package cn.appsys.controller.developer;
import java.io.File;
import java.io.IOException;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.annotation.Resource;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpSession;
import org.apache.commons.io.FilenameUtils;
import org.apache.log4j.Logger;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.multipart.MultipartFile;
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONObject;
import cn.appsys.pojo.AppCategory;
import cn.appsys.pojo.AppInfo;
import cn.appsys.pojo.AppVersion;
import cn.appsys.pojo.DataDictionary;
import cn.appsys.pojo.DevUser;
import cn.appsys.service.AppCategoryService;
import cn.appsys.service.AppInfoService;
import cn.appsys.service.AppVersionService;
import cn.appsys.service.DataDictionaryService;
import cn.appsys.tools.Page;
@Controller
@RequestMapping("/dev/flatform/app/")
public class AppController {
private Logger logger = Logger.getLogger(AppController.class);
@Resource
private AppInfoService appInfoService;
public void setAppService(AppInfoService appService) {
this.appInfoService = appService;
}
@Resource
private DataDictionaryService dataDictionaryService;
public void setDataService(DataDictionaryService dataService) {
this.dataDictionaryService = dataService;
}
@Resource
private AppCategoryService appCategoryService;
public void setCategoryService(AppCategoryService categoryService) {
this.appCategoryService = categoryService;
}
@Resource
private AppVersionService appVersionService;
public void setVersionService(AppVersionService versionService) {
this.appVersionService = versionService;
}
/**
* App列表
* @return
*/
@RequestMapping(value="/Applist.html",method=RequestMethod.GET)
