package com.controller;
import java.util.ArrayList;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import com.entity.Article;
import com.entity.Bbs;
import com.entity.Cart;
import com.entity.Cate;
import com.entity.Complains;
import com.entity.Goods;
import com.entity.Orders;
import com.entity.Rebbs;
import com.entity.Seller;
import com.entity.Topic;
import com.entity.Users;
import com.service.ArticleService;
import com.service.BbsService;
import com.service.CartService;
import com.service.CateService;
import com.service.ComplainsService;
import com.service.GoodsService;
import com.service.OrdersService;
import com.service.RebbsService;
import com.service.SellerService;
import com.service.TopicService;
import com.service.UsersService;
import com.util.PageHelper;
import com.util.VeDate;
//定义为控制器
@Controller
// 设置路径
@RequestMapping("/index")
public class IndexController extends BaseController {
@Autowired
private UsersService usersService;
@Autowired
private SellerService sellerService;
@Autowired
private ArticleService articleService;
@Autowired
private CateService cateService;
@Autowired
private GoodsService goodsService;
@Autowired
private CartService cartService;
@Autowired
private OrdersService ordersService;
@Autowired
private TopicService topicService;
@Autowired
private ComplainsService complainsService;
@Autowired
private BbsService bbsService;
@Autowired
private RebbsService rebbsService;
// 公共方法 提供公共查询数据
private void front() {
this.getRequest().setAttribute("title", "学校食堂管理系统");
List<Cate> cateList = this.cateService.getAllCate();
this.getRequest().setAttribute("cateList", cateList);
List<Goods> hotList = this.goodsService.getGoodsByHot();
