package jdbc;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.List;
import util.DBConnection;
public class StudentCRUD {
//add
public static void add( String stuid,String sname,String sex,String age,String birthday,String classname) throws SQLException {
Connection conn = null;
PreparedStatement ps = null;
ResultSet rs = null;
try{
conn = DBConnection.getConnection();
String sql = "insert into student(stuid,sname,sex,age,birthday,classname) values(?,?,?,?,?,?)";
ps = conn.prepareStatement(sql);
ps.setString(1, stuid);
ps.setString(2, sname);
ps.setString(3, sex);
ps.setString(4, age);
ps.setString(5, birthday);
ps.setString(6, classname);
ps.executeUpdate();
}finally{
DBConnection.close(rs, ps, conn);
}
}
//add with transaction
/*public static void addwithtrans() throws SQLException {
Connection conn = null;
PreparedStatement ps1 = null;
PreparedStatement ps2 = null;
ResultSet rs = null;
try{
conn = DBConnection.getConnection();
conn.setAutoCommit(false);
String sql1 = "insert into user(id,name,password,sex,age,birthday) values(2,'zhangsan','123','man',21,'1990-12-31')";
String sql2="insert into student(stuid,name,sex,age,birthday) values(2,'zhangsan','man',21,'1990-12-31')";
ps1 = conn.prepareStatement(sql1);
ps1.executeUpdate();
ps2 = conn.prepareStatement(sql2);
ps2.executeUpdate();
conn.commit();
}catch(SQLException e){
conn.rollback();
}finally{
conn.setAutoCommit(true);
DBConnection.close(null, ps1, null);
DBConnection.close(rs, ps2, conn);
}
}*/
//update
/*public static void update() throws SQLException {
Connection conn = null;
Statement st = null;
ResultSet rs = null;
try{
conn = DBConnection.getConnection();
String sql = "update student set sname='lisi' where stuid='stuid'";
st = conn.createStatement();
st.executeUpdate(sql);
}finally{
DBConnection.close(rs, st, conn);
}
}*/
//delete
/*public static void delete() throws SQLException {
Connection conn = null;
Statement st = null;
ResultSet rs = null;
try{
conn = DBConnection.getConnection();
String sql = "delete from student where stuid='stuid'";
st = conn.createStatement();
st.executeUpdate(sql);
}finally{
DBConnection.close(rs, st, conn);
}
}*/
//get
/*public static void get() throws SQLException {
Connection conn = null;
Statement st = null;
ResultSet rs = null;
try{
conn = DBConnection.getConnection();
String sql = "select stuid,sname, sex,age,birthday,classname from student";
st = conn.createStatement();
rs = st.executeQuery(sql);
while(rs.next()){
int id=rs.getInt("id");
String name=rs.getString("name");
String password=rs.getString("password");
String sex=rs.getString("sex");
int age=rs.getInt("age");
Date birthday = rs.getDate("birthday");
System.out.println("id=" + id + ";name=" + name + ";password="
+ password + ";sex=" + sex + ";age=" + age + ";birthday="
+ birthday);
}
}finally{
DBConnection.close(rs, st, conn);
}
}*/
public static List<Student> get()throws SQLException {
List<Student> stuList = new ArrayList<Student>();
Connection conn =null;
Statement st = null;
ResultSet rs = null;
try{
conn = DBConnection.getConnection();
String sql = "select * from student;";
st = conn.createStatement();
rs = st.executeQuery(sql);
while (rs!=null&&rs.next()) {
Student stu = new Student();
String stuid = rs.getString("stuid");
String sname = rs.getString("sname");
String sex = rs.getString("sex");
int age = rs.getInt("age");
String birthday = rs.getString("birthday");
