import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import javax.swing.ButtonGroup;
import javax.swing.JButton;
import javax.swing.JCheckBox;
import javax.swing.JComboBox;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JRadioButton;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.JTextField;
import javax.swing.table.DefaultTableModel;
public class teaPanel extends JPanel {
private JComboBox comboBox_1;
private JComboBox comboBox;
private JCheckBox checkBox_6;
/**
*
*/
private static final long serialVersionUID = 6678676176512765166L;
private JTextField textField_7;
private JTextField textField_6;
private JButton button_5;
JButton button_2,button,button_1,button_3,button_4 ;
private JTable table;
private ButtonGroup buttonGroup = new ButtonGroup();
private JTextField textField_2;
private JTextField textField_1;
private JTextField textField;
private JCheckBox checkBox,checkBox_1,checkBox_2,checkBox_3,checkBox_4;
private JLabel label,label_1;
private JRadioButton radioButton,radioButton_1;
private JScrollPane scrollPane;
String zhuanye[]={"软件工程","计算机","数字媒体","动漫","数学","俄语","英语"};
String kecheng[]={"Java语言","操作系统","计算机原","数据库","计组","高等数学1","离散数学","数值计算","大学英语"};
String teainf[]={"教工号","姓名","年龄","性别","系别"};
DataBase db;
Connection con=null;
Statement st=null;
ResultSet rs=null;
String sql;
DefaultTableModel teaTableModel;
Teacher tea=null;
/**
* Create the panel
*/
public teaPanel() {
super();
setVisible(true);
setLayout(null);
checkBox = new JCheckBox();
checkBox.setText("教工号:");
checkBox.setBounds(0, 10, 79, 26);
add(checkBox);
checkBox_1 = new JCheckBox();
checkBox_1.setText("姓名:");
checkBox_1.setBounds(178, 10, 64, 26);
add(checkBox_1);
checkBox_2 = new JCheckBox();
checkBox_2.setText("年龄:");
checkBox_2.setBounds(0, 42, 64, 26);
add(checkBox_2);
textField = new JTextField();
textField.setBounds(248, 10, 87, 26);
add(textField);
textField_1 = new JTextField();
textField_1.setBounds(85, 10, 87, 26);
add(textField_1);
textField_2 = new JTextField();
textField_2.setBounds(85, 42, 39, 26);
add(textField_2);
radioButton = new JRadioButton();
buttonGroup.add(radioButton);
radioButton.setText("男");
radioButton.setSelected(true);
radioButton.setBounds(248, 42, 39, 26);
add(radioButton);
checkBox_3 = new JCheckBox();
checkBox_3.setText("性别:");
checkBox_3.setBounds(178, 42, 64, 26);
add(checkBox_3);
radioButton_1 = new JRadioButton();
buttonGroup.add(radioButton_1);
radioButton_1.setText("女");
radioButton_1.setBounds(293, 42, 39, 26);
add(radioButton_1);
checkBox_4 = new JCheckBox();
checkBox_4.setText("系别:");
checkBox_4.setBounds(0, 81, 64, 26);
add(checkBox_4);
button = new JButton();
