#include<windows.h>
#include<process.h>
#include<time.h>
#include<math.h>
#include<wingdi.h>
#include"resource.h"
#pragma comment(lib,"winmm.lib")
typedef struct
{
HWND hwnd;
int cxClient;
int cyClient;
int cyChar;
BOOL bKill;
}
PARAMS,*PPARAMS;
TCHAR szChildClass[]=TEXT("ChildWnd");
LRESULT CALLBACK WndProc (HWND ,UINT ,WPARAM ,LPARAM);
LRESULT CALLBACK ChildWndProc(HWND,UINT,WPARAM,LPARAM);
void loadImages(HINSTANCE);
void Thread(PVOID);
void hammerhit(int,PPARAMS);
void random(int,PPARAMS);
//void moleGo(int, PPARAMS);
void Draw( HDC );
HANDLE thread;
HWND hAppHwnd;
HDC bufferDC=NULL;
HDC bkDC = NULL;
HDC hdc;
HBITMAP bufferBMP = NULL;
HBITMAP hBkImage[5],hBkMask[5];
RECT g_ClientRect;
RECT g_GameValueRect;
BOOL isHit=FALSE;
TCHAR szBuffer[20];
int g_iClientWidth;
int g_iClientHeight;
int hitClock;
static int threadN=0;
static int score=0;// 分数
static int level = 1;//等级
static int Time =80;//时间
static int count =0 ;//控制游戏速度的计数器
static int isOver = 0;//结束标志位
static int stayTime[3]={20,20,10};
static BOOL iconState=FALSE;
static int state[3][3]={0},clocks[3][3]={0};
static POINT point[3][3]={{{135,113},{272,113},{408,113}},{{107,198},{267,198},{423,198}},{{65,305},{254,305},{439,305}}};//地鼠的位置坐标
static int nSpeed[3]={9,12,18};//三个地鼠的速度
static int nyTemp[3][3]={0};
static int isLive[3][3]={0};//老鼠是否存活
static int isPause=0;
static int isStop = 0;
static int cxClient,cyClient,cxBitmap,cyBitmap;
static POINT pt,pe;
static BITMAP bitmap[13],BKbitmap[5];
static HBITMAP hBitmapImage[13],hBitmapMask[13];
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, PSTR szCmdLine, int iCmdShow)