public String getAppList(Model model,
@RequestParam(value="querySoftwareName",required=false)String softwareName,@RequestParam(value="queryStatus",required=false)Integer queryStatus,
@RequestParam(value="queryFlatformId",required=false)Integer flatformId,@RequestParam(value="queryCategoryLevel1",required=false)Integer categoryLevel1,
@RequestParam(value="queryCategoryLevel2",required=false)Integer categoryLevel2,@RequestParam(value="queryCategoryLevel3",required=false)Integer categoryLevel3,
@RequestParam(value="pageIndex",required=false)Integer index){
Map<String,Object> map=new HashMap<String,Object>();
//当前页码
if(index==null||"".equals(index)){
index=1;
}
//软件名称
if(softwareName!=null&&!"".equals(softwareName)){
map.put("softwareName", softwareName);
}
//状态
if(queryStatus!=null&&!"".equals(queryStatus)){
map.put("status", queryStatus);
}
//所属平台
if(flatformId!=null&&!"".equals(flatformId)){
map.put("flatformId", flatformId);
}
//一级分类
if(categoryLevel1!=null&&!"".equals(categoryLevel1)){
map.put("categoryLevel1", categoryLevel1);
}
//二级分类
if(categoryLevel2!=null&&!"".equals(categoryLevel2)){
map.put("categoryLevel2", categoryLevel2);
}
//三级分类
if(categoryLevel3!=null&&!"".equals(categoryLevel3)){
map.put("categoryLevel3", categoryLevel3);
}
map.put("index", (index-1)*Page.SIZE);
map.put("size", Page.SIZE);
//程序调用
Page<AppInfo> page=appInfoService.getAppList(map);
List<DataDictionary> statusList=dataDictionaryService.findDataList("APP_STATUS");
List<DataDictionary> flatFormList=dataDictionaryService.findDataList("APP_FLATFORM");
List<AppCategory> categoryLevel1List=appCategoryService.appCategoryList(null);
List<AppCategory> categoryLevel2List=null;
