package com.example.yin.controller;
import com.example.yin.common.FatalMessage;
import com.example.yin.common.ErrorMessage;
import com.example.yin.common.SuccessMessage;
import com.example.yin.common.WarningMessage;
import com.example.yin.constant.Constants;
import com.example.yin.domain.Consumer;
import com.example.yin.service.impl.ConsumerServiceImpl;
import org.apache.commons.lang3.ObjectUtils.Null;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Configuration;
import org.springframework.dao.DuplicateKeyException;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
import org.springframework.web.servlet.config.annotation.ResourceHandlerRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpSession;
import java.io.File;
import java.io.IOException;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.List;
@RestController
public class ConsumerController {
@Autowired
private ConsumerServiceImpl consumerService;
@Configuration
public static class MyPicConfig implements WebMvcConfigurer {
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
registry.addResourceHandler("/img/avatorImages/**")
.addResourceLocations(Constants.AVATOR_IMAGES_PATH);
}
}
/**
* 用户注册
*/
@ResponseBody
@RequestMapping(value = "/user/add", method = RequestMethod.POST)
public Object addUser(HttpServletRequest req) {
String username = req.getParameter("username").trim();
String password = req.getParameter("password").trim();
String sex = req.getParameter("sex").trim();
String phone_num = req.getParameter("phone_num").trim();
String email = req.getParameter("email").trim();
String birth = req.getParameter("birth").trim();
String introduction = req.getParameter("introduction").trim();
String location = req.getParameter("location").trim();
