/* =========================================================
* Bootstrap year calendar v1.1.0
* Repo: https://github.com/Paul-DS/bootstrap-year-calendar
* =========================================================
* Created by Paul David-Sivelle
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ========================================================= */
(function($) {
var Calendar = function(element, options) {
this.element = element;
this.element.addClass('calendar');
this._initializeEvents(options);
this._initializeOptions(options);
this._render();
};
Calendar.prototype = {
constructor: Calendar,
_initializeOptions: function(opt) {
if(opt == null) {
opt = [];
}
this.options = {
startYear: !isNaN(parseInt(opt.startYear)) ? parseInt(opt.startYear) : new Date().getFullYear(),
minDate: opt.minDate instanceof Date ? opt.minDate : null,
maxDate: opt.maxDate instanceof Date ? opt.maxDate : null,
language: (opt.language != null && dates[opt.language] != null) ? opt.language : 'en',
allowOverlap: opt.allowOverlap != null ? opt.allowOverlap : true,
displayWeekNumber: opt.displayWeekNumber != null ? opt.displayWeekNumber : false,
alwaysHalfDay: opt.alwaysHalfDay != null ? opt.alwaysHalfDay : false,
enableRangeSelection: opt.enableRangeSelection != null ? opt.enableRangeSelection : false,
disabledDays: opt.disabledDays instanceof Array ? opt.disabledDays : [],
roundRangeLimits: opt.roundRangeLimits != null ? opt.roundRangeLimits : false,
dataSource: opt.dataSource instanceof Array != null ? opt.dataSource : [],
style: opt.style == 'background' || opt.style == 'border' || opt.style == 'custom' ? opt.style : 'border',
enableContextMenu: opt.enableContextMenu != null ? opt.enableContextMenu : false,
contextMenuItems: opt.contextMenuItems instanceof Array ? opt.contextMenuItems : [],
customDayRenderer : $.isFunction(opt.customDayRenderer) ? opt.customDayRenderer : null,
customDataSourceRenderer : $.isFunction(opt.customDataSourceRenderer) ? opt.customDataSourceRenderer : null
};
this._initializeDatasourceColors();
},
_initializeEvents: function(opt) {
if(opt == null) {
opt = [];
}
if(opt.renderEnd) { this.element.bind('renderEnd', opt.renderEnd); }
if(opt.clickDay) { this.element.bind('clickDay', opt.clickDay); }
if(opt.dayContextMenu) { this.element.bind('dayContextMenu', opt.dayContextMenu); }
if(opt.selectRange) { this.element.bind('selectRange', opt.selectRange); }
if(opt.mouseOnDay) { this.element.bind('mouseOnDay', opt.mouseOnDay); }
if(opt.mouseOutDay) { this.element.bind('mouseOutDay', opt.mouseOutDay); }
},
_initializeDatasourceColors: function() {
for(var i in this.options.dataSource) {
if(this.options.dataSource[i].color == null) {
this.options.dataSource[i].color = colors[i % colors.length];
}
}
},
_render: function() {
this.element.empty();
this._renderHeader();
this._renderBody();
this._renderDataSource();
this._applyEvents();
this.element.find('.months-container').fadeIn(500);
this._triggerEvent('renderEnd', { currentYear: this.options.startYear });
},
_renderHeader: function() {
var header = $(document.createElement('div'));
header.addClass('calendar-header panel panel-default');
var headerTable = $(document.createElement('table'));
var prevDiv = $(document.createElement('th'));
prevDiv.addClass('prev');
if(this.options.minDate != null && this.options.minDate > new Date(this.options.startYear - 1, 11, 31)) {
prevDiv.addClass('disabled');
}
var prevIcon = $(document.createElement('span'));
prevIcon.addClass('glyphicon glyphicon-chevron-left');
prevDiv.append(prevIcon);
headerTable.append(prevDiv);
var prev2YearDiv = $(document.createElement('th'));
prev2YearDiv.addClass('year-title year-neighbor2 hidden-sm hidden-xs');
prev2YearDiv.text(this.options.startYear - 2);
if(this.options.minDate != null && this.options.minDate > new Date(this.options.startYear - 2, 11, 31)) {
prev2YearDiv.addClass('disabled');
}
headerTable.append(prev2YearDiv);
var prevYearDiv = $(document.createElement('th'));
prevYearDiv.addClass('year-title year-neighbor hidden-xs');
prevYearDiv.text(this.options.startYear - 1);
if(this.options.minDate != null && this.options.minDate > new Date(this.options.startYear - 1, 11, 31)) {
prevYearDiv.addClass('disabled');
}
headerTable.append(prevYearDiv);
var yearDiv = $(document.createElement('th'));
yearDiv.addClass('year-title');
yearDiv.text(this.options.startYear);
headerTable.append(yearDiv);
var nextYearDiv = $(document.createElement('th'));
nextYearDiv.addClass('year-title year-neighbor hidden-xs');
nextYearDiv.text(this.options.startYear + 1);
if(this.options.maxDate != null && this.options.maxDate < new Date(this.options.startYear + 1, 0, 1)) {
nextYearDiv.addClass('disabled');
}
headerTable.append(nextYearDiv);
var next2YearDiv = $(document.createElement('th'));
next2YearDiv.addClass('year-title year-neighbor2 hidden-sm hidden-xs');
next2YearDiv.text(this.options.startYear + 2);
if(this.options.maxDate != null && this.options.maxDate < new Date(this.options.startYear + 2, 0, 1)) {
next2YearDiv.addClass('disabled');
}
headerTable.append(next2YearDiv);
var nextDiv = $(document.createElement('th'));
nextDiv.addClass('next');
if(this.options.maxDate != null && this.options.maxDate < new Date(this.options.startYear + 1, 0, 1)) {
nextDiv.addClass('disabled');
}
var nextIcon = $(document.createElement('span'));
nextIcon.addClass('glyphicon glyphicon-chevron-right');
nextDiv.append(nextIcon);
headerTable.append(nextDiv);
header.append(headerTable);
this.element.append(header);
},
_renderBody: function() {
var monthsDiv = $(document.createElement('div'));
monthsDiv.addClass('months-container');
for(var m = 0; m < 12; m++) {
/* Container */
var monthDiv = $(document.createElement('div'));
monthDiv.addClass('month-container');
monthDiv.data('month-id', m);
var firstDate = new Date(this.options.startYear, m, 1);
var table = $(document.createElement('table'));
table.addClass('month');
/* Month header */
var thead = $(document.createElement('thead'));
var titleRow = $(document.createElement('tr'));
var titleCell = $(document.createElement('th'));
titleCell.addClass('month-title');
titleCell.attr('colspan', this.options.displayWeekNumber ? 8 : 7);
titleCell.text(dates[this.options.language].months[m]);
titleRow.append(titleCell);
thead.append(titleRow);
var headerRow = $(document.createElement('tr'));
if(this.options.displayWeekNumber) {
var weekNumberCell = $(document.createElement('th'));
weekNumberCell.addClass('week-number');
weekNumberCell.text(dates[this.options.language].weekShort);
headerRow.append(weekNumberCell);
}
var d = dates[this.options.language].weekStart;
do
{
var headerCell = $(document.createElement('th'));
headerCell.addClass('day-header');
headerCell.text(dates[this.options.language].daysMin[d]);
headerRow.append(headerCell);
d++;
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
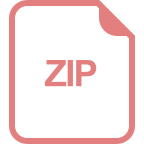
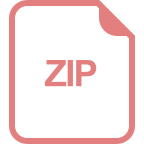
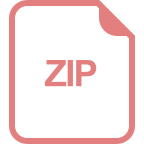
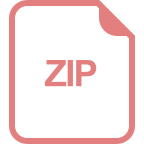
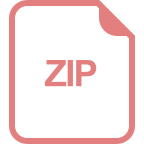
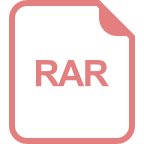
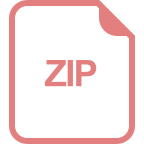
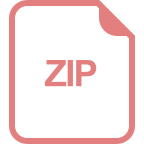
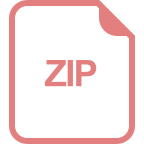
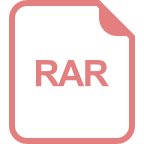
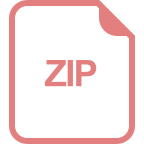
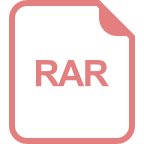
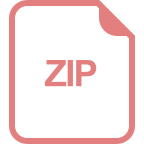
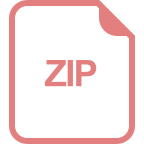
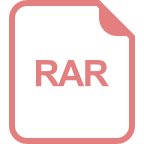
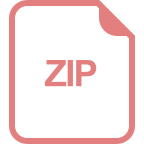
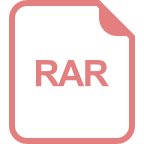
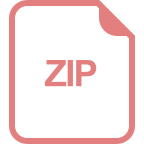
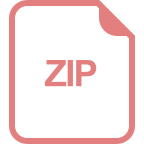
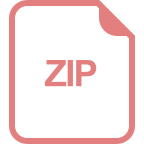
收起资源包目录


