/*M///////////////////////////////////////////////////////////////////////////////////////
//
// IMPORTANT: READ BEFORE DOWNLOADING, COPYING, INSTALLING OR USING.
//
// By downloading, copying, installing or using the software you agree to this license.
// If you do not agree to this license, do not download, install,
// copy or use the software.
//
//
// License Agreement
// For Open Source Computer Vision Library
//
// Copyright (C) 2000-2008, Intel Corporation, all rights reserved.
// Copyright (C) 2009, Willow Garage Inc., all rights reserved.
// Third party copyrights are property of their respective owners.
//
// Redistribution and use in source and binary forms, with or without modification,
// are permitted provided that the following conditions are met:
//
// * Redistribution's of source code must retain the above copyright notice,
// this list of conditions and the following disclaimer.
//
// * Redistribution's in binary form must reproduce the above copyright notice,
// this list of conditions and the following disclaimer in the documentation
// and/or other materials provided with the distribution.
//
// * The name of the copyright holders may not be used to endorse or promote products
// derived from this software without specific prior written permission.
//
// This software is provided by the copyright holders and contributors "as is" and
// any express or implied warranties, including, but not limited to, the implied
// warranties of merchantability and fitness for a particular purpose are disclaimed.
// In no event shall the Intel Corporation or contributors be liable for any direct,
// indirect, incidental, special, exemplary, or consequential damages
// (including, but not limited to, procurement of substitute goods or services;
// loss of use, data, or profits; or business interruption) however caused
// and on any theory of liability, whether in contract, strict liability,
// or tort (including negligence or otherwise) arising in any way out of
// the use of this software, even if advised of the possibility of such damage.
//
//M*/
#ifndef __OPENCV_CORE_C_H__
#define __OPENCV_CORE_C_H__
#include "opencv2/core/types_c.h"
#ifdef __cplusplus
extern "C" {
#endif
/****************************************************************************************\
* Array allocation, deallocation, initialization and access to elements *
\****************************************************************************************/
/* <malloc> wrapper.
If there is no enough memory, the function
(as well as other OpenCV functions that call cvAlloc)
raises an error. */
CVAPI(void*) cvAlloc( size_t size );
/* <free> wrapper.
Here and further all the memory releasing functions
(that all call cvFree) take double pointer in order to
to clear pointer to the data after releasing it.
Passing pointer to NULL pointer is Ok: nothing happens in this case
*/
CVAPI(void) cvFree_( void* ptr );
#define cvFree(ptr) (cvFree_(*(ptr)), *(ptr)=0)
/* Allocates and initializes IplImage header */
CVAPI(IplImage*) cvCreateImageHeader( CvSize size, int depth, int channels );
/* Inializes IplImage header */
CVAPI(IplImage*) cvInitImageHeader( IplImage* image, CvSize size, int depth,
int channels, int origin CV_DEFAULT(0),
int align CV_DEFAULT(4));
/* Creates IPL image (header and data) */
CVAPI(IplImage*) cvCreateImage( CvSize size, int depth, int channels );
