#coding=utf-8
#import tensorflow as tf
import tensorflow.compat.v1 as tf
tf.disable_v2_behavior()
import CNN_train_data as train_data
import cv2
import random
import matplotlib.pyplot as plt
import numpy as np
from sklearn.metrics import confusion_matrix, classification_report
train_epochs=3000
batch_size = 9
drop_prob = 0.4
learning_rate=0.00001
def weight_init(shape):
weight = tf.truncated_normal(shape,stddev=0.1,dtype=tf.float32)
return tf.Variable(weight)
def bias_init(shape):
bias = tf.random_normal(shape,dtype=tf.float32)
return tf.Variable(bias)
images_input = tf.placeholder(tf.float32,[None,112*92*3],name='input_images')
labels_input = tf.placeholder(tf.float32,[None,2],name='input_labels')
def fch_init(layer1,layer2,const=1):
min = -const * (6.0 / (layer1 + layer2));
max = -min;
weight = tf.random_uniform([layer1, layer2], minval=min, maxval=max, dtype=tf.float32)
return tf.Variable(weight)
def conv2d(images,weight):
return tf.nn.conv2d(images,weight,strides=[1,1,1,1],padding='SAME')
def max_pool2x2(images,tname):
return tf.nn.max_pool(images,ksize=[1,2,2,1],strides=[1,2,2,1],padding='SAME',name=tname)
x_input = tf.reshape(images_input,[-1,112,92,3])
# 卷积核3*3*3 16个 第一层卷积
w1 = weight_init([3,3,3,16])
b1 = bias_init([16])
# 结果 NHWC N H W C
conv_1 = conv2d(x_input,w1)+b1
relu_1 = tf.nn.relu(conv_1,name='relu_1')
max_pool_1 = max_pool2x2(relu_1,'max_pool_1')
# 卷积核3*3*16 32个 第二层卷积
w2 = weight_init([3,3,16,32])