String classname = rs.getString("classname");
stu.setStuid(stuid);
stu.setSname(sname);
stu.setSex(sex);
stu.setAge(age);
stu.setBirthday(birthday);
stu.setClassname(classname);
stuList.add(stu);
}
}catch(Exception e){
e.printStackTrace();
System.out.println(e.getMessage());
}
return stuList;
}
public static Student get(String stuid)throws SQLException{
Connection conn = null;
PreparedStatement ps = null;
ResultSet rs = null;
Student stu = new Student();
try{
conn = DBConnection.getConnection();
String sql = "select sname,sex,age,birthday,classname from student where stuid=?";
ps = conn.prepareStatement(sql);
ps.setString(1, stuid);
rs = ps.executeQuery();
while(rs.next()){
String sname = rs.getString("sname");
String sex = rs.getString("sex");
int age = rs.getInt("age");
String birthday = rs.getString("birthday");
String classname = rs.getString("classname");
stu.setSname(sname);
stu.setSex(sex);
stu.setAge(age);
stu.setBirthday(birthday);
stu.setClassname(classname);
}
}catch(SQLException e){
System.out.println("错误:"+e.getMessage());
}finally{
DBConnection.close(rs, ps, conn);
}
return stu;
}
public static boolean update(String stuid,String sname,String sex,String age,String birthday,String classname) throws SQLException{
Connection conn = null;
PreparedStatement ps = null;
boolean successflag=false;
try{
conn = DBConnection.getConnection();
String sql = "update student set sname=?,sex=?,age=?,birthday=?,classname=? where stuid=?";
ps = conn.prepareStatement(sql);
ps.setString(1, sname);
ps.setString(2, sex);
ps.setString(3, age);
ps.setString(4, birthday);
ps.setString(5, classname);
ps.setString(6, stuid);
ps.executeUpdate();
successflag=true;
}catch(SQLException e){
System.out.println("错误"+e.getMessage());
}finally{
DBConnection.close(null, ps, conn);
}
return successflag;
}
public static boolean del(String stuid) throws SQLException{
boolean successflag = false;
Connection conn = null;
PreparedStatement ps = null;
try{
conn = DBConnection.getConnection();
String sql = "delete from student where stuid=?";
ps = conn.prepareStatement(sql);
ps.setString(1, stuid);
ps.executeUpdate();
successflag = true;
}catch(SQLException e){
System.out.println("错误"+e.getMessage());
}finally{
DBConnection.close(null, ps, conn);
}
return successflag;
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
【资源说明】 1、该资源包括项目的全部源码,下载可以直接使用! 2、本项目适合作为计算机、数学、电子信息等专业的课程设计、期末大作业和毕设项目,作为参考资料学习借鉴。 3、本资源作为“参考资料”如果需要实现其他功能,需要能看懂代码,并且热爱钻研,自行调试。 javaweb基于jsp+servlet+javabean的学校管理系统源码(高分课设).zip
资源推荐
资源详情
资源评论
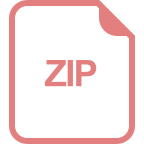
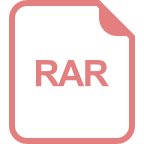
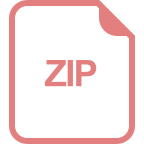
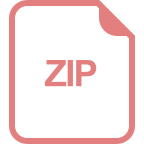
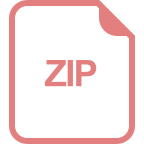
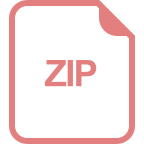
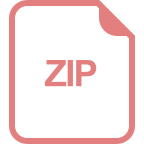
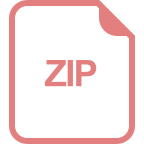
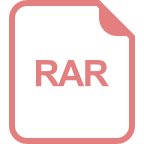
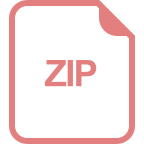
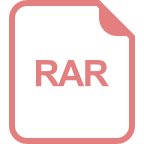
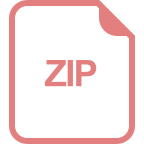
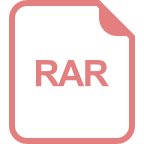
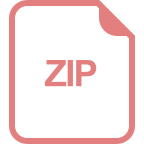
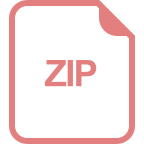
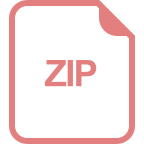
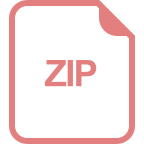
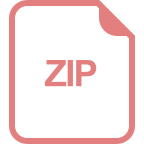
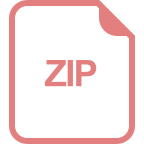
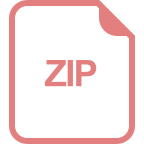
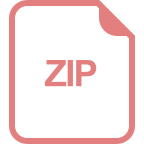
收起资源包目录


