package com.yuanlrc.campus_market.controller.home;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import com.yuanlrc.campus_market.bean.CodeMsg;
import com.yuanlrc.campus_market.bean.Result;
import com.yuanlrc.campus_market.constant.SessionConstant;
import com.yuanlrc.campus_market.entity.common.Comment;
import com.yuanlrc.campus_market.entity.common.Goods;
import com.yuanlrc.campus_market.entity.common.ReportGoods;
import com.yuanlrc.campus_market.entity.common.Student;
import com.yuanlrc.campus_market.entity.common.WantedGoods;
import com.yuanlrc.campus_market.service.common.CommentService;
import com.yuanlrc.campus_market.service.common.GoodsCategoryService;
import com.yuanlrc.campus_market.service.common.GoodsService;
import com.yuanlrc.campus_market.service.common.ReportGoodsService;
import com.yuanlrc.campus_market.service.common.StudentService;
import com.yuanlrc.campus_market.service.common.WantedGoodsService;
import com.yuanlrc.campus_market.util.SessionUtil;
import com.yuanlrc.campus_market.util.ValidateEntityUtil;
/**
* 学生中心控制器
* @author Administrator
*
*/
@RequestMapping("/home/student")
@Controller
public class HomeStudentController {
@Autowired
private GoodsCategoryService goodsCategoryService;
@Autowired
private StudentService studentService;
@Autowired
private GoodsService goodsService;
@Autowired
private WantedGoodsService wantedGoodsService;
@Autowired
private ReportGoodsService reportGoodsService;
@Autowired
private CommentService commentService;
/**
* 学生登录主页
* @param model
* @return
*/
@RequestMapping(value="/index",method=RequestMethod.GET)