b2 = bias_init([32])
conv_2 = conv2d(max_pool_1,w2) + b2
relu_2 = tf.nn.relu(conv_2,name='relu_2')
max_pool_2 = max_pool2x2(relu_2,'max_pool_2')
# 卷积核3*3*32 64个 第三层卷积
w3 = weight_init([3,3,32,64])
b3 = bias_init([64])
conv_3 = conv2d(max_pool_2,w3)+b3
relu_3 = tf.nn.relu(conv_3,name='relu_3')
max_pool_3 = max_pool2x2(relu_3,'max_pool_3')
f_input = tf.reshape(max_pool_3,[-1,14*12*64])
#全连接第一层 31*31*32,512
f_w1= fch_init(14*12*64,512)
f_b1 = bias_init([512])
f_r1 = tf.matmul(f_input,f_w1) + f_b1
f_relu_r1 = tf.nn.relu(f_r1)
f_dropout_r1 = tf.nn.dropout(f_relu_r1,drop_prob)
f_w2 = fch_init(512,128)
f_b2 = bias_init([128])
f_r2 = tf.matmul(f_dropout_r1,f_w2) + f_b2
f_relu_r2 = tf.nn.relu(f_r2)
f_dropout_r2 = tf.nn.dropout(f_relu_r2,drop_prob)
#全连接第二层 512,2
f_w3 = fch_init(128,2)
f_b3 = bias_init([2])
f_r3 = tf.matmul(f_dropout_r2,f_w3) + f_b3
f_softmax = tf.nn.softmax(f_r3,name='f_softmax')
#定义交叉熵
cross_entry = tf.reduce_mean(tf.reduce_sum(-labels_input*tf.log(f_softmax)))
optimizer = tf.train.AdamOptimizer(learning_rate).minimize(cross_entry)
#计算准确率
arg1 = tf.argmax(labels_input,1)
arg2 = tf.argmax(f_softmax,1)
cos = tf.equal(arg1,arg2)
acc = tf.reduce_mean(tf.cast(cos,dtype=tf.float32))
init = tf.global_variables_initializer()
sess = tf.Session()
sess.run(init)
Cost = []
Accuracy=[]
for i in range(train_epochs):
idx=random.randint(0,len(train_data.images)-20)
batch= random.randint(6,18)
train_input = train_data.images[idx:(idx+batch)]