this.getRequest().setAttribute("hotList", hotList);
}
// 首页显示
@RequestMapping("index.action")
public String index() {
this.front();
List<Cate> cateList = this.cateService.getCateFront();
List<Cate> frontList = new ArrayList<Cate>();
for (Cate cate : cateList) {
List<Goods> goodsList = this.goodsService.getGoodsByCate(cate.getCateid());
cate.setGoodsList(goodsList);
frontList.add(cate);
}
this.getRequest().setAttribute("frontList", frontList);
return "users/index";
}
// 公告
@RequestMapping("article.action")
public String article(String number) {
this.front();
List<Article> tempList = this.articleService.getAllArticle();
PageHelper.getIndexPage(tempList, "article", "article", null, 10, number, this.getRequest());
return "users/article";
}
// 阅读公告
@RequestMapping("read.action")
public String read(String id) {
this.front();
Article article = this.articleService.getArticleById(id);
article.setHits("" + (Integer.parseInt(article.getHits()) + 1));
this.articleService.updateArticle(article);
this.getRequest().setAttribute("article", article);
return "users/read";
}
// 准备登录
@RequestMapping("preLogin.action")
public String prelogin() {
this.front();
return "users/login";
}
// 用户登录
@RequestMapping("login.action")
public String login() {
this.front();
String username = this.getRequest().getParameter("username");
String password = this.getRequest().getParameter("password");
Users u = new Users();
u.setUsername(username);
List<Users> usersList = this.usersService.getUsersByCond(u);
if (usersList.size() == 0) {
this.getSession().setAttribute("message", "用户名不存在");
return "redirect:/index/preLogin.action";
} else {
Users users = usersList.get(0);
if ("锁定".equals(users.getStatus())) {
