
extern int wait3 ();
# endif
# define WAIT_NOHANG(status) wait3 ((status), WNOHANG, (struct rusage *) 0)
# endif /* Have wait3. */
#endif /* Have waitpid. */
#if !defined (wait) && !defined (POSIX)
extern int wait ();
#endif
#ifndef HAVE_UNION_WAIT
# define WAIT_T int
# ifndef WTERMSIG
# define WTERMSIG(x) ((x) & 0x7f)
# endif
# ifndef WCOREDUMP
# define WCOREDUMP(x) ((x) & 0x80)
# endif
# ifndef WEXITSTATUS
# define WEXITSTATUS(x) (((x) >> 8) & 0xff)
# endif
# ifndef WIFSIGNALED
# define WIFSIGNALED(x) (WTERMSIG (x) != 0)
# endif
# ifndef WIFEXITED
# define WIFEXITED(x) (WTERMSIG (x) == 0)
# endif
#else /* Have `union wait'. */
# define WAIT_T union wait
# ifndef WTERMSIG
# define WTERMSIG(x) ((x).w_termsig)
# endif
# ifndef WCOREDUMP
# define WCOREDUMP(x) ((x).w_coredump)
# endif
# ifndef WEXITSTATUS
# define WEXITSTATUS(x) ((x).w_retcode)
# endif
# ifndef WIFSIGNALED
# define WIFSIGNALED(x) (WTERMSIG(x) != 0)
# endif
# ifndef WIFEXITED
# define WIFEXITED(x) (WTERMSIG(x) == 0)
# endif
#endif /* Don't have `union wait'. */
/* How to set close-on-exec for a file descriptor. */
#if !defined F_SETFD
# define CLOSE_ON_EXEC(_d)
#else
# ifndef FD_CLOEXEC
# define FD_CLOEXEC 1
# endif
# define CLOSE_ON_EXEC(_d) (void) fcntl ((_d), F_SETFD, FD_CLOEXEC)
#endif
#ifdef VMS
static int vms_jobsefnmask = 0;
#endif /* !VMS */
#ifndef HAVE_UNISTD_H
extern int dup2 ();
extern int execve ();
extern void _exit ();
# ifndef VMS
extern int geteuid ();
extern int getegid ();
extern int setgid ();
extern int getgid ();
# endif
#endif
extern char *allocated_variable_expand_for_file PARAMS ((char *line, struct file *file));
extern int getloadavg PARAMS ((double loadavg[], int nelem));
extern int start_remote_job PARAMS ((char **argv, char **envp, int stdin_fd,
int *is_remote, int *id_ptr, int *used_stdin));
extern int start_remote_job_p PARAMS ((int));
extern int remote_status PARAMS ((int *exit_code_ptr, int *signal_ptr,
int *coredump_ptr, int block));
RETSIGTYPE child_handler PARAMS ((int));
static void free_child PARAMS ((struct child *));
static void start_job_command PARAMS ((struct child *child));
static int load_too_high PARAMS ((void));
static int job_next_command PARAMS ((struct child *));
static int start_waiting_job PARAMS ((struct child *));
#ifdef VMS
static void vmsWaitForChildren PARAMS ((int *));
#endif
/* Chain of all live (or recently deceased) children. */
struct child *children = 0;
/* Number of children currently running. */
unsigned int job_slots_used = 0;
/* Nonzero if the `good' standard input is in use. */
static int good_stdin_used = 0;
/* Chain of children waiting to run until the load average goes down. */
static struct child *waiting_jobs = 0;
/* Non-zero if we use a *real* shell (always so on Unix). */
int unixy_shell = 1;
#ifdef WINDOWS32
/*
* The macro which references this function is defined in make.h.
*/
int w32_kill(int pid, int sig)
{
return ((process_kill(pid, sig) == TRUE) ? 0 : -1);
}
#endif /* WINDOWS32 */
/* Write an error message describing the exit status given in
EXIT_CODE, EXIT_SIG, and COREDUMP, for the target TARGET_NAME.