List<AppCategory> categoryLevel3List=null;
if ((categoryLevel2 != null) && (!categoryLevel2.equals("")))
{
categoryLevel2List = appCategoryService.appCategoryList(categoryLevel1.toString());
}
if ((categoryLevel3 != null) && (!categoryLevel3.equals("")))
{
categoryLevel3List = appCategoryService.appCategoryList(categoryLevel2.toString());
}
//传页码
page.setCurrentPageNo(index);
//传参
model.addAttribute("appInfoList", page.getList());
model.addAttribute("statusList", statusList);
model.addAttribute("flatFormList", flatFormList);
model.addAttribute("categoryLevel1List", categoryLevel1List);
model.addAttribute("categoryLevel2List", categoryLevel2List);
model.addAttribute("categoryLevel3List", categoryLevel3List);
model.addAttribute("queryStatus", queryStatus);
model.addAttribute("queryFlatformId", flatformId);
model.addAttribute("querySoftwareName", softwareName);
model.addAttribute("queryCategoryLevel1", categoryLevel1);
model.addAttribute("queryCategoryLevel2", categoryLevel2);
model.addAttribute("queryCategoryLevel3", categoryLevel3);
model.addAttribute("pages", page);
return "/developer/appinfolist";
}
/**
* 分级查询(json)
* @return
* json数据
*/
@RequestMapping(value="/categorylevellist.json",method=RequestMethod.GET,produces="application/json")
@ResponseBody
public Object categorylevellist(@RequestParam("pid")String pid){
JSONArray json=new JSONArray();
List<AppCategory> categoryLevelList=null;
if(pid!=null||!"".equals(pid)){
categoryLevelList=appCategoryService.appCategoryList(pid);
}
if(pid==null||"".equals(pid)){
categoryLevelList=appCategoryService.appCategoryList(null);
}
for (AppCategory appCategory : categoryLevelList) {