public String index(Model model){
Student loginedStudent = (Student)SessionUtil.get(SessionConstant.SESSION_STUDENT_LOGIN_KEY);
model.addAttribute("goodsList", goodsService.findByStudent(loginedStudent));
model.addAttribute("wantedGoodsList", wantedGoodsService.findByStudent(loginedStudent));
model.addAttribute("reportGoodsList", reportGoodsService.findByStudent(loginedStudent));
return "home/student/index";
}
/**
* 修改个人信息提交表单
* @param student
* @return
*/
@RequestMapping(value="/edit_info",method=RequestMethod.POST)
@ResponseBody
public Result<Boolean> editInfo(Student student){
Student loginedStudent = (Student)SessionUtil.get(SessionConstant.SESSION_STUDENT_LOGIN_KEY);
loginedStudent.setAcademy(student.getAcademy());
loginedStudent.setGrade(student.getGrade());
loginedStudent.setMobile(student.getMobile());
loginedStudent.setNickname(student.getNickname());
loginedStudent.setQq(student.getQq());
loginedStudent.setSchool(student.getSchool());
if(studentService.save(loginedStudent) == null){
return Result.error(CodeMsg.HOME_STUDENT_EDITINFO_ERROR);
}
SessionUtil.set(SessionConstant.SESSION_STUDENT_LOGIN_KEY,loginedStudent);
return Result.success(true);
}
/**
* 保存用户头像
* @param headPic
* @return
*/
@RequestMapping(value="/update_head_pic",method=RequestMethod.POST)
@ResponseBody
public Result<Boolean> updateHeadPic(@RequestParam(name="headPic",required=true)String headPic){
Student loginedStudent = (Student)SessionUtil.get(SessionConstant.SESSION_STUDENT_LOGIN_KEY);
loginedStudent.setHeadPic(headPic);;
if(studentService.save(loginedStudent) == null){
return Result.error(CodeMsg.HOME_STUDENT_EDITINFO_ERROR);
