package com.nncq.sunmoon.controller;
import java.io.File;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.util.ArrayList;
import java.util.Date;
import java.util.Iterator;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.multipart.MultipartFile;
import org.springframework.web.multipart.MultipartHttpServletRequest;
import org.springframework.web.multipart.commons.CommonsMultipartResolver;
import com.nncq.sunmoon.entity.Contract;
import com.nncq.sunmoon.entity.Department;
import com.nncq.sunmoon.entity.DepartmentStaff;
import com.nncq.sunmoon.entity.Login;
import com.nncq.sunmoon.entity.Msg;
import com.nncq.sunmoon.entity.Notice;
import com.nncq.sunmoon.entity.Rank;
import com.fasterxml.jackson.annotation.JsonTypeInfo.Id;
import com.nncq.sunmoon.entity.AdminTransferStaff;
import com.nncq.sunmoon.entity.Apply;
import com.nncq.sunmoon.entity.ChartsKeyValue;
import com.nncq.sunmoon.entity.Staff;
import com.nncq.sunmoon.entity.StaffAndPosition;
import com.nncq.sunmoon.entity.StaffContract;
import com.nncq.sunmoon.entity.StaffIdName;
import com.nncq.sunmoon.entity.StaffIdSetting;
import com.nncq.sunmoon.entity.Transfer;
import com.nncq.sunmoon.service.ApplyService;
import com.nncq.sunmoon.service.ContractManageService;
import com.nncq.sunmoon.service.DepartmentService;
import com.nncq.sunmoon.service.LoginService;
import com.nncq.sunmoon.service.MsgService;
import com.nncq.sunmoon.service.NoticeService;
import com.nncq.sunmoon.service.StaffApplyService;
import com.nncq.sunmoon.service.StaffManageService;
import com.nncq.sunmoon.tools.Datetool;
import com.nncq.sunmoon.tools.SendMail;
