<?php
/**
* PHPExcel
*
* Copyright (c) 2006 - 2010 PHPExcel
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
*
* @category PHPExcel
* @package PHPExcel_Calculation
* @copyright Copyright (c) 2006 - 2010 PHPExcel (http://www.codeplex.com/PHPExcel)
* @license http://www.gnu.org/licenses/old-licenses/lgpl-2.1.txt LGPL
* @version ##VERSION##, ##DATE##
*/
/** PHPExcel root directory */
if (!defined('PHPEXCEL_ROOT')) {
/**
* @ignore
*/
define('PHPEXCEL_ROOT', dirname(__FILE__) . '/../../');
require(PHPEXCEL_ROOT . 'PHPExcel/Autoloader.php');
PHPExcel_Autoloader::Register();
PHPExcel_Shared_ZipStreamWrapper::register();
// check mbstring.func_overload
if (ini_get('mbstring.func_overload') & 2) {
throw new Exception('Multibyte function overloading in PHP must be disabled for string functions (2).');
}
}
/** EPS */
define('EPS', 2.22e-16);
/** MAX_VALUE */
define('MAX_VALUE', 1.2e308);
/** LOG_GAMMA_X_MAX_VALUE */
define('LOG_GAMMA_X_MAX_VALUE', 2.55e305);
/** SQRT2PI */
define('SQRT2PI', 2.5066282746310005024157652848110452530069867406099);
/** 2 / PI */
define('M_2DIVPI', 0.63661977236758134307553505349006);
/** XMININ */
define('XMININ', 2.23e-308);
/** MAX_ITERATIONS */
define('MAX_ITERATIONS', 256);
/** FINANCIAL_MAX_ITERATIONS */
define('FINANCIAL_MAX_ITERATIONS', 128);
/** PRECISION */
define('PRECISION', 8.88E-016);
/** FINANCIAL_PRECISION */
define('FINANCIAL_PRECISION', 1.0e-08);
/** EULER */
define('EULER', 2.71828182845904523536);
$savedPrecision = ini_get('precision');
if ($savedPrecision < 16) {
ini_set('precision',16);
}
/** Matrix */
require_once PHPEXCEL_ROOT . 'PHPExcel/Shared/JAMA/Matrix.php';
require_once PHPEXCEL_ROOT . 'PHPExcel/Shared/trend/trendClass.php';
/**
* PHPExcel_Calculation_Functions
*
* @category PHPExcel
* @package PHPExcel_Calculation
* @copyright Copyright (c) 2006 - 2010 PHPExcel (http://www.codeplex.com/PHPExcel)
*/
class PHPExcel_Calculation_Functions {
/** constants */
const COMPATIBILITY_EXCEL = 'Excel';
const COMPATIBILITY_GNUMERIC = 'Gnumeric';
const COMPATIBILITY_OPENOFFICE = 'OpenOfficeCalc';
const RETURNDATE_PHP_NUMERIC = 'P';
const RETURNDATE_PHP_OBJECT = 'O';
const RETURNDATE_EXCEL = 'E';
/**
* Compatibility mode to use for error checking and responses
*
* @access private
* @var string
*/
private static $compatibilityMode = self::COMPATIBILITY_EXCEL;
/**
* Data Type to use when returning date values
*
* @access private
* @var string
*/
private static $ReturnDateType = self::RETURNDATE_EXCEL;
/**
* List of error codes
*
* @access private
* @var array
*/
private static $_errorCodes = array( 'null' => '#NULL!',
'divisionbyzero' => '#DIV/0!',
'value' => '#VALUE!',
'reference' => '#REF!',
'name' => '#NAME?',
'num' => '#NUM!',
'na' => '#N/A',
'gettingdata' => '#GETTING_DATA'
);
/**
* Set the Compatibility Mode
*
* @access public
* @category Function Configuration
* @param string $compatibilityMode Compatibility Mode
* Permitted values are:
* PHPExcel_Calculation_Functions::COMPATIBILITY_EXCEL 'Excel'
* PHPExcel_Calculation_Functions::COMPATIBILITY_GNUMERIC 'Gnumeric'
* PHPExcel_Calculation_Functions::COMPATIBILITY_OPENOFFICE 'OpenOfficeCalc'
* @return boolean (Success or Failure)
*/
public static function setCompatibilityMode($compatibilityMode) {
if (($compatibilityMode == self::COMPATIBILITY_EXCEL) ||
($compatibilityMode == self::COMPATIBILITY_GNUMERIC) ||
($compatibilityMode == self::COMPATIBILITY_OPENOFFICE)) {
self::$compatibilityMode = $compatibilityMode;
return True;
}
return False;
} // function setCompatibilityMode()
/**
* Return the current Compatibility Mode
*
* @access public
* @category Function Configuration
* @return string Compatibility Mode
* Possible Return values are:
