<?php
namespace Tool\Qiniu\Storage;
use Tool\Qiniu\Auth;
use Tool\Qiniu\Config;
use Tool\Qiniu\Zone;
use Tool\Qiniu\Http\Client;
use Tool\Qiniu\Http\Error;
/**
* 主要涉及了空间资源管理及批量操作接口的实现,具体的接口规格可以参考
*
* @link http://developer.qiniu.com/docs/v6/api/reference/rs/
*/
final class BucketManager
{
private $auth;
private $zone;
public function __construct(Auth $auth, Zone $zone = null)
{
$this->auth = $auth;
if ($zone === null) {
$this->zone = new Zone();
}
}
/**
* 获取指定账号下所有的空间名。
*
* @return string[] 包含所有空间名
*/
public function buckets()
{
return $this->rsGet('/buckets');
}
/**
* 列取空间的文件列表
*
* @param $bucket 空间名
* @param $prefix 列举前缀
* @param $marker 列举标识符
* @param $limit 单次列举个数限制
* @param $delimiter 指定目录分隔符
*
* @return array 包含文件信息的数组,类似:[
* {
* "hash" => "<Hash string>",
* "key" => "<Key string>",
* "fsize" => "<file size>",
* "putTime" => "<file modify time>"
* },
* ...
* ]
* @link http://developer.qiniu.com/docs/v6/api/reference/rs/list.html
*/
public function listFiles($bucket, $prefix = null, $marker = null, $limit = 1000, $delimiter = null)
{
$query = array('bucket' => $bucket);
\Qiniu\setWithoutEmpty($query, 'prefix', $prefix);
\Qiniu\setWithoutEmpty($query, 'marker', $marker);
\Qiniu\setWithoutEmpty($query, 'limit', $limit);
\Qiniu\setWithoutEmpty($query, 'delimiter', $delimiter);
$url = Config::RSF_HOST . '/list?' . http_build_query($query);
list($ret, $error) = $this->get($url);
if ($ret === null) {
return array(null, null, $error);
}
$marker = array_key_exists('marker', $ret) ? $ret['marker'] : null;
return array($ret['items'], $marker, null);
}
/**
* 获取资源的元信息,但不返回文件内容
*
* @param $bucket 待获取信息资源所在的空间
* @param $key 待获取资源的文件名
*
* @return array 包含文件信息的数组,类似:
* [
* "hash" => "<Hash string>",
* "key" => "<Key string>",
* "fsize" => "<file size>",
* "putTime" => "<file modify time>"
* ]
*
* @link http://developer.qiniu.com/docs/v6/api/reference/rs/stat.html
*/
public function stat($bucket, $key)
{
$path = '/stat/' . \Qiniu\entry($bucket, $key);
return $this->rsGet($path);
}
/**
* 删除指定资源
*
* @param $bucket 待删除资源所在的空间
* @param $key 待删除资源的文件名
*
* @return mixed 成功返回NULL,失败返回对象Qiniu\Http\Error
* @link http://developer.qiniu.com/docs/v6/api/reference/rs/delete.html
*/
public function delete($bucket, $key)
{
$path = '/delete/' . \Qiniu\entry($bucket, $key);
list(, $error) = $this->rsPost($path);
return $error;
}
/**
* 给资源进行重命名,本质为move操作。
*
* @param $bucket 待操作资源所在空间
* @param $oldname 待操作资源文件名
* @param $newname 目标资源文件名
*
* @return mixed 成功返回NULL,失败返回对象Qiniu\Http\Error
*/
public function rename($bucket, $oldname, $newname)
{
return $this->move($bucket, $oldname, $bucket, $newname);
}
/**
* 给资源进行重命名,本质为move操作。
*
* @param $from_bucket 待操作资源所在空间
* @param $from_key 待操作资源文件名
* @param $to_bucket 目标资源空间名
* @param $to_key 目标资源文件名
*
* @return mixed 成功返回NULL,失败返回对象Qiniu\Http\Error
* @link http://developer.qiniu.com/docs/v6/api/reference/rs/copy.html
*/
public function copy($from_bucket, $from_key, $to_bucket, $to_key, $force = false)
{
$from = \Qiniu\entry($from_bucket, $from_key);
$to = \Qiniu\entry($to_bucket, $to_key);
$path = '/copy/' . $from . '/' . $to;
if ($force) {
$path .= '/force/true';
}
list(, $error) = $this->rsPost($path);
return $error;
}
/**
* 将资源从一个空间到另一个空间
*
* @param $from_bucket 待操作资源所在空间
* @param $from_key 待操作资源文件名
* @param $to_bucket 目标资源空间名
* @param $to_key 目标资源文件名
*
* @return mixed 成功返回NULL,失败返回对象Qiniu\Http\Error
* @link http://developer.qiniu.com/docs/v6/api/reference/rs/move.html
*/
public function move($from_bucket, $from_key, $to_bucket, $to_key, $force = false)
{
$from = \Qiniu\entry($from_bucket, $from_key);
$to = \Qiniu\entry($to_bucket, $to_key);
$path = '/move/' . $from . '/' . $to;
if ($force) {
$path .= '/force/true';
}
list(, $error) = $this->rsPost($path);
return $error;
}
/**
* 主动修改指定资源的文件类型
*
* @param $bucket 待操作资源所在空间
* @param $key 待操作资源文件名
* @param $mime 待操作文件目标mimeType
*
* @return mixed 成功返回NULL,失败返回对象Qiniu\Http\Error
* @link http://developer.qiniu.com/docs/v6/api/reference/rs/chgm.html
*/
public function changeMime($bucket, $key, $mime)
{
$resource = \Qiniu\entry($bucket, $key);
$encode_mime = \Qiniu\base64_urlSafeEncode($mime);
$path = '/chgm/' . $resource . '/mime/' .$encode_mime;
list(, $error) = $this->rsPost($path);
return $error;
}
/**
* 从指定URL抓取资源,并将该资源存储到指定空间中
*
* @param $url 指定的URL
* @param $bucket 目标资源空间
* @param $key 目标资源文件名
*
* @return array 包含已拉取的文件信息。
* 成功时: [
* [
* "hash" => "<Hash string>",
* "key" => "<Key string>"
* ],
* null
* ]
*
* 失败时: [
* null,
* Qiniu/Http/Error
* ]
* @link http://developer.qiniu.com/docs/v6/api/reference/rs/fetch.html
*/
public function fetch($url, $bucket, $key = null)
{
$resource = \Qiniu\base64_urlSafeEncode($url);
$to = \Qiniu\entry($bucket, $key);
$path = '/fetch/' . $resource . '/to/' . $to;
没有合适的资源?快使用搜索试试~ 我知道了~
ThinkPHP3.2整合七牛云最新第三方sdk,版本Release v7.1.3
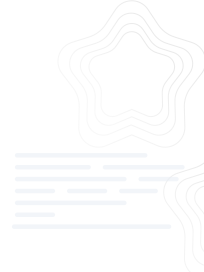
共32个文件
php:32个