/* Releases (i.e. deallocates) IPL image header */
CVAPI(void) cvReleaseImageHeader( IplImage** image );
/* Releases IPL image header and data */
CVAPI(void) cvReleaseImage( IplImage** image );
/* Creates a copy of IPL image (widthStep may differ) */
CVAPI(IplImage*) cvCloneImage( const IplImage* image );
/* Sets a Channel Of Interest (only a few functions support COI) -
use cvCopy to extract the selected channel and/or put it back */
CVAPI(void) cvSetImageCOI( IplImage* image, int coi );
/* Retrieves image Channel Of Interest */
CVAPI(int) cvGetImageCOI( const IplImage* image );
/* Sets image ROI (region of interest) (COI is not changed) */
CVAPI(void) cvSetImageROI( IplImage* image, CvRect rect );
/* Resets image ROI and COI */
CVAPI(void) cvResetImageROI( IplImage* image );
/* Retrieves image ROI */
CVAPI(CvRect) cvGetImageROI( const IplImage* image );
/* Allocates and initializes CvMat header */
CVAPI(CvMat*) cvCreateMatHeader( int rows, int cols, int type );
#define CV_AUTOSTEP 0x7fffffff
/* Initializes CvMat header */
CVAPI(CvMat*) cvInitMatHeader( CvMat* mat, int rows, int cols,
int type, void* data CV_DEFAULT(NULL),
int step CV_DEFAULT(CV_AUTOSTEP) );
/* Allocates and initializes CvMat header and allocates data */
CVAPI(CvMat*) cvCreateMat( int rows, int cols, int type );
/* Releases CvMat header and deallocates matrix data
(reference counting is used for data) */
CVAPI(void) cvReleaseMat( CvMat** mat );
/* Decrements CvMat data reference counter and deallocates the data if
it reaches 0 */
CV_INLINE void cvDecRefData( CvArr* arr )
{
if( CV_IS_MAT( arr ))
{
CvMat* mat = (CvMat*)arr;
mat->data.ptr = NULL;
if( mat->refcount != NULL && --*mat->refcount == 0 )
cvFree( &mat->refcount );
mat->refcount = NULL;
}
else if( CV_IS_MATND( arr ))
{
CvMatND* mat = (CvMatND*)arr;
mat->data.ptr = NULL;
if( mat->refcount != NULL && --*mat->refcount == 0 )
cvFree( &mat->refcount );
mat->refcount = NULL;
}
}
/* Increments CvMat data reference counter */
CV_INLINE int cvIncRefData( CvArr* arr )
{
int refcount = 0;
if( CV_IS_MAT( arr ))
{
CvMat* mat = (CvMat*)arr;
if( mat->refcount != NULL )
refcount = ++*mat->refcount;
}
else if( CV_IS_MATND( arr ))
{
CvMatND* mat = (CvMatND*)arr;
if( mat->refcount != NULL )
refcount = ++*mat->refcount;
}
return refcount;
}
/* Creates an exact copy of the input matrix (except, may be, step value) */
CVAPI(CvMat*) cvCloneMat( const CvMat* mat );
/* Makes a new matrix from <rect> subrectangle of input array.
No data is copied */
CVAPI(CvMat*) cvGetSubRect( const CvArr* arr, CvMat* submat, CvRect rect );
#define cvGetSubArr cvGetSubRect
/* Selects row span of the input array: arr(start_row:delta_row:end_row,:)
(end_row is not included into the span). */
CVAPI(CvMat*) cvGetRows( const CvArr* arr, CvMat* submat,
int start_row, int end_row,
int delta_row CV_DEFAULT(1));
CV_INLINE CvMat* cvGetRow( const CvArr* arr, CvMat* submat, int row )
{
return cvGetRows( arr, submat, row, row + 1, 1 );
}
/* Selects column span of the input array: arr(:,start_col:end_col)
(end_col is not included into the span) */
CVAPI(CvMat*) cvGetCols( const CvArr* arr, CvMat* submat,
int start_col, int end_col );
CV_INLINE CvMat* cvGetCol( const CvArr* arr, CvMat* submat, int col )
{
return cvGetCols( arr, submat, col, col + 1 );
}
/* Select a diagonal of the input array.
(diag = 0 means the main diagonal, >0 means a diagonal above the main one,
<0 - below the main one).
