package src.Kyodai;
import javax.swing.*;
import java.awt.*;
import java.net.*;
import java.util.*;
import java.awt.event.*;
import javax.swing.border.*;
import src.Kyodai.*;
import src.Kyodai.map.*;
import src.Kyodai.map.Map;
import src.Kyodai.topbar.*;
/**
* <p>Title: LianLianKan</p>
* <p>Description: 连连看</p>
* <p>Copyright: Copyright (c) 2004</p>
* <p>Company: www.wuhantech.com</p>
* @author ZhangJian
* @version 1.0
*/
public class Kyodai extends JFrame implements ActionListener {
public static Color DarkColor = new Color(55, 77, 118); //暗色
public static Color LightColor = new Color(111, 146, 212); //亮色
public static ImageIcon[] BlocksIcon = new ImageIcon[39]; //游戏中方块的图标
public static ImageIcon GuideIcon; //连线的图标
public static Border unSelected = BorderFactory.createLineBorder(DarkColor, 1); //未选中时的边框
public static Border Selected = BorderFactory.createLineBorder(Color.white, 1); //选中后的边框
public static Border Hint = BorderFactory.createLineBorder(Color.green, 1); //提示的边框
Dimension faceSize = new Dimension(780, 500);
Image icon;
private int counter = 0;
JPanel toolBar = new JPanel(); //工具栏
JPanel actionPanel = new JPanel(); //用户操作栏
JPanel contentPanel = new JPanel(); //容器
JPanel statusPanel = new JPanel(); //状态栏
Border emptyBorder = BorderFactory.createEmptyBorder(); //未选中时的边框
JButton startButton = new JButton(); //"开始"
JButton refreshButton = new JButton(); //"刷新"
JButton hintButton = new JButton(); //"提示"
JButton bombButton = new JButton(); //"炸弹"
JButton demoButton = new JButton(); //"演示"
JButton setupButton = new JButton(); //设置
JButton helpButton = new JButton(); //帮助
JButton aboutButton = new JButton(); //关于
JButton goTop10 = new JButton("Go top 10");
HelpDialog helpDialog; //帮助对话框
AboutDialog aboutDialog; //关于对话框
