package servlet;
import java.awt.Font;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.PrintWriter;
import java.sql.ResultSet;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import org.jfree.chart.ChartFactory;
import org.jfree.chart.ChartUtilities;
import org.jfree.chart.JFreeChart;
import org.jfree.chart.axis.CategoryAxis;
import org.jfree.chart.axis.NumberAxis;
import org.jfree.chart.axis.NumberTickUnit;
import org.jfree.chart.plot.CategoryPlot;
import org.jfree.chart.plot.PlotOrientation;
import org.jfree.chart.title.TextTitle;
import org.jfree.data.category.DefaultCategoryDataset;
import bean.Indagate;
import bean.OperateDb;
public class Servlet2 extends HttpServlet {
private static final long serialVersionUID = 1L;
OperateDb operatedb;
Indagate indagate;
ResultSet rs;
HttpSession session;
/**
* The doGet method of the servlet. <br>
*
* This method is called when a form has its tag value method equals to get.
*
* @param request
* the request send by the client to the server
* @param response
* the response send by the server to the client
* @throws ServletException
* if an error occurred
* @throws IOException
* if an error occurred
*/
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// 实例化对象
operatedb = new OperateDb();
indagate = new Indagate();
session = request.getSession();
response.setContentType("text/html;charset=utf-8");
PrintWriter out = response.getWriter();
// 数据库中读取调查第一个问题的数据
String sql = "select * from indagate where id = 2";
rs = operatedb.query(sql);
try {
if (rs.next()) {
// 将查询结果封装到indagate中
indagate.setId(rs.getInt(1));
indagate.setTitle(rs.getString(2));
indagate.setFirsta(rs.getString(3));
indagate.setFirstv(rs.getInt(4));
indagate.setSeconda(rs.getString(5));
indagate.setSecondv(rs.getInt(6));
indagate.setThirda(rs.getString(7));
indagate.setThirdv(rs.getInt(8));
indagate.setFourtha(rs.getString(9));
indagate.setFourthv(rs.getInt(10));
indagate.setTotal(rs.getInt(11));
}
} catch (Exception e) {
System.out.println("数据读取错误!");
e.printStackTrace();
}
// 判断用户选择选项
String radio = request.getParameter("radio2");
if (radio.equals("radio")) {
indagate.setFirstv(indagate.getFirstv() + 1);
}
if (radio.equals("radio2")) {
indagate.setSecondv(indagate.getSecondv() + 1);
}
if (radio.equals("radio3")) {
indagate.setThirdv(indagate.getThirdv() + 1);
}
if (radio.equals("radio4")) {
indagate.setFourthv(indagate.getFourthv() + 1);
}
indagate.setTotal(indagate.getTotal() + 1);
// 在session中保存调查题目
session.setAttribute("question", indagate.getTitle());
// 更新数据库中的数据
operatedb.update(indagate);
// 关闭数据库链接
operatedb.close();
// 创建柱状图的数据集,从indagate中调用
DefaultCategoryDataset dataset = new DefaultCategoryDataset();
dataset.setValue(indagate.getFirstv(), indagate.getFirsta(), indagate
.getFirsta());
dataset.setValue(indagate.getSecondv(), indagate.getSeconda(), indagate
.getSeconda());
dataset.setValue(indagate.getThirdv(), indagate.getThirda(), indagate
.getThirda());
dataset.setValue(indagate.getFourthv(), indagate.getFourtha(), indagate
.getFourtha());
// 使用工厂创建饼图图形对象
Integer i = new Integer(indagate.getTotal());
JFreeChart chart = ChartFactory.createBarChart3D("问题:"+indagate.getTitle(),
"回答过此问题的总人数是:" + i.toString() + "人", "人 数", dataset,
PlotOrientation.VERTICAL, true, false, false);
// 设置图形标题、标签字体,防止乱码出现
TextTitle txtTitle = chart.getTitle();
txtTitle.setFont(new Font("黑体", 10, 20));
CategoryPlot categoryplot = (CategoryPlot) chart.getCategoryPlot();
CategoryAxis domainAxis = categoryplot.getDomainAxis();
NumberAxis numberaxis = (NumberAxis) categoryplot.getRangeAxis();
domainAxis.setTickLabelFont(new Font("宋体", 5, 11));
domainAxis.setLabelFont(new Font("宋体", 5, 12));
numberaxis.setTickLabelFont(new Font("宋体", 5, 12));
numberaxis.setLabelFont(new Font("宋体", 5, 12));
chart.getLegend().setItemFont(new Font("黑体", 5, 14));
//设置纵坐标刻度单位
numberaxis.setTickUnit(new NumberTickUnit(3D));
// 保存图形到指定目录
File file = new File("..\\webapps\\indagate\\images\\indagate.jpg");
FileOutputStream jpgchart = new FileOutputStream(file);
ChartUtilities.writeChartAsJPEG(jpgchart, chart, 600, 400);
// 转向到结果显示页面
response.sendRedirect("../result.jsp");
out.flush();
out.close();
}
/**
* The doPost method of the servlet. <br>
*
* This method is called when a form has its tag value method equals to
* post.
