# Stripe PHP bindings
[](https://github.com/stripe/stripe-php/actions?query=branch%3Amaster)
[](https://packagist.org/packages/stripe/stripe-php)
[](https://packagist.org/packages/stripe/stripe-php)
[](https://packagist.org/packages/stripe/stripe-php)
[](https://coveralls.io/r/stripe/stripe-php?branch=master)
The Stripe PHP library provides convenient access to the Stripe API from
applications written in the PHP language. It includes a pre-defined set of
classes for API resources that initialize themselves dynamically from API
responses which makes it compatible with a wide range of versions of the Stripe
API.
## Requirements
PHP 5.6.0 and later.
## Composer
You can install the bindings via [Composer](http://getcomposer.org/). Run the following command:
```bash
composer require stripe/stripe-php
```
To use the bindings, use Composer's [autoload](https://getcomposer.org/doc/01-basic-usage.md#autoloading):
```php
require_once 'vendor/autoload.php';
```
## Manual Installation
If you do not wish to use Composer, you can download the [latest release](https://github.com/stripe/stripe-php/releases). Then, to use the bindings, include the `init.php` file.
```php
require_once '/path/to/stripe-php/init.php';
```
## Dependencies
The bindings require the following extensions in order to work properly:
- [`curl`](https://secure.php.net/manual/en/book.curl.php), although you can use your own non-cURL client if you prefer
- [`json`](https://secure.php.net/manual/en/book.json.php)
- [`mbstring`](https://secure.php.net/manual/en/book.mbstring.php) (Multibyte String)
If you use Composer, these dependencies should be handled automatically. If you install manually, you'll want to make sure that these extensions are available.
## Getting Started
Simple usage looks like:
```php
$stripe = new \Stripe\StripeClient('sk_test_BQokikJOvBiI2HlWgH4olfQ2');
$customer = $stripe->customers->create([
'description' => 'example customer',
'email' => 'email@example.com',
'payment_method' => 'pm_card_visa',
]);
echo $customer;
```
### Client/service patterns vs legacy patterns
You can continue to use the legacy integration patterns used prior to version [7.33.0](https://github.com/stripe/stripe-php/blob/master/CHANGELOG.md#7330---2020-05-14). Review the [migration guide](https://github.com/stripe/stripe-php/wiki/Migration-to-StripeClient-and-services-in-7.33.0) for the backwards-compatible client/services pattern changes.
## Documentation
See the [PHP API docs](https://stripe.com/docs/api/?lang=php#intro).
See [video demonstrations][youtube-playlist] covering how to use the library.
## Legacy Version Support
### PHP 5.4 & 5.5
If you are using PHP 5.4 or 5.5, you should consider upgrading your environment as those versions have been past end of life since September 2015 and July 2016 respectively.
Otherwise, you can still use Stripe by downloading stripe-php v6.43.1 ([zip](https://github.com/stripe/stripe-php/archive/v6.43.1.zip), [tar.gz](https://github.com/stripe/stripe-php/archive/6.43.1.tar.gz)) from our [releases page](https://github.com/stripe/stripe-php/releases). This version will work but might not support recent features we added since the version was released and upgrading PHP is the best course of action.
### PHP 5.3
If you are using PHP 5.3, you should upgrade your environment as this version has been past end of life since August 2014.
Otherwise, you can download v5.9.2 ([zip](https://github.com/stripe/stripe-php/archive/v5.9.2.zip), [tar.gz](https://github.com/stripe/stripe-php/archive/v5.9.2.tar.gz)) from our [releases page](https://github.com/stripe/stripe-php/releases). This version will continue to work with new versions of the Stripe API for all common uses.
## Custom Request Timeouts
> **Note**
> We do not recommend decreasing the timeout for non-read-only calls (e.g. charge creation), since even if you locally timeout, the request on Stripe's side can still complete. If you are decreasing timeouts on these calls, make sure to use [idempotency tokens](https://stripe.com/docs/api/?lang=php#idempotent_requests) to avoid executing the same transaction twice as a result of timeout retry logic.
To modify request timeouts (connect or total, in seconds) you'll need to tell the API client to use a CurlClient other than its default. You'll set the timeouts in that CurlClient.