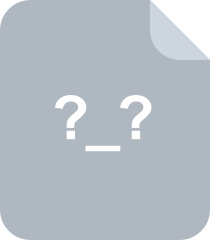

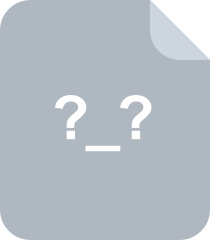
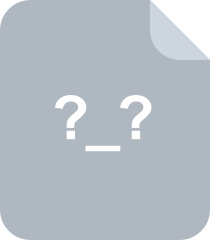
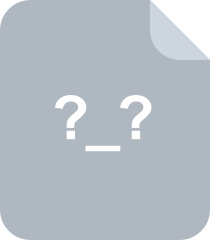
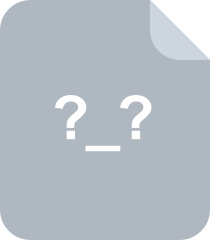
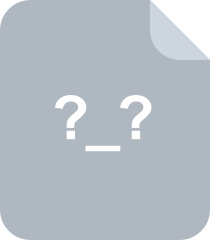
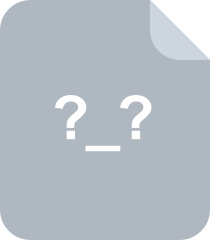
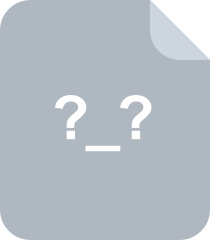


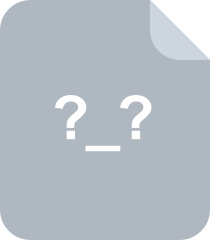
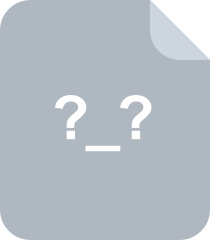
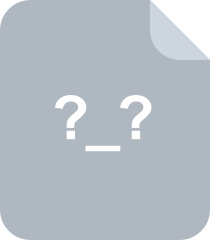
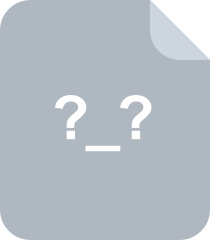

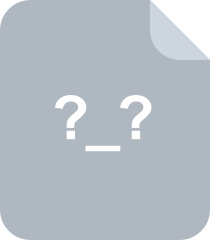
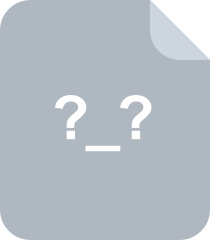

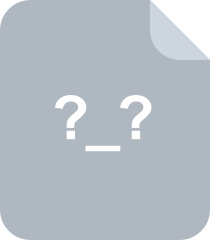
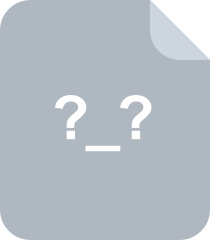

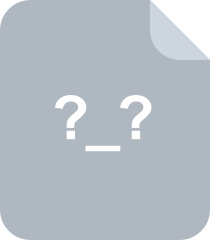

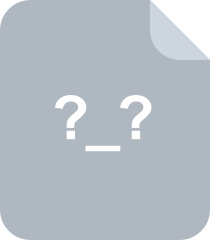
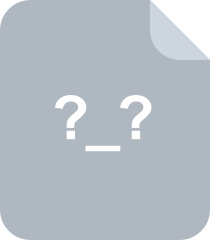
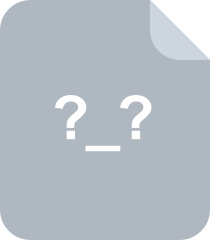
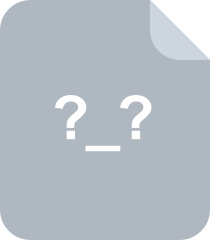

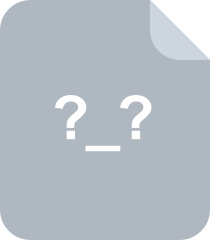
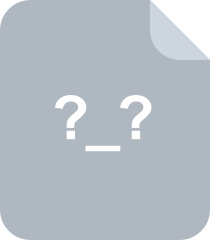
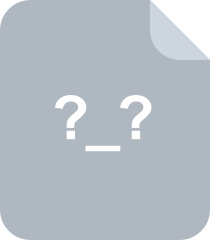