*
* @param request
* the request send by the client to the server
* @param response
* the response send by the server to the client
* @throws ServletException
* if an error occurred
* @throws IOException
* if an error occurred
*/
public void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doGet(request, response);
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
JSP网络开发逐步深入源代码
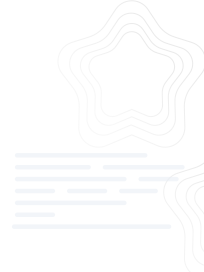
共230个文件
jsp:42个
java:35个
class:35个


温馨提示
《JSP网络开发逐步深入》详细介绍了怎样使用JSP逐步深入进行网络系统设计,从最基础的JSP概念入手,逐步介绍语法和深入开发技术,通过从几种典型模块到4个具体应用系统示例的开发设计,逐步将读者带入JSP的殿堂。 《JSP网络开发逐步深入》内容由浅入深、循序渐进,把理论知识与实验结合讲解,注重提高学习JSP的趣味性、知识性和生动性。通过对《JSP网络开发逐步深入》的学习,读者可以系统地掌握JSP技术的相关概念、方法、编程思路和技巧。 《JSP网络开发逐步深入》不仅可以作为JSP开发的学习用书,还可以作为从事JSP开发的程序员的参考用书和必备手册。此外,《JSP网络开发逐步深入》的配套光盘包含了《JSP网络开发逐步深入》教学视频,对JSP开发进行了全面讲解,可以帮助读者快速地从JSP基础知识的学习过渡到JSP应用开发。
资源推荐
资源详情
资源评论
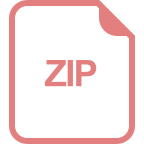
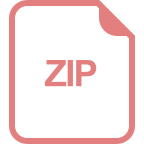
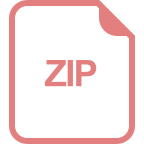
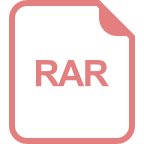
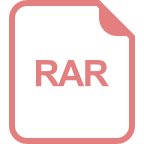
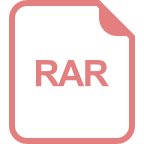