}
SessionUtil.set(SessionConstant.SESSION_STUDENT_LOGIN_KEY,loginedStudent);
return Result.success(true);
}
/**
* 物品发布页面
* @param model
* @return
*/
@RequestMapping(value="/publish",method=RequestMethod.GET)
public String publish(Model model){
return "home/student/publish";
}
/**
* 商品发布表单提交
* @param goods
* @return
*/
@RequestMapping(value="/publish",method=RequestMethod.POST)
@ResponseBody
public Result<Boolean> publish(Goods goods){
CodeMsg validate = ValidateEntityUtil.validate(goods);
if(validate.getCode() != CodeMsg.SUCCESS.getCode()){
return Result.error(validate);
}
if(goods.getGoodsCategory() == null || goods.getGoodsCategory().getId() == null || goods.getGoodsCategory().getId().longValue() == -1){
return Result.error(CodeMsg.HOME_STUDENT_PUBLISH_CATEGORY_EMPTY);
}
Student loginedStudent = (Student)SessionUtil.get(SessionConstant.SESSION_STUDENT_LOGIN_KEY);
goods.setStudent(loginedStudent);
if(goodsService.save(goods) == null){
return Result.error(CodeMsg.HOME_STUDENT_PUBLISH_ERROR);
}
return Result.success(true);
}
/**
* 物品编辑页面
* @param id
* @param model
* @return
*/
@RequestMapping(value="/edit_goods",method=RequestMethod.GET)
public String publish(@RequestParam(name="id",required=true)Long id,Model model){
Student loginedStudent = (Student)SessionUtil.get(SessionConstant.SESSION_STUDENT_LOGIN_KEY);
Goods goods = goodsService.find(id, loginedStudent.getId());
if(goods == null){
model.addAttribute("msg", "物品不存在!");
return "error/runtime_error";
}
model.addAttribute("goods", goods);
return "home/student/edit_goods";
}
/**
* 物品编辑表单提交
* @param goods
* @return
*/