import com.nncq.sunmoon.tools.SessionListener;
import com.nncq.sunmoon.tools.StaticValues;
import com.nncq.sunmoon.tools.entity.SelectEntity;
import net.sf.json.JSONArray;
import net.sf.json.JSONObject;
/**
* 职员管理 控制层
*
* @author duoduo
*
*/
@RequestMapping("staffManage")
@Controller
public class StaffManageController {
@Autowired
private ContractManageService contractManageService;
@Autowired
private StaffManageService staffManageService;
@Autowired
private MsgService msgService;
@Autowired
private DepartmentService departmentService;
@Autowired
private StaffApplyService staffApplyService;
@Autowired
private ApplyService applyService;
@Autowired
private NoticeService noticeService;
/**
* 查询员工
*
* @param response
* @param page
* @param limit
* @param field
* @param order
* @param key
* @param filter
*/
@RequestMapping(value = "getStaffsBySE", method = RequestMethod.GET)
public void getStaffsBySE(HttpServletRequest request, HttpServletResponse response, int page, int limit,
String field, String order, String key, String[] filter, String department_id) {
// 构建查询语句
StaffAndPosition staff = (StaffAndPosition) request.getSession().getAttribute("staff");
if (staff == null) {
return;
}
String sql = "";
if (filter != null) {
for (int i = 0; i < filter.length; i++) {
filter[i] = "IFNULL(" + filter[i] + ",'')";
}
sql = String.join(",", filter);
} else {
sql = "IFNULL(staff_id,'')";
}
if (key == null) {
key = "";
}
if (department_id != null && department_id != "") {
sql = "department_id = '" + department_id + "' and CONCAT(" + sql + ")";
} else if (staff.getPowerMap().get(StaticValues.powerMap.get("span/department")) == null) {
