#include <windows.h>
#include <tchar.h>
#pragma comment(lib, "winmm.lib") //调用PlaySound函数所需库文件t
#pragma comment(lib, "Msimg32.lib")
#define WINDOW_WIDTH 500
#define WINDOW_HEIGHT 700
#define WINDOW_TITLE L"飞机大战"
const int SceneNum = 5; //关卡数
const int BulletSpeed = 10; //子弹速度
const int GoodsSpeed = 6; //道具速度
const int BulletNum = 3; //子弹种类
const int LaserNum = 2; //激光种类
const int MaxEnemyCount = 30; //最大敌机数目
const int MaxBulletCount = 100; //最大子弹数目
const int MaxLaserCount = 20; //最大激光数目
const int MaxExplosionCount = 40; //最大特效数目
const int MaxBuffTime = 30; //最大双倍弹药时间
const int MaxProtectedTime = 30; //最大防护罩时间
const int BossCreateTime = 1; //boss创建时间
const int BackGroundSpeed = 5; //背景滚动速度
//玩家弹道间隔距离数组(单倍,双倍弹药)
const int IntervalP[2][10] =
{
{19,38},
{0, 19, 38, 57}
};
//敌机、Boss弹道间隔距离数组
const int IntervalE[6][10] =
{
{15,65},
{35,70,110},
{15,45,90,120},
{12,100},
{15,60,105,150,195,240},
{16}
};
//飞机
struct Plane
{
int x, y; //位置
int hp, maxHp; //生命值,最大生命值
int w, h; //宽度与长度
int hBullet; //子弹
int hLaser; //激光
int LaserNum; //激光数目
int ProtectedNum; //防护罩数目
bool Protected; //是否处于防护状态
bool exist; //是否存在
int wait, waitTime; //发射经过时间,发射间隔时间
int motionX, motionY; //每帧移动距离
int speed; //速度
bool buff; //是否有双倍弹药状态
int BulletNum; //同时发射子弹数目
const int *Interval; //弹道距离数组
//初始化
void Init(int _w, int _h, int _hBullet, int _hLaser,int _waitTime,
int _motionX, int _motionY, int _maxHp, int _BulletNum, const int *_Interval)
{
w = _w;
h = _h;
hBullet = _hBullet;
hLaser = _hLaser;
waitTime = _waitTime;
motionX = _motionX;
motionY = _motionY;
exist = false;
maxHp = _maxHp;
BulletNum = _BulletNum;
Interval = _Interval;
}
//创建飞机
void Create(int _x, int _y, int _speed)
{
x = _x;
y = _y;
hp = maxHp;
exist = true;
speed = _speed;
buff = false;
Protected = false;
LaserNum = 0;
ProtectedNum = 0;
Protected = false;
wait = 0;
}
};
//游戏对象(道具,子弹)
struct Objects
{
int x, y; //位置
int w, h; //宽度与长度
int motionY; //每帧移动距离
int power; //伤害
bool exist; //是否存在
//初始化
void Init(int _w, int _h, int _motionY, int _power)
{
w = _w;
h = _h;
motionY = _motionY;
exist = false;
power = _power;
}
//创建对象
void Create(int _x, int _y)
{
x = _x;
y = _y;
exist = true;
}
};
//爆炸特效
struct Explosion
{
int x, y; //位置
int w, h; //宽度与长度
int iNum; //爆炸图片索引
bool exist; //是否存在
//初始化
void Init(int _w, int _h)
{
w = _w;