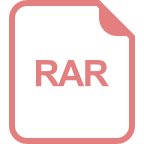
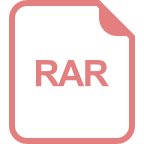
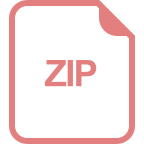
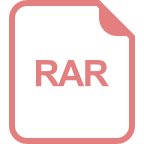
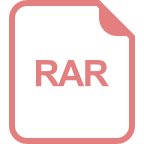
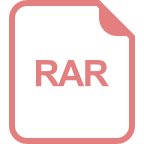
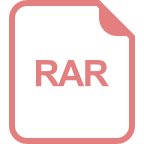
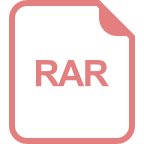
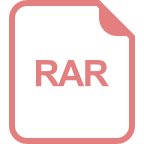
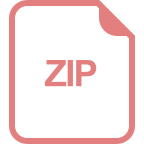
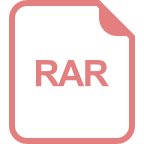
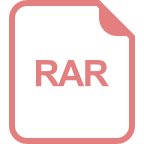
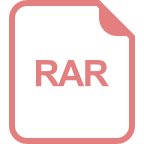
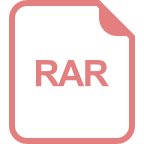
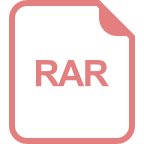
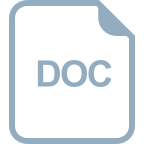
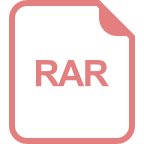
收起资源包目录

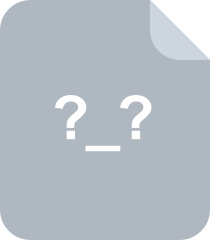
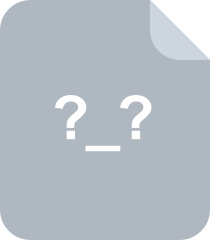
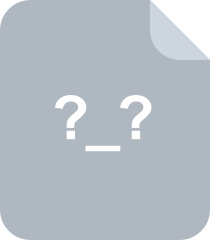
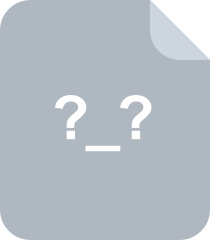
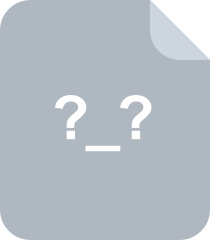
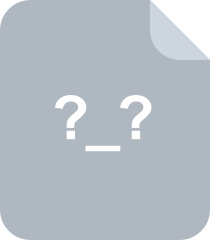
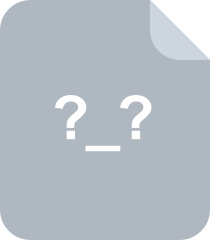
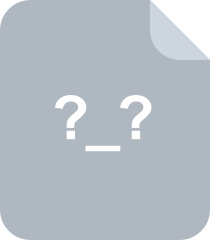
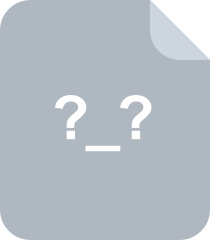