//SetupDialog setupDialog; //设置对话框
public static JTextField statusField = new JTextField(
"Weclome to Kyodai 1.0 alpha");
ImageIcon imgStart, imgHint, imgRefresh, imgBomb, imgDemo;
ImageIcon imgSetup, imgHelp, imgAbout;
JButton[] dots = new JButton[Setting.ROW * Setting.COLUMN];
Setting setting = new Setting();
MapUI ui;
Map map;
ClockAnimate clock = new ClockAnimate(); //时钟
ScoreAnimate score = new ScoreAnimate(); //分数
AnimateDelete animateDelete = new AnimateDelete(dots);
Music music = new Music();
public Kyodai() {
this.setDefaultCloseOperation(this.EXIT_ON_CLOSE);
initResource();
map = new Map();
ui = new MapUI(map,dots);
initUI();
ui.setScore(score);
ui.setClock(clock);
ui.setTop10Button(goTop10);
animateDelete.setSpeed(setting.Animate);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.pack();
this.setSize(faceSize);
Dimension screenSize = Toolkit.getDefaultToolkit().getScreenSize();
this.setLocation( (int) (screenSize.width - faceSize.getWidth()) / 2,
(int) (screenSize.height - faceSize.getHeight()) / 2);
this.setResizable(false);
this.setTitle("Kyodai 1.0 alpha"); //设置标题
this.setIconImage(icon); //设置程序图标
//设置动画光标
URLClassLoader urlLoader = (URLClassLoader)this.getClass().getClassLoader();
URL url = urlLoader.findResource("images/cursor.gif");
Image animateImage = new ImageIcon(url).getImage();
Cursor cursor = Toolkit.getDefaultToolkit().createCustomCursor(
animateImage, new Point(0, 0), "cursor");
this.setCursor(cursor);
this.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent we) {
setting.save();
}
});
if (setting.Music == 1) {
music.play();
}
}
/**
* 初始化系统所需要的资源
*/
public void initResource() {
URLClassLoader urlLoader = (URLClassLoader)this.getClass().getClassLoader();
URL url;
//程序图标
icon = getImage("images/kyodai16.gif");
