package com.wang.vire.service.impl;
import com.github.pagehelper.Page;
import com.github.pagehelper.PageHelper;
import com.wang.vire.mapper.*;
import com.wang.vire.pojo.RepairApply;
import com.wang.vire.service.AuditEndService;
import com.wang.vire.service.AuditService;
import com.wang.vire.service.WangService;
import com.wang.vire.utils.EmptyChecker;
import com.wang.vire.utils.JsonUtils;
import com.wang.vire.utils.ServiceUtils;
import com.ycx.lend.pojo.Audit;
import com.ycx.lend.pojo.AuditEnd;
import com.ycx.lend.pojo.Auditor;
import com.ycx.lend.pojo.Status;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.text.ParseException;
import java.util.*;
/**
* @Author ycx
* @Date 2022/1/24 13:40
* @Description
*/
@Service
public class AuditServiceImpl implements AuditService {
@Autowired
RepairApplyMapper repairApplyMapper;
@Autowired
AuditEndService auditEndService;
@Autowired
WangService wangService;
private final String randomNum(){
String i = String.valueOf(Math.abs(new Random().nextInt()));
Object auditSelByKey = JsonUtils.JsonToPojo(wangService.auditSelByKey(i), Audit.class);
Audit auditSelByKey1 = (Audit) auditSelByKey;
if(auditSelByKey1!=null){
this.randomNum();
}
return i;
}
//派审核单到不同审核员,平均分配
@Override
public int allotAudit(String applicationId) {
Audit audit = new Audit();
if (EmptyChecker.isEmpty(applicationId)) {
return 0;
}
//判断申请编号是否存在于申请表
if (EmptyChecker.isEmpty(repairApplyMapper.selectByPrimaryKey(applicationId))) {
return -2;
}
audit.setApplicationId(applicationId);
//添加主键
audit.setAuditId(this.randomNum());
HashMap<String, Object> h1 = new HashMap<>();
h1.put("auditCount", 1000000);
//查找没有任务的审核员 分配审核
Object auditorSelAllNormal = JsonUtils.JsonToListString(wangService.auditorSelAllNormal());
List<String> auditorSelAllNormals = (List<String>) auditorSelAllNormal;
for (String s : auditorSelAllNormals) {
Object auditSelByAuditorId = JsonUtils.JsonToListPojo(wangService.auditSelByAuditorId(s), Audit.class);
List<Audit> audit1 = (List<Audit>) auditSelByAuditorId;
// List<Audit> audit1 = wangService.auditSelByAuditorId(s);
if (EmptyChecker.isEmpty(audit1)) {
h1.put("auditorId", s);
break;
}
}
if (EmptyChecker.isEmpty(h1.get("auditorId"))) {
Object auditSelNumByNormalAuditor = JsonUtils.JsonToListPojo(wangService.auditSelNumByNormalAuditor(), HashMap.class);
List<HashMap<String, Object>> auditSelNumByNormalAuditor1 = (List<HashMap<String, Object>>) auditSelNumByNormalAuditor;
for (HashMap<String, Object> hashMap : auditSelNumByNormalAuditor1) {
String auditorId = (String) hashMap.get("auditorId");
//判断该审核员是否已经被分配该审核单
Object auditSelIfAllot = JsonUtils.JsonToPojo(wangService.auditSelIfAllot(auditorId,applicationId), Audit.class);
Audit auditSelIfAllot1 = (Audit) auditSelIfAllot;
if (EmptyChecker.isEmpty(auditSelIfAllot1)){
int auditCount = ServiceUtils.NumberToInt(hashMap.get("auditCount"));
if (auditCount < (Integer) h1.get("auditCount")) {
h1.put("auditorId", auditorId);
h1.put("auditCount", auditCount);
}
}
}
}
// //如果没有审核员信息被存入,那么随机分配给一个普通审核员
// if (EmptyChecker.isEmpty(h1.get("auditorId"))) {
// while (EmptyChecker.isEmpty(auditorMapper.selectByPrimaryKey(h1.get("auditorId")))) {
// Random random = new Random();
// int i = Integer.parseInt(idService.getMinId("auditor", "auditor_id"));
// String auditorId = String.valueOf(random.nextInt(Integer.parseInt(idService.getMaxId("auditor", "auditor_id")) - i) + i);
// if (auditorMapper.selectByPrimaryKey(auditorId).getAuditorType() == 0) {
// h1.put("auditorId", auditorId);
// }
// }
// }
//过滤完成,进行赋值
audit.setAuditorId((String) h1.get("auditorId"));
Object o = wangService.auditInsertSelective(audit);
int auditInsertSelective = JsonUtils.JsonToInt(o);
