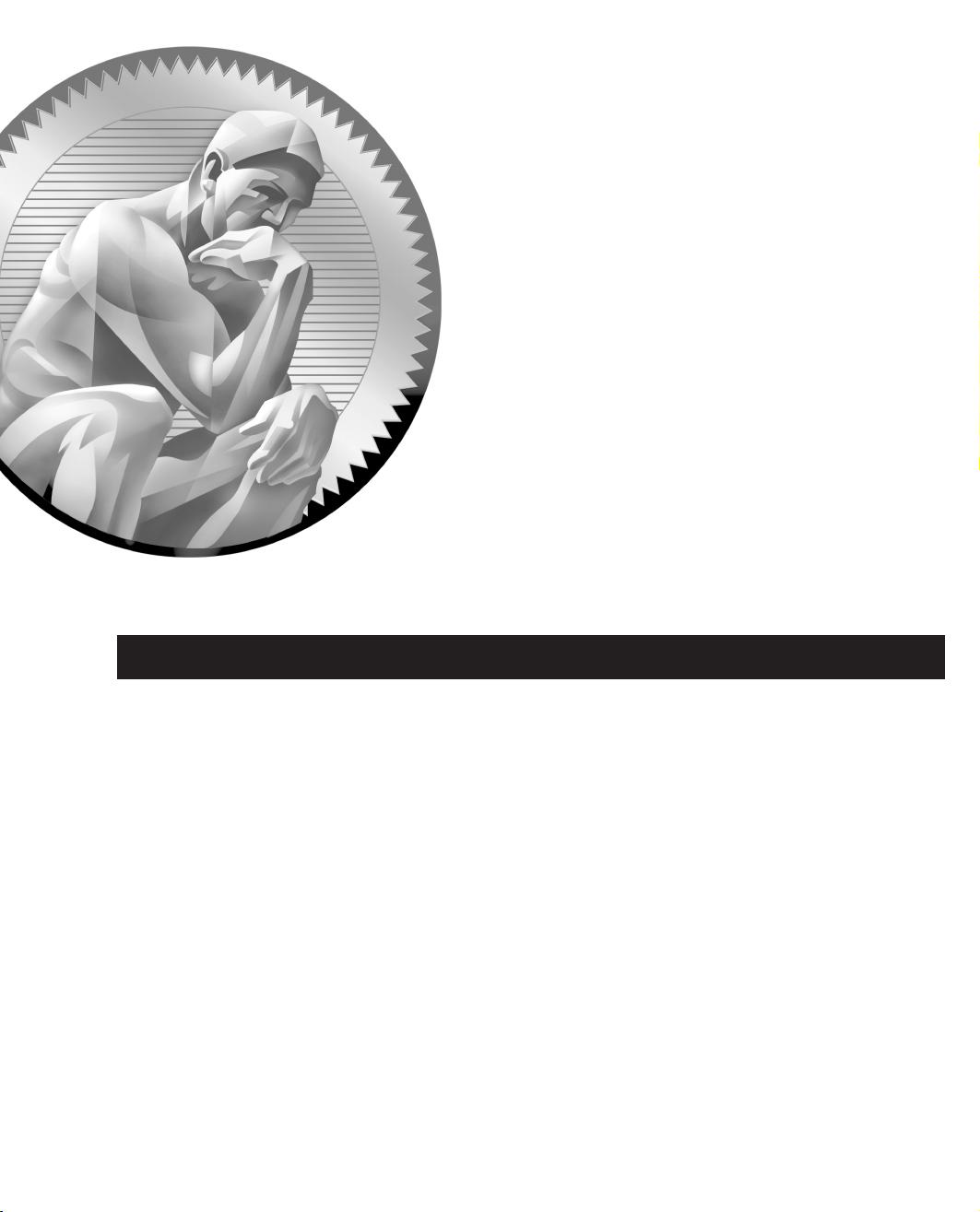
CertPrs8/Java 6 Cert. StudyGuide/Sierra-Bates/159106-0/Chapter 1
Blind Folio 1
1
Declarations and
Access Control
CERTIFICATION OBJECTIVES
l Declare Classes & Interfaces
l Develop Interfaces &
Abstract Classes
l Use Primitives, Arrays, Enums, &
Legal Identifiers
l Use Static Methods, JavaBeans
Naming, & Var-Args
3 Two-Minute Drill
Q&A Self Test
ch01.indd 1 5/21/08 12:58:18 PM
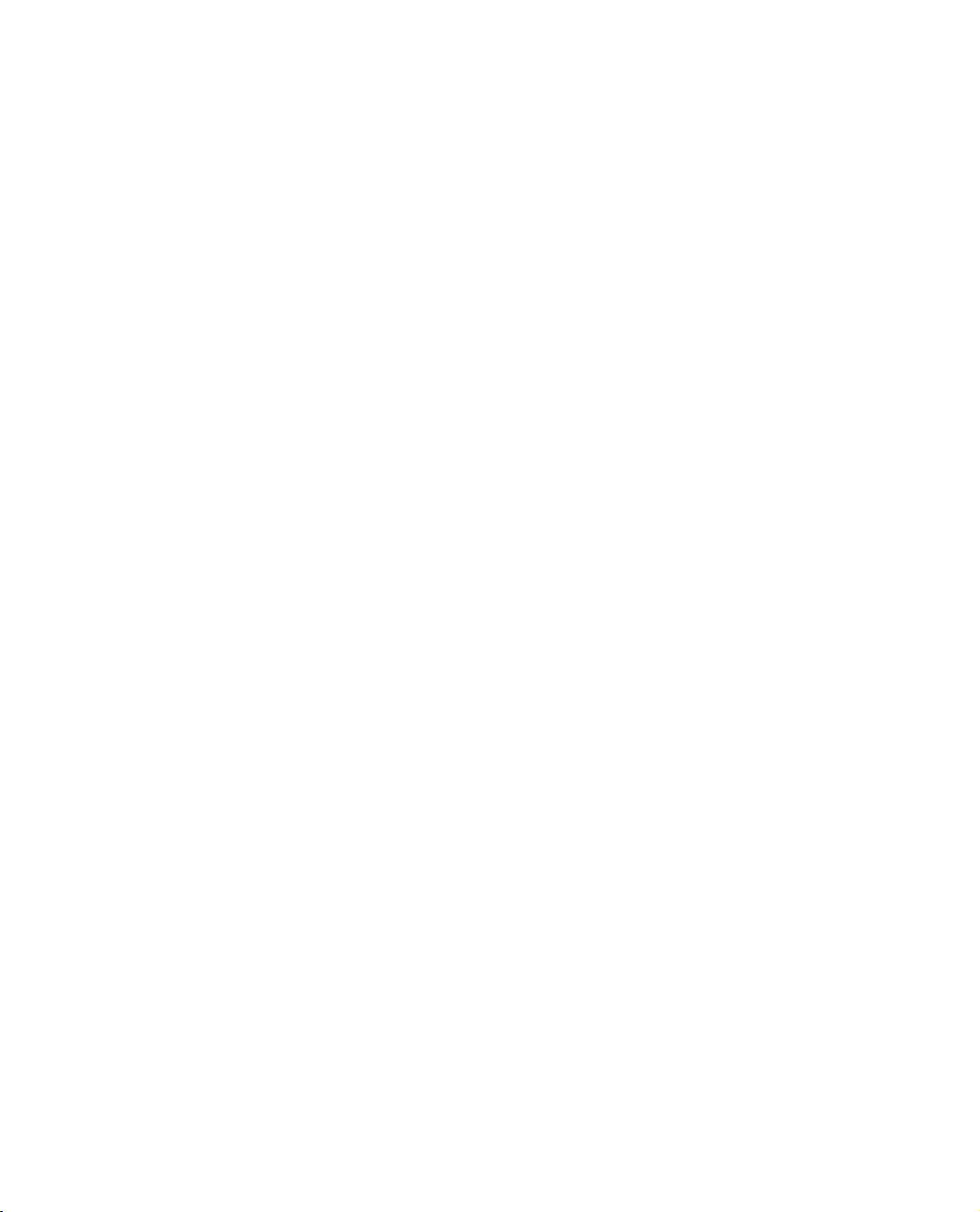
CertPrs8/Java 6 Cert. StudyGuide/Sierra-Bates/159106-0/Chapter 1
2
Chapter 1: Declarations and Access Control
W
e assume that because you're planning on becoming certified, you already know
the basics of Java. If you're completely new to the language, this chapter—and the
rest of the book—will be confusing; so be sure you know at least the basics of the
language before diving into this book. That said, we're starting with a brief, high-level refresher to
put you back in the Java mood, in case you've been away for awhile.
