package com.anbyke.entity;
import java.util.Date;
/**
* KeUsers entity.
*
* @author MyEclipse Persistence Tools
*/
public class KeUsers implements java.io.Serializable {
// Fields
private Integer id;
private String userName;
private String password;
private String realName;
private Integer sex;
private String mobilePhone;
private String companyName;
private String companyPhone;
private String companyFax;
private String companyEmail;
private String companyAddress;
private String category;
private Integer status;
private Integer state;
private Integer trial;
private Date trialEndtime;
private Date registerTime;
private Date expireTime;
// Constructors
/** default constructor */
public KeUsers() {
}
/** minimal constructor */
public KeUsers(String userName, String password, String realName) {
this.userName = userName;
this.password = password;
this.realName = realName;
}
/** full constructor */
public KeUsers(String userName, String password, String realName, Integer sex, String mobilePhone, String companyName, String companyPhone, String companyFax, String companyEmail, String companyAddress, String category, Integer status, Integer state, Integer trial, Date trialEndtime, Date registerTime, Date expireTime) {
this.userName = userName;
this.password = password;
this.realName = realName;
this.sex = sex;
this.mobilePhone = mobilePhone;
this.companyName = companyName;
this.companyPhone = companyPhone;
this.companyFax = companyFax;
this.companyEmail = companyEmail;
this.companyAddress = companyAddress;
this.category = category;
this.status = status;
this.state = state;
this.trial = trial;
this.trialEndtime = trialEndtime;
this.registerTime = registerTime;
this.expireTime = expireTime;
}
// Property accessors
public Integer getId() {
return this.id;
}
public void setId(Integer id) {
this.id = id;
}
public String getUserName() {
return this.userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public String getPassword() {
return this.password;
}
public void setPassword(String password) {
this.password = password;
}
public String getRealName() {
return this.realName;
}
public void setRealName(String realName) {
this.realName = realName;
}
public Integer getSex() {
return this.sex;
}
public void setSex(Integer sex) {
this.sex = sex;
}
public String getMobilePhone() {
return this.mobilePhone;
}
public void setMobilePhone(String mobilePhone) {
this.mobilePhone = mobilePhone;
}
public String getCompanyName() {
return this.companyName;
}
public void setCompanyName(String companyName) {
this.companyName = companyName;
}
public String getCompanyPhone() {
return this.companyPhone;
}
public void setCompanyPhone(String companyPhone) {
this.companyPhone = companyPhone;
}
public String getCompanyFax() {
return this.companyFax;
}
public void setCompanyFax(String companyFax) {
this.companyFax = companyFax;
}
public String getCompanyEmail() {
return this.companyEmail;
}
public void setCompanyEmail(String companyEmail) {
this.companyEmail = companyEmail;
}
public String getCompanyAddress() {
return this.companyAddress;
}
public void setCompanyAddress(String companyAddress) {
this.companyAddress = companyAddress;
}
public String getCategory() {
return this.category;
}
public void setCategory(String category) {
this.category = category;
}
public Integer getStatus() {
return this.status;
}
public void setStatus(Integer status) {
this.status = status;
}
public Integer getState() {
return this.state;
}
public void setState(Integer state) {
this.state = state;
}
public Integer getTrial() {
return this.trial;
}
public void setTrial(Integer trial) {
this.trial = trial;
}
public Date getTrialEndtime() {
return this.trialEndtime;
}
public void setTrialEndtime(Date trialEndtime) {
this.trialEndtime = trialEndtime;
}
public Date getRegisterTime() {
return this.registerTime;
}
public void setRegisterTime(Date registerTime) {
this.registerTime = registerTime;
}
public Date getExpireTime() {
return this.expireTime;
}
public void setExpireTime(Date expireTime) {
this.expireTime = expireTime;
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
SSH三层架构MVC,Hibernate(ehcache)二级缓存技术,源代码
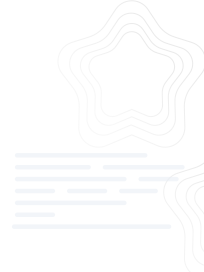
共72个文件
jar:39个
xml:8个
class:7个