String avator = "/img/avatorImages/user.jpg";
if(consumerService.existUser(username)) {
return new WarningMessage("用户名已注册").getMessage();
}
Consumer consumer = new Consumer();
DateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
Date myBirth = new Date();
try {
myBirth = dateFormat.parse(birth);
} catch (Exception e) {
e.printStackTrace();
}
consumer.setUsername(username);
consumer.setPassword(password);
consumer.setSex(new Byte(sex));
if ("".equals(phone_num)) {
consumer.setPhoneNum(null);
} else {
consumer.setPhoneNum(phone_num);
}
if ("".equals(email)) {
consumer.setEmail(null);
} else {
consumer.setEmail(email);
}
consumer.setBirth(myBirth);
consumer.setIntroduction(introduction);
consumer.setLocation(location);
consumer.setAvator(avator);
consumer.setCreateTime(new Date());
consumer.setUpdateTime(new Date());
try {
boolean res = consumerService.addUser(consumer);
if (res) {
return new SuccessMessage<Null>("注册成功").getMessage();
} else {
return new ErrorMessage("注册失败").getMessage();
}
} catch (DuplicateKeyException e) {
return new FatalMessage(e.getMessage()).getMessage();
}
}
/**
* 登录判断
*/
@ResponseBody
@RequestMapping(value = "/user/login/status", method = RequestMethod.POST)
public Object loginStatus(HttpServletRequest req, HttpSession session) {
String username = req.getParameter("username");
String password = req.getParameter("password");
boolean res = consumerService.veritypasswd(username, password);
if (res) {
session.setAttribute("username", username);
return new SuccessMessage<List<Consumer>>("登录成功", consumerService.loginStatus(username)).getMessage();
} else {
return new ErrorMessage("用户名或密码错误").getMessage();
}
}
/**
* 返回所有用户
*/
@RequestMapping(value = "/user", method = RequestMethod.GET)
