package com.mathlab.action;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import javax.swing.JFileChooser;
import javax.swing.filechooser.FileFilter;
import jxl.Workbook;
import jxl.format.Alignment;
import jxl.format.Colour;
import jxl.format.UnderlineStyle;
import jxl.format.VerticalAlignment;
import jxl.write.Label;
import jxl.write.WritableCellFormat;
import jxl.write.WritableFont;
import jxl.write.WritableSheet;
import jxl.write.WritableWorkbook;
import jxl.write.WriteException;
import jxl.write.biff.RowsExceededException;
import com.mathlab.model.Student;
import com.mathlab.service.StuService;
import com.opensymphony.xwork2.ActionSupport;
public class ModelToExcelAction extends ActionSupport {
StuService stuService;
public StuService getStuService() {
return stuService;
}
public void setStuService(StuService stuService) {
this.stuService = stuService;
}
@Override
public String execute() throws Exception {
JFileChooser jfc = new JFileChooser();
jfc.setAcceptAllFileFilterUsed(false);
jfc.setFileSelectionMode(JFileChooser.DIRECTORIES_ONLY);//只是针对文件夹有效
jfc.setFileFilter(
new FileFilter(){
public boolean accept(File f) {
// TODO Auto-generated method stub
return f.getName().toLowerCase().endsWith(".xls");
}
public String getDescription() {
// TODO Auto-generated method stub
return "Excel File";
}
}
);
jfc.showSaveDialog(null);
String filePath = jfc.getSelectedFile().getPath();
System.out.println(filePath);
WritableWorkbook wwb;
FileOutputStream fos;
