**J paginate 分页插件优化使用详解** 在Web开发中,分页是常见的功能,用于处理大量数据并提高用户体验。J paginate 是一个流行的JavaScript分页插件,它可以帮助开发者轻松实现网页上的数据分页。本教程将深入探讨如何优化使用J paginate 插件,并结合MySQL数据库,提供一个实际的DEMO示例。 了解J paginate 的基本用法至关重要。J paginate 提供了丰富的样式和自定义选项,可以通过简单的HTML和JavaScript代码实现分页界面。在HTML中,你需要创建一个容器元素来承载分页链接,然后在JavaScript中初始化J paginate 插件。例如: ```html <div id="pagination"></div> <script> $(document).ready(function() { $('#pagination').paginate({ count: 总页数, start: 当前页数, display: 显示页数, text: '页', border: true, buttons: true, images: false, .onclick: function(page) { // 在这里处理页面跳转逻辑,比如重新加载数据 } }); }); </script> ``` 接下来,我们将讨论如何与MySQL数据库配合使用。在描述中提到了`mysql数据sql文件在META-INF下`,这意味着项目包含了一个SQL文件,可能包含了创建表、插入数据等操作。通常,你可以使用这些SQL语句在本地或者服务器上创建一个测试数据库,以便于演示分页功能。例如,你可能有一个`create_table.sql`和`insert_data.sql`文件,分别用于创建表结构和填充数据。 为了实现分页,你需要执行SQL查询,获取当前页的数据。在MySQL中,可以使用`LIMIT`和`OFFSET`关键字进行分页。假设每页显示10条记录,当前页为3,查询语句如下: ```sql SELECT * FROM table_name LIMIT 10 OFFSET (3 - 1) * 10; ``` 这段SQL将获取第21到30条记录(页数从0开始计数)。在你的DEMO中,`pagedemo`可能是一个包含此类查询逻辑的文件,如`index.php`或`app.js`,它会根据用户在J paginate 上的选择动态更新查询条件。 在J paginate 的`onclick`回调函数中,你需要处理页面切换事件,更新查询参数并重新加载数据。这通常涉及向后端发送AJAX请求,获取新页的数据,并更新页面上的内容。