sql = "department_id in( select department_id from department where FIND_IN_SET(department_id,getChildList('"
+ staff.getDepartment_id() + "')) ) and CONCAT(" + sql + ")";
} else {
sql = "CONCAT(" + sql + ")";
}
response.setCharacterEncoding("UTF-8");
SelectEntity selectEntity = new SelectEntity();
selectEntity.setStart((page - 1) * limit);
selectEntity.setLimit(limit);
selectEntity.setKey(key);
selectEntity.setSql(sql);
if (field == null) {
field = "staff_id";
order = "desc";
}
selectEntity.setField(field);
selectEntity.setOrder(order);
// 执行查询
List<Staff> list = staffManageService.getStaffsBySE(selectEntity);
int num = staffManageService.getStaffsNumberBySE(selectEntity);
JSONObject json = new JSONObject();
JSONArray data = JSONArray.fromObject(list);
json.put("code", 0);
json.put("msg", "");
json.put("count", num);
json.put("data", data);
try {
response.getWriter().print(json);
} catch (IOException e) {
e.printStackTrace();
}
}
/**
* 查询员工花名册
*
* @param response
* @param page
* @param limit
* @param field
* @param order
* @param key
* @param filter
*/
@RequestMapping(value = "getStaffsRosterBySE", method = RequestMethod.GET)
public void getStaffsRosterBySE(HttpServletResponse response, int page, int limit, String field, String order,
String key, String[] filter) {
// 构建查询语句
String sql = "";
if (filter != null) {
for (int i = 0; i < filter.length; i++) {
filter[i] = "IFNULL(" + filter[i] + ",'')";
}
sql = String.join(",", filter);
} else {
sql = "IFNULL(staff_id,'')";
}
if (key == null) {
key = "";
}
sql = "staff_state='在职' and CONCAT(" + sql + ")";
response.setCharacterEncoding("UTF-8");
SelectEntity selectEntity = new SelectEntity();