JSONObject object=new JSONObject();
object.put("id", appCategory.getId());
object.put("categoryName", appCategory.getCategoryName());
json.add(object);
}
return json;
}
/**
* 新增app(跳转)
*
*创建时间: 2017年11月2日 上午11:48:50
*@author: Angelo yin
*@return
*/
@RequestMapping("/appinfoadd.html")
public String appinfoAdd(@ModelAttribute("appinfo")AppInfo appinfo){
return "developer/appinfoadd";
}
/**
* 加载平台
*
*创建时间: 2017年11月2日 上午11:54:14
*@author: Angelo yin
*@return
*/
@RequestMapping("/datadictionarylist.json")
@ResponseBody
public Object datadictionarylist(@RequestParam("tcode")String typeName){
JSONArray json=new JSONArray();
List<DataDictionary> flatFormList=dataDictionaryService.findDataList(typeName);
for (DataDictionary dataDictionary : flatFormList) {
JSONObject object=new JSONObject();
object.put("valueId", dataDictionary.getValueId());
object.put("valueName", dataDictionary.getValueName());
json.add(object);
}
return json;
}
/**
* 查询是否存在APKName
*
*创建时间: 2017年11月2日 下午1:34:08
*@author: Angelo yin
*@return
*/
@RequestMapping("/apkexist.json")
@ResponseBody
public Object apkexist(@RequestParam("APKName")String APKName){
JSONObject object=new JSONObject();
Integer count=5;
if(APKName!=null&&!"".equals(APKName)){
count=appInfoService.findAPKName(APKName);
}
if(APKName.equals("")){
object.put("APKName", "empty");
}else if(count>=1){
object.put("APKName", "exist");
}else if(count==0){
object.put("APKName", "noexist");
}
return object;
}
/**
* 修改上下架状态
*
*创建时间: 2017年11月3日 下午4:00:43
*@author: Angelo yin
*@return
*/
@Reque
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
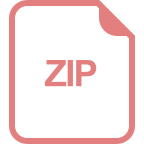
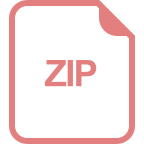