{
static TCHAR szAppName[]=TEXT("WacamoleWin");
HWND hwnd;
MSG msg;
WNDCLASS wndclass;
wndclass.style = CS_HREDRAW|CS_VREDRAW;
wndclass.lpfnWndProc = WndProc;
wndclass.cbClsExtra = 0;
wndclass.cbWndExtra = 0;
wndclass.hInstance = hInstance;
wndclass.hIcon = LoadIcon(NULL,IDI_APPLICATION);
wndclass.hCursor = LoadCursor(hInstance,MAKEINTRESOURCE(IDC_CURSOR1));
wndclass.hbrBackground = (HBRUSH)GetStockObject(WHITE_BRUSH);
wndclass.lpszMenuName = NULL;
wndclass.lpszClassName = szAppName;
if(!RegisterClass(&wndclass))
{
MessageBox(NULL,TEXT("This program requires Windows NT!"),szAppName,MB_ICONERROR);
return 0;
}
wndclass.lpfnWndProc = ChildWndProc;
wndclass.cbWndExtra = sizeof(long);
wndclass.hIcon = NULL;
wndclass.lpszClassName = szChildClass;
RegisterClass(&wndclass);
hwnd = CreateWindow(szAppName,
TEXT("Wacamole"),
WS_OVERLAPPEDWINDOW,
400,
50,
655,
630,
NULL,
NULL,
hInstance,
NULL
);
hAppHwnd=hwnd;
ShowWindow(hwnd,iCmdShow);
UpdateWindow(hwnd);
hdc=GetDC(hwnd);
bufferDC=CreateCompatibleDC(hdc);
bkDC=CreateCompatibleDC(hdc);
GetClientRect(hwnd, &g_ClientRect);
g_iClientWidth = g_ClientRect.right-g_ClientRect.left;
g_iClientHeight = g_ClientRect.bottom-g_ClientRect.top;
bufferBMP=CreateCompatibleBitmap(hdc,g_iClientWidth,g_iClientHeight);
//hBK=(HBITMAP)LoadImage(hInstance,LPCWSTR(IDB_BK),IMAGE_BITMAP,0,0,LR_DEFAULTCOLOR);
SelectObject(bufferDC,bufferBMP);
//SelectObject(bkDC,hBK);
while(GetMessage(&msg,NULL,0,0))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return msg.wParam;
}
LRESULT CALLBACK WndProc(HWND hwnd, UINT message, WPARAM wParam, LPARAM lParam)
{