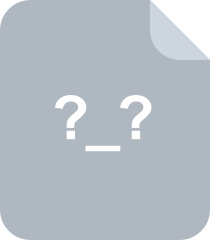
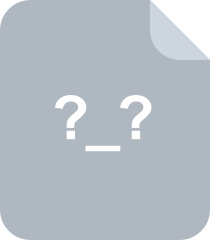
共 2 条
- 1
资源评论
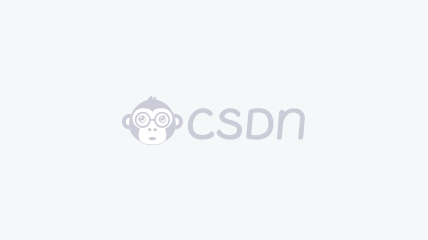

走啊走走到九月九
- 粉丝: 0
- 资源: 5
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

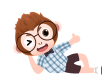
最新资源
- (源码)基于Arduino和Python的实时歌曲信息液晶显示屏展示系统.zip
- (源码)基于C++和C混合模式的操作系统开发项目.zip
- (源码)基于Arduino的全球天气监控系统.zip
- OpenCVForUnity2.6.0.unitypackage
- (源码)基于SimPy和贝叶斯优化的流程仿真系统.zip
- (源码)基于Java Web的个人信息管理系统.zip
- (源码)基于C++和OTL4的PostgreSQL数据库连接系统.zip
- (源码)基于ESP32和AWS IoT Core的室内温湿度监测系统.zip
- (源码)基于Arduino的I2C协议交通灯模拟系统.zip
- coco.names 文件
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


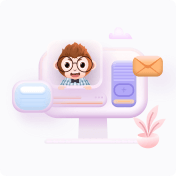
安全验证
文档复制为VIP权益,开通VIP直接复制
