Objective-C 开发入门

### Objective-C 开发入门知识点详解 #### 一、Objective-C简介 Objective-C是一种简单而强大的计算机语言,旨在支持复杂的面向对象编程。它扩展了标准的ANSI C语言,增加了定义类和方法的语法,以及一系列促进类动态扩展的构造。 #### 二、Objective-C与C的关系 **Objective-C是C的超集**: - Objective-C兼容ANSI C的基本语法。 - 和C代码一样,Objective-C中也使用头文件和源文件来分离公共声明和代码实现细节。 - **头文件扩展名**:通常使用`.h`作为Objective-C头文件的扩展名,这些文件包含类、类型、函数和常量的声明。 - **源文件扩展名**: - `.m`:典型的Objective-C源文件扩展名,可以同时包含Objective-C和C代码。 - `.mm`:这种扩展名用于同时包含Objective-C/C和C++代码的文件,仅在实际引用C++类或特性时使用。 #### 三、面向对象概念 如果之前有面向对象语言编程经验,以下内容将有助于理解Objective-C的基本语法: - **封装**:封装是面向对象编程的核心原则之一,它通过隐藏内部状态和行为来保护数据的安全性。 - **继承**:继承允许创建一个新类(子类)从现有类(父类)继承属性和方法,从而减少代码重复并提高代码复用性。 - **多态**:多态性允许使用父类的引用来指向子类的对象,并调用子类的方法,从而提供接口一致性和灵活性。 #### 四、初学者注意事项 如果你从未使用过面向对象的语言,建议先了解以下基本概念: - **对象**:对象是类的实例,具有特定的属性和方法。 - **设计模式**:设计模式是解决特定问题的模板,Objective-C中的设计模式如单例模式、工厂模式等对于理解和构建Cocoa应用至关重要。 #### 五、资源推荐 - **《面向对象编程与Objective-C》**:本书提供了一个面向对象编程概念的概述,非常适合初学者。 - **《Cocoa基础指南》**:这本书介绍了Cocoa框架中使用的各种设计模式,对理解Cocoa应用的设计至关重要。 - **《Objective-C编程语言》**:该文档提供了Objective-C语言及其语法的全面介绍,是深入学习的最佳资源之一。 #### 六、实战练习建议 为了更好地掌握Objective-C,建议进行实践练习: - **编写简单的命令行程序**:例如计算器或地址簿应用,帮助理解类、对象、属性和方法的基本使用。 - **阅读开源项目代码**:GitHub上有大量的Objective-C项目,阅读这些项目的源代码可以帮助理解更高级的编程技巧和最佳实践。 - **参与社区讨论**:加入Stack Overflow等社区,参与讨论和技术交流,能够及时获得反馈和解答疑问。 #### 七、小结 Objective-C是一种功能强大且易于学习的面向对象语言,它是iOS开发的基础。通过理解面向对象的核心概念、熟悉Objective-C的语法特点以及不断实践,可以快速提高编程技能。希望以上内容能为初学者提供一定的指导和帮助。
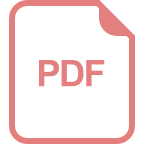
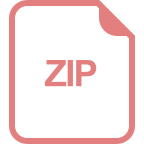
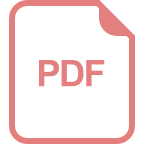
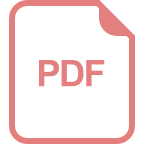
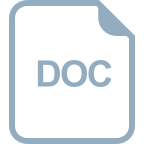
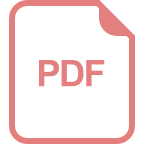
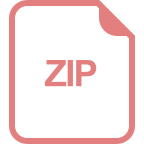
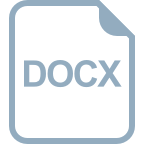
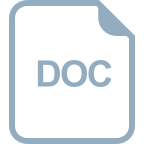
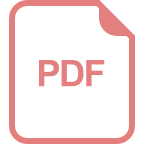
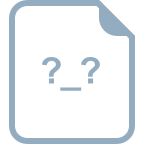
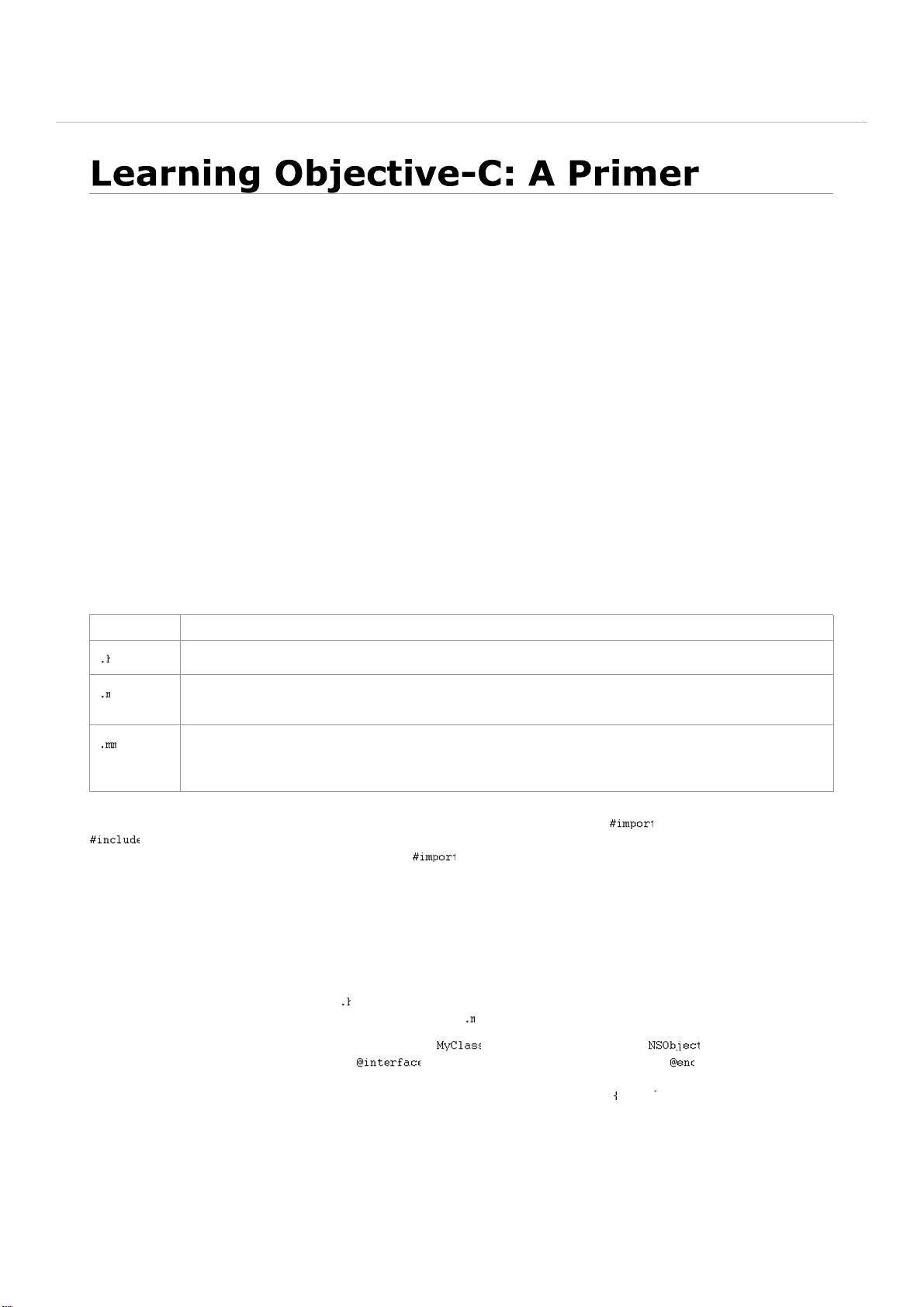
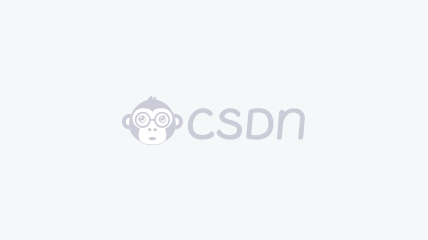
- 天真无邪12013-01-14看起来费劲了点 不过是好书!
- vin2382014-05-10內容不會太多,初學者(需要有其他OO編程經驗)比較容易看的完
- pody2012-04-20全英文版的,英语一般,得慢慢看
- fengpiaoxianle2012-04-03全英文版的,英语一般,得慢慢看