for (int i = 0; i < BlocksIcon.length; i++) {
BlocksIcon[i] = new ImageIcon(getImage("images/" + (i + 1) + ".gif"));
}
imgStart = new ImageIcon(getImage("images/start.gif"));
imgRefresh = new ImageIcon(getImage("images/refresh.gif"));
imgHint = new ImageIcon(getImage("images/hint.gif"));
imgBomb = new ImageIcon(getImage("images/bomb.gif"));
imgDemo = new ImageIcon(getImage("images/demo.gif"));
imgSetup = new ImageIcon(getImage("images/setup.gif"));
imgHelp = new ImageIcon(getImage("images/help.gif"));
imgAbout = new ImageIcon(getImage("images/about.gif"));
GuideIcon = new ImageIcon(getImage("images/dots.gif"));
//初始化方块
for (int i = 0; i < dots.length; i++) {
dots[i] = new JButton();
dots[i].setActionCommand("" + i);
dots[i].setBorder(unSelected);
dots[i].setBackground(DarkColor);
}
//读取用户设置
setting.load();
//初始化对话框
helpDialog = new HelpDialog(this); //帮助对话框
aboutDialog = new AboutDialog(this); //关于对话框
}
/**
* 初始化用户界面
*/
public void initUI() {
//界面整体布局
Border border = BorderFactory.createBevelBorder(BevelBorder.LOWERED,
new Color(45, 92, 162),
new Color(43, 66, 97),
new Color(45, 92, 162),
new Color(84, 123, 200));
BorderLayout borderLayout = new BorderLayout();
toolBar.setBackground(DarkColor);
toolBar.setBorder(border);
toolBar.setPreferredSize(new Dimension(780, 48));
toolBar.setMinimumSize(new Dimension(780, 48));
toolBar.setLayout(new FlowLayout(FlowLayout.LEFT));
actionPanel.setBackground(LightColor);
actionPanel.setBorder(border);
actionPanel.setPreferredSize(new Dimension(160, 380));
actionPanel.setMinimumSize(new Dimension(160, 380));
contentPanel.setBackground(DarkColor);
contentPanel.setBorder(border);
contentPanel.setPreferredSize(new Dimension(620, 380));
contentPanel.setMinimumSize(new Dimension(620, 380));
