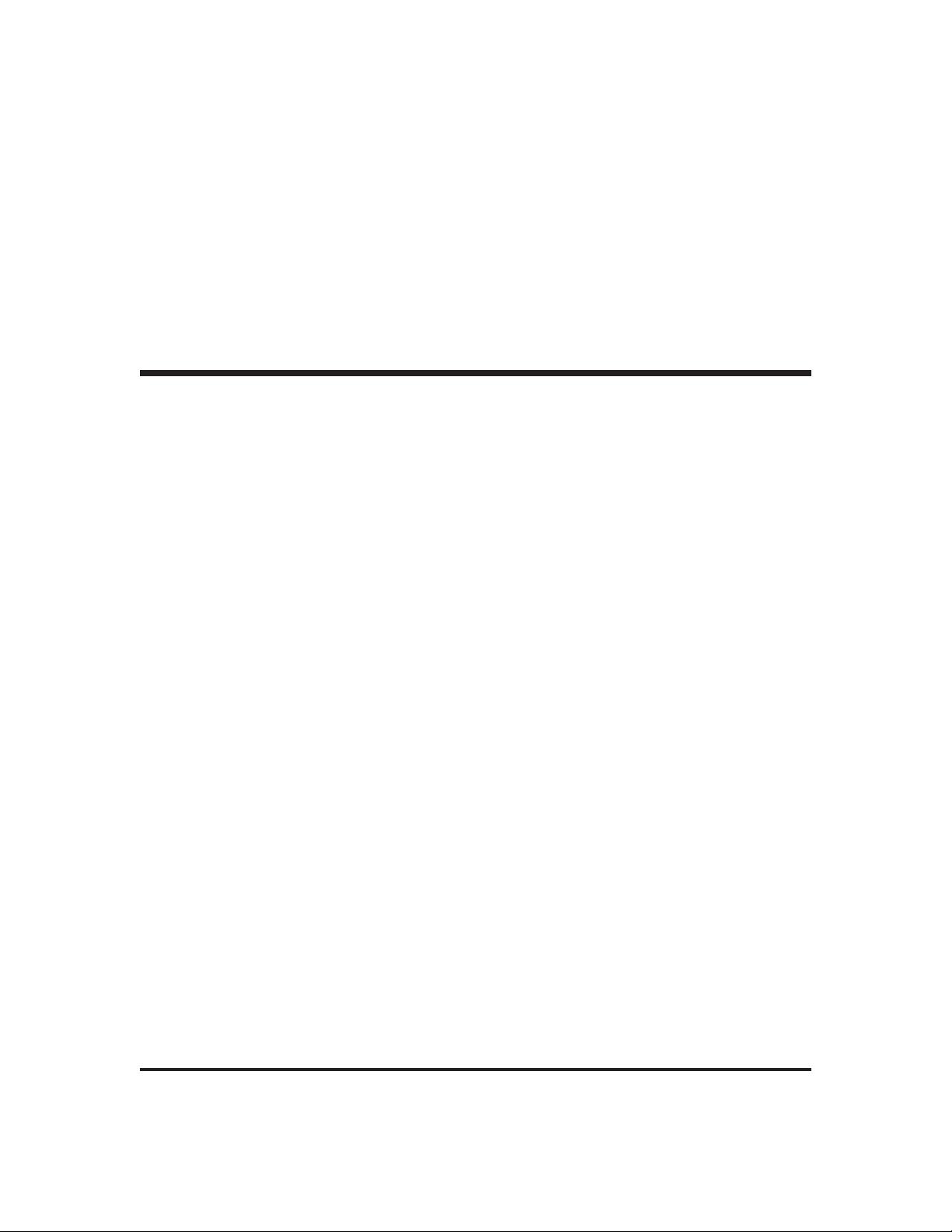
Using the GNU Compiler Collection
For gcc version 4.5.0
(GCC)
Richard M. Stallman and the GC C Developer Community
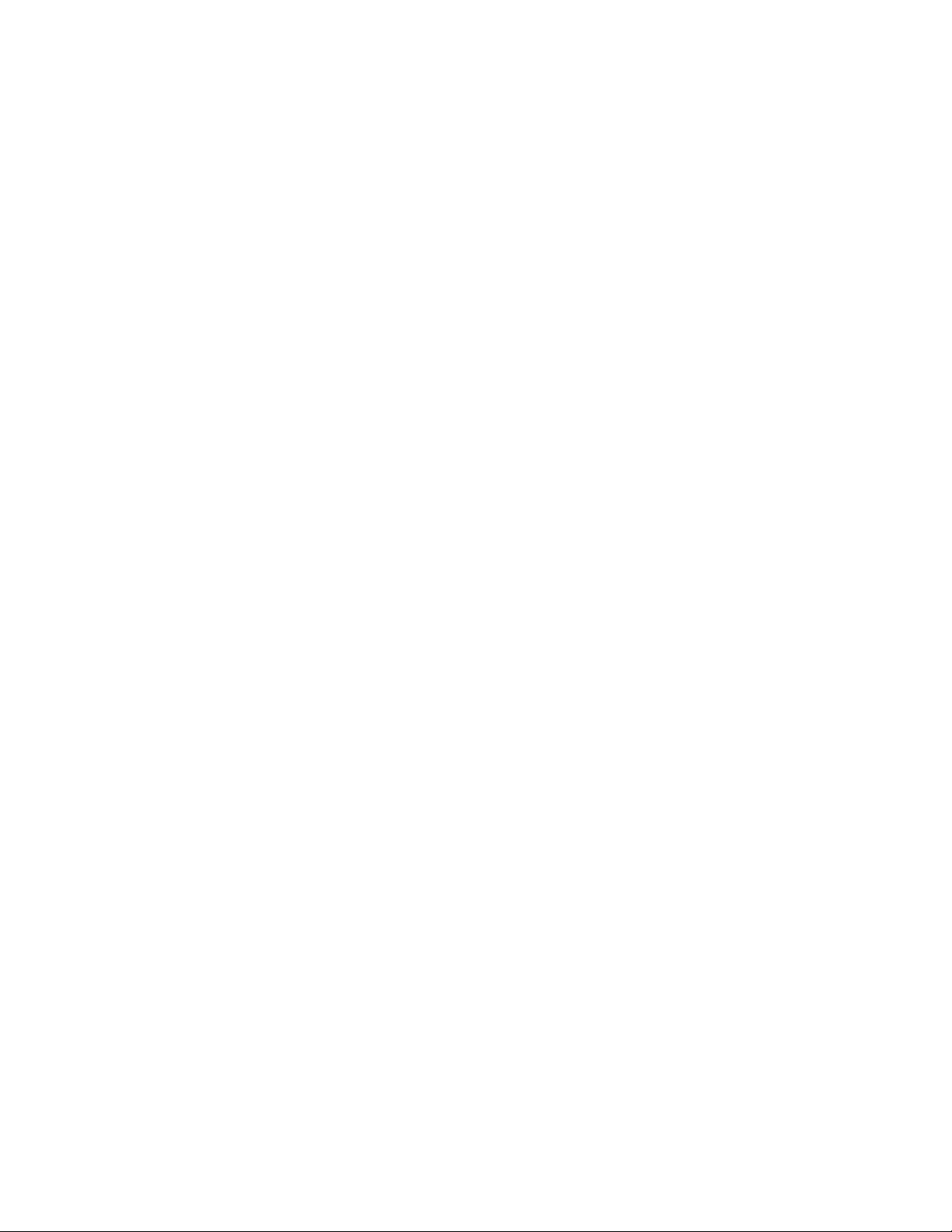
Published by:
GNU Press Website: www.gnupress.org
a division of the General: press@gnu.org
Free Software Foundation Orders: sales@gnu.org
51 Franklin Street, Fifth Floor Tel 617-542-5942
Boston, MA 02110-1301 USA Fax 617-542-2652
Last printed October 2003 for GCC 3.3.1.
Printed copies are available for $45 each.