h = _h;
exist = false;
}
//创建对象
void Create(int _x, int _y)
{
iNum = 0;
x = _x;
y = _y;
exist = true;
}
};
HINSTANCE hInst;
HDC hdc, mdc, bufdc;
bool start, over, super, pause; //是否开始,结束,无敌模式,暂停
int scene, score; //场景数,分数
HBITMAP hStart, hOver, hScore[SceneNum], hScene[SceneNum], hBullet[BulletNum], hExplosion, hGetHp, hPlus,
hGetLaser, hGetBuff, hGetProtect, hLaser[LaserNum], hPlayer, hProtect, hBoss[SceneNum], hEnemy, hReturn;
HFONT hFont[2];
HBRUSH hBrush;
DWORD g_tNow, g_tPre;
HWND hwnd;
Plane player, enemy[MaxEnemyCount], boss[SceneNum]; //玩家,敌机,boss
//子弹,激光,游戏道具(恢复生命,双倍弹药,防护罩,激光武器)
Objects bullet[BulletNum][MaxBulletCount], laser[LaserNum][MaxLaserCount], getHp, getBuff, getProtect, getLaser;
Explosion explosion[MaxExplosionCount]; //爆炸特效
int g_iBGOffset; //背景滚动量
int ProtectedTime, BuffTime, BossTime; //防护罩、双倍弹药存在时间,Boss未产生时间
RECT rect; //获取客户区窗口
int MyWindowClass(HINSTANCE);
BOOL InitInstance(HINSTANCE, int);
LRESULT CALLBACK WndProc(HWND, UINT, WPARAM, LPARAM);
void PlaneGame(HWND);
void MyDraw(HDC);
void StartGame();
void OverGame();
void Clear();
void AI_Motion();
void AI_Create();
void AI_Fire(Plane &);
void CollisionDetection();
void CreateExplosion(int, int);
bool isCollision(int, int, int, int, int, int, int, int);
void LoadMusic();
/*****************************************************************************************************************
在不同的应用程序中,在此处添加相关的全局变量
******************************************************************************************************************/
int APIENTRY WinMain(HINSTANCE hInstance, HINSTANCE hPreInstace,
LPSTR lpCmdLine, int nCmdShow)
{
MyWindowClass(hInstance);
if(!InitInstance(hInstance, nCmdShow))
return FALSE;
MSG msg = {0}; //初始化
while(msg.message != WM_QUIT)
{
//检测目前是否有需要处理的消息,有返回非0,否则返回0
if(PeekMessage(&msg, NULL, 0, 0, PM_REMOVE))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
else
{
g_tNow = GetTickCount(); //获取当前系统时间
if(g_tNow - g_tPre >= 40) //当此次循环运行与上次绘图时间相差0.1s进行重绘操作
{
PlaneGame(hwnd);
g_tPre = GetTickCount(); //记录此次绘图时间,供下次游戏循环中判断是否达到画面更新操作
}
}
}
return msg.wParam;
}
int MyWindowClass(HINSTANCE hInstance)
{
WNDCLASSEX wcex;
wcex.cbSize = sizeof(WNDCLASSEX);
wcex.style = CS_HREDRAW | CS_VREDRAW;
wcex.lpfnWndProc = WndProc;
wcex.cbClsExtra = 0;
wcex.cbWndExtra = 0;
wcex.hInstance = hInstance;