```php
// set up your tweaked Curl client
$curl = new \Stripe\HttpClient\CurlClient();
$curl->setTimeout(10); // default is \Stripe\HttpClient\CurlClient::DEFAULT_TIMEOUT
$curl->setConnectTimeout(5); // default is \Stripe\HttpClient\CurlClient::DEFAULT_CONNECT_TIMEOUT
echo $curl->getTimeout(); // 10
echo $curl->getConnectTimeout(); // 5
// tell Stripe to use the tweaked client
\Stripe\ApiRequestor::setHttpClient($curl);
// use the Stripe API client as you normally would
```
## Custom cURL Options (e.g. proxies)
Need to set a proxy for your requests? Pass in the requisite `CURLOPT_*` array to the CurlClient constructor, using the same syntax as `curl_stopt_array()`. This will set the default cURL options for each HTTP request made by the SDK, though many more common options (e.g. timeouts; see above on how to set those) will be overridden by the client even if set here.
```php
// set up your tweaked Curl client
$curl = new \Stripe\HttpClient\CurlClient([CURLOPT_PROXY => 'proxy.local:80']);
// tell Stripe to use the tweaked client
\Stripe\ApiRequestor::setHttpClient($curl);
```
Alternately, a callable can be passed to the CurlClient constructor that returns the above array based on request inputs. See `testDefaultOptions()` in `tests/CurlClientTest.php` for an example of this behavior. Note that the callable is called at the beginning of every API request, before the request is sent.
### Configuring a Logger
The library does minimal logging, but it can be configured
