/*
Fork by Martin Lucas Golini
Original author
Jonathan Dummer
2007-07-26-10.36
Simple OpenGL Image Library 2
Public Domain
using Sean Barret's stb_image as a base
Thanks to:
* Sean Barret - for the awesome stb_image
* Dan Venkitachalam - for finding some non-compliant DDS files, and patching some explicit casts
* everybody at gamedev.net
*/
#define SOIL_CHECK_FOR_GL_ERRORS 0
#if defined( __APPLE_CC__ ) || defined ( __APPLE__ )
#include <TargetConditionals.h>
#if defined( __IPHONE__ ) || ( defined( TARGET_OS_IPHONE ) && TARGET_OS_IPHONE ) || ( defined( TARGET_IPHONE_SIMULATOR ) && TARGET_IPHONE_SIMULATOR )
#define SOIL_PLATFORM_IOS
#include <dlfcn.h>
#else
#define SOIL_PLATFORM_OSX
#endif
#elif defined( __ANDROID__ ) || defined( ANDROID )
#define SOIL_PLATFORM_ANDROID
#elif ( defined ( linux ) || defined( __linux__ ) || defined( __FreeBSD__ ) || defined(__OpenBSD__) || defined( __NetBSD__ ) || defined( __DragonFly__ ) || defined( __SVR4 ) )
#define SOIL_X11_PLATFORM
#endif
#if ( defined( SOIL_PLATFORM_IOS ) || defined( SOIL_PLATFORM_ANDROID ) ) && ( !defined( SOIL_GLES1 ) && !defined( SOIL_GLES2 ) )
#define SOIL_GLES2
#endif
#if ( defined( SOIL_GLES2 ) || defined( SOIL_GLES1 ) ) && !defined( SOIL_NO_EGL ) && !defined( SOIL_PLATFORM_IOS )
#include <EGL/egl.h>
#endif
#if defined( SOIL_GLES2 )
#ifdef SOIL_PLATFORM_IOS
#include <OpenGLES/ES2/gl.h>
#include <OpenGLES/ES2/glext.h>
#else
#include <GLES2/gl2.h>
#include <GLES2/gl2ext.h>
#endif
#define APIENTRY GL_APIENTRY
#elif defined( SOIL_GLES1 )
#ifndef GL_GLEXT_PROTOTYPES
#define GL_GLEXT_PROTOTYPES
#endif
#ifdef SOIL_PLATFORM_IOS
#include <OpenGLES/ES1/gl.h>
#include <OpenGLES/ES1/glext.h>
#else
#include <GLES/gl.h>
#include <GLES/glext.h>
#endif
#define APIENTRY GL_APIENTRY
