# -*- coding: utf-8 -*-
# import time
try:
#from osgeo import gdal
from osgeo import ogr
from osgeo import osr
except ImportError:
#import gdal
import ogr
import osr
from osgeo.ogr import Geometry
from shutil import copyfile
import os
#from coordTransform_utils import gcj02tobd09, bd09togcj02, wgs84togcj02, gcj02towgs84, wgs84tomercator, mercatortowgs84,bd09tomercator, mercatortobd09
from coordTransform_utils import gcj02tobd09, bd09togcj02, wgs84togcj02, gcj02towgs84
# 写入shp文件,polygon
# coord_type,code_name, field_list, result_list
# coord_original == 'gcj02' and coord_target == 'wgs84',可灵活组合
def polygon_transform(geom_polygon, coord_original, coord_target):
geom_type = geom_polygon.GetGeometryType()
z_value = ogr.GT_HasZ(geom_type)
# print(z_value)
geom_col_polygon = ogr.Geometry(ogr.wkbGeometryCollection)
geom_col_polygon.AddGeometry(geom_polygon)
geom_poly = geom_polygon
# print(geom_poly.GetGeometryCount())
geom_poly_o_count = geom_poly.GetGeometryCount()
geom_poly = ogr.Geometry(ogr.wkbPolygon)
# for m in range(0, geom_poly_o_count):
# geom_poly.RemoveGeometry(geom_poly_o_count - m - 1)
# print('geom_poly')
# print(geom_poly)
# 获取polygon对象的边界
boundary_polygon = geom_col_polygon.GetGeometryRef(0).GetBoundary()
# print('boundary_polygon')
# print(boundary_polygon)
# 获取边界的数量,如果大于1,说明有孔洞
boundary_polygon_count = boundary_polygon.GetGeometryCount()
# print('boundary_polygon_count')
# print(boundary_polygon_count)
if boundary_polygon_count == 0:
boundary_polygon_point_count = boundary_polygon.GetPointCount()
ring = ogr.Geometry(ogr.wkbLinearRing)
for j in range(boundary_polygon_point_count):
point_polygon = boundary_polygon.GetPoint(j)
# 坐标系转换
if coord_original == 'gcj02' and coord_target == 'wgs84':
point_polygon_trans = gcj02towgs84(point_polygon[0], point_polygon[1])
elif coord_original == 'wgs84' and coord_target == 'gcj02':
point_polygon_trans = wgs84togcj02(point_polygon[0], point_polygon[1])
elif coord_original == 'gcj02' and coord_target == 'bd09':
point_polygon_trans = gcj02tobd09(point_polygon[0], point_polygon[1])
elif coord_original == 'bd09' and coord_target == 'gcj02':
point_polygon_trans = bd09togcj02(point_polygon[0], point_polygon[1])
elif coord_original == 'wgs84' and coord_target == 'bd09':
point_polygon_trans_gcj02 = wgs84togcj02(point_polygon[0], point_polygon[1])
point_polygon_trans = gcj02tobd09(point_polygon_trans_gcj02[0], point_polygon_trans_gcj02[1])
elif coord_original == 'bd09' and coord_target == 'wgs84':
point_polygon_trans_gcj02 = bd09togcj02(point_polygon[0], point_polygon[1])
point_polygon_trans = gcj02towgs84(point_polygon_trans_gcj02[0], point_polygon_trans_gcj02[1])
else:
point_polygon_trans = [point_polygon[0], point_polygon[1]]
# 添加坐标点
if z_value == 1:
ring.AddPoint(point_polygon_trans[0], point_polygon_trans[1], point_polygon[2])
else:
ring.AddPoint_2D(point_polygon_trans[0], point_polygon_trans[1])
geom_poly.AddGeometry(ring)
else:
for i in range(boundary_polygon_count):
boundary_polygon_ring = boundary_polygon.GetGeometryRef(i)
boundary_polygon_ring_point_count = boundary_polygon_ring.GetPointCount()
ring = ogr.Geometry(ogr.wkbLinearRing)
for j in range(0, boundary_polygon_ring_point_count):
point_polygon = boundary_polygon_ring.GetPoint(j)
# print('point_polygon')
# print(point_polygon)
# 坐标系转换
if coord_original == 'gcj02' and coord_target == 'wgs84':
point_polygon_trans = gcj02towgs84(point_polygon[0], point_polygon[1])
elif coord_original == 'wgs84' and coord_target == 'gcj02':
point_polygon_trans = wgs84togcj02(point_polygon[0], point_polygon[1])