with a [`PSR-3` compatible logger][psr3] so that messages
end up there instead of `error_log`:
```php
\Stripe\Stripe::setLogger($logger);
```
### Accessing response data
You can access the data from the last API response on any object via `getLastResponse()`.
```php
$customer = $stripe->customers->create([
'description' => 'example customer',
]);
echo $customer->getLastResponse()->headers['Request-Id'];
```
### SSL / TLS compatibility issues
Stripe's API now requires that [all connections use TLS 1.2](https://stripe.com/blog/upgrading-tls). Some systems (most notably some older CentOS and RHEL versions) are capable of using TLS 1.2 but will use TLS 1.0 or 1.1 by default. In this case, you'd get an `invalid_request_error` with the following error message: "Stripe no longer supports API requests made with TLS 1.0. Please initiate HTTPS connections with TLS 1.2 or later. You can learn more about this at [https://stripe.com/blog/upgrading-tls](https://stripe.com/blog/upgrading-tls).".
The recommended course of action is to [upgrade your cURL and OpenSSL packages](https://support.stripe.com/questions/how-do-i-upgrade-my-stripe-integration-from-tls-1-0-to-tls-1-2#php) so that TLS 1.2 is used by default, but if that is not possible, you might be able to solve the issue by setting the `CURLOPT_SSLVERSION` option to either `CURL_SSLVERSION_TLSv1` or `CURL_SSLVERSION_TLSv1_2`:
```php
$curl = new \Stripe\HttpClient\CurlClient([CURLOPT_SSLVERSION => CURL_SSLVERSION_TLSv1]);
\Stripe\ApiRequestor::setHttpClient($curl);