Java Refresher
A Java program is mostly a collection of objects talking to other objects by invoking
each other's methods. Every object is of a certain type, and that type is defined by a
class or an interface. Most Java programs use a collection of objects of many different
types.
n Class A template that describes the kinds of state and behavior that objects
of its type support.
n Object At runtime, when the Java Virtual Machine (JVM) encounters the
new keyword, it will use the appropriate class to make an object which is an
instance of that class. That object will have its own state, and access to all of
the behaviors defined by its class.
n State (instance variables) Each object (instance of a class) will have its
own unique set of instance variables as defined in the class. Collectively, the
values assigned to an object's instance variables make up the object's state.
n Behavior (methods) When a programmer creates a class, she creates meth-
ods for that class. Methods are where the class' logic is stored. Methods are
where the real work gets done. They are where algorithms get executed, and
data gets manipulated.
Identifiers and Keywords
All the Java components we just talked about—classes, variables, and methods—
need names. In Java these names are called identifiers, and, as you might expect,
there are rules for what constitutes a legal Java identifier. Beyond what's legal,
ch01.indd 2 5/21/08 12:58:19 PM
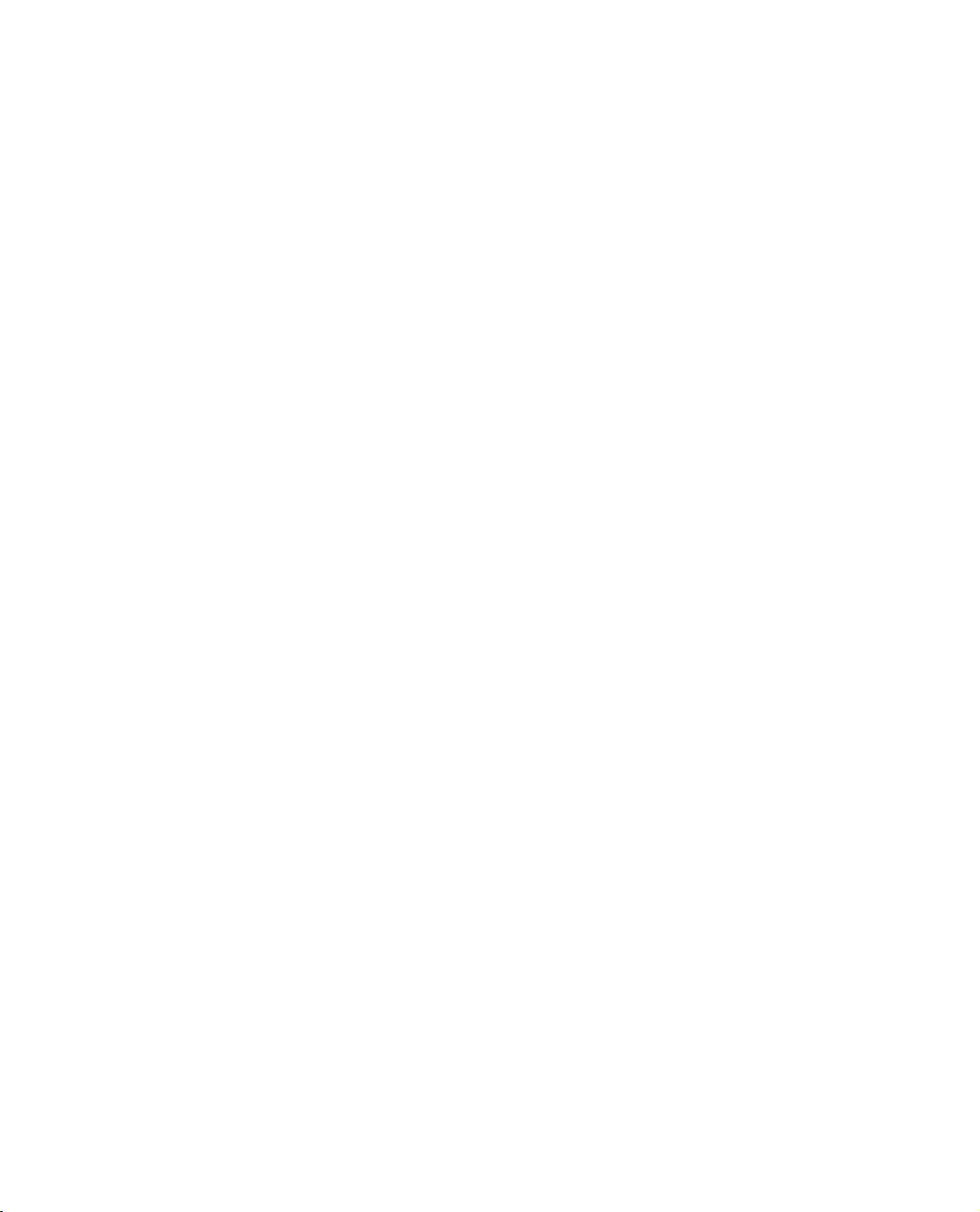
CertPrs8/Java 6 Cert. StudyGuide/Sierra-Bates/159106-0/Chapter 1
Java Refresher
3
though, Java programmers (and Sun) have created conventions for naming methods,
variables, and classes.