The diagonal will be represented as a column (nx1 matrix). */
CVAPI(CvMat*) cvGetDiag( const CvArr* arr, CvMat* submat,
int diag CV_DEFAULT(0));
/* low-level scalar <-> raw data conversion functions */
CVAPI(void) cvScalarToRawData( const CvScalar* scalar, void* data, int type,
int extend_to_12 CV_DEFAULT(0) );
CVAPI(void) cvRawDataToScalar( const void* data, int type, CvScalar* scalar );
/* Allocates and initializes CvMatND header */
CVAPI(CvMatND*) cvCreateMatNDHeader( int dims, const int* sizes, int type );
/* Allo
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
一个OpenCV人脸识别的小程序matlab源码.zip 一个OpenCV人脸识别的小程序matlab源码.zip 一个OpenCV人脸识别的小程序matlab源码.zip 一个OpenCV人脸识别的小程序matlab源码.zip 一个OpenCV人脸识别的小程序matlab源码.zip 一个OpenCV人脸识别的小程序matlab源码.zip 一个OpenCV人脸识别的小程序matlab源码.zip 一个OpenCV人脸识别的小程序matlab源码.zip 一个OpenCV人脸识别的小程序matlab源码.zip 一个OpenCV人脸识别的小程序matlab源码.zip 一个OpenCV人脸识别的小程序matlab源码.zip 一个OpenCV人脸识别的小程序matlab源码.zip 一个OpenCV人脸识别的小程序matlab源码.zip 【资源说明】 1、该资源内项目代码都是经过测试运行成功,功能正常的情况下才上传的,请放心下载使用。 2、适用人群:主要针对计算机相关专业(如计科、信息安全、数据科学与大数据技术、人工智能、通信、物联网、数学、电子信息等)的同学或企业员工下载使用,具有较高的学习借鉴价值。 3、不仅适合小白学习实战练习,也可作为大作业、课程设计、毕设项目、初期项目立项演示等,欢迎下载,互相学习,共同进步!