try{
fos = new FileOutputStream(filePath+"\\"+"学生名单"+".xls");
wwb = Workbook.createWorkbook(fos);
WritableSheet ws = wwb.createSheet("sheet1", 0); // 创建一个工作表
// 设置单元格的文字格式
WritableFont wf = new WritableFont(WritableFont.ARIAL,12,WritableFont.NO_BOLD,false,
UnderlineStyle.NO_UNDERLINE,Colour.BLUE);
WritableCellFormat wcf = new WritableCellFormat(wf);
wcf.setVerticalAlignment(VerticalAlignment.CENTRE);
wcf.setAlignment(Alignment.CENTRE);
ws.setRowView(1, 500);
ws.addCell(new Label(0, 0, "记录编号",wcf));
ws.addCell(new Label(1, 0, "用户名", wcf));
ws.addCell(new Label(2, 0, "用户密码", wcf));
ws.addCell(new Label(3, 0, "学生姓名", wcf));
ws.addCell(new Label(4, 0, "学号", wcf));
ws.addCell(new Label(5, 0, "所属班级", wcf));
ws.addCell(new Label(6, 0, "权限", wcf));
//填充数据的内容
Student[] stu = new Student[stuService.listStu().size()];
for (int i = 0; i < stuService.listStu().size(); i++){
stu[i] = (Student)stuService.listStu().get(i);
ws.addCell(new Label(0, i + 1, stu[i].getStuID().toString()));
System.out.println(stu[i].getStuID().toString());
ws.addCell(new Label(1, i + 1, stu[i].getStuName()));
ws.addCell(new Label(2, i + 1, stu[i].getStuPwd()));
ws.addCell(new Label(3, i + 1, stu[i].getRealName()));
ws.addCell(new Label(4, i + 1, stu[i].getStuNum()));
ws.addCell(new Label(5, i + 1, stu[i].getGrade()));
ws.addCell(new Label(6, i + 1, stu[i].getAuthority()));
if(i == 0)
wcf = new WritableCellFormat();
}
wwb.write();
wwb.close();
} catch (IOException e){
} catch (RowsExceededException e){