this.getSession().setAttribute("message", "账户被锁定");
return "redirect:/index/preLogin.action";
}
if (password.equals(users.getPassword())) {
this.getSession().setAttribute("userid", users.getUsersid());
this.getSession().setAttribute("username", users.getUsername());
this.getSession().setAttribute("users", users);
return "redirect:/index/index.action";
} else {
this.getSession().setAttribute("message", "密码错误");
return "redirect:/index/preLogin.action";
}
}
}
// 商家准备注册
@RequestMapping("preRegSeller.action")
public String preRegSeller() {
this.front();
return "users/sellerregister";
}
@RequestMapping("sellerregister.action")
public String sellerregister(Seller seller) {
this.front();
Seller u = new Seller();
u.setUsername(seller.getUsername());
List<Seller> usersList = this.sellerService.getSellerByCond(u);
if (usersList.size() == 0) {
seller.setStatus("锁定");
seller.setRegdate(VeDate.getStringDateShort());
this.sellerService.insertSeller(seller);
} else {
this.getSession().setAttribute("message", "用户名已存在");
return "redirect:/index/preRegSeller.action";
}
return "redirect:/seller/index.action";
}
// 准备注册
@RequestMapping("preReg.action")
public String preReg() {
this.front();
return "users/register";
}
// 用户注册
@RequestMapping("register.action")
public String register(Users users) {
this.front();
Users u = new Users();
u.setUsername(users.getUsername());
List<Users> usersList = this.usersService.getUsersByCond(u);
if (usersList.size() == 0) {
users.setStatus("锁定");
users.setRegdate(VeDate.getStringDateShort());
this.usersService.insertUsers(users);
} else {
this.getSession().setAttribute("message", "用户名已存在");
return "redirect:/index/preReg.action";
}
return "redirect:/index/preLogin.action";