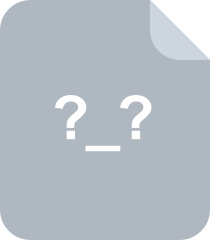
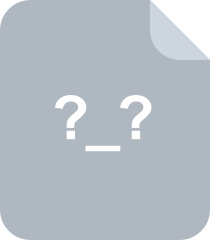
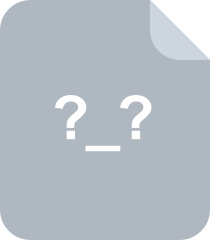
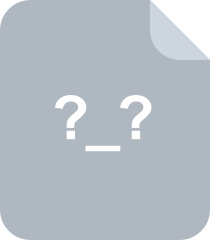
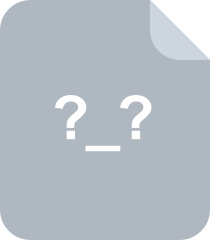
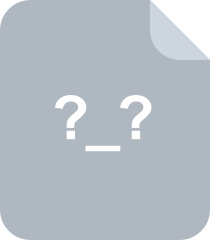
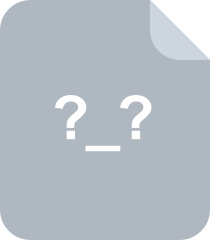
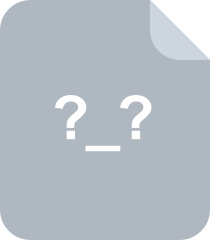
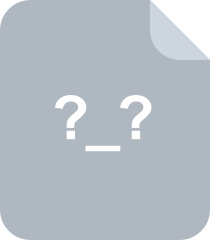
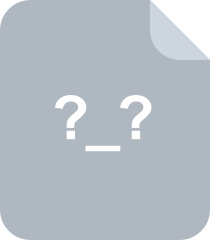
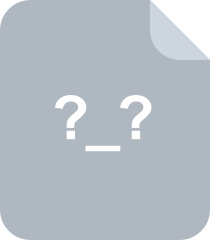
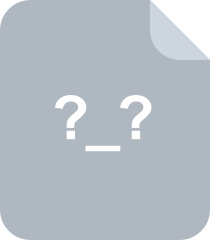
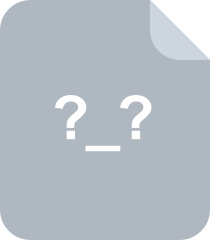
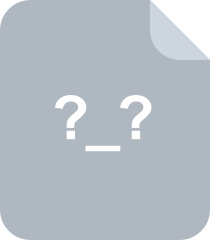
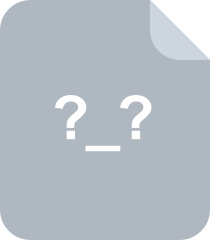
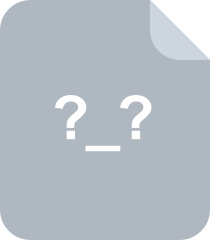
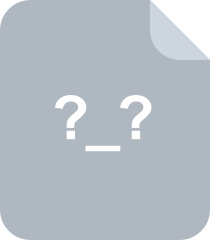
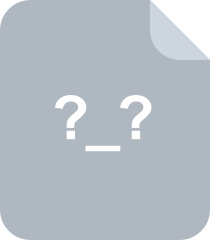
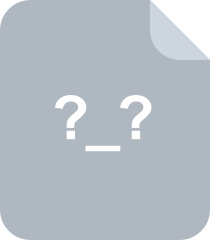
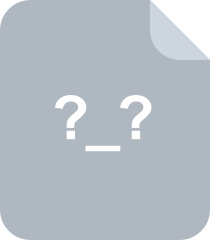
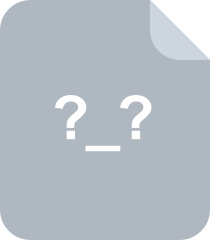
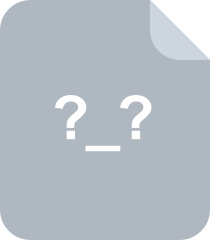
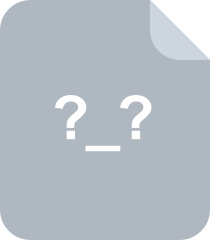
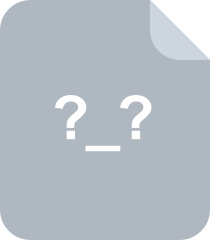
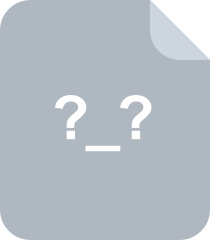
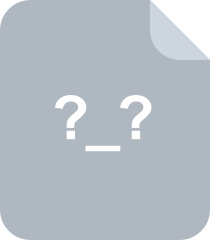
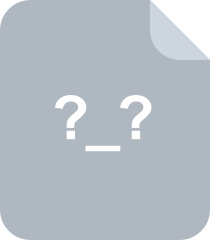
