C#操作INI文件源码--完整版)
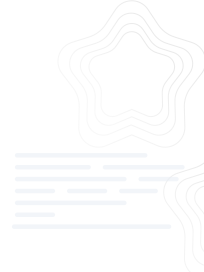

在C#编程中,操作INI配置文件是一种常见的需求,它用于存储应用程序的设置和配置信息。INI文件是一种轻量级的、易于读写的文本格式,通常由键值对组成,结构简单,便于人类理解和编辑。本篇将详细介绍如何在C#中实现对INI文件的读写操作,并提供完整的源码示例。 我们需要了解INI文件的基本结构。一个典型的INI文件可能包含多个节(Section),每个节下有多个键(Key)和对应的值(Value)。例如: ``` [Section1] Key1=Value1 Key2=Value2 [Section2] Key3=Value3 Key4=Value4 ``` 在C#中,我们可以通过使用`System.IO`命名空间中的`StreamReader`和`StreamWriter`类来读写文本文件,但为了方便操作,通常会封装一个专门处理INI文件的类。以下是一个简单的C#操作INI文件的类实现: ```csharp using System; using System.IO; public class IniFile { private string filePath; public IniFile(string path) { this.filePath = path; } // 读取键值 public string Read(string section, string key) { string value = ""; using (StreamReader reader = new StreamReader(filePath)) { string line; while ((line = reader.ReadLine()) != null) { if (line.Trim().StartsWith("[") && line.Trim().EndsWith("]")) { if (line.Trim().Substring(1, line.Trim().Length - 2) == section) { while ((line = reader.ReadLine()) != null) { if (line.Trim().StartsWith(key + "=")) { value = line.Trim().Substring(key.Length + 1); break; } } break; } } } } return value; } // 写入键值 public void Write(string section, string key, string value) { bool foundSection = false; using (StreamWriter writer = new StreamWriter(filePath, false)) { string line; using (StreamReader reader = new StreamReader(filePath)) { while ((line = reader.ReadLine()) != null) { if (line.Trim().StartsWith("[") && line.Trim().EndsWith("]")) { if (line.Trim().Substring(1, line.Trim().Length - 2) == section) { foundSection = true; } } else if (foundSection && line.Trim().StartsWith(key + "=")) { line = key + "=" + value; } writer.WriteLine(line); } if (!foundSection) { writer.WriteLine("[{0}]", section); writer.WriteLine("{0}={1}", key, value); } } } } } ``` 上述代码定义了一个名为`IniFile`的类,包含了读取和写入INI文件的方法。`Read`方法用于获取指定节和键的值,而`Write`方法用于设置或更新键值。注意,`Write`方法会保留原有文件的结构,如果键已经存在,就更新其值;如果节不存在,会在文件末尾添加新的节和键值。 在实际使用时,你可以这样调用这个类: ```csharp IniFile ini = new IniFile("config.ini"); string value = ini.Read("Section1", "Key1"); ini.Write("Section1", "Key1", "NewValue"); ``` 通过这个类,你可以轻松地处理INI文件中的配置信息。在C#项目中,可以将此代码封装成一个静态类或单独的库,以便在多个地方复用。 以上就是关于C#操作INI文件的完整知识,包括了基本概念、文件结构、以及具体的C#源码实现。这个源码示例可以帮助你理解如何在实际项目中处理INI文件,方便进行配置管理和设置存储。
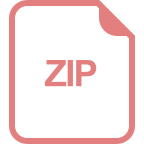
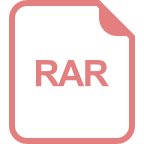
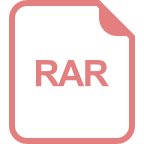
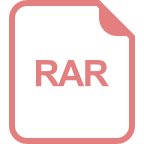
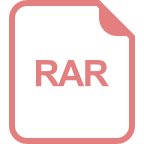
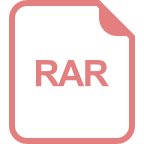
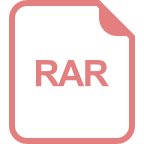
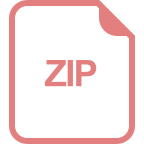
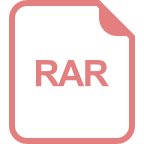
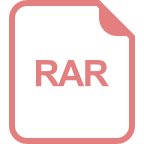
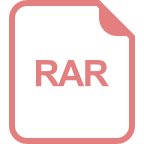
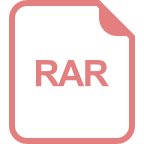
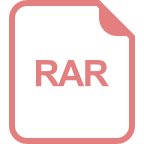
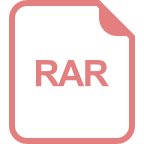
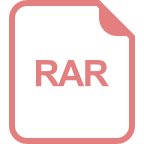
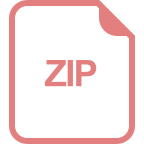