#else
#if defined( __WIN32__ ) || defined( _WIN32 ) || defined( WIN32 )
#define SOIL_PLATFORM_WIN32
#define WIN32_LEAN_AND_MEAN
#include <windows.h>
#include <wingdi.h>
#include <GL/gl.h>
#elif defined(__APPLE__) || defined(__APPLE_CC__)
/* I can't test this Apple stuff! */
#include <OpenGL/gl.h>
#include <Carbon/Carbon.h>
#define APIENTRY
#elif defined( SOIL_X11_PLATFORM )
#include <GL/gl.h>
#include <GL/glx.h>
#else
#include <GL/gl.h>
#endif
#endif
#ifndef GL_BGRA
#define GL_BGRA 0x80E1
#endif
#ifndef GL_RG
#define GL_RG 0x8227
#endif
#ifndef GL_UNSIGNED_SHORT_4_4_4_4
#define GL_UNSIGNED_SHORT_4_4_4_4 0x8033
#endif
#ifndef GL_UNSIGNED_SHORT_5_5_5_1
#define GL_UNSIGNED_SHORT_5_5_5_1 0x8034
#endif
#ifndef GL_UNSIGNED_SHORT_5_6_5
#define GL_UNSIGNED_SHORT_5_6_5 0x8363
#endif
#ifndef GL_UNSIGNED_BYTE_3_3_2
#define GL_UNSIGNED_BYTE_3_3_2 0x8032
#endif
#include "SOIL2.h"
#define STB_IMAGE_IMPLEMENTATION
#include "stb_image.h"
#define STB_IMAGE_WRITE_IMPLEMENTATION
#include "stb_image_write.h"
#include "image_helper.h"
#include "image_DXT.h"
#include "pvr_helper.h"
#include "pkm_helper.h"
#include "jo_jpeg.h"
#include <stdlib.h>
#include <string.h>
/* error reporting */
const char *result_string_pointer = "SOIL initialized";
/* for loading cube maps */
enum{
SOIL_CAPABILITY_UNKNOWN = -1,
SOIL_CAPABILITY_NONE = 0,
SOIL_CAPABILITY_PRESENT = 1
};
static int has_cubemap_capability = SOIL_CAPABILITY_UNKNOWN;
int query_cubemap_capability( void );
#define SOIL_TEXTURE_WRAP_R 0x8072
#define SOIL_CLAMP_TO_EDGE 0x812F
#define SOIL_NORMAL_MAP 0x8511
#define SOIL_REFLECTION_MAP 0x8512
#define SOIL_TEXTURE_CUBE_MAP 0x8513
#define SOIL_TEXTURE_BINDING_CUBE_MAP 0x8514
#define SOIL_TEXTURE_CUBE_MAP_POSITIVE_X 0x8515
