设置文本颜色和字体API

根据给定的信息,本文将详细解释C++中用于设置文本颜色和字体的API,并通过具体的API函数进行逐一解析,帮助读者更好地理解如何在C++环境下控制文本的颜色与样式。 ### 设置文本颜色 #### `SetTextColor` `SetTextColor`是Windows API中的一个函数,用于设置设备上下文中前景色的颜色。这个函数的返回值是之前设置的颜色,可以用来保存以备后续恢复原始设置时使用。函数原型如下: ```cpp COLORREF SetTextColor( HDC hdc, // handle to DC COLORREF crColor // new text color ); ``` - `hdc`: 设备上下文(Device Context)句柄。 - `crColor`: 新的文本颜色。 ### 设置字体 #### `AddFontResource` `AddFontResource`用于向系统添加一个新的字体资源。此函数会增加对字体文件的引用计数,并且使得该字体对所有应用程序可见。如果字体已存在,则不会重复加载。 ```cpp LONG AddFontResource( LPCSTR lpszFilename // font filename or font resource ID ); ``` - `lpszFilename`: 字体文件名或字体资源ID。 #### `CreateFont` 和 `CreateFontIndirect` 这两个函数都用于创建一个逻辑字体对象。其中`CreateFont`通过一系列参数来定义字体属性,而`CreateFontIndirect`则是通过传入一个`LOGFONT`结构体来创建。 ##### `CreateFont` ```cpp HFONT CreateFont( int nHeight, // height of font int nWidth, // average character width int nEscapement, // angle of escapement int nOrientation, // base-line orientation angle int fnWeight, // font weight DWORD bItalic, // italic attribute selector DWORD bUnderline, // underline attribute selector DWORD bStrikeOut, // strike-out attribute selector DWORD iCharset, // character set identifier DWORD iOutPrecision, // output precision DWORD iClipPrecision,// clipping precision DWORD iQuality, // output quality DWORD iPitchAndFamily,// pitch and family LPCSTR lpszFacename // typeface name ); ``` ##### `CreateFontIndirect` ```cpp HFONT CreateFontIndirect( const LOGFONT *lpLogFont // specifies logical font ); ``` - `lpLogFont`: 指向包含字体属性的`LOGFONT`结构的指针。 #### `CreateScalableFontResource` 这个函数为TrueType字体创建一个字体资源,并注册到系统中,使得其他应用程序也能使用它。 ```cpp LONG CreateScalableFontResource( UINT uiInstanceID, // resource instance identifier LPCTSTR pszFaceName, // typeface name HMODULE hmod, // handle to module containing resource LPCTSTR pszResourceName // name of font resource ); ``` ### 文本绘制相关API #### `DrawText` `DrawText`函数用来绘制文本,并且可以指定多种格式化选项。 ```cpp int DrawText( HDC hdc, // handle to DC LPCSTR lpchText, // pointer to text string int cchText, // number of characters LPRECT lprc, // pointer to rectangle UINT uFormat // text formatting ); ``` - `lpchText`: 指向字符串的指针。 - `uFormat`: 格式化标志。 #### `DrawTextEx` `DrawTextEx`函数扩展了`DrawText`的功能,提供了更多的文本绘制选项。 ```cpp int DrawTextEx( HDC hdc, // handle to DC LPCSTR lpszText, // pointer to text string int cchText, // number of characters LPRECT lprc, // pointer to rectangle UINT dwDTFormat, // text formatting DTTOPTS *lpDTTOpts// text options ); ``` ### 获取文本信息相关API #### `GetTextMetrics` `GetTextMetrics`用于获取当前选定设备上下文中的字体度量信息。 ```cpp BOOL GetTextMetrics( HDC hdc, // handle to DC LPTMETRIC lptm // pointer to text metric structure ); ``` - `lptm`: 指向`TEXTMETRIC`结构的指针。 ### 其他相关API #### `EnumFontFamilies`、`EnumFontFamiliesEx`、`EnumFonts` 这三个函数用于枚举设备上下文中可用的字体。 - `EnumFontFamilies`:枚举指定字体家族的字体。 - `EnumFontFamiliesEx`:枚举指定字体家族的字体,并提供更多的信息。 - `EnumFonts`:枚举设备上下文中所有字体。 ### 示例代码 下面给出一个简单的示例,展示了如何使用`graphics.h`库中的函数来设置文本样式。 ```cpp #include <graphics.h> #include <stdlib.h> #include <stdio.h> #include <conio.h> char* fname[] = { "DEFAULTfont", "TRIPLEXfont", "SMALLfont", "SANSSERIFfont", "GOTHICfont" }; int main(void) { int gdriver = DETECT, gmode, errorcode; int style, midx, midy; int size = 1; initgraph(&gdriver, &gmode, ""); /* initialize graphics */ errorcode = graphresult(); /* read result of initialization */ if (errorcode != grOk) { /* an error occurred */ printf("Graphics error: %s\n", grapherrormsg(errorcode)); printf("Press any key to halt:"); getch(); exit(1); /* terminate with an error code */ } /* ... 进行绘图操作 ... */ closegraph(); /* close everything down */ return 0; } ``` 以上是对C++中用于设置文本颜色和字体API的详细介绍。这些API在开发基于Windows的应用程序时非常有用,能够帮助开发者灵活地控制界面中的文字样式和颜色。
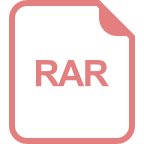
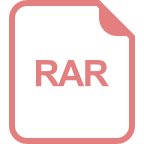
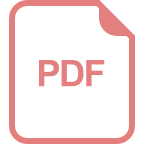
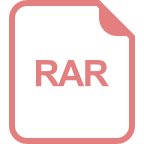
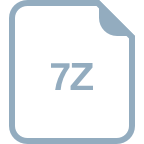
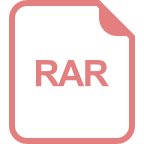
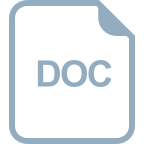
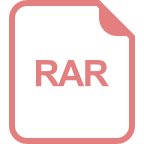
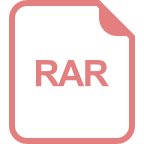
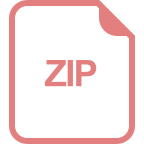
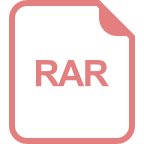
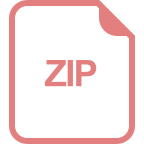
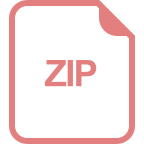
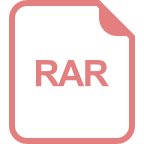
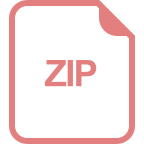
C中与图形有关的在#include "graphics.h"里面.
setcolor 颜色. 可以用数字表示色彩.. 好象1-12
API之文本和字体函数
AddFontResource 在Windows系统中添加一种字体资源
CreateFont 用指定的属性创建一种逻辑字体
CreateFontIndirect 用指定的属性创建一种逻辑字体
CreateScalableFontResource 为一种TureType字体创建一个资源文件,以便能用API函数AddFontResource将其加入Windows系统
DrawText 将文本描绘到指定的矩形中
DrawTextEx 与DrawText相似,只是加入了更多的功能
EnumFontFamilies 列举指定设备可用的字体
EnumFontFamiliesEx 列举指定设备可用的字体
EnumFonts 列举指定设备可用的字体
ExtTextOut 经过扩展的文本描绘函数。也请参考SetTextAlign函数
GetAspectRatioFilterEx 用SetMapperFlags要求Windows只选择与设备当前纵横比相符的光栅字体时,本函数可判断纵横比大小
GetCharABCWidths 判断TureType字体中一个或多个字符的A-B-C大小
GetCharABCWidthsFloat 查询一种字体中一个或多个字符的A-B-C尺寸
GetCharacterPlacement 该函数用于了解如何用一个给定的字符显示一个字串
GetCharWidth 调查字体中一个或多个字符的宽度
GetFontData 接收一种可缩放字体文件的数据
GetFontLanguageInfo 返回目前选入指定设备场景中的字体的信息
GetGlyphOutline 取得TureType字体中构成一个字符的曲线信息
GetKerningPairs 取得指定字体的字距信息
GetOutlineTextMetrics 接收与TureType字体内部特征有关的详细信息
GetRasterizerCaps 了解系统是否有能力支持可缩放的字体
GetTabbedTextExtent 判断一个字串占据的范围,同时考虑制表站扩充的因素
GetTextAlign 接收一个设备场景当前的文本对齐标志
GetTextCharacterExtra 判断额外字符间距的当前值