statusPanel.setBackground(DarkColor);
statusPanel.setBorder(border);
statusPanel.setPreferredSize(new Dimension(620, 24));
statusPanel.setMinimumSize(new Dimension(620, 24));
statusPanel.setLayout(new BorderLayout());
this.getContentPane().setLayout(borderLayout);
this.getContentPane().add(toolBar, BorderLayout.NORTH);
this.getContentPane().add(actionPanel, BorderLayout.EAST);
this.getContentPane().add(contentPanel, BorderLayout.CENTER);
this.getContentPane().add(statusPanel, BorderLayout.SOUTH);
//加入地图
contentPanel.add(ui);
//加入计分
actionPanel.add(score);
//加入开始按钮
actionPanel.add(startButton);
startButton.setBorder(emptyBorder);
startButton.setIcon(imgStart);
startButton.addActionListener(this);
//加入刷新按钮
actionPanel.add(refreshButton);
refreshButton.setBorder(emptyBorder);
refreshButton.setIcon(imgRefresh);
refreshButton.addActionListener(this);
//加入提示按钮
actionPanel.add(hintButton);
hintButton.setBorder(emptyBorder);
hintButton.setIcon(imgHint);
hintButton.addActionListener(this);
//加入炸弹按钮
actionPanel.add(bombButton);
bombButton.setBorder(emptyBorder);
bombButton.setIcon(imgBomb);
bombButton.addActionListener(this);
//加入自动演示
actionPanel.add(demoButton);
demoButton.setBorder(emptyBorder);
demoButton.setIcon(imgDemo);
demoButton.addActionListener(this);
//加入设置
toolBar.add(setupButton);
setupButton.setBorder(emptyBorder);
setupButton.setIcon(imgSetup);
setupButton.addActionListener(this);
//加入帮助
toolBar.add(helpButton);
helpButton.setBorder(emptyBorder);
helpButton.setIcon(imgHelp);
helpButton.addActionListe
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
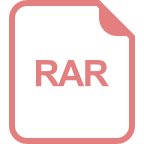
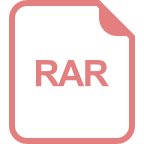
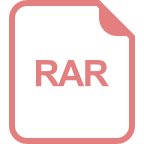
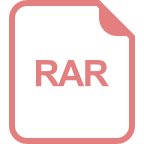
