/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
/*
* ChartGui.java
*
* Created on 2012-7-24, 10:00:16
*/
package com.itjob.contact.client.gui;
import com.itjob.contact.client.Client;
import com.itjob.contact.client.ClientWorker;
import com.itjob.contact.common.IMessageService;
import com.itjob.contact.common.Message;
import com.itjob.contact.common.MessageType;
import com.itjob.contact.common.SocketMessageService;
import com.itjob.contact.common.User;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import javax.swing.DefaultListModel;
/**
*
* @author Administrator
*/
public class ChartGui extends javax.swing.JFrame {
private Client c;
private User u;
/** Creates new form ChartGui */
public ChartGui(User u, Client c) {
this.c = c;
this.u = u;
initComponents();
this.setTitle(u.getLoginName());
//必须要不断地接收消息
//构造客户端的秘书类
new ClientWorker(this).start();
}
public Client getC() {
return c;
}
public void setC(Client c) {
this.c = c;
}
/** This method is called from within the constructor to
* initialize the form.
* WARNING: Do NOT modify this code. The content of this method is
* always regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">//GEN-BEGIN:initComponents
private void initComponents() {
jScrollPane1 = new javax.swing.JScrollPane();
jList1 = new javax.swing.JList();
jScrollPane2 = new javax.swing.JScrollPane();
jTextArea1 = new javax.swing.JTextArea();
jScrollPane3 = new javax.swing.JScrollPane();
jTextArea2 = new javax.swing.JTextArea();
jButton1 = new javax.swing.JButton();
jCheckBox1 = new javax.swing.JCheckBox();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
addWindowListener(new java.awt.event.WindowAdapter() {
public void windowClosing(java.awt.event.WindowEvent evt) {
formWindowClosing(evt);
}
});
jList1.setModel(new DefaultListModel());
jList1.addMouseListener(new java.awt.event.MouseAdapter() {
public void mouseClicked(java.awt.event.MouseEvent evt) {
jList1MouseClicked(evt);
}
});
jScrollPane1.setViewportView(jList1);
jTextArea1.setColumns(20);
jTextArea1.setRows(5);
jScrollPane2.setViewportView(jTextArea1);
jTextArea2.setColumns(20);
jTextArea2.setRows(5);
jScrollPane3.setViewportView(jTextArea2);
jButton1.setText("发送");
jButton1.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButton1ActionPerformed(evt);
}
});
jCheckBox1.setText("私聊");
jCheckBox1.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jCheckBox1ActionPerformed(evt);
}
});
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jScrollPane2, javax.swing.GroupLayout.PREFERRED_SIZE, 277, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jScrollPane3, javax.swing.GroupLayout.DEFAULT_SIZE, 277, Short.MAX_VALUE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jScrollPane1, javax.swing.GroupLayout.PREFERRED_SIZE, 75, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(32, 32, 32))
.addGroup(layout.createSequentialGroup()
.addGap(132, 132, 132)
.addComponent(jButton1)
.addGap(18, 18, 18)
.addComponent(jCheckBox1, javax.swing.GroupLayout.PREFERRED_SIZE, 60, javax.swing.GroupLayout.PREFERRED_SIZE)
.addContainerGap(133, Short.MAX_VALUE))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addComponent(jScrollPane2, javax.swing.GroupLayout.PREFERRED_SIZE, 152, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, 34, Short.MAX_VALUE)
.addComponent(jScrollPane3, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE))
.addComponent(jScrollPane1, javax.swing.GroupLayout.PREFERRED_SIZE, 259, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(jButton1)
.addContainerGap())
.addGroup(layout.createSequentialGroup()
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jCheckBox1)
.addContainerGap())))
);
pack();
}// </editor-fold>//GEN-END:initComponents
private void jButton1ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton1ActionPerformed
// TODO add your handling code here:
Message msg = new Message(MessageType.MSG_TEXT, u);
List<User> msgTo = null;
//发送消息
DefaultListModel dlm = (DefaultListModel) jList1.getModel();
int[] i = jList1.getSelectedIndices();
if (i[0] == 0) {
System.out.println("chartgui publicchat");
msg = new Message(MessageType.MSG_PUBLICCHAT, u);
} else {
msgTo = new ArrayList<User>();
for (int j = 0; j < i.length; j++) {
msgTo.add((User) dlm.elementAt(i[j]));
}
/* c.sendMessage((new User(this.getTitle())),msgTo,chatMessage);
msg.setText(msg.getText()+new User(this.getTitle())+":"+chatMessage+"\n");//娑堟伅鍘嗗彶+鍙戦€佽€咃細鍙戦€佺殑娑堟伅
sendMsg.setText(" ");//灏嗘瘡娆″彂閫佹秷鎭殑闈㈡澘缃┖
*/
}
msg.setMsgSender(u);//5
// User tu=new User("2","2");
// List<User> tul=new ArrayList<User> ();
// tul.add(tu);
/// msg.setType(MessageType.MSG_TEXT);
msg.setMsgTo(msgTo);
msg.setMsgBody(jTextArea2.getText());
IMessageService proxy = new SocketMessageService(c.getS());
proxy.sendMessage(msg);
//接收注册结果
}//GEN-LAST:event_jButton1ActionPerformed
private void jCheckBox1ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jCheckBox1ActionPerformed
// TODO add your handling code here:
}//GEN-LAST:event_jCheckBox1ActionPerformed
private void jList1MouseClicked(java.awt.event.MouseEvent evt) {//GEN-FIRST:event_jList1MouseClicked
// TODO add your handling code here:
}//GEN-LAST:event_jList1MouseClicked
private void formWindowClosing(java.awt.event.WindowEvent
没有合适的资源?快使用搜索试试~ 我知道了~
NETBEANS下的网络QQ聊天系统
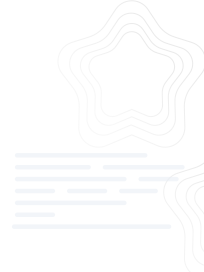
共75个文件
class:26个
java:16个
xml:12个