Append "(ignored)" if IGNORED is nonzero. */
static void
child_error (target_name, exit_code, exit_sig, coredump, ignored)
char *target_name;
int exit_code, exit_sig, coredump;
int ignored;
{
if (ignored && silent_flag)
return;
#ifdef VMS
if (!(exit_code & 1))
error (NILF,
(ignored ? _("*** [%s] Error 0x%x (ignored)")
: _("*** [%s] Error 0x%x")),
target_name, exit_code);
#else
if (exit_sig == 0)
error (NILF, ignored ? _("[%s] Error %d (ignored)") :
_("*** [%s] Error %d"),
target_name, exit_code);
else
error (NILF, "*** [%s] %s%s",
target_name, strsignal (exit_sig),
coredump ? _(" (core dumped)") : "");
#endif /* VMS */
}
#ifdef VMS
/* Wait for nchildren children to terminate */
static void
vmsWaitForChildren(int *status)
{
while (1)
{
if (!vms_jobsefnmask)
{
*status = 0;
return;
}
*status = sys$wflor (32, vms_jobsefnmask);
}
return;
}
/* Set up IO redirection. */
char *
vms_redirect (desc, fname, ibuf)
struct dsc$descriptor_s *desc;
char *fname;
char *ibuf;
{
char *fptr;
extern char *vmsify ();
ibuf++;
while (isspace (*ibuf))
ibuf++;
fptr = ibuf;
while (*ibuf && !isspace (*ibuf))
ibuf++;
*ibuf = 0;
if (strcmp (fptr, "/dev/null") != 0)
{
strcpy (fname, vmsify (fptr, 0));