```
### Per-request Configuration
For apps that need to use multiple keys during the lifetime of a process, like
one that uses [Stripe Connect][connect], it's also possible
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
浪子易支付源码优化缓存机制 更新日志 2023/06/30: 1.跳转微信客服支持多企业多客服轮询 2.新增黑名单列表,订单界面可一键添加 3.新商户订单支付成功率检测 4.新增通道订单连续失败检测(升级前先备份之前的黑名单列表) 2023/06/17: 1.新增H5页面跳转微信客服支付功能 2.优化缓存机制
资源推荐
资源详情
资源评论
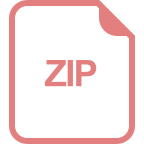
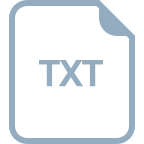
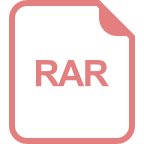
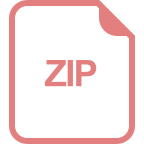
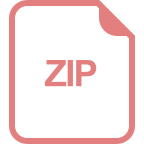
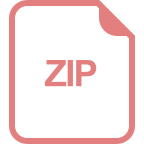
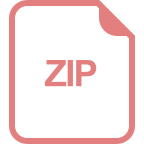
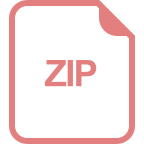
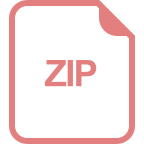
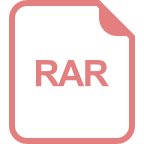
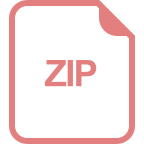
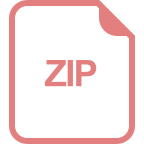
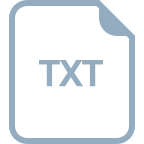
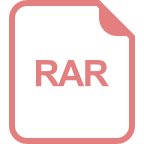
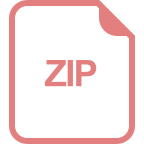
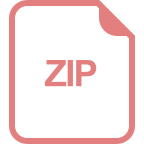
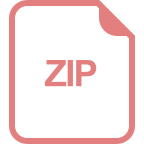
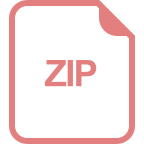
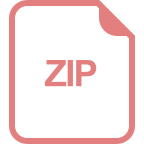
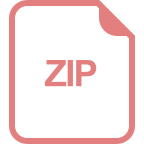
收起资源包目录

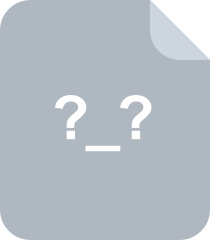
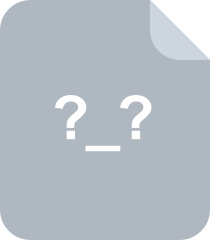
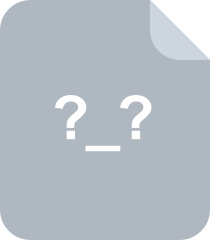
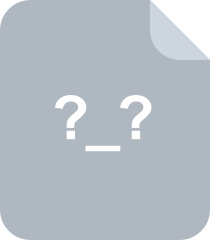
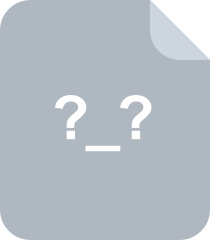
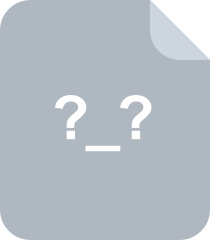