@RequestMapping(value="/edit_goods",method=RequestMethod.POST)
@ResponseBody
public Result<Boolean> editGoods(Goods goods){
CodeMsg validate = ValidateEntityUtil.validate(goods);
if(validate.getCode() != CodeMsg.SUCCESS.getCode()){
return Result.error(validate);
}
if(goods.getGoodsCategory() == null || goods.getGoodsCategory().getId() == null || goods.getGoodsCategory().getId().longValue() == -1){
return Result.error(CodeMsg.HOME_STUDENT_PUBLISH_CATEGORY_EMPTY);
}
Student loginedStudent = (Student)SessionUtil.get(SessionConstant.SESSION_STUDENT_LOGIN_KEY);
Goods existGoods = goodsService.find(goods.getId(), loginedStudent.getId());
if(existGoods == null){
return Result.error(CodeMsg.HOME_STUDENT_GOODS_NO_EXIST);
}
existGoods.setBuyPrice(goods.getBuyPrice());
existGoods.setContent(goods.getContent());
existGoods.setGoodsCategory(goods.getGoodsCategory());
existGoods.setName(goods.getName());
existGoods.setPhoto(goods.getPhoto());
existGoods.setSellPrice(goods.getSellPrice());
if(goodsService.save(existGoods) == null){
return Result.error(CodeMsg.HOME_STUDENT_GOODS_EDIT_ERROR);
}
return Result.success(true);
}
/**
* 用户设置是否擦亮物品
* @param id
* @param flag
* @return
*/
@RequestMapping(value="/update_flag",method=RequestMethod.POST)
@ResponseBody
public Result<Boolean> updateFlag(@RequestParam(name="id",required=true)Long id,
@RequestParam(name="flag",required=true,defaultValue="0")Integer flag){
Student loginedStudent = (Student)SessionUtil.get(SessionConstant.SESSION_STUDENT_LOGIN_KEY);
Goods existGoods = goodsService.find(id, loginedStudent.getId());
if(existGoods == null){