资源推荐
资源详情
资源评论
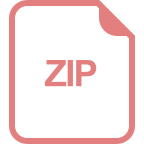
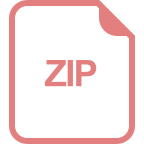
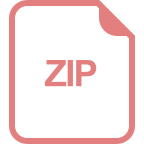
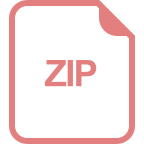
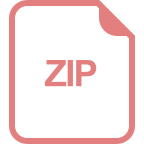
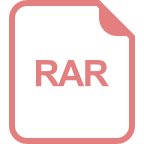
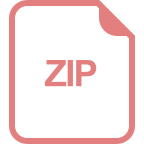
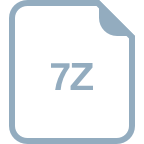
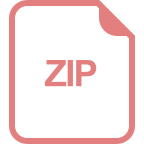
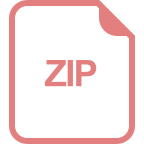
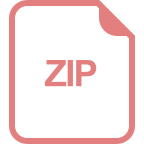
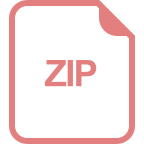
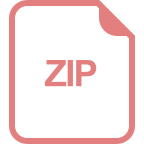
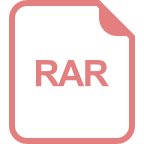
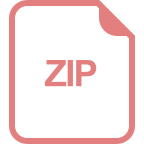
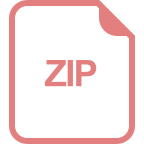
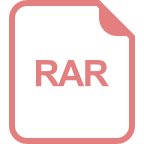
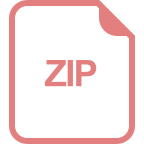
收起资源包目录

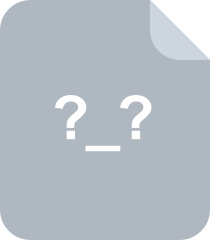
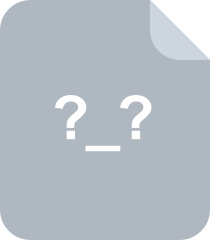
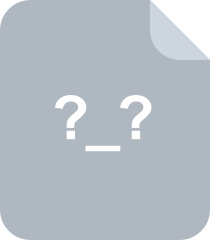
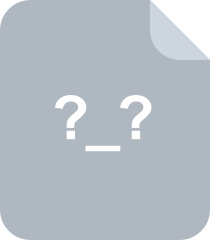
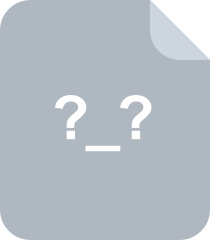
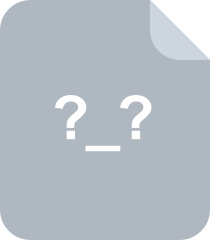
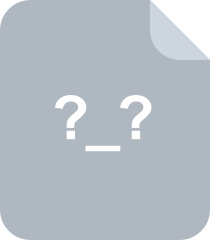
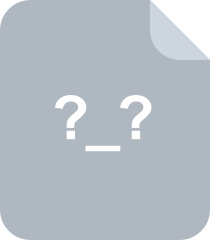
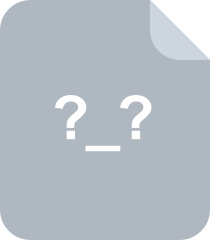