例如,使用jQuery的`$.ajax`方法: ```javascript onclick: function(page) { var url = 'your_server_script.php?page=' + page; $.ajax({ url: url, type: 'GET', success: function(data) { // 更新页面内容,如表格或列表 $('#dataContainer').html(data); }, error: function(jqXHR, textStatus, errorThrown) { // 处理错误情况 } }); } ``` 在这个DEMO中,`your_server_script.php`将处理请求,执行相应的SQL查询,并返回当前页的数据。然后前端使用这些数据更新`#dataContainer`元素,展示新页面的内容。 总结来说,J paginate 分页插件通过与MySQL数据库的配合,可以有效地处理大量数据的显示。通过理解其基本用法,结合适当的SQL查询和前端逻辑,你可以构建出高效、用户友好的分页系统。在实际项目中,还要考虑性能优化,如缓存、预加载等策略,以提升用户体验。
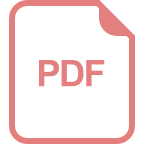
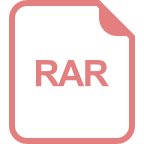
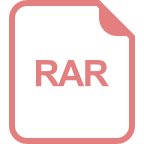
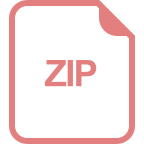
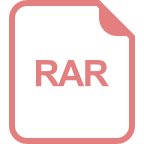
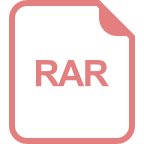
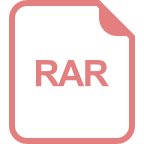
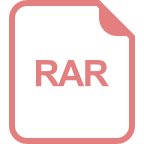
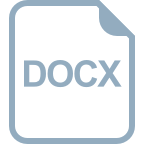
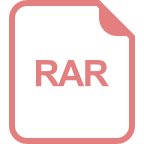
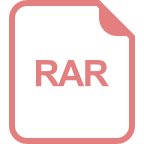
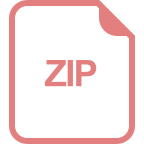
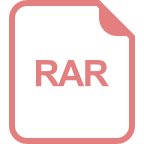
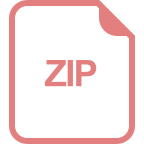
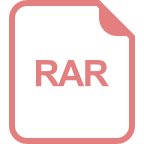
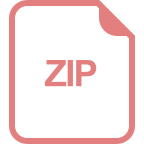
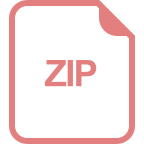
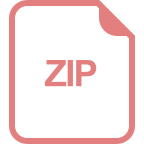
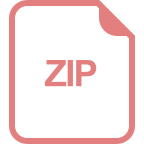
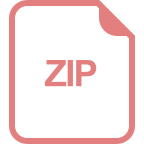
