# ESPAsyncWebServer
[](https://travis-ci.org/me-no-dev/ESPAsyncWebServer)  [](https://www.codacy.com/manual/me-no-dev/ESPAsyncWebServer?utm_source=github.com&utm_medium=referral&utm_content=me-no-dev/ESPAsyncWebServer&utm_campaign=Badge_Grade)
A fork of the [ESPAsyncWebServer](https://github.com/me-no-dev/ESPAsyncWebServer) library by [@me-no-dev](https://github.com/me-no-dev) for [ESPHome](https://esphome.io).
For help and support [](https://gitter.im/me-no-dev/ESPAsyncWebServer?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)
Async HTTP and WebSocket Server for ESP8266 Arduino
For ESP8266 it requires [ESPAsyncTCP](https://github.com/me-no-dev/ESPAsyncTCP)
To use this library you might need to have the latest git versions of [ESP8266](https://github.com/esp8266/Arduino) Arduino Core
For ESP32 it requires [AsyncTCP](https://github.com/me-no-dev/AsyncTCP) to work
To use this library you might need to have the latest git versions of [ESP32](https://github.com/espressif/arduino-esp32) Arduino Core
## Table of contents
- [ESPAsyncWebServer](#espasyncwebserver)
- [Table of contents](#table-of-contents)
- [Installation](#installation)
- [Using PlatformIO](#using-platformio)
- [Why should you care](#why-should-you-care)
- [Important things to remember](#important-things-to-remember)
- [Principles of operation](#principles-of-operation)
- [The Async Web server](#the-async-web-server)
- [Request Life Cycle](#request-life-cycle)
- [Rewrites and how do they work](#rewrites-and-how-do-they-work)
- [Handlers and how do they work](#handlers-and-how-do-they-work)
- [Responses and how do they work](#responses-and-how-do-they-work)
- [Template processing](#template-processing)
- [Libraries and projects that use AsyncWebServer](#libraries-and-projects-that-use-asyncwebserver)
- [Request Variables](#request-variables)
- [Common Variables](#common-variables)
- [Headers](#headers)
- [GET, POST and FILE parameters](#get-post-and-file-parameters)
- [FILE Upload handling](#file-upload-handling)
- [Body data handling](#body-data-handling)
- [JSON body handling with ArduinoJson](#json-body-handling-with-arduinojson)
- [Responses](#responses)
- [Redirect to another URL](#redirect-to-another-url)
- [Basic response with HTTP Code](#basic-response-with-http-code)
- [Basic response with HTTP Code and extra headers](#basic-response-with-http-code-and-extra-headers)
- [Basic response with string content](#basic-response-with-string-content)
- [Basic response with string content and extra headers](#basic-response-with-string-content-and-extra-headers)
- [Send large webpage from PROGMEM](#send-large-webpage-from-progmem)
- [Send large webpage from PROGMEM and extra headers](#send-large-webpage-from-progmem-and-extra-headers)
- [Send large webpage from PROGMEM containing templates](#send-large-webpage-from-progmem-containing-templates)
- [Send large webpage from PROGMEM containing templates and extra headers](#send-large-webpage-from-progmem-containing-templates-and-extra-headers)
- [Send binary content from PROGMEM](#send-binary-content-from-progmem)
- [Respond with content coming from a Stream](#respond-with-content-coming-from-a-stream)
- [Respond with content coming from a Stream and extra headers](#respond-with-content-coming-from-a-stream-and-extra-headers)
- [Respond with content coming from a Stream containing templates](#respond-with-content-coming-from-a-stream-containing-templates)
- [Respond with content coming from a Stream containing templates and extra headers](#respond-with-content-coming-from-a-stream-containing-templates-and-extra-headers)
- [Respond with content coming from a File](#respond-with-content-coming-from-a-file)
- [Respond with content coming from a File and extra headers](#respond-with-content-coming-from-a-file-and-extra-headers)
- [Respond with content coming from a File containing templates](#respond-with-content-coming-from-a-file-containing-templates)
- [Respond with content using a callback](#respond-with-content-using-a-callback)
- [Respond with content using a callback and extra headers](#respond-with-content-using-a-callback-and-extra-headers)
- [Respond with content using a callback containing templates](#respond-with-content-using-a-callback-containing-templates)