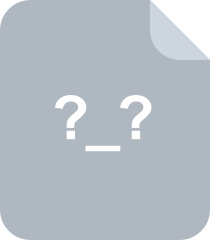
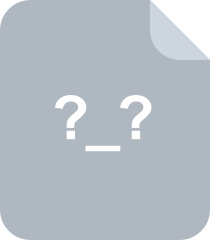
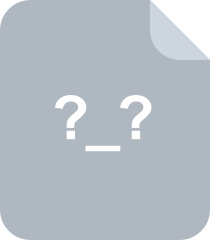
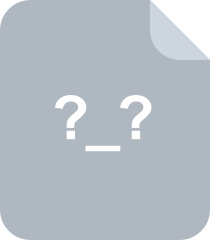
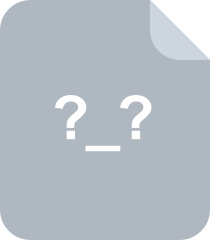
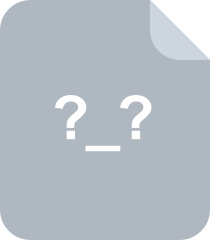
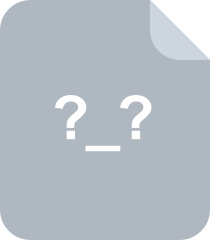
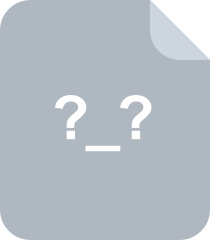
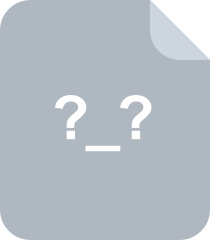
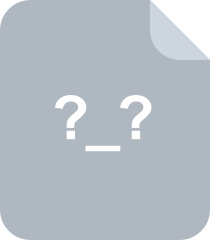
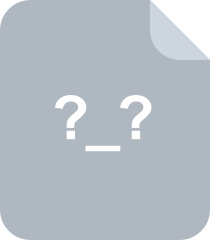
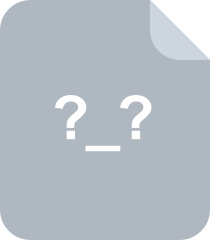
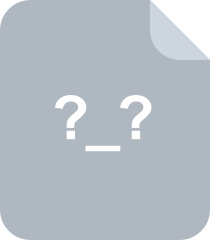
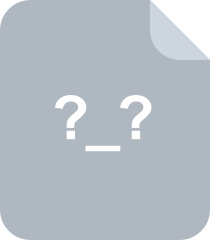
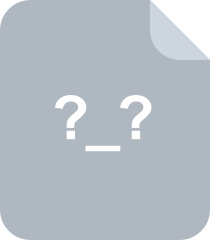
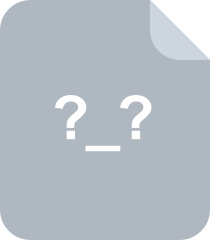
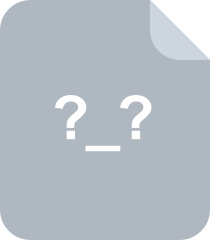
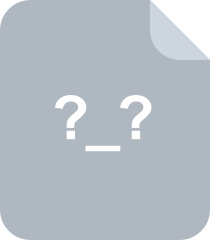
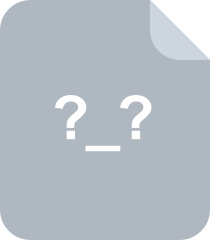
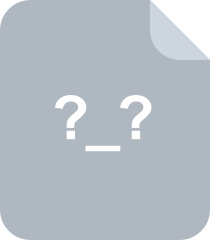
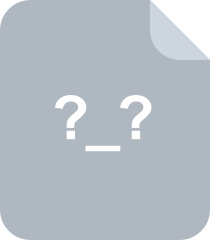
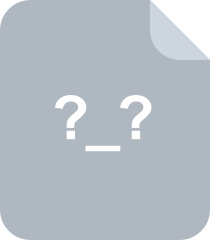
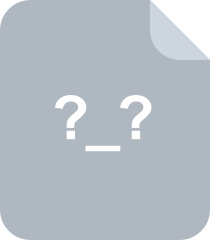
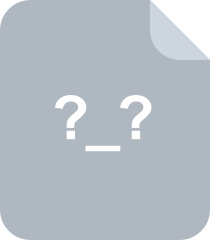
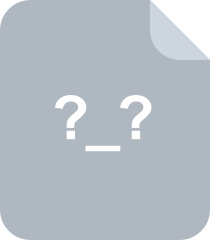
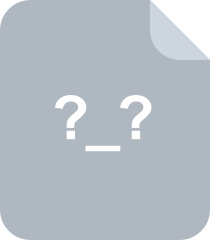
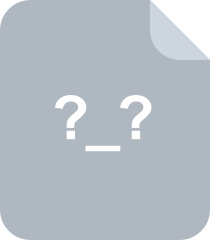
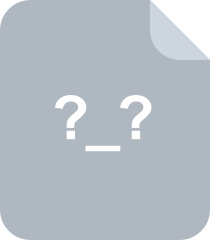