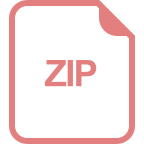
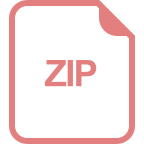
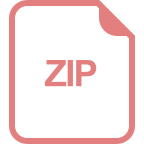
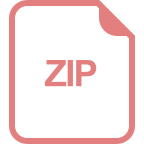
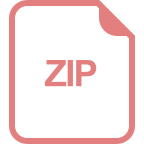
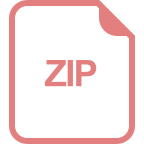
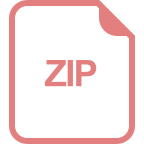
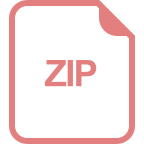
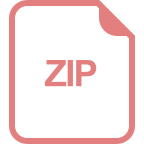
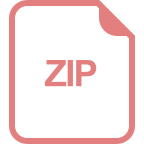
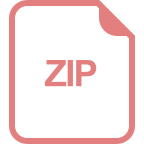
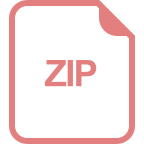
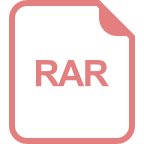
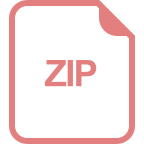
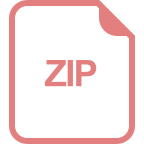
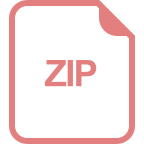
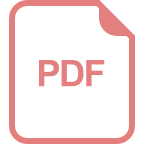
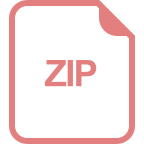
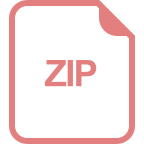
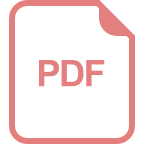
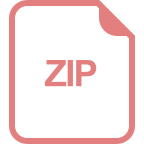
收起资源包目录

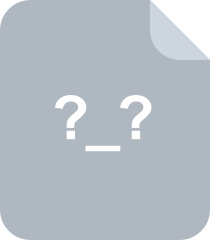
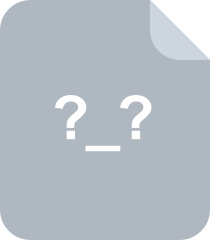
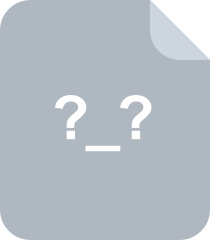
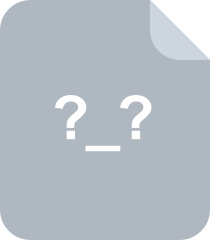
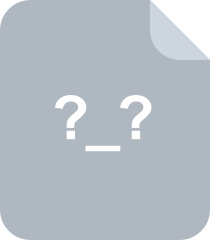
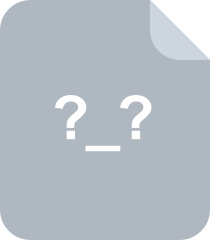
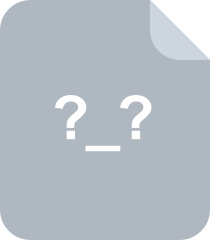
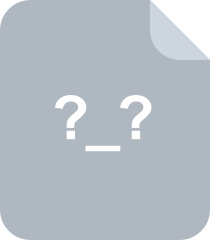
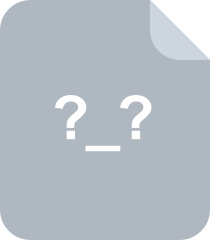
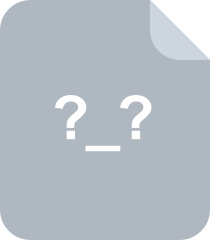
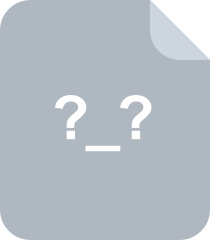
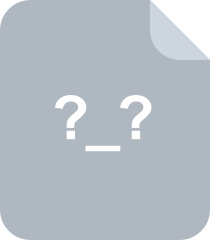
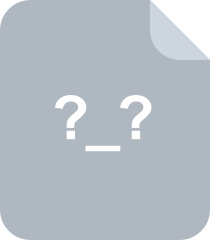
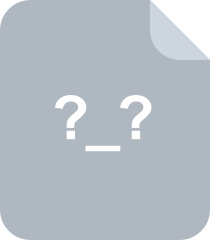
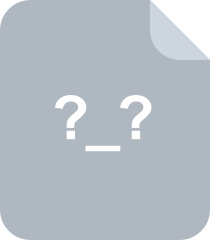
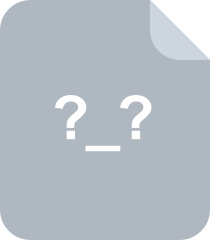
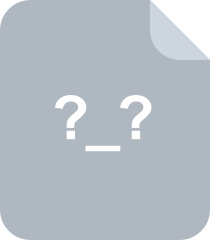
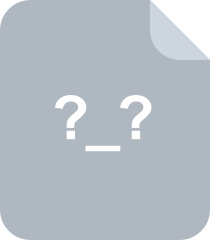
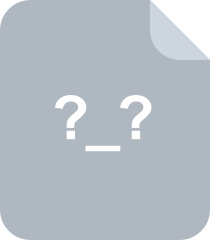
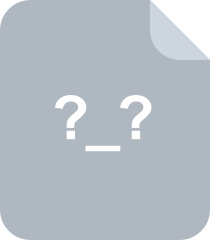
