#include "pch.h"
#include "MainPage.xaml.h"
#if __has_include("MainPage.g.cpp")
#include "MainPage.g.cpp"
#endif
#include <random>
#include <chrono>
#include <sstream>
#include <ShObjIdl.h>
#include <MainWindow.xaml.h>
using winrt::Windows::Foundation::IInspectable;
using winrt::Windows::Foundation::Rect;
using winrt::Windows::Foundation::TimeSpan;
using winrt::Windows::Foundation::Size;
using winrt::Windows::Graphics::Imaging::SoftwareBitmap;
using winrt::Windows::Graphics::Imaging::BitmapEncoder;
using winrt::Windows::Storage::Pickers::FileOpenPicker;
using winrt::Windows::Storage::Pickers::FileSavePicker;
using winrt::Windows::Storage::Pickers::PickerLocationId;
using winrt::Windows::Storage::StorageFile;
using winrt::Windows::Storage::Streams::IBuffer;
using winrt::Windows::Storage::Streams::IRandomAccessStream;
using winrt::Windows::UI::Color;
using winrt::Microsoft::Graphics::Canvas::CanvasRenderTarget;
using winrt::Microsoft::Graphics::Canvas::CanvasDrawingSession;
using winrt::Microsoft::Graphics::Canvas::CanvasBitmap;
using winrt::Microsoft::Graphics::Canvas::CanvasRenderTarget;
using winrt::Microsoft::Graphics::Canvas::Brushes::CanvasSolidColorBrush;
using winrt::Microsoft::Graphics::Canvas::Text::CanvasTextFormat;
using winrt::Microsoft::Graphics::Canvas::UI::Xaml::CanvasImageSource;
using winrt::Microsoft::UI::Xaml::RoutedEventArgs;
using winrt::Microsoft::UI::Xaml::SizeChangedEventArgs;
using winrt::Microsoft::UI::Xaml::Media::Imaging::RenderTargetBitmap;
constexpr Color CANVAS_BACKGROUND_COLOR{ 0xff, 0xa9, 0xa9, 0xa9 };
constexpr Color CURVES_BACKGROUND_COLOR{ 0xff, 0xff, 0xff, 0xff };
constexpr Color LINE_ONE_COLOR{ 0xff, 0x00, 0xa2, 0xe8 };
constexpr Color LINE_TWO_COLOR{ 0xff, 0x75, 0xfa, 0x8d };
constexpr Color TEXT_COLOR{ 0xff, 0x00, 0x00, 0x00 };
constexpr Color GRID_LINE_COLOR{ 0xff, 0x80, 0x80, 0x80 };
constexpr int LINE_COUNT{ 2 };
constexpr int LINE_POINTS{ 300 };
constexpr int32_t SAMPLING_PERIOD{ 100 };
constexpr size_t DRAWING_PERIOD{ 3 };
constexpr float X_LEFT_PADDING = 40.0f;