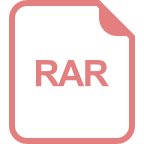
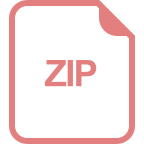
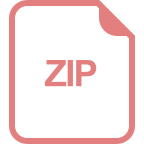
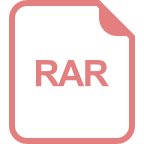
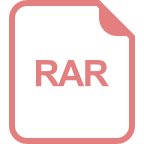
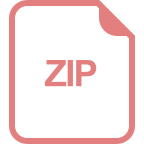
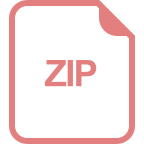
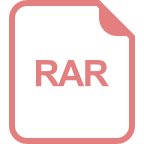
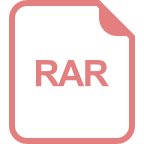
收起资源包目录

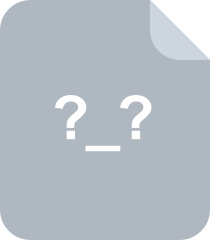
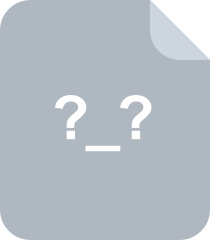
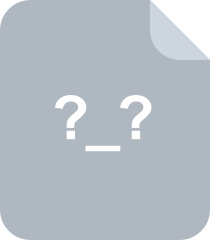
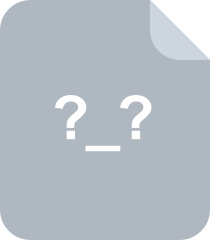
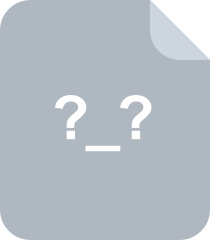
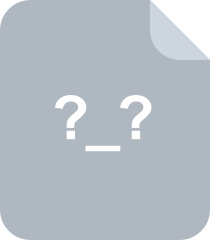
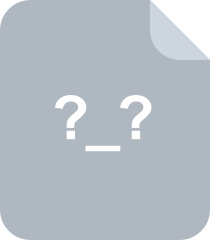
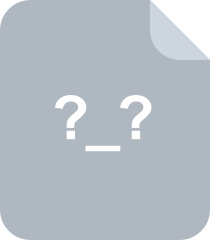
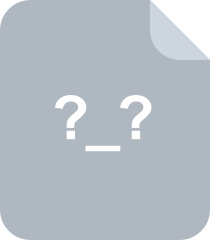
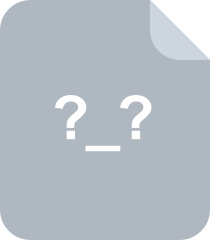
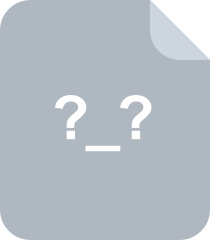
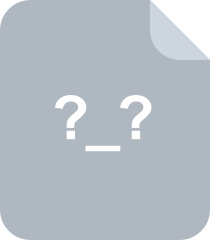
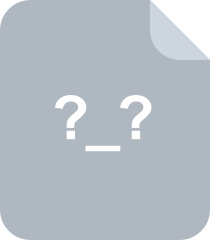
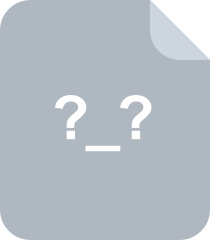
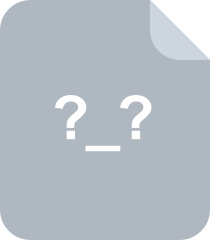
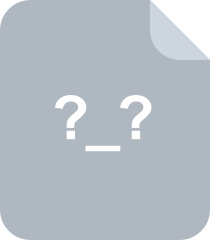
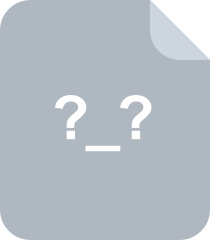
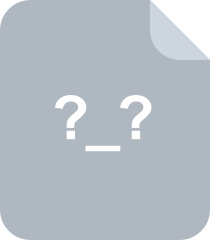
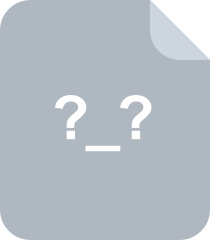
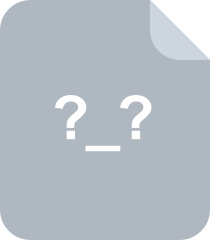
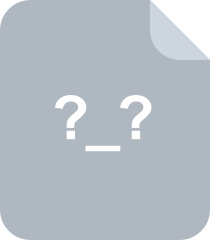
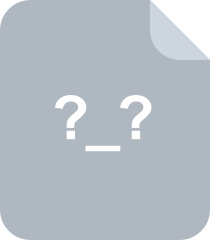
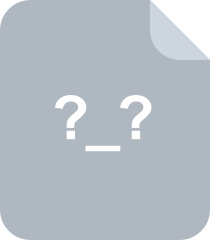
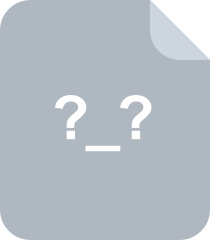
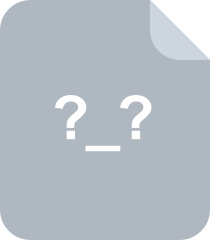
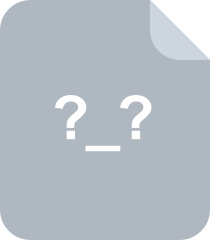
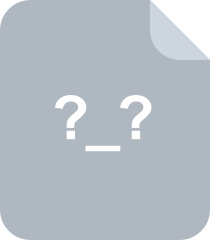
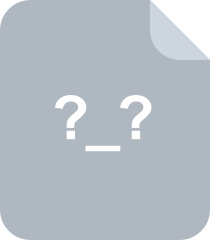
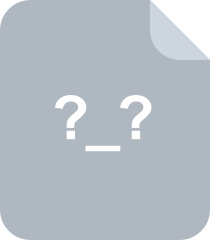
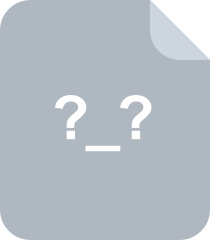
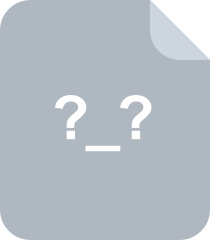
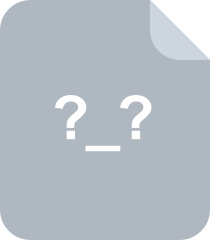
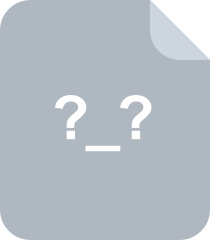
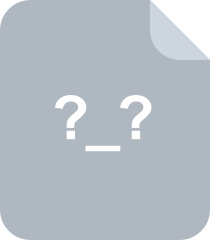