selectEntity.setStart((page - 1) * limit);
selectEntity.setLimit(limit);
selectEntity.setKey(key);
selectEntity.setSql(sql);
if (field == null) {
field = "staff_id";
order = "desc";
}
selectEntity.setField(field);
selectEntity.setOrder(order);
// 执行查询
List<Staff> list = staffManageService.getStaffsBySE(selectEntity);
int num = staffManageService.getStaffsNumberBySE(selectEntity);
JSONObject json = new JSONObject();
JSONArray data = JSONArray.fromObject(list);
json.put("code", 0);
json.put("msg", "");
json.put("count", num);
json.put("data", data);
try {
response.getWriter().print(json);
} catch (IOException e) {
e.printStackTrace();
}
}
@RequestMapping(value = "getAllStaffIdName", method = RequestMethod.GET)
public void getAllStaffIdName(HttpServletResponse response, HttpServletRequest request) {
JSONObject json = new JSONObject();
int code = 100;
response.setCharacterEncoding("UTF-8");
List<StaffIdName> list = staffManageService.getAllStaffIdName();
json.put("code", code);
json.put("data", list);
try {
response.getWriter().print(json);
} catch (IOException e) {
e.printStackTrace();
}
}
@RequestMapping(value = "getStaffByDepId", method = RequestMethod.GET)
public void getStaffByDepId(HttpServletRequest request, HttpServletResponse response, int page, int limit,
String field, String order, String key, String[] filter, String department_id) {
// 构建查询语句
StaffAndPosition staff = (StaffAndPosition) request.getSession().getAttribute("staff");
if (staff == null) {
return;
}
String sql = "";
if (filter != null) {
for (int i = 0; i < filter.length; i++) {
filter[i] = "IFNULL(" + filter[i] + ",'')";