if (strchr (fname, '.') == 0)
strcat (fname, ".");
}
desc->dsc$w_length = strlen(fname);
desc->dsc$a_pointer = fname;
desc->dsc$b_dtype = DSC$K_DTYPE_T;
desc->dsc$b_class = DSC$K_CLASS_S;
if (*fname == 0)
printf (_("Warning: Empty redirection\n"));
return ibuf;
}
/*
found apostrophe at (p-1)
inc p until after closing apostrophe. */
static char *
handle_apos (char *p)
{
int alast;
int inside;
#define SEPCHARS ",/()= "
inside = 0;
while (*p != 0)
{
if (*p == '"')
{
if (inside)
{
while ((alast > 0)
&& (*p == '"'))
{
p++;
alast--;
}
if (alast == 0)
inside = 0;
else
{
fprintf (stderr, _
没有合适的资源?快使用搜索试试~ 我知道了~
make-3.79.tar.gz
需积分: 1 0 下载量 157 浏览量
2024-01-30
00:27:41
上传
评论
收藏 937KB GZ 举报
温馨提示
GNU Make 是一种广泛使用的工具,它使得开发者能够自动化编译和构建过程,特别是在处理大型项目和复杂的源代码结构时。这个工具读取包含有关如何构建程序的指令的文件(通常称为 Makefile),然后执行这些指令来自动编译和链接源代码,生成可执行文件或库。GNU Make 对于那些需要精确控制构建过程和自动化复杂构建任务的开发项目来说尤其重要。它不仅被用于大型软件开发项目,还被广泛用于小型项目,以提高开发效率和减少重复性工作。
资源推荐
资源详情
资源评论
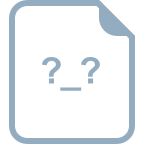
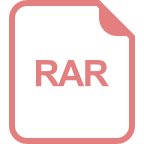
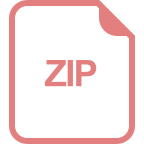
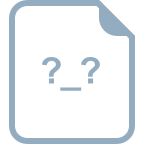
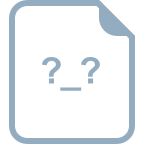
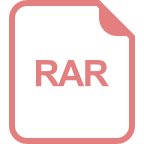
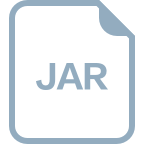