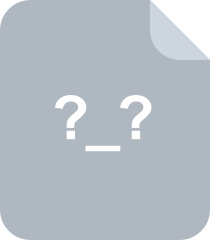
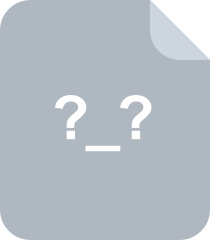
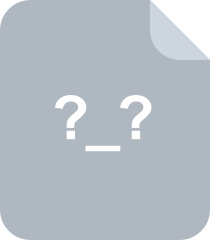
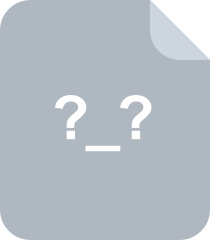
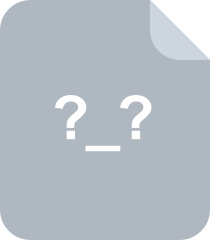
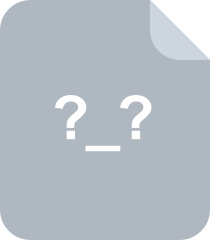
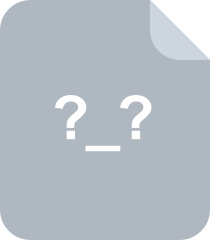
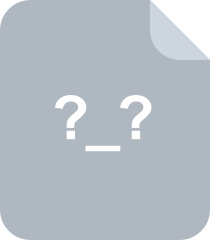
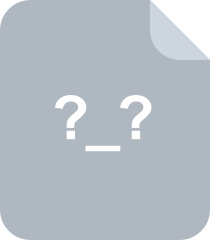
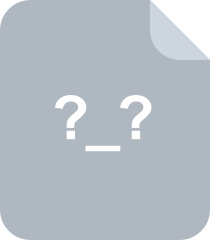
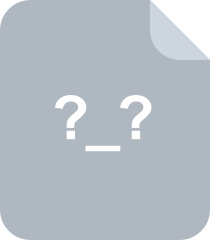
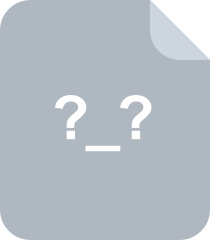
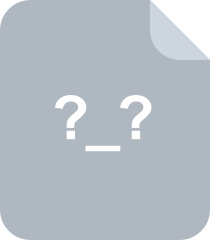
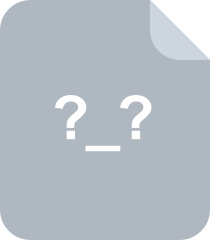
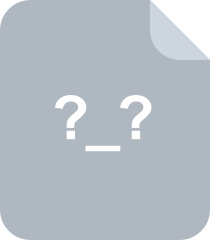
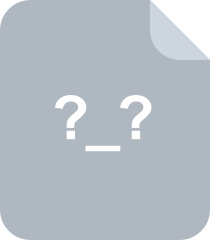
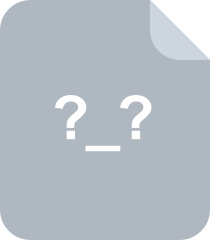
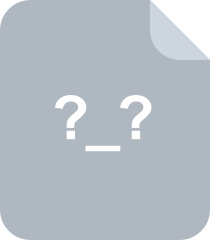
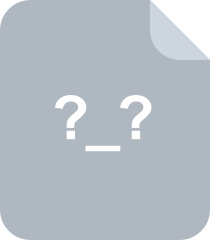
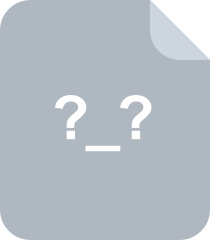
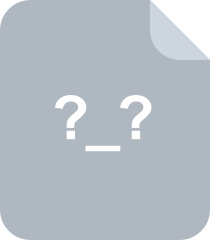
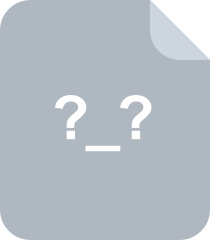
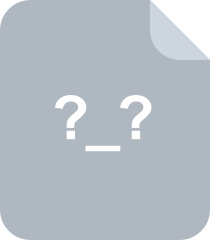
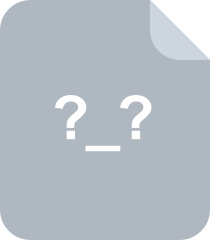
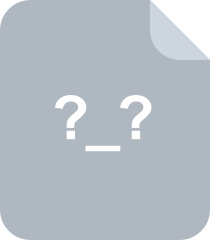
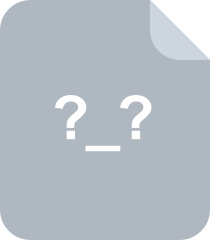
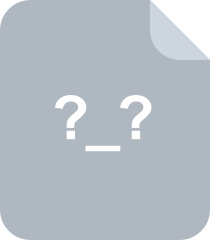
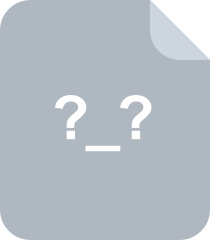