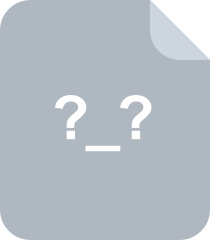
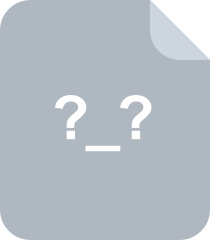
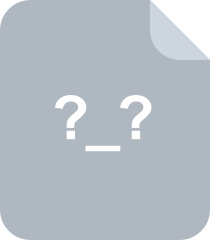
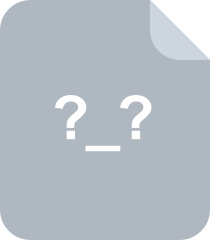
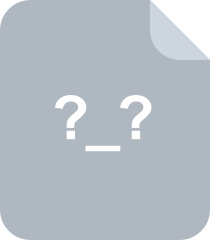
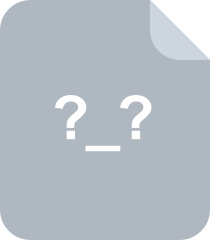
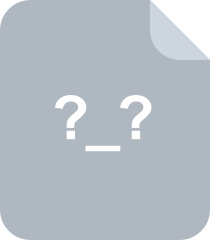
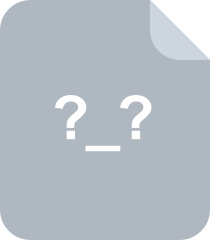
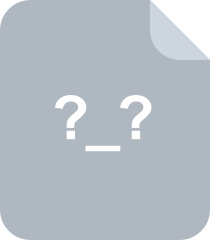
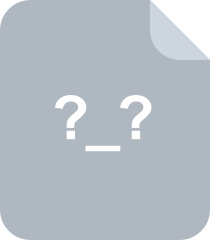
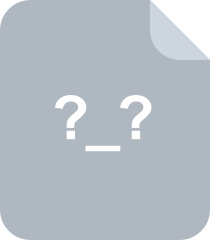
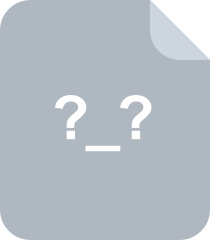
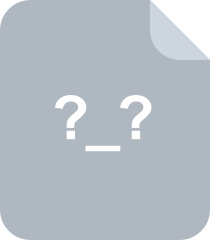
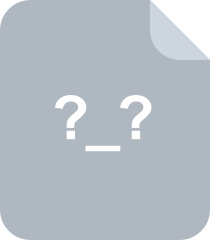
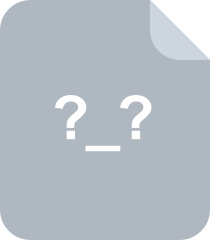
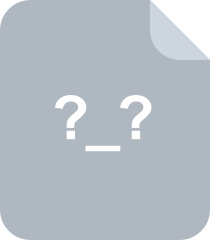
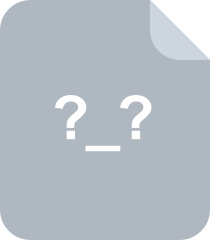
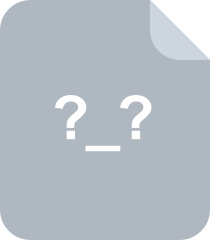
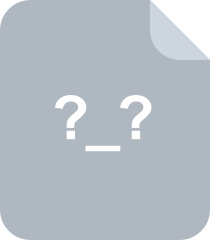
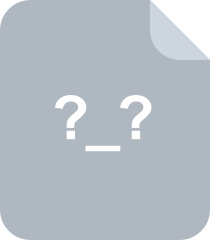
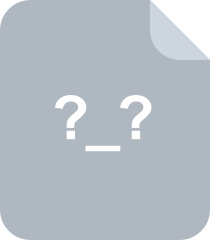
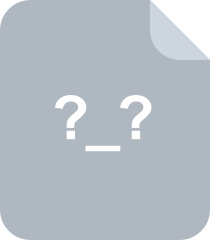
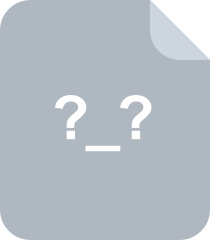
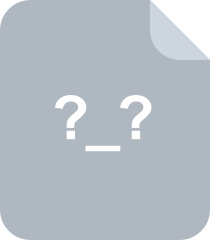
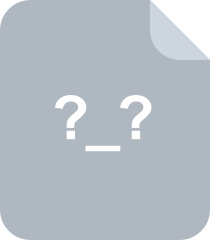
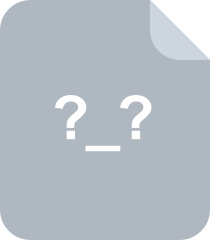
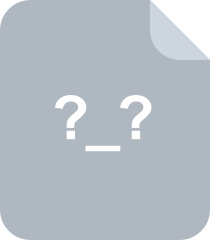
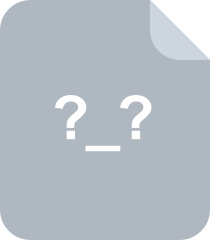
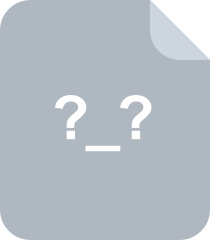
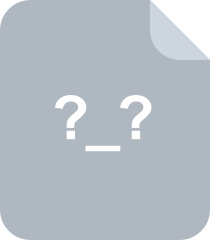
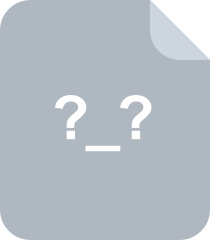
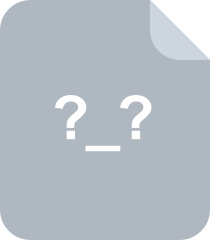
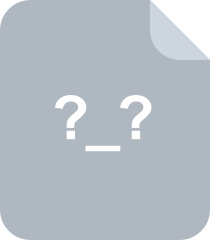
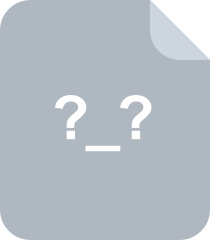
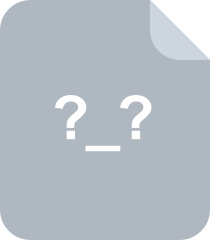
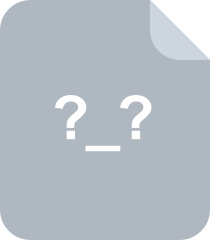
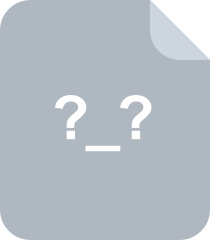
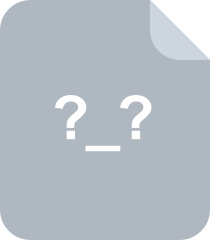
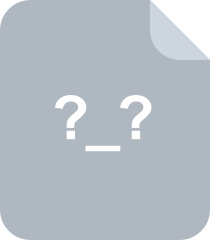
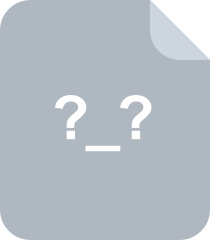
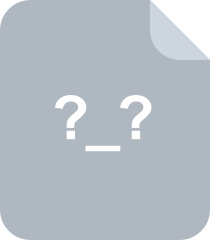