#define SOIL_TEXTURE_CUBE_MAP_NEGATIVE_X 0x8516
#define SOIL_TEXTURE_CUBE_MAP_POSITIVE_Y 0x8517
#define SOIL_TEXTURE_CUBE_MAP_NEGATIVE_Y 0x8518
#define SOIL_TEXTURE_CUBE_MAP_POSITIVE_Z 0x8519
#define SOIL_TEXTURE_CUBE_MAP_NEGATIVE_Z 0x851A
#define SOIL_PROXY_TEXTURE_CUBE_MAP 0x851B
#define SOIL_MAX_CUBE_MAP_TEXTURE_SIZE 0x851C
/* for non-power-of-two texture */
#define SOIL_IS_POW2( v ) ( ( v & ( v - 1 ) ) == 0 )
static int has_NPOT_capability = SOIL_CAPABILITY_UNKNOWN;
int query_NPOT_capability( void );
/* for texture rectangles */
static int has_tex_rectangle_capability = SOIL_CAPABILITY_UNKNOWN;
int query_tex_rectangle_capability( void );
#define SOIL_TEXTURE_RECTANGLE_ARB 0x84F5
#define SOIL_MAX_RECTANGLE_TEXTURE_SIZE_ARB 0x84F8
/* for using DXT compression */
static int has_DXT_capability = SOIL_CAPABILITY_UNKNOWN;
int query_DXT_capability( void );
static int has_3Dc_capability = SOIL_CAPABILITY_UNKNOWN;
int query_3Dc_capability( void );
#define SOIL_GL_SRGB 0x8C40
#define SOIL_GL_SRGB_ALPHA 0x8C42
#define SOIL_RGB_S3TC_DXT1 0x83F0
#define SOIL_RGBA_S3TC_DXT1 0x83F1
#define SOIL_RGBA_S3TC_DXT3 0x83F2
#define SOIL_RGBA_S3TC_DXT5 0x83F3
#define SOIL_COMPRESSED_RG_RGTC2 0x8DBD
#define SOIL_GL_COMPRESSED_SRGB_S3TC_DXT1_EXT 0x8C4C
#define SOIL_GL_COMPRESSED_SRGB_ALPHA_S3TC_DXT5_EXT 0x8C4F
static int has_sRGB_capability = SOIL_CAPABILITY_UNKNOWN;
int query_sRGB_capability( void );
typedef void (APIENTRY * P_SOIL_GLCOMPRESSEDTEXIMAGE2DPROC) (GLenum target, GLint level, GLenum internalformat, GLsizei width, GLsizei height, GLint border, GLsizei imageSize, const GLvoid * data);
static P_SOIL_GLCOMPRESSEDTEXIMAGE2DPROC soilGlCompressedTexImage2D = NULL;
typedef void (APIENTRY *P_SOIL_GLGENERATEMIPMAPPROC)(GLenum target);