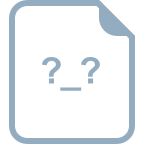
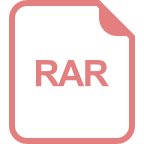
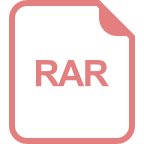
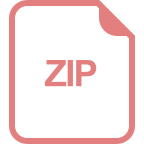

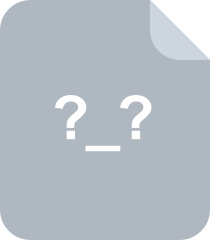
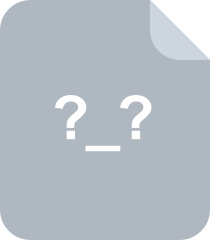
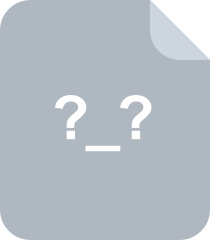
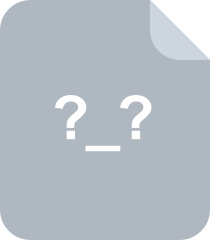
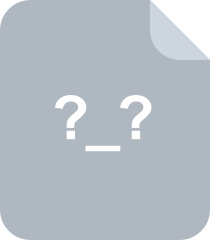
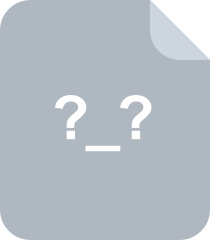
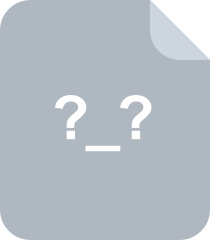
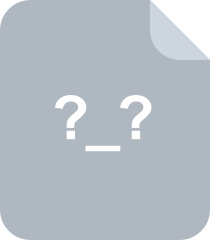
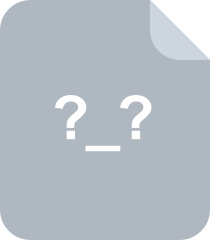
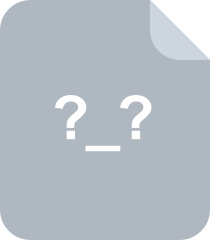
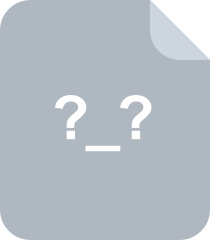
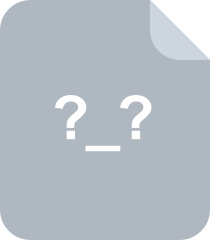
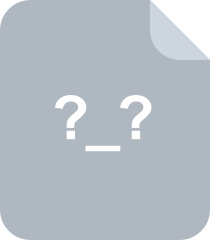
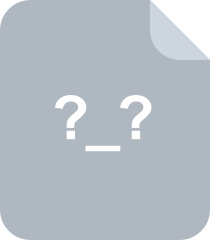
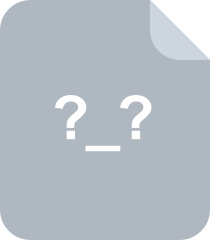
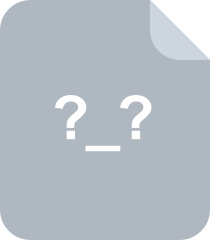
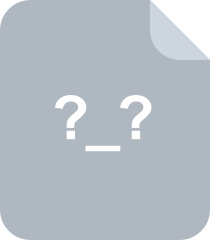
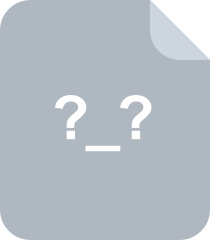
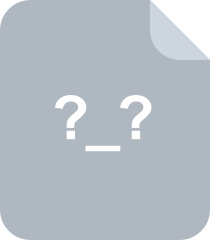
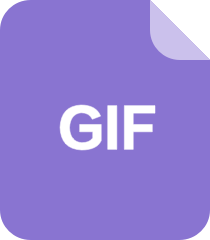
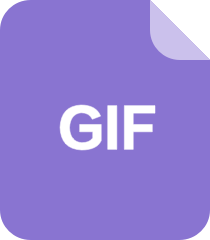
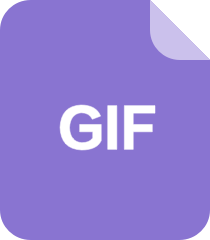
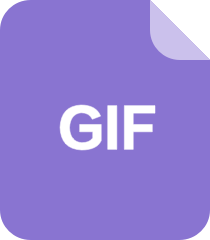
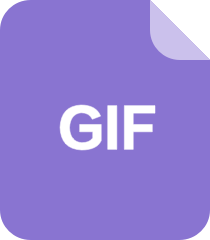
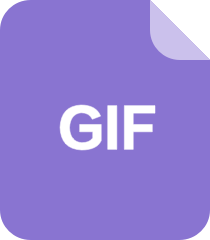
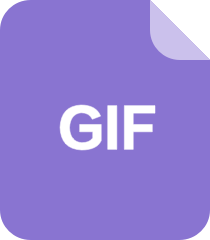
