// MainFrm.cpp : implementation of the CMainFrame class
//
#include "stdafx.h"
#include "SimpleBrowse.h"
#include "MainFrm.h"
#include "LeftView.h"
#include "SimpleBrowseView.h"
#include "Aid.h"
#include "io.h"
#ifdef _DEBUG
#define new DEBUG_NEW
#undef THIS_FILE
static char THIS_FILE[] = __FILE__;
#endif
/////////////////////////////////////////////////////////////////////////////
// CMainFrame
IMPLEMENT_DYNCREATE(CMainFrame, CFrameWnd)
BEGIN_MESSAGE_MAP(CMainFrame, CFrameWnd)
//{{AFX_MSG_MAP(CMainFrame)
ON_WM_CREATE()
ON_COMMAND(ID_VIEW_THUMBNAIL, OnViewThumbnail)
ON_UPDATE_COMMAND_UI(ID_VIEW_THUMBNAIL, OnUpdateViewThumbnail)
ON_COMMAND(ID_HTML_HELP, OnHtmlHelp)
ON_COMMAND(ID_DIY, OnDiy)
//}}AFX_MSG_MAP
ON_UPDATE_COMMAND_UI_RANGE(AFX_ID_VIEW_MINIMUM, AFX_ID_VIEW_MAXIMUM, OnUpdateViewStyles)
ON_COMMAND_RANGE(AFX_ID_VIEW_MINIMUM, AFX_ID_VIEW_MAXIMUM, OnViewStyle)
END_MESSAGE_MAP()
static UINT indicators[] =
{
ID_SEPARATOR, // status line indicator
ID_INDICATOR_CAPS,
ID_INDICATOR_NUM,
ID_INDICATOR_SCRL,
};
/////////////////////////////////////////////////////////////////////////////
// CMainFrame construction/destruction
CMainFrame::CMainFrame()
{
// TODO: add member initialization code here
}
CMainFrame::~CMainFrame()
{
}
int CMainFrame::OnCreate(LPCREATESTRUCT lpCreateStruct)
{
if (CFrameWnd::OnCreate(lpCreateStruct) == -1)
return -1;
if (!m_wndToolBar.CreateEx(this) ||
!m_wndToolBar.LoadToolBar(IDR_MAINFRAME))
{
TRACE0("Failed to create toolbar\n");
return -1; // fail to create
}
// if (!m_wndDlgBar.Create(this, IDR_MAINFRAME,
// CBRS_ALIGN_TOP, AFX_IDW_DIALOGBAR))
// {
// TRACE0("Failed to create dialogbar\n");
// return -1; // fail to create
// }
if (!m_wndReBar.Create(this) ||
!m_wndReBar.AddBar(&m_wndToolBar))// ||
// !m_wndReBar.AddBar(&m_wndDlgBar))
{
TRACE0("Failed to create rebar\n");
return -1; // fail to create
}
if (!m_wndStatusBar.Create(this) ||
!m_wndStatusBar.SetIndicators(indicators,
sizeof(indicators)/sizeof(UINT)))
{
TRACE0("Failed to create status bar\n");
return -1; // fail to create
}
// TODO: Remove this if you don't want tool tips
m_wndToolBar.SetBarStyle(m_wndToolBar.GetBarStyle() |
CBRS_TOOLTIPS | CBRS_FLYBY);
return 0;
}
BOOL CMainFrame::OnCreateClient(LPCREATESTRUCT /*lpcs*/,
CCreateContext* pContext)
{
// create splitter window
if (!m_wndSplitter.CreateStatic(this, 1, 2))
return FALSE;
if (!m_wndSplitter.CreateView(0, 0, RUNTIME_CLASS(CLeftView), CSize(100, 100), pContext) ||
!m_wndSplitter.CreateView(0, 1, RUNTIME_CLASS(CSimpleBrowseView), CSize(100, 100), pContext))
{
m_wndSplitter.DestroyWindow();
return FALSE;
}
return TRUE;
}
BOOL CMainFrame::PreCreateWindow(CREATESTRUCT& cs)
{
if( !CFrameWnd::PreCreateWindow(cs) )
return FALSE;
// TODO: Modify the Window class or styles here by modifying
// the CREATESTRUCT cs
return TRUE;
}
/////////////////////////////////////////////////////////////////////////////
// CMainFrame diagnostics
#ifdef _DEBUG
void CMainFrame::AssertValid() const
{
CFrameWnd::AssertValid();
}
void CMainFrame::Dump(CDumpContext& dc) const
{
CFrameWnd::Dump(dc);
}
#endif //_DEBUG
/////////////////////////////////////////////////////////////////////////////
// CMainFrame message handlers
CSimpleBrowseView* CMainFrame::GetRightPane()
{
CWnd* pWnd = m_wndSplitter.GetPane(0, 1);
CSimpleBrowseView* pView = DYNAMIC_DOWNCAST(CSimpleBrowseView, pWnd);
return pView;
}
void CMainFrame::OnUpdateViewStyles(CCmdUI* pCmdUI)
{
// TODO: customize or extend this code to handle choices on the
// View menu.