温馨提示
SSH三层架构MVC(struts1.3+spring2.x+hibernate3.2),Hibernate(ehcache)二级缓存技术,Spring 注解形式依赖注入,ehcache缓存 源代码,内有MySql anbyke.sql文件,方便创建数据库演示效果!
资源推荐
资源详情
资源评论
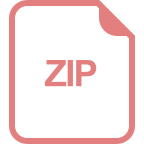
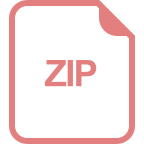
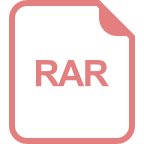
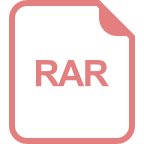
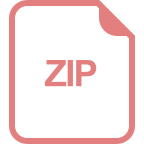
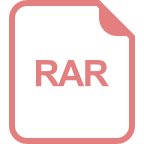
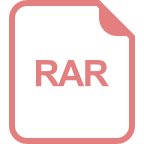
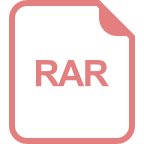
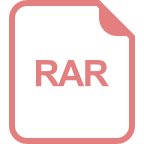
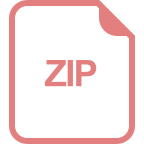
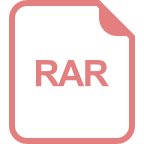
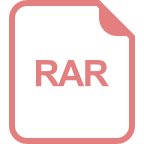
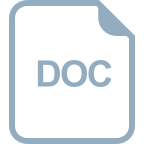
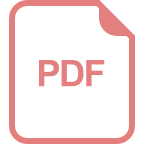
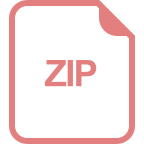
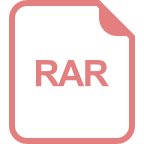
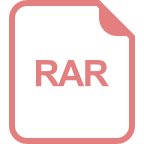
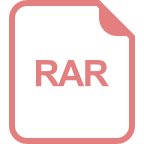
收起资源包目录






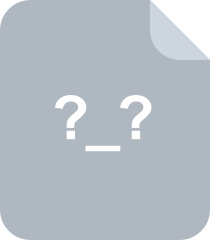
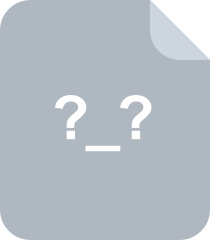


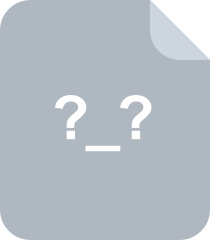
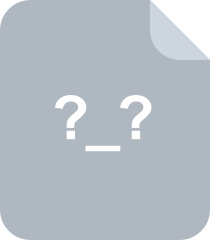


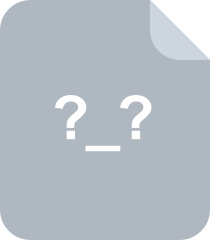
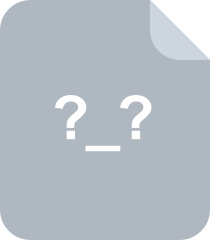


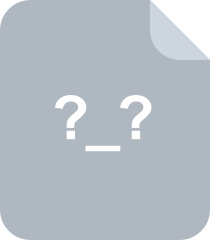

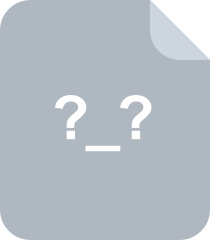
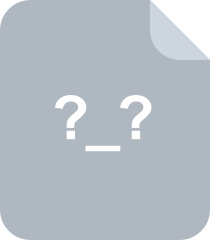
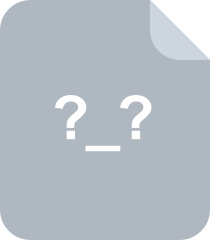
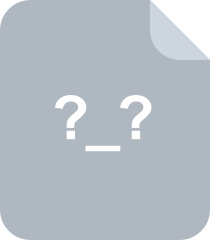
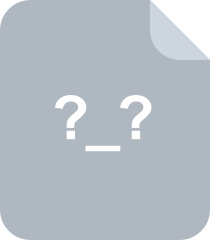
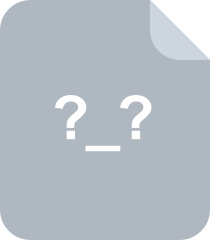

