在IT领域,数据结构是计算机科学的基础之一,而链表作为一种基本的数据结构,广泛应用于各种算法和程序设计中。本文将详细讲解C++中实现丰富功能的单链表类,帮助你更好地理解和运用这一概念。 单链表是一种线性数据结构,其中的元素不是在内存中的连续位置存储,而是每个元素(节点)包含一个指向下一个元素的指针。这种结构允许动态地增加或减少元素,而不需要预先知道元素的总数。 在提供的文件中,`LLink.h`和`Link.h`很可能是两个不同的链表类实现。通常,`Link.h`可能包含了链表节点的定义,而`LLink.h`则可能是一个完整的链表类,包含了一系列操作链表的方法。 让我们看看链表节点的定义。在C++中,一个链表节点通常包含两部分:数据成员(存储实际的元素)和指针成员(指向下一个节点)。例如: ```cpp struct ListNode { int data; // 数据成员,可以是任意类型 ListNode* next; // 指针成员,指向下一个节点 }; ``` 接下来,我们讨论单链表类`LLink`。这个类可能包括以下方法: 1. **构造函数**:初始化空链表。 2. **析构函数**:释放链表中所有节点的内存。 3. **插入元素**:在链表的特定位置插入新的节点,例如在头部(`prepend`)或尾部(`append`)。 4. **删除元素**:根据给定值删除链表中的第一个匹配节点,或者删除指定位置的节点。 5. **查找元素**:返回链表中第一个匹配给定值的节点,如果没有找到则返回`nullptr`。 6. **打印链表**:遍历链表并打印每个节点的值。 7. **长度**:返回链表中的元素数量。 8. **反转链表**:将链表的顺序反转。 9. **合并链表**:合并两个已排序的链表,保持排序顺序。 这些方法都是基于链表的特性设计的,它们通过改变节点的`next`指针来实现对链表的操作。例如,插入一个新节点需要创建一个新的节点对象,设置其数据成员,并将它的`next`指针指向原链表的第一个节点。原链表的头节点的`next`指针则被设置为新节点。 理解并能熟练使用链表类对于任何C++开发者来说都至关重要,特别是在涉及动态数据结构、高级算法如排序和搜索时。链表提供了灵活性,允许在不连续的内存空间中管理数据,这与数组等其他数据结构相比有其独特的优势。 `C++单链表类(实现丰富)`的学习涵盖了链表的基本操作和高级应用,是数据结构和算法学习的重要组成部分。通过深入研究`LLink`类的源代码,你可以更深入地了解链表的工作原理,并提升在实际项目中的应用能力。
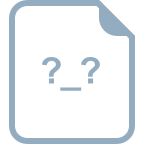
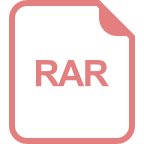
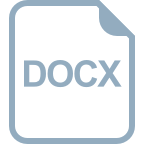
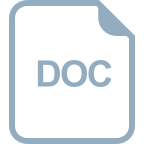
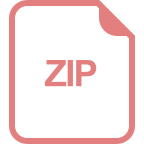
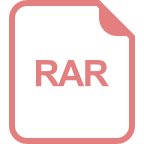
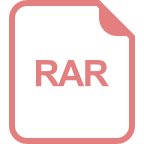
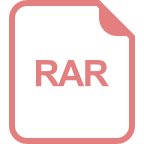
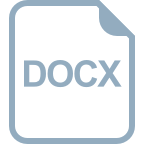
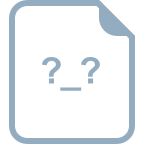
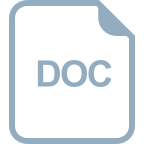

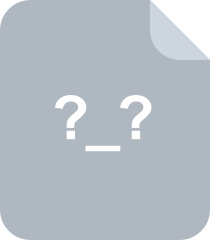
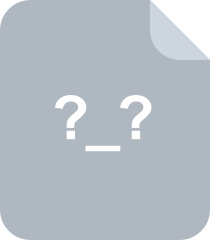
- 1
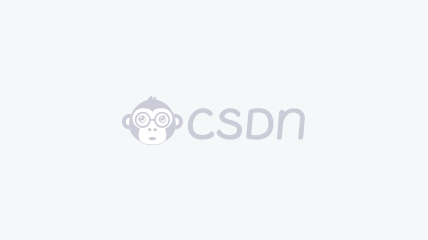

- 粉丝: 0
- 资源: 1
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

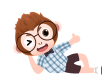
最新资源
- 创意个人相册图片网站整站模板.zip
- 创意黑板风格的BLOG网页模板下载.zip
- 创意广告介绍网站蓝色宽屏风格的模板下载.zip
- 创意红色调的公司企业整站模板.rar
- 创意简单白调的博客网页模板下载.zip
- 创意简洁白调的个人博客网页模板下载.zip
- 创意简洁的单页视差网站模板下载.zip
- 创意简洁风的欧美运动网站模板下载.zip
- 创意简洁风的个人网站设计模板下载.zip
- 创意简洁风的思维教育企业网站模板下载.zip
- 创意简洁风格的商务单页企业网站模板下载.zip
- 创意简洁风的营销策划公司网站模板下载.zip
- 创意简实灰色效果的摄影企业整站模板下载.zip
- 创意简洁风格的设计简介网页源码下载.zip
- 创意精美的IT数码产品网站模板下载.rar
- 创意简实酷炫的企业整站模板下载.zip

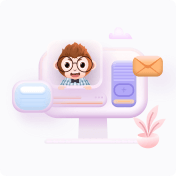