- 粉丝: 30
- 资源: 32
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

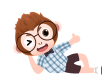
最新资源
- springboot项目 房源信息管理系统.zip
- springboot项目 高速公路收费管理系统.zip
- springboot项目 大学生兼职系统.zip
- springboot项目 爱康医院专家预约管理系统.zip
- springboot项目 成绩管理系统.zip
- springboot项目 驾校预约管理系统.zip
- springboot项目 医药管理系统.zip
- HHO哈里斯鹰算法算法优化KELM核极限学习机(HHO-KELM)回归预测MATLAB代码 代码注释清楚 main为主程序,可以读取EXCEL数据 很方便,容易上手 (电厂运行数据为例) 温
- springboot项目 咖啡厅管理系统.zip
- springboot项目 房屋交易系统.zip
- springboot项目 共享型汽车租赁系统.zip
- GWO灰狼优化算法算法优化KELM核极限学习机(GWO-KELM)回归预测MATLAB代码 代码注释清楚 main为主程序,可以读取EXCEL数据 很方便,容易上手 (电厂运行数据为例) 温
- 多租户物联网平台服务器框架是根据多年经验以及实际客户需求而研发 主要技术基于高性能、高并发的IOCP Sokect基础上研发而成 目前使用的C#语言开发,运行于Windows平台,后期会推出跨平台
- NPC整流器,三电平整流器,中点钳位 PWM整流器三电平模型 simulink matlab,电子文件
- 两阶段微电网鲁棒优化代码 分别以投资成本和运行成本为目标 采用C&CG算法将模型分为主问题和子问题 用KKT条件将双层模型进行转化 使用MATLAB环境下的yalmip+cplex进行求解
- 2000-2020年分城市分行业新注册企业数目数据-最新出炉.zip

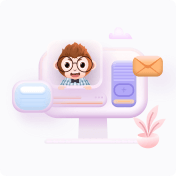

- 1
- 2
前往页