Like all programming languages, Java has a set of built-in keywords. These
keywords must not be used as identifiers. Later in this chapter we'll review the details
of these naming rules, conventions, and the Java keywords.
Inheritance
Central to Java and other object-oriented (OO) languages is the concept of
inheritance, which allows code defined in one class to be reused in other classes. In
Java, you can define a general (more abstract) superclass, and then extend it with
more specific subclasses. The superclass knows nothing of the classes that inherit from
it, but all of the subclasses that inherit from the superclass must explicitly declare the
inheritance relationship. A subclass that inherits from a superclass is automatically
given accessible instance variables and methods defined by the superclass, but is also
free to override superclass methods to define more specific behavior.
For example, a Car superclass class could define general methods common to all
automobiles, but a Ferrari subclass could override the accelerate() method.
Interfaces
A powerful companion to inheritance is the use of interfaces. Interfaces are like a
100-percent abstract superclass that defines the methods a subclass must support, but
not how they must be supported. In other words, an Animal interface might declare
that all Animal implementation classes have an eat() method, but the Animal
interface doesn't supply any logic for the eat() method. That means it's up to the
classes that implement the Animal interface to define the actual code for how that
particular Animal type behaves when its eat() method is invoked.
Finding Other Classes
As we'll see later in the book, it's a good idea to make your classes cohesive. That
means that every class should have a focused set of responsibilities. For instance,
if you were creating a zoo simulation program, you'd want to represent aardvarks
with one class, and zoo visitors with a different class. In addition, you might have
a Zookeeper class, and a Popcorn vendor class. The point is that you don't want a
class that has both Aardvark and Popcorn behaviors (more on that in Chapter 2).
Even a simple Java program uses objects from many different classes: some that