return Result.error(CodeMsg.HOME_STUDENT_GOODS_NO_EXIST);
}
existGoods.setFlag(flag);
if(goodsService.save(existGoods) == null){
return Result.error(CodeMsg.HOME_STUDENT_GOODS_EDIT_ERROR);
}
return Result.success(true);
}
/**
* 修改物品状态
* @param id
* @param status
* @return
*/
@RequestMapping(value="/update_status",method=RequestMethod.POST)
@ResponseBody
public Result<Boolean> updateStatus(@RequestParam(name="id",required=true)Long id,
@RequestParam(name="status",required=true,defaultValue="2")Integer status){
Student loginedStudent = (Student)SessionUtil.get(SessionConstant.SESSION_STUDENT_LOGIN_KEY);
Goods existGoods = goodsService.find(id, loginedStudent.getId())
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
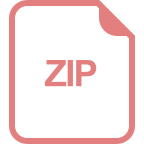
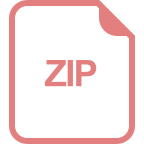
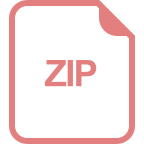
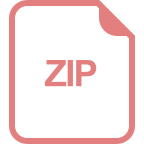
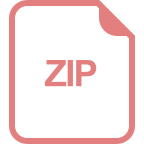
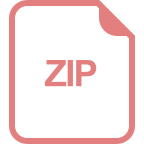
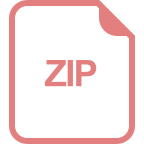
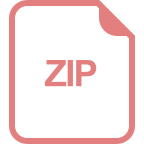
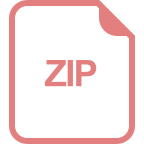
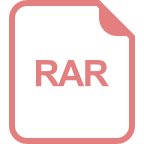
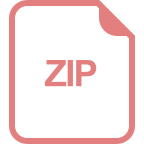
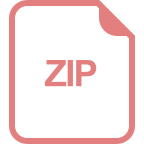
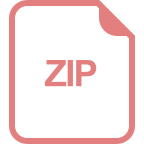
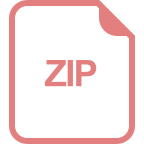
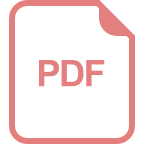
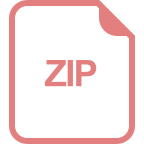
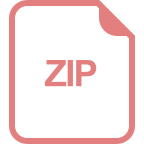
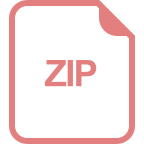
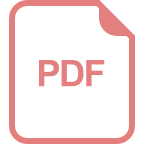
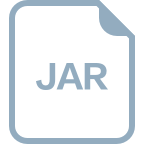
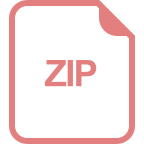
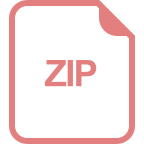