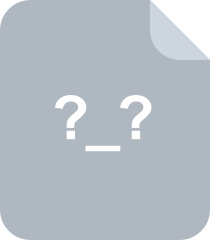
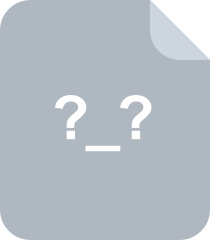
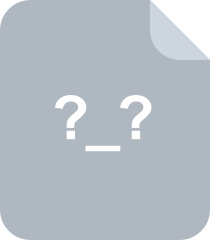
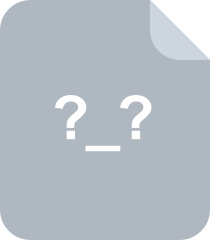
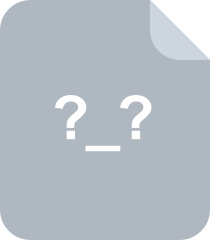
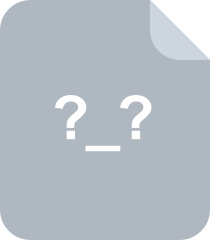
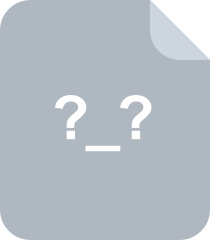
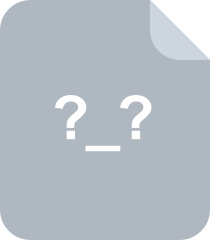
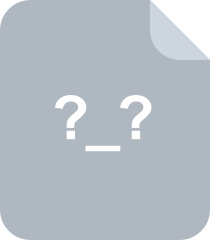
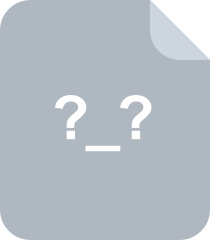
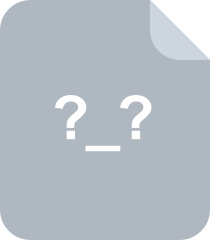
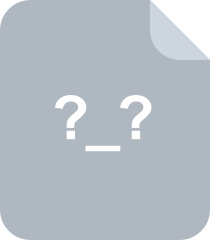
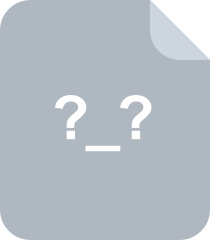
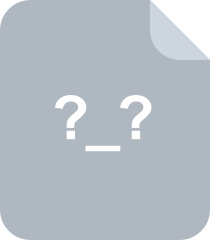
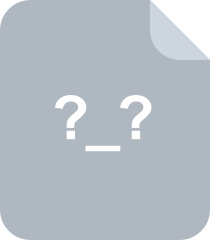
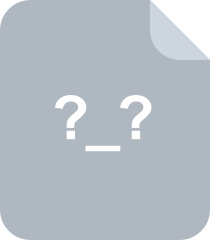
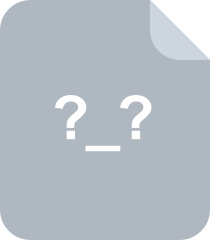
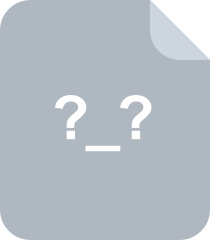
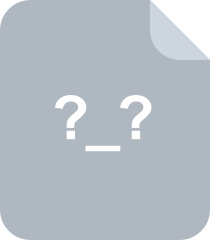
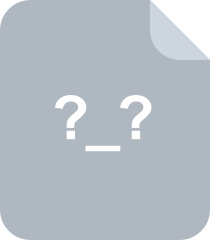
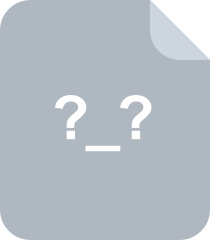
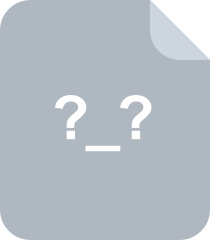
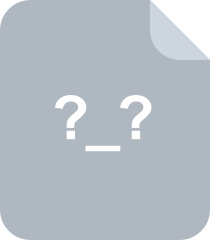
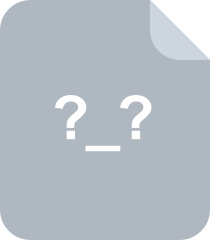
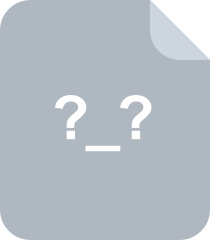
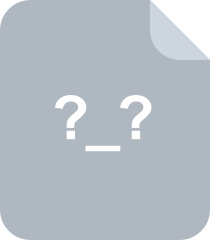
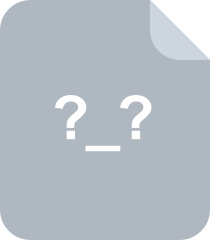
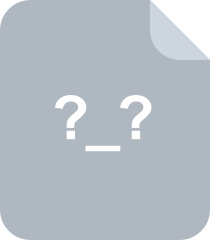
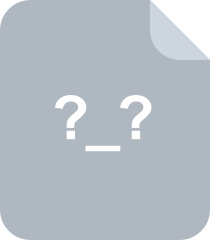
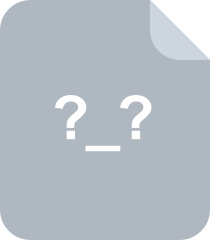
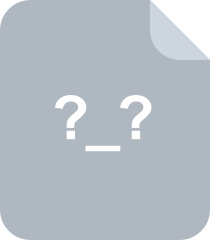
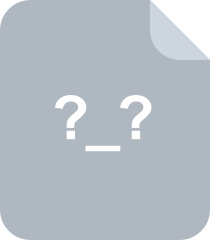
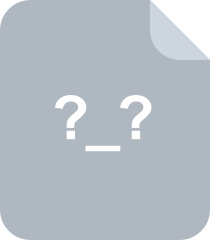
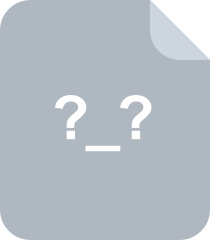
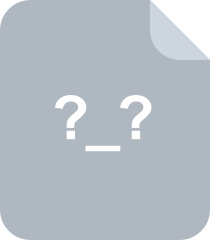
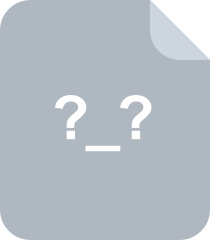
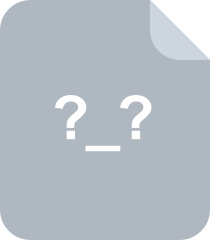
