自定义对话框
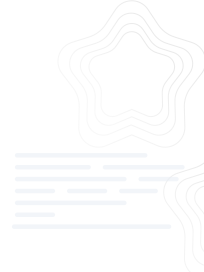

在Android或iOS等移动开发平台,以及Windows、Mac等桌面应用程序中,自定义对话框(Custom Dialog)是一种常见的用户界面元素。它允许开发者为用户提供更加个性化、功能丰富的交互体验,相比系统默认的对话框,自定义对话框可以更好地融入应用的整体设计风格。本教程将深入探讨如何创建和实现一个自定义的登录对话框。 ### 1. 自定义对话框的基本概念 自定义对话框是开发者根据具体需求设计的一种弹出式窗口,它可以包含任何视图元素,如输入框、按钮、图片、进度条等。自定义对话框通常用于显示警告信息、获取用户输入或进行特定操作前的确认。 ### 2. Android中的自定义对话框 在Android中,我们可以使用`AlertDialog.Builder`或者自定义布局的方式来实现。我们需要创建一个XML布局文件,用于定义对话框的视图结构。例如,`dialog_login.xml`可能包含两个`EditText`(用户名和密码)、一个“登录”按钮和一个“取消”按钮。 ```xml <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="vertical" android:padding="16dp"> <EditText android:id="@+id/et_username" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="用户名" /> <EditText android:id="@+id/et_password" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="密码" android:inputType="textPassword" /> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal" android:gravity="end"> <Button android:id="@+id/btn_cancel" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="取消" /> <Button android:id="@+id/btn_login" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="登录" /> </LinearLayout> </LinearLayout> ``` 然后,在Java或Kotlin代码中,我们可以加载这个布局并设置对话框: ```java // 创建Builder AlertDialog.Builder builder = new AlertDialog.Builder(context); // 加载自定义布局 View dialogView = LayoutInflater.from(context).inflate(R.layout.dialog_login, null); builder.setView(dialogView); // 获取并设置控件 EditText etUsername = dialogView.findViewById(R.id.et_username); EditText etPassword = dialogView.findViewById(R.id.et_password); Button btnLogin = dialogView.findViewById(R.id.btn_login); Button btnCancel = dialogView.findViewById(R.id.btnCancel); // 设置点击事件 btnLogin.setOnClickListener(v -> { // 处理登录逻辑 }); btnCancel.setOnClickListener(v -> dialog.dismiss()); // 创建并显示对话框 AlertDialog dialog = builder.create(); dialog.show(); ``` ### 3. iOS中的自定义对话框 在iOS开发中,我们通常使用`UIAlertController`来创建自定义对话框。创建一个Storyboard或Xib文件,包含所需的输入字段和按钮。然后,通过代码加载这个视图,并将其添加到`UIAlertController`中: ```swift let storyboard = UIStoryboard(name: "CustomDialog", bundle: .main) guard let dialogViewController = storyboard.instantiateInitialViewController() as? CustomDialogViewController else { return } let alertController = UIAlertController(title: "登录", message: nil, preferredStyle: .alert) alertController.setValue(dialogViewController, forKey: "contentViewController") // 添加按钮并设置点击事件 let loginAction = UIAlertAction(title: "登录", style: .default) { _ in // 处理登录逻辑 } let cancelAction = UIAlertAction(title: "取消", style: .cancel) { _ in self.dismiss(animated: true, completion: nil) } alertController.addAction(loginAction) alertController.addAction(cancelAction) present(alertController, animated: true, completion: nil) ``` ### 4. 设计原则与最佳实践 - 对话框应简洁明了,避免过多的元素导致用户困惑。 - 提供清晰的关闭或取消选项,以遵循用户期望。 - 使用一致的设计语言,保持与应用其他部分的一致性。 - 避免滥用对话框,它们可能会打断用户的操作流程。 自定义对话框是一个展示应用个性和提升用户体验的重要手段。通过合理设计和实现,我们可以创建出既美观又实用的自定义登录对话框,为用户提供更好的互动体验。
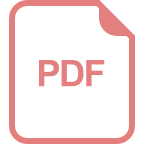
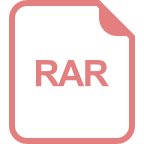
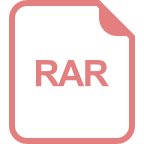
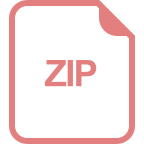
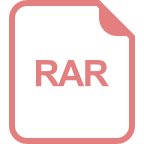



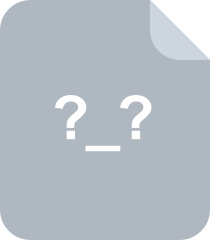



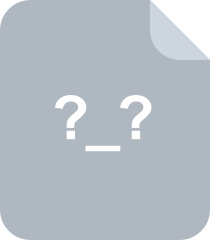
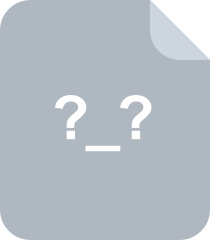
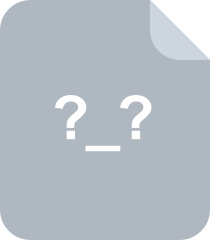
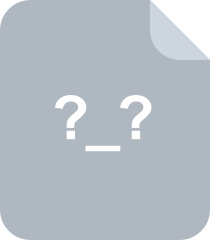
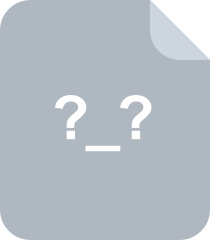
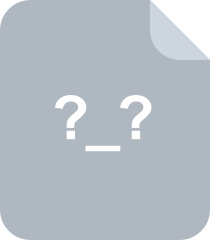
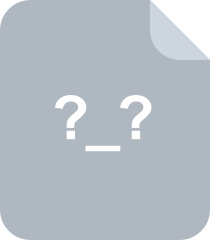
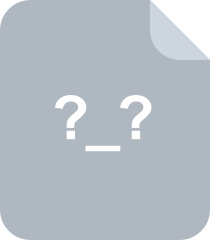
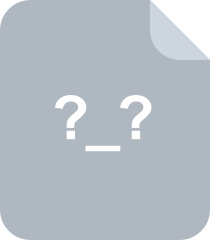
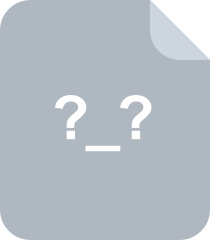