温馨提示
NETBEANS下的网络QQ聊天系统,精研实用,值得收藏!
资源推荐
资源详情
资源评论
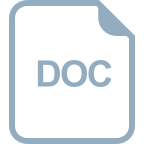
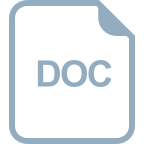
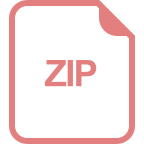
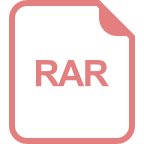
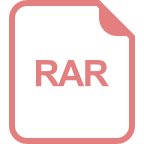
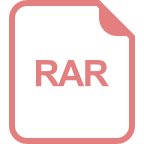
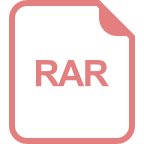
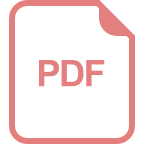
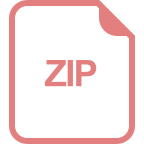
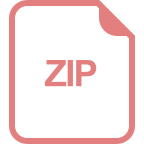
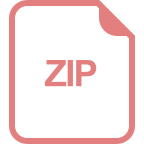
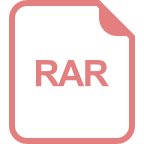
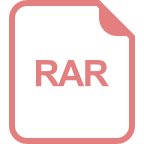
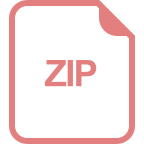
收起资源包目录



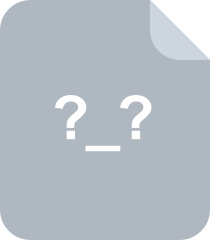
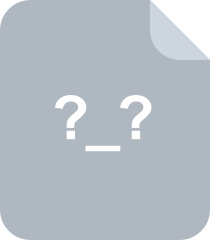