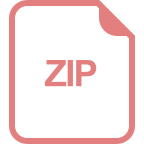
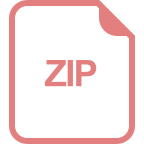
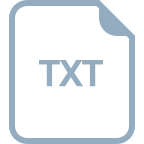
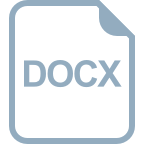
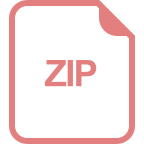
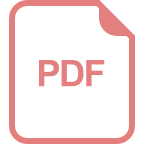
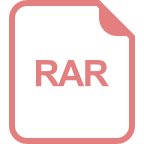
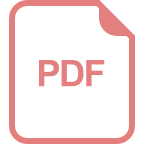
收起资源包目录

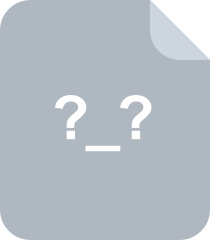
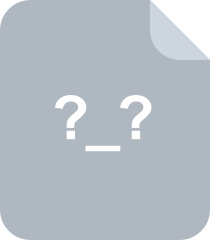
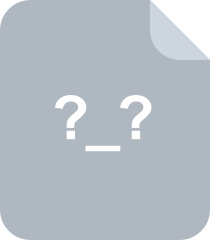
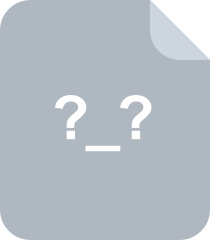
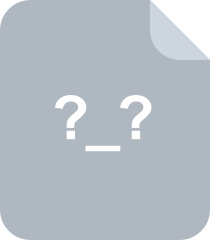
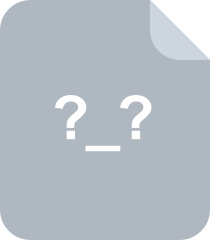
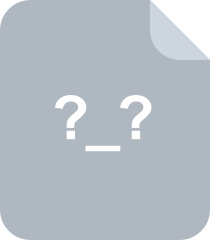
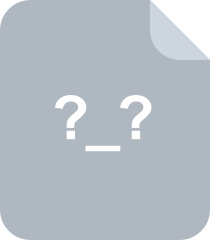
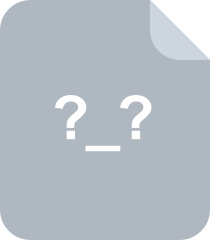
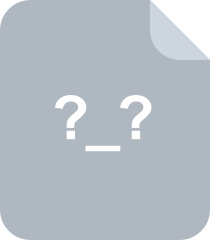
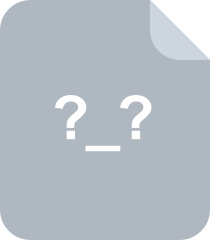
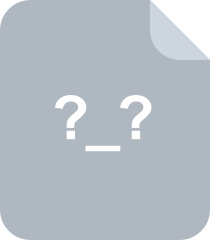
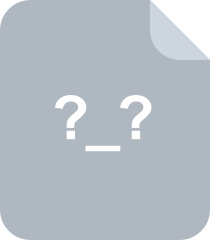
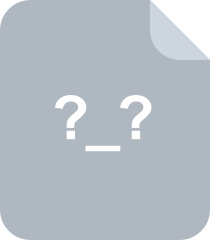
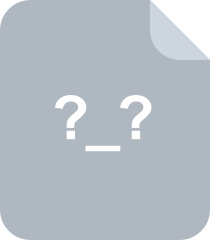
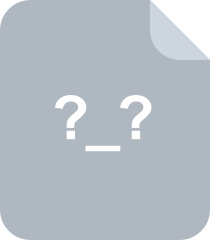
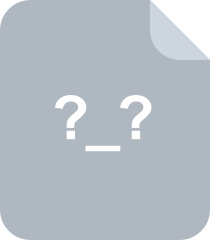
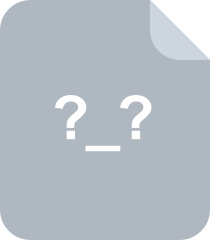
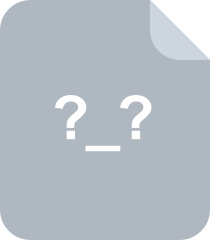
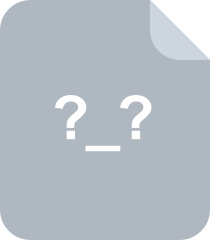