// int auditInsertSelective1 = (int) auditInsertSelective;
return auditInsertSelective;
}
//将审核单分配给三个不同的人
@Override
public int allotAllAudits() {
int i = 1;
int auditCount;
for (int j = 0; j < 3; j++) {
for (String s : repairApplyMapper.queryAllApplicationId()) {
//判断有无值,无值时设置计数为0,防止空指针
Object auditSelNumByAuditorId = JsonUtils.JsonToPojo(wangService.auditSelNumByAuditorId(s), HashMap.class);
HashMap<String, Object> auditSelNumByAuditorIds = (HashMap<String, Object>) auditSelNumByAuditorId;
if (EmptyChecker.notEmpty(auditSelNumByAuditorIds)) {
//防止冗余分配
Object auditSelAuditNumAllotAuditor = JsonUtils.JsonToPojo(wangService.auditSelAuditNumAllotAuditor(s), HashMap.class);
HashMap<String, Object> countMap = (HashMap<String, Object>) auditSelAuditNumAllotAuditor;
// HashMap<String, Object> countMap = wangService.auditSelAuditNumAllotAuditor(s);
//判空
if (countMap == null) {
auditCount = 0;
} else auditCount = ServiceUtils.NumberToInt(countMap.get("applicationCount"));
} else auditCount = 0;
if (auditCount < 3)
i = allotAudit(s);
if (i <= 0) {
return i;
}
}
}
return i;
}
@Override
public int delAudit(String auditId) {
// 判空
if (EmptyChecker.isEmpty(auditId)) {
return 0;
}
// 操作对象判定
Object auditSelByKey = JsonUtils.JsonToPojo(wangService.auditSelByKey(auditId), Audit.class);
Audit auditSelByKey1 = (Audit) auditSelByKey;
if (EmptyChecker.isEmpty(auditSelByKey1)) {
return -3;
}
Object auditDelByKey = JsonUtils.JsonToInt(wangService.auditDelByKey(auditId));
int auditDelByKey1 = (int) auditDelByKey;
return auditDelByKey1;
}
@Override
public int updateAuditor(String auditId, String auditorId) {
if (EmptyChecker.isAnyOneEmpty(auditId, auditorId)) {
return 0;
}
Audit audit = new Audit();
Object auditorSelByKey = JsonUtils.JsonToPojo(wangService.auditorSelByKey(auditorId), Auditor.class);
Auditor auditorSelByKey1 = (Auditor) auditorSelByKey;
if (EmptyChecker.isEmpty(auditorSelByKey1)) {
return -2;
}
Object auditSelByKey = JsonUtils.JsonToPojo(wangService.auditSelByKey(auditId), Audit.class);
Audit auditSelByKey1 = (Audit) auditSelByKey;
if (EmptyChecker.isEmpty(auditSelByKey1)) {
return -3;
}
audit.setAuditId(auditId);
audit.setAuditorId(auditorId);
Object auditUpdSelective = JsonUtils.JsonToInt(wangService.auditUpdSelective(audit));
int auditUpdSelective1 = (int) auditUpdSelective;
return auditUpdSelective1;
}
@Override
public int changeStatus(String auditId, Integer status, String changeTime) th

龙年行大运
- 粉丝: 1402
- 资源: 3960
最新资源
- Comsol案例研究:离散裂缝网络中单相流计算的精确计算方法与结果分析,COMSOL案例研究:离散裂缝网络中的单相流计算策略与应用,comsol案例离散裂缝网络中的单相流计算 ,comsol案例; 离
- (源码)基于Arduino的机床转速显示系统.zip
- 基于达西定律流动模式的两相流体COMSOL基质裂缝双重介质案例研究,基于基质裂缝双重介质达西定律的COMSOL两相流体流动模式案例研究,comsol案例,两相流体,基于基质裂缝双重介质达西定律流动模式
- (源码)基于ESP32摄像头和OpenCV的AR对象识别系统.zip
- 基于地质统计学的岩体裂隙渗流模拟:Comsol模拟粗糙裂隙的渗流特性及优势通道研究,基于地质统计学的岩体裂隙渗流模拟:Comsol模拟粗糙裂隙的渗流特性及优势通道研究,基于地质统计学的建模comsol
- (源码)基于 React 和 CesiumJS 的无人机飞行演示系统.zip
- COMSOL模拟双层多孔介质中油类地下渗透现象:时间影响下的油扩散趋势分析,COMSOL模拟双层多孔介质中油类地下渗透现象:时间演进下的油扩散过程可视化研究,comsol模拟油往地下渗透现象,考虑两层
- COMSOL多孔介质渗漏模拟案例:物质在双相介质中流动过程的精确模拟与可视化分析,多孔介质中渗漏模拟的COMSOL案例研究:探索某相物质在双相介质环境中的流态模拟过程,comsol案例提供多孔介质中渗
- (源码)基于ESP32和MQTT的物联网数据采集与通知系统.zip
- COMSOL案例:实现隧道衬砌结构多场耦合细观损伤模型与多因素交互作用的数值模拟研究,基于COMSOL的隧道衬砌结构多场耦合细观损伤模型实现与应用:热-湿-力场三场耦合效应下的混凝土损伤研究,COMS
- (源码)基于C++的缓存一致性性能验证模型CC.zip
- 基于COMSOL软件的三维多孔介质生成方法与探究,基于COMSOL模拟的三维多孔介质生成技术研究,COMSOL生成三维多孔介质 ,COMSOL; 三维; 多孔介质; 生成,COMSOL生成三维多孔介
- (源码)基于Python的多功能网络爬虫项目.zip
- VT仿真教程升级版:多轴与车铣复合实战笔记,CIMCO Edit专业仿真解析,快速进阶高手指南,VT仿真教程大升级:多轴与车铣复合实战笔记,专业解析助你快速进阶,全面解析MC导入VT仿真设置及问题解决
- (源码)基于Java语言的树莓派多功能应用项目.zip
- 风光储联合发电系统Simulink仿真模型研究:光伏风电储能能量管理之实证与应用教学,风光储联合发电系统Simulink仿真模型:实现光伏风电储能能量管理的最佳实践及文献解析,风光储联合发电系统;光伏
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


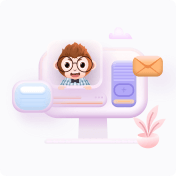