}
sql = String.join(",", filter);
} else {
sql = "IFNULL(staff_id,'')";
}
if (key == null) {
key = "";
}
sql = "department_id='"+staff.getDepartment_id()+"' and staff_id <> '"+staff.getStaff_id()+"' and CONCAT(" + sql + ")";
response.setCharacterEncoding("UTF-8");
SelectEntity selectEntity = new SelectEntity();
selectEntity.setStart((page - 1) * limit);
selectEntity.setLimit(limit);
selectEntity.setKey(key);
selectEntity.setSql(sql);
if (field == null) {
field = "staff_id";
order = "desc";
}
selectEntity.setField(field);
selectEntity.setOrder(order);
// 执�
没有合适的资源?快使用搜索试试~ 我知道了~
小型人力资源管理系统源码+项目说明+数据库.zip
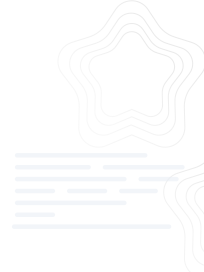
共759个文件
java:189个
class:175个
gif:83个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 48 浏览量
2024-03-05
15:27:51
上传
评论
收藏 231.5MB ZIP 举报
温馨提示
【资源说明】 1、该资源包括项目的全部源码,下载可以直接使用! 2、本项目适合作为计算机、数学、电子信息等专业的课程设计、期末大作业和毕设项目,作为参考资料学习借鉴。 3、本资源作为“参考资料”如果需要实现其他功能,需要能看懂代码,并且热爱钻研,自行调试。 小型人力资源管理系统源码+项目说明+数据库.zip 小型人力资源管理系统源码+项目说明+数据库.zip 小型人力资源管理系统源码+项目说明+数据库.zip 小型人力资源管理系统源码+项目说明+数据库.zip 小型人力资源管理系统源码+项目说明+数据库.zip 小型人力资源管理系统源码+项目说明+数据库.zip 小型人力资源管理系统源码+项目说明+数据库.zip 小型人力资源管理系统源码+项目说明+数据库.zip 小型人力资源管理系统源码+项目说明+数据库.zip 小型人力资源管理系统源码+项目说明+数据库.zip 小型人力资源管理系统源码+项目说明+数据库.zip 小型人力资源管理系统源码+项目说明+数据库.zip 小型人力资源管理系统源码+项目说明+数据库.zip 小型人力资源管理系统源码+项目说明+数据库.zip
资源推荐
资源详情
资源评论
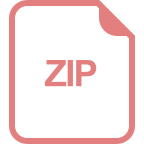
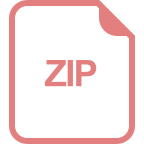
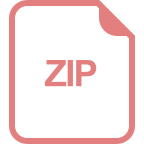
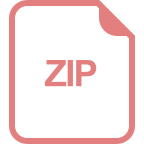
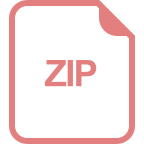
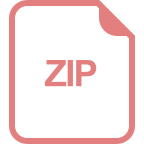
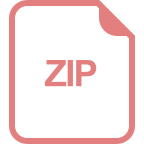
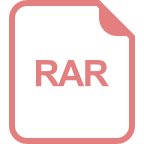