static P_SOIL_GLGENERATEMIPMAPPROC soilGlGenerateMipmap = NULL;
static int has_gen_mipmap_capability = SOIL_CAPABILITY_UNKNOWN;
static int query_gen_mipmap_capability( void );
static int has_PVR_capability = SOIL_CAPABILITY_UNKNOWN;
int query_PVR_capability( void );
static int has_BGRA8888_capability = SOIL_CAPABILITY_UNKNOWN;
int query_BGRA8888_capability( void );
static int has_ETC1_capability = SOIL_CAPABILITY_UNKNOWN;
int query_ETC1_capability( void );
/* GL_IMG_texture_compression_pvrtc */
#define SOIL_COMPRESSED_RGB_PVRTC_4BPPV1_IMG 0x8C00
#define SOIL_COMPRESSED_RGB_PVRTC_2BPPV1_IMG 0x8C01
#define SOIL_COMPRESSED_RGBA_PVRTC_4BPPV1_IMG 0x8C02
#define SOIL_COMPRESSED_RGBA_PVRTC_2BPPV1_IMG 0x8C03
#define SOIL_GL_ETC1_RGB8_OES 0x8D64
#if defined( SOIL_X11_PLATFORM ) || defined( SOIL_PLATFORM_WIN32 ) || defined( SOIL_PLATFORM_OSX ) || defined(__HAIKU__)
typedef const GLubyte *(APIENTRY * P_SOIL_glGetStringiFunc) (GLenum, GLuint);
static P_SOIL_glGetStringiFunc soilGlGetStringiFunc = NULL;
static int isAtLeastGL3()
{
static int is_gl3 = SOIL_CAPABILITY_UNKNOWN;
if ( SOIL_CAPABILITY_UNKNOWN == is_gl3 )
{
const char * verstr = (const char *) glGetString( GL_VERSION );
is_gl3 = ( verstr && ( atoi(verstr) >= 3 ) &&
strstr( verstr, " ES " ) == NULL );
}
return is_gl3;
}
#else
static int isAtLeastGL3()
{
return SOIL_CAPABILITY_NONE;
}
#endif
#ifdef SOIL_PLATFORM_WIN32
static HMODULE openglModule = NULL;
static int soilTestWinProcPointer(const PROC pTest)
{
ptrdiff_t iTest;
if(!pTest) return 0;
iTest = (ptrdiff_t)pTest;
if(iTest == 1 || iTest == 2 || iTest == 3 || iTest == -1) return 0;
return 1;
}
#endif
#if defined(__sgi) || defined (__sun) || defined(__HAIKU__)
#include <dlfcn.h>
void* dlGetProcAddress (const char* name)
{
static void* h = NULL;