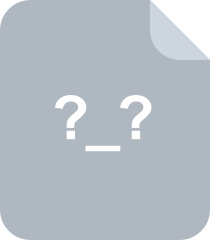
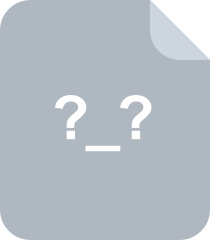

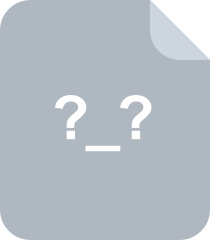
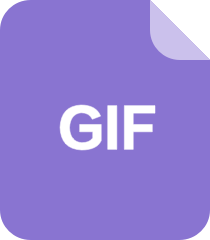
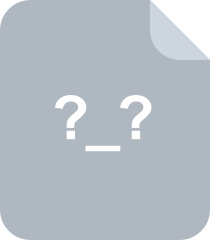
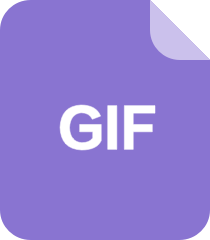
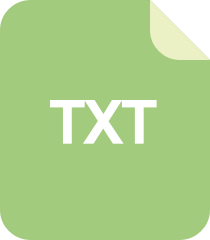




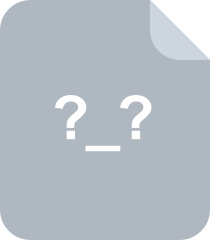
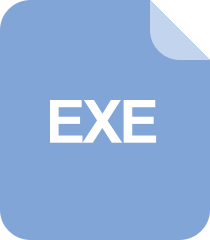
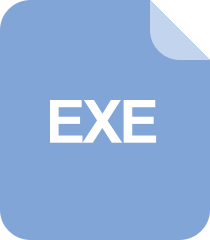
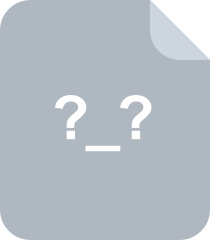
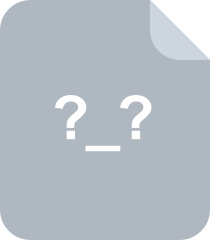
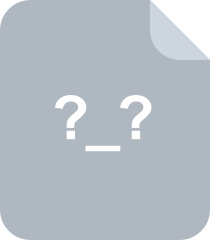
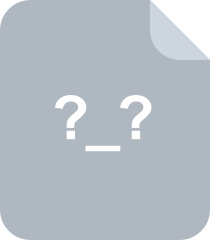


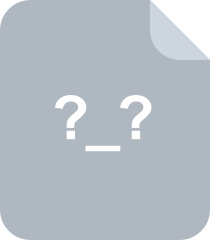
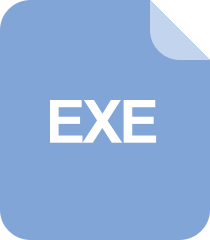
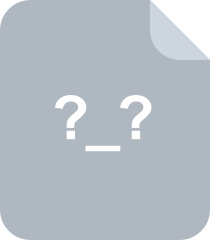
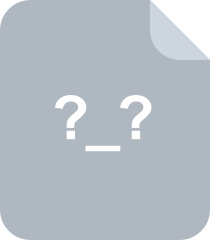
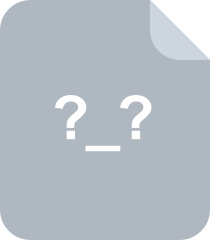