constexpr float X_RIGHT_PADDING = 30.0F;
constexpr float Y_TOP_PADDING = 10.0F;
constexpr float Y_BOTTOM_PADDING = 30.0F;
constexpr int GRID_X_COUNT = 15;
constexpr int GRID_Y_COUNT = 20;
constexpr int GRID_FONT_SIZE = 15;
constexpr float X_VALUE_MIN = 0.0F;
constexpr float X_VALUE_MAX = 100.0F;
constexpr float Y_VALUE_MIN = -100.0F;
constexpr float Y_VALUE_MAX = 100.0f;
namespace winrt::Win2dDemo::implementation
{
MainPage::MainPage()
:m_linesData{LINE_COUNT, LINE_POINTS, X_LEFT_PADDING, Y_TOP_PADDING, X_RIGHT_PADDING, Y_BOTTOM_PADDING}
{
}
/// <summary>
/// 获取信号值。
/// 这里采用随机数,模拟采集到的数据。
/// </summary>
/// <param name="minValue">数据范围最小值。</param>
/// <param name="maxValue">数据范围最大值。</param>
/// <returns>每次调用该函数,都返回一个模拟的信号值。</returns>
float MainPage::GetSignalValue(float minValue, float maxValue)
{
static std::random_device device{};
static std::mt19937_64 engine{ device() };
std::uniform_real_distribution<float> dist{ minValue, maxValue };
return dist(engine);
}
void MainPage::Resize()
{
auto size = Win2dCanvas().ActualSize();
m_canvasWidth = size.x;
m_canvasHeight = size.y;
m_linesData.Resize(m_canvasWidth, m_canvasHeight);
}
/// <summary>
/// 初始化绘图区域。
/// </summary>
void MainPage::InitializeCanvas()
{
// 1. 重新计算图片大小。
Resize();
// 2. 重新计算 x 坐标。
if (!m_shouldDrawLines)
{
m_linesData.SetXCoordinates(m_canvasWidth);
m_pointsCounter = 0;
}
// 3. 绘制界面
m_canvasImageSource = CanvasImageSource(Win2dCanvas().Device(), m_canvasWidth, m_canvasHeight, 96.0f);
UIImage().Source(m_canvasImageSource);
auto ds = m_canvasImageSource.CreateDrawingSession(CANVAS_BACKGROUND_COLOR);
DrawGrids(ds);
if (m_shouldDrawLines)
{
DrawLines(ds);
}
}
/// <summary>
/// UI 加载完成,才能初始化绘图区域,否则会报错。
/// </summary>
/// <param name=""></param>
/// <param name=""></param>
void MainPage::LoadedTimer_Tick(IInspectable const&, IInspectable const&)
{
m_textBrush = CanvasSolidColorBrush{ Win2dCanvas(), TEXT_COLOR };
m_textFormat = CanvasTextFormat{};
m_textFormat.FontFamily(L"Consolas");
m_textFormat.FontSize(GRID_FONT_SIZE);
m_textFormat.FontStyle(Windows::UI::Text::FontStyle::Italic);