public Object allUser() {
return new SuccessMessage<List<Consumer>>(null, consumerService.allUser()).getMessage();
}
/**
* 返回指定 ID 的用户
*/
@RequestMapping(value = "/user/detail", method = RequestMethod.GET)
public Object userOfId(HttpServletRequest req) {
String id = req.getParameter("id");
return new SuccessMessage<List<Consumer>>(null, consumerService.userOfId(Integer.parseInt(id))).getMessage();
}
/**
* 删除用户
*/
@RequestMapping(value = "/user/delete", method = RequestMethod.GET)
public Object deleteUser(HttpServletRequest req) {
String id = req.getParameter("id");
boolean res = consumerService.deleteUser(Integer.parseInt(id));
if (res) {
return new SuccessMessage<Null>("删除成功").getMessage();
} else {
return new ErrorMessage("删除失败").getMessage();
}
}
/**
* 更新用户信息
*/
@ResponseBody
@RequestMapping(value = "/user/update", method = RequestMethod.POST)
public Object updateUserMsg(HttpServletRequest req) {
String id = req.getParameter("id").trim();
String username = req.getParameter("username").trim();
String sex = req.getParameter("sex").trim();
String phone_num = req.getParameter("phone_num").trim();
String email = req.getParameter("email").trim();
String birth = req.getParameter("birth").trim();
String introduction = req.getParameter("introduction").trim();
String location = req.getParameter("location").trim();
// System.out.println(username);
Consumer consumer = new Consumer();
DateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
Date myBirth = new Date();
try {
myBirth = dateFormat.parse(birth);
} catch (Exception e) {
e.printStackTrace();
}
consumer.setId(Integer.parseInt(id));
consumer.setUsername(username);
consumer.setSex(new Byte(sex));
consumer.setPhoneNum(phone_num);
consumer.setEmail(email);