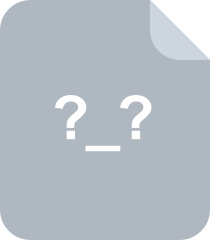
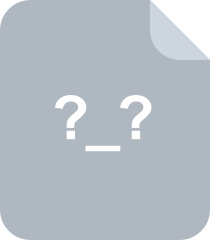
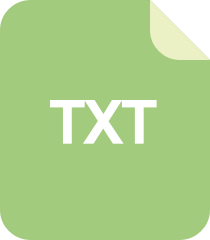
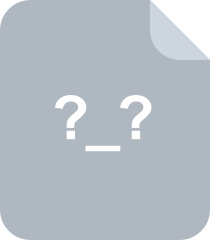
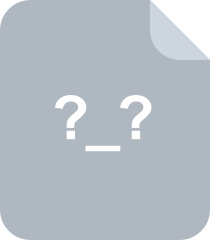
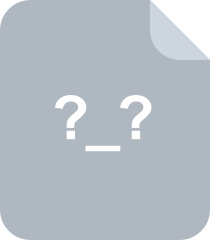
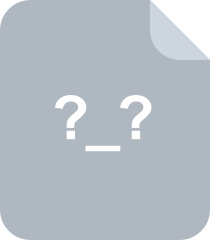
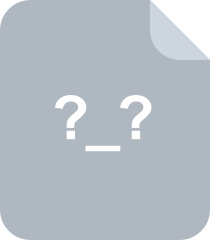

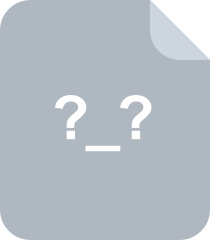
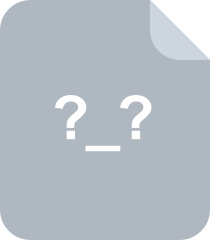
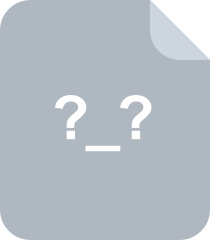
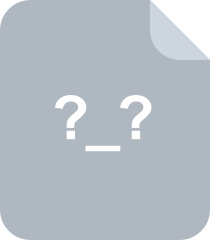
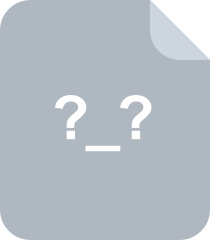


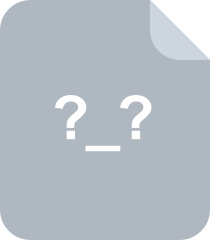
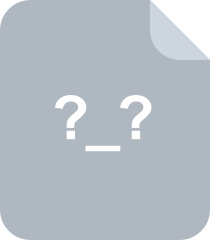
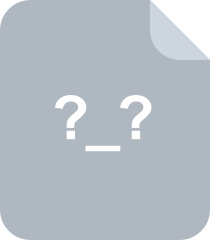
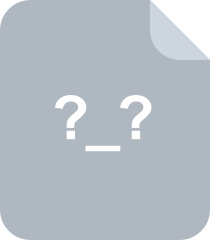
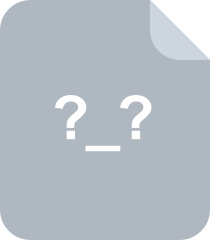

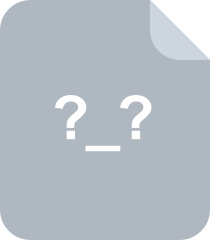
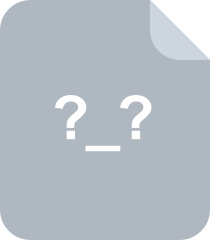
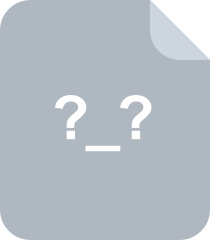
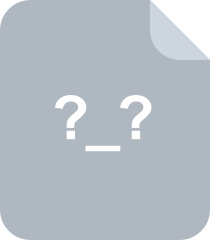
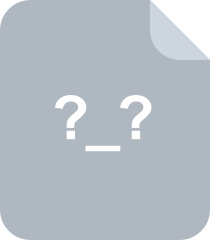
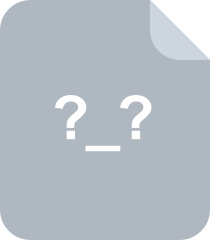
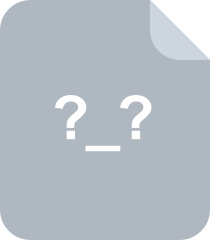
- 1
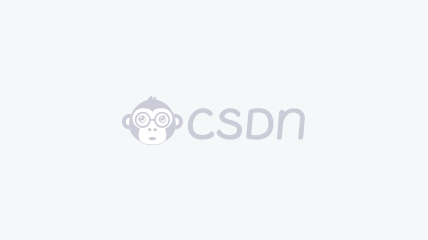

- 粉丝: 562
- 资源: 994
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

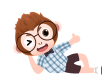
最新资源

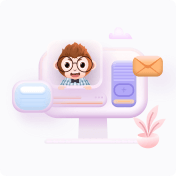