static void* gpa;
if (h == NULL)
{
if ((h = dlopen(NULL, RTLD_LAZY | RTLD_LOCAL)) == NULL) return NULL;
gpa = dlsym(h, "glXGetProcAddress");
}
if (gpa != NULL)
return ((void*(*)(const GLubyte*))gpa)((const GLubyte*)name);
else
return dlsym(h, (const char*)name);
}
#endif
void * SOIL_GL_GetProcAddress(const char *proc)
{
void *func = NULL;
#if defined( SOIL_PLATFORM_IOS )
func = dlsym( RTLD_DEFAULT, proc );
#elif defined( SOIL_GLES2
没有合适的资源?快使用搜索试试~ 我知道了~
openGL高度贴图,使用纹理图像来存储高度值,然后使用该高度值来提升(或降低)顶 点位置。
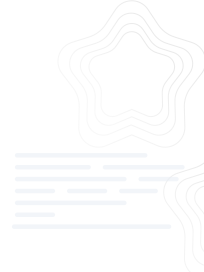
共437个文件
hpp:151个
inl:116个
h:109个

需积分: 50 11 下载量 28 浏览量
2022-03-26
16:16:59
上传
评论
收藏 42.5MB RAR 举报
温馨提示
1.现在我们扩展法线贴图的概念——从纹理图像用于扰动法向量到扰乱顶点位置本身。实 际上,以这种方式修改对象的几何体具有一定的优势,例如使表面特征沿着对象的边缘可 见,并使特征能够响应阴影贴图。我们将会看到,它还可以帮助构建地形。 2.openGL高度贴图,使用纹理图像来存储高度值,然后使用该高度值来提升(或降低)顶 点位置。含有高度信息的图像称为高度图,使用高度图更改对象的顶点的方法称为高度贴 图 3. 高度图通常将高度信息编码为灰度颜色:(0,0,0)(黑色)=低高度,(1,1,1)(白色)=高高度。这样一来通过算法或使用“画图”程序就可以轻松创建高度图。图像的对比度越高,其表示的高度变化越大。 4.改变顶点位置是否有用取决于改变的模型。顶点操作可以在顶点着色器中轻松完成,当 模型顶点细节级别够高(例如在足够高精度的球体中)时,改变顶点高度的方法效果很好。但是,当模型的顶点数量很少(例如立方体的角)时,渲染对象的表面需要依赖于光栅器中的顶点插值来填充细节。 5.当顶点着色器中可用于改变高度的顶点很少时,许多像素的高 度将无法从高度图中检索,而需要由插值生成,从而导致表面细节较差。当然
资源详情
资源评论
资源推荐
收起资源包目录

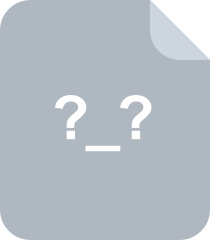
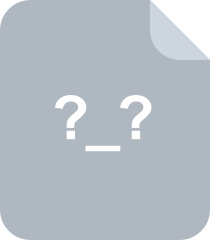
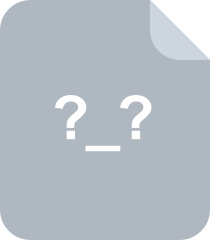
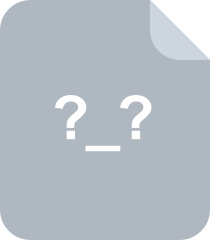
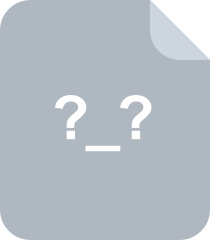
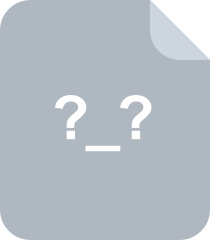
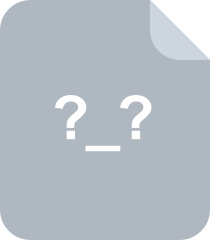
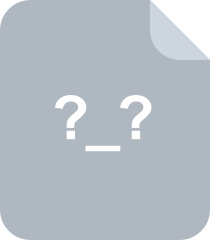
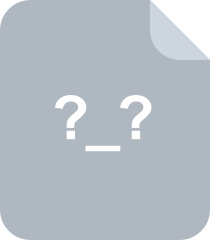
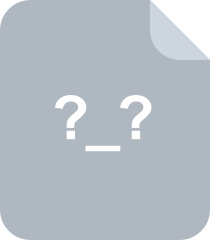
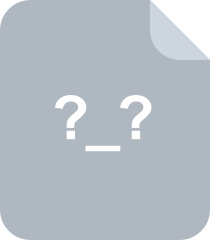
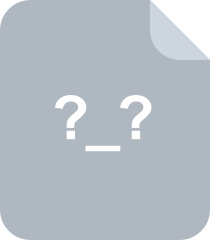
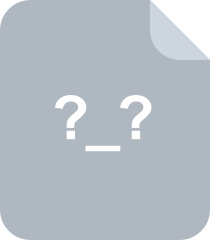
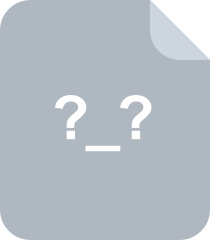