static HWND hwndChild[3];
HDC hdcMemImage,hdcMemMask;
PAINTSTRUCT ps;
RECT rect;
HINSTANCE hInstance;
int hitx,hity;//击打时的坐标
//int cyBitmap,cxBitmap;
switch(message)
{
case WM_CREATE:
hInstance = ((LPCREATESTRUCT)lParam)->hInstance;
//hBK = (HBITMAP)LoadImage(hInstance,(LPCWSTR)IDB_BK,IMAGE_BITMAP,
// 0,0,LR_DEFAULTCOLOR);
loadImages(hInstance);
for(int i = 0;i<3;i++)
for(int j= 0;j<3;j++)
{
state[i][j]=0;
clocks[i][j]=0;
}
for(int i = 0;i<1;i++)
{
hwndChild[i]=CreateWindow(szChildClass,
NULL,
WS_CHILDWINDOW/*|WS_VISIBLE*/,
0,0,0,0,
hwnd,
(HMENU)(i),
(HINSTANCE)GetWindowLong(hwnd,GWL_HINSTANCE),
NULL);
}
//PlaySound(TEXT("123.wav"),NULL,SND_FILENAME|SND_ASYNC|SND_LOOP);
return 0;
case WM_SIZE:
cxClient=LOWORD(lParam);
cyClient=HIWORD(lParam);
//BitBlt(hdc,0,0,cxClient,cyClient,bkDC,0,0,SRCCOPY);
/*for(int i = 0;i<3;i++){
cxBitmap = bitmap[8].bmWidth;
cyBitmap = bitmap[8].bmHeight;
MoveWindow(hwndChild[i],150*(i+1),500,cxBitmap,cyBitmap,TRUE);
}*/
return 0;
case WM_LBUTTONDOWN:
hitx = LOWORD(lParam);
hity = HIWORD(lParam);
isHit=TRUE;
hitClock=4;
for(int i=0;i<3;i++)
for(int j=0;j<3;j++)
{
if(clocks[i][j]>=10&&clocks[i][j]<=stayTime[1]+10&&isLive[i][j]==1)
{
if(hitx-65>point[i][j].x&&hitx-65<point[i][j].x+bitmap[i*2].bmWidth
&&hity-60>point[i][j].y&&hity-60<point[i][j].y+bitmap[i*2].bmHeight)
{
PlaySound(TEXT("1381.wav"),NULL,SND_FILENAME|SND_ASYNC|SND_NOSTOP);
isLive[i][j]=0;
clocks[i][j]=10;
score++;
if(score>=20)
level=2;
if(score>=40)
level=3;
}
}
}
if(hitx>150&&hitx<150+bitmap[8].bmWidth&&hity>500&&hity<500+bitmap[8].bmHeight)
{
if(isPause==0&&threadN==0){
SendMessage(hwndChild[0],WM_LBUTTONDOWN,0,0);