} catch (WriteException e){}
return "success";
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
【资源说明】 1、该资源包括项目的全部源码,下载可以直接使用! 2、本项目适合作为计算机、数学、电子信息等专业的课程设计、期末大作业和毕设项目,作为参考资料学习借鉴。 3、本资源作为“参考资料”如果需要实现其他功能,需要能看懂代码,并且热爱钻研,自行调试。 基于SSH的在线实验系统源码(在线实验部分用matlab库实现).zip
资源推荐
资源详情
资源评论
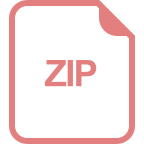
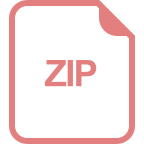
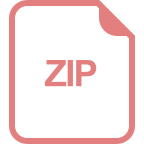
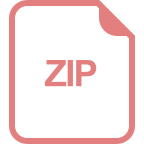
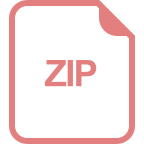
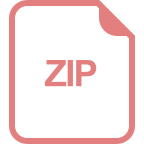
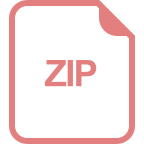
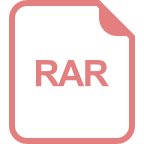
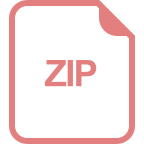
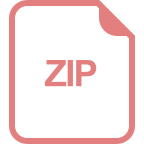
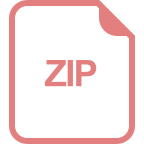
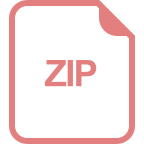
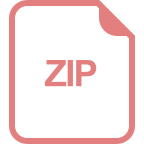
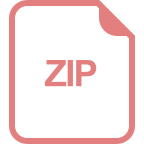
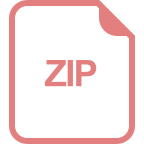
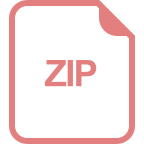
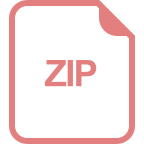
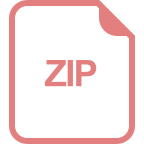
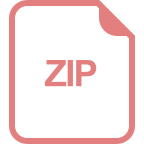
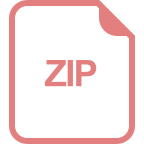
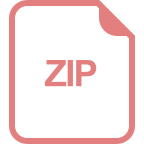
收起资源包目录

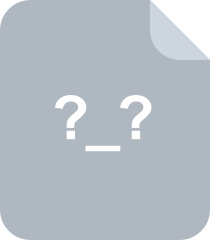
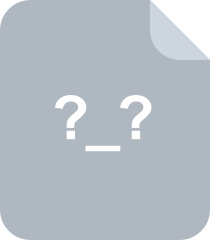
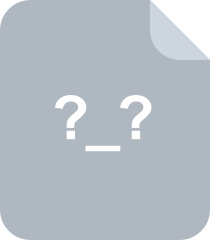
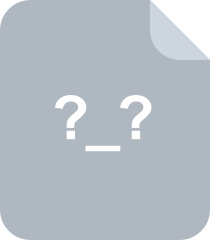
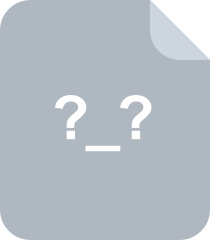
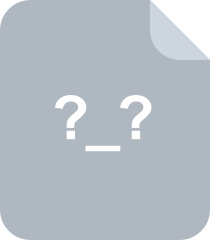
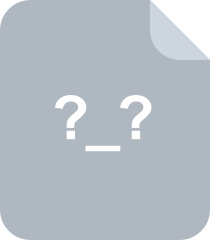
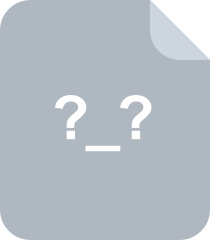