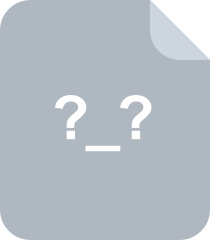




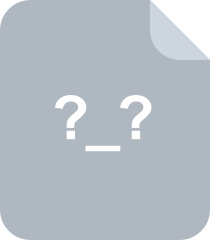
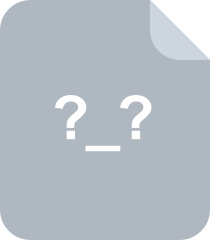



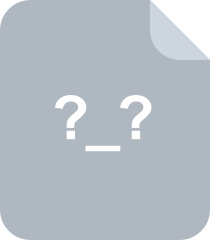




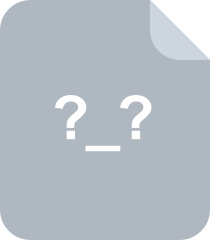
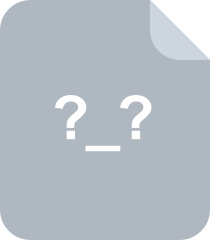
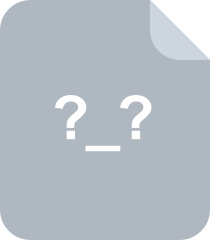
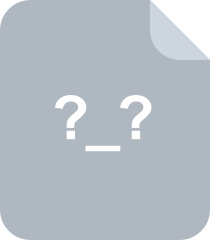

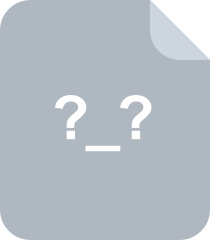
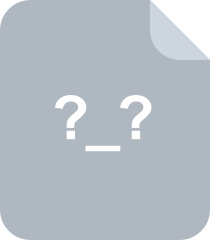

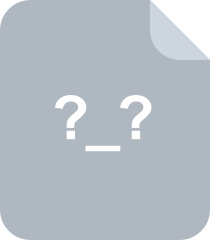
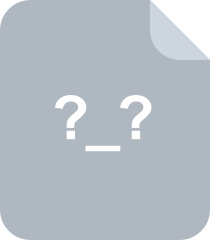
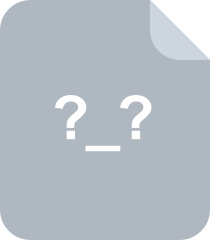
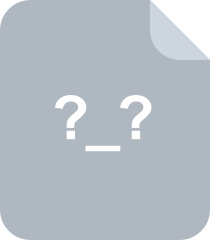
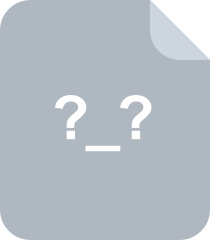