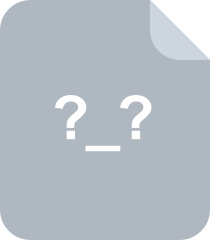
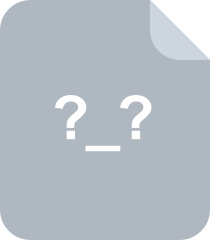
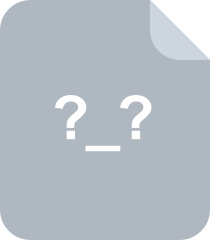
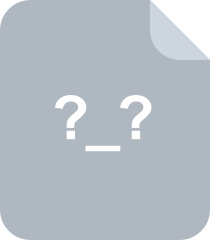
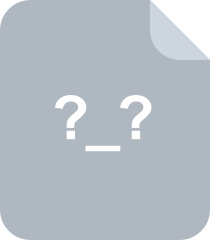
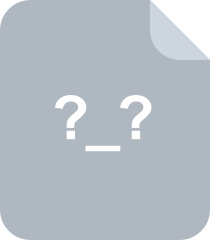
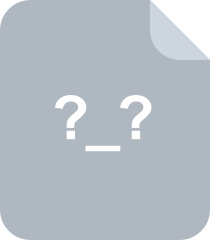
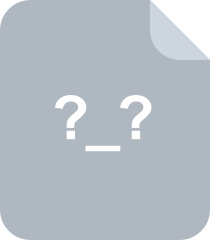
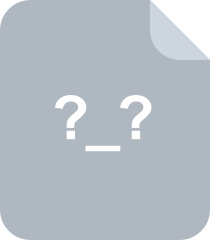
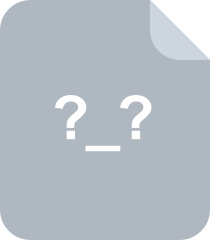
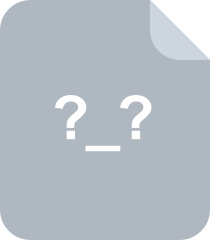
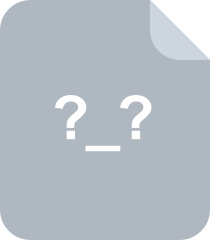
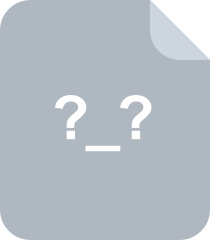
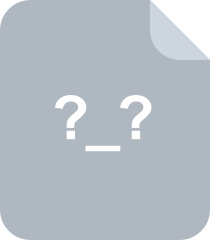
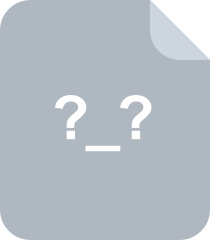
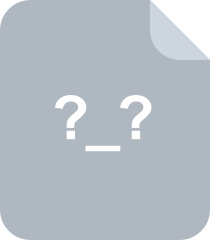
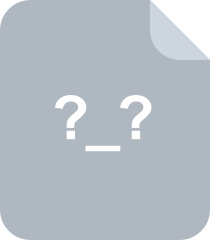
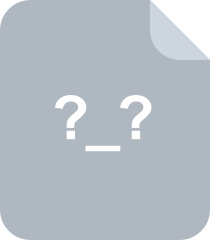
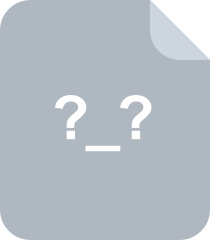
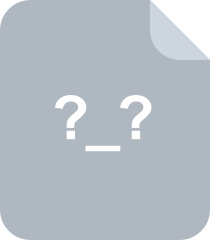
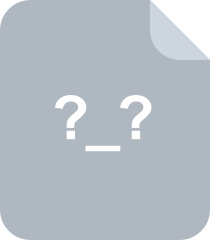
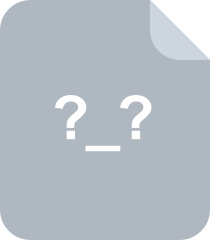
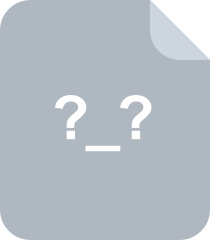
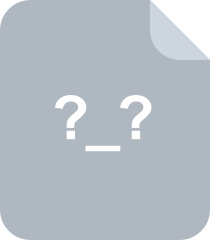
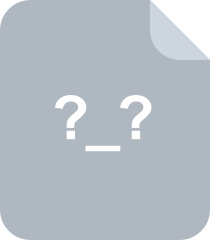
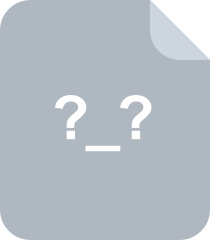
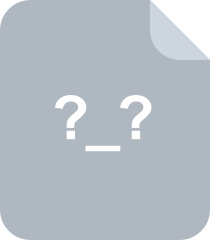
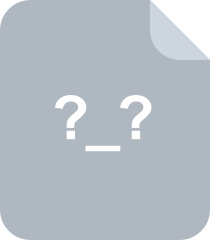
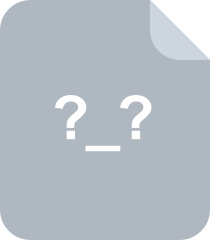
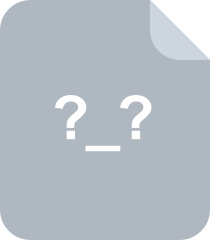
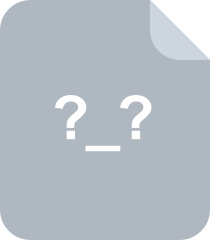
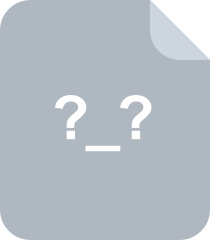
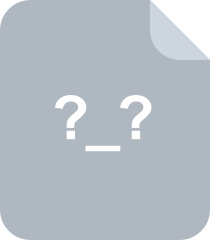
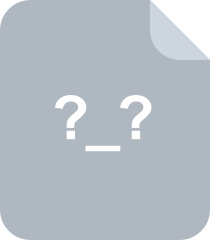
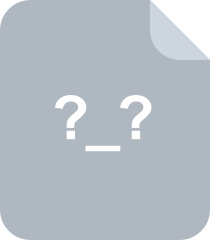
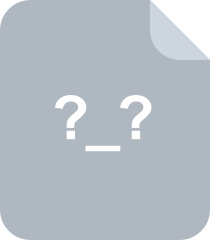
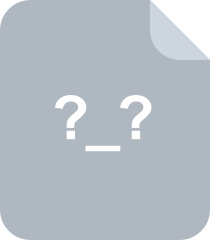
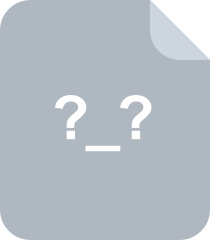
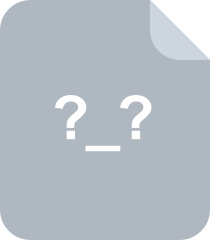
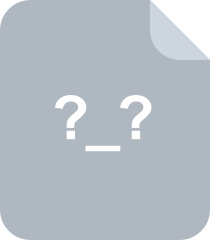
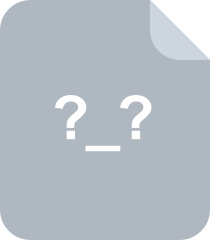
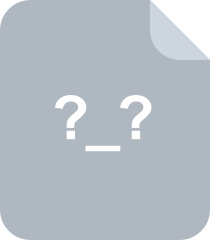
