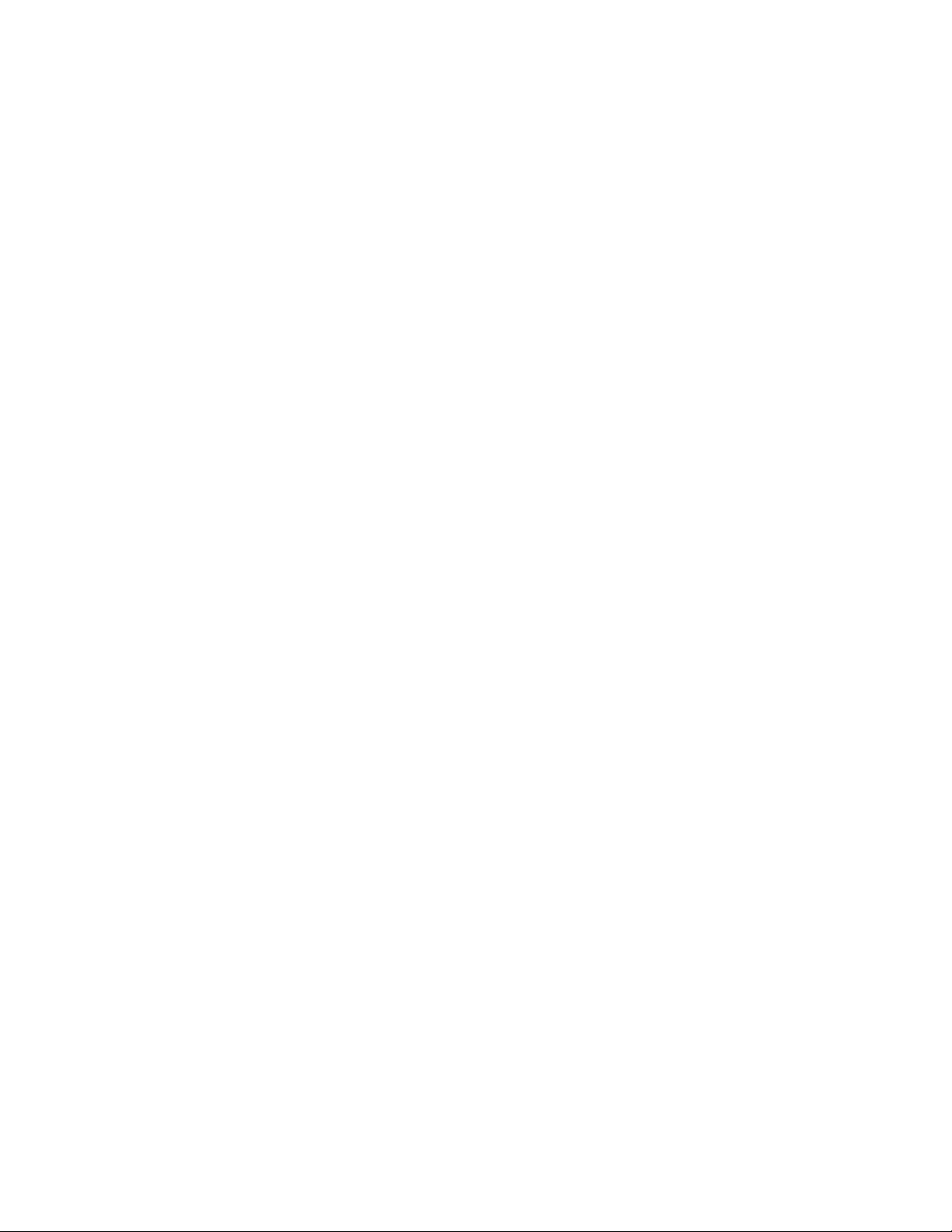
repeat ..................................................................................................... 59
Numerical for ........................................................................................... 60
Generic for .............................................................................................. 60
break, return, and goto ..................................................................................... 61
II. Real Programming ......................................................................................................... 65
9. Closures ............................................................................................................... 68
Functions as First-Class Values ............................................................................ 68
Non-Global Functions ........................................................................................ 69
Lexical Scoping ................................................................................................ 71
A Taste of Functional Programming ..................................................................... 74
10. Pattern Matching .................................................................................................. 77
The Pattern-Matching Functions ........................................................................... 77
The function string.find ...................................................................... 77
The function string.match .................................................................... 77
The function string.gsub ...................................................................... 78
The function string.gmatch .................................................................. 78
Patterns ............................................................................................................ 78
Captures ........................................................................................................... 82
Replacements .................................................................................................... 83
URL encoding .......................................................................................... 84
Tab expansion ........................................................................................... 86
Tricks of the Trade ............................................................................................ 86
11. Interlude: Most Frequent Words ............................................................................. 90
12. Date and Time ..................................................................................................... 92
The Function os.time ..................................................................................... 92
The Function os.date ..................................................................................... 93
Date–Time Manipulation .................................................................................... 95
13. Bits and Bytes ..................................................................................................... 97
Bitwise Operators .............................................................................................. 97
Unsigned Integers .............................................................................................. 97
Packing and Unpacking Binary Data ..................................................................... 99
Binary files ..................................................................................................... 101
14. Data Structures ................................................................................................... 104
Arrays ............................................................................................................ 104
Matrices and Multi-Dimensional Arrays ............................................................... 105
Linked Lists .................................................................................................... 107
Queues and Double-Ended Queues ..................................................................... 107
Reverse Tables ................................................................................................ 108
Sets and Bags ................................................................................................. 109
String Buffers .................................................................................................. 110
Graphs ........................................................................................................... 111
15. Data Files and Serialization .................................................................................. 114
Data Files ....................................................................................................... 114
Serialization .................................................................................................... 116
Saving tables without cycles ...................................................................... 118
Saving tables with cycles .......................................................................... 119
16. Compilation, Execution, and Errors ....................................................................... 122
Compilation .................................................................................................... 122
Precompiled Code ............................................................................................ 125
Errors ............................................................................................................. 126
Error Handling and Exceptions .......................................................................... 127
Error Messages and Tracebacks .......................................................................... 128
17. Modules and Packages ........................................................................................ 131
The Function require .................................................................................... 132