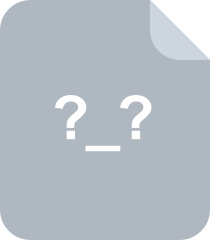
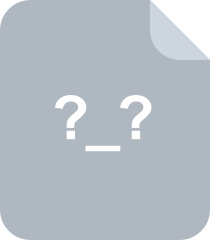
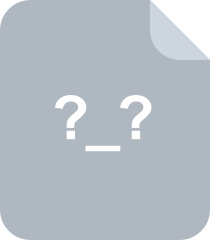
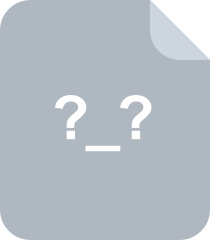
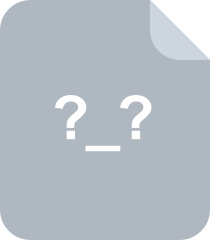
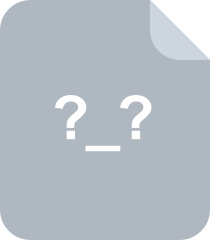
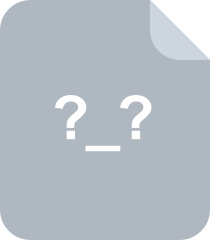
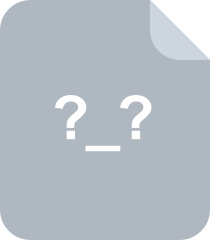
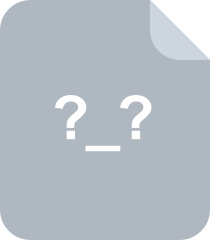
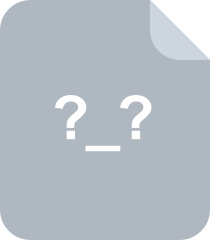
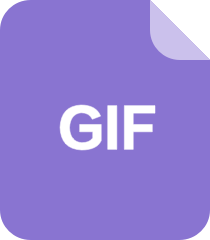
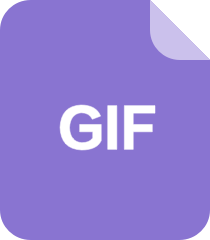
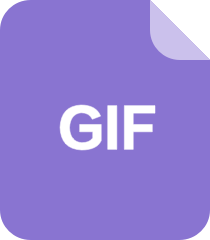
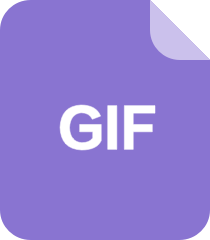
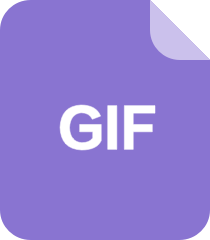
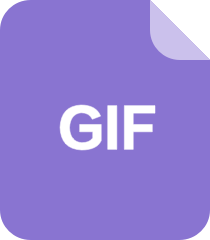
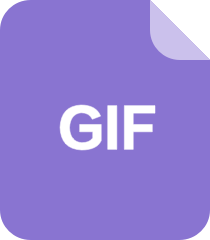
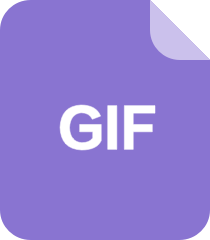
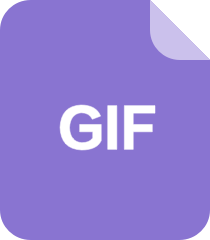
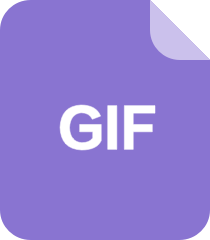
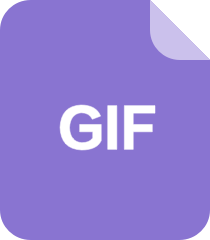
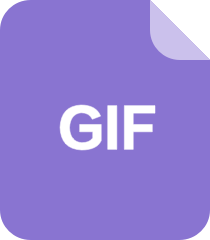
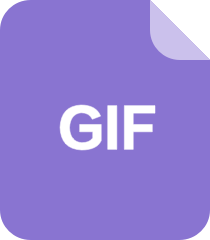
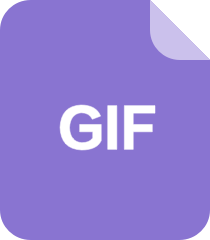
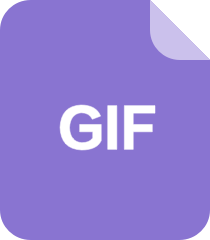
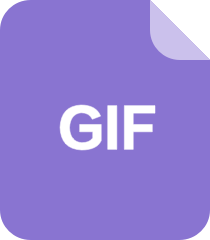