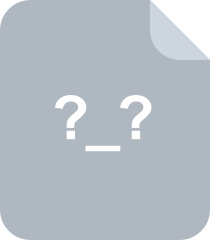
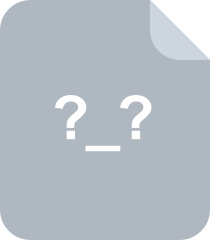
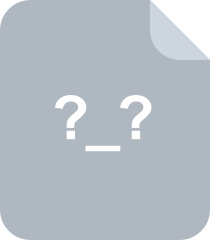
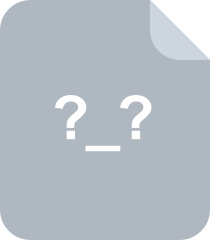
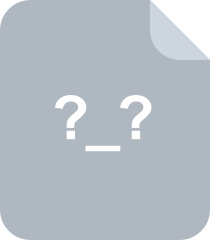
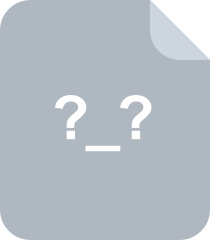
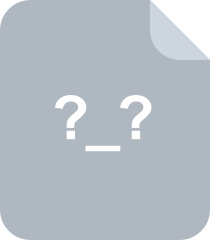


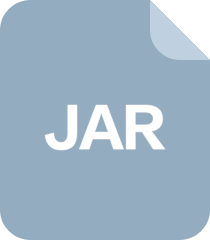
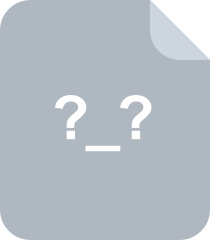
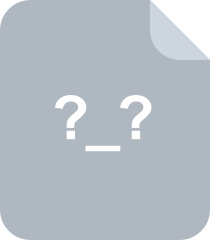
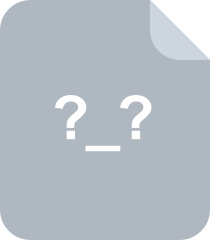

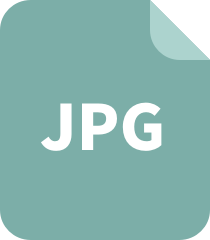
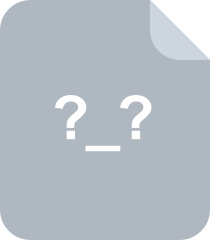

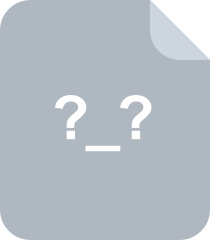
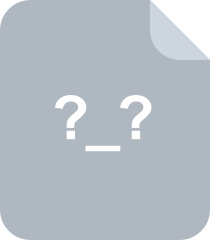
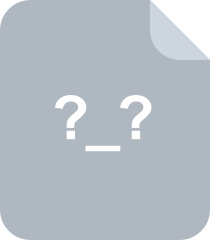
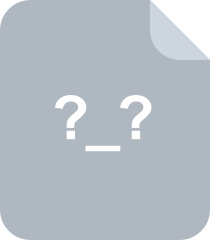
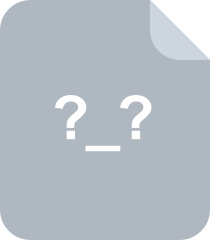
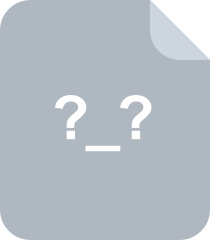
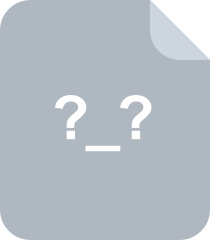
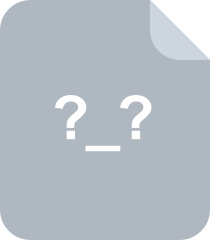
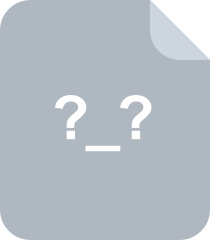
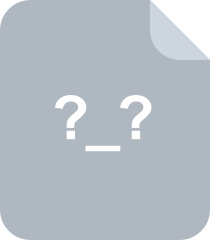
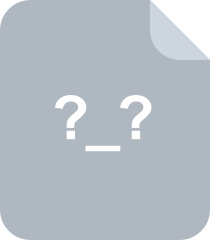
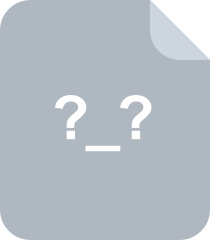
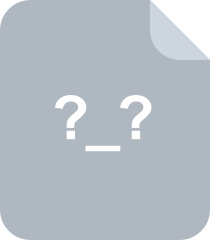