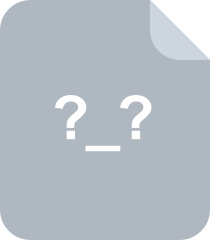


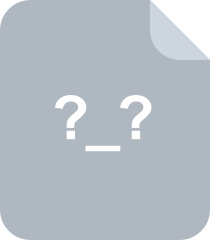





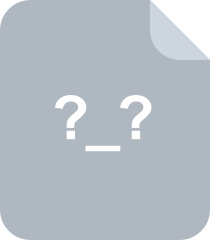
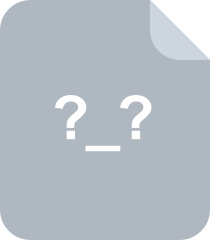
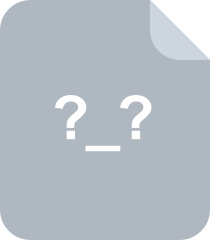
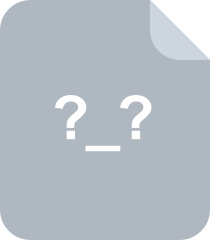
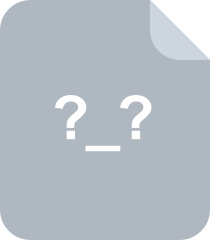
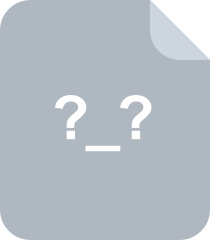
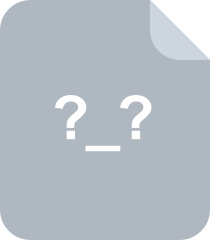
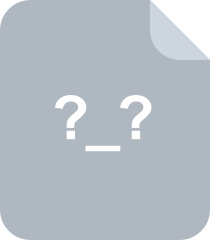



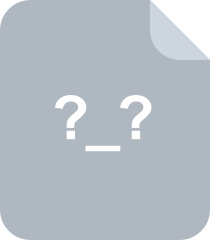




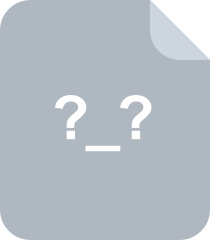

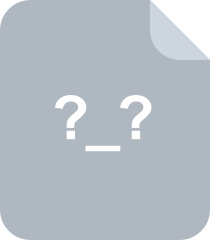
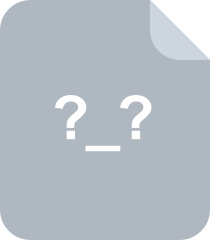
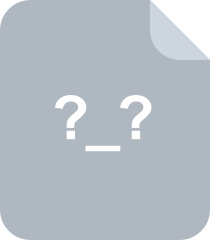
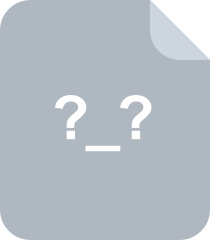
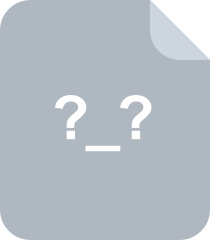
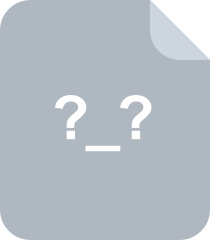
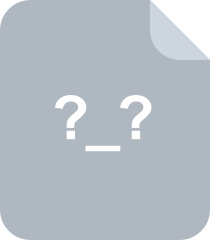
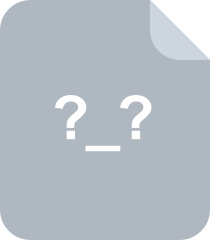
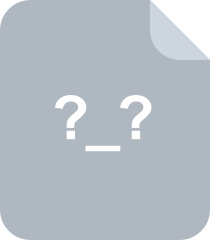
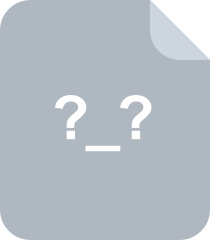
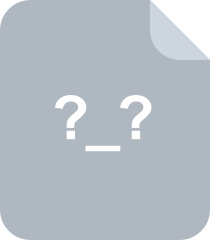
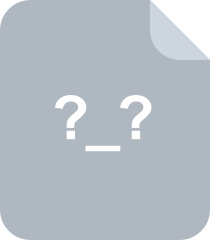
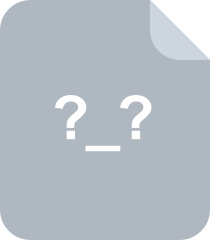
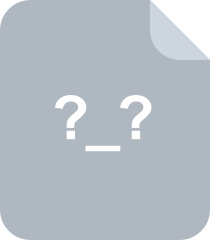
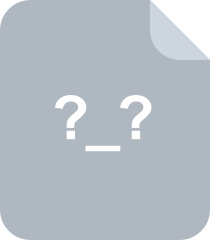
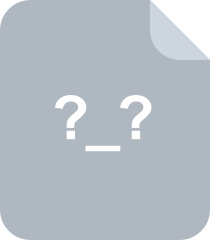