train_labels = train_data.labels[idx:(idx+batch)]
result,acc1,cross_entry_r,cos1,f_softmax1,relu_1_r= sess.run([optimizer,acc,cross_entry,cos,f_softmax,relu_1],feed_dict={images_input:train_input,labels_input:train_labels})
print (acc1)
Cost.append(cross_entry_r)
Accuracy.append(acc1)
# 代价函数曲线
fig1,ax1 = plt.subplots(figsize=(10,7))
plt.plot(Cost)
ax1.set_xlabel('Epochs')
ax1.set_ylabel('Cost')
plt.title('Cross Loss')
plt.grid()
plt.show()
# 准确率曲线
fig7,ax7 = plt.subplots(figsize=(10,7))
plt.plot(Accuracy)
ax7.set_xlabel('Epochs')
ax7.set_ylabel('Accuracy Rate')
plt.title('Train Accuracy Rate')
plt.grid()
plt.show()
#测试
arg2_r = sess.run(arg2,feed_dict={images_input:train_data.test_images,labels_input:train_data.test_labels})
arg1_r = sess.run(arg1,feed_dict={images_input:train_data.test_images,labels_input:train_data.test_labels})
print (classification_report(arg1_r, arg2_r))
#保存模型
saver = tf.train.Saver()
saver.save(sess, './model/my-gender-v1.0')
没有合适的资源?快使用搜索试试~ 我知道了~
基于卷积神经网络算法的人脸识别项目源码+项目说明.zip
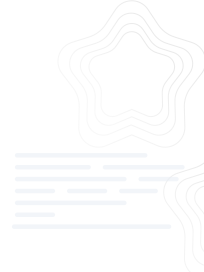
共402个文件
bmp:399个
py:3个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 34 浏览量
2024-02-19
10:52:57
上传
评论 1
收藏 4.24MB ZIP 举报
温馨提示
【资源说明】 1、该资源包括项目的全部源码,下载可以直接使用! 2、本项目适合作为计算机、数学、电子信息等专业的课程设计、期末大作业和毕设项目,作为参考资料学习借鉴。 3、本资源作为“参考资料”如果需要实现其他功能,需要能看懂代码,并且热爱钻研,自行调试。 基于卷积神经网络算法的人脸识别项目源码+项目说明.zip 基于卷积神经网络算法的人脸识别项目源码+项目说明.zip 基于卷积神经网络算法的人脸识别项目源码+项目说明.zip 基于卷积神经网络算法的人脸识别项目源码+项目说明.zip 基于卷积神经网络算法的人脸识别项目源码+项目说明.zip 基于卷积神经网络算法的人脸识别项目源码+项目说明.zip 基于卷积神经网络算法的人脸识别项目源码+项目说明.zip 基于卷积神经网络算法的人脸识别项目源码+项目说明.zip 基于卷积神经网络算法的人脸识别项目源码+项目说明.zip