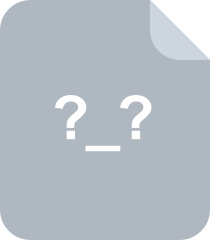
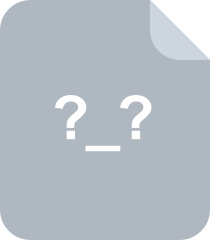
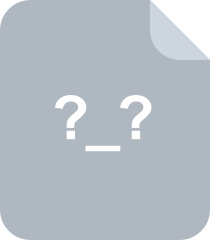
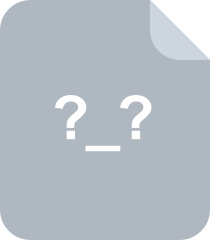
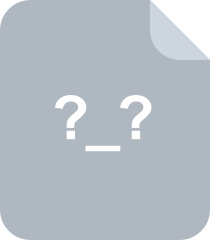
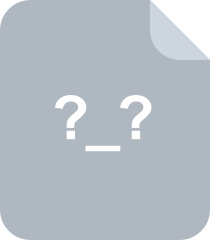
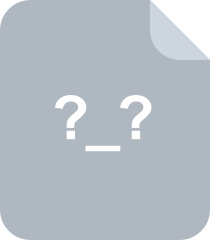
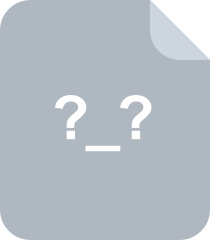
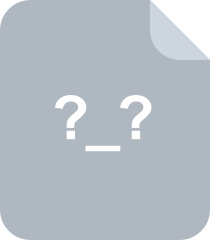
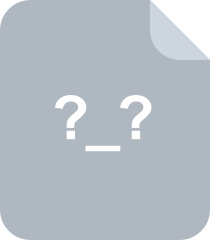
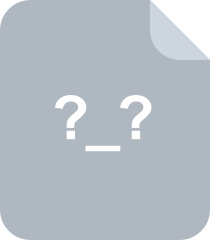
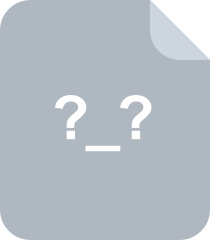
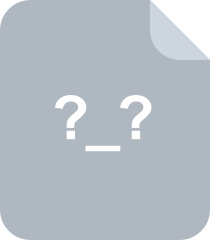
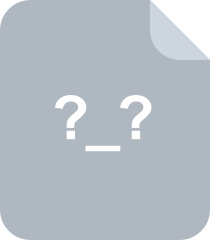
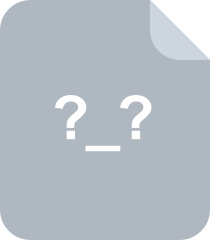
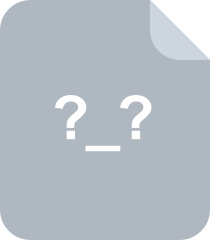
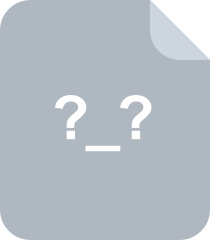
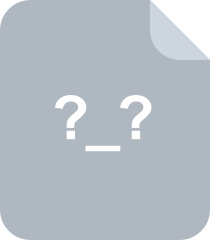
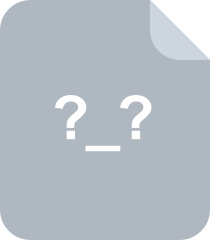
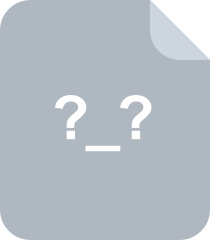
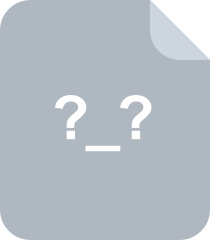
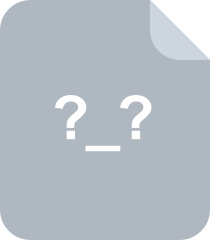
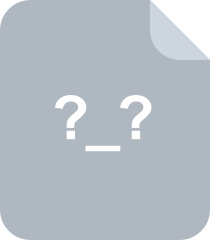
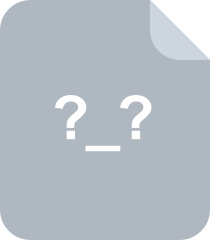
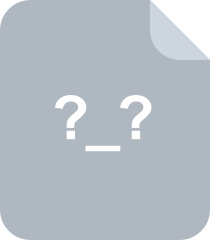
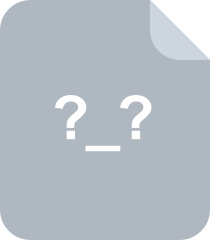
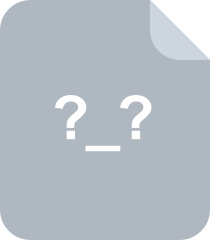
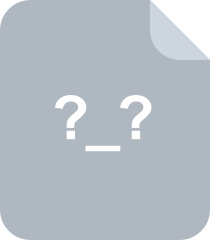
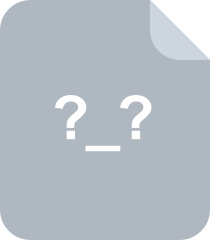
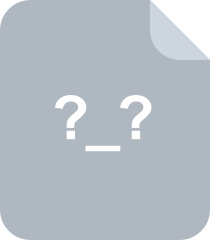