button.addActionListener(new ActionListener() {
public void actionPerformed(final ActionEvent e) {
try{
db=new DataBase();
con=db.getCon();
st=con.createStatement();
}catch(SQLException e2){
JOptionPane.showMessageDialog(null, "数据连接错误!");
System.exit(0);
}
String hasjiaogonghao="",hasxingming="",hasnianling="",hasxingbie="",hasxihao="",haskecheng="",needshouke="";
if(checkBox.isSelected()){
if(textField_1.getText().equals("")){
JOptionPane.showMessageDialog(null, "请输入要查询的教工号!");
}
hasjiaogonghao=" and teacher.教工号='"+textField_1.getText()+"'";
}
if(checkBox_1.isSelected()){
if(textField.getText().equals("")){
JOptionPane.showMessageDialog(null, "请输入教师姓名!");
}
else{
hasxingming=" and 姓名='"+textField.getText()+"'";
}
}
if(checkBox_2.isSelected()){
if(textField_2.getText().equals("")){
JOptionPane.showMessageDialog(null, "请输入教师的年龄!");
}
else{
hasnianling=" and 年龄='"+textField_2.getText()+"'";
}
}
if(checkBox_3.isSelected()){
if(radioButton.isSelected()){
hasxingbie=" and 性别='"+radioButton.getText()+"'";
}
else{
hasxingbie=" and 性别='"+radioButton_1.getText()+"'";
}
}
if(checkBox_4.isSelected()){
sql="select 系号 from depart where 系别='"+comboBox_1.getSelectedItem()+"'";
System.out.println(sql);
try{
System.out.println(sql);
rs=st.executeQuery(sql);
if(rs.next()){
hasxihao=" and teacher.系号='"+rs.getString(1)+"'";
rs.close();
}
else{
//donothing
}
}catch(SQLException e1){
e1.printStackTrace();
JOptionPane.showMessageDialog(null, "执行SQL语句"+sql+"时出错!");
}
}
if(checkBox_6.isSelected()){
needshouke=",shouke,course ";
haskecheng=" and teacher.教工号=shouke.教工号 and shouke.课程号=course.课程号 and course.课程名='"+comboBox.getSelectedItem()+"'";
}
sql="select teacher.教工号,teacher.姓名,teacher.年龄,teacher.性别,depart.系别 from teacher,depart"+needshouke+" where teacher.系号=depart.系号 "+haskecheng+hasjiaogonghao+hasnianling+hasxingbie+hasxingming+hasxihao ;
try {
String[] s = new String[5];
int rows = teaTableModel.getRowCount();
if (rows != 0){
for (int i = 0; i < rows; i++)
teaTableModel.removeRow(0);}
int i=0;
if(!checkBox.isSelected()&&!checkBox_1.isSelected()&&!checkBox_2.isSelected()&&!checkBox_3.isSelected()&&!checkBox_4.isSelected()&&!checkBox_6.isSelected()){
JOptionPane.showMessageDialog(null, "请选择你要查询的条件和内容!");
}
else if((checkBox.isSelected()&&textField_1.getText().equals(""))||
(checkBox_1.isSelected()&&textField.getText().equals(""))||
(checkBox_2.isSelected()&&textField_2.getText().equals(""))||
(checkBox.isSelected()&&textField.getText().equals(""))
){
//donothing
}
else{
System.out.println(sql);
rs=st.executeQuery(sql);
while (rs.next()) {
for ( i = 1; i <= 5; i++)
s[i - 1] = rs.getString(i);
teaTableModel.addRow(s);