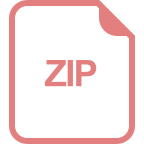
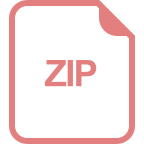
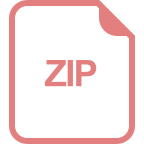
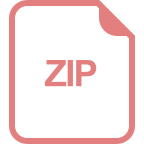
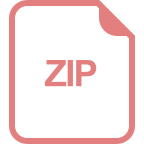
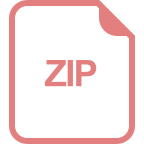
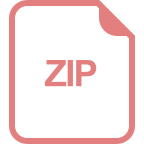
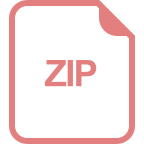
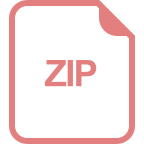
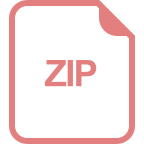
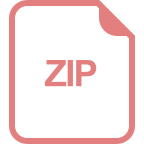
收起资源包目录

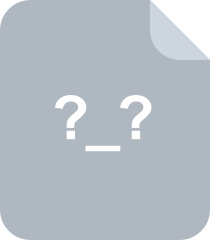
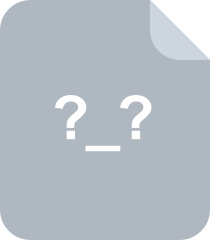
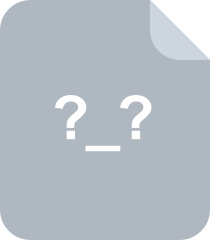
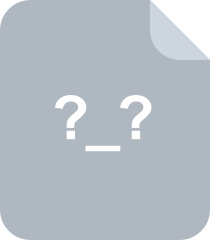
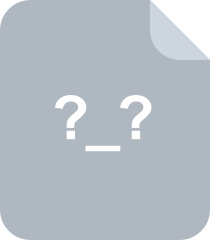
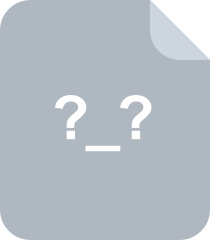
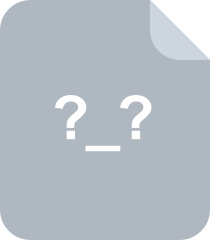
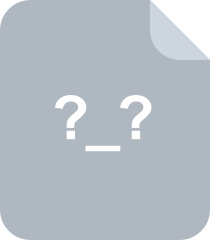
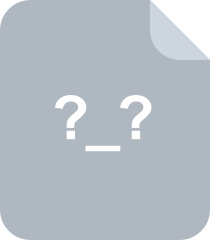
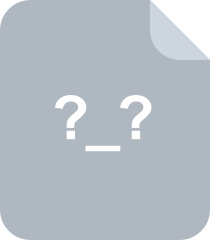
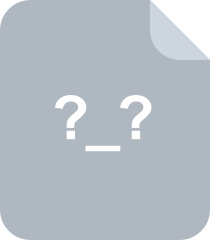
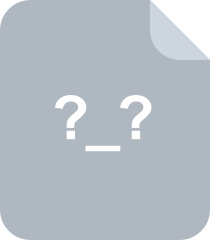
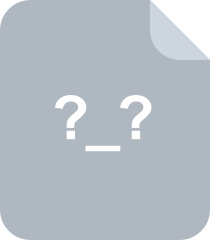
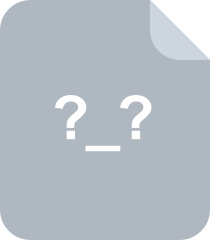
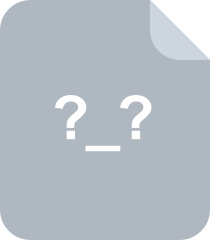
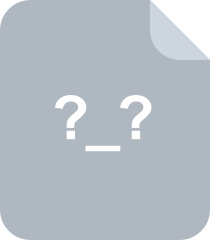
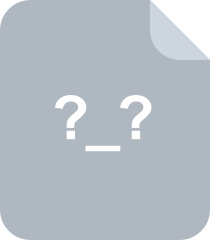
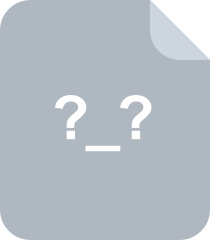
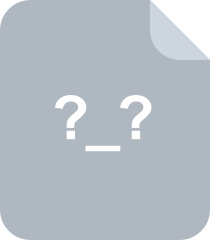
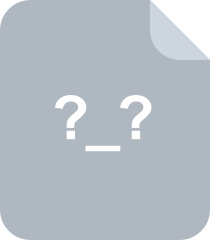
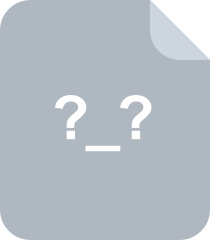
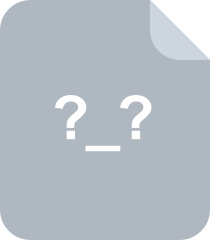
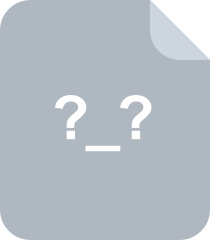
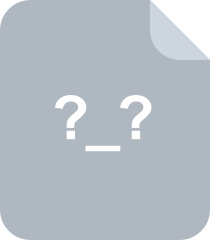
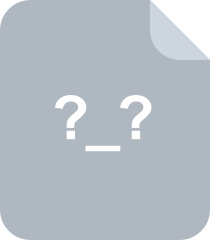
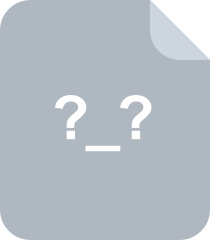
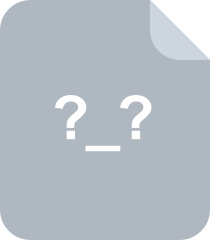
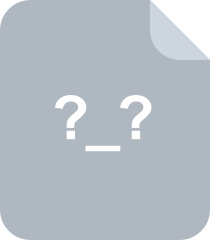