资源推荐
资源详情
资源评论
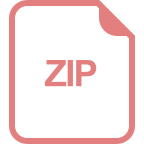
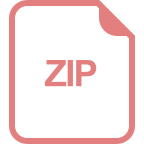
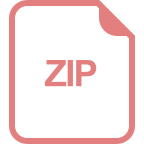
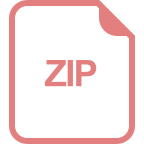
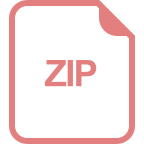
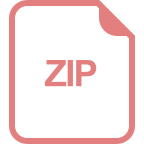
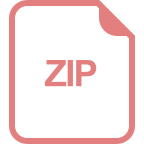
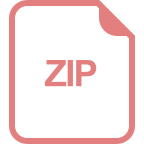
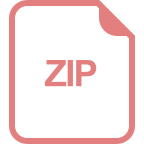
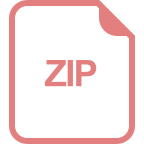
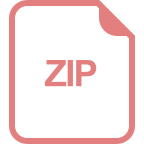
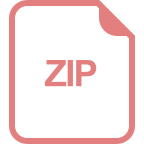
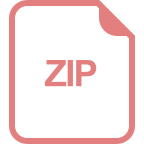
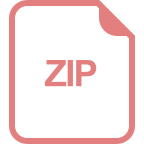
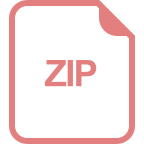
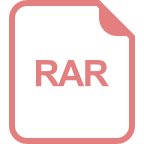
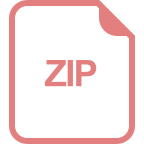
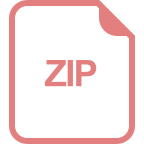
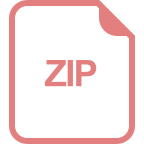
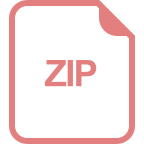
收起资源包目录

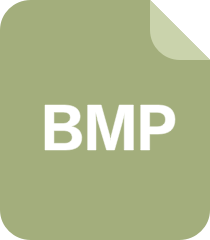
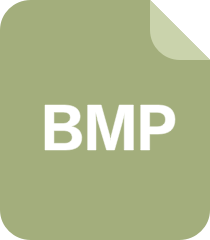
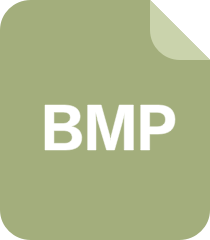
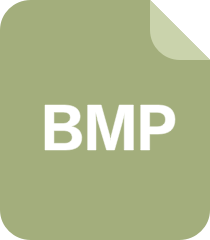
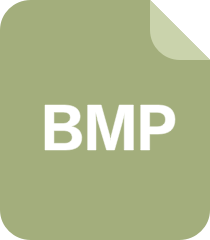
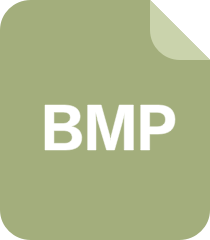
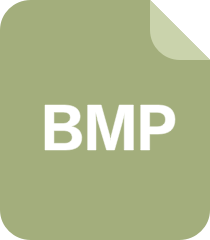
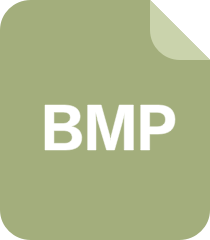
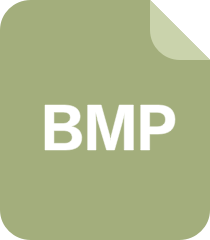
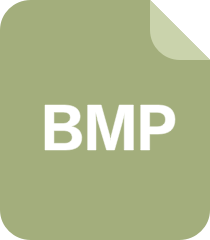
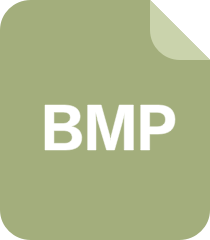