Copyright
c
1988, 1989, 1992, 1993, 1994, 1995, 1996, 1997, 1998, 1999, 2000, 2001, 2002,
2003, 2004, 2005, 2006, 2007, 2008 Free Software Foundation, Inc.
Permission is granted to copy, distribute and/or modify this document under the terms of
the GNU Free Documentation License, Version 1.2 or any later version published by the
Free Software Foundation; with the Invariant Sections being “Funding Free Software”, the
Front-Cover Texts being (a) (see below), and with the Back-Cover Texts being (b) (see
below). A copy of the license is included in the section entitled “GNU Free Documentation
License”.
(a) The FSF’s Front-Cover Text is:
A GNU Manual
(b) The FSF’s Back-Cover Text is:
You have freedom to c opy and modify this GNU Manual, like GNU software. Copies
published by the Free Software Foundation raise funds for GNU development.
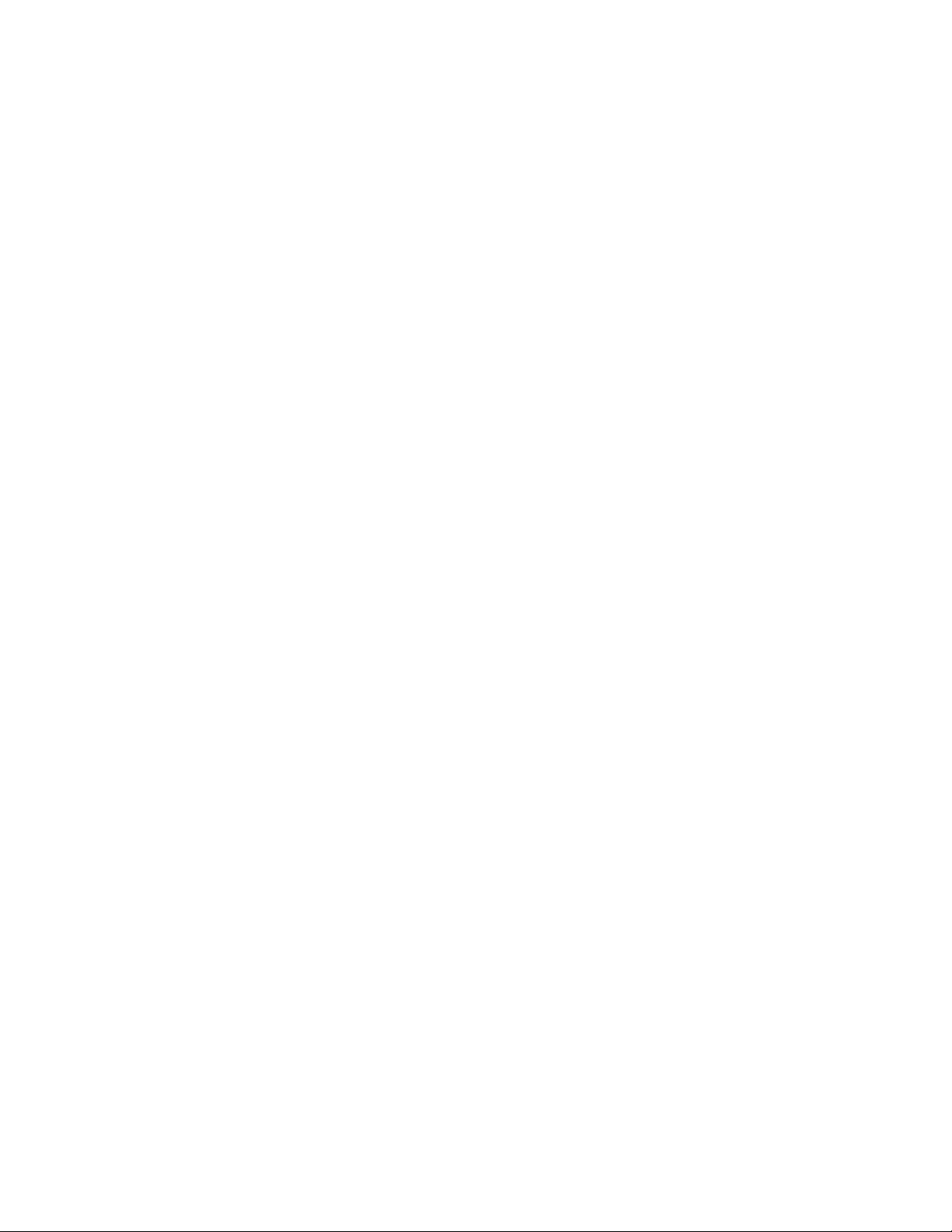
i
Short Contents
Introduction . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1
1 Programming Languages Supported by GCC . . . . . . . . . . . . . . . 3
2 Language Standards Supported by GCC . . . . . . . . . . . . . . . . . . 5
3 GCC Command Options . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 9
4 C Implementation-defined behavior . . . . . . . . . . . . . . . . . . . . . 267
5 C++ Implementation-defined behavior . . . . . . . . . . . . . . . . . . 275
6 Extensions to the C Language Family . . . . . . . . . . . . . . . . . . . 277