wcex.hIcon = (HICON)LoadImage(hInstance, L"Textures\\icon.ico", IMAGE_ICON, 0, 0, LR_DEFAULTSIZE|LR_LOADFROMFILE);
wcex.hCursor = LoadCursor(NULL, IDC_ARROW);
wcex.hbrBackground = (HBRUSH)(COLOR_WINDOW+1);
wcex.lpszMenuName = NULL;
wcex.lpszClassName = L"gamebase";
wcex.hIconSm = NULL;
return RegisterClassEx(&wcex);
}
BOOL InitInstance(HINSTANCE hInstance, int nCmdShow)
{
hInst = hInstance;
hwnd = CreateWindow(L"gamebase", WINDOW_TITLE, WS_OVERLAPPEDWINDOW,
CW_USEDEFAULT, CW_USEDEFAULT, WINDOW_WIDTH, WINDOW_HEIGHT, NULL, NULL, hInstance, NULL);
if(!hwnd)
return FALSE;
MoveWindow(hwnd, 400, 10, WINDOW_WIDTH, WINDOW_HEIGHT, true);
ShowWindow(hwnd, nCmdShow);
UpdateWindow(hwnd);
LoadMusic(); //加载音乐资源
mciSendString(L"play bgm repeat", NULL, 0, NULL); //重复播放背景音乐
hdc = GetDC(hwnd);
mdc = CreateCompatibleDC(hdc);
bufdc = CreateCompatibleDC(hdc);
//位图的加载
wchar_t filename[50] = {};
hStart = (HBITMAP)LoadImage(NULL, L"Textures\\start.bmp", IMAGE_BITMAP, WINDOW_WIDTH, WINDOW_HEIGHT, LR_LOADFROMFILE); //开始界面
hOver = (HBITMAP)LoadImage(NULL, L"Textures\\over.bmp", IMAGE_BITMAP, WINDOW_WIDTH, WINDOW_HEIGHT, LR_LOADFROMFILE); //结束界面
hBoss[0] = (HBITMAP)LoadImage(NULL, L"Textures\\boss0.bmp", IMAGE_BITMAP, 88, 50, LR_LOADFROMFILE); //boss
hBoss[1] = (HBITMAP)LoadImage(NULL, L"Textures\\boss1.bmp", IMAGE_BITMAP, 151, 94, LR_LOADFROMFILE);
hBoss[2] = (HBITMAP)LoadImage(NULL, L"Textures\\boss2.bmp", IMAGE_BITMAP, 134, 91, LR_LOADFROMFILE);
hBoss[3] = (HBITMAP)LoadImage(NULL, L"Textures\\boss3.bmp", IMAGE_BITMAP, 126, 106, LR_LOADFROMFILE);
hBoss[4] = (HBITMAP)LoadImage(NULL, L"Textures\\boss4.bmp", IMAGE_BITMAP, 260, 197, LR_LOADFROMFILE);
hBullet[0] = (HBITMAP)LoadImage(NULL, L"Textures\\bullet0.bmp", IMAGE_BITMAP, 21, 50, LR_LOADFROMFILE); //子弹
hBullet[1] = (HBITMAP)Lo
没有合适的资源?快使用搜索试试~ 我知道了~
飞机大战-Win32程序开发
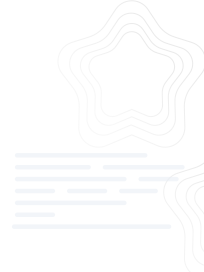
共71个文件
bmp:34个
tlog:11个
mp3:4个

需积分: 16 9 下载量 26 浏览量
2018-12-25
14:14:24
上传
评论 4
收藏 25.31MB ZIP 举报
温馨提示
运行环境:vs2010 游戏多个关卡,多个boss,多种道具,可玩行还可以吧。跟之前的差不太多。 此游戏为飞机大战,胜利条件为玩家消灭最终boss,失败条件为玩家被消灭。 游戏玩法: 开始界面:点击鼠标进入普通模式,按下空格键进入无敌模式,按下Esc键退出游戏。 游戏界面:玩家鼠标控制飞机的移动与射击,A键发射激光,S键使用防护罩,按下Esc键退出游戏,P键暂停。 --------------------- 作者:辣条不爱辣 来源:CSDN 原文:https://blog.csdn.net/baidu_38304645/article/details/82836535 版权声明:本文为博主原创文章,转载请附上博文链接!