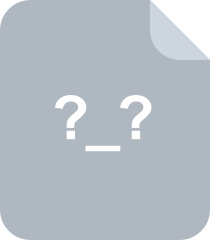
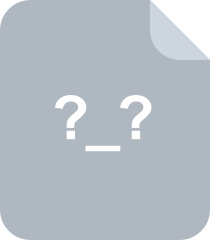
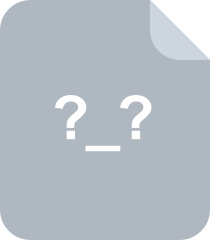
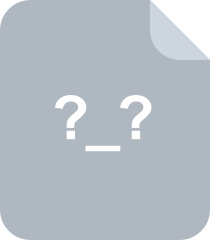
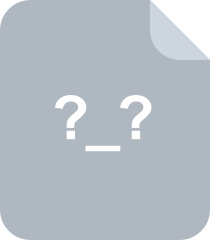
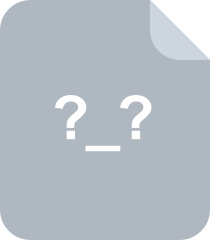
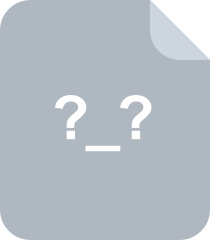
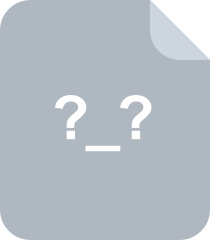
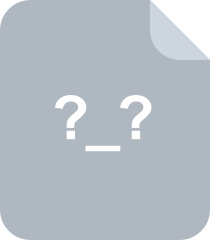
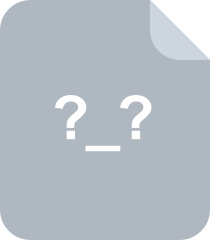
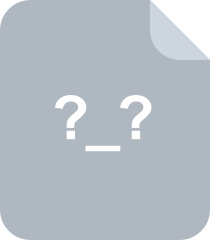
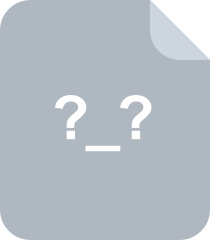
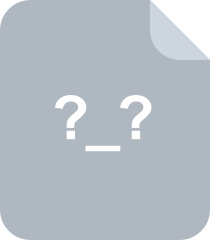
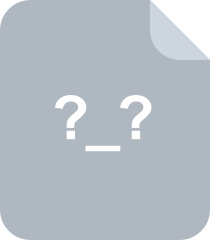
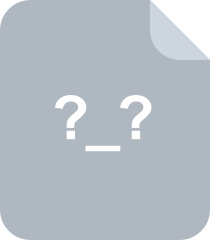
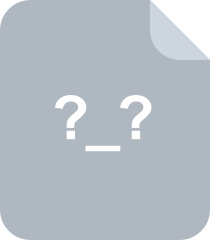
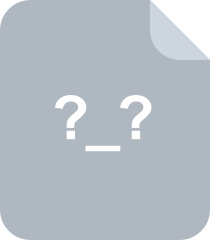
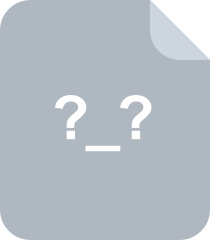
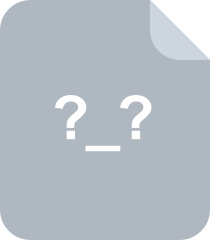
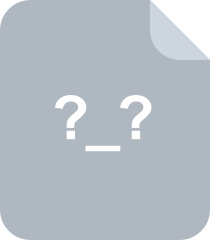
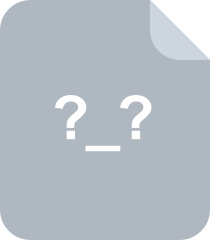
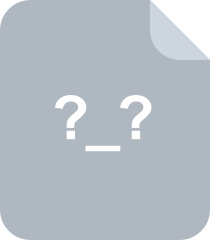
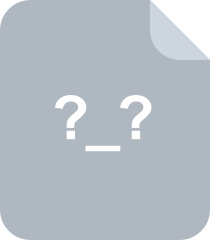
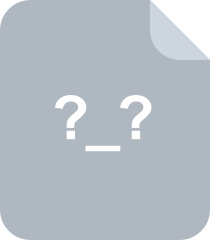
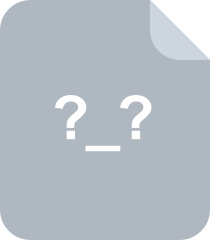
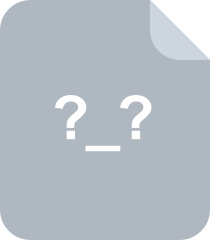
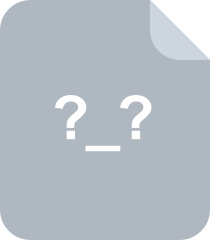
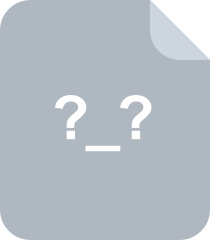
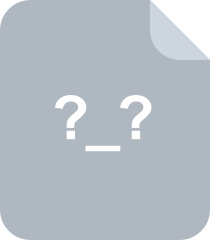
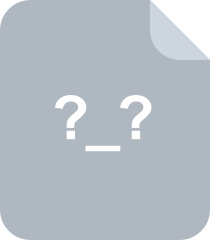
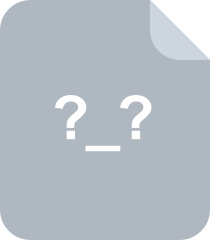
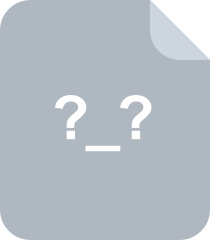
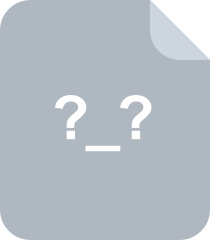
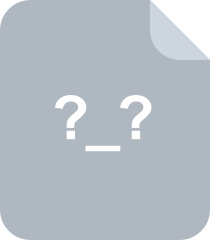
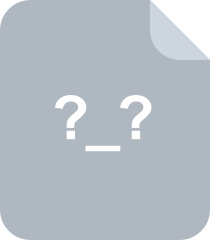
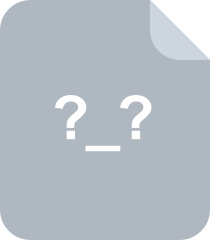