7 Extensions to the C++ Language . . . . . . . . . . . . . . . . . . . . . . 559
8 GNU Objective-C runtime features . . . . . . . . . . . . . . . . . . . . . 571
9 Binary Compatibility . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 577
10 gcov—a Test Coverage Program . . . . . . . . . . . . . . . . . . . . . . . 581
11 Known Causes of Trouble with GCC . . . . . . . . . . . . . . . . . . . . 589
12 Reporting Bugs . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 605
13 How To Get Help with GCC . . . . . . . . . . . . . . . . . . . . . . . . . . 607
14 Contributing to GCC Development . . . . . . . . . . . . . . . . . . . . . 609
Funding Free Software . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 611
The GNU Project and GNU/Linux. . . . . . . . . . . . . . . . . . . . . . . . . 613
GNU General Public License . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 615
GNU Free Documentation License . . . . . . . . . . . . . . . . . . . . . . . . . 627
Contributors to GCC . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 635
Option I ndex . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 651
Keyword Index . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 667
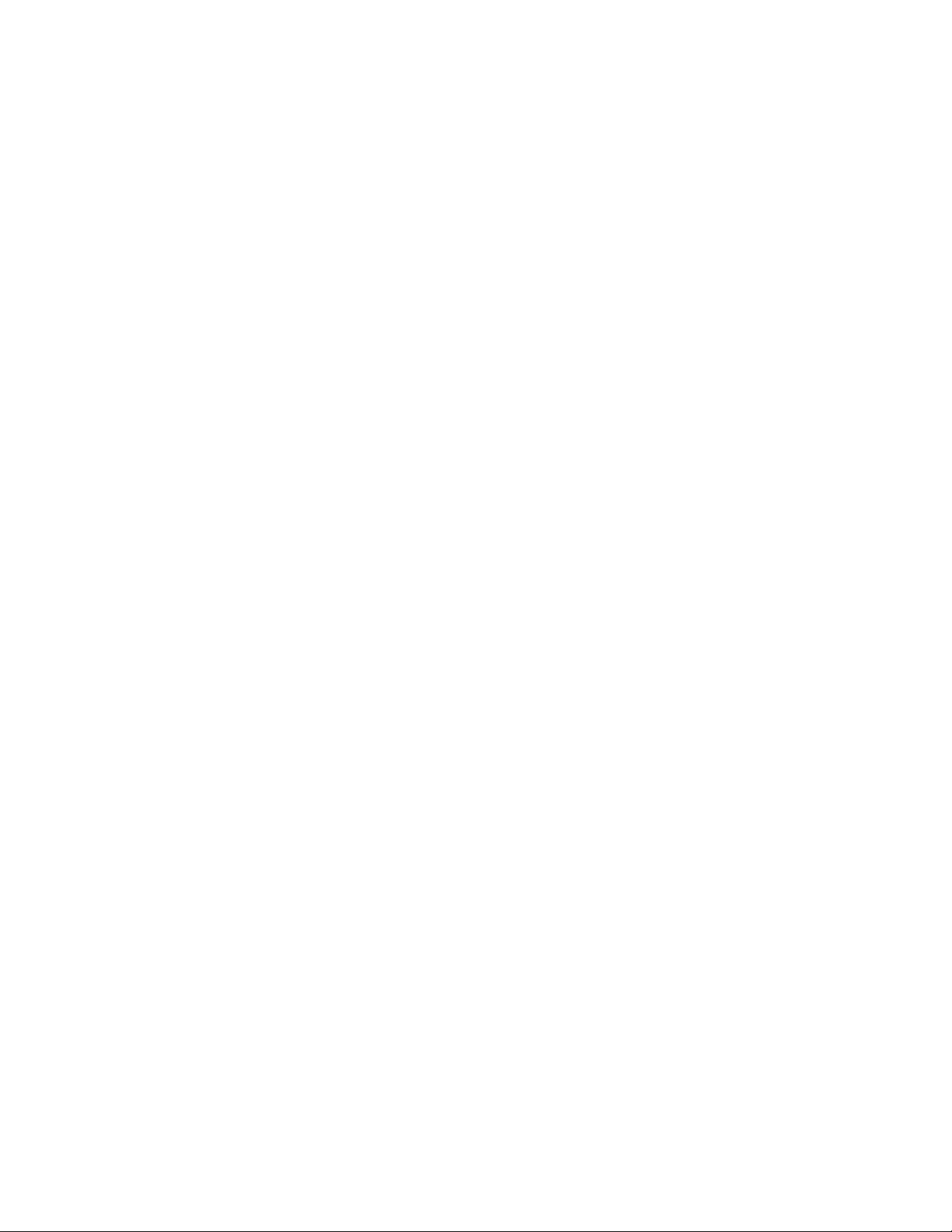
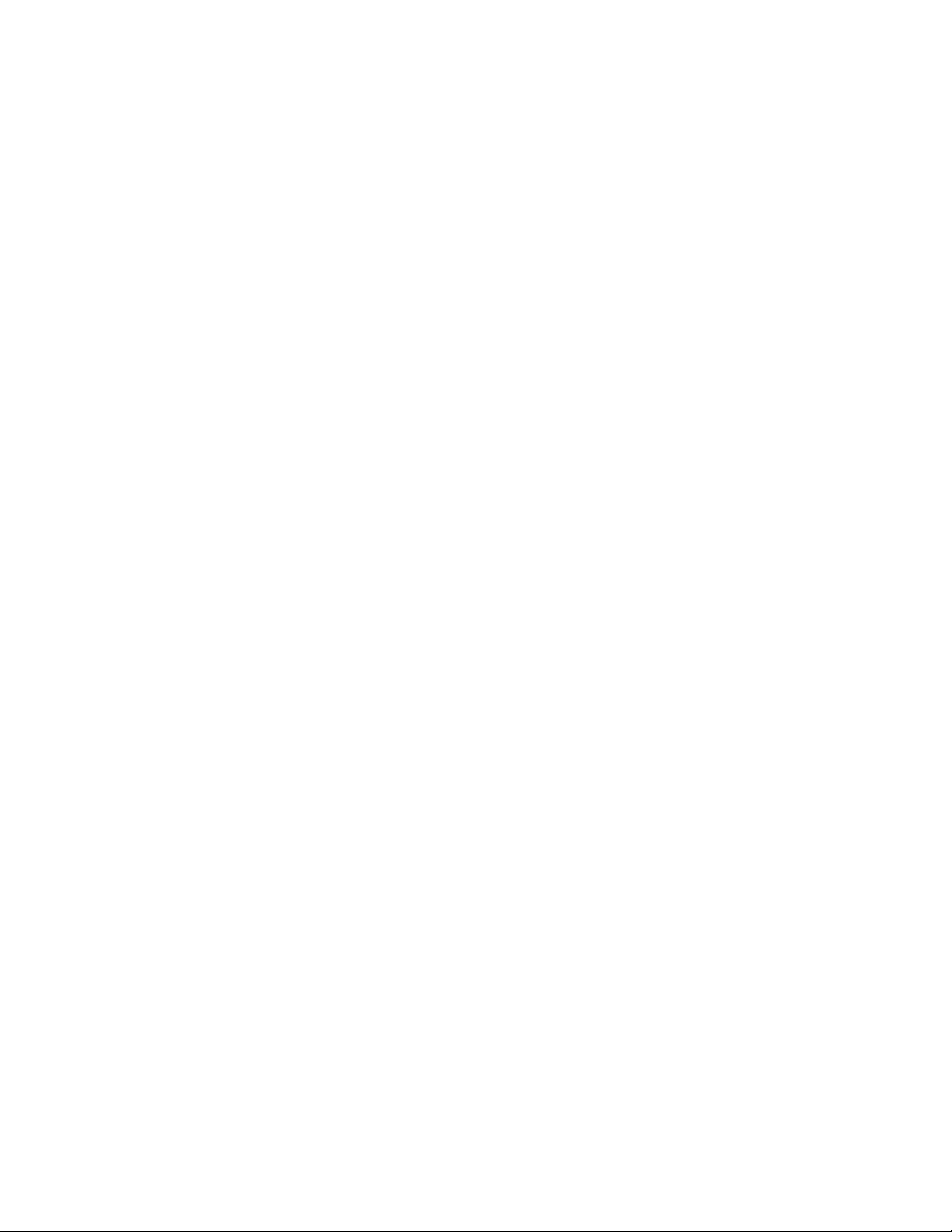
iii
Table of Contents
Introduction . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1
1 Programming Languages Supported by GCC
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 3
2 Language Standards Supported by GCC . . . . . 5