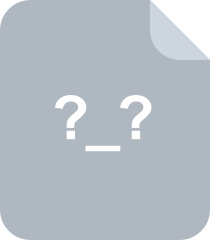

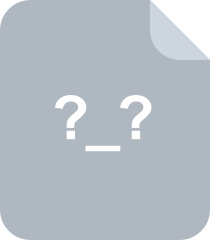
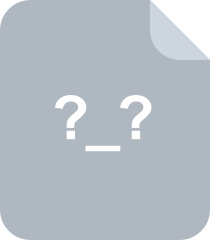


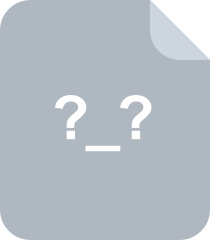
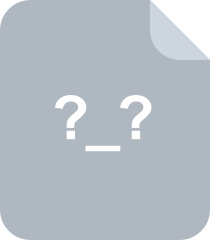





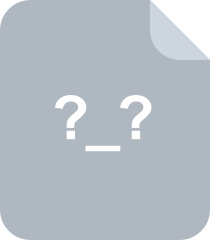
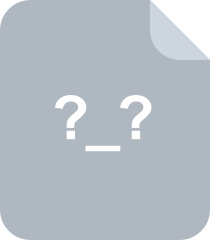

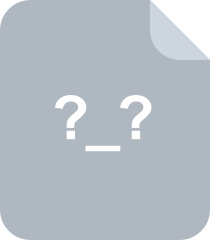

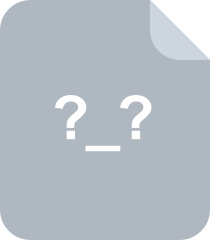

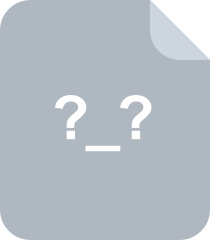

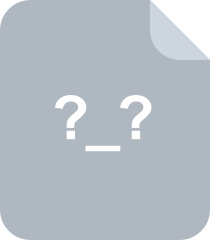


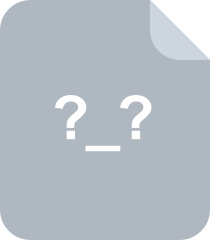

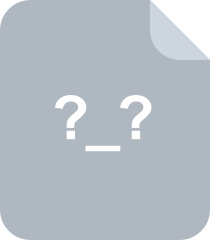
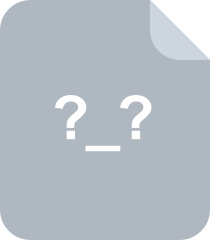
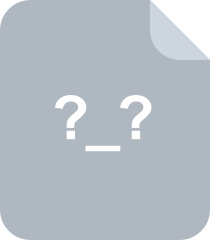
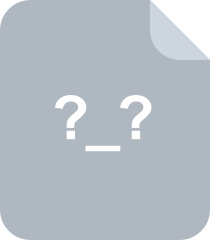
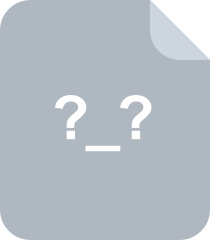
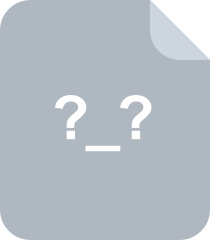

