import numpy as np
class Population:
def __init__(self, pop_size, chromosome_size):
self.pop_size = pop_size
self.chromosome_size = chromosome_size
self.population = np.round(np.random.rand(pop_size, chromosome_size)).astype(np.int)
self.fit_value = np.zeros((pop_size, 1))
def select_chromosome(self):
total_fitness_value = self.fit_value.sum()
p_fit_value = self.fit_value/total_fitness_value
p_fit_value = np.cumsum(p_fit_value)
point = np.sort(np.random.rand(self.pop_size, 1), 0)
fit_in = 0
new_in = 0
new_population = np.zeros_like(self.population)
while new_in < self.pop_size:
if point[new_in] < p_fit_value[fit_in]:
new_population[new_in, :] = self.population[fit_in, :]
new_in += 1
else:
fit_in += 1
self.population = new_population
def cross_chromosome(self, cross_rate):
x = self.pop_size
y = self.chromosome_size
new_population = np.zeros_like(self.population)
for i in range(0, x-1, 2):
if np.random.rand(1) < cross_rate:
insert_point = int(np.round(np.random.rand(1) * y).item())
new_population[i, :] = np.concatenate([self.population[i, 0:insert_point], self.population[i+1, insert_point:y]], 0)
new_population[i+1, :] = np.concatenate([self.population[i+1, 0:insert_point], self.population[i, insert_point:y]], 0)
else:
new_population[i, :] = self.population[i, :]
new_population[i + 1, :] = self.population[i + 1, :]
self.population = new_population
def best(self):
best_individual = self.population[0, :]
best_fit = self.fit_value[0]
for i in range(1, self.pop_size):
if self.fit_value[i] > best_fit:
best_individual = self.population[i, :]
best_fit = self.fit_value[i]
return best_individual, best_fit
def mutation_chromosome(self, mutation_rate):
x = self.pop_size
for i in range(x):
if np.random.rand(1) < mutation_rate:
m_point = int(np.round(np.random.rand(1) * self.chromosome_size).item())
if self.population[i, m_point] == 1:
self.population[i, m_point] = 0
else:
self.population[i, m_point] = 1
def binary2decimal(self, population):
pop1 = np.zeros_like(population)
y = self.chromosome_size
for i in range(y):
pop1[:, i] = 2 ** (y - i - 1) * population[:, i]
pop = np.sum(pop1, 1)
pop2 = pop * 10/(1 << y)
return pop2
def cal_obj_value(self):
x = self.binary2decimal(self.population)
self.fit_value = 10 * np.sin(5 * x) + 7 * np.abs(x - 5) + 10
if __name__ == "__main__":
pop = Population(10, 3)
a = np.random.rand(1, 1)
b = np.random.rand(1, 2)
c = np.concatenate([a, b], 1)
print(c)
point = np.random.rand(10, 1)
point = np.sort(point, 0)
print(point)
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
## 什么是遗传算法 我们了解过深度学习的都知道,我们在进行网络优化的过程都是通过反向传播求导进行参数的不断优化,而这种类型的优化参数采用前向传播的方式继续优化网络,不断找出最优解,或者最优的参数。很多的优化算法都来自于大自然的启发,来一种算法叫做蚁群算法,灵感就是来自于蚂蚁,所以观察大自然有时也是灵感的来源。 遗传算法,也叫Genetic Algorithm,简称 GA 算法他既然叫遗传算法,那么遗传之中必然有基因,那么基因染色体(Chromosome)就是它的需要调节的参数。我们在生物中了解到,大自然的法则是“物竞天择,适者生存”,我觉得遗传算法更适用于“**优胜劣汰**”。 ### 适用人群:MATLAB 爱好者,智能算法,AI
资源推荐
资源详情
资源评论
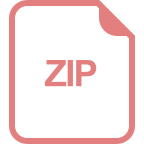
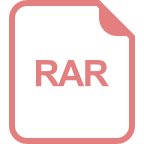
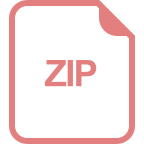
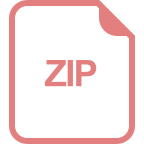
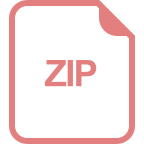
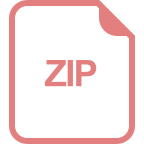
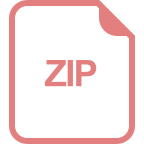
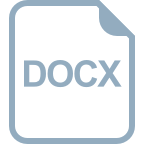
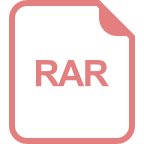
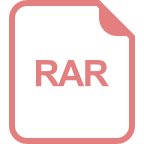
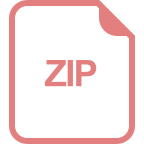
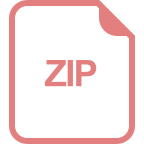
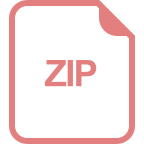
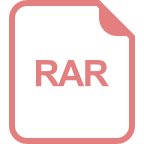
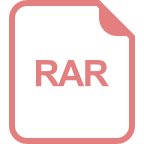
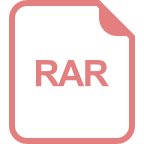
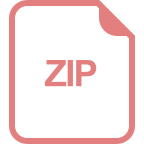
收起资源包目录



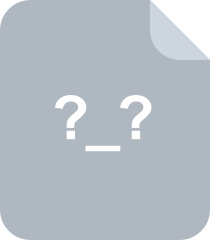
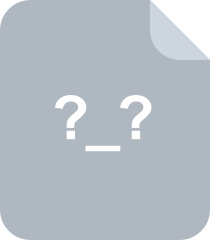
共 2 条
- 1
资源评论
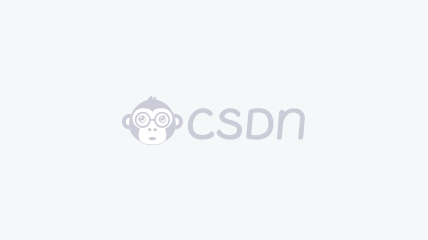

sanbaofengs
- 粉丝: 509
- 资源: 711
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

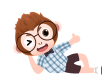
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


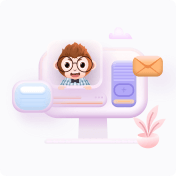
安全验证
文档复制为VIP权益,开通VIP直接复制