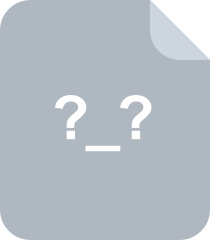
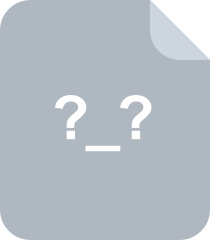
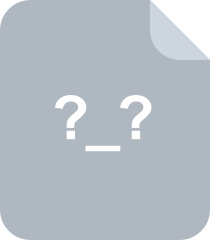
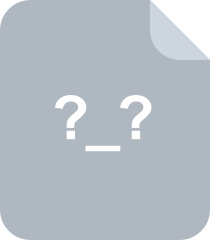
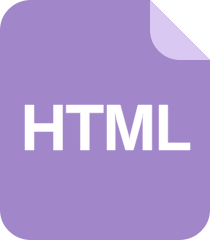
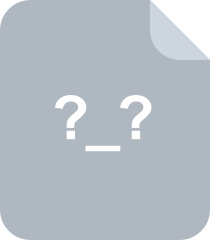
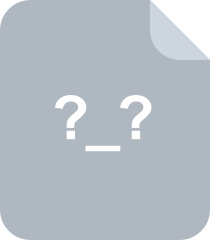
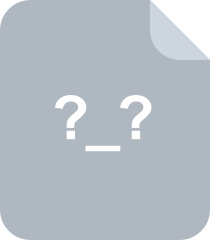
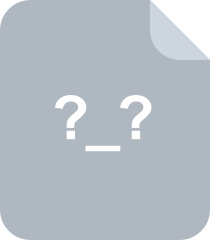
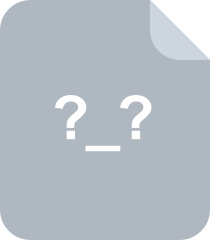
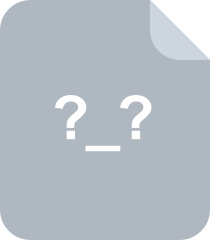
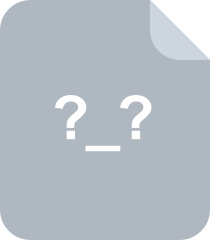

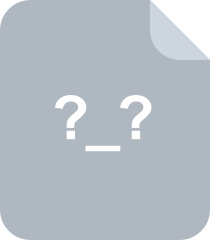
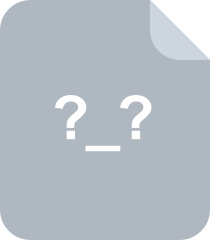
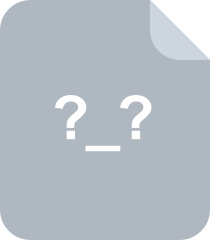
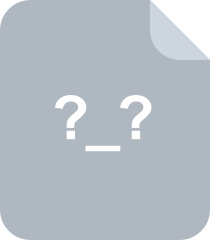
共 66 条
- 1
资源评论
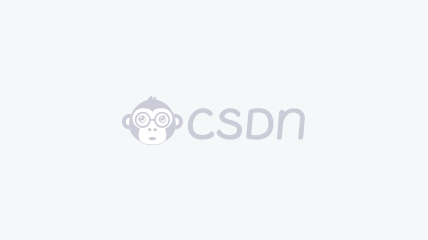

土豆片片
- 粉丝: 1844
- 资源: 5849
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

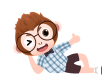
最新资源
- 用于查看,提取《命运 2》资产的多功能工具,重点是精确地重新创建渲染器.zip
- 基于 Java 实现的多任务下载器(进度条+断点续传)课程设计
- 用于更改着色器以修复 3D Vision 中的游戏的 DirectX 挂钩库.zip
- 用于托管 Discord Overlay 的 DirectX 11 窗口.zip
- 用于开发实时图形应用程序的现代 C++14 库.zip
- 用于处理 DirectX .x 文件的 .Net 库 .zip
- 用于增强现实 Oculus Rift 的 DirectX 立体渲染引擎.zip
- 用于制作 directx 和 opengl 屏幕截图的 Qt 库 .zip
- 用于优化各种 DirectX 数学函数的游乐场.zip
- 用于从 DirectX 应用程序中提取网格和纹理数据的命令行工具 .zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


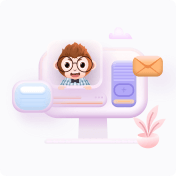
安全验证
文档复制为VIP权益,开通VIP直接复制