consumer.setIntroduction(introduction);
consumer.setLocation(location);
consumer.setUpdateTime(new Date());
consumer.setBirth(myBirth);
boolean res = consumerService.updateUserMsg(consumer);
if (res) {
return new SuccessMessage<Null>("修改成功").getMessage();
} else {
return new ErrorMessage("修改失败").getMessage();
}
}
/**
* 更新用户密码
*/
@ResponseBody
@RequestMapping(value = "/user/updatePassword", method = RequestMethod.POST)
public Object updatePassword(HttpServletRequest req) {
String id = req.getParameter("id").trim();
String username = req.getParameter("username").trim();
String old_password = req.getParameter("old_password").trim();
String password = req.getParameter("password").trim();
boolean res = consumerService.veritypasswd(username, old_password);
if (!res) {
return new ErrorMessage("密码输入错误").getMessage();
}
Consumer consumer = new Consumer();
consumer.setId(Integer.parseInt(id));
consumer.setPassword(password);
boolean result = consumerService.updatePassword(consumer);
if (result) {
ret
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
软件架构 - java后台:music-server - 用户前台:music-client - 管理员前台:music-manage 前端采用的是基于VUE的ElmentUI框架开发,后端是基于springboot框架开发,数据库使用的是Mysql,分为三个项目,分别是用户前端,管理员前端,后台接口集成 技术选型 | Spring Boot| 2.1.6| MVC核心框架 | | Spring Security oauth2 | 2.1.5| 认证和授权框架| | MyBatis| 3.5.0| ORM框架 | | MyBatisPlus| 3.1.0| 基于mybatis,使用lambda表达式的 | | Swagger-UI | 2.9.2| 文档生产工具| | Hibernator-Validator | 6.0.17 | 验证框架| | redisson | 3.10.6 | 对redis进行封装、集成分布式锁等 | | hikari | 3.2.0| 数据库连接池| | log4j2 | 2.11.2 | 更快的log日志工具 | | fst| 2.57 | 更快的序列化
资源推荐
资源详情
资源评论
















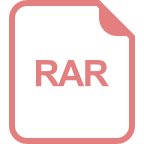







收起资源包目录

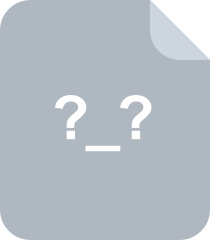
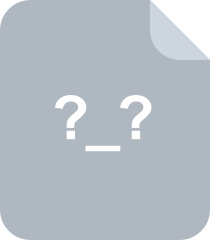