}
if(i==0){
JOptionPane.showMessageDialog(null, "未找到与该条件匹配的教师!");
}
}} catch (SQLException e2) {
JOptionPane.showMessageDialog(null, "执行SQL语句"+sql+"时出错!");
e2.printStackTrace();
}
}
});
button.setText("查询");
button.setBounds(341, 9, 63, 28);
add(button);
button_1 = new JButton();
button_1.addActionListener(new ActionListener() {
public void actionPerformed(final ActionEvent e) {
try{
db=new DataBase();
con=db.getCon();
st=con.createStatement();
}catch(SQLException e2){
JOptionPane.showMessageDialog(null, "数据连接错误!");
System.exit(0);
}
tea=new Teacher();
if(!checkBox.isSelected()){
JOptionPane.showMessageDialog(null, "教工号必须选择!");
}
else if(checkBox.isSelected()&&textField_1.getText().equals("")){
JOptionPane.showMessageDialog(null, "请填写教工号!");
}
else{
tea.setTno(textField_1.getText());
sql="select * from teacher where 教工号='"+tea.getTno()+"'";
try {
System.out.println(sql);
rs=st.executeQuery(sql);
if(rs.next()){
System.out.println("you");
tea.setTname(rs.getString(2));
tea.setAge(rs.getInt(4));
tea.setSex(rs.getString(3));
tea.setXihao(rs.getString(5));
if(checkBox_1.isSelected()){
if(textField.getText().equals("")){
JOptionPane.showMessageDialog(null, "请输入教师姓名!");
}
else{
tea.set

c1032176200
- 粉丝: 41
- 资源: 31
最新资源
- simulink 三机九节点系统风电调频,mppt运行下附有下垂控制和惯性控制,风电渗透率20%,带参考文献
- 西门子smart200plc与4台台达变频器modbus通讯 1,读写变频器的内部参数 2,控制变频器启停,读频率电流 3,设置变频器输出频率 4,有彩色接线图,和参数设置说明, 昆仑通泰触摸程序 有
- MATLAB环境下EMG信号降噪(去除心脏干扰)方法 算法运行环境为MAT;AB R2018a,执行MATLAB环境下EMG信号降噪(去除心脏干扰),采用概率自适应模板减法PATS,经验模态分解方法E
- 条形码检测 avt相机 halcon联合C++联合C#读条码源码 AVT的CCD相机飞拿采集图片,流水线上面运行,传感器感应条形码,相机采图,识别二维码,当读取二维码不联系后,开始通过串口控制输出点停
- 基于Windows.RTX64实时系统的仿真系统框架,用于分析工作时序(ns级),系统动态分析,集采集、分析、处理等于一体的多功能系统 可搭载光纤卡,图像卡,高速A D等板卡
- Simulink集成外部C语言实现PID功能,与Simulink自带pid模块功能一致 可直接进行DSP28335等的代码生成,拖过来直接用,加快开发进度 下图三显示这6种方式达到了基本一致的效果
- 分布式驱动电动汽车LQR DYC 直接横摆力矩控制 最优 规则扭矩分配控制pid计算纵向扭矩需求, 上层lqr计算 下层最小附着利用率分配 扭矩分配 效果优良 稳定性控制 操纵稳定性 matlab
- 四轮独立驱动电动汽车的车辆状态估计,分别采用无迹卡尔曼,容积卡尔曼,高阶容积卡尔曼观测器等,可估计包括纵向速度,质心侧偏角,横摆角速度,以及四个车轮角速度七个状态 模型中第一个模块是四轮驱动电机用f
- 开发板STM32 三轴联动 带插补 加减速 源代码 MDK 源码 分别基于STM32F1和STM32F4两套的三轴联动插补(直线圆弧两种带)加减速的源码,基于国外写的脱机简易雕刻机源码的项目修改,添加
- 三相电压型PWM整流器+双向buck boost matlab仿真 电压电流双闭环控制 dq变 波形完美 ps:可代做仿真
- 基于固定400Hz正弦信号注入的在线搜索永磁同步电机MTPA自动追踪运行的仿真,考虑了电感的饱和以及电机参数的变化,不需要电机的具体参数便可以自动寻求到最佳MTPA工作点,模型采用离散化的方式运行,与
- 基恩士KV7500 程序 ~ 基恩士KV7500系列程序,KV7500+KV-C64X+KV-C64T等输入输出模块,KV-XH16EC定位控制模块 检测机程序 松下A6系列总线控制伺服电机和总
- IronPython-2.6.2-Src-Net20.zip
- Labview条码追踪系统JKI+ AMC结合的框架,扩展性强,适用于各种项目
- PFC单轴压缩代码,非均质模型,包括声发射,根据裂纹数截图同时输出应力和位移云图数据,拉剪裂纹等
- IronPython-2.6.2-Net20.msi
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


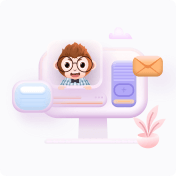
- 1
- 2
前往页