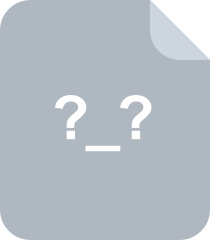
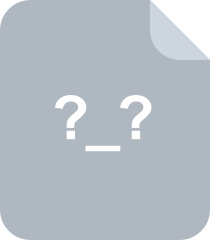
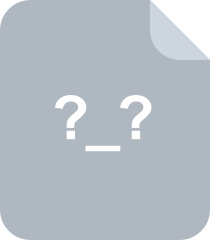
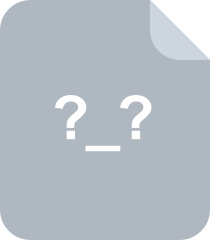
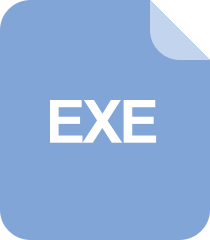
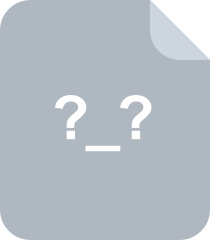
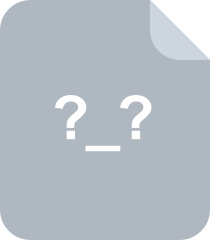
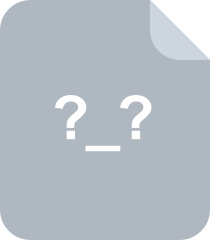
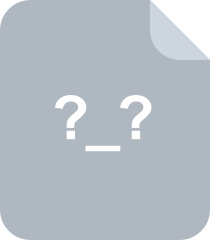
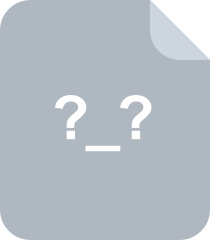
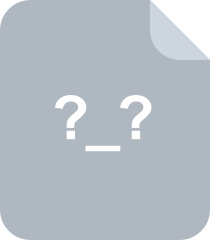
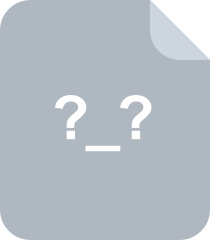
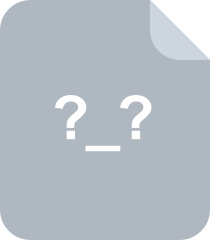
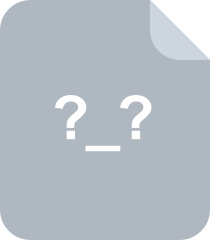
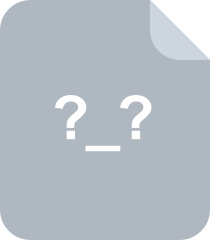
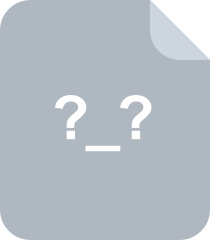
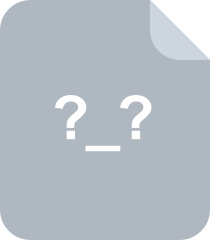
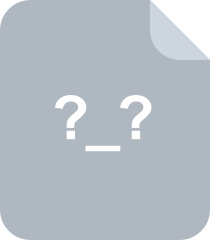
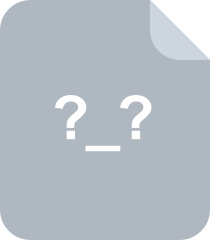
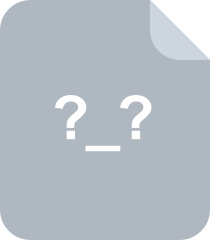
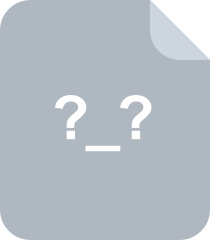
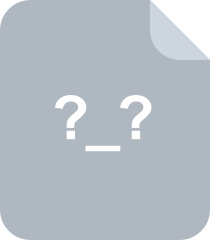
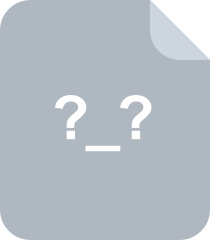
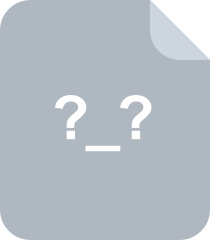
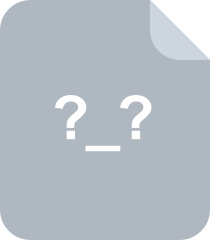
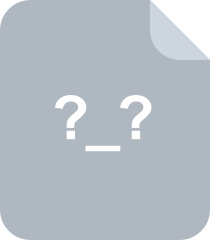
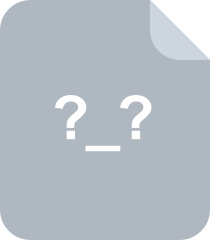
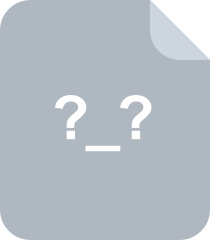
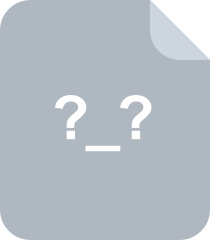
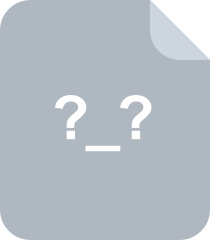
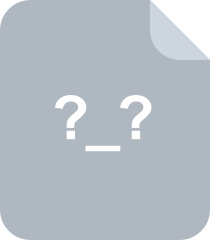
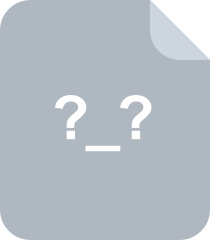
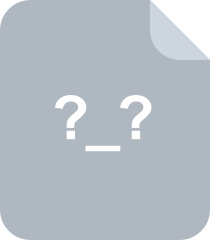
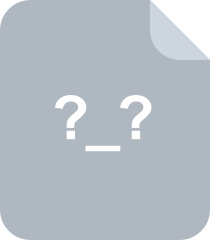
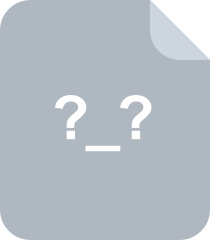
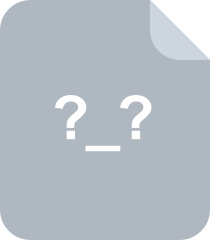
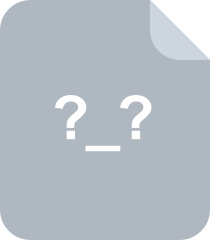
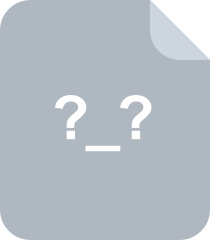
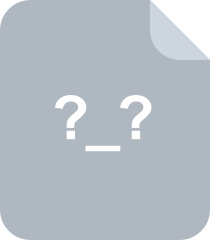
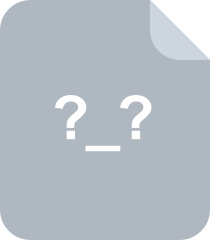
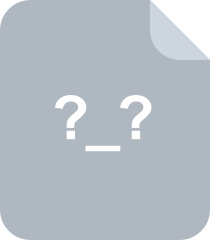
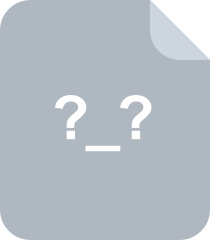