收起资源包目录

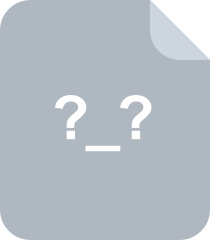
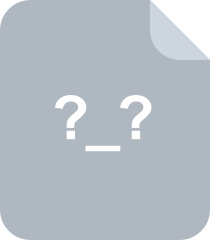
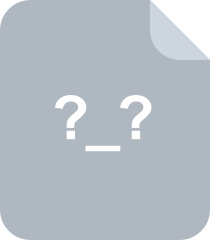
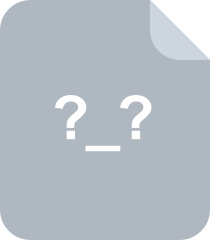
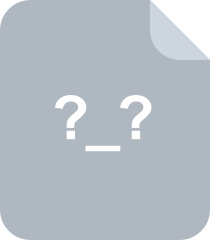
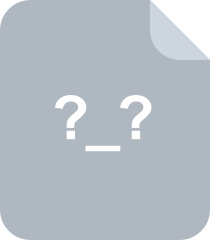
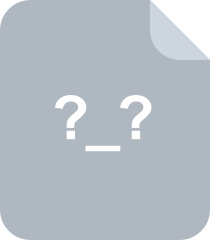
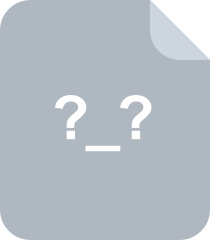
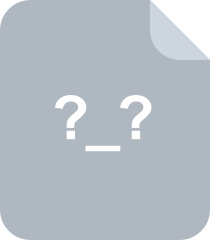
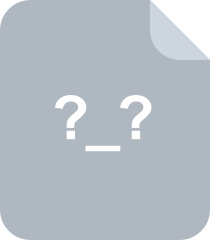
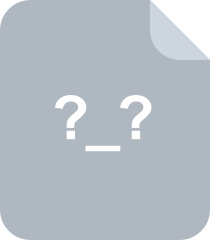
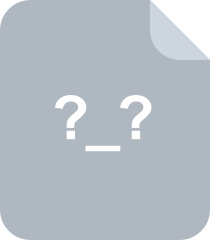
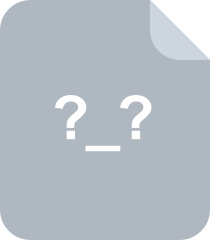
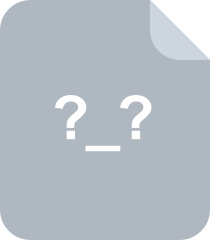
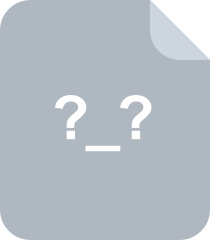
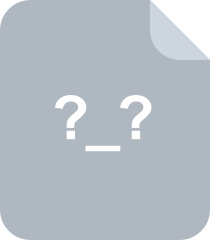
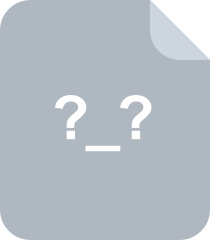
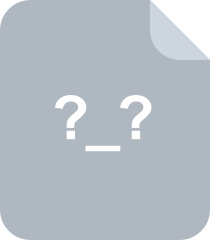
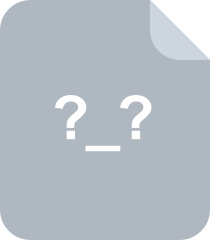
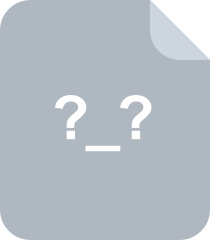
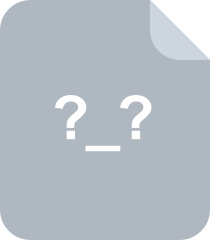
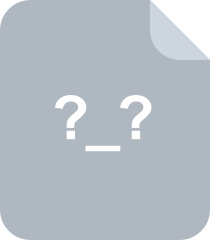
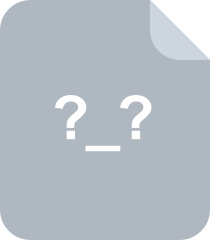
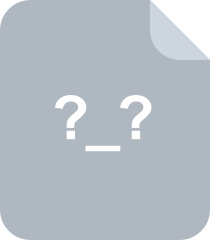
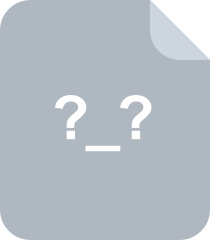
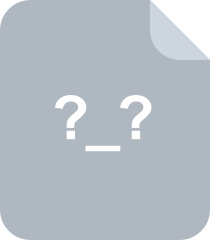
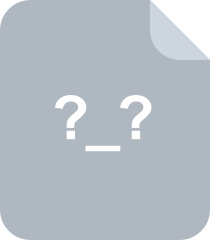
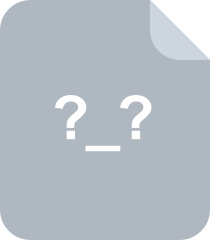
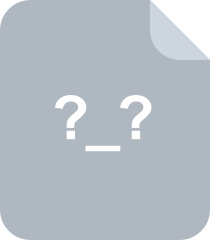
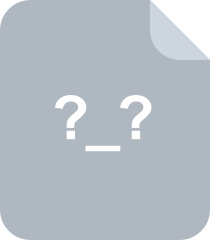
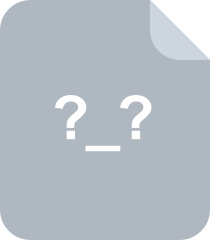
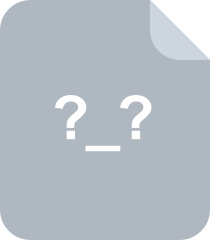
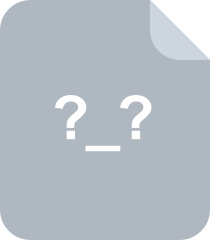
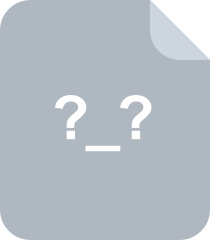
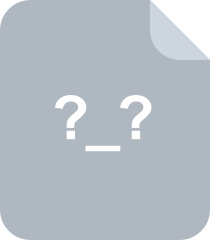
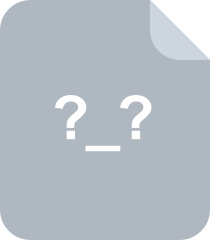
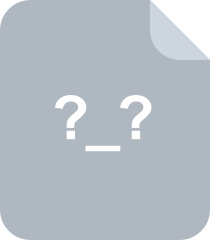
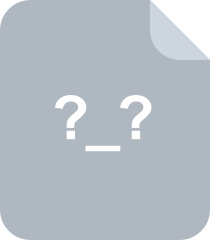
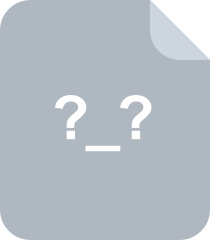