threadN=1;
}
else
isPause=0;
if(isStop==1)
{
isPause=0;
isStop=0;
isOver=0;
}
}
if(hitx>300&&hitx<300+bitmap[8].bmWidth&&hity>500&&hity<500+bitmap[8].bmHeight)
{
isPause=1;
}
if(hitx>450&&hitx<450+bitmap[8].bmWidth&&hity>500&&hity<500+bitmap[8].bmHeight)
{
isStop=1;
// threadN=0;
}
/* if(clocks[i][j]>=10)*/
InvalidateRect(hwnd,NULL,FALSE);
return 0;
case WM_MOUSEMOVE:
pt.x = LOWORD(lParam);
pt.y = HIWORD(lParam);
if(pt.y>490)
iconState=TRUE;
else
iconState=FALSE;
InvalidateRect(hwnd,NULL,FALSE);
return 0;
case WM_PAINT:
hdc=BeginPaint(hwnd,&ps);
GetClientRect(hwnd,&rect);
//DrawState(hdc,NULL,NULL,(LPARAM)hBK,0,0,0,0,0,DST_BITMAP);
Draw(bufferDC);
BitBlt(hdc,0,0,cxClient,cyClient,bufferDC,0,0,SRCCOPY);
EndPaint(hwnd,&ps);
return 0;
case WM_DESTROY:
DeleteObject (hBitmapImage) ;
DeleteObject (hBitmapMask) ;
PostQuitMessage(0);
return 0;
}
return DefWindowProc(hwnd, message, wParam,lParam);
}
LRESULT CALLBACK ChildWndProc(HWND hwnd,UINT message,WPARAM wParam,LPARAM lParam)
{
HDC hdc,hdcMemImage,hdcMemMask;
PAINTSTRUCT ps;
RECT rect;
int cxBitmap,cyBitmap;
static PARAMS params;
switch(message)
{
case WM_CREATE:
return 0;
case WM_LBUTTONDOWN:
//MessageBox(hwnd,TEXT("sss"),NULL,MB_OK);
params.hwnd = GetParent(hwnd);
//thread = CreateThread(NULL,0,Thread(),)
_beginthread(Thread,0,¶ms);
InvalidateRect(hwnd, NULL,FALSE);
return 0;
case WM_PAINT:
/*hdc = BeginPaint(hwnd,&ps);
hdcMemImage = CreateCompatibleDC (hdc) ;
SelectObject (hdcMemImage, hBitmapImage[8]) ;
hdcMemMask = CreateCompatibleDC (hdc) ;
SelectObject (hdcMemMask, hBitmapMask[8]) ;
cxBitmap = bitmap[8].bmWidth;