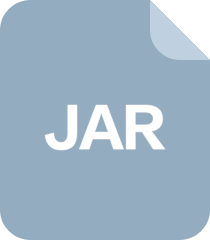
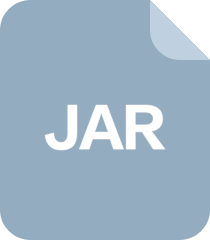
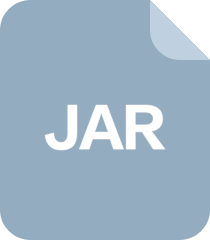
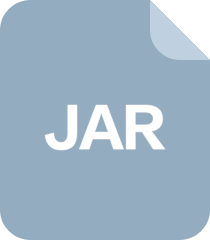
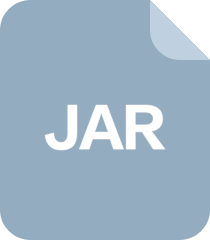
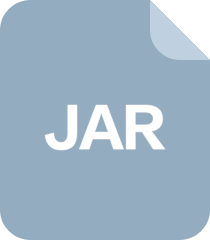
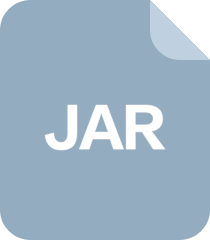
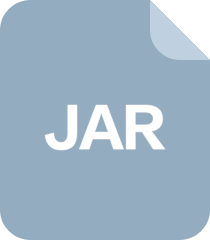
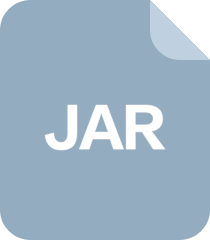
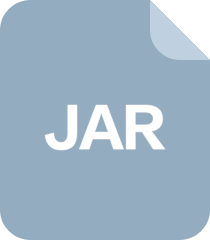
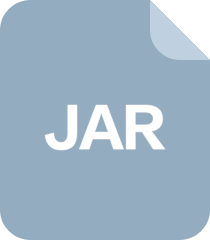
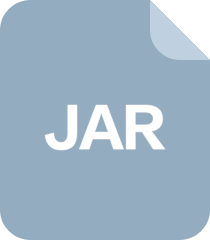
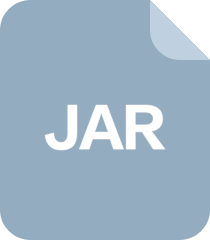
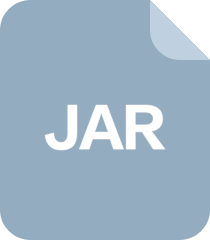
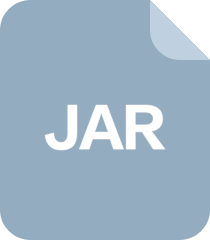
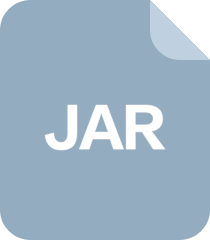
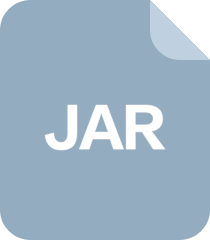
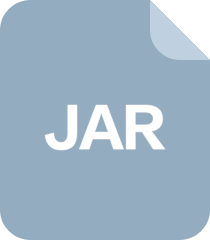
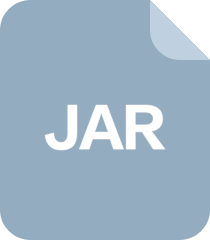
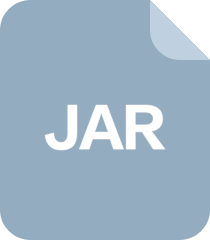
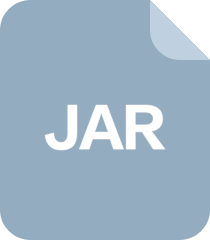
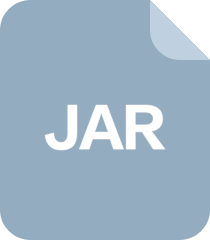
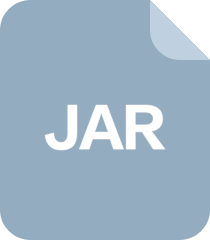
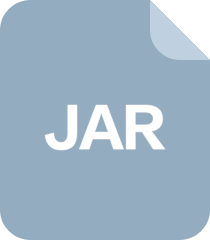
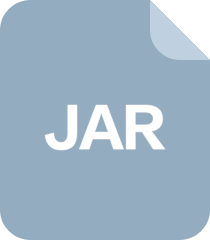
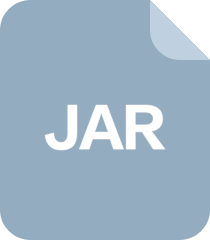
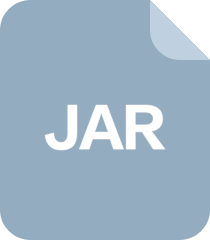
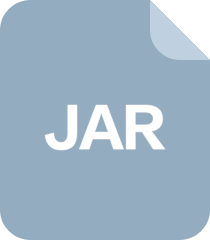