you created, and some built by others (such as Sun's Java API classes). Java organizes
classes into packages, and uses import statements to give programmers a consistent
ch01.indd 3 5/21/08 12:58:19 PM
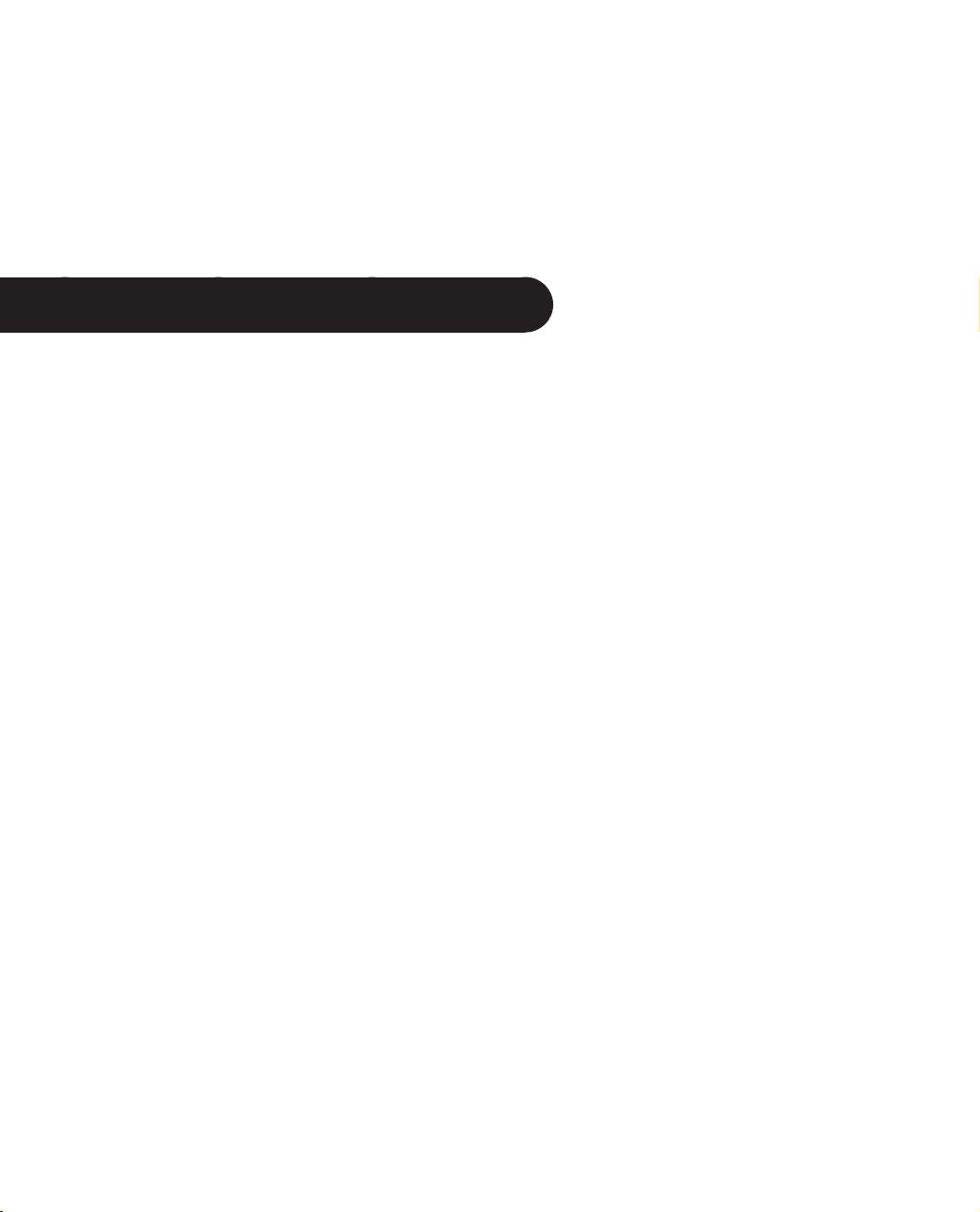
CertPrs8/Java 6 Cert. StudyGuide/Sierra-Bates/159106-0/Chapter 1
way to manage naming of, and access to, classes they need. The exam covers a lot of
concepts related to packages and class access; we'll explore the details in this—and
later—chapters.
CERTIFICATION OBJECTIVE
Identifiers & JavaBeans (Objectives 1.3 and 1.4)
1.3 Develop code that declares, initializes, and uses primitives, arrays, enums, and
objects as static, instance, and local variables. Also, use legal identifiers for variable names.
1.4 Develop code that declares both static and non-static methods, and—if appropriate—
use method names that adhere to the JavaBeans naming standards. Also develop code that
declares and uses a variable-length argument list.
Remember that when we list one or more Certification Objectives in the book,
as we just did, it means that the following section covers at least some part of that
objective. Some objectives will be covered in several different chapters, so you'll see
the same objective in more than one place in the book. For example, this section
covers declarations, identifiers, and JavaBeans naming, but using the things you
declare is covered primarily in later chapters.
So, we'll start with Java identifiers. The three aspects of Java identifiers that we
cover here are
n Legal Identifiers The rules the compiler uses to determine whether a
name is legal.
n Sun's Java Code Conventions Sun's recommendations for naming classes,
variables, and methods. We typically adhere to these standards throughout
the book, except when we're trying to show you how a tricky exam question
might be coded. You won't be asked questions about the Java Code Conven-
tions, but we strongly recommend that programmers use them.
n JavaBeans Naming Standards The naming requirements of the JavaBeans
specification. You don't need to study the JavaBeans spec for the exam,
but you do need to know a few basic JavaBeans naming rules we cover in
this chapter.