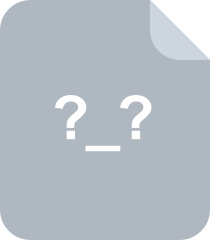
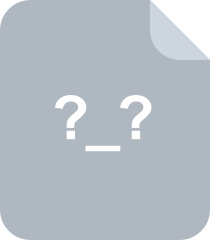
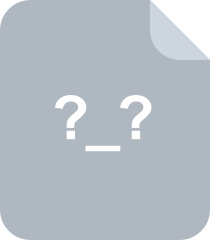
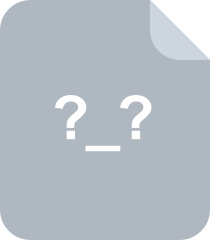
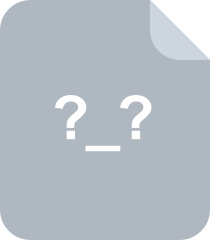
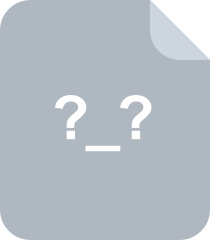
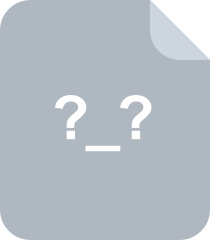
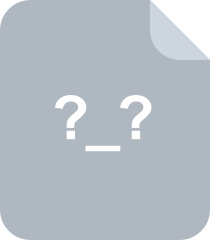
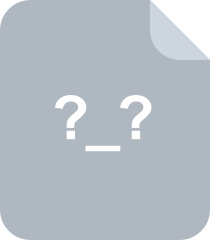
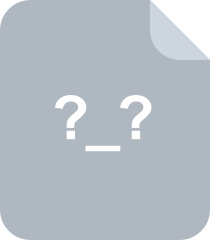
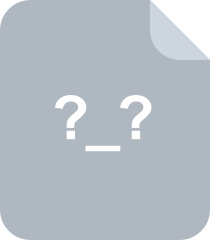
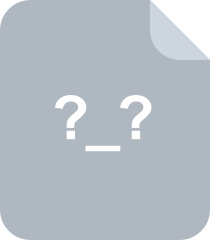
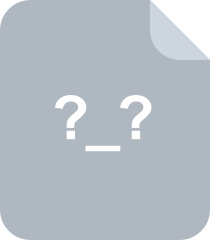
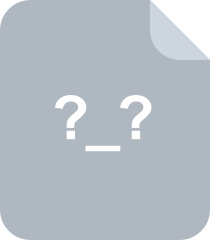
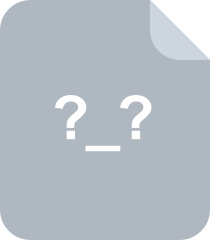
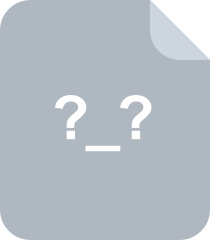
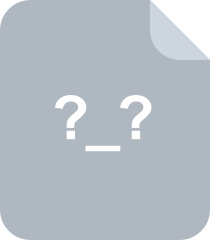
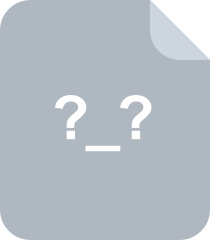
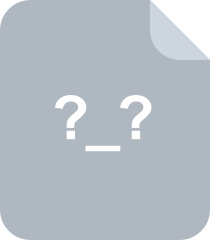
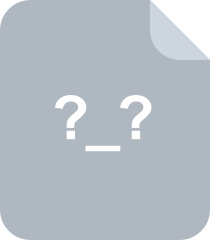
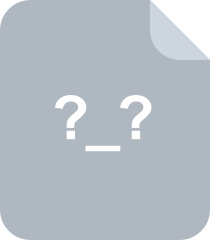
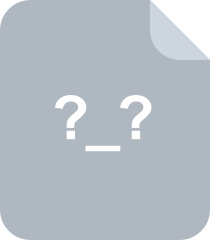
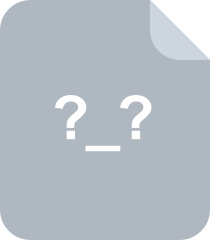
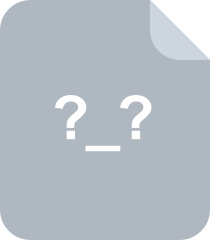
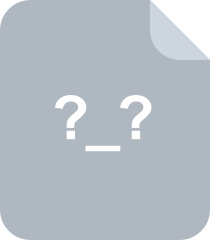
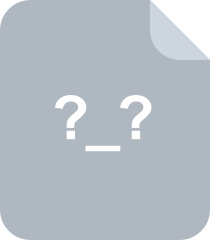
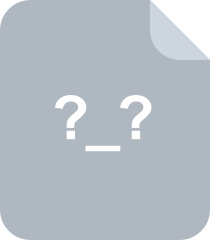
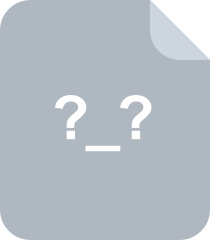
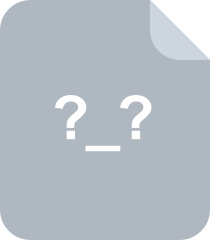
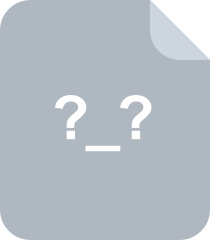
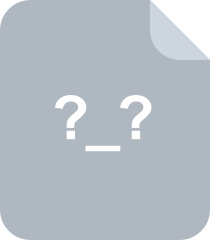
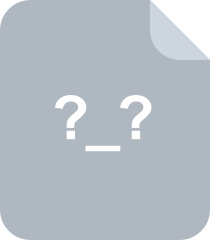
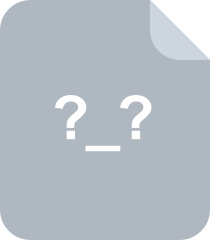
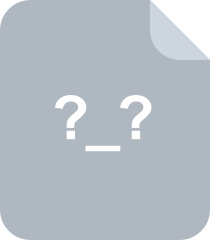
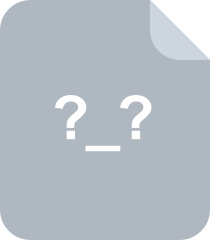
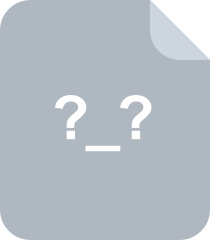
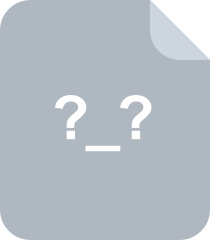
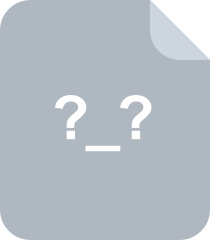
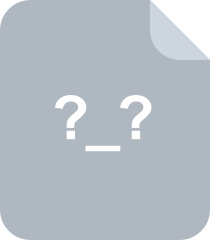
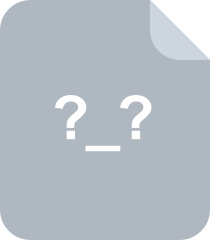
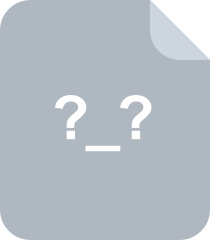
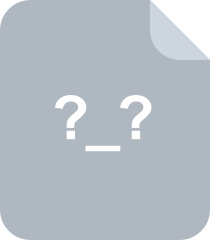