资源推荐
资源详情
资源评论
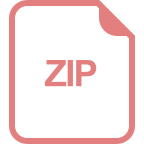
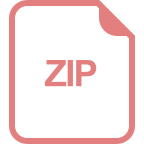
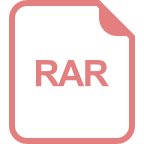
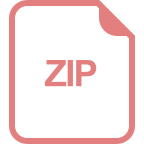
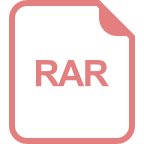
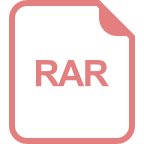
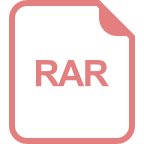
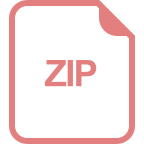
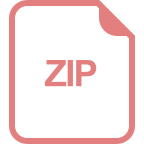
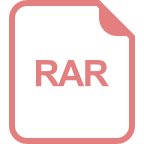
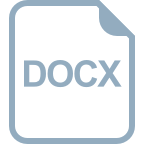
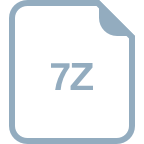
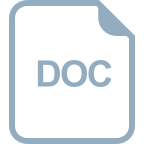
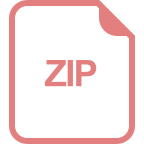
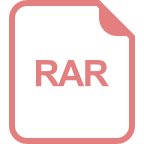
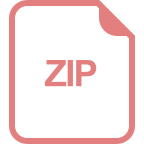
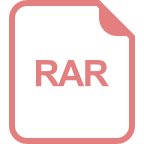
收起资源包目录


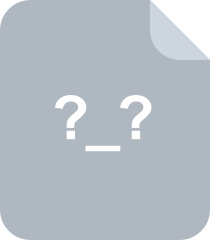
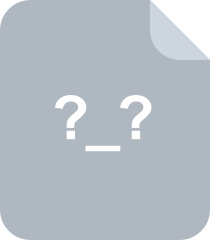
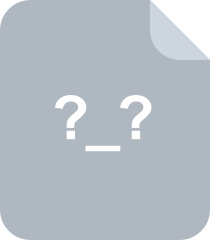

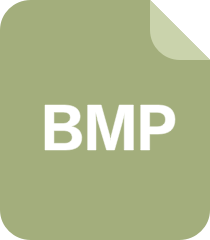
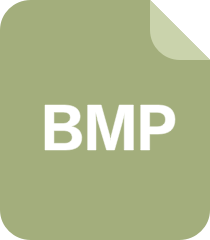
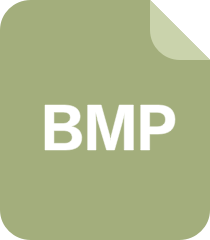
