<div align="center">
<br>
<br>
<img width="360" src="media/logo.svg" alt="Got">
<br>
<br>
<br>
<p align="center">Huge thanks to <a href="https://moxy.studio"><img src="https://sindresorhus.com/assets/thanks/moxy-logo.svg" valign="middle" width="150"></a> for sponsoring Sindre Sorhus!
</p>
<p align="center"><sup>(they love Got too!)</sup></p>
<br>
<br>
</div>
> Human-friendly and powerful HTTP request library for Node.js
[](https://travis-ci.com/github/sindresorhus/got)
[](https://coveralls.io/github/sindresorhus/got?branch=master)
[](https://npmjs.com/got)
[](https://packagephobia.now.sh/result?p=got)
[Moving from Request?](documentation/migration-guides.md) [*(Note that Request is unmaintained)*](https://github.com/request/request/issues/3142)
[See how Got compares to other HTTP libraries](#comparison)
For browser usage, we recommend [Ky](https://github.com/sindresorhus/ky) by the same people.
## Highlights
- [Promise API](#api)
- [Stream API](#streams)
- [Pagination API](#pagination)
- [HTTP2 support](#http2)
- [Request cancelation](#aborting-the-request)
- [RFC compliant caching](#cache-adapters)
- [Follows redirects](#followredirect)
- [Retries on failure](#retry)
- [Progress events](#onuploadprogress-progress)
- [Handles gzip/deflate/brotli](#decompress)
- [Timeout handling](#timeout)
- [Errors with metadata](#errors)
- [JSON mode](#json-mode)
- [WHATWG URL support](#url)
- [HTTPS API](#advanced-https-api)
- [Hooks](#hooks)
- [Instances with custom defaults](#instances)
- [Types](#types)
- [Composable](documentation/advanced-creation.md#merging-instances)
- [Plugins](documentation/lets-make-a-plugin.md)
- [Used by 4K+ packages and 1.8M+ repos](https://github.com/sindresorhus/got/network/dependents)
- [Actively maintained](https://github.com/sindresorhus/got/graphs/contributors)
- [Trusted by many companies](#widely-used)
## Install
```
$ npm install got
```
## Usage
###### Promise
```js
const got = require('got');
(async () => {
try {
const response = await got('https://sindresorhus.com');
console.log(response.body);
//=> '<!doctype html> ...'
} catch (error) {
console.log(error.response.body);
//=> 'Internal server error ...'
}
})();
```
###### JSON
```js
const got = require('got');
(async () => {
const {body} = await got.post('https://httpbin.org/anything', {
json: {
hello: 'world'
},
responseType: 'json'
});
console.log(body.data);
//=> {hello: 'world'}
})();
```
See [JSON mode](#json-mode) for more details.
###### Streams
```js
const stream = require('stream');
const {promisify} = require('util');
const fs = require('fs');
const got = require('got');
const pipeline = promisify(stream.pipeline);
(async () => {
await pipeline(
got.stream('https://sindresorhus.com'),
fs.createWriteStream('index.html')
);
// For POST, PUT, PATCH, and DELETE methods, `got.stream` returns a `stream.Writable`.
await pipeline(
fs.createReadStream('index.html'),
got.stream.post('https://sindresorhus.com')
);
})();
```
**Tip:** `from.pipe(to)` doesn't forward errors. Instead, use [`stream.pipeline(from, ..., to, callback)`](https://nodejs.org/api/stream.html#stream_stream_pipeline_streams_callback).
**Note:** While `got.post('https://example.com')` resolves, `got.stream.post('https://example.com')` will hang indefinitely until a body is provided. If there's no body on purpose, remember to `.end()` the stream or set the [`body`](#body) option to an empty string.
### API
It's a `GET` request by default, but can be changed by using different methods or via [`options.method`](#method).
**By default, Got will retry on failure. To disable this option, set [`options.retry`](#retry) to `0`.**
#### got(url?, options?)
Returns a Promise giving a [Response object](#response) or a [Got Stream](#streams-1) if `options.isStream` is set to true.
##### url
Type: `string | object`
The URL to request, as a string, a [`https.request` options object](https://nodejs.org/api/https.html#https_https_request_options_callback), or a [WHATWG `URL`](https://nodejs.org/api/url.html#url_class_url).
Properties from `options` will override properties in the parsed `url`.
If no protocol is specified, it will throw a `TypeError`.
**Note:** The query string is **not** parsed as search params. Example:
```js
got('https://example.com/?query=a b'); //=> https://example.com/?query=a%20b
got('https://example.com/', {searchParams: {query: 'a b'}}); //=> https://example.com/?query=a+b
// The query string is overridden by `searchParams`
got('https://example.com/?query=a b', {searchParams: {query: 'a b'}}); //=> https://example.com/?query=a+b
```
##### options
Type: `object`
Any of the [`https.request`](https://nodejs.org/api/https.html#https_https_request_options_callback) options.
**Note:** Legacy URL support is disabled. `options.path` is supported only for backwards compatibility. Use `options.pathname` and `options.searchParams` instead. `options.auth` has been replaced with `options.username` & `options.password`.
###### method
Type: `string`\
Default: `GET`
The HTTP method used to make the request.
###### prefixUrl
Type: `string | URL`
When specified, `prefixUrl` will be prepended to `url`. The prefix can be any valid URL, either relative or absolute.\
A trailing slash `/` is optional - one will be added automatically.