CSimpleBrowseView* pView = GetRightPane();
// if the right-hand pane hasn't been created or isn't a view,
// disable commands in our range
if (pView == NULL)
pCmdUI->Enable(FALSE);
else
{
BOOL bThumbnail = pView->m_ThumbListCtrl.IsInThumbnailView();
DWORD dwStyle = pView->m_ThumbListCtrl.GetStyle() & LVS_TYPEMASK;
// if the command is ID_VIEW_LINEUP, only enable command
// when we're in LVS_ICON or LVS_SMALLICON mode
if (pCmdUI->m_nID == ID_VIEW_LINEUP)
{
if (dwStyle == LVS_ICON || dwStyle == LVS_SMALLICON)
pCmdUI->Enable();
else
pCmdUI->Enable(FALSE);
}
else
{
// otherwise, use dots to reflect the style of the view
pCmdUI->Enable();
BOOL bChecked = FALSE;
switch (pCmdUI->m_nID)
{
case ID_VIEW_DETAILS:
bChecked = (dwStyle == LVS_REPORT);
break;
case ID_VIEW_SMALLICON:
bChecked = (dwStyle == LVS_SMALLICON);
break;
case ID_VIEW_LARGEICON:
bChecked = (dwStyle == LVS_ICON);
break;
case ID_VIEW_LIST:
bChecked = (dwStyle == LVS_LIST);
break;
default:
bChecked = FALSE;
break;
}
bChecked = bChecked && !bThumbnail;
pCmdUI->SetRadio(bChecked ? 1 : 0);
}
}
}
void CMainFrame::OnViewStyle(UINT nCommandID)
{
// TODO: customize or extend this code to handle choices on the
// View menu.
CSimpleBrowseView* pView = GetRightPane();
// if the right-hand pane has been created and is a CSimpleBrowseView,
// process the menu commands...
if (pView != NULL)
{
DWORD dwStyle = -1;
switch (nCommandID)
{
case ID_VIEW_LINEUP:
{
// ask the list control to snap to grid
CListCtrl& refListCtrl = (CListCtrl&)pView->m_ThumbListCtrl;
refListCtrl.Arrange(LVA_SNAPTOGRID);
}
break;
// other commands change the style on the list control
case ID_VIEW_DETAILS:
dwStyle = LVS_REPORT;
break;
case ID_VIEW_SMALLICON:
dwStyle = LVS_SMALLICON;
break;
case ID_VIEW_LARGEICON:
dwStyle = LVS_ICON;
break;
case ID_VIEW_LIST:
dwStyle = LVS_LIST;
break;
}
// change the style; window will repaint automatically
if (dwStyle != -1)
{
// call CThumbListCtrl::LeaveThumbnailView() if leave "thumbnail view"
pView->m_ThumbListCtrl.LeaveThumbnailView();
pView->m_ThumbListCtrl.ModifyStyle(LVS_TYPEMASK, dwStyle);
}
}
}
void CMainFrame::OnViewThumbnail()
{
// TODO: Add your command handler code here
CSimpleBrowseView* pView = GetRightPane();
// if the right-hand pane has been created and is a CSimpleBrowseView,
// process the menu commands...