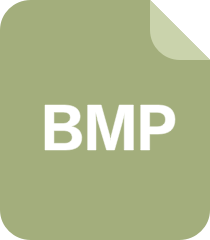
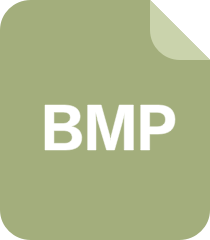
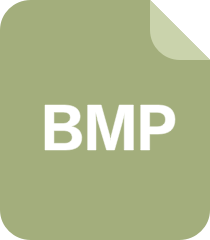
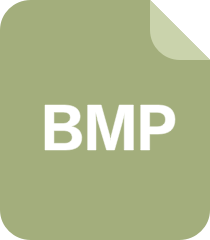
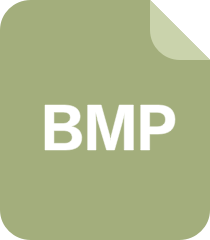
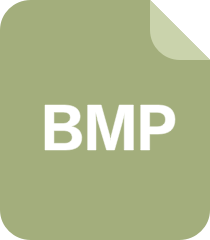
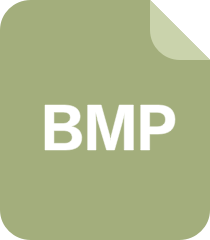
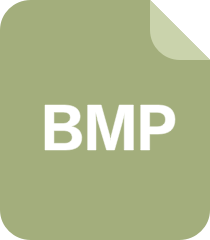
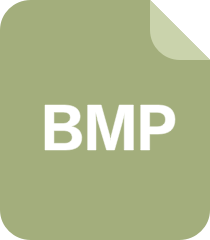
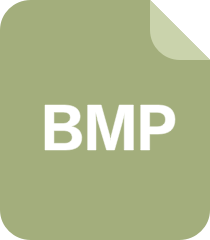
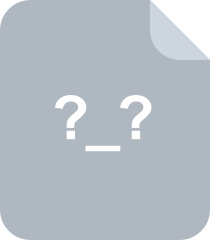
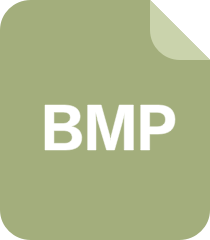
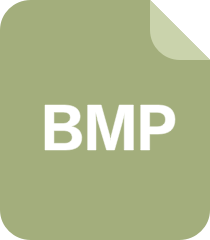
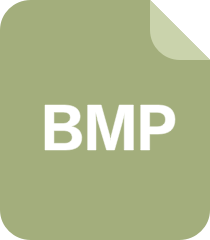
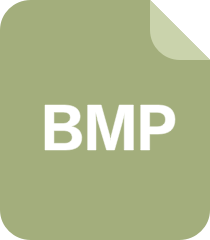
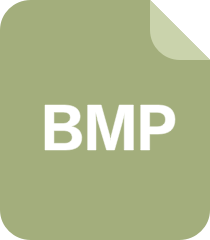
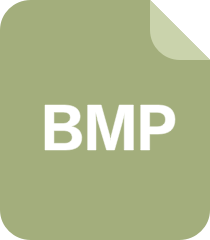
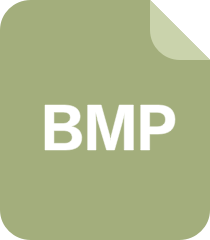
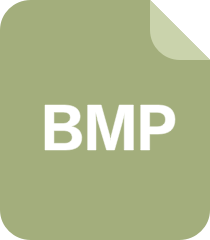
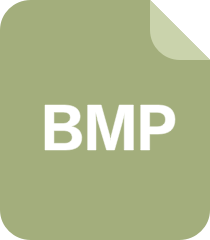
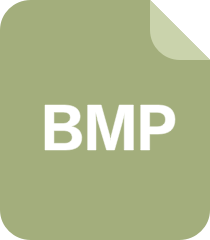
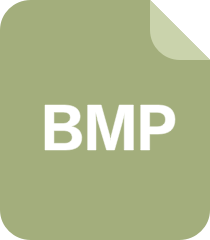
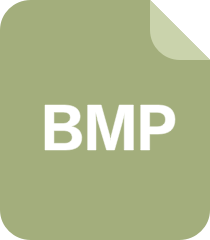
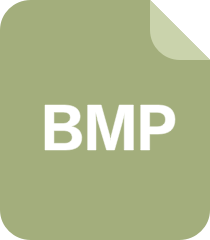