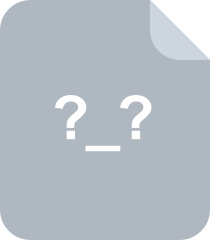
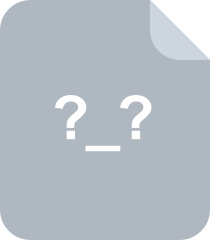
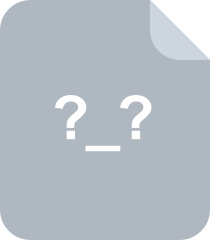
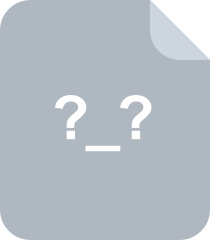
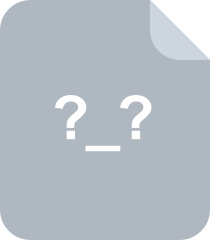
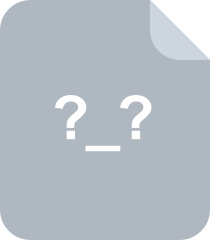
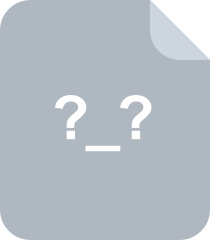
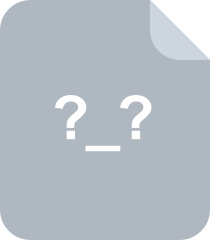
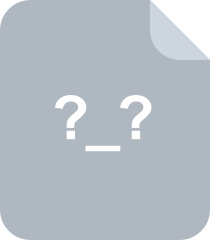
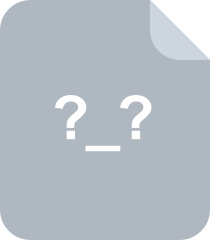
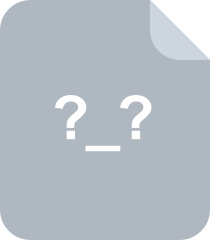
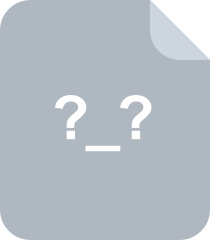
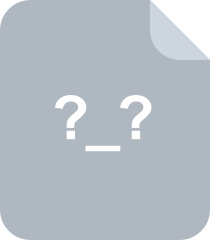
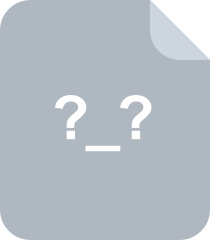
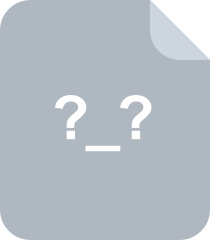
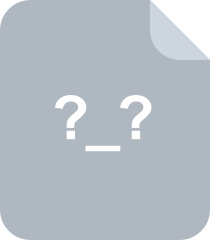
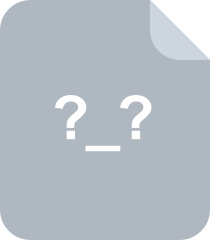
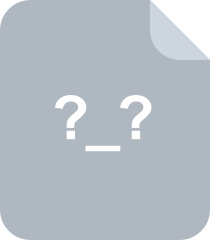
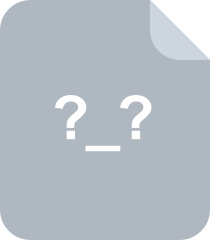
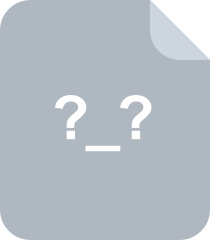
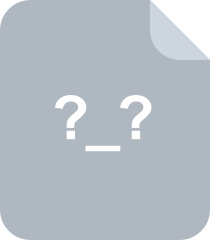
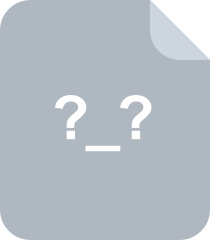
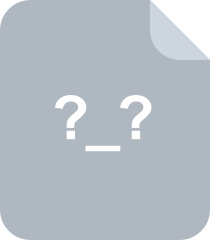
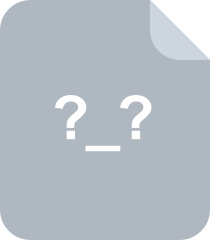
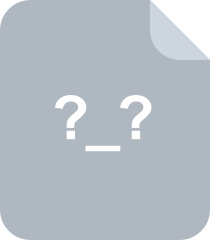
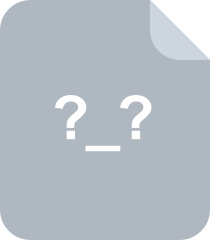
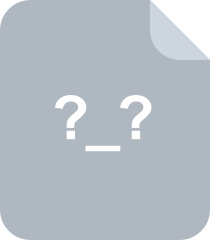
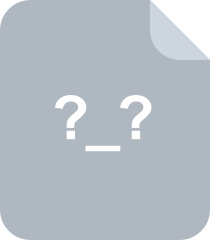
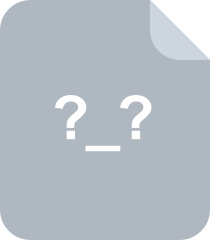
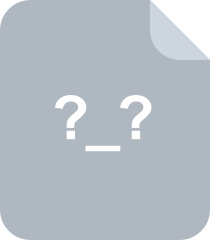
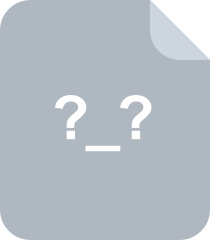
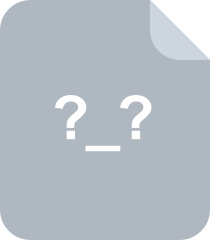
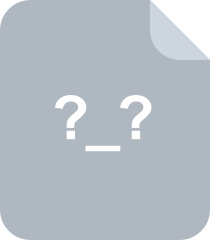
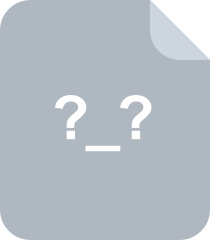
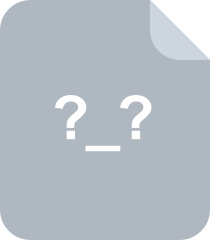
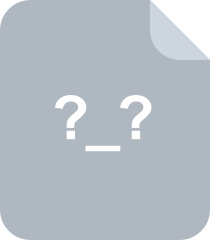
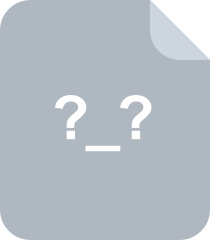
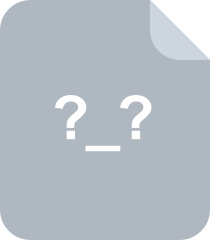
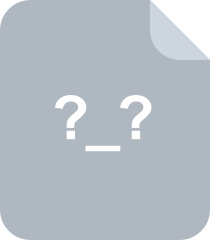
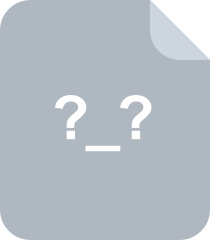