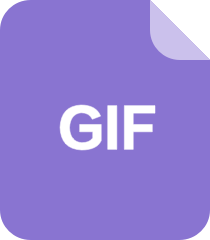
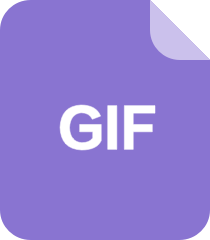
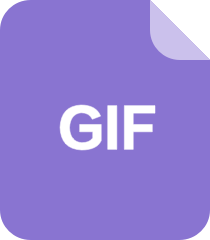
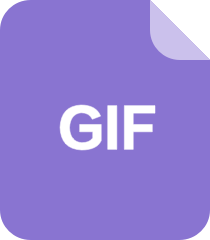
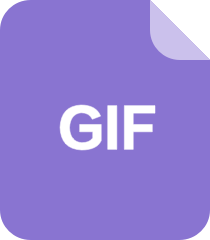
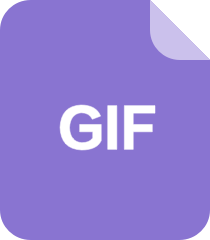
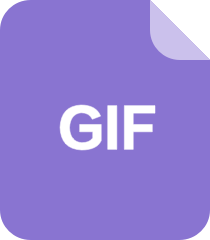
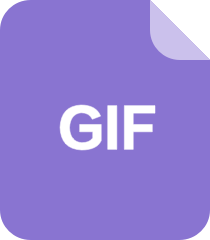
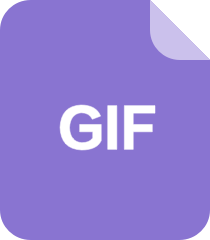
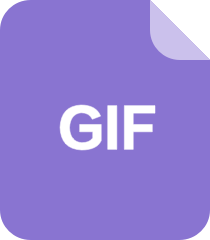
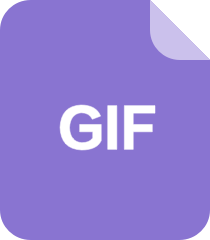
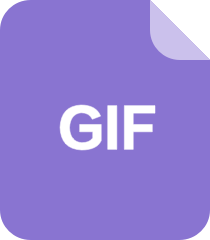
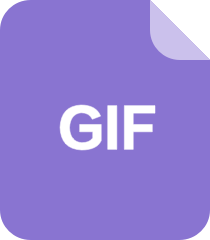
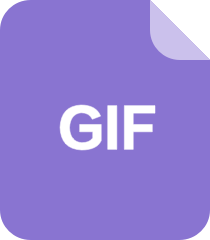
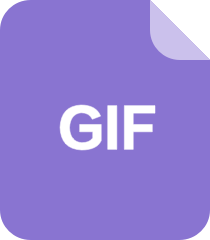
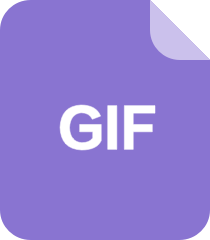
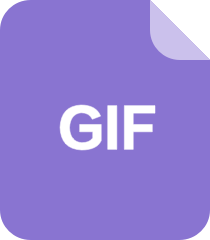
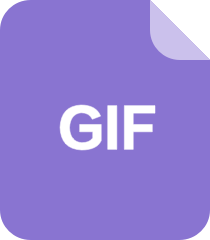
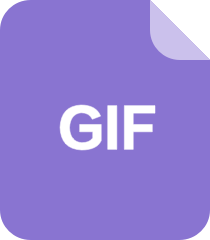
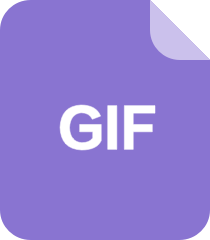
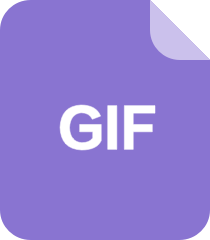
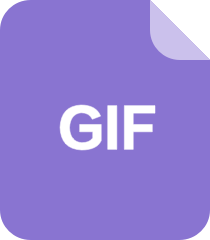
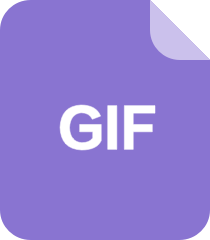
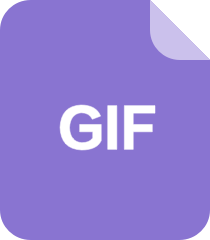
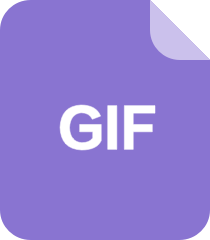
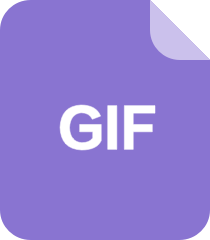
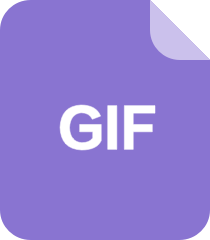
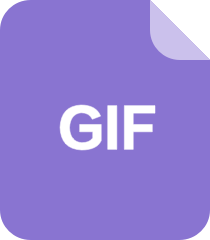
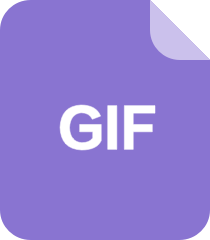
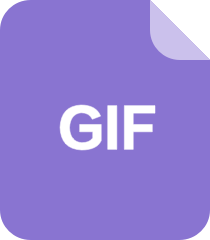