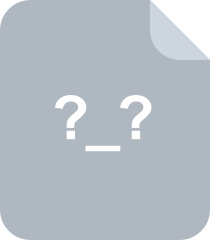
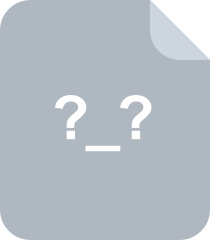
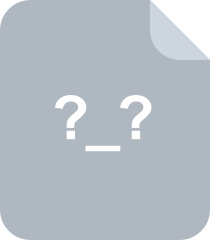
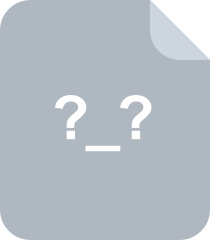
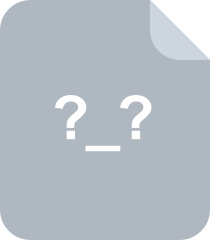
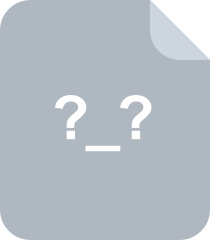
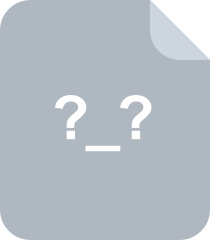
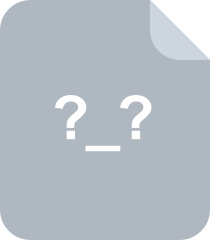
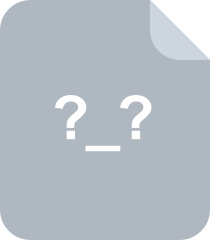
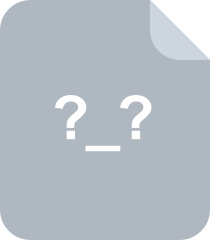
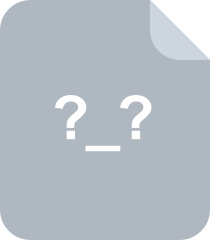
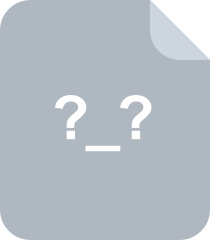
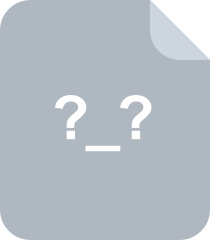
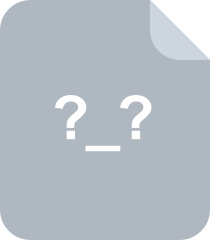
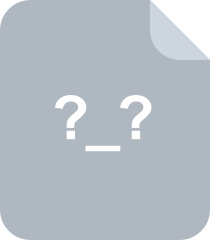
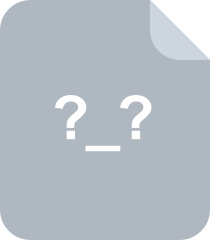
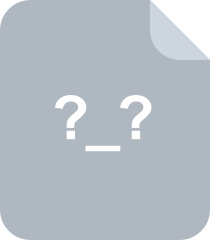
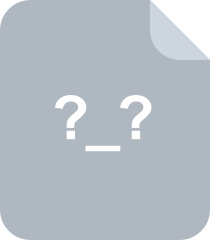
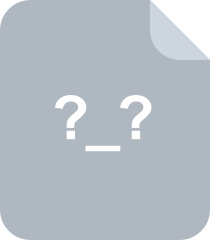
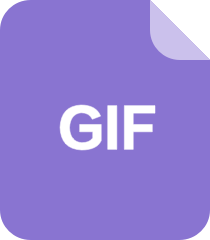
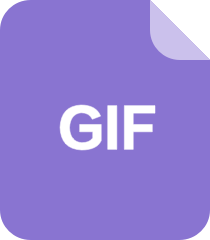
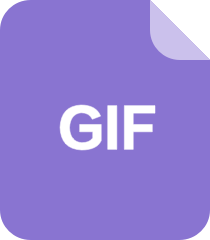
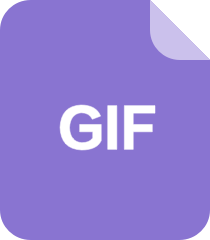
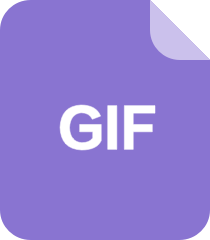
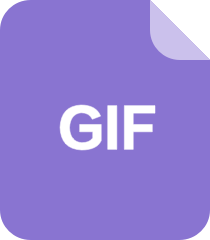
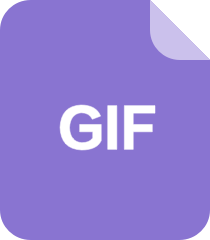
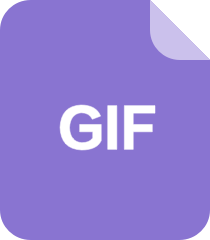
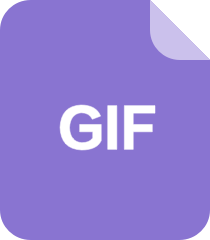
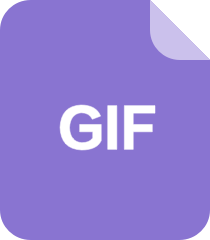
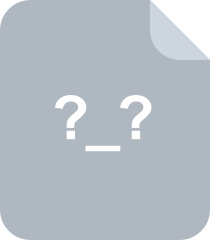
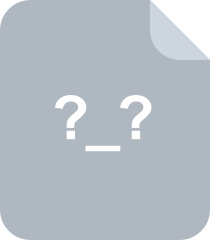
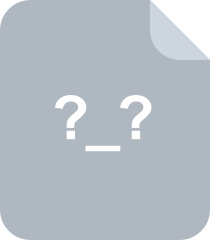
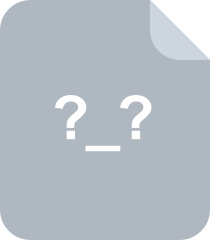
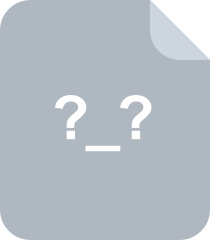
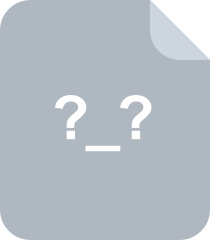
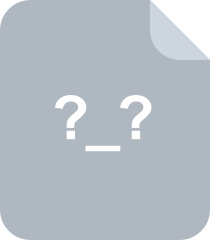
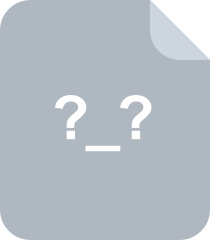