cyBitmap = bitmap[8].bmHeight;
BitBlt (hdc, 0, 0, cxBitmap, cyBitmap, hdcMemMask, 0, 0, 0x220326) ;
BitBlt (hdc, 0, 0, cxBitmap, cyBitmap, hdcMemImage, 0, 0, SRCPAINT) ;
DeleteDC (hdcMemImage) ;
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
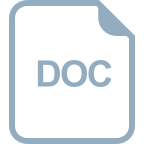
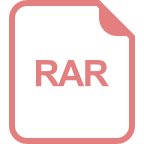
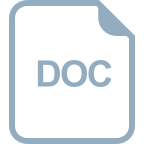
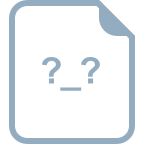
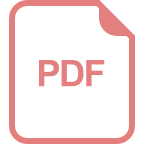
收起资源包目录

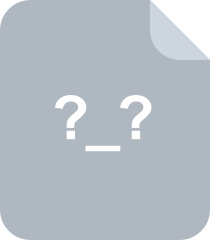
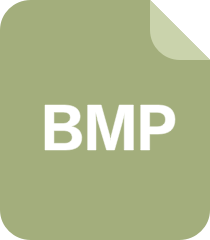
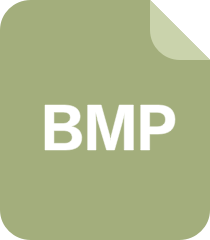
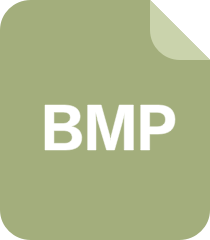
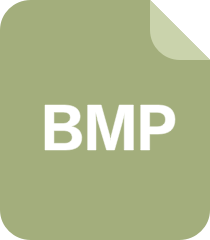
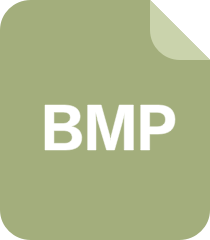
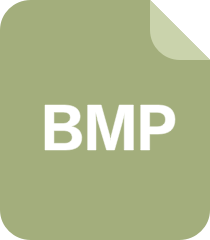
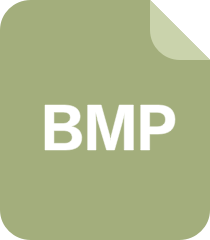
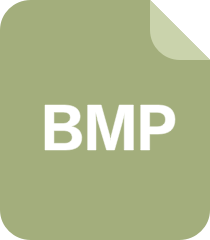
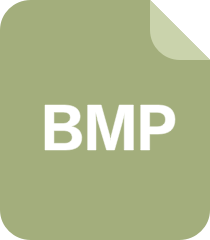
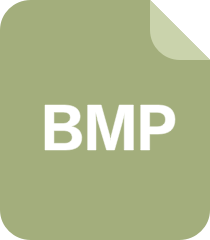
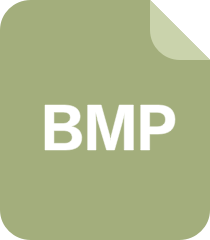
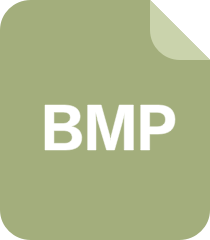
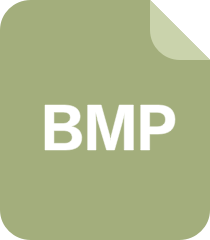
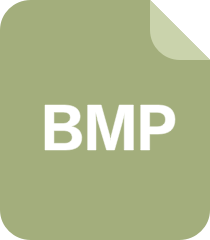
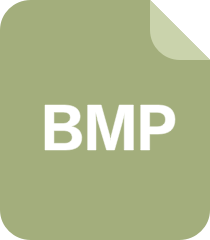
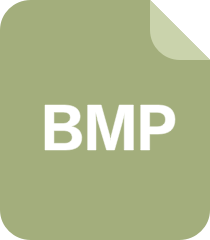
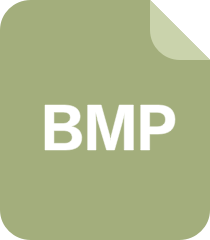
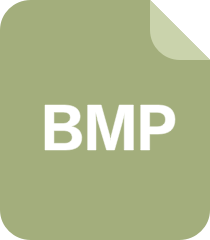
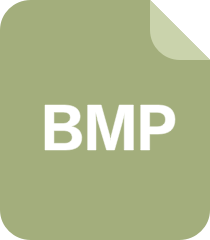
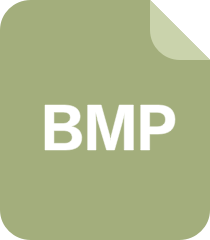
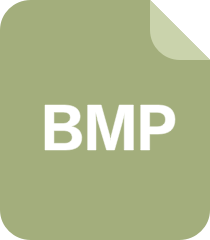
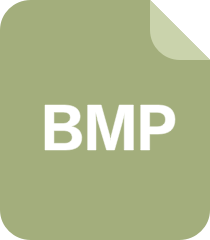
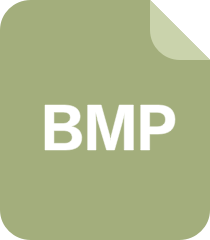
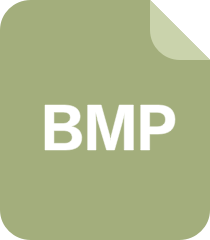
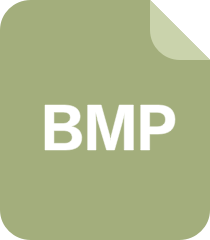
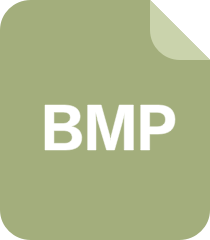
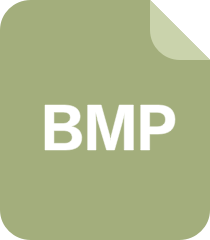