}
// 退出登录
@RequestMapping("exit.action")
public String exit() {
this.front();
this.getSession().removeAttribute("userid");
this.getSession().removeAttribute("username");
this.getSession().removeAttribute("users");
return "redirect:/index/index.action";
}
// 准备修改密码
@RequestMapping("prePwd.action")
public String prePwd() {
this.front();
if (this.getSession().getAttribute("userid") == null) {
return "redirect:/index/preLogin.action";
}
return "users/editpwd";
}
// 修改密码
@RequestMapping("editpwd.action")
public String editpwd() {
this.front();
if (this.getSession().getAttribute("userid") == null) {
return "redirect:/index/preLogin.action";
}
String userid = (String) this.getSession().getAttribute("userid");
String password = this.getRequest().getParameter("password");
String repassword = this.getRequest().getParameter("repassword");
Users users = this.usersService.getUsersById(userid);
if (password.equals(users.getPassword())) {
users.setPassword(repassword);
this.usersService.updateUsers(users);
} else {
this.getSession().setAttribute("message", "旧密码错误");
return "redirect:/index/prePwd.action";
}
return "redirect:/index/prePwd.action";
}
@RequestMapping("usercenter.action")
public String usercenter() {
this.front();
if (this.getSession().getAttribute("userid") == null) {
return "redirect:/index/preLogin.action";
}
return "users/usercenter";
}
@RequestMapping("userinfo.action")
public String userinfo() {
this.front();
if (this.getSession().getAttribute("userid") == null) {
return "redirect:/index/preLogin.action";
}
String userid = (String) this.getSession().getAttribute("userid");
this.getSession().setAttribute("users", this.usersService.getUsersById(userid));