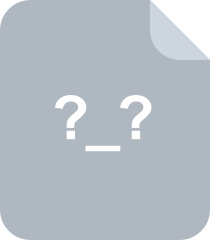
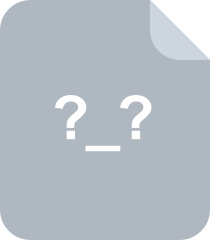
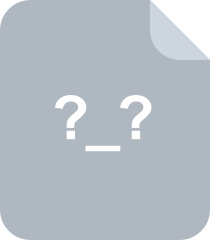
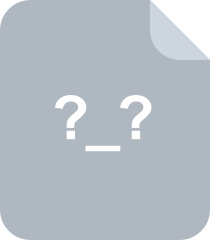
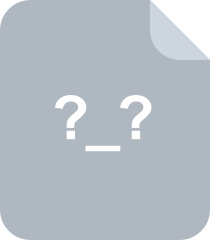
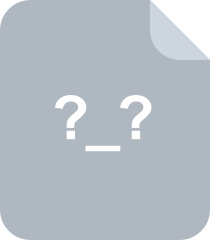
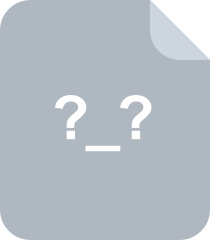
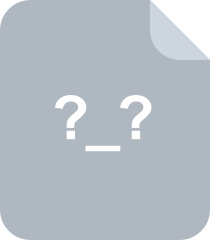
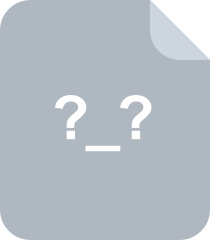
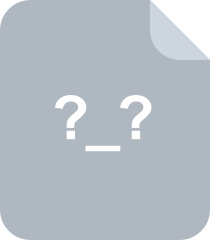
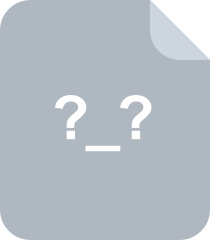
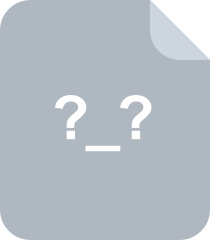
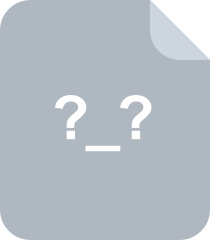
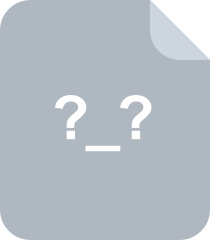
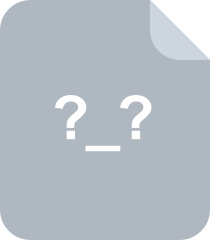
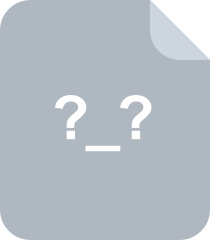
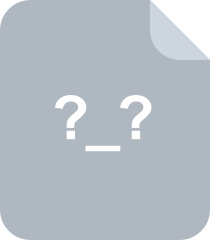
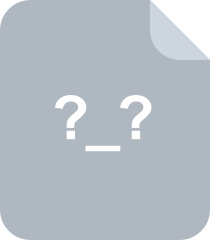
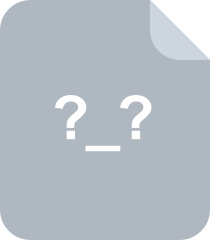
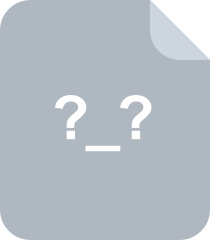
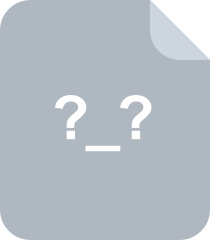
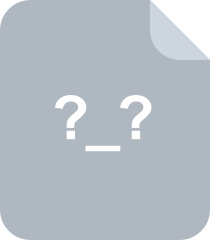
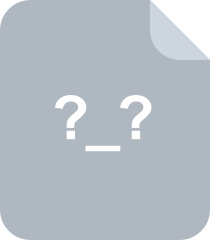
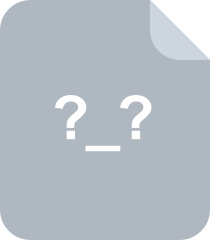
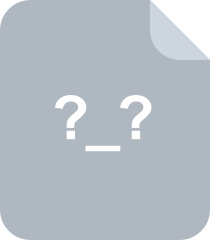
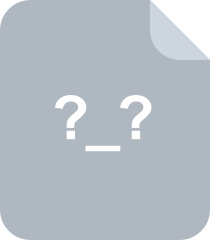
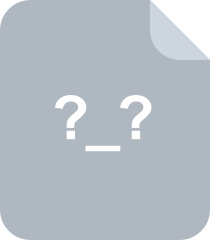
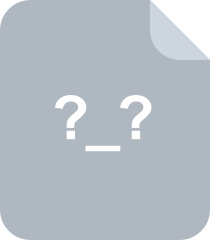
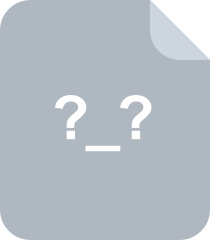