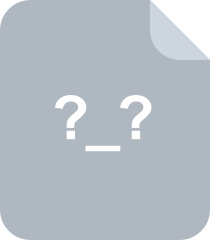
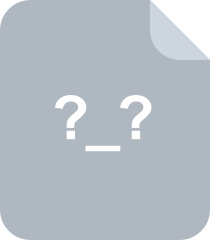
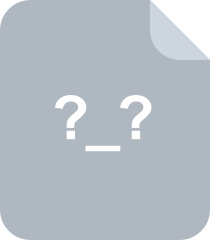
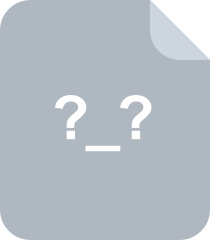
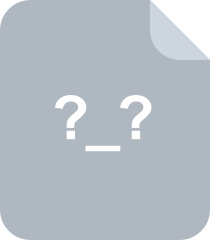
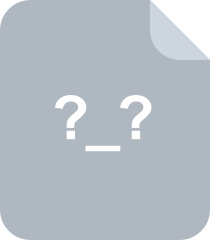
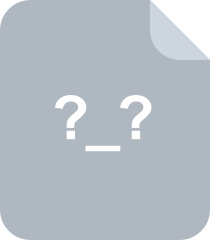
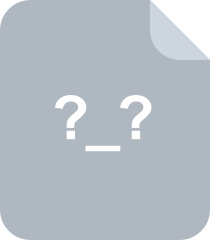
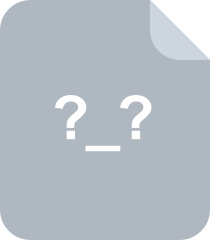
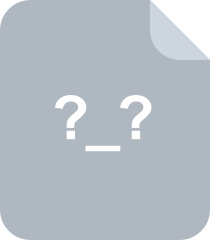
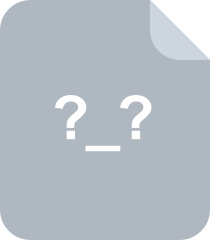
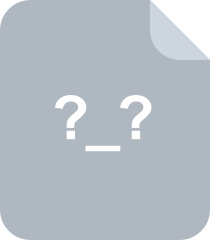
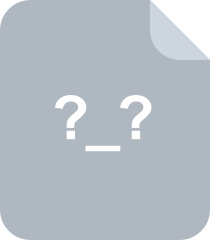
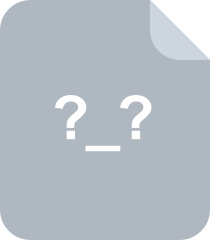
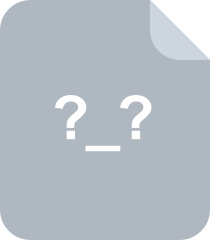
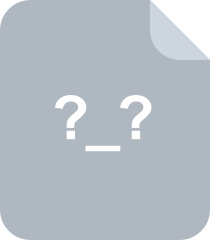
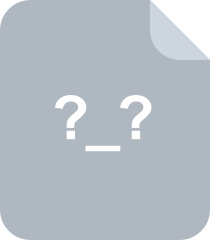
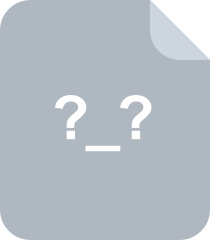
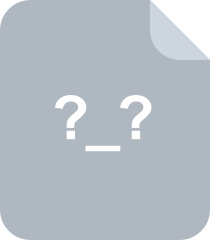
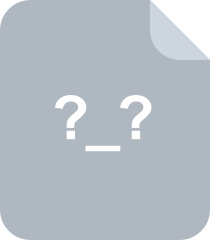
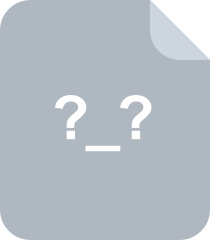
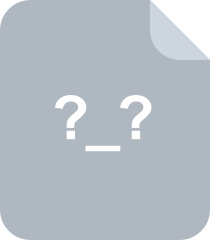
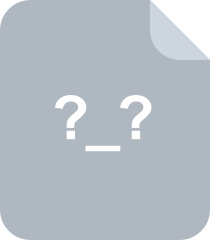
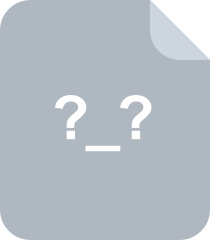
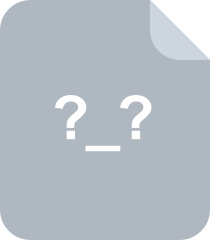
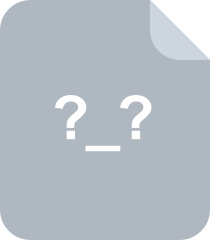
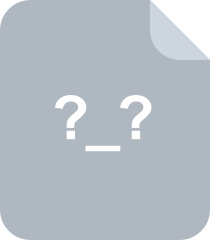
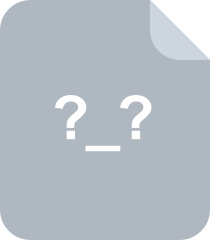
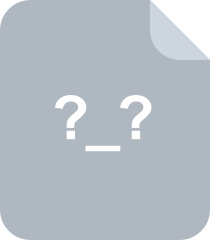
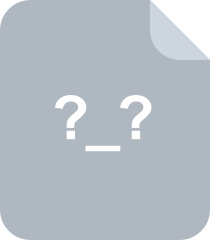
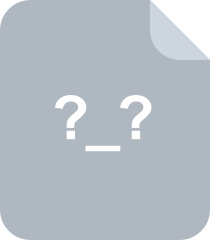
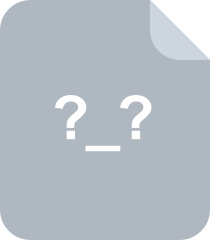
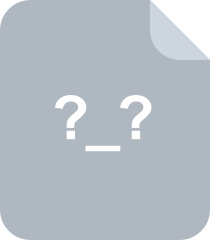
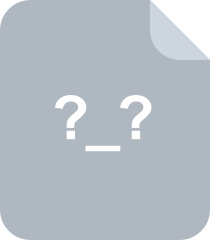
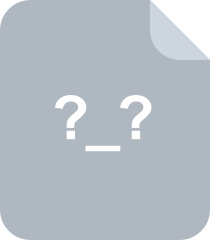
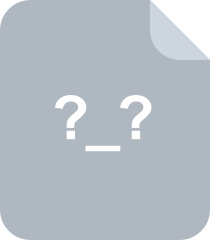
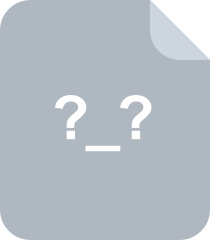
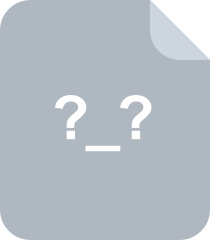
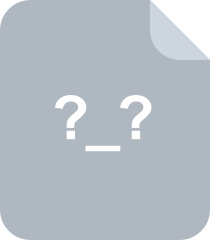
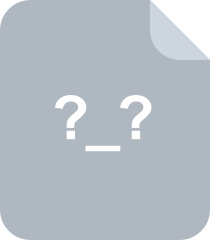
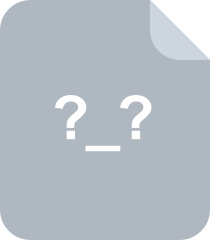
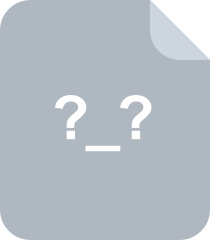
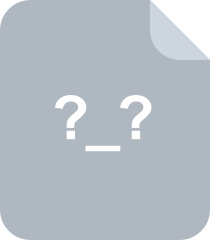