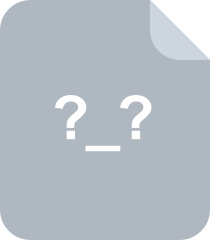
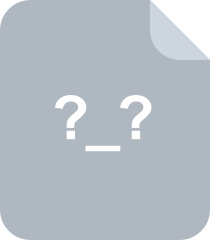
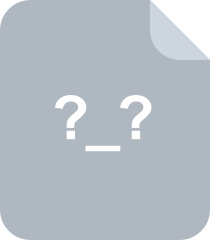
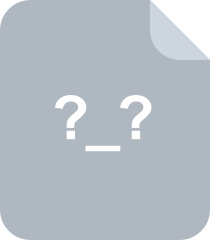
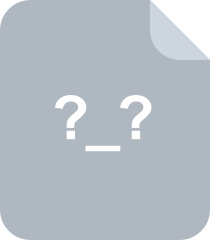
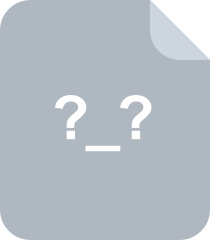
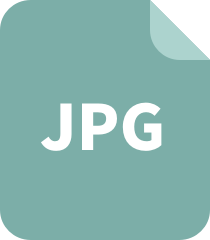
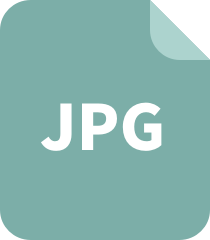
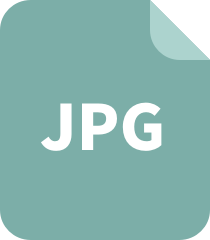
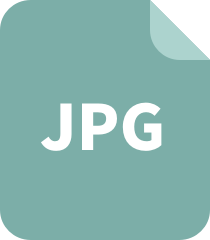
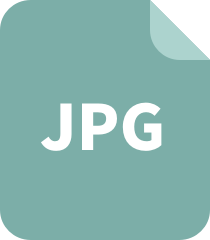
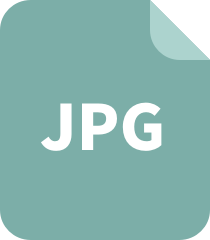
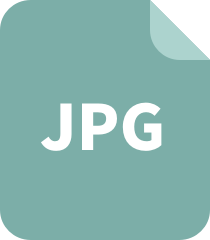
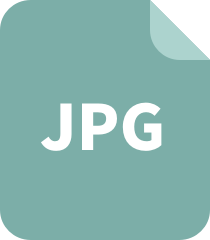
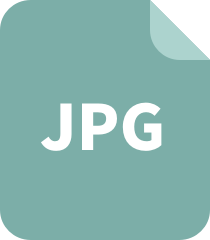
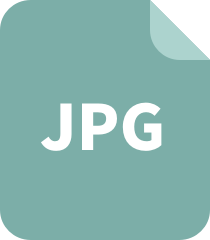
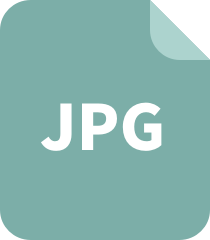
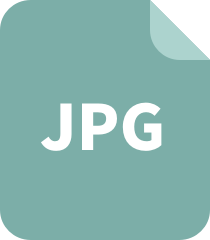
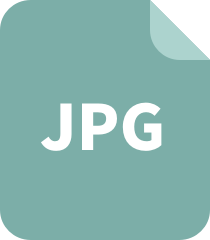
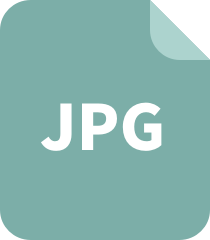
共 1320 条
- 1
- 2
- 3
- 4
- 5
- 6
- 14
资源评论
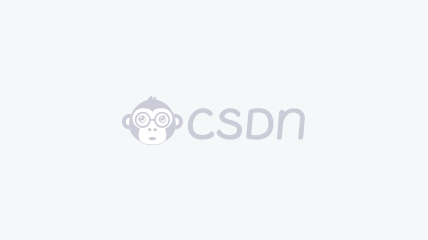

小正太浩二
- 粉丝: 238
- 资源: 5943
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

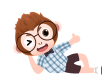
最新资源
- baby-llama2-chinese
- 个人毕业设计-基于Hadoop的校园资源云存储的设计与开发.zip
- MicrosoftEdgeEnterpriseX64(Edge浏览器)
- 个人毕业设计 健身房管理系统 java后端.zip
- 绝缘体检测66-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord、VOC数据集合集.rar
- 个人毕业设计 - 基于树莓派、OpenCV及Python语言的人脸识别.zip
- 个人毕业设计01234.zip
- 基于python的高考志愿填报系统源码+数据库+项目说明(高分项目)
- H矩阵 深度学习 python
- 个人博客系统毕业设计.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


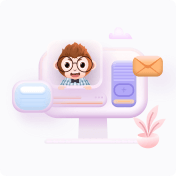
安全验证
文档复制为VIP权益,开通VIP直接复制