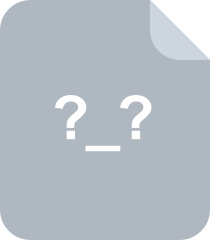
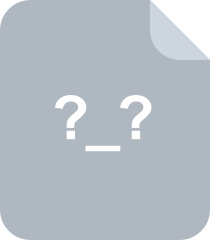
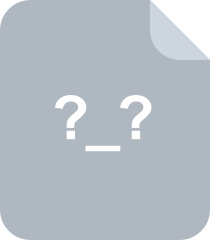
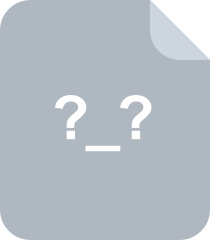
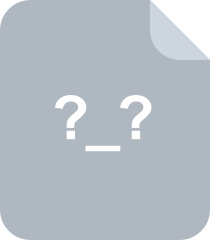
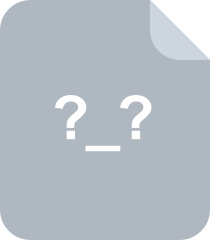
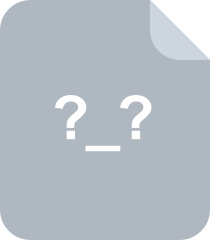
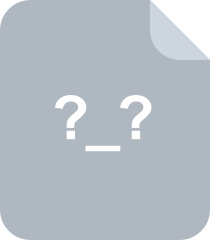
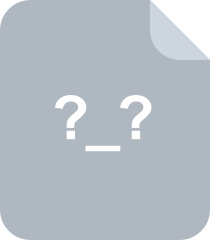
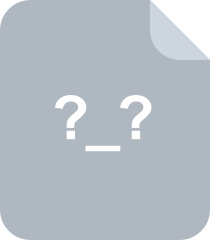
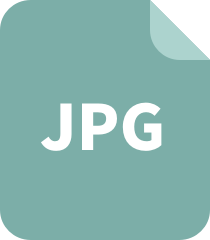
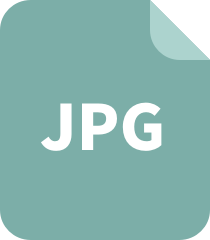
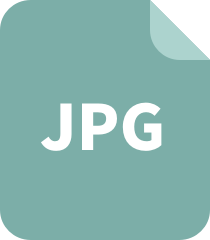
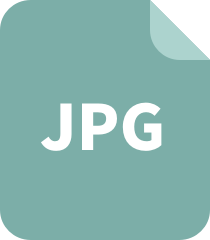
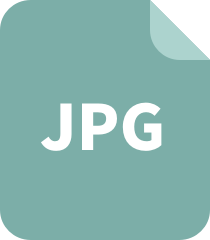
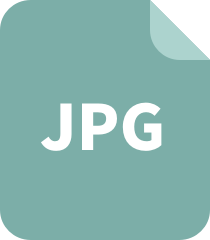
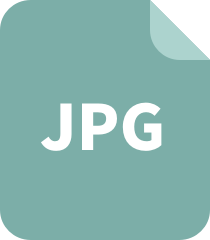
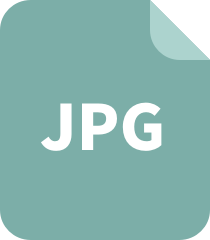
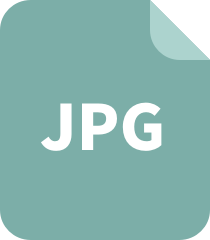
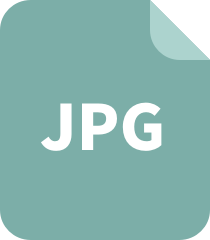
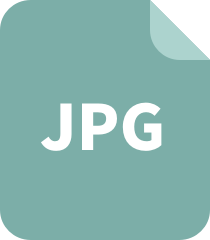
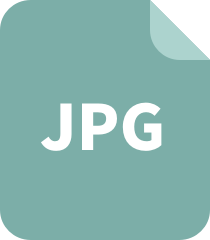
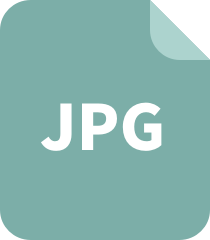
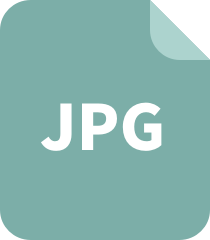
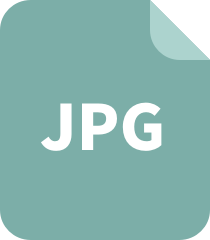
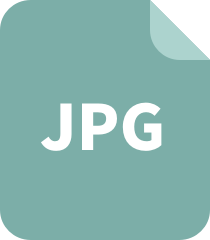
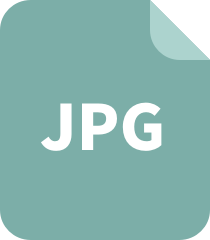
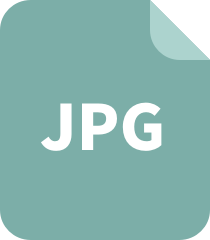
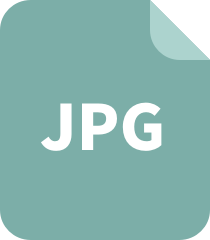
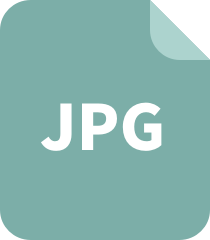
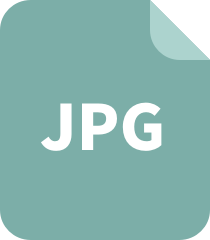
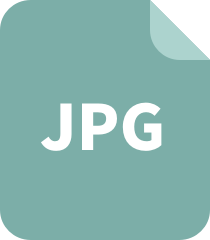
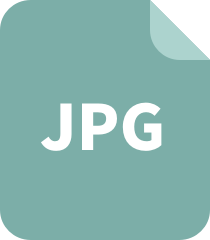
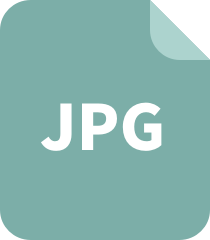
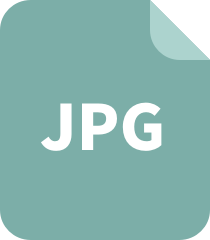
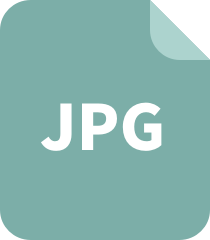
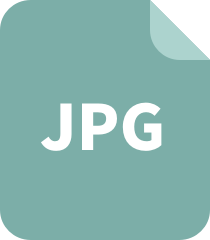
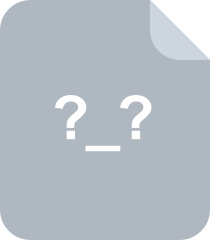
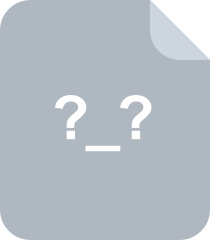
共 175 条
- 1
- 2
资源评论
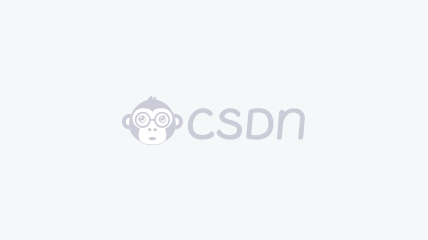