- 粉丝: 0
- 资源: 12
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

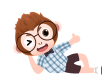
最新资源
- 一种电池极耳贴双面胶设备sw18可编辑全套技术资料100%好用.zip
- matlab实现智能优化算法-Kmean-Transformer-BiLSTM负荷预测-电力负荷预测-智能优化算法-BiLSTM-matlab
- 基于ssm的电影购票系统源码(java毕业设计完整源码+LW).zip
- PCB电路板缺陷检测识别数据集,使用COCO JSON格式标注 99.8的识别准确率,1297张图片
- PCB电路板缺陷检测识别数据集,使用YOLOv5格式标注 99.8的识别准确率,1297张图片
- PCB电路板缺陷检测识别数据集,使用PASICAL VOC XML格式标注 99.8的识别准确率,1297张图片
- 不同颜色机器人检测55-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- PCB电路板缺陷检测识别数据集,使用YOLOv7格式标注 99.8的识别准确率,1297张图片
- PCB电路板缺陷检测识别数据集,使用yolov8格式标注 99.8的识别准确率,1297张图片
- PCB电路板缺陷检测识别数据集,使用yolov9格式标注 99.8的识别准确率,1297张图片
- matlab实现智能优化算法-K-means-Transformer-BiLSTM组合状态识别分类算法研究-智能优化算法-状态识别-matlab
- 基于ssm的在线商品管理系统源码(java毕业设计完整源码+LW).zip
- PCB电路板缺陷检测识别数据集,使用yolov11格式标注 99.8的识别准确率,1297张图片
- Java项目资源:智能图书管理系统
- 基于ssm的在线图书管理源码(java毕业设计完整源码+LW).zip
- 基于Javaweb的物流管理系统源码(java毕业设计完整源码+LW).zip

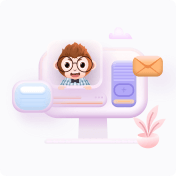