温馨提示
ThinkPHP3.2整合七牛云最新第三方sdk,版本Release v7.1.3 详情:http://blog.csdn.net/baikeliang/article/details/58619655
资源推荐
资源详情
资源评论
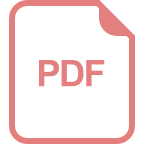
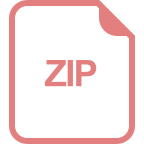
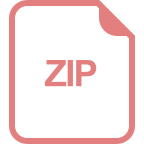
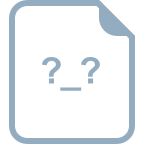
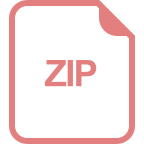
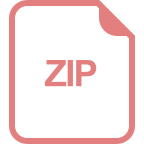
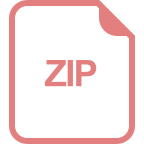
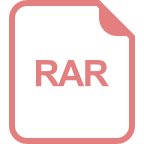
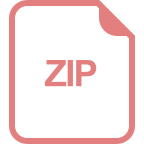
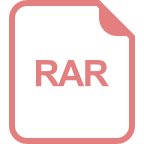
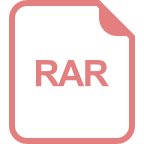
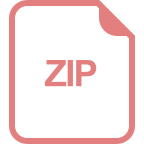
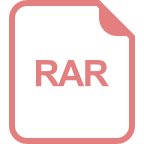
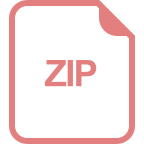
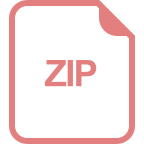
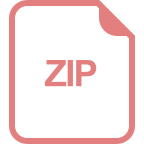
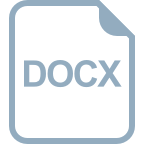
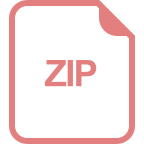
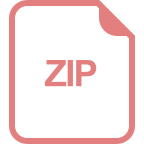
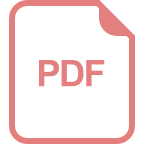
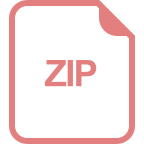
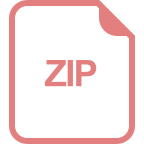
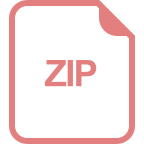
收起资源包目录