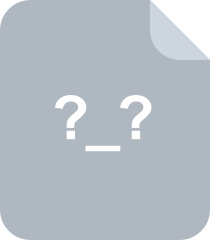
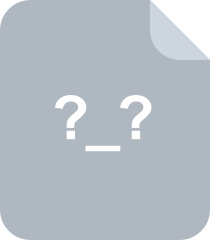

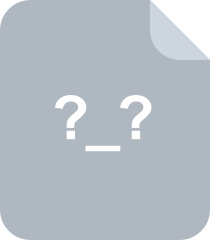
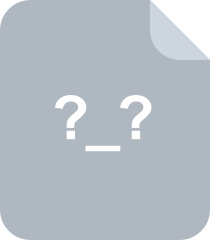
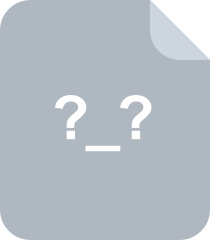
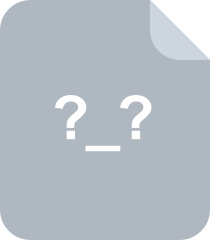
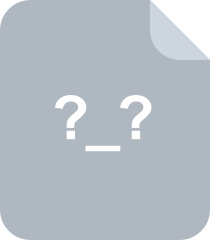

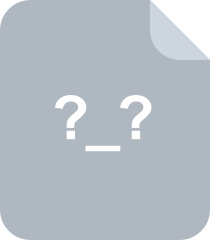





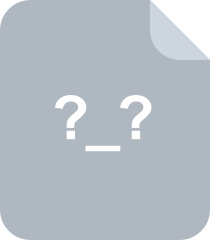
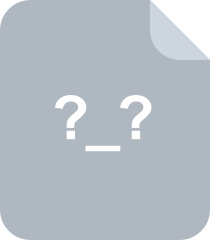
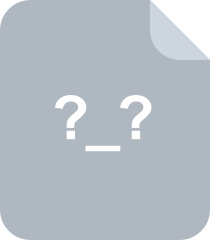
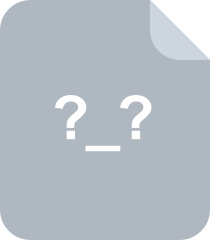
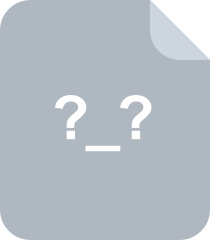
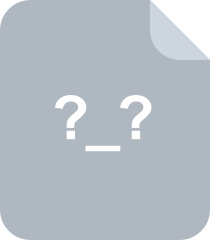
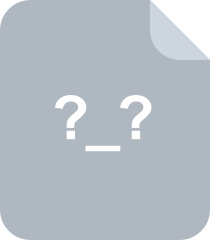