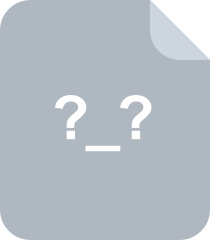
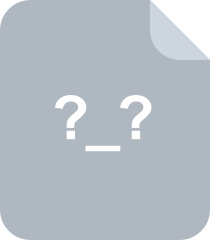
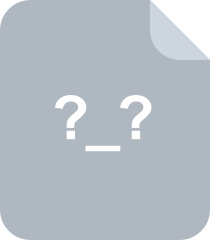
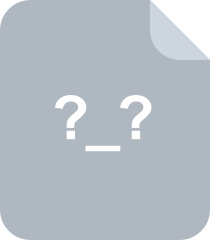
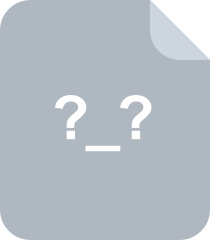
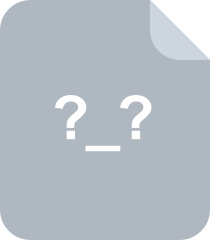
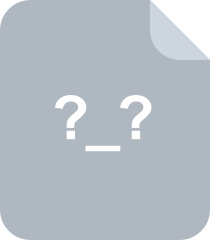
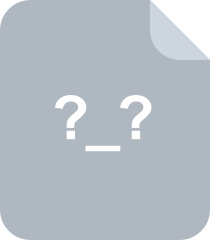
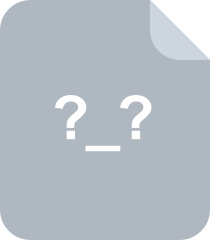
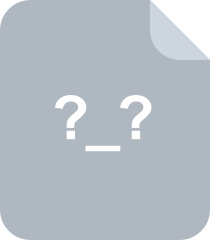
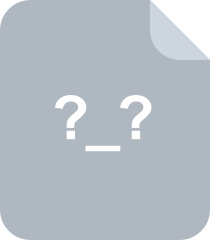
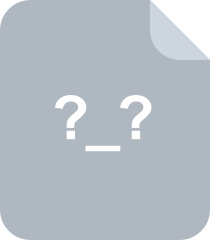
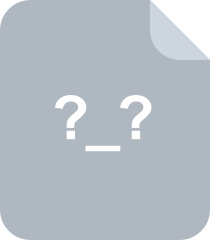
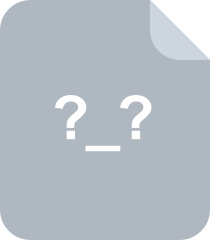
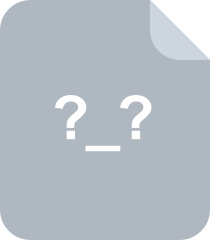
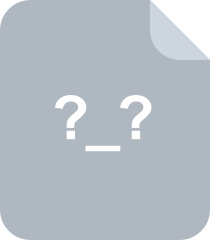
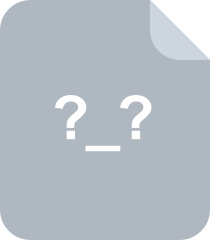
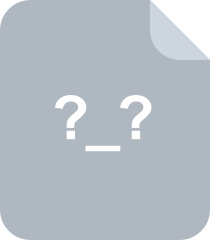
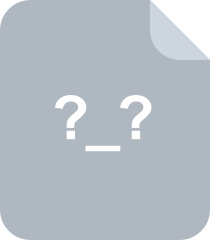
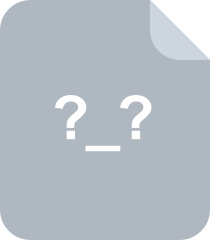
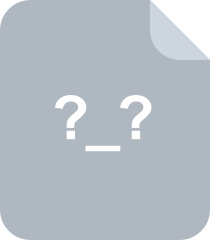
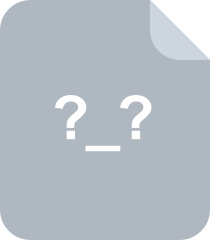
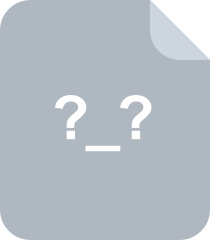
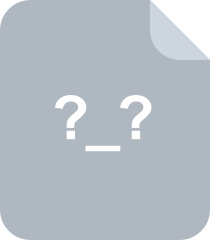
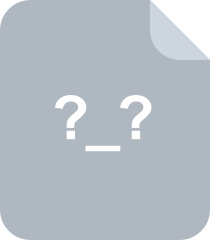
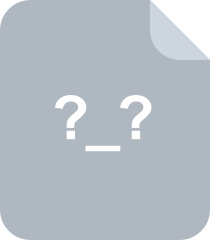
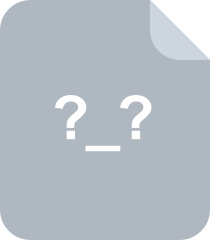
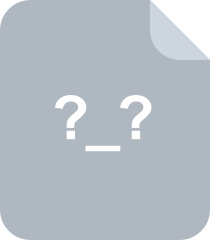
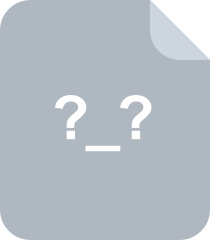
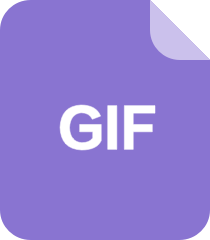
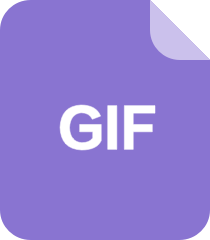
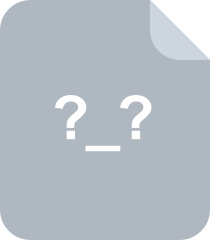
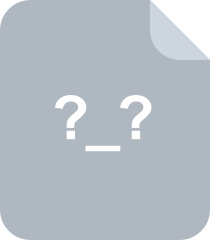
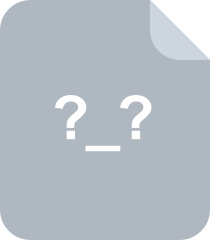
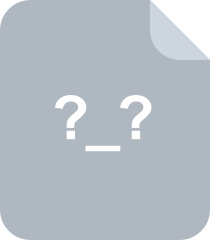
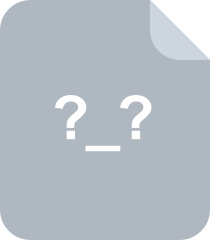
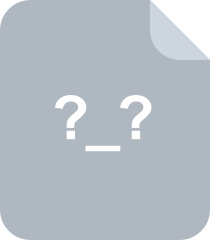
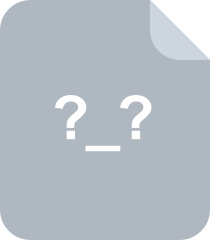
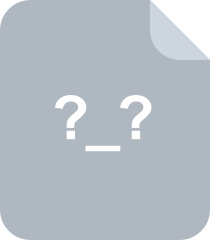