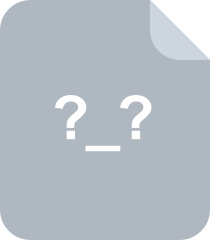
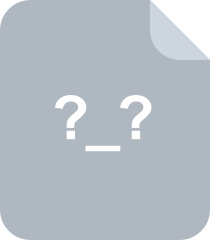
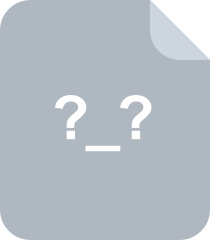
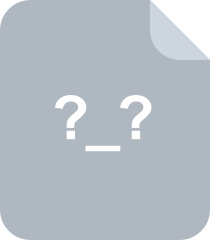
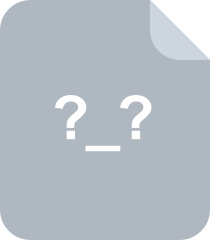
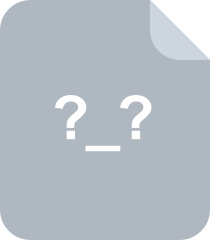
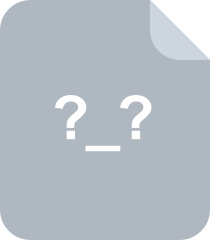
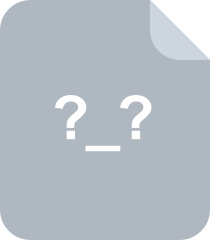
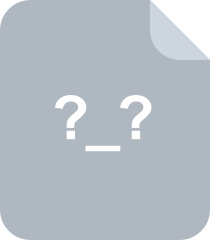
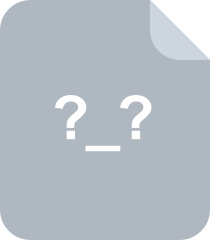
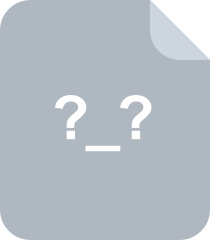
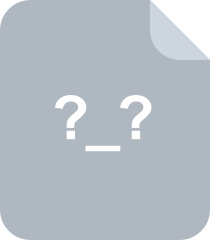
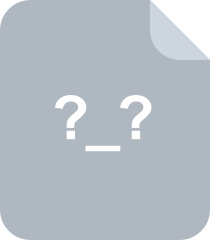
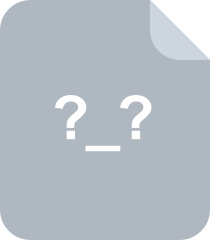
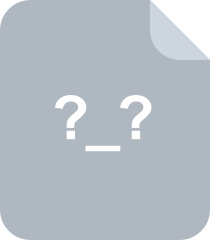
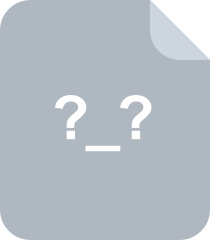
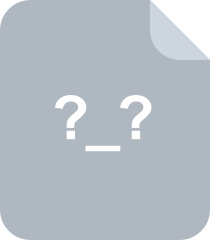
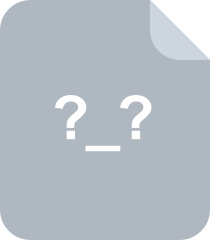
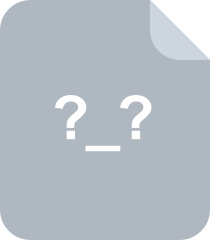
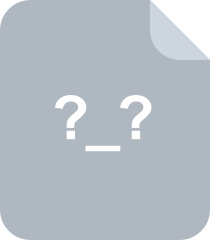
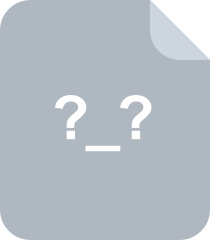
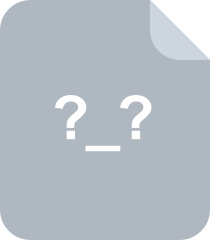
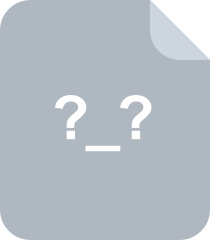
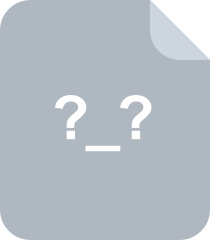
共 307 条
- 1
- 2
- 3
- 4
资源评论
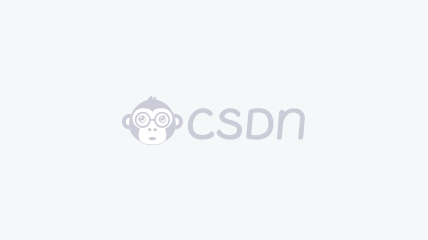

boyluke
- 粉丝: 3
- 资源: 12
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

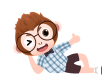
最新资源
- 本地安装GSVA,有很多选择,我选了相对最新的
- yolo算法-动物类别数据集-21613张图像带标签-人-奶牛-鹰-大象-汽车-猪-水牛-熊-鹿-雨伞-狗-老虎-浣熊-狼.zip
- Go语言实现高质量代理池构建与部署
- yolo算法-多类别动物数据集-8893张图像带标签-猴子-奶牛-大象-水牛-美洲虎-熊-鹿-马-狗-老虎-鸟-狮子-猎豹-山羊.zip
- Video_1732514072178.mp4
- yolo算法-手套-无手套-人数据集-14163张图像带标签-手套-无手套.zip
- WordPress主题 多本小说阅读模板
- yolo算法-道路损伤检测数据集-17145张图像带标签-纵向的-坑洼.zip
- yolo算法-猴子-大象-猪动物数据集-6229张图像带标签-猴子-大象-猪-牛-鹿-熊-棕熊-老虎.zip
- yolo算法-动物数据集-8944张图像带标签-自行车-背景-大象-豹-牛-熊-鹿-马-摩托车-猎豹-福克斯-猴子-美洲虎-太阳能电池板-老虎-犀牛-狮子-山羊-人-狗-天鱼-鸟.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


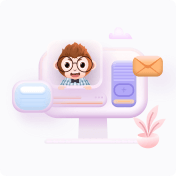
安全验证
文档复制为VIP权益,开通VIP直接复制
