### C语言库函数详解 #### 1. 函数名: abort **功能**: 异常终止一个进程。 **用法**: `void abort(void);` **程序示例**: ```c #include <stdio.h> #include <stdlib.h> int main(void) { printf("Calling abort()\n"); abort(); // 终止当前进程 return 0; // 此行代码永远不会被执行 } ``` **解释**: `abort` 函数用于立即终止当前进程,并且不执行任何清理操作(如关闭打开的文件)。通常用于处理不可恢复的错误情况。 #### 2. 函数名: abs **功能**: 求整数的绝对值。 **用法**: `int abs(int i);` **程序示例**: ```c #include <stdio.h> #include <stdlib.h> int main(void) { int number = -1234; printf("number: %d absolute value: %d\n", number, abs(number)); return 0; } ``` **解释**: `abs` 函数可以获取整数参数的绝对值。如果传入的参数是负数,则返回它的相反数;如果是正数或零,则返回它本身。 #### 3. 函数名: absread, abswrite **功能**: 在指定磁盘驱动器上进行绝对磁盘扇区的读取与写入操作。 **用法**: - `int absread(int drive, int nsects, int sectno, void *buffer);` - `int abswrite(int drive, int nsects, int sectno, void *buffer);` **程序示例**: ```c #include <conio.h> #include <dos.h> #include <stdio.h> #include <stdlib.h> int main(void) { int i, strt, ch_out, sector; char buf[512]; printf("Insert a diskette into drive A and press any key\n"); getch(); // 等待用户按键 sector = 0; if (absread(0, 1, sector, &buf) != 0) { perror("Disk problem"); // 输出错误信息 exit(1); // 异常退出 } printf("Read OK\n"); strt = 3; for (i = 0; i < 80; i++) { ch_out = buf[strt + i]; putchar(ch_out); // 输出读取的数据 } printf("\n"); return(0); } ``` **解释**: `absread` 和 `abswrite` 是用于直接操作磁盘扇区的函数,它们允许程序直接读写磁盘上的特定位置,这对于低级的磁盘操作非常有用。 #### 4. 函数名: access **功能**: 确定文件是否存在以及是否可以被访问。 **用法**: `int access(const char *filename, int amode);` **程序示例**: ```c #include <stdio.h> #include <unistd.h> int file_exists(char *filename); int main(void) { printf("Does NOTEXIST.FIL exist: %s\n", file_exists("NOTEXISTS.FIL") ? "YES" : "NO"); return 0; } int file_exists(char *filename) { return (access(filename, 0) == 0); } ``` **解释**: `access` 函数用于检查文件是否可以被访问。函数接受两个参数:文件名和模式(amode)。在本例中,模式设置为0表示只检查文件是否存在。 #### 5. 函数名: acos **功能**: 计算反余弦函数。 **用法**: `double acos(double x);` **程序示例**: ```c #include <math.h> #include <stdio.h> int main(void) { double result; double x = 0.5; result = acos(x); printf("The arccosine of %lf is %lf\n", x, result); return 0; } ``` **解释**: `acos` 函数计算给定数值的反余弦值,即返回介于0到π之间的角度θ,使得cos(θ)等于给定的数值。 #### 6. 函数名: allocmem **功能**: 分配DOS存储段。 **用法**: `int allocmem(unsigned size, unsigned *seg);` **程序示例**: ```c #include <dos.h> #include <stdio.h> #include <stdlib.h> int main(void) { unsigned int size, segp; int stat; size = 64; /* (64x16)=1024 bytes */ stat = allocmem(size, &segp); if (stat == -1) printf("Allocated memory at segment: %x\n", segp); else printf("Failed: maximum number of paragraphs available is %u\n", stat); return 0; } ``` **解释**: `allocmem` 函数用于分配DOS存储段。这个函数主要用于旧版本的DOS系统,在现代操作系统中很少使用。 #### 7. 函数名: arc **功能**: 画一条弧线。 **用法**: `void far arc(int x, int y, int stangle, int endangle, int radius);` **程序示例**: ```c #include <graphics.h> #include <stdlib.h> #include <stdio.h> int main(void) { int gdriver = DETECT, gmode, errorcode; int midx, midy; int stangle = 45, endangle = 135; int radius = 100; initgraph(&gdriver, &gmode, ""); // 初始化图形环境 // 继续完成图形绘制... return 0; } ``` **解释**: `arc` 函数用于在图形环境中绘制弧线。通常需要与 `graphics.h` 头文件一起使用,并且需要初始化图形环境才能正常工作。该函数接受五个参数:弧线中心的坐标(x, y),起始角度(stangle),结束角度(endangle)和半径(radius)。 以上是对给定文档中的部分C语言库函数的详细介绍,这些函数覆盖了从文件操作到数学计算等多个方面,对于C语言编程具有重要意义。
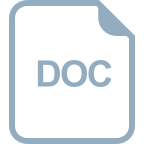
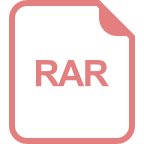
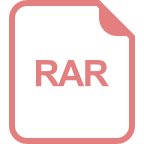
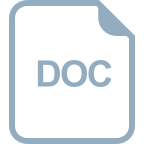
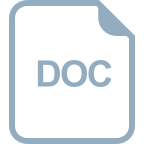
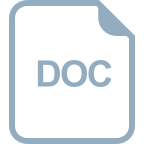
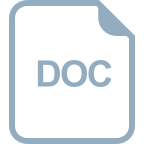
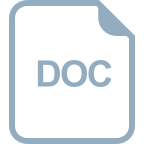
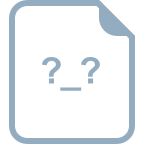
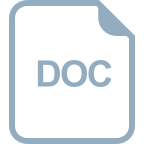
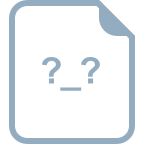
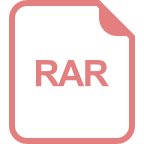
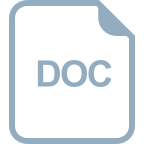
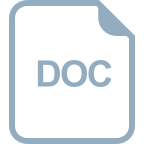
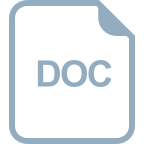
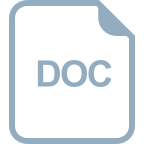
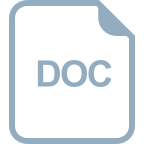
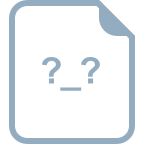
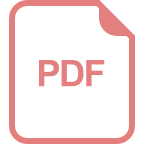
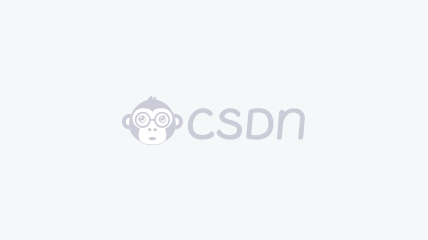

- 粉丝: 18
- 资源: 76
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

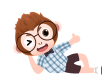
最新资源

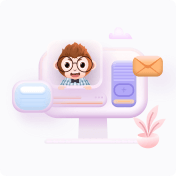