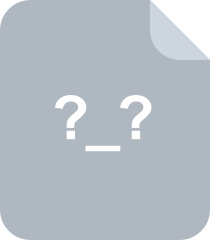
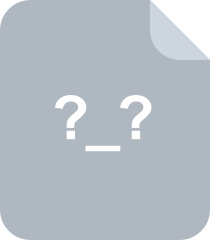
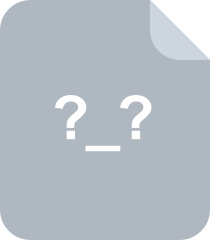
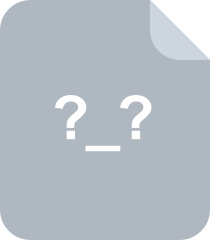
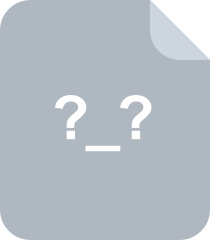
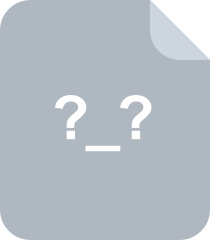
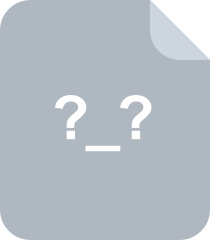
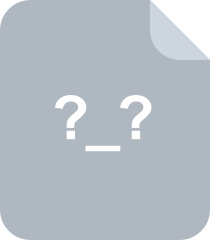
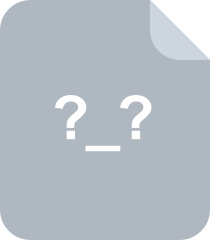
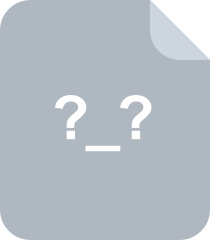
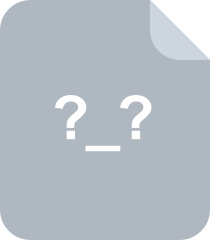
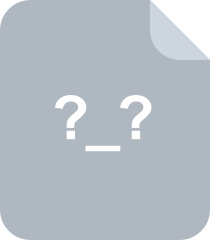
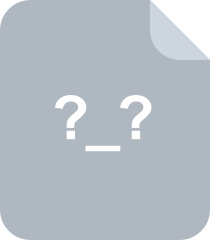
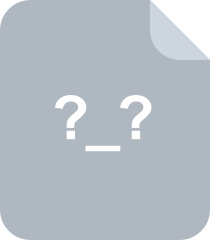
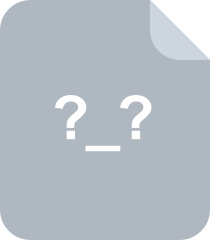
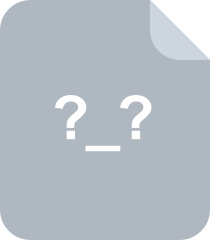
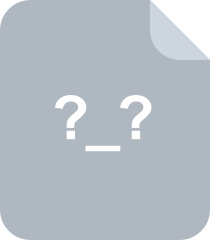
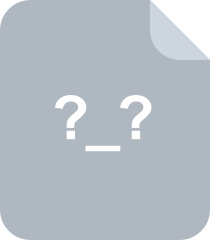
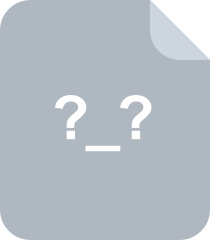
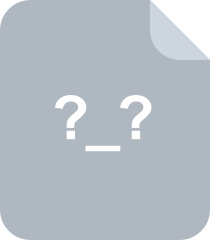
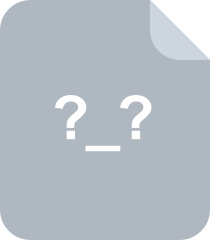
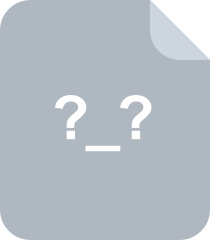
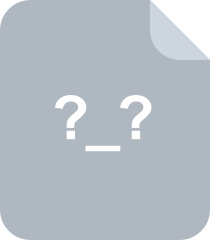
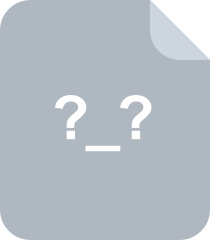
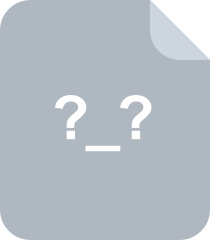
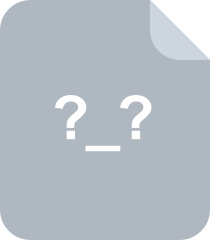
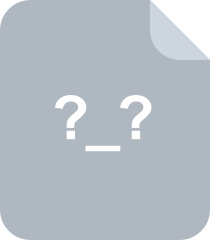
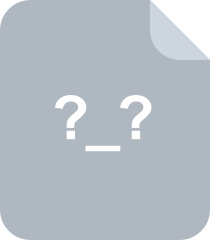