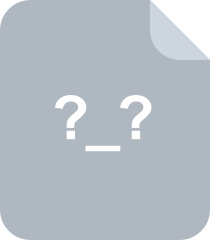
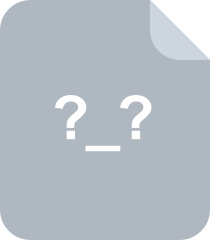
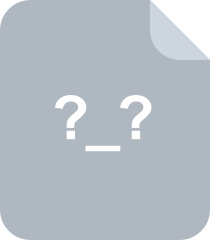
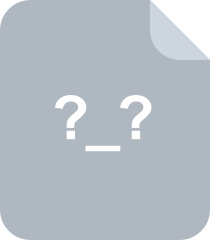
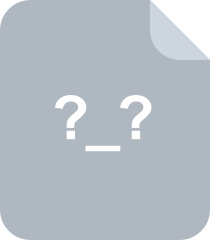
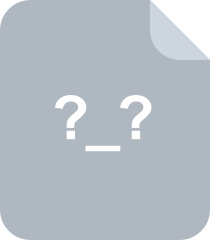
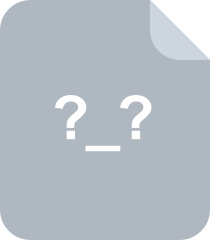
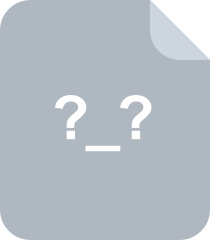
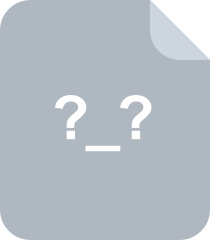
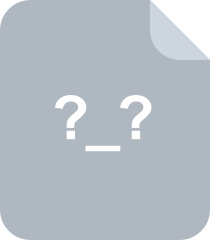
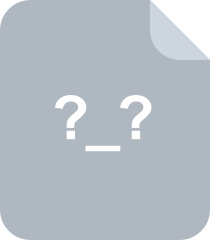
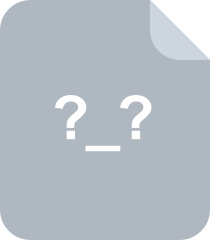
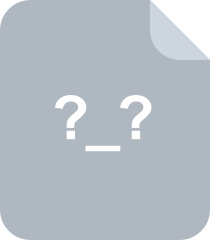
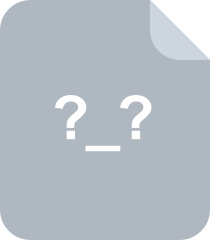
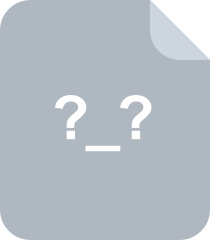
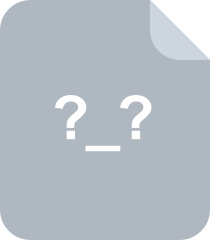
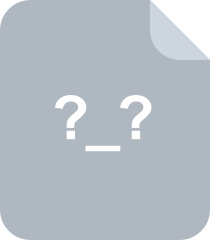
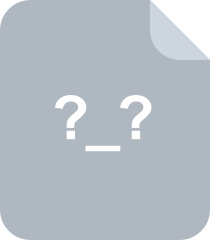
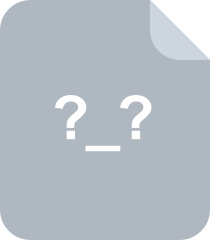
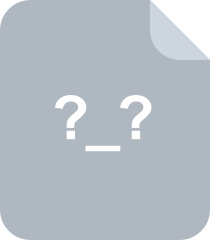
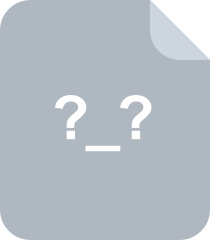
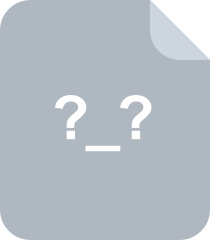
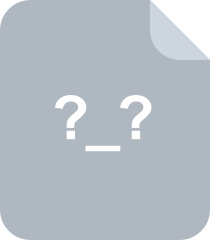
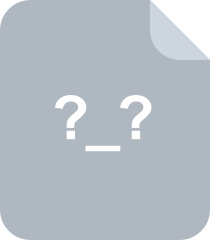
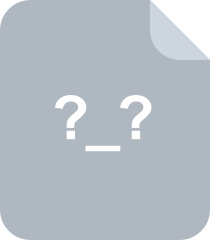
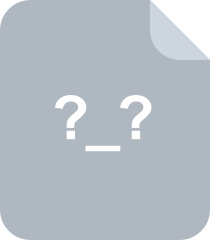
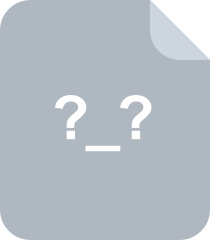
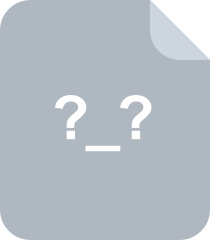
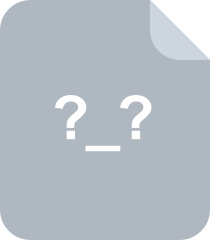
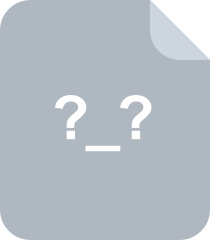
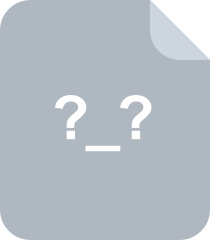
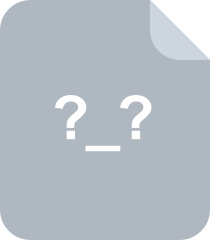
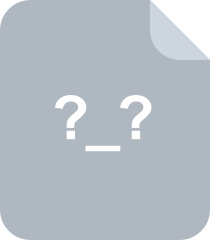
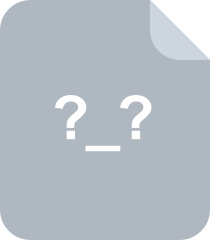
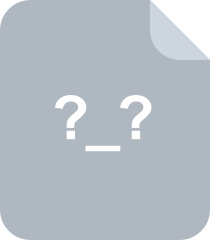
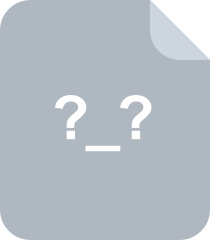