InitializeCanvas();
m_loadedTimer.Stop();
}
/// <summary>
/// 每100ms,调用一次这个函数。模拟数据采集,并在此更新绘图。
/// </summary>
/// <param name=""></param>
/// <param name=""></param>
void MainPage::DrawLinesTimer_Tick(IInspectable const&, IInspectable const&)
{
if (m_isPaused)
return;
auto t1 = std::chrono::high_resolution_clock::now();
auto validHeight = m_canvasHeight - Y_TOP_PADDING - Y_BOTTOM_PADDING;
m_linesData.SetYCoordinates(0, m_pointsCounter, (1.0f - GetSignalValue(0.1f, 0.3f)) * validHeight + Y_TOP_PADDING);
m_linesData.SetYCoordinates(1, m_pointsCounter, (1.0f - GetSignalValue(0.6f, 0.7f)) * validHeight + Y_TOP_PADDING);
if (++m_pointsCounter % DRAWING_PERIOD == (DRAWING_PERIOD - 1))
{
auto ds = m_canvasImageSource.CreateDrawingSession(CANVAS_BACKGROUND_COLOR);
DrawGrids(ds);
DrawLines(ds);
auto t2 = std::chrono::high_resolution_clock::now();
ShowUsedTime(t1, t2);
}
}
/// <summary>
/// 绘制 X,Y 坐标系。
/// </summary>
/// <param name="ds"></param>
void MainPage::DrawGrids(Microsoft::Graphics::Canvas::CanvasDrawingSession const& ds) const
{
auto validHeight = m_canvasHeight - Y_TOP_PADDING - Y_BOTTOM_PADDING;
auto validWidth = m_canvasWidth - X_LEFT_PADDING - X_RIGHT_PADDING;
float dx = validWidth / GRID_X_COUNT;
float dy = validHeight / GRID_Y_COUNT;
float xOffset = -14.0f;
float yOffset = -10.0f;
ds.FillRectangle(Rect{ X_LEFT_PADDING, Y_TOP_PADDING, validWidth, validHeight }, CURVES_BACKGROUND_COLOR);
constexpr double dxValue = (X_VALUE_MAX - X_VALUE_MIN) / GRID_X_COUNT;
double xValueOffset = max(0.0, 1.0 * m_pointsCounter - LINE_POINTS) * (X_VALUE_MAX - X_VALUE_MIN) / LINE_POINTS;
for (int i = 0; i <= GRID_X_COUNT; i++)
{
auto x = i * dx + X_LEFT_PADDING;
auto y = m_canvasHeight - Y_BOTTOM_PADDING;
double value = round(dxValue * i + xValueOffset);
ds.DrawTextW(winrt::to_hstring(value), float2{ x + xOffset, y }, m_textBrush, m_textFormat);
if (i > 0 && i < GRID_X_COUNT)
ds.DrawLine(float2{ x, y }, float2{ x, Y_TOP_PADDING }, GRID_LINE_COLOR, 1.0f);
}
constexpr double dyValue = (Y_VALUE_MAX - Y_VALUE_MIN) / GRID_Y_COUNT;