return "users/userinfo";
}
@RequestMapping("personal.action")
public String personal(Users users) {
this.front();
if (this.getSession().getAttribute("userid") == null) {
return "redirect:/index/preLogin.action";
}
this.usersService.updateUsers(users);
return "redirect:/index/userinfo.action";
}
// 留言板
@RequestMapping("bbs.action")
public String bbs() {
this.front();
List<Bbs> bbsList = this.bbsService.getAllBbs();
this.getReques
没有合适的资源?快使用搜索试试~ 我知道了~
基于的SSM校园食堂点餐系统源码+项目说明.zip
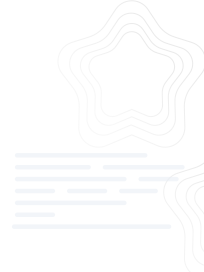
共1088个文件
gif:305个
js:237个
png:143个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 180 浏览量
2024-03-15
00:38:01
上传
评论
收藏 25.03MB ZIP 举报
温馨提示
【资源说明】 1、该资源包括项目的全部源码,下载可以直接使用! 2、本项目适合作为计算机、数学、电子信息等专业的课程设计、期末大作业和毕设项目,作为参考资料学习借鉴。 3、本资源作为“参考资料”如果需要实现其他功能,需要能看懂代码,并且热爱钻研,自行调试。 基于的SSM校园食堂点餐系统源码+项目说明.zip基于的SSM校园食堂点餐系统源码+项目说明.zip基于的SSM校园食堂点餐系统源码+项目说明.zip基于的SSM校园食堂点餐系统源码+项目说明.zip基于的SSM校园食堂点餐系统源码+项目说明.zip基于的SSM校园食堂点餐系统源码+项目说明.zip基于的SSM校园食堂点餐系统源码+项目说明.zip基于的SSM校园食堂点餐系统源码+项目说明.zip基于的SSM校园食堂点餐系统源码+项目说明.zip基于的SSM校园食堂点餐系统源码+项目说明.zip基于的SSM校园食堂点餐系统源码+项目说明.zip基于的SSM校园食堂点餐系统源码+项目说明.zip基于的SSM校园食堂点餐系统源码+项目说明.zip基于的SSM校园食堂点餐系统源码+项目说明.zip
资源推荐
资源详情
资源评论
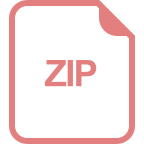
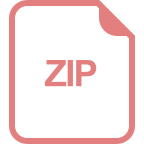
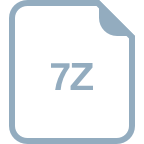
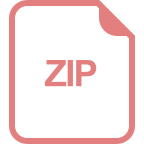
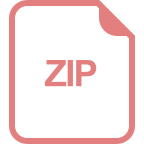
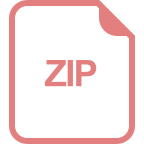
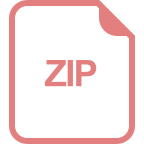
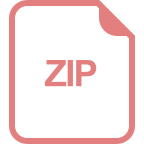
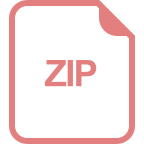
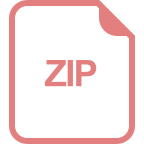
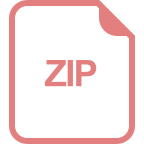
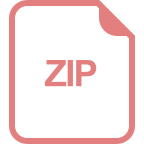
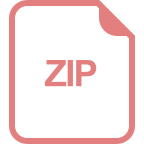