elif coord_original == 'gcj02' and coord_target == 'bd09':
point_polygon_trans = gcj02tobd09(point_polygon[0], point_polygon[1])
elif coord_original == 'bd09' and coord_target == 'gcj02':
point_polygon_trans = bd09togcj02(point_polygon[0], point_polygon[1])
elif coord_original == 'wgs84' and coord_target == 'bd09':
point_polygon_trans_gcj02 = wgs84togcj02(point_polygon[0], point_polygon[1])
point_polygon_trans = gcj02tobd09(point_polygon_trans_gcj02[0], point_polygon_trans_gcj02[1])
elif coord_original == 'bd09' and coord_target == 'wgs84':
point_polygon_trans_gcj02 = bd09togcj02(point_polygon[0], point_polygon[1])
point_polygon_trans = gcj02towgs84(point_polygon_trans_gcj02[0], point_polygon_trans_gcj02[1])
else:
point_polygon_trans = [point_polygon[0], point_polygon[1]]
# 添加坐标点
if z_value == 1:
ring.AddPoint(point_polygon_trans[0], point_polygon_trans[1], point_polygon[2])
else:
ring.AddPoint_2D(point_polygon_trans[0], point_polygon_trans[1])
geom_poly.AddGeometry(ring)
return geom_poly
def multi_polygon_transform(geom_polygon, coord_original, coord_target):
geom_col = ogr.Geometry(ogr.wkbGeometryCollection)
for g in geom_polygon:
geom_col.AddGeometry(g)
geom_polygon_count = geom_polygon.GetGeometryCount()
# for m in range(0, geom_polygon_count):
# geom_polygon.RemoveGeometry(geom_polygon_count - m - 1)
geom_polygon = ogr.Geometry(ogr.wkbMultiPolygon)
for g in geom_col:
g_trans = polygon_transform(g, coord_original, coord_target)
geom_polygon.AddGeometry(g_trans)
return geom_polygon
def polyline_transform(geom_polyline, coord_original, coord_target):
print ('================ 进入 polyline_transform ===================')
#print ('================ 进入 polyline_transform ===================,原始geom_polyline:'+str(geom_polyline))
geom_type = geom_polyline.GetGeometryType()
z_value = ogr.GT_HasZ(geom_type)
# print(z_value)
geom_polyline_count = geom_polyline.GetPointCount()
for i in range(0, geom_polyline_count):
point_orginal = geom_polyline.GetPoint(i)
# 坐标系转换
print ('================ 进入 polyline_transform ===================开始转换'+str(i)+"/"+str(geom_polyline_count))
if coord_original == 'gcj02' and coord_target == 'wgs84':
point_polygon_trans = gcj02towgs84(point_orginal[0], point_orginal[1])
elif coord_original == 'wgs84' and coord_target == 'gcj02':
point_polygon_trans = wgs84togcj02(point_orginal[0], point_orginal[1])
elif coord_original == 'gcj02' and coord_target == 'bd09':
point_polygon_trans = gcj02tobd09(point_orginal[0], point_orginal[1])
elif coord_original == 'bd09' and coord_target == 'gcj02':
point_polygon_trans = bd09togcj02(point_orginal[0], point_orginal[1])
elif coord_original == 'wgs84' and coord_target == 'bd09':
point_polygon_trans_gcj02 = wgs84togcj02(point_orginal[0], point_orginal[1])
point_polygon_trans = gcj02tobd09(point_polygon_trans_gcj02[0], point_polygon_trans_gcj02[1])
elif coord_original == 'bd09' and coord_target == 'wgs84':
point_polygon_trans_gcj02 = bd09togcj02(point_orginal[0], point_orginal[1])
point_polygon_trans = gcj02towgs84(point_pol

arno_wzk
- 粉丝: 20
- 资源: 10
最新资源
- 基于web的中小型企业医药管理系统.doc
- PM产品管理流程总结整理
- 基于web的大学生社团平台的开发与实现论文.doc
- 基于SSM框架的建筑市场监管与诚信信息发布平台.doc
- Delphi 12 控件之Dism++10.1.1002.1B.rar
- 自动化水洗标机sw18可编辑全套技术资料100%好用.zip
- photocc2020处理不了webp文件插件
- 跑步社区界面管理系统基于Struts2技术的设计与实现
- 基于struts和hibernate的手机销售系统的设计与实现论文.doc
- 山东大学编译原理期末复习-概念汇总
- 基于web的畅读小说管理系统设计论文.doc
- 基于Web的电影点评系统分析与设计-提高用户观影选择及影院管理效率的JSP开发
- 基于web的房屋出租管理系统的设计与实现.doc
- 基于web的老年公寓管理平台的设计与实现.doc
- 基于web的农产品销售管理系统
- 基于web的人才招聘网站论文 .doc
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


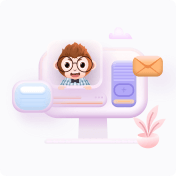