2.1 C language. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 5
2.2 C++ language . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 6
2.3 Objective-C and Objective-C++ languages . . . . . . . . . . . . . . . . . . . . . 7
3 GCC Command Options . . . . . . . . . . . . . . . . . . . . . . . 9
3.1 Option Summary. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 9
3.2 Options Controlling the Kind of Output . . . . . . . . . . . . . . . . . . . . . . . 22
3.3 Compiling C++ Programs . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 28
3.4 Options Controlling C Dialect . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 28
3.5 Options Controlling C++ Dialect . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 33
3.6 Options Controlling Objective-C and Objective-C++ Dialects . . 41
3.7 Options to Control Diagnostic Messages Formatting . . . . . . . . . . . 45
3.8 Options to Request or Suppress Warnings . . . . . . . . . . . . . . . . . . . . . 46
3.9 Options for Debugging Your Program or GCC . . . . . . . . . . . . . . . . . 67
3.10 Options That Control Optimization . . . . . . . . . . . . . . . . . . . . . . . . . . 85
3.11 Options Controlling the Preprocess or. . . . . . . . . . . . . . . . . . . . . . . . 131
3.12 Passing Options to the Assembler . . . . . . . . . . . . . . . . . . . . . . . . . . . 141
3.13 Options for Linking . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 141
3.14 Options for Directory Search . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 145
3.15 Specifying subprocesses and the switches to pass to them . . . . 147
3.16 Specifying Target Machine and Compiler Version . . . . . . . . . . . . 154
3.17 Hardware Models and Configurations . . . . . . . . . . . . . . . . . . . . . . . 154
3.17.1 ARC Options . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 154
3.17.2 ARM Options. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 155
3.17.3 AVR Options . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 159
3.17.4 Blackfin Options . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 160
3.17.5 CRIS Options. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 163
3.17.6 CRX Options . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 165
3.17.7 Darwin Options . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 165
3.17.8 DEC Alpha Options . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 169
3.17.9 DEC Alpha/VMS Options . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 173
3.17.10 FR30 Options . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 174
3.17.11 FRV Options . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 174
3.17.12 GNU/Linux Options . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 177
3.17.13 H8/300 Options. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 178
3.17.14 HPPA Options. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 178
- 1
- 2
- 3
- 4
- 5
前往页