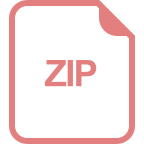
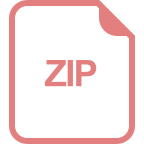
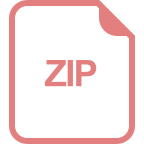
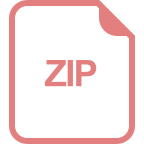
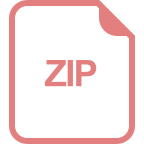
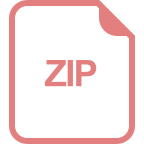
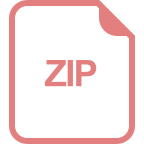
收起资源包目录

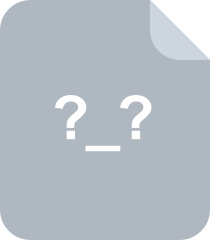
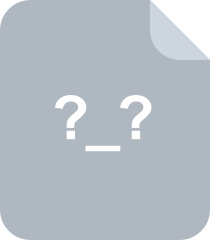
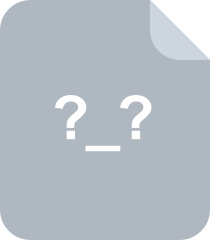
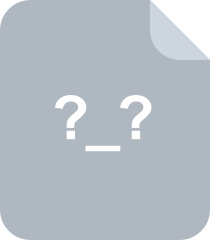
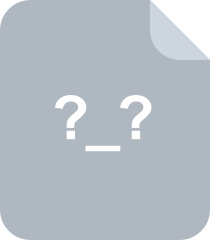
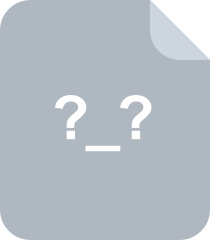
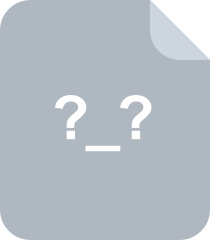
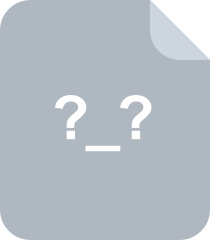
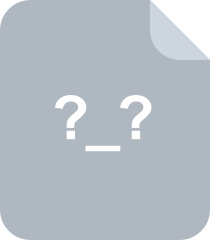
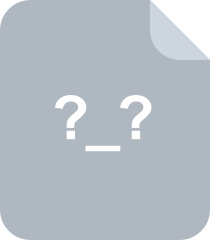
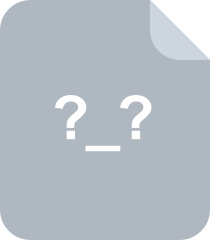
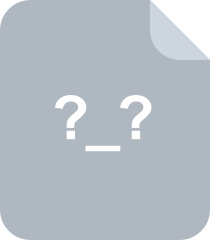
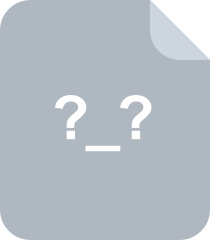
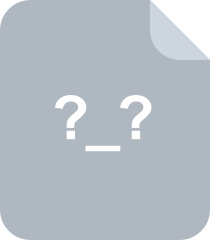
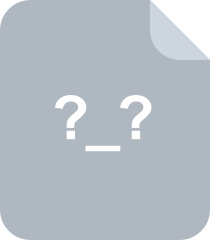
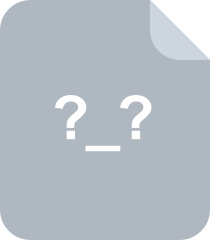
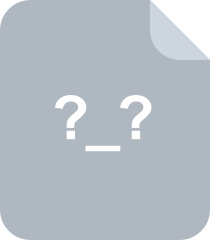
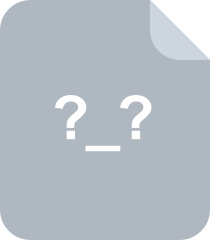
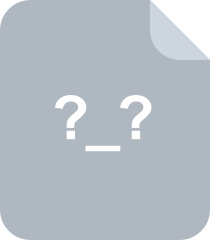
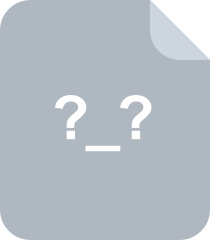
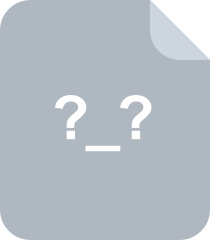
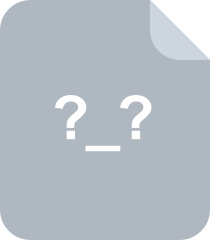
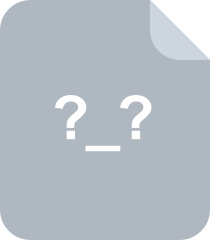
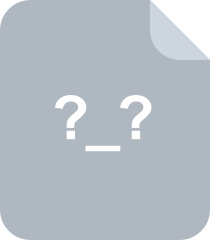
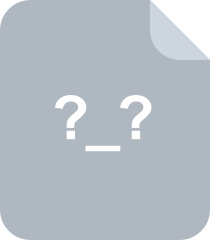
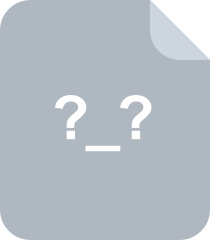
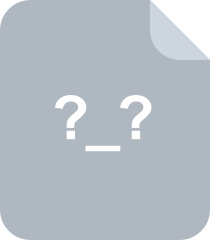
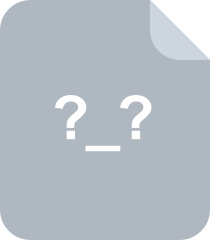
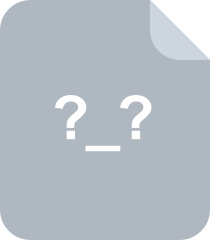
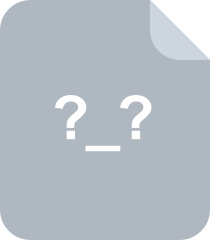
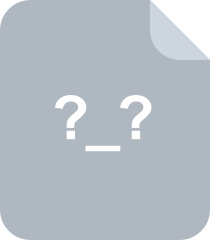
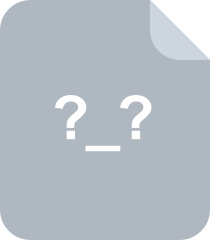
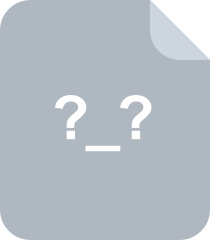
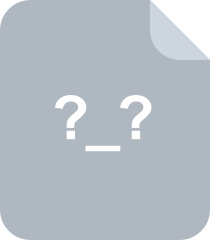