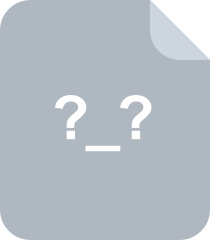
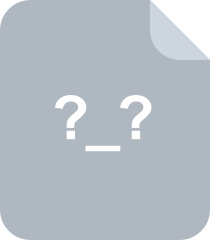
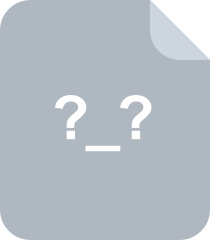
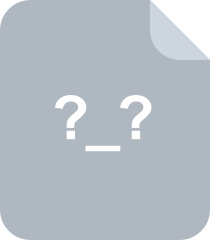
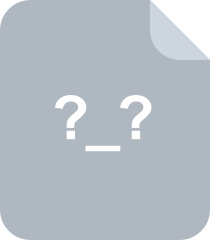
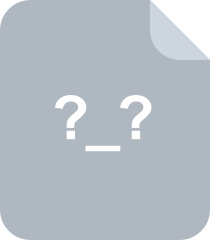
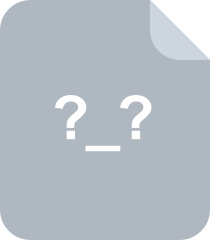
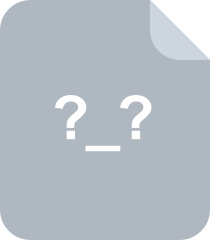
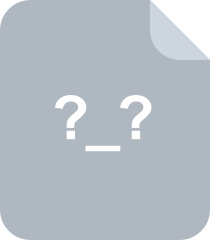
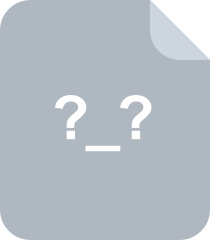
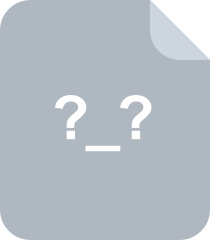
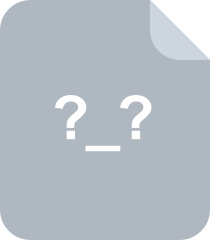
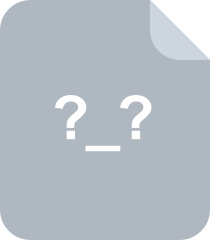
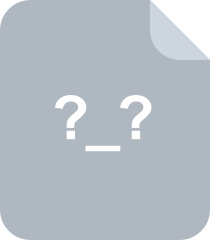
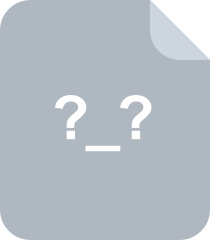
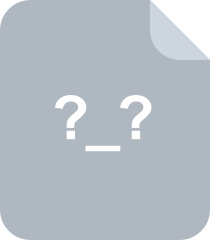
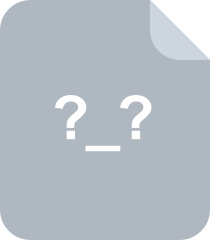
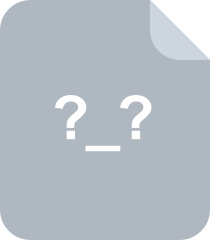
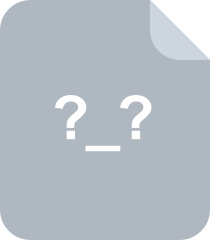
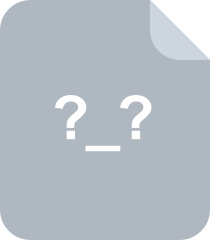
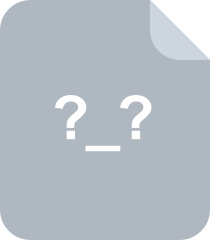
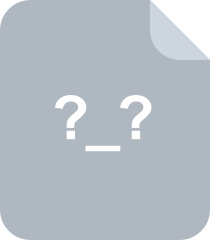
共 516 条
- 1
- 2
- 3
- 4
- 5
- 6
资源评论
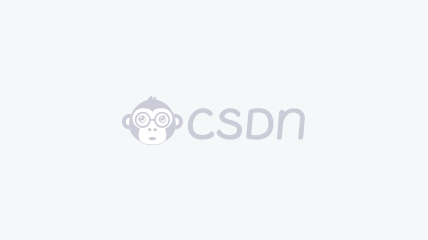

博士僧小星
- 粉丝: 1985
- 资源: 5910
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

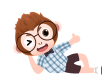
最新资源
- 一个基于 tauri + rust + vue 构建的抢票软件,全部调用大麦的接口
- 大麦网 演唱会抢票软件,一个基于 tauri + rust + vue 调用接口的抢票软件
- nessus-10.6.3.-x64
- 编程实战:基于Javamail的邮件收发系统的设计与实现(源码+文档+开题报告+答辩PPT).zip
- 用Java写五子棋小游戏swing,有运行教程拿着就能运行的源码
- HCM2.0(中文)英威腾通讯软件
- 毕设项目:基于Javamail的邮件收发系统的设计与实现(系统+文档+开题报告+答辩PPT).zip
- 基于python+pygame实现的酷跑游戏,只有5关课程设计
- 编程实战:基于JAVA局域网的聊天室系统(源代码+文档).zip
- medicalclient-2024-6-28.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


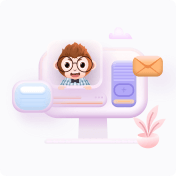
安全验证
文档复制为VIP权益,开通VIP直接复制