**Note:** `prefixUrl` will be ignored if the `url` argument is a URL instance.
**Note:** Leading slashes in `input` are disallowed when using this option to enforce consistency and avoid confusion. For example, when the prefix URL is `https://example.com/foo` and the input is `/bar`, there's ambiguity whether the resulting URL would become `https://example.com/foo/bar` or `https://example.com/bar`. The latter is used by browsers.
**Tip:** Useful when used with [`got.extend()`](#custom-endpoints) to create niche-specific Got instances.
**Tip:** You can change `prefixUrl` using hooks as long as the URL still includes the `prefixUrl`. If the URL doesn't include it anymore, it will throw.
```js
const got = require('got');
(async () => {
await got('unicorn', {prefixUrl: 'https://cats.com'});
//=> 'https://cats.com/unicorn'
const instance = got.extend({
prefixUrl: 'https://google.com'
});
await instance('unicorn', {
hooks: {
beforeRequest: [
options => {
options.prefixUrl = 'https://cats.com';
}
]
}
});
//=> 'https://cats.com/unicorn'
})();
```
###### headers
Type: `object`\
Default: `{}`
Request headers.
Existing headers will be overwritten. Headers set to `undefined` will be omitted.
###### isStream
Type: `boolean`\
Default: `false`
Returns a `Stream` instead of a `Promise`. This is equivalent to calling `got.stream(url, options?)`.
###### body
Type: `string | Buffer | stream.Readable` or [`form-data` instance](https://github.com/form-data/form-data)
**Note #1:** The `body` option cannot be used with the `json` or `form` option.
**Note #2:** If you provide this option, `got.stream()` will be read-only.
**Note #3:** If you provide a payload with the `GET` or `HEAD` method, it will throw a `TypeError` unless the method is `GET` and the `allowGetBody` option is set to `true`.
**Note #4:** This option is not enumerable and will not be merged with the instance defaults.
The `content-length` header will be automatically set if `body` is a `string` / `Buffer` / `fs.createReadStream` instance / [`form-data` instance](https://github.com/form-data/form-data), and `content-length` and `transfer-encoding` are not manually set in `options.headers`.
###### json
Type: `object | Array | number | string | boolean | null` *(JSON-serializable values)*
**Note #1:** If you provide thi

土豆片片
- 粉丝: 1857
- 资源: 5869
最新资源
- 日立hgp电梯mcub03主板维修原理图 日立gvf电梯mcub01主板维修原理图 日立hgp电梯evecd03变频器维修原理图 日立gvf电梯evecd01变频器维修原理图 日立hge电梯els-4
- 基于Python3的Mysql数据库操作封装类解析及其应用
- S7-200Smart PLC,MCGS触摸屏,使用中的机组程序,采用通讯方式同步控制3台变频器调速,温度采集程序
- RS422-ARINC429通讯转模块 RS422支持全双工通讯接口,通讯速率可设置,ARINC429支持发送和接收,每通道发送接收速率可单独设置,可卖板卡,也可以根据具体要求设计硬件,支持FPGA
- 无人艇(机)仿真,带gui 具体内容看图片 文字资料已遗失,主参数通过initial来生成,具体模型全部在simulink里面,可自行推导,或者加价我去推导 gui界面打开方式:在主界面输入gu
- MATLAB代码:基于多时间尺度滚动优化的多能源微网双层调度模型红旗红旗红旗火火 关键词:多能源微网 多时间尺度 滚动优化 微网双层模型 调度 红旗红旗 主要内容:代码主要
- 重庆大学 Python 考试题目解析与计算机基础知识点复习资料
- 西门子300PLC 分时产量计数程序,在地址不冲突情况下,改个启动计数条件,就可以拿来在项目上直接使用,间接寻址,程序直观明了,触摸屏有系统时间更改功能
- LNOI绝缘体上铌酸锂薄膜电光调制特性
- Apollo7.0-行为预测模块思维导图及该模块注释代码 , Apollo 7.0为Apollo最新版本,此次重点针对感知和预测算法,进行了模块升级 引入Inter-TNT行为预测模式,通过障碍物与
- 数学建模竞赛之城市交通拥堵与优化-华数杯赛题解析及应用
- 西门子UG后处理三轴后处理840D828D系统 界面简洁,没那么多字幕 无使用限制 带刀具信息 带备刀 带ij圆弧输出 输出m08冷却液 程序段m1暂停 g41半径补偿 结尾回零点 带pui 840没
- simulink 风电调频,双馈风机调频,VSG同步机控制,风电场调频,三机九节点,带有惯性控制,下垂控制 同步机为火电机组,水轮机,可实现同步机调频,火电调频,水轮机调频等 风电渗透20%,ph
- Matlab路径规划算法代码 传统A star算法源码+详细注释 可固定地图和起点终点 适合初学者入门学习使用 保证运行
- 冲床送料机程序,送料机程序,伺服送料机程序,伺服电机,程序,三菱,台达,中达一体机,送料机程序,PLC多段数据不同,可任意调节A段B段c段长度,并定长切断 程序能存储5段工件数据,使用调出非常方便
- 项目:超声波-基于Arduino的超声波距离测量(LCD1602显示) 设计;proteus 仿真(版本8.9-可提供软件安装包) 主控:Arduino UNO 外设:超声波,LCD1602 程序:
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


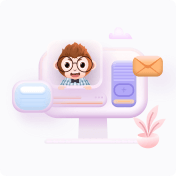