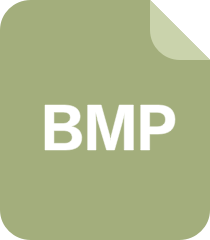
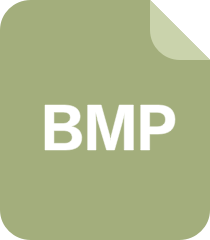
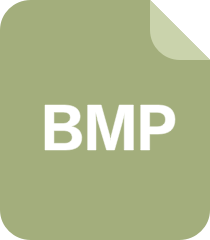
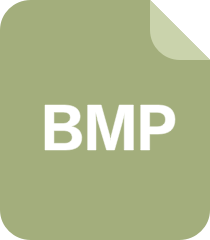
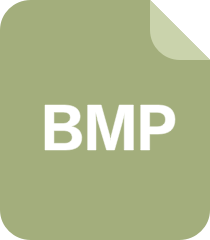
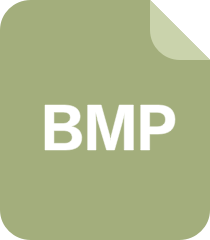
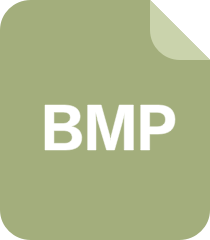
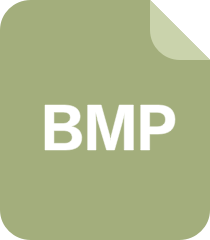
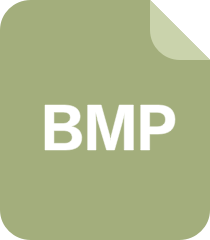
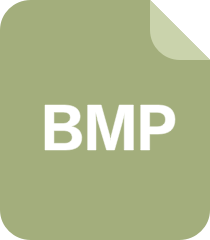
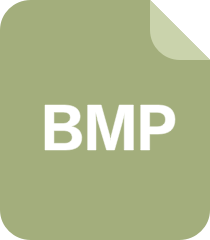
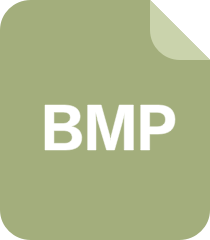
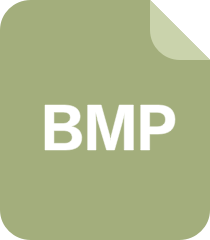
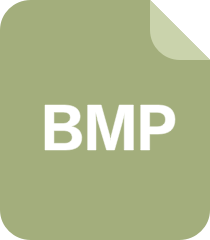
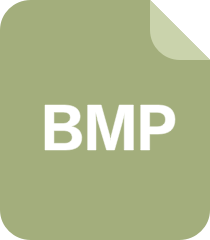
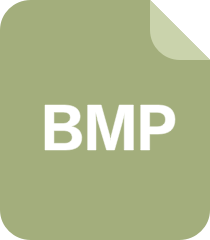
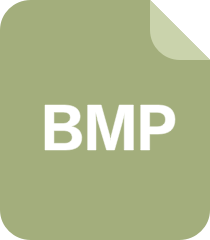
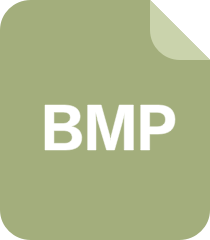
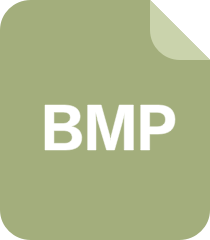
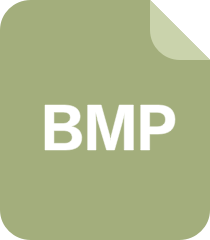
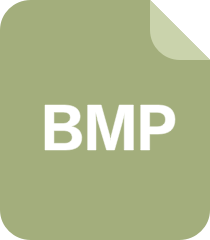
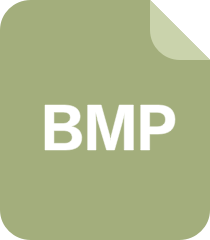
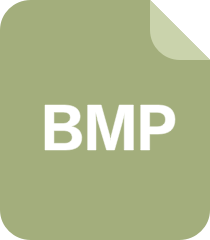
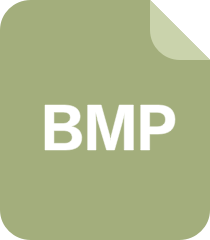
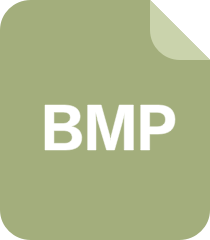
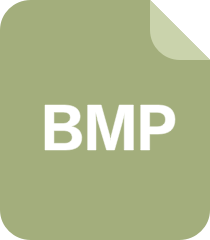
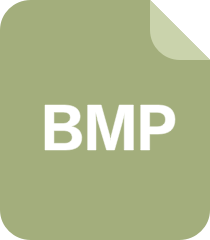
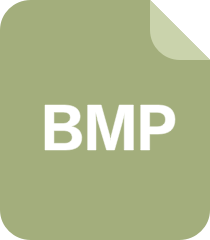
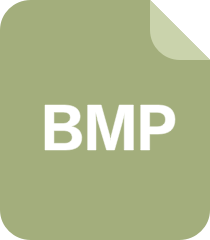
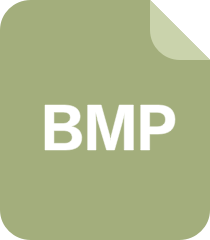
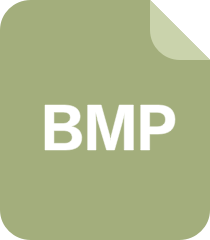
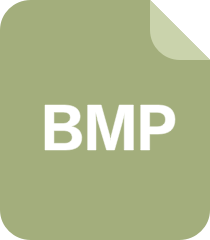
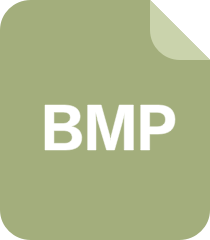
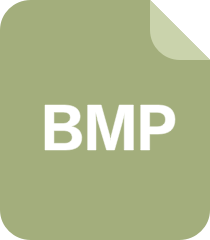
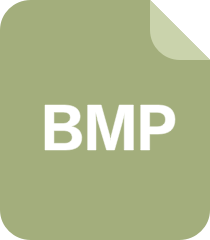