* PHPExcel_Calculation_Functions::COMPATIBILITY_EXCEL 'Excel'
* PHPExcel_Calculation_Functions::COMPATIBILITY_GNUMERIC 'Gnumeric'
* PHPExcel_Calculation_Functions::COMPATIBILITY_OPENOFFICE 'OpenOfficeCalc'
*/
public static function getCompatibilityMode() {
return self::$compatibilityMode;
} // function getCompatibilityMode()
/**
* Set the Return Date Format used by functions that return a date/time (Excel, PHP Serialized Numeric or PHP Object)
*
* @access public
* @category Function Configuration
* @param string $returnDateType Return Date Format
* Permitted values are:
* PHPExcel_Calculation_Functions::RETURNDATE_PHP_NUMERIC 'P'
* PHPExcel_Calculation_Functions::RETURNDATE_PHP_OBJECT 'O'
* PHPExcel_Calculation_Functions::RETURNDATE_EXCEL 'E'
* @return boolean Success or failure
*/
public static function setReturnDateType($returnDateType) {
if (($returnDateType == self::RETURNDATE_PHP_NUMERIC) ||
($returnDateType == self::RETURNDATE_PHP_OBJECT) ||
($returnDateType == self::RETURNDATE_EXCEL)) {
self::$ReturnDateType = $returnDateType;
return True;
}
return False;
} // function setReturnDateType()
/**
* Return the current Return Date Format for functions that return a date/time (Excel, PHP Serialized Numeric or PHP Object)
*
* @access public
* @category Function Configuration
* @return string Return Date Format
* Possible Return values are:
* PHPExcel_Calculation_Functions::RETURNDATE_PHP_NUMERIC 'P'
* PHPExcel_Calculation_Functions::RETURNDATE_PHP_OBJECT 'O'
* PHPExcel_Calculation_Functions::RETURNDATE_EXCEL 'E'
*/
public static function getReturnDateType() {
return self::$ReturnDateType;
} // function getReturnDateType()
/**
* DUMMY
*
* @access public
* @category Error Returns
* @return string #Not Yet Implemented
*/
public static function DUMMY() {
return '#Not Yet Implemented';
} // function DUMMY()
/**
* NA
*
* Excel Function:
* =NA()
*
* Returns the error value #N/A
* #N/A is the error value that means "no value is available."
*
* @access public
* @category Logical Functions
* @return string #N/A!
*/
public static function NA() {
return self::$_errorCodes['na'];
} // function NA()
/**
* NAN
*
* Returns the error value #NUM!
*
* @access public
* @category Error Returns
* @return string #NUM!
*/
public static function NaN() {
return self::$_errorCodes['num'];
} // function NAN()
/**
* NAME
*
* Returns the error value #NAME?
*
* @access public
* @category Error Returns
* @return string #NAME?
*/
public static function NAME() {
return self::$_errorCodes['name'];
} // function NAME()
/**
* REF
*
* Returns the error value #REF!
*
* @access public
* @category Error Returns
* @return string #REF!
*/
public static function REF() {
return se

baibenq
- 粉丝: 6
- 资源: 9
最新资源
- 基于Go-micro微服务的秒杀系统详细文档+优秀项目+全部资料.zip
- 基于golang实现在线客服系统,包含用户端(h5,微信小程序),客服端(PC),方便跟已有的系统整合。适用于小程序自带的客服系统无法满足或有多端业务需求的情况详细文档+优秀项目+全部资料.zip
- 基于gorillawebsocket封装的websocket库,实现基于系统维度的消息推送,基于群组维度的消息推送,基于单个和多个客户端消息推送详细文档+优秀项目+全部资料.zip
- 基于Go-Zero + Vue3 + TypeScript + Element-Plus开发的简单高效权限管理系统详细文档+优秀项目+全部资料.zip
- 基于Go-Zero Nestjs + Vue3 + TypeScript + Element-Plus开发的简单高效权限管理系统详细文档+优秀项目+全部资料.zip
- linux常用命令大全.txt
- 基于go-zero的影票售卖系统详细文档+优秀项目+全部资料.zip
- 基于Go-Zero + vue-element-admin的前后端分离微服务管理系统的前端模块详细文档+优秀项目+全部资料.zip
- 基于go-zero 框架实现的电商系统的后端服务详细文档+优秀项目+全部资料.zip
- 基于go-zero实现的网盘系统详细文档+优秀项目+全部资料.zip
- 基于go-zero框架数据中台系统详细文档+优秀项目+全部资料.zip
- 基于go-zero和gorm开发的分布式微服务后端权限管理系统脚手架。十分合适新手入手go-zero、gorm、casbin、jwt等。详细文档+优秀项目+全部
- 基于Go的WebSocket直播间推送系统详细文档+优秀项目+全部资料.zip
- 基于Go和GraphQL的微型进销存系统:服务器端(基于Golang,GraphQL,GORM,jwt-go等开发)详细文档+优秀项目+全部资料.zip
- 基于go的自托管博客系统详细文档+优秀项目+全部资料.zip
- 基于go开发的分布式高并发web电商系统详细文档+优秀项目+全部资料.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


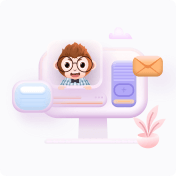
- 1
- 2
前往页