for (int i = 1; i < GRID_Y_COUNT; i++)
{
auto x = X_LEFT_PADDING - 30.0f;
auto y = i * dy + Y_TOP_PADDING;
double value = round(dyValue * (GRID_Y_COUNT - i) + Y_VALUE_MIN);
ds.DrawTextW(winrt::to_hstring(value), float2{ x,y + yOffset }, m_textBrush, m_textFormat);
ds.DrawLine(float2{ X_LEFT_PADDING, y }, float2{ m_canvasWidth - X_RIGHT_PADDING, y }, GRID_LINE_COLOR, 1.0F);
}
}
/// <summary>
/// 绘制两条曲线。
/// </summary>
/// <param name="ds"></param>
/// <param name="count"></param>
void MainPage::DrawLines(Microsoft::Graphics::Canvas::CanvasDrawingSession const& ds) const
{
size_t count = min(m_pointsCounter, LINE_POINTS);
for (size_t i = 1; i < count; ++i)
{
ds.DrawLine(m_linesData[0][i - 1], m_linesData[0][i], LINE_ONE_COLOR, 2.0F);
ds.DrawLine(m_linesData[1][i - 1], m_linesData[1][i], LINE_TWO_COLOR, 2.0F);
}
}
void MainPage::ShowUsedTime(std::chrono::high_resolution_clock::time_point t1, std::chrono::high_resolution_clock::time_point t2)
{
auto usedTime = t2 - t1;
std::wstringstream ss;
ss << L"绘图时间: " << usedTime.count() / 1e6 << L"ms";
CostTimeTextBlock().Text(ss.str());
}
/// <summary>
/// 页面加载完成,启动定时器。
/// </summary>
/// <param name=""></param>
/// <param name=""></param>
void MainPage::Pag
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
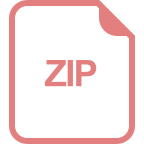
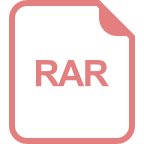
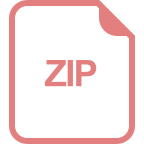
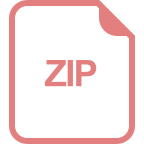
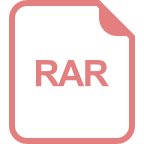
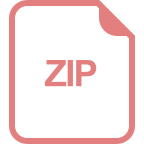
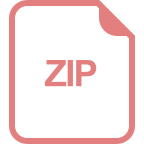
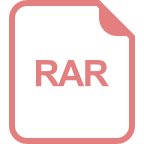
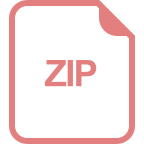
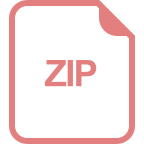
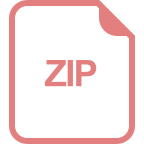
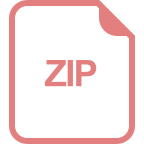
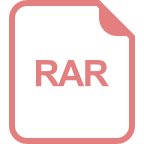
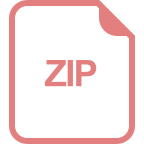
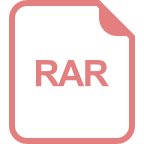
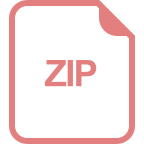
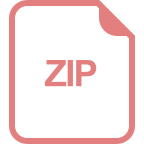
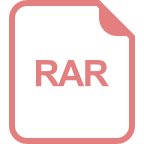
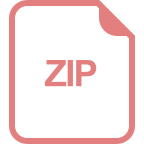