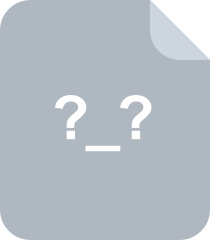
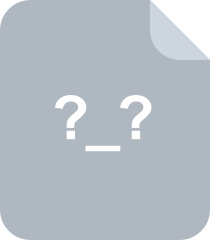
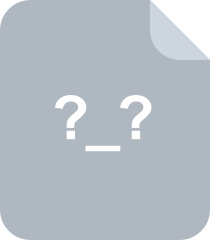
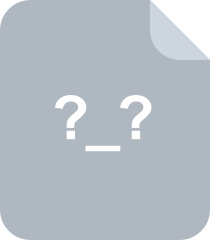
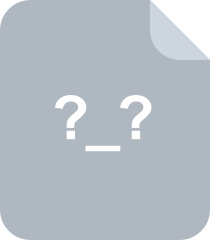
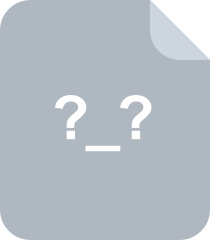
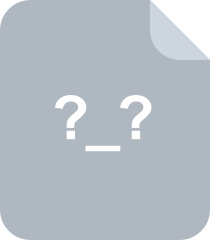
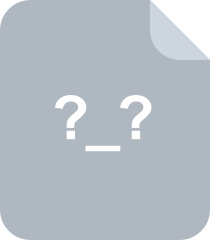
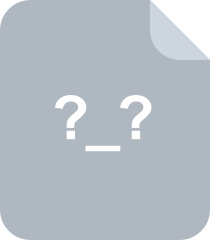
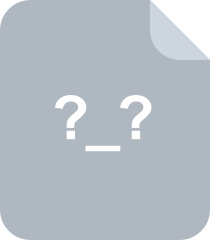
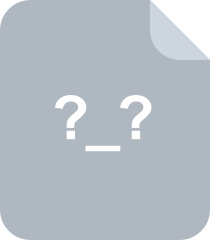
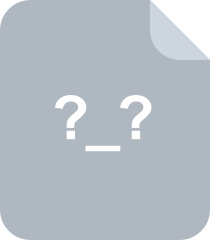
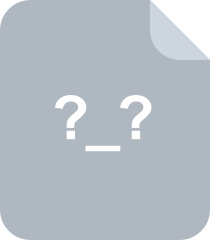
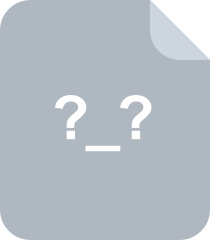
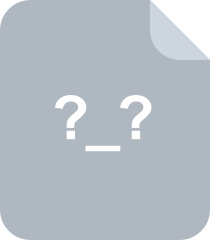
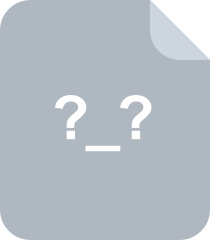
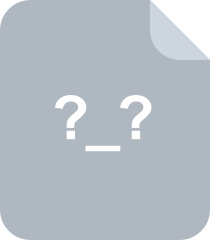
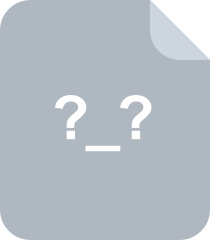
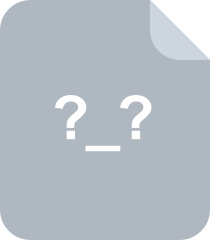
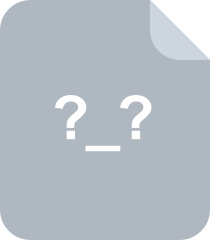
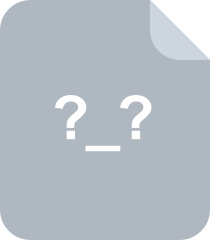
共 217 条
- 1
- 2
- 3
资源评论
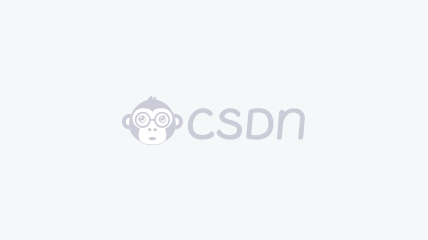

程序员Chino的日记
- 粉丝: 3672
- 资源: 5万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

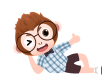
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


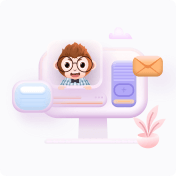
安全验证
文档复制为VIP权益,开通VIP直接复制