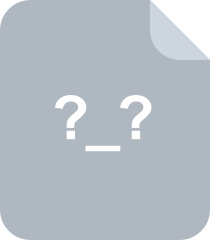
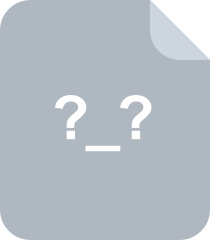
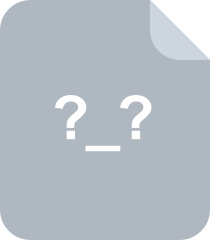
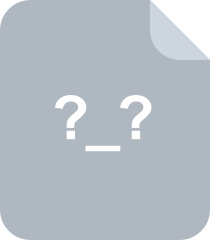

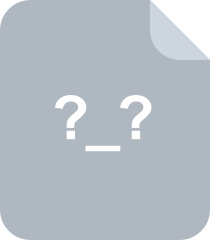
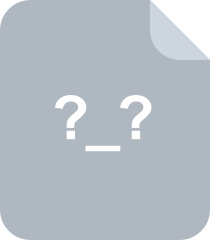
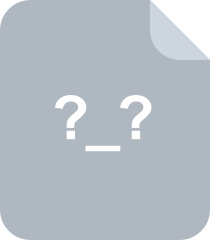
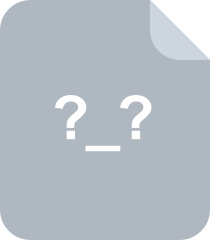

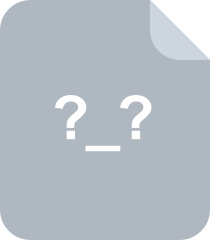
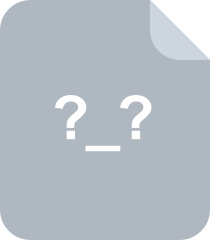
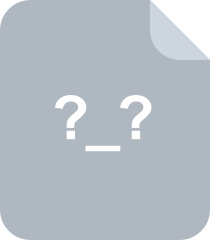
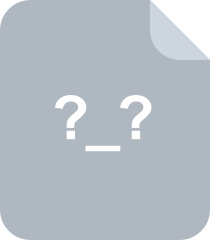
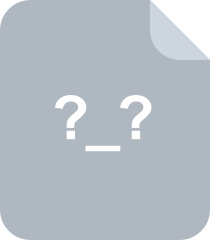
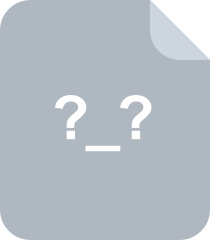
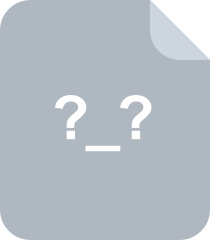
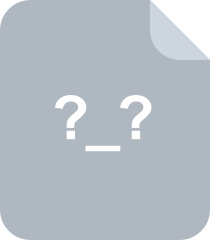