4
Chapter 1: Declarations and Access Control
ch01.indd 4 5/21/08 12:58:19 PM
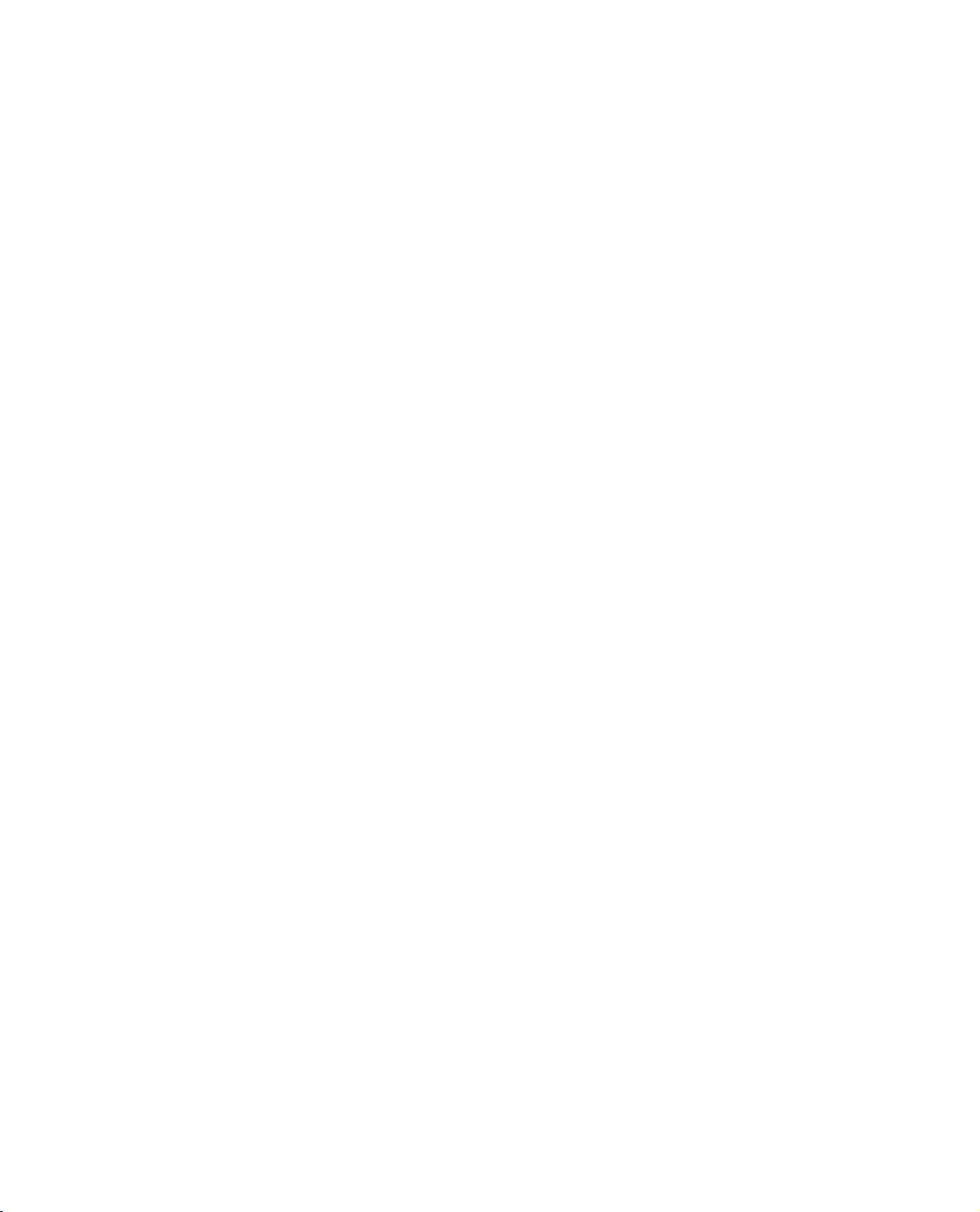
CertPrs8/Java 6 Cert. StudyGuide/Sierra-Bates/159106-0/Chapter 1
Legal Identifiers
Technically, legal identifiers must be composed of only Unicode characters,
numbers, currency symbols, and connecting characters (like underscores). The
exam doesn't dive into the details of which ranges of the Unicode character set are
considered to qualify as letters and digits. So, for example, you won't need to know
that Tibetan digits range from \u0420 to \u0f29. Here are the rules you do need
to know:
n Identifiers must start with a letter, a currency character ($), or a connecting
character such as the underscore ( _ ). Identifiers cannot start with a number!
n After the first character, identifiers can contain any combination of letters,
currency characters, connecting characters, or numbers.
n In practice, there is no limit to the number of characters an identifier can
contain.
n You can't use a Java keyword as an identifier. Table 1-1 lists all of the Java
keywords including one new one for 5.0, enum.
n Identifiers in Java are case-sensitive; foo and FOO are two different identifiers.
Examples of legal and illegal identifiers follow, first some legal identifiers:
int _a;
int $c;
int ______2_w;
int _$;
int this_is_a_very_detailed_name_for_an_identifier;
The following are illegal (it's your job to recognize why):
int :b;
int -d;
int e#;
int .f;
int 7g;
Legal Identifiers (Exam Objectives 1.3 and 1.4)
5
ch01.indd 5 5/21/08 12:58:19 PM