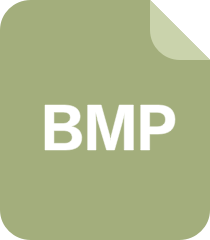
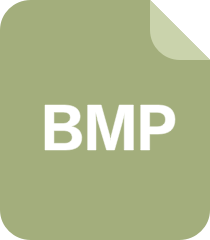
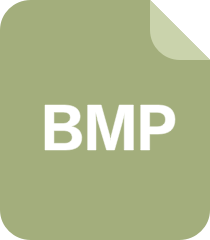
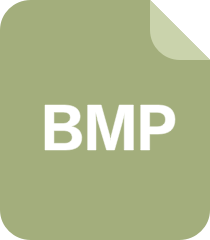
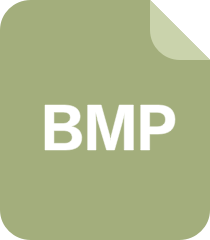
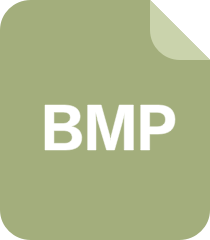
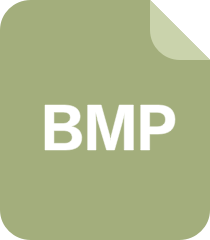
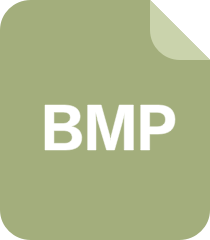

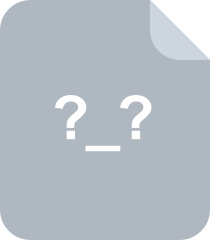
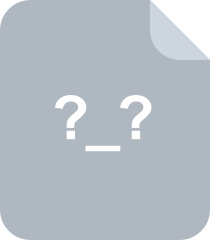
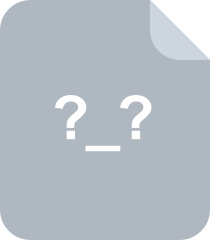
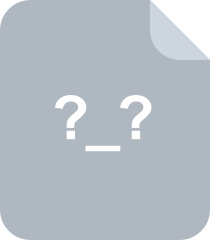
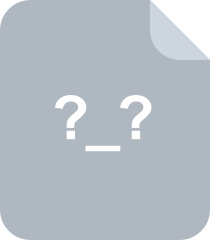
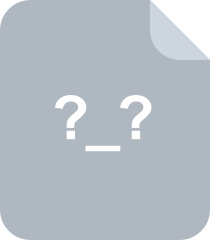
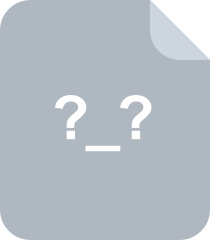

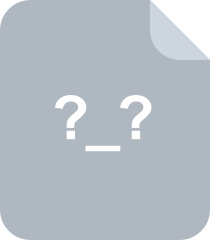
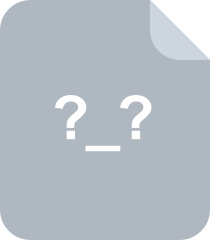
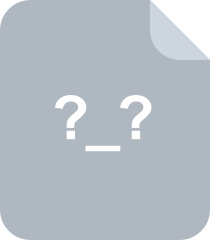
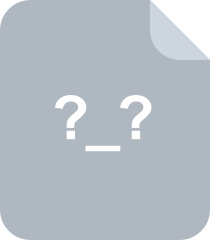
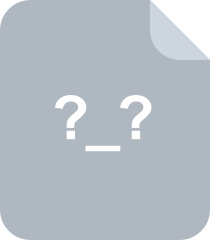
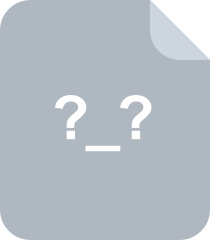
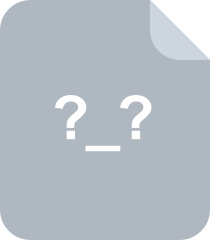
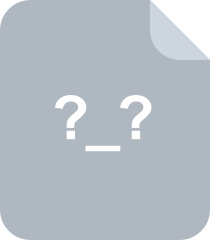
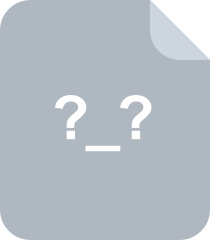