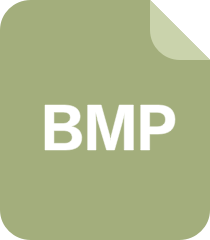
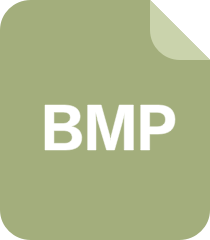
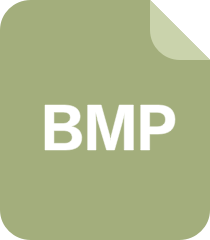
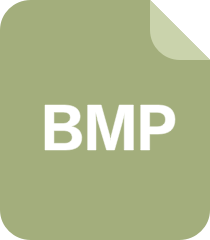
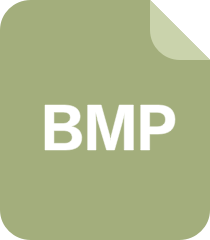
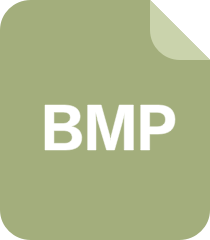
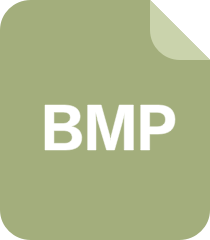
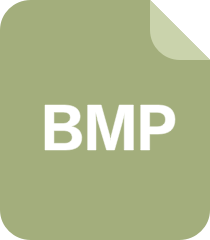
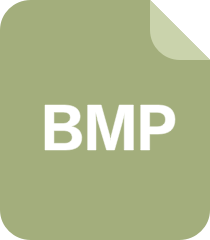
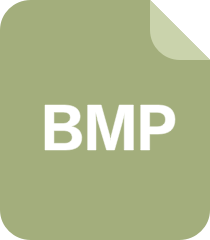
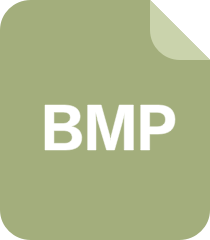
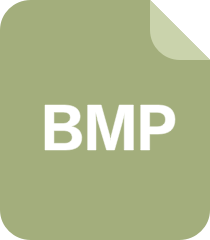
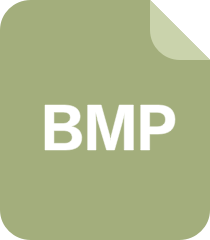
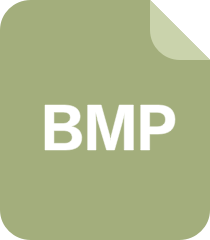
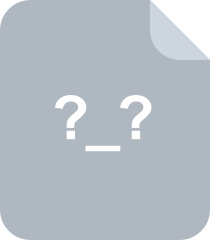
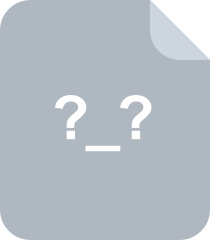
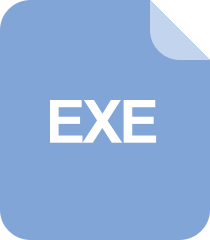
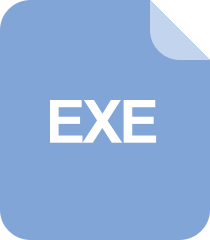
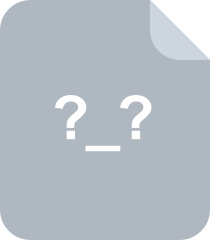
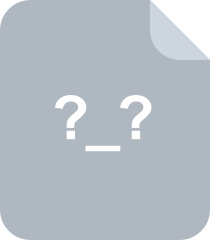
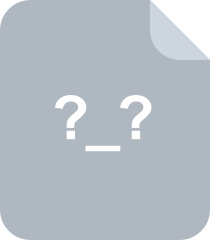
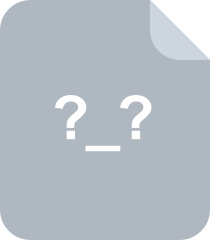
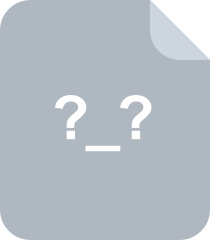
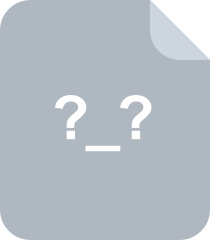
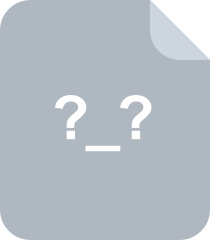
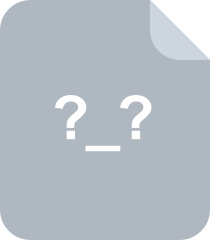
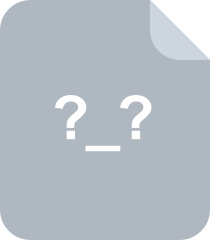
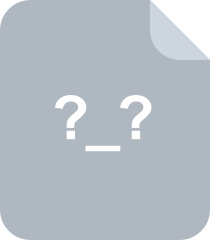
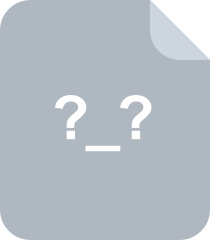
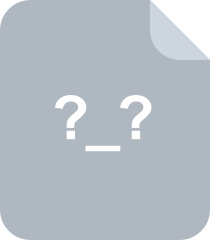
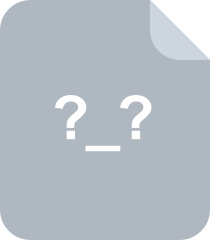
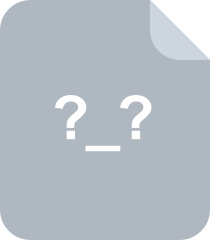
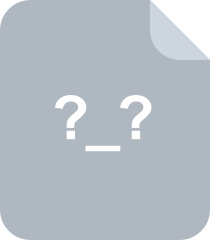
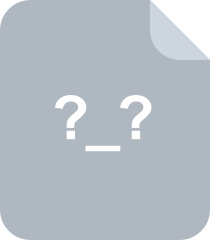
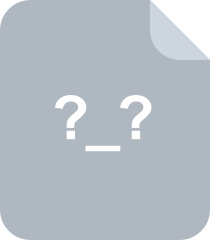
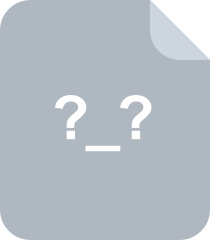
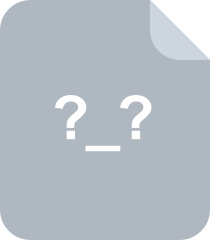
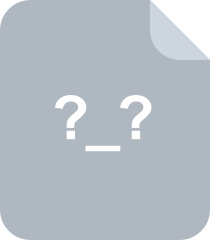
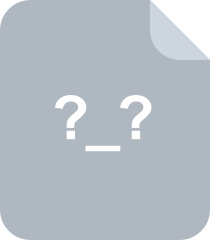