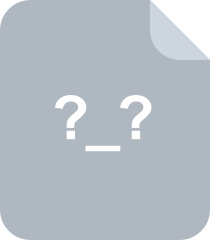
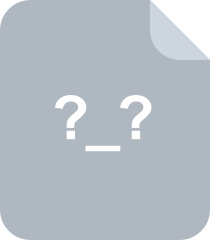
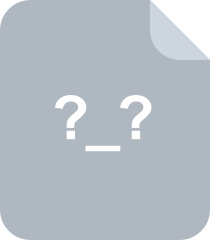
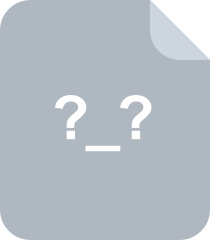
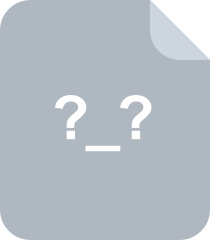
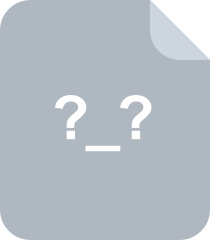
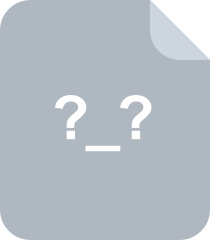
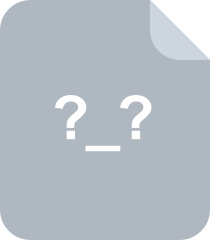
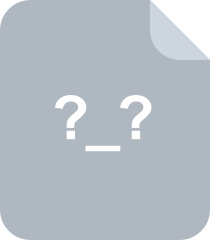
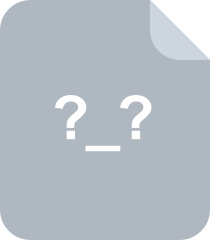
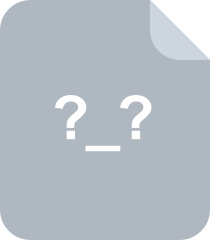
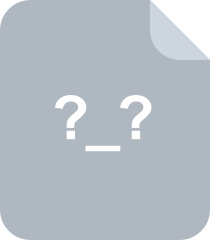
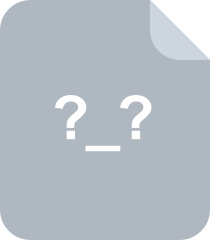
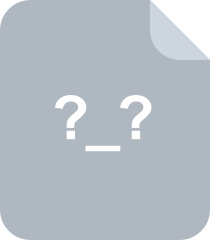
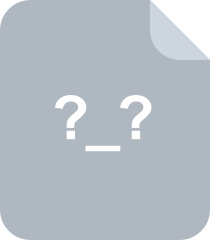
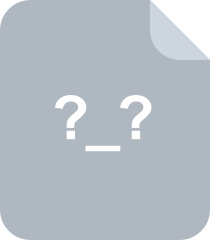
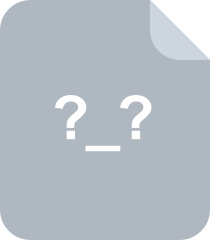
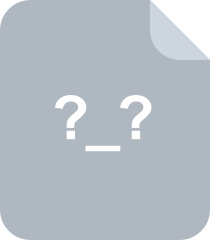
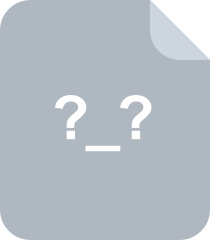
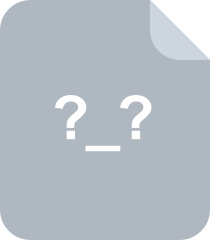
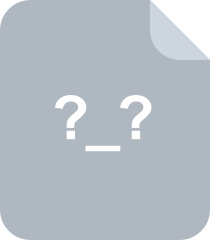
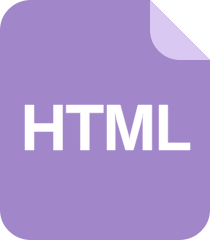
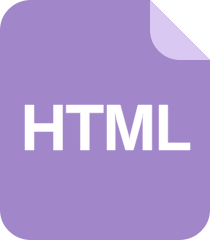
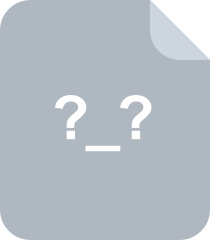
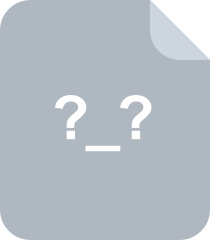
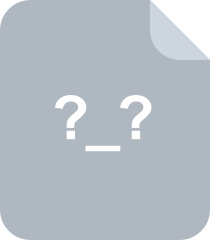
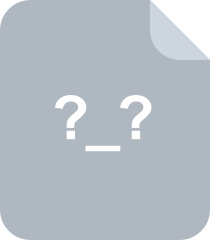
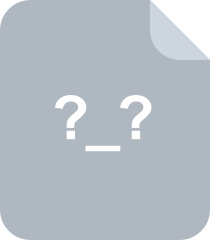
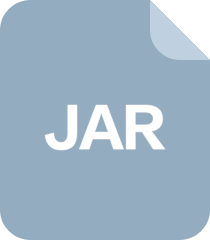
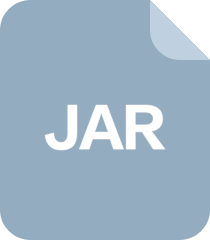
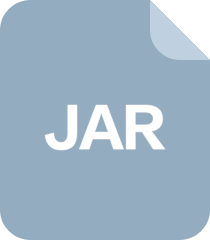
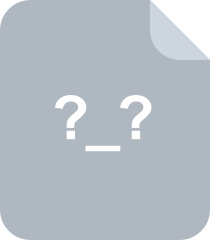
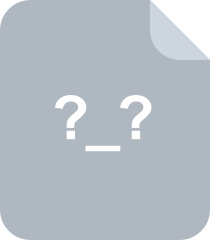
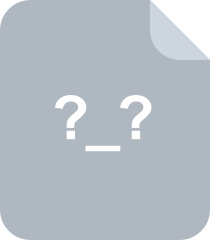
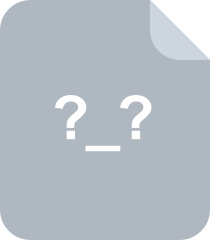
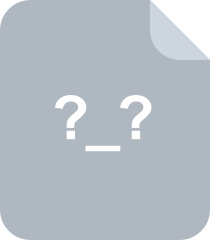
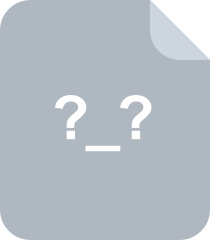
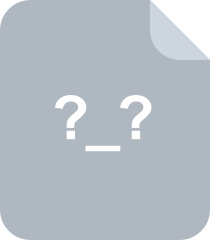
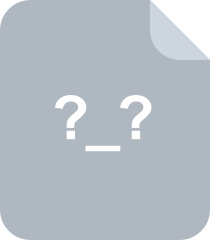
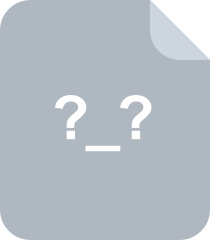
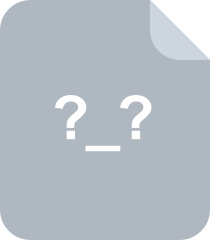
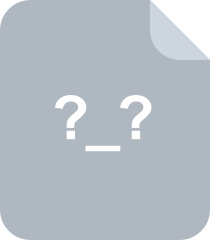
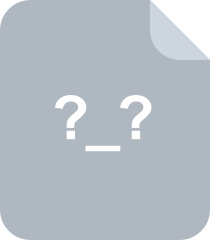