if (pView != NULL)
{
// call CThumbListCtrl::GotoThumbnailView() if entry "thumbnail view"
pView->m_ThumbListCtrl.GotoThumbnailView();
DWORD dwStyle = LVS_ICON;
pView->m_ThumbListCtrl.ModifyStyle(LVS_TYPEMASK, dwStyle);
}
}
void CMainFrame::OnUpdateViewThumbnail(CCmdUI* pCmdUI)
{
// TODO: Add your command update UI handler code here
CSimpleBrowseView* pView = GetRightPane();
// if the right-hand pane hasn't been created or isn't a view,
// disable commands in our range
if (pView == NULL)
pCmdUI->Enable(FALSE);
else
{
pCmdUI->Enable();
BOOL bThumbnail = pView->m_ThumbListCtrl.IsInThumbnailView();
DWORD dwStyle = pView->m_ThumbListCtrl.GetStyle() & LVS_TYPEMASK;
BOOL bChecked = (dwStyle == LVS_ICON) && bThumbnail;
pCmdUI->SetRadio(bChecked ? 1 : 0);
}
}
void CMainFrame::OnHtmlHelp()
{
// TODO: Add your command handler code here
CString strPath, strFullName;
strPath = CAid::GetExeFilePath();
strPath = strPath.Left(strPath.GetLength() - 1);
int nIndex = -1;
nIndex = strPath.ReverseFind('\\');
if (nIndex != -1)
{
strPath = strPath.Left(nIndex);
}
strFullName = strPath + "\\Docs\\simplebrowse.htm";
if (_access(strFullName, 00) != -1)
::ShellExecute(NULL, TEXT("open"), strFullName,
NULL, NULL, SW_SHOWNORMAL);
}
void CMainFrame::OnDiy()
{
// TODO: Add your command handler code here
CString strPath, strFullName;
strPath = CAid::GetExeFilePath();
strPath = strPath.Left(strPath.GetLength

billqiao00
- 粉丝: 0
- 资源: 2
最新资源
- 旅游推荐-JAVA-基于springBoot的旅游推荐系统设计与实现(毕业论文+开题+PPT)
- PFC5.0,6.0花岗岩单轴GBM,可定义矿物种类,含量,预制孔隙/裂隙单轴压缩实验,孔隙,裂隙可直接CAD导入,可监测应力应变曲线,裂纹数量和种类 代码百分百正常运行,有中文备注,对于后添加的功能
- 基于yolov8-firedetection的火灾探测部署.zip
- 6劳动合同书范本5页.doc
- 全国各省Kml边界,WGS84格式
- 安徽新省劳动合同范本.doc
- 复制leveldb的主要目的是学习LSM-Tree的具体实现,提高C++水平 将附上具体的实施文件,以便更好地阅读项目(以及理解leveldb的实施)-xdb LSM树.zip
- 劳动合同范本(西安市).doc
- 武汉市新版劳动合同.doc
- 药店管理-JAVA-基于springBoot的药店管理系统的设计与实现(毕业论文+开题)
- 大学生就业合同.doc
- 餐厅服务员用工合同范本.doc
- 黑龙江省各市Kml边界数据
- 在e-vue-download中实现文件下载、下载暂停、下载恢复和下载撤销.zip
- 八月最新终极修复版阿里金融蚂蚁金服完整运营版28+修复开奖+机器人
- FlexiFed实验初学者学生复制品- FlexiFed留级生.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


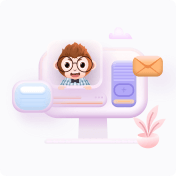
- 1
- 2
前往页