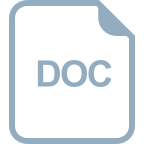
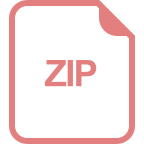
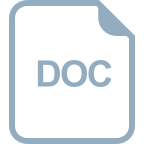
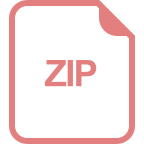
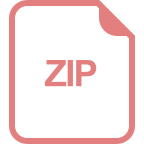
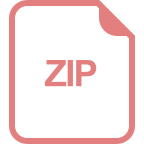
收起资源包目录

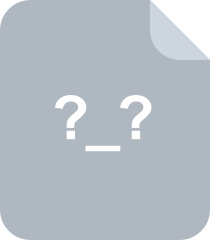
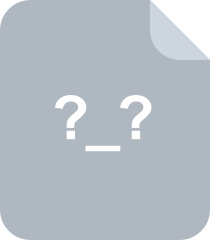

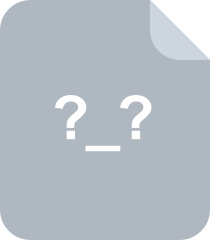
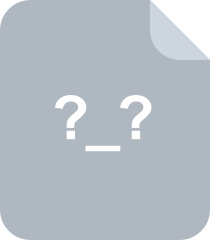
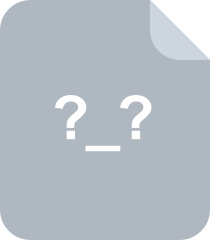
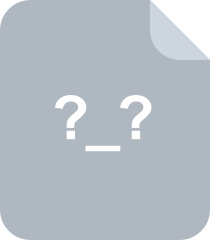
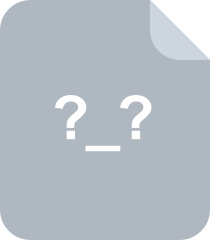
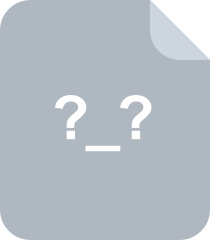
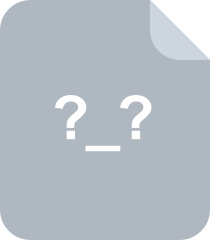
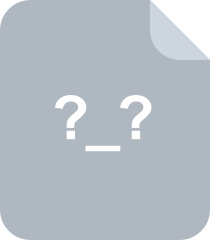
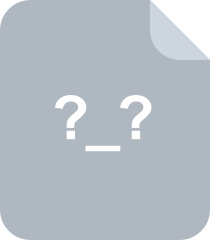
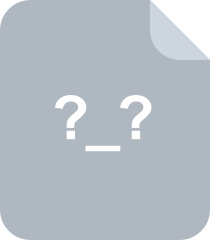
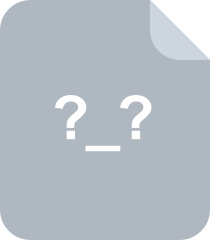
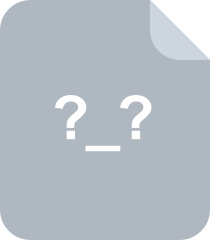
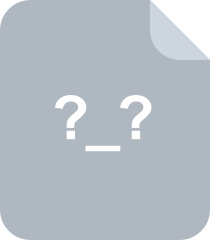

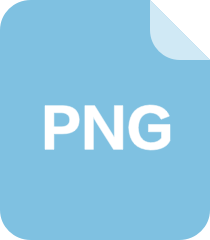
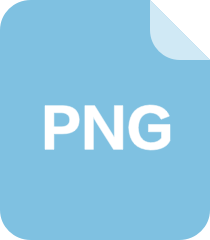
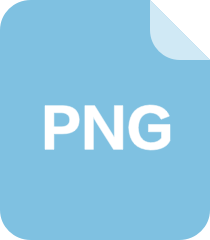
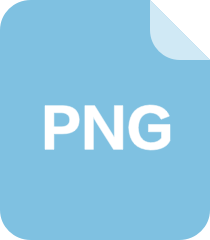
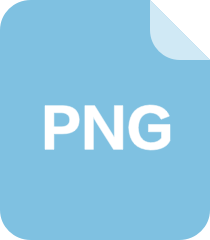
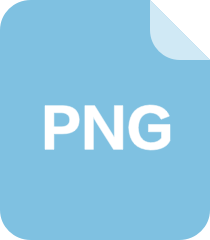
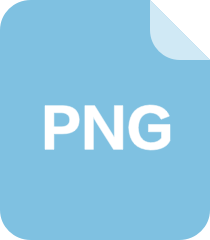
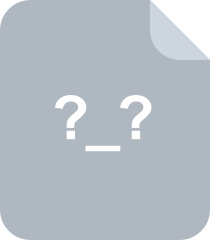
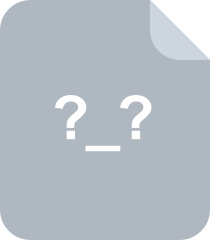
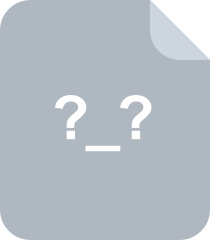
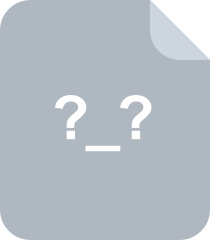
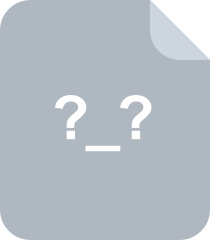
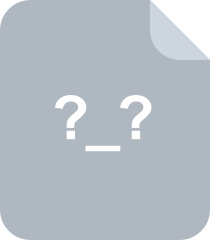
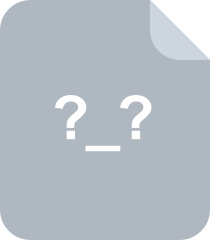
共 29 条
- 1
资源评论
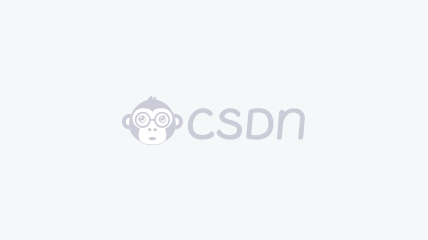

DENG-TAO
- 粉丝: 48
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

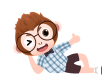
最新资源
- 通过go语言实现单例模式(Singleton Pattern).rar
- 通过python实现简单贪心算法示例.rar
- C语言中指针基本概念及应用详解
- (源码)基于Websocket和C++的咖啡机器人手臂控制系统.zip
- (源码)基于深度学习和LoRA技术的图书问答系统.zip
- (源码)基于Servlet和Vue的机动车车辆车库管理系统.zip
- (源码)基于ESP32C3和WiFi的LED控制系统.zip
- (源码)基于Spring Boot和Quartz的定时任务管理系统.zip
- (源码)基于jnetpcap框架的网络流量监控系统.zip
- (源码)基于Spring Boot和WebSocket的FTP部署管理系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


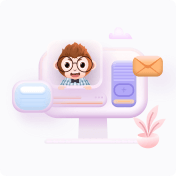
安全验证
文档复制为VIP权益,开通VIP直接复制