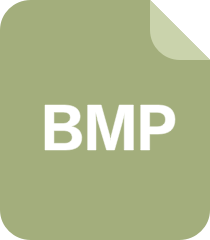
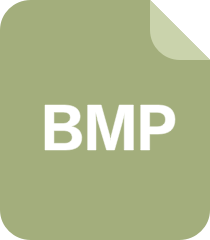
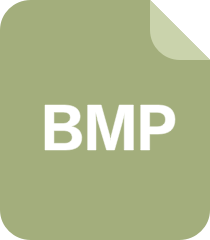
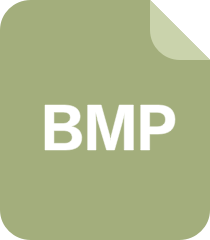
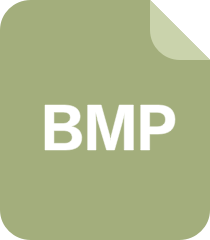
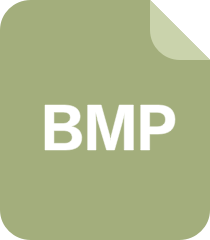
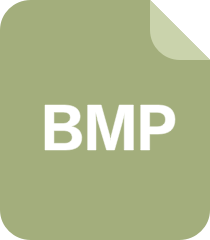
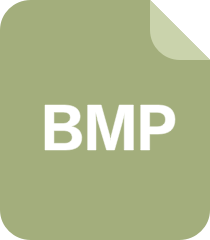
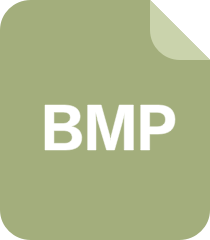
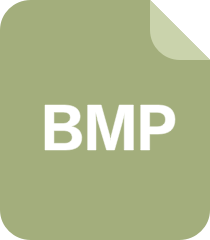
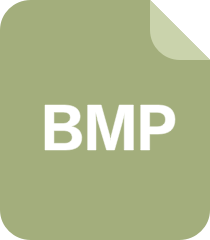
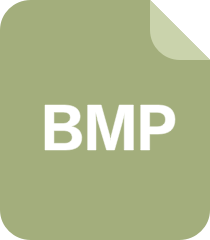
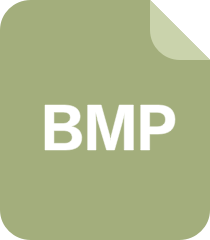
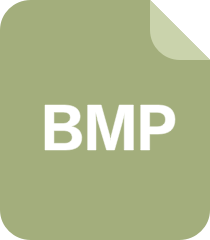
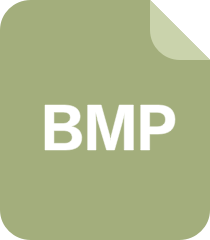
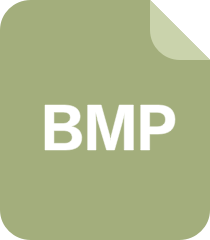
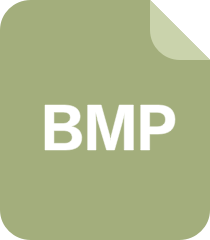
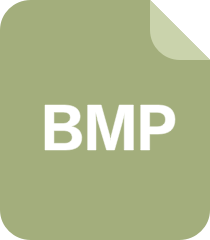
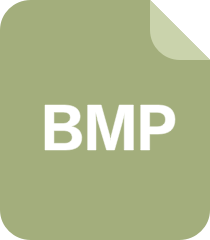
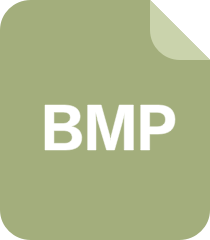
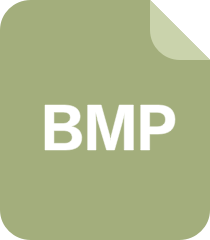
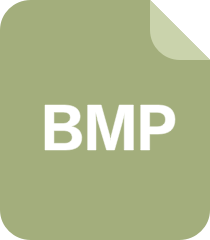
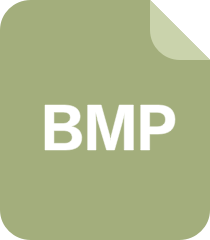
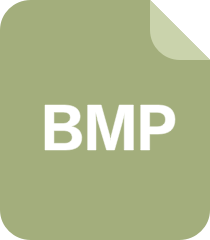
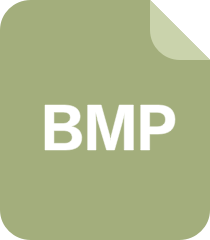
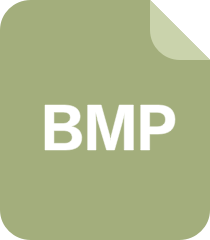
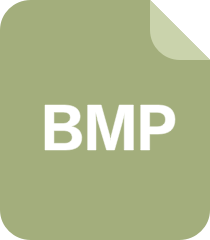
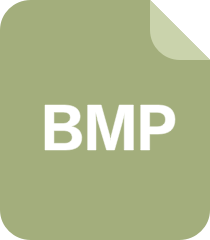
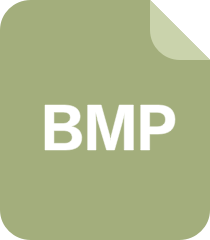
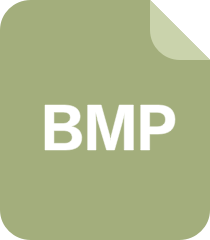
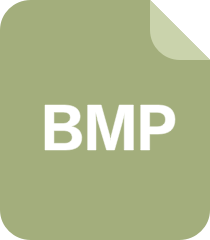
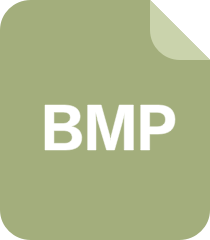
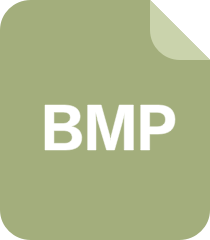
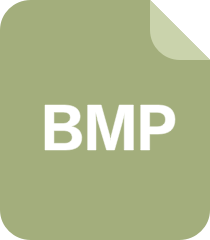
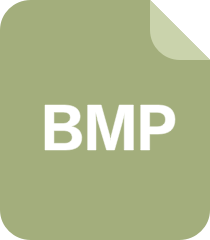