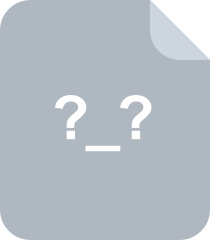
共 437 条
- 1
- 2
- 3
- 4
- 5
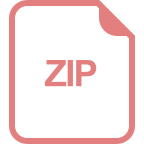
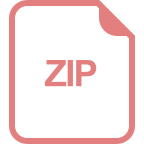
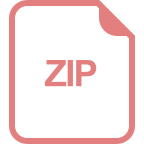
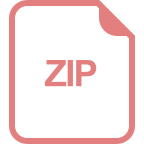
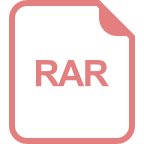
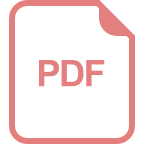
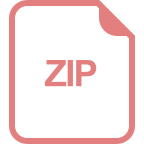
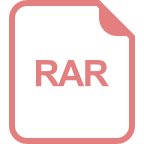
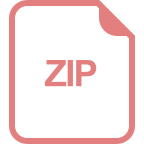
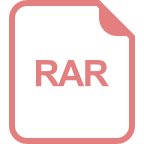
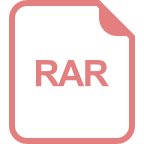
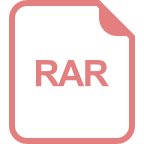
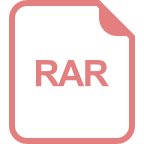
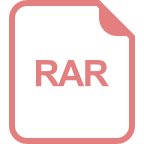
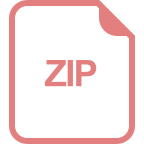
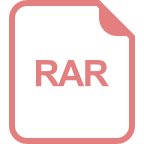
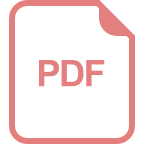
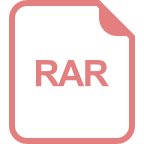
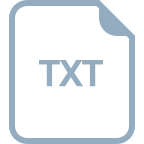
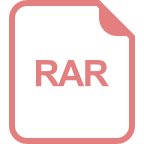
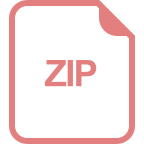
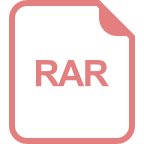
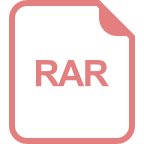
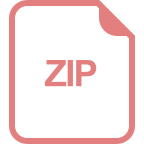
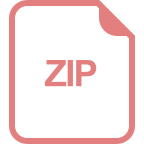


妙为
- 粉丝: 897
- 资源: 206
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

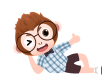
最新资源
- (源码)基于Spring Boot和Vue的后台管理系统.zip
- 用于将 Power BI 嵌入到您的应用中的 JavaScript 库 查看文档网站和 Wiki 了解更多信息 .zip
- (源码)基于Arduino、Python和Web技术的太阳能监控数据管理系统.zip
- (源码)基于Arduino的CAN总线传感器与执行器通信系统.zip
- (源码)基于C++的智能电力系统通信协议实现.zip
- 用于 Java 的 JSON-RPC.zip
- 用 JavaScript 重新实现计算机科学.zip
- (源码)基于PythonOpenCVYOLOv5DeepSort的猕猴桃自动计数系统.zip
- 用 JavaScript 编写的贪吃蛇游戏 .zip
- (源码)基于ASP.NET Core的美术课程管理系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


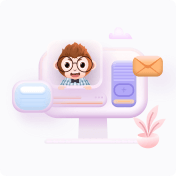
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0