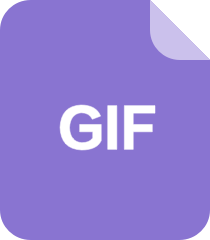
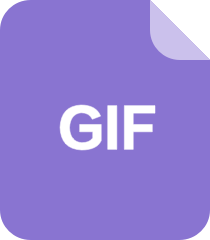
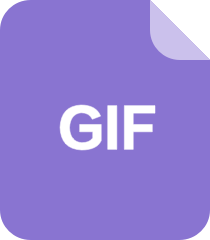
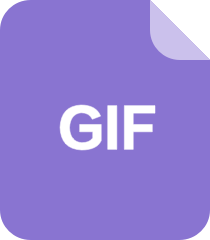
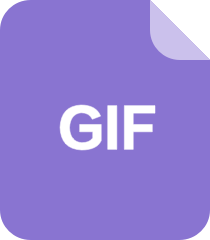
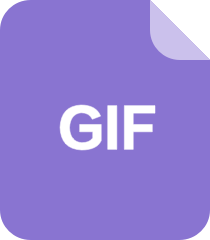
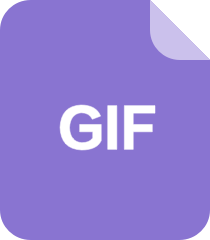
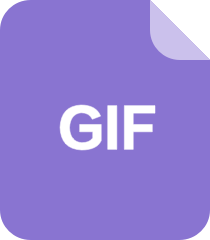
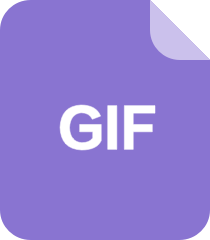
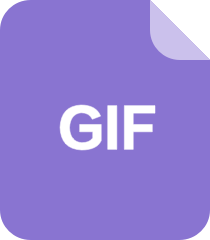
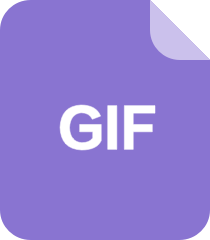
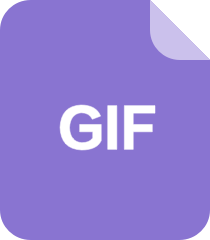
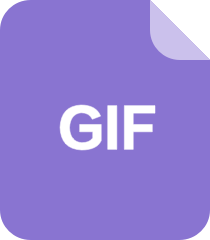
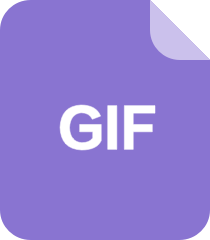
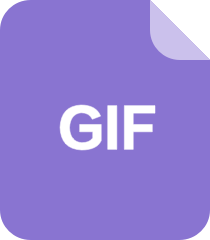
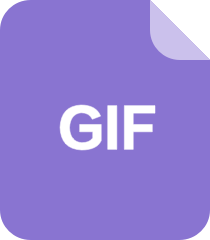
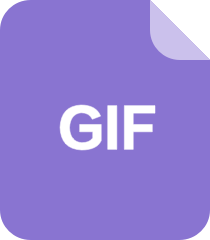
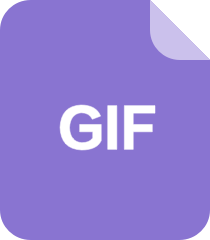
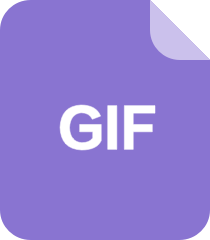
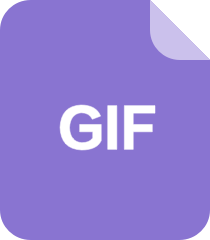
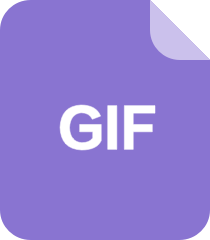
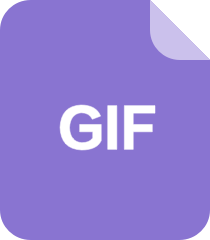
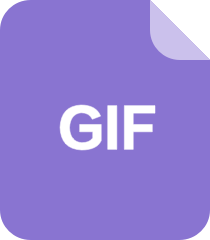
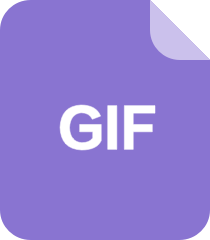
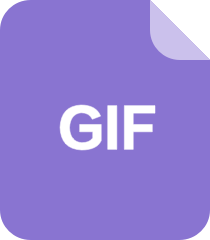
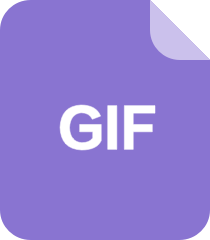
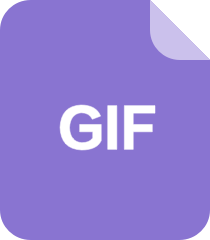
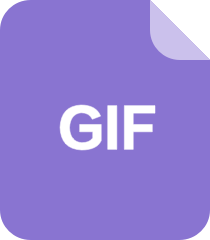
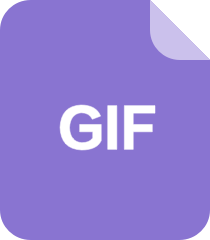
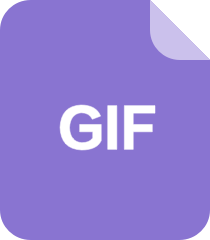