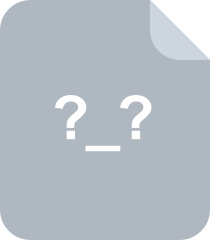
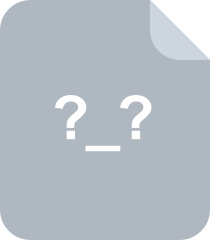
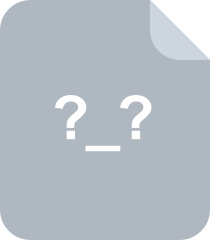
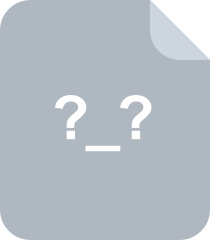
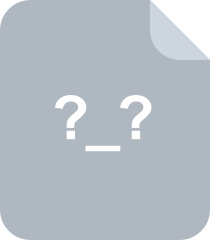
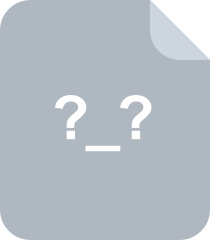
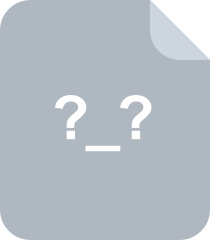
共 162 条
- 1
- 2
资源评论
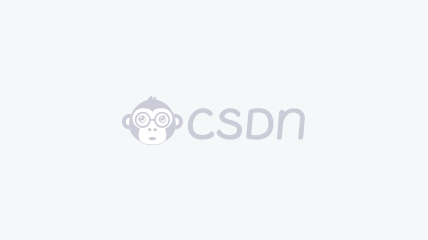

龙年行大运
- 粉丝: 1385
- 资源: 3960
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

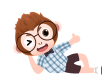
最新资源
- STM32固件库使用手册的中文翻译版
- 机械设计玉米收割台sw18可编辑非常好的设计图纸100%好用.zip
- 量子计算中的逻辑电路与算法实验
- SLAM常见数据集汇总.zip
- springboot集成netty,使用protobuf作为数据传输格式,包含心跳检测、断开重连、上传数据、主动推送功能.zip
- TiDB 数据库 DBA 常用工具集.zip
- VOC类型数据集操作库函数.zip
- 2024关于圣诞树的素材
- 如何在 Linux 上安装 Python Pycharm?
- HCNP实验室搭建指南:网络设备配置与线缆连接教程 (V2.0)
- 【Python】基于Python的微信群助手机器人.zip
- 【C#】Telegram投稿机器人,支持多图与权限管理.zip
- 【Python】一个简单、灵活、优雅的中文语音对话机器人:智能音箱项.zip
- 【Python】基于Python的用于爬取gitlab上的数据的python项目.zip
- 【C#】QQ机器人用于在群组内提供便捷的Ai对话应答服务.zip
- 【Python】一款可以工作在RaspberryPi上的中文语音对话机器人-智能音箱项目.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


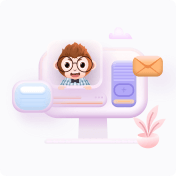
安全验证
文档复制为VIP权益,开通VIP直接复制