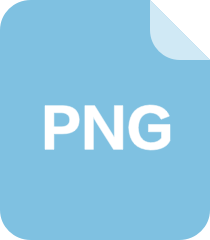

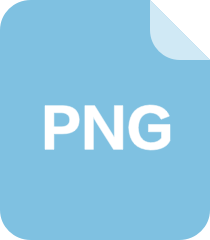

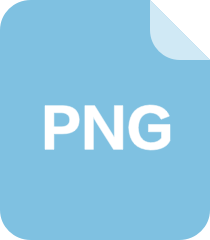
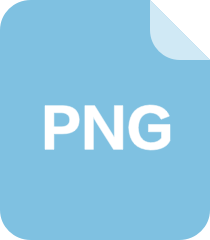
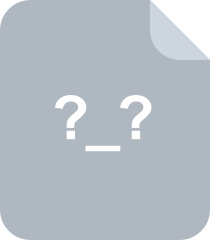
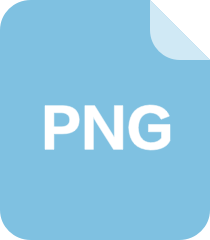
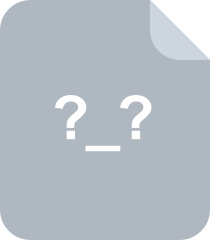
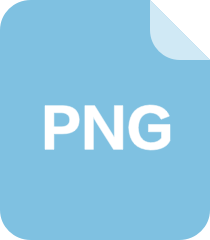
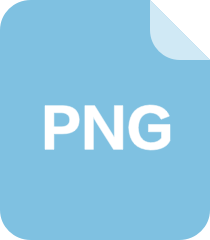

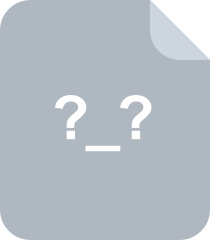
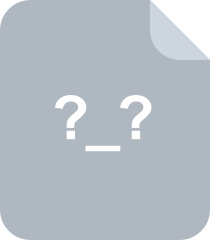

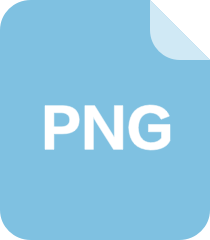

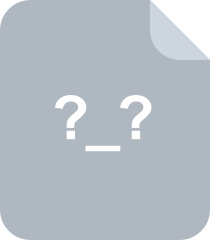
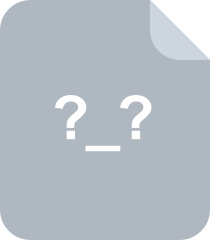

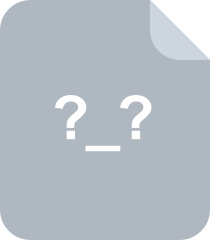




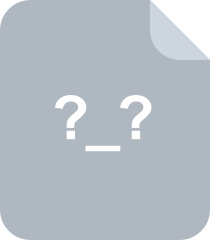




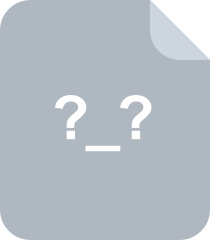
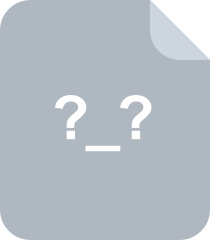
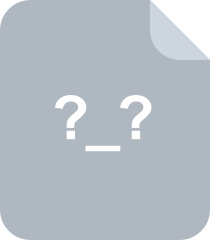
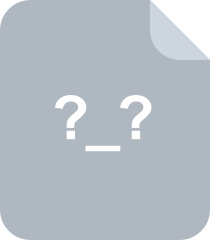
- 1
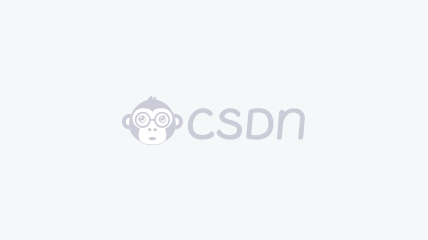

- 粉丝: 0
- 资源: 5
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

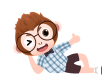
最新资源
- python-leetcode python题解之第482题密钥格式化
- python-leetcode python题解之第479题最大回文数乘积
- python-leetcode python题解之第475题供暖器
- python-leetcode python题解之第463题岛屿的周长
- python-leetcode python题解之第461题汉明距离
- python-leetcode python题解之第458题可怜的小猪
- python-leetcode python题解之第457题环形数组是否存在循环
- python-leetcode python题解之第453题最小操作次数使数组元素相等
- python-leetcode python题解之第448题找到所有数组中消失的数字
- python-leetcode python题解之第443题压缩字符串

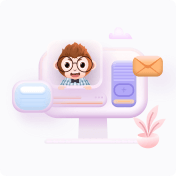