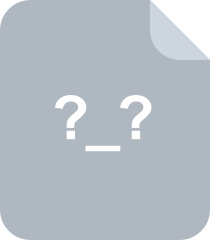
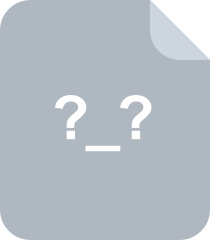
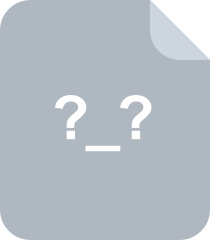
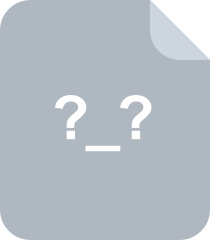
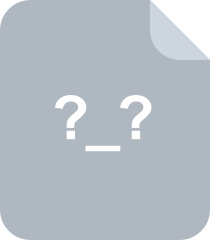
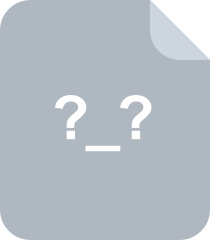
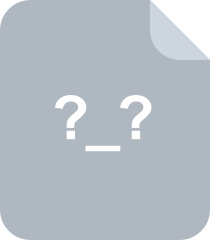
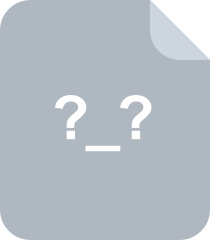
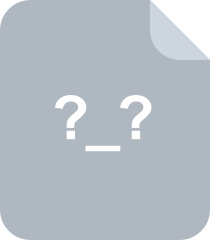
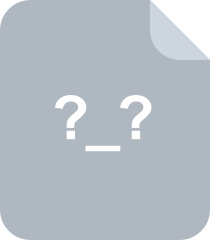
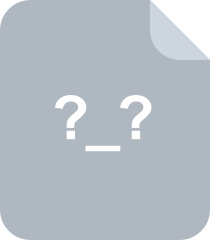
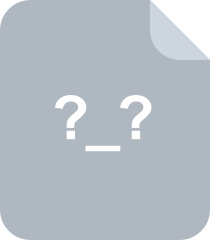
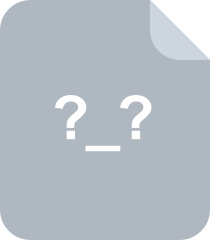
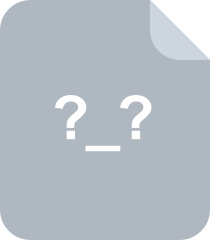
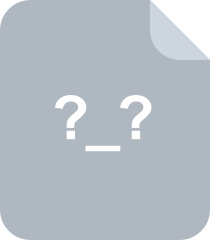
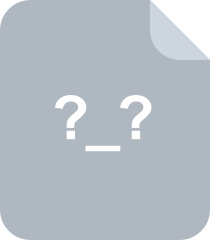
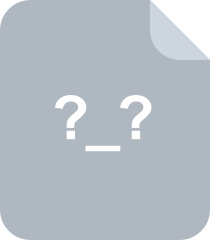
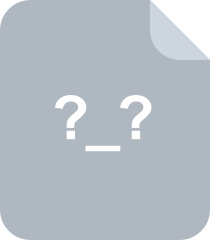
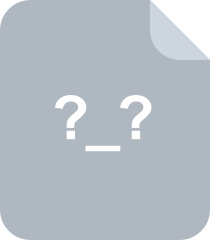
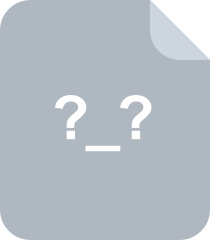
共 579 条
- 1
- 2
- 3
- 4
- 5
- 6
资源评论
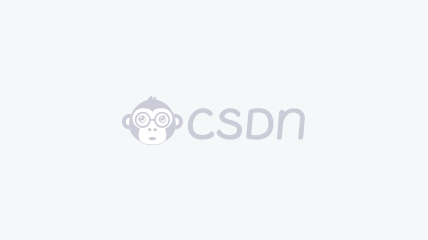

土豆片片
- 粉丝: 1851
- 资源: 5869
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

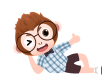
最新资源
- 车辆、人员、标志检测26-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 一款完全免费的屏幕水印工具
- 基于PLC的空调控制原理图
- 基于VUE的短视频推荐系统
- Windows环境下Hadoop安装配置与端口管理指南
- 起重机和汽车检测17-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord、VOC数据集合集.rar
- XAMPP 是一个免费且易于安装的Apache发行版
- 汽车软件需求开发与管理-从需求分析到实现的全流程解析
- 汽车电子中的证书认证需求及CANoe测试工程实践
- Cursor Setup 0.43.6 - Build
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


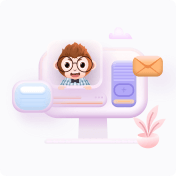
安全验证
文档复制为VIP权益,开通VIP直接复制