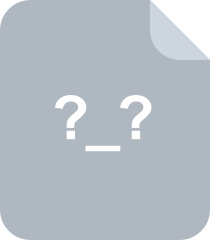
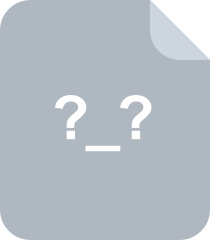
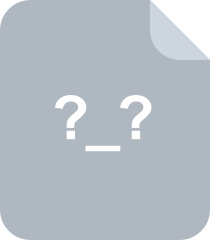
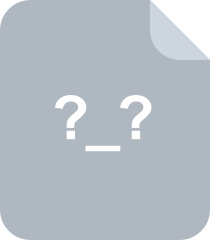
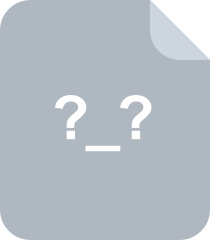
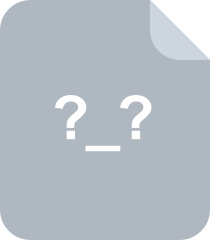
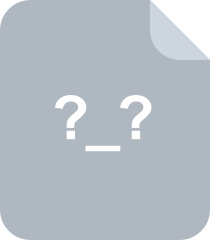
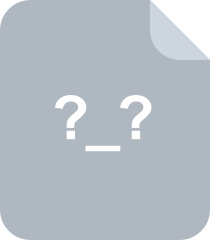
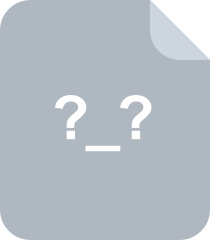
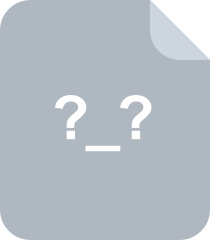
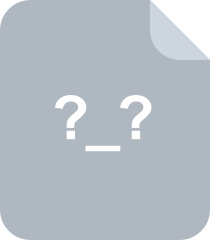
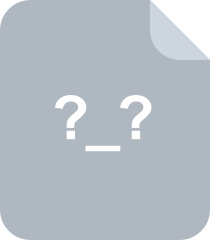
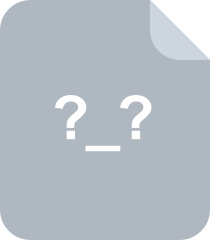
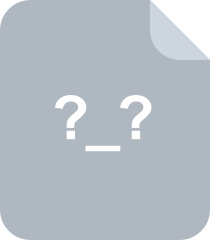
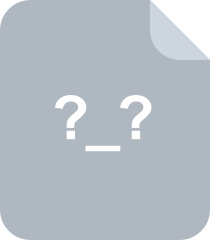
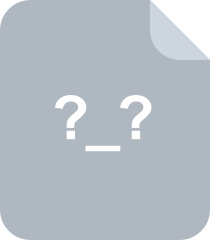
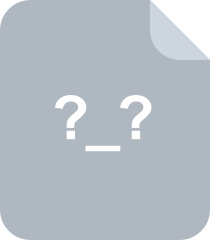
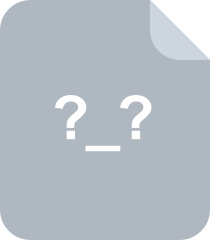
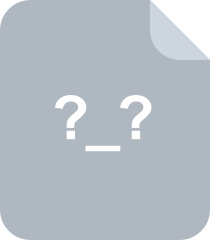
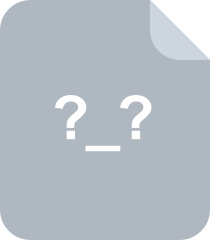
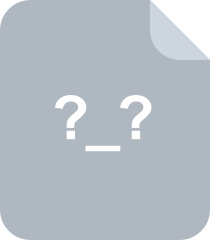
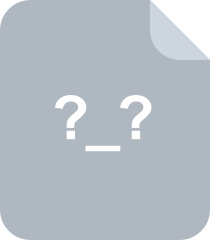
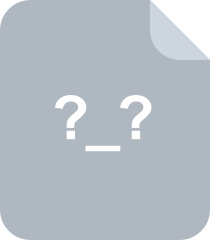
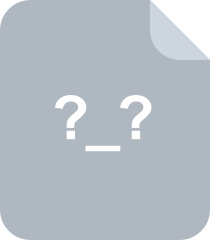
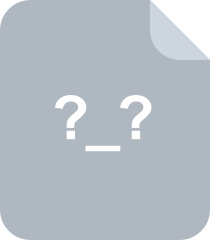
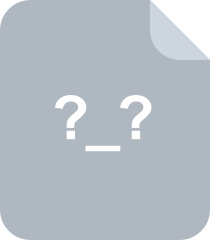
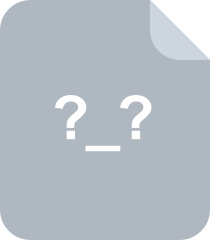
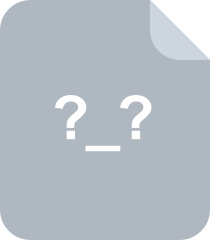
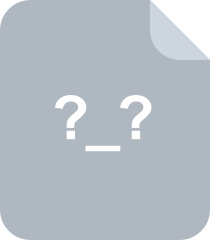
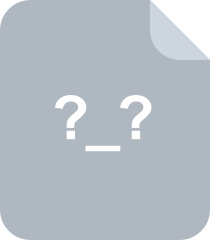
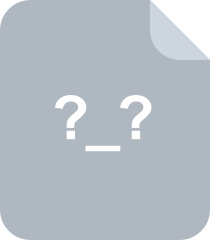
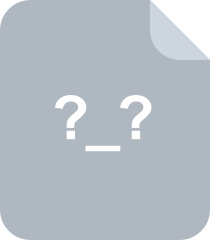
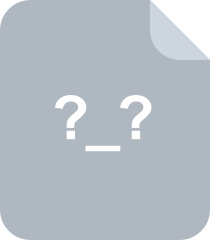
共 109 条
- 1
- 2
资源评论
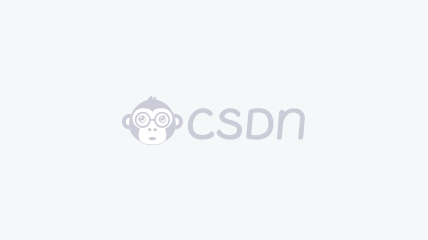
- qq_169856012015-06-28很不错的资源,代码详细,界面清晰。

caoshupng
- 粉丝: 12
- 资源: 4
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

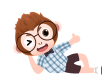
安全验证
文档复制为VIP权益,开通VIP直接复制