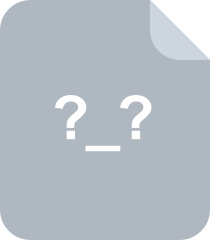
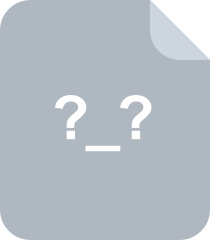
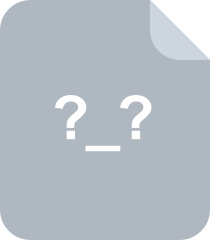
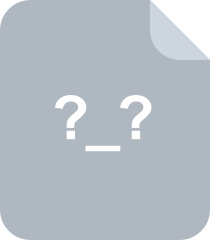
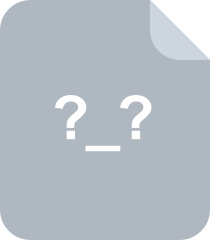
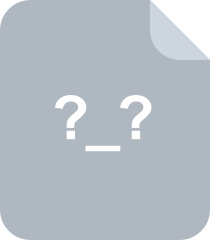
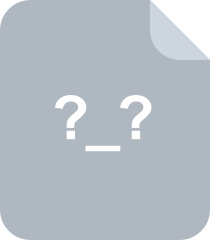
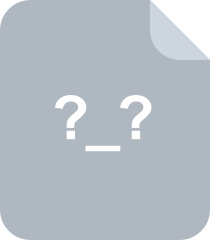
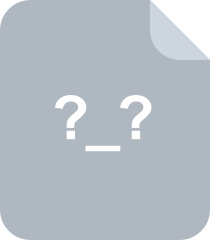
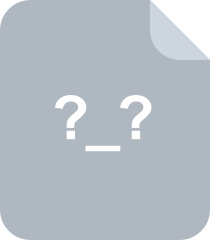
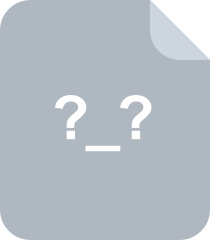
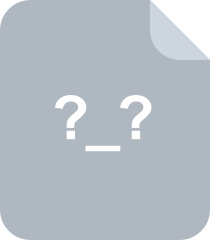
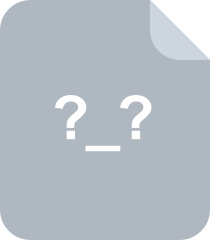
共 381 条
- 1
- 2
- 3
- 4
资源评论
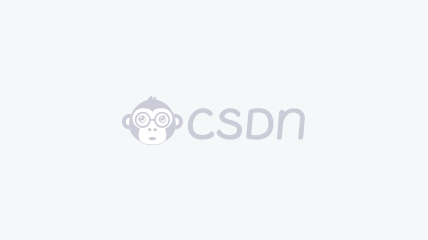

心兰相随引导者
- 粉丝: 1183
- 资源: 5639

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

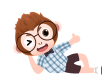
最新资源
- Kotlin编程入门:基础语法、类与对象、进阶特性以及实战案例详解
- EF框架 MySQL数据库依赖
- 使用COMSOL 6.1版模拟光纤及其他波导三维弯曲的模场分布与波束包络方法探索,使用COMSOL 6.1仿真波导的三维弯曲及其模场分布和波束包络分析,COMSOL模型仿真光纤等波导的三维弯曲,模场分
- 电机控制器IGBT结温精确估算方法与模型:国际大厂机密算法公开,涵盖直流交流仿真及底层算法库,精准保护产品性能提升 ,电机控制器核心算法揭秘:高精度IGBT结温估算模型与simulink仿真库 此模型
- 美赛MCM/ICM备战指南:数学建模、数据分析与论文写作全攻略
- 并网逆变器阻抗建模与扫频验证:伍文华博士论文内容复现与仿真程序解析,并网逆变器阻抗建模与扫频验证:伍文华博士的lunwen复现与仿真模型研究,附带注释的程序实现,并网逆变器阻抗建模,扫频模型扫频验证
- 汇编语言入门 简要的说明了入门方法
- 储能双向DCDC变流器模型预测控制:结合下垂控制与PI电压环和模型预测电流环的创新策略参考模型文献,储能双向DCDC变流器模型预测控制研究:结合下垂控制与PI电压环的高级控制策略参考文献解析,储能双向
- linux常用命令大全.txt
- AI领域基础概念、算法及应用实例解析及学习路径
- linux常用命令大全.txt
- linux常用命令大全.txt
- 高效稳定的BLDC低压方波无感控制策略:直流无刷电机驱动器闭环启动方案与实践,BLDC低压方波无感无霍尔驱动器:闭环启动方案与PI稳速功能的实践与应用,BLDC低压方波控制方案 直流无刷电机驱动器 无
- 基于IEEE 33标准的含分布式电源配电网潮流计算方法:采用牛顿拉夫逊法及Matlab编程,调整电压器变比、添加无功补偿装置与灵活布置光伏风机电源位置的探讨,基于IEEE 33标准的配电网潮流计算:含
- 面向双碳目标的新型电力系统演进路径与挑战.pptx
- 集团企业信息化管理平台建设方案(43页).pptx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


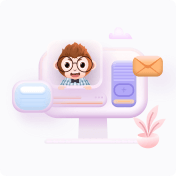
安全验证
文档复制为VIP权益,开通VIP直接复制