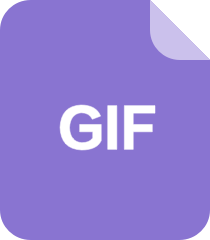
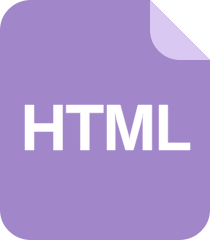
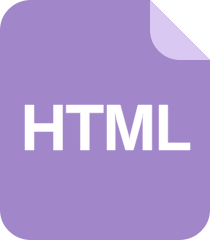
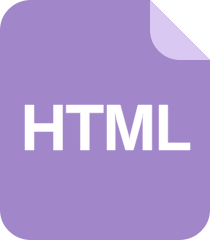
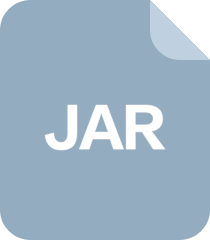
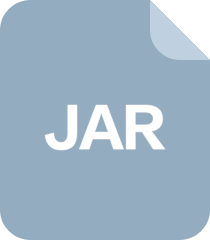
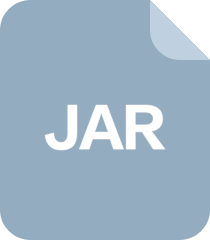
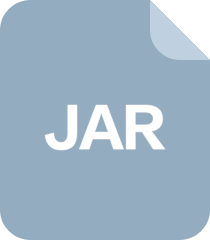
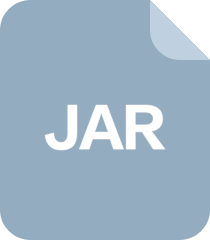
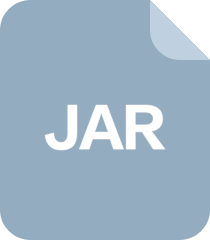
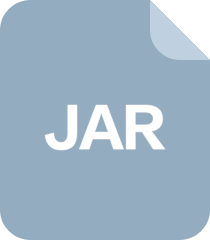
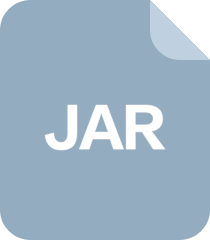
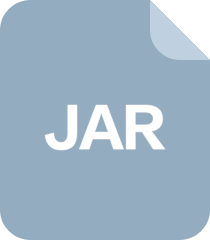
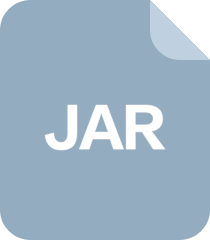
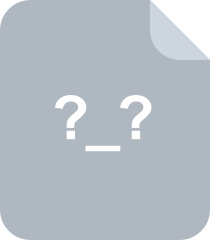
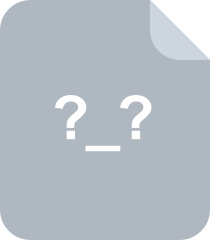
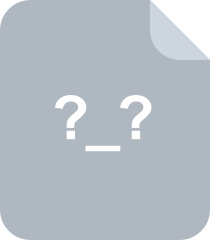
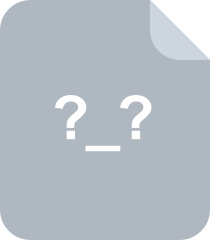
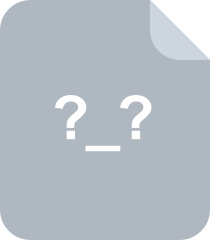
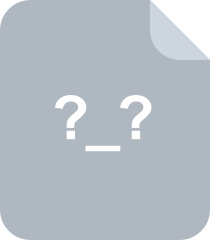
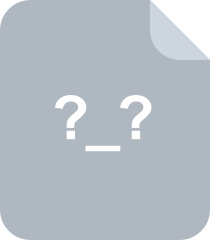
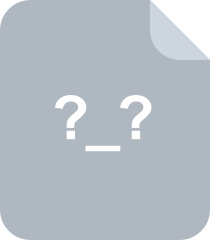
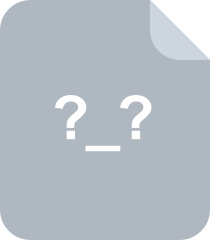
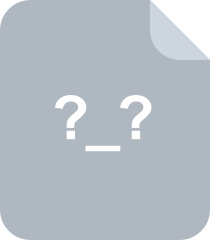
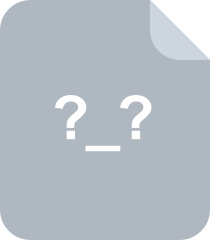
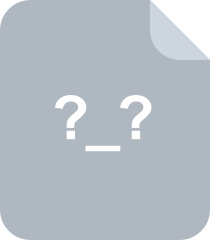
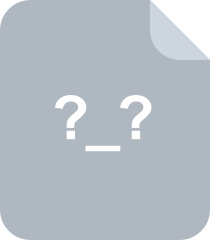
