package flight.file;
import dao.FB_db;
import dao.FB_id;
import java.io.*;
import java.io.IOException;
import java.io.PrintWriter;
import java.io.UnsupportedEncodingException;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.Iterator;
import java.util.List;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import org.jfree.chart.ChartFactory;
import org.jfree.chart.JFreeChart;
import org.jfree.chart.plot.PlotOrientation;
import org.jfree.chart.servlet.ServletUtilities;
import org.jfree.data.DefaultCategoryDataset;
import org.jfree.data.DefaultPieDataset;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import flight.dao.FlightFile;
import flight.dao.FileDao;
import utility.LogEvent;
import utility.MD5Util;
//根据前端发来的action指令采取对应措施
//将数据库取出的数据jsonList传到前端
public class ServletAction extends HttpServlet {
public String module = "flight";
public String sub = "file";
public SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
public LogEvent flightLog = new LogEvent();
public void service(HttpServletRequest request, HttpServletResponse response) throws IOException, ServletException {
try {
processAction(request,response);
} catch (Exception e) {
e.printStackTrace();
}
}
public void processAction(HttpServletRequest request, HttpServletResponse response) throws IOException, ServletException {
request.setCharacterEncoding("UTF-8");
String action = request.getParameter("action");
showDebug("processAction收到的action是:"+action);
if (action.equals("get_record")){
try {
getRecord(request, response);
} catch (SQLException e) {
e.printStackTrace();
} catch (JSONException e) {
e.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
}
}
else if(action.equals("delete_record")) {
try {
deleteRecord(request, response);
} catch (SQLException e) {
e.printStackTrace();
} catch (JSONException e) {
e.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
}
}
else if(action.equals("modify_record")) {
try {
modifyRecord(request, response);
} catch (SQLException e) {
e.printStackTrace();
} catch (JSONException e) {
e.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
}
}
else if(action.equals("add_record")) {
try {
addRecord(request, response);
} catch (SQLException e) {
e.printStackTrace();
} catch (JSONException e) {
e.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
}
}
}
public void processError(HttpServletRequest request, HttpServletResponse response,int errorNo,String errorMsg) throws JSONException, IOException{
String action = request.getParameter("action");
//errorNo=0->没有错误
//errorNo=1->action是空值
//errorNo=2->没有对应的处理该action的函数
//errorNo=3->session超时
showDebug("错误信息:"+errorMsg+",代码:"+errorNo);
JSONObject jsonObj=new JSONObject();
boolean isAjax=true;
if(request.getHeader("x-requested-with")==null){isAjax=false;} //判断是异步请求还是同步请求
if(isAjax){
jsonObj.put("result_code",errorNo);
jsonObj.put("result_msg",errorMsg);
jsonObj.put("action",action);
response.setContentType("application/json; charset=UTF-8");
PrintWriter out;
try {
out = response.getWriter();
out.print(jsonObj);
out.flush();
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}else{
errorMsg = java.net.URLEncoder.encode(errorMsg, "UTF-8");
String url = errorMsg;
showDebug(url);
response.sendRedirect(url);
}
}
public void showDebug(String msg){
System.out.println("["+(new SimpleDateFormat("yyyy-MM-dd HH:mm:ss")).format(new Date())+"]["+module+"/ServletAction]"+msg);
}
//从前端获取数据存入变量中,用以调用数据库其他数据
public void getRecord(HttpServletRequest request, HttpServletResponse response) throws Exception {
HttpSession session = request.getSession();
String existResultset= request.getParameter("exist_resultset");
if((existResultset==null) ||(existResultset.equals("null") || existResultset.isEmpty())) existResultset="0";
//获取userID和username
String userId=session.getAttribute("userID")==null?null:(String)session.getAttribute("userID");
String srcPlace=request.getParameter("srcPlace");
String dstPlace=request.getParameter("dstPlace");
String srcDate=request.getParameter("srcDate");
System.out.println(srcPlace);
System.out.println(dstPlace);
System.out.println(srcDate);
//数据获取完毕,开始和数据库交互
FlightFile query=new FlightFile();
query.setDbName("FlightBooking");
query.setUserID(userId);
query.setSrcPlace(srcPlace);
query.setDstPlace(dstPlace);
query.setSrcDate(srcDate);
JSONObject jsonObj=null;
if(existResultset.equals("1")){
//要求提取之前查询结果,如果有就取出来,如果没有就重新查询一次,并且保存进session里
if(session.getAttribute(module+"_"+sub+"_get_record_result")!=null){
jsonObj=(JSONObject)session.getAttribute(module+"_"+sub+"_get_record_result");
}else{
//没有就报错
jsonObj=new JSONObject();
jsonObj.put("result_code",10);
jsonObj.put("result_msg","exist_resultset参数不当,服务器当前没有结果数据,请重新设置!");
}
}else{
//如果是新查询
FileDao fileDao=new FileDao();
jsonObj = fileDao.getRecord(query);
session.setAttribute(module+"_"+sub+"_get_record_result",jsonObj);
}
jsonObj.put("userID",userId);
//数据查询完毕,根据交互方式返回数据
boolean isAjax=true;
if(request.getHeader("x-requested-with")==null){isAjax=false;} //判断是异步请求还是同步请求
if(isAjax){
response.setContentType("application/json; charset=UTF-8");
try {
PrintWriter out = response.getWriter();
out.print(jsonObj); //数据写入缓冲区
out.flush();
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}else{
String resultMsg="操作已经执行,请按返回按钮返回列表页面!";
int resultCode=0;
resultMsg=java.net.URLEncoder.encode(resultMsg, "UTF-8");
//String url = redirectPath+"/"+redirectUrl;
showDebug(resultMsg);
//response.sendRedirect(url);
}
}
public void deleteRecord(HttpServletRequest request, HttpServletResponse response)

土豆片片
- 粉丝: 1853
- 资源: 5869
最新资源
- NSArgumentNullException如何解决.md
- VueError解决办法.md
- buvid、did参数生成算法
- tiny-cuda-cnn.zip
- 关于月度总结的PPT模板
- 手表品牌与型号数据集,手表型号数据
- 基于Java实现(IDEA)的贪吃蛇游戏-源码+jar文件+项目报告
- 数字按键3.2考试代码
- 颜色拾取器 for Windows
- 台球检测40-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- # 基于MATLAB的导航科学计算库
- Qt源码ModbusTCP 主机客户端通信程序 基于QT5 QWidget, 实现ModbusTCP 主机客户端通信,支持以下功能: 1、支持断线重连 2、通过INI文件配置自定义服务器I
- tesseract ocr 训练相关的环境部署包,包括jdk-8u331-windows-x64.exe、jTessBoxEditorFX-2.6.0.zip 等
- 好用的Linux终端管理工具,支持自定义多行脚本命令,密码保存、断链续接,SFTP等功能
- 大学毕业设计写作与答辩指南:选题、研究方法及PPT制作
- 小偏差线性化模型,航空发动机线性化,非线性系统线性化,求解线性系统具体参数,最小二乘拟合 MATLAB Simulink 航空发动机,非线性,线性,非线性系统,线性系统,最小二乘,拟合,小偏差,系统辨
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


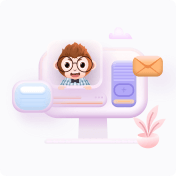