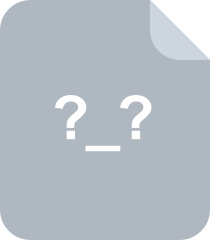
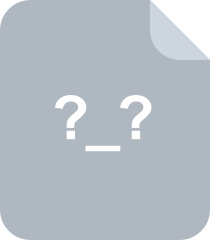
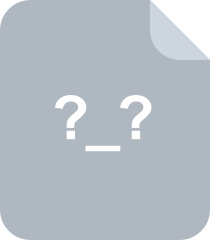
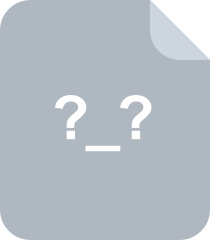
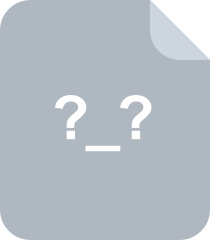
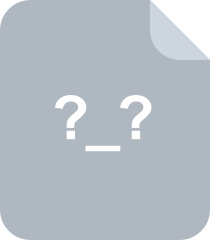
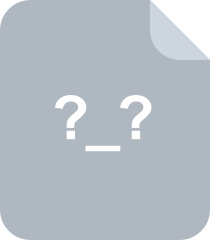
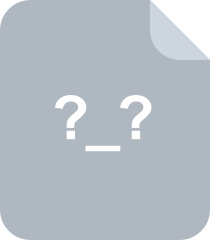
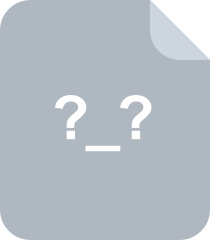
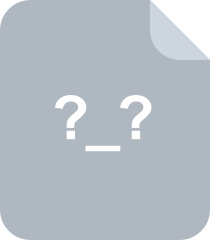
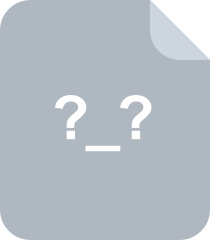
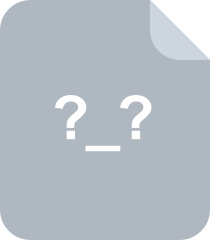
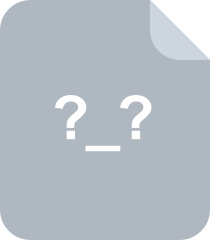
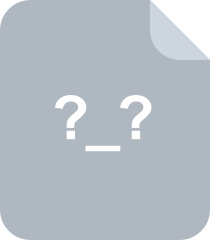
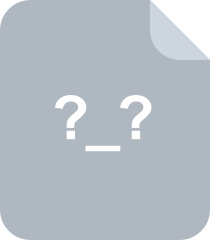
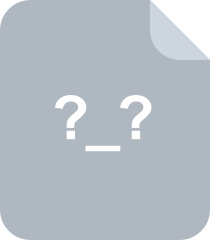
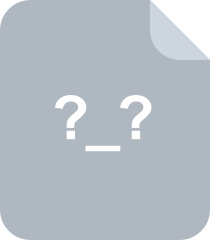
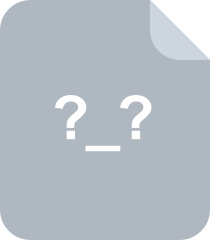
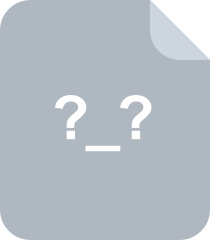
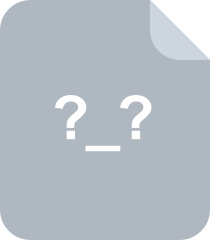
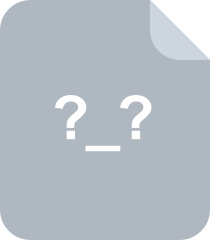
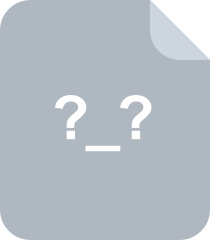
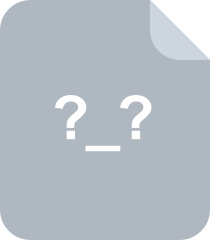
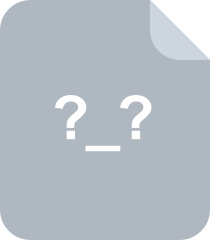
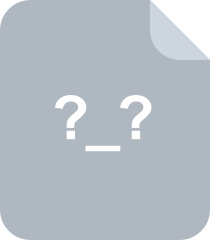
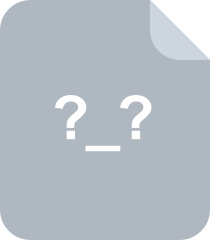
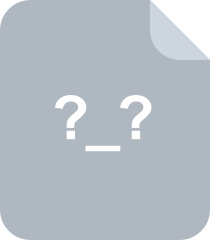
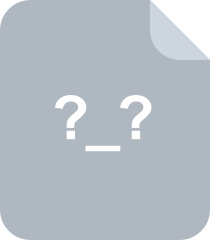
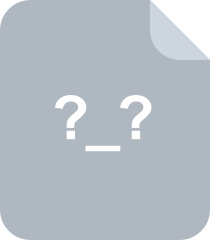
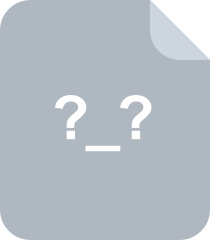
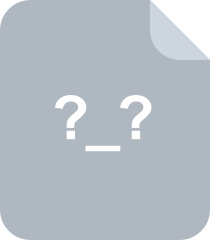
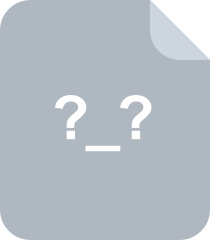
共 759 条
- 1
- 2
- 3
- 4
- 5
- 6
- 8
资源评论
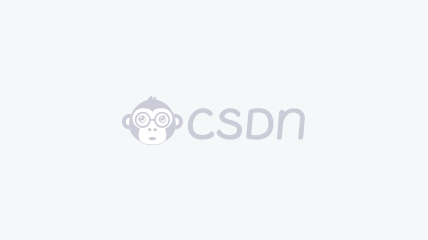

土豆片片
- 粉丝: 1843
- 资源: 5862
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

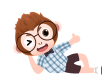
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


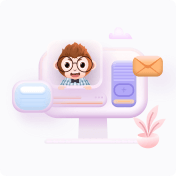
安全验证
文档复制为VIP权益,开通VIP直接复制