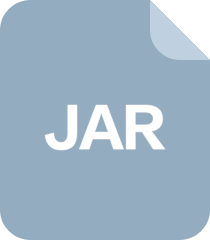
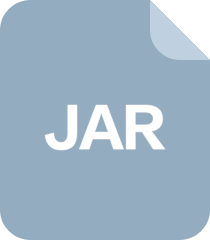
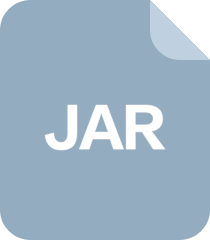
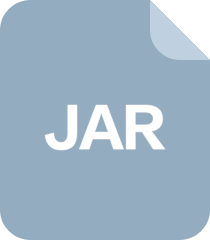
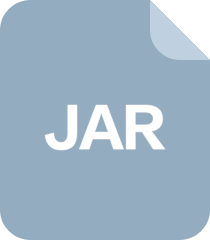
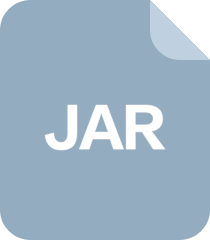
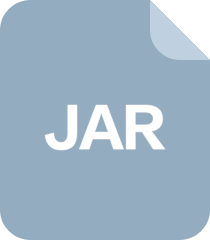
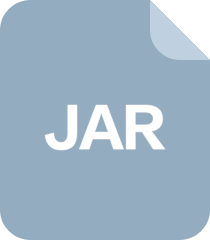
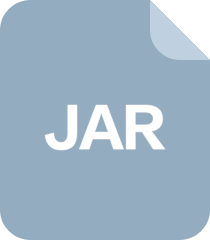
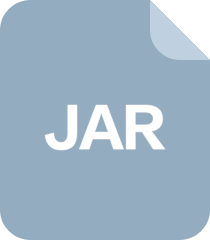
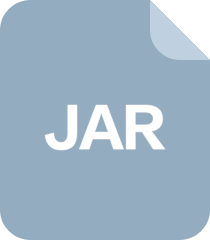
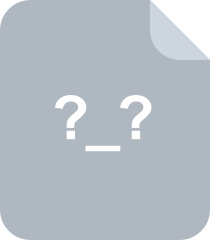
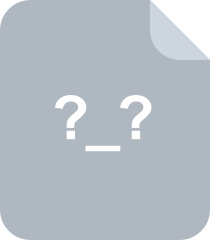
共 72 条
- 1

anbyke
- 粉丝: 3
- 资源: 8
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

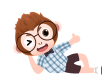
最新资源
- (源码)基于SimPy和贝叶斯优化的流程仿真系统.zip
- (源码)基于Java Web的个人信息管理系统.zip
- (源码)基于C++和OTL4的PostgreSQL数据库连接系统.zip
- (源码)基于ESP32和AWS IoT Core的室内温湿度监测系统.zip
- (源码)基于Arduino的I2C协议交通灯模拟系统.zip
- coco.names 文件
- (源码)基于Spring Boot和Vue的房屋租赁管理系统.zip
- (源码)基于Android的饭店点菜系统.zip
- (源码)基于Android平台的权限管理系统.zip
- (源码)基于CC++和wxWidgets框架的LEGO模型火车控制系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


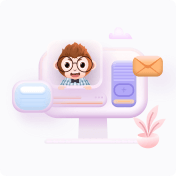
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
前往页