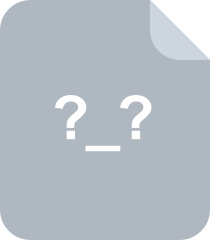
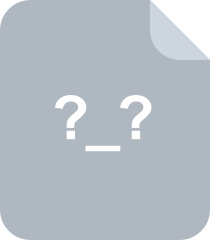
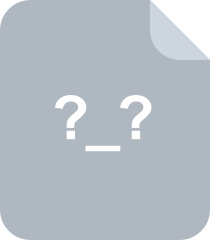
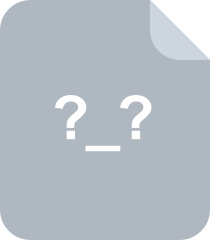
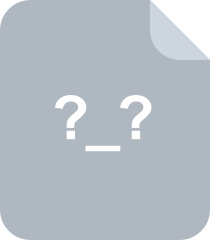
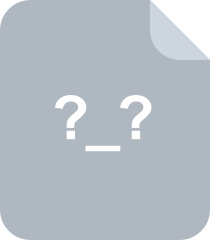
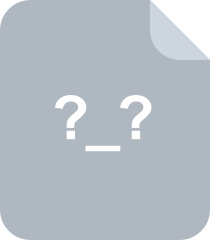
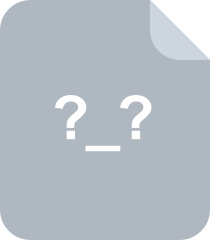
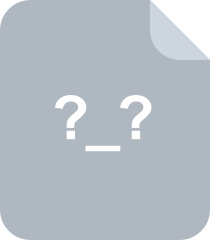
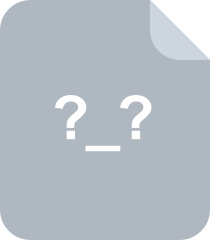
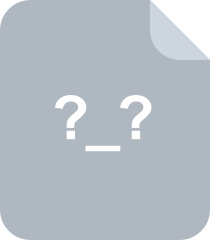
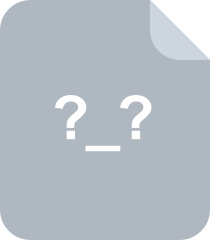
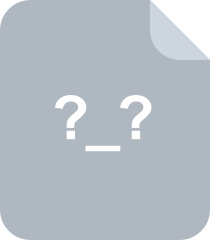
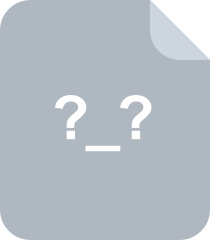
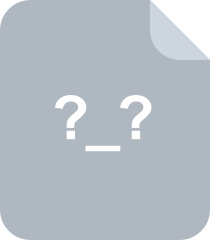
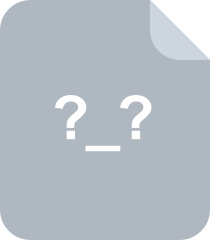
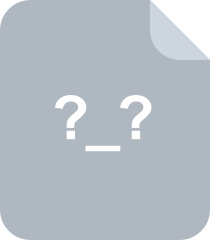
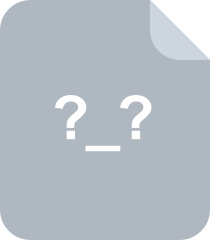
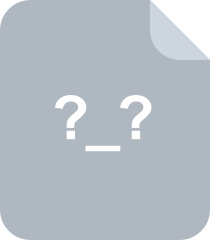
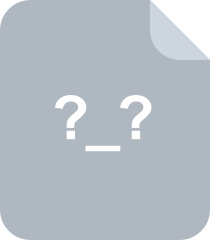
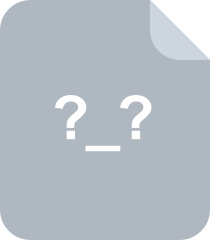
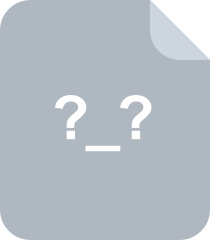
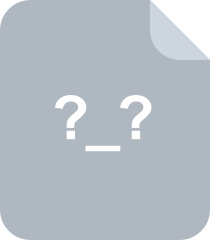
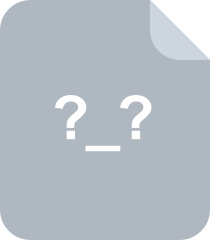
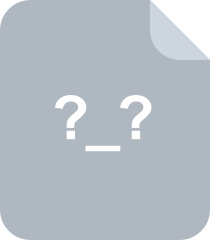
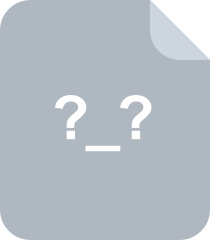
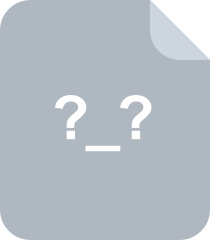
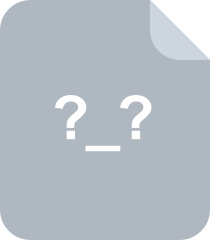
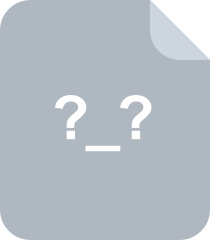
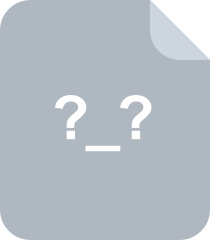
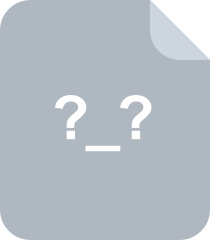
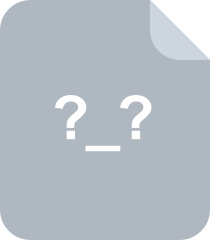
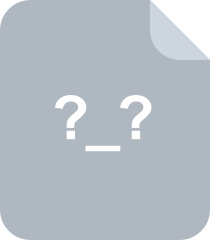
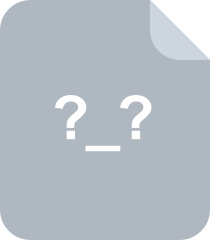
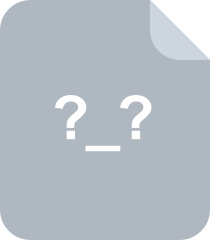
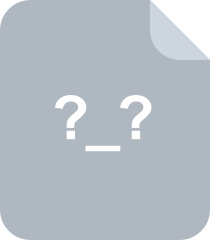
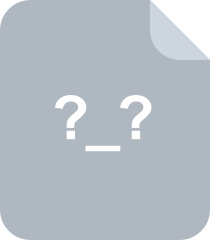
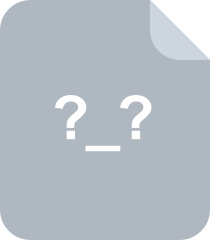
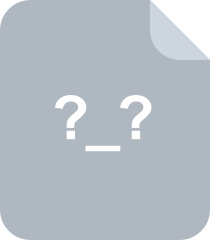
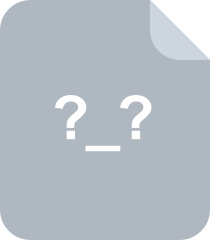
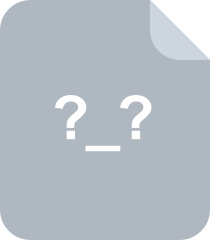