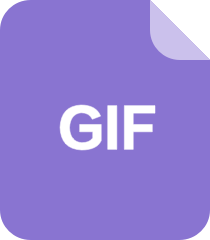
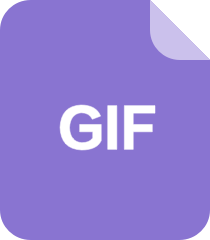
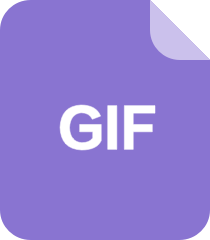
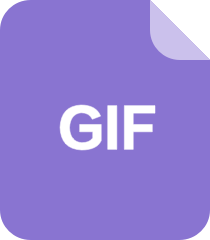
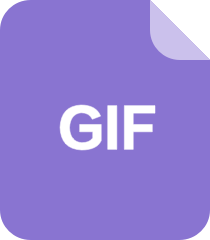
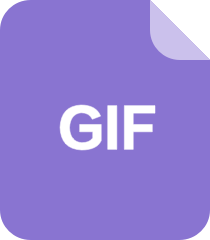
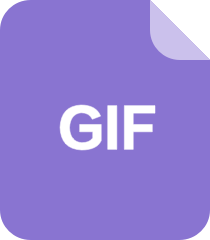
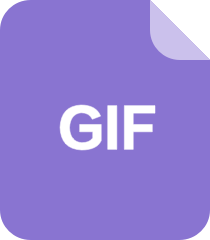
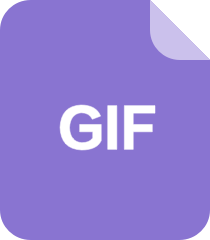
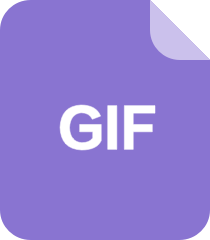
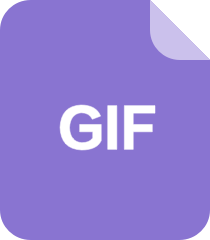
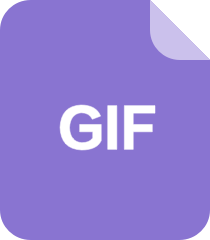
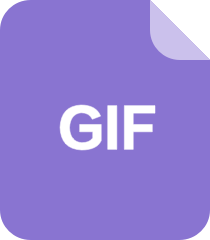
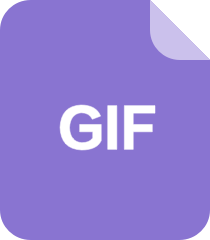
- 1
- 2
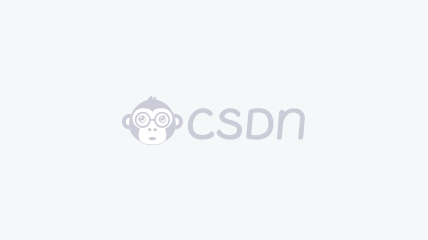
- zzpde20122013-07-17很好的一个分页控件
- zhanliang12172015-07-09非常好,虽然我还是初级水平,用插件还是要多学习
- 腊树丫2014-11-17非常好,集成到项目中很漂亮
- 盖世飞翔2013-09-04不错,很值得学习的分页控件!

- 粉丝: 1w+
- 资源: 113
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

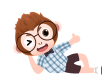
最新资源
- 纯真IP库,用于ip查询地址使用的数据库文件
- 基于javaweb的动漫网站管理系统毕业设计论文.doc
- 废物垃圾检测28-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 探索CSDN博客数据:使用Python爬虫技术
- 基于tensorflow和cnn做的图像识别,对四种花卉进行了分类项目源代码+使用说明,可识别:玫瑰花、郁金香、蒲公英、向日葵
- 基于Java的电影订票网站的设计与开发毕业设计论文.doc
- ODrive 固件 0.5.6
- 停电自动关机程序.EXE
- RabbitMQ 的7种工作模式
- 基于java的大学生二手书在线买卖系统论文.doc

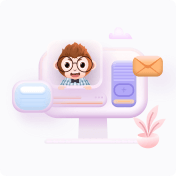