- [Respond with content using a callback containing templates and extra headers](#respond-with-content-using-a-callback-containing-templates-and-extra-headers)
- [Chunked Response](#chunked-response)
- [Chunked Response containing templates](#chunked-response-containing-templates)
- [Print to response](#print-to-response)
- [ArduinoJson Basic Response](#arduinojson-basic-response)
- [ArduinoJson Advanced Response](#arduinojson-advanced-response)
- [Serving static files](#serving-static-files)
- [Serving specific file by name](#serving-specific-file-by-name)
- [Serving files in directory](#serving-files-in-directory)
- [Serving static files with authentication](#serving-static-files-with-authentication)
- [Specifying Cache-Control header](#specifying-cache-control-header)
- [Specifying Date-Modified header](#specifying-date-modified-header)
- [Specifying Template Processor callback](#specifying-template-processor-callback)
- [Param Rewrite With Matching](#param-rewrite-with-matching)
- [Using filters](#using-filters)
- [Serve different site files in AP mode](#serve-different-site-files-in-ap-mode)
- [Rewrite to different index on AP](#rewrite-to-different-index-on-ap)
- [Serving different hosts](#serving-different-hosts)
- [Determine interface inside callbacks](#determine-interface-inside-callbacks)
- [Bad Responses](#bad-responses)
- [Respond with content using a callback without content length to HTTP/1.0 clients](#respond-with-content-using-a-callback-without-content-length-to-http10-clients)
- [Async WebSocket Plugin](#async-websocket-plugin)
- [Async WebSocket Event](#async-websocket-event)
- [Methods for sending data to a socket client](#methods-for-sending-data-to-a-socket-client)
- [Direct access to web socket message buffer](#direct-access-to-web-socket-message-buffer)
- [Limiting the number of web socket clients](#limiting-the-number-of-web-socket-clients)
- [Async Event Source Plugin](#async-event-source-plugin)
- [Setup Event Source on the server](#setup-event-source-on-the-server)
- [Setup Event Source in the browser](#setup-event-source-in-the-browser)
- [Scanning for available WiFi Networks](#scanning-for-available-wifi-networks)
- [Remove handlers and rewrites](#remove-handlers-and-rewrites)
- [Setting up the server](#setting-up-the-server)
- [Setup global and class functions as request handlers](#setup-global-and-class-functions-as-request-handlers)
- [Methods for controlling websocket connections](#methods-for-controlling-websocket-connections)
- [Adding Default Headers](#adding-default-headers)
- [Path variable](#path-variable)
## Installation
### Using PlatformIO
[PlatformIO](http://platformio.org) is an open source ecosystem for IoT development with cross platform build system, library manager and full support for Espressif ESP8266/ESP32 development. It works on the popular host OS: Mac OS X, Windows, Linux 32/64, Linux ARM (like Raspberry Pi, BeagleBone, CubieBoard).
1. Install [PlatformIO IDE](http://platformio.org/platformio-ide)
2. Create new project

armcsdn
- 粉丝: 256
- 资源: 5
最新资源
- 【未发表】基于牛顿拉夫逊优化算法NRBO优化宽度学习BLS实现光伏数据预测算法研究附Matlab代码.rar
- 【未发表】基于蜣螂优化算法DBO优化集成学习结合核极限学习机KELM-Adaboost实现风电数据时序预测算法研究附Matlab代码.rar
- 【未发表】基于蜣螂优化算法DBO优化鲁棒极限学习机RELM实现负荷数据回归预测算法研究附Matlab代码.rar
- 【未发表】基于蜣螂优化算法DBO优化宽度学习BLS实现光伏数据预测算法研究附Matlab代码.rar
- 【未发表】基于蜣螂优化算法DBO优化集成学习结合鲁棒极限学习机RELM-Adaboost实现负荷数据回归预测算法研究附Matlab代码.rar
- 【未发表】基于人工蜂鸟优化算法AHA优化宽度学习BLS实现光伏数据预测算法研究附Matlab代码.rar
- 【未发表】基于人工蜂鸟优化算法AHA优化集成学习结合核极限学习机KELM-Adaboost实现风电数据时序预测算法研究附Matlab代码.rar
- 【未发表】基于人工蜂鸟优化算法AHA优化集成学习结合鲁棒极限学习机RELM-Adaboost实现负荷数据回归预测算法研究附Matlab代码.rar
- 【未发表】基于人工蜂鸟优化算法AHA优化鲁棒极限学习机RELM实现负荷数据回归预测算法研究附Matlab代码.rar
- 【未发表】基于人工蜂群优化算法ABC优化集成学习结合鲁棒极限学习机RELM-Adaboost实现负荷数据回归预测算法研究附Matlab代码.rar
- 【未发表】基于人工蜂群优化算法ABC优化集成学习结合核极限学习机KELM-Adaboost实现风电数据时序预测算法研究附Matlab代码.rar
- 【未发表】基于人工蜂群优化算法ABC优化宽度学习BLS实现光伏数据预测算法研究附Matlab代码.rar
- 【未发表】基于人工蜂群优化算法ABC优化鲁棒极限学习机RELM实现负荷数据回归预测算法研究附Matlab代码.rar
- 【未发表】基于三角测量拓扑聚合优化器TTAO优化集成学习结合核极限学习机KELM-Adaboost实现风电数据时序预测算法研究附Matlab代码.rar
- 【未发表】基于三角测量拓扑聚合优化器TTAO优化鲁棒极限学习机RELM实现负荷数据回归预测算法研究附Matlab代码.rar
- 【未发表】基于三角测量拓扑聚合优化器TTAO优化宽度学习BLS实现光伏数据预测算法研究附Matlab代码.rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


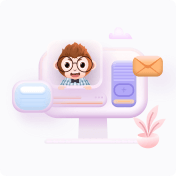