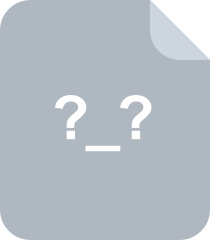
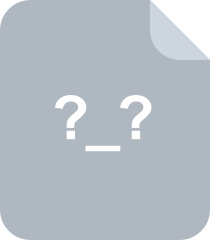
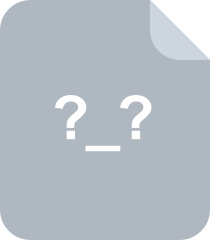
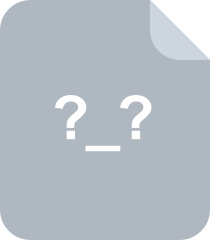
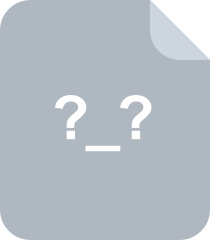
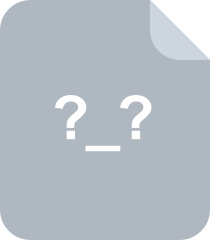
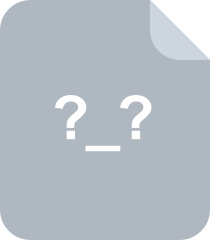
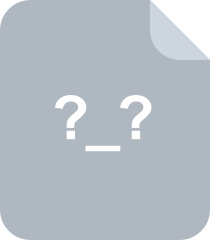
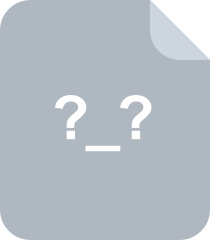
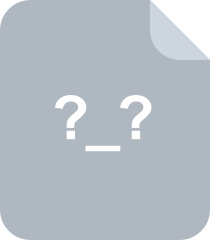
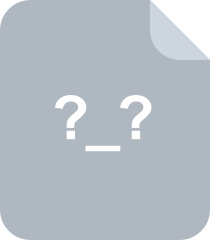
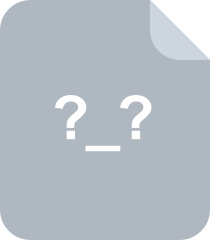
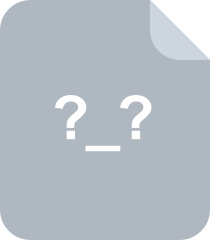
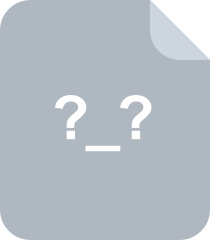
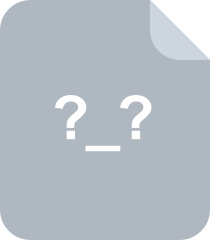
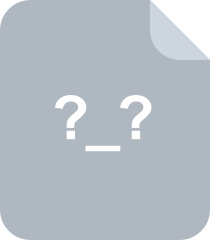
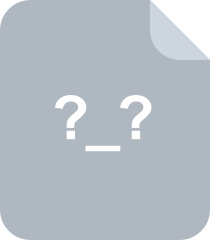
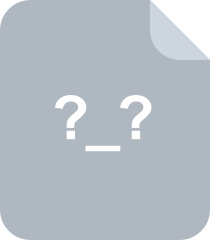
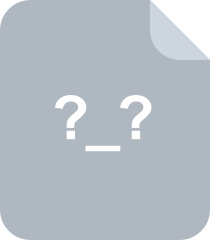
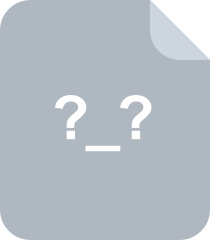
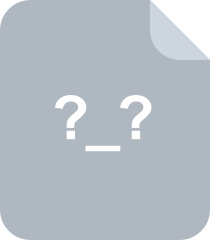
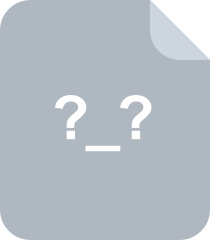
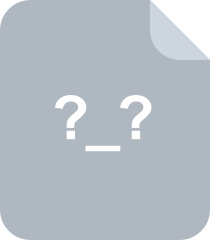
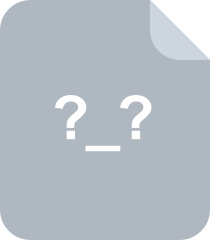
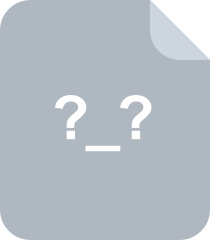
共 1088 条
- 1
- 2
- 3
- 4
- 5
- 6
- 11
资源评论
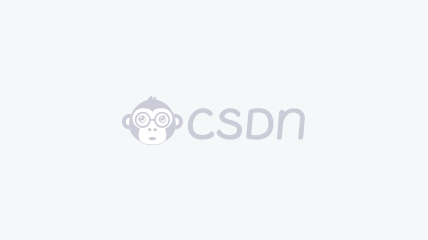

土豆片片
- 粉丝: 1839
- 资源: 5654
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

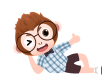
最新资源
- C语言-leetcode题解之83-remove-duplicates-from-sorted-list.c
- C语言-leetcode题解之79-word-search.c
- C语言-leetcode题解之78-subsets.c
- C语言-leetcode题解之75-sort-colors.c
- C语言-leetcode题解之74-search-a-2d-matrix.c
- C语言-leetcode题解之73-set-matrix-zeroes.c
- 树莓派物联网智能家居基础教程
- YOLOv5深度学习目标检测基础教程
- (源码)基于Arduino和Nextion的HMI人机界面系统.zip
- (源码)基于 JavaFX 和 MySQL 的影院管理系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


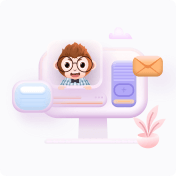
安全验证
文档复制为VIP权益,开通VIP直接复制