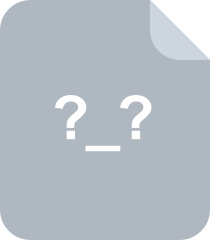
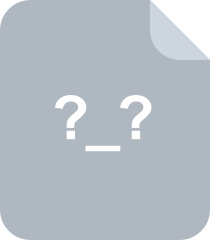
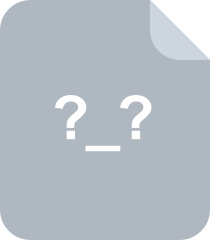
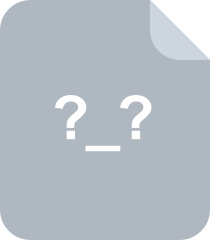

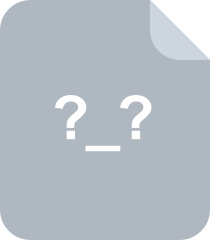
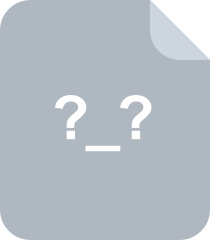
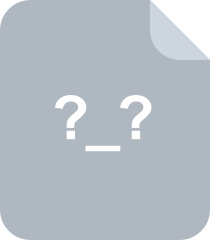
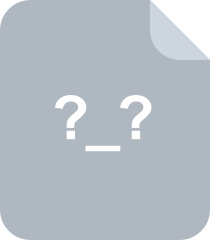

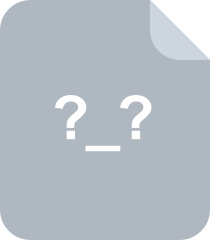
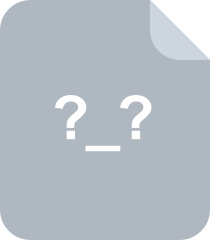
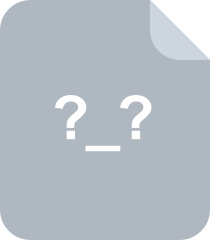
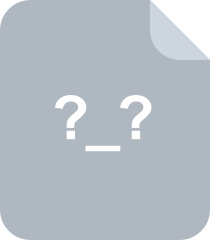
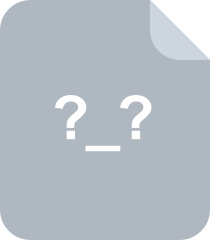
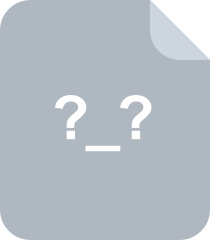
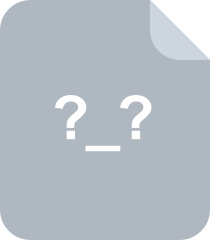
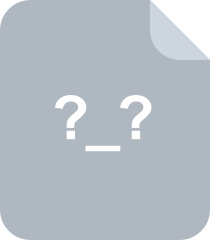
共 32 条
- 1
资源评论
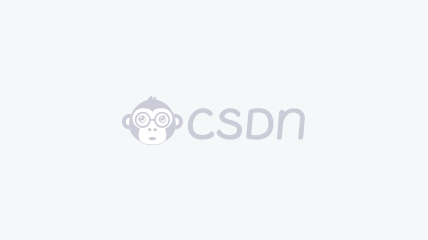
- zyu672018-01-10:( Call to undefined function Qiniu\json_decode() 错误位置 FILE: /home/wwwroot/17vs/Application/Tool/Qiniu/Http/Response.class.php LINE: 133 出现这个错误了呀

编程技术初学者
- 粉丝: 17
- 资源: 3
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

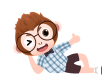
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


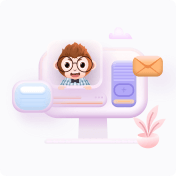
安全验证
文档复制为VIP权益,开通VIP直接复制