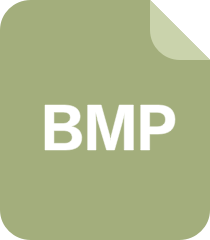
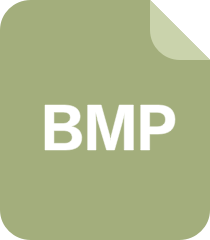
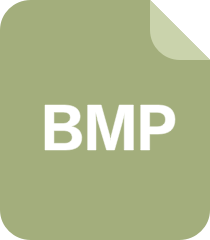
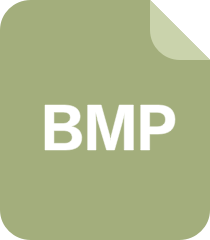
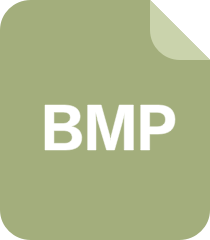
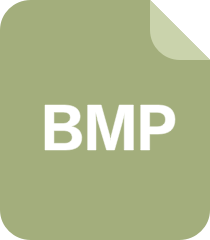
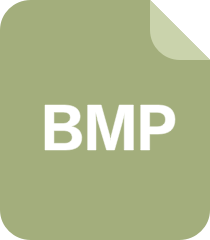
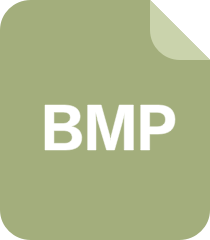
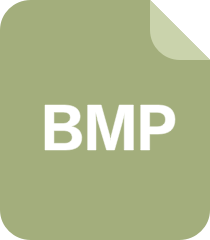
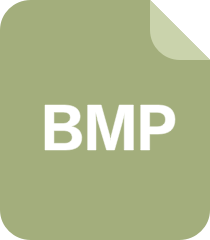
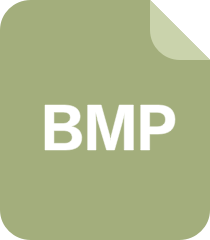
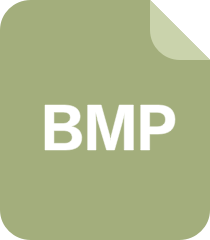
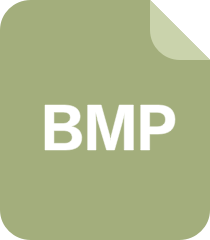
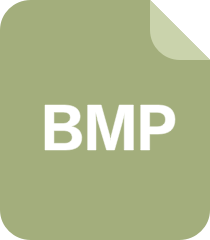
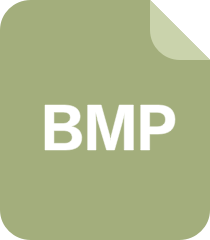
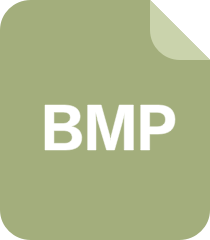
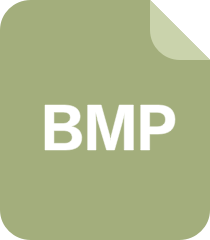
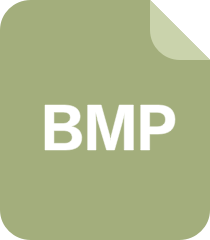
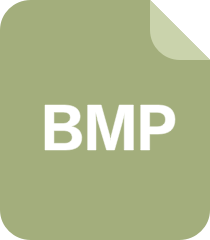
共 402 条
- 1
- 2
- 3
- 4
- 5
资源评论
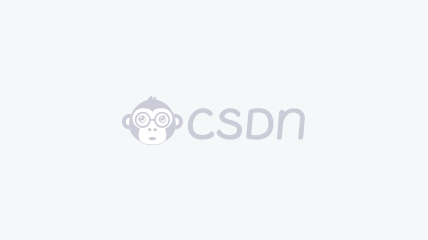

土豆片片
- 粉丝: 1857
- 资源: 5869
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

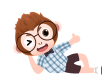
最新资源
- STM32Cube高效开发教程基础篇第二部分 第20-22章
- 校园社团招新活动模板.pptx
- 中国风工作汇报模板.pptx
- 校园艺术节活动策划主题模板.pptx
- 大气党建党政风政府机关工作计划模板.pptx
- 六一儿童节活动策划模板.pptx
- vscode配置c++环境.md
- vscode配置c++环境.md
- 可爱卡通幼儿教育教学模板.pptx
- 大学生创业计划书模板.pptx
- 中国风工作总结汇报模板.pptx
- vscode配置c++环境.md
- 芋道 yudao ruoyi-vue-pro mall sql
- 新生开学班会家长会模板.pptx
- 音乐课开学第一课教育模板.pptx
- 小清新教师说课模板.pptx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


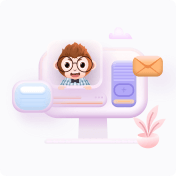
安全验证
文档复制为VIP权益,开通VIP直接复制