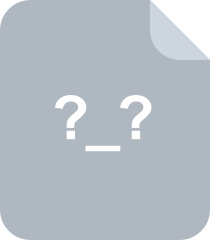
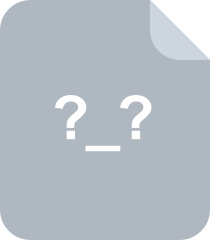
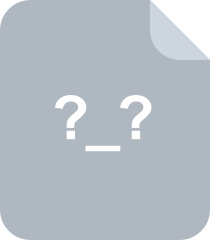
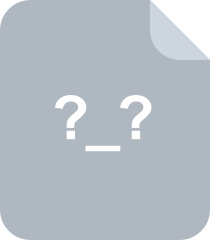
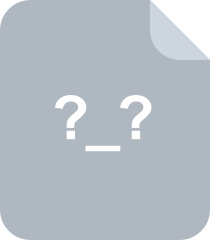
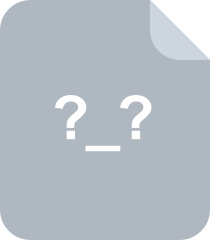
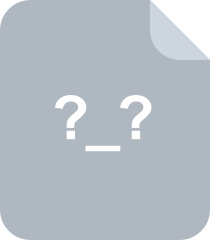
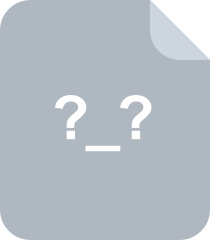
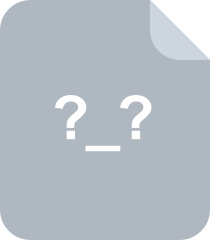

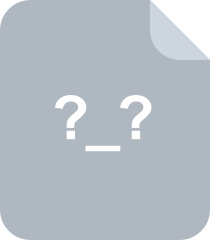
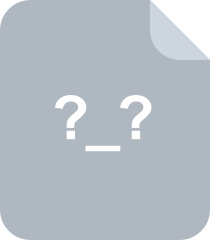

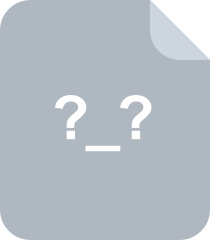
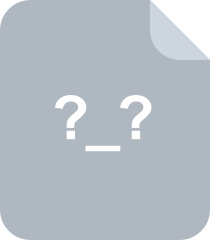
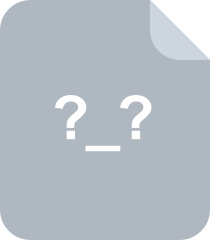
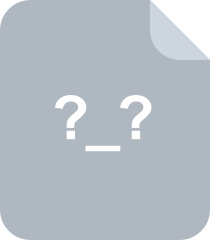
共 75 条
- 1
资源评论
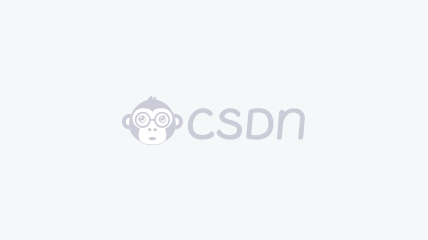
- tianmiaoDaisy2013-11-16刚刚学习netbeans,对学习很有帮助
- NJNBJL2014-01-13还可以,有需要更改的地方
- RTAAAAAQBHJE2018-01-11对初学者还可以
- amyitishao2014-04-06挺好的,但还是有需要更改的地方

buddha17
- 粉丝: 37
- 资源: 56
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

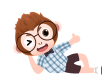
最新资源
- 内部讲师评选方案.doc
- 培训师职业训练教材《教学媒体应用技能训练》.doc
- 培训之网络写作指南.doc
- 培训师职业技能训练教材《良好心理素质养成与心理辅导技能训》.doc
- HR师认证复习资料-人力资源规划.ppt
- 关于如何对待工作的一些建议(ppt 45页).ppt
- 培训导师.ppt
- 管理领导力与激励.ppt
- 培训导师的职责与任务.ppt
- 培训艺术与技术(教材).ppt
- 企业内部培训师培训分享资料.ppt
- 培训与开发(ppt 157页).ppt
- 人力资源培训教材-人员招募与甄选(PPT 24页).ppt
- 巧妙处理听众的问题.ppt
- 企业培训讲师形象.ppt
- 人力资源培训教材-如何设计发展空间(PPT 26页).ppt
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


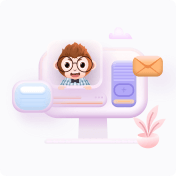
安全验证
文档复制为VIP权益,开通VIP直接复制