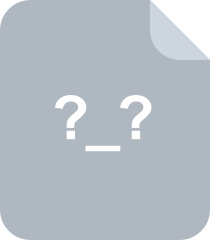
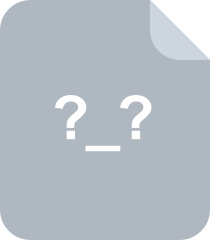
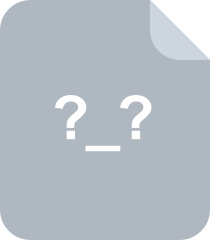
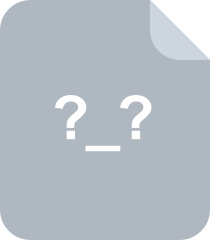
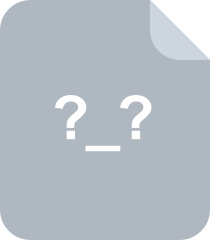
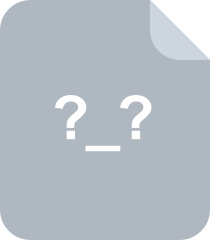
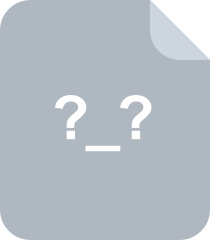
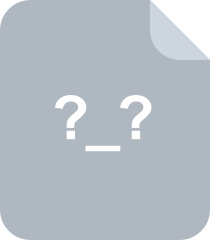
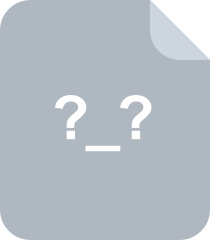
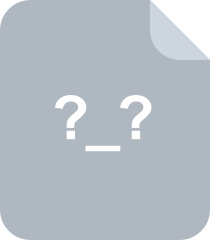
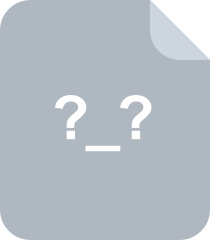
共 230 条
- 1
- 2
- 3

biehuixieleni
- 粉丝: 1
- 资源: 12
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

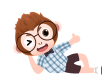
最新资源
- 人、垃圾、非垃圾检测18-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 金智维RPA server安装包
- 二维码图形检测6-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord数据集合集.rar
- Matlab绘制绚丽烟花动画迎新年
- 厚壁圆筒弹性应力计算,过盈干涉量计算
- 网络实践11111111111111
- GO编写图片上传代码.txt
- LabVIEW采集摄像头数据,实现图像数据存储和浏览
- 几种不同方式生成音乐的 Python 源码示例.txt
- python红包打开后出现烟花代码.txt
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


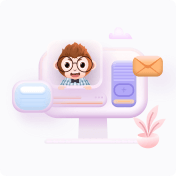
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
前往页