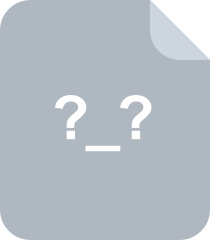
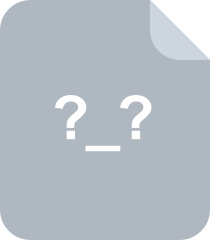
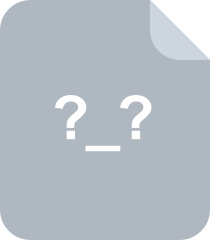
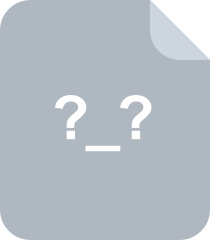
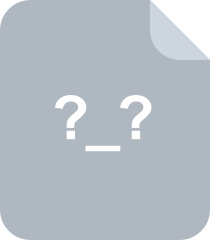
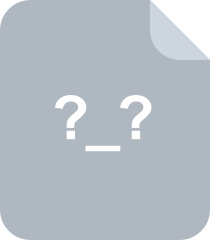
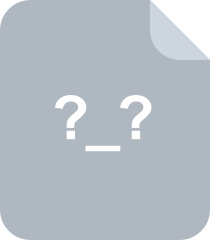
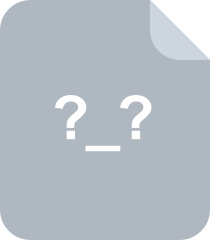
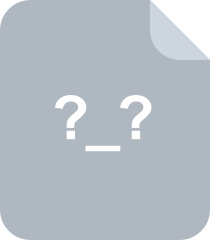
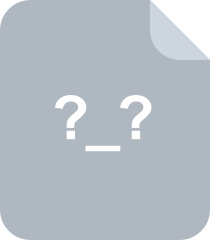
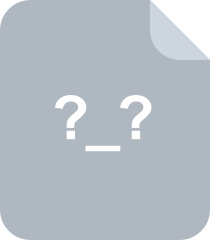
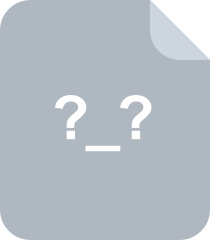


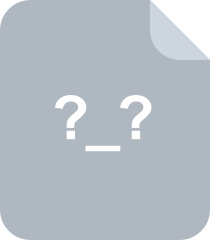

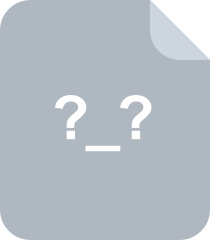
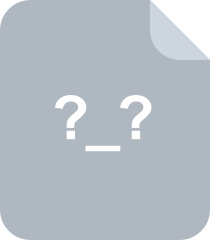
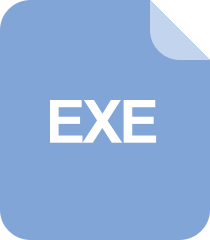
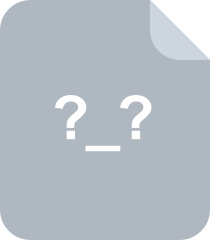
共 71 条
- 1
资源评论
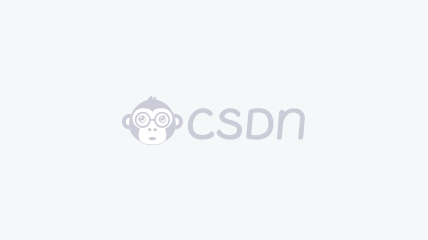

辣条不爱辣
- 粉丝: 148
- 资源: 3
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

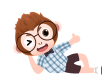
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


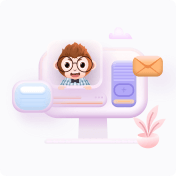
安全验证
文档复制为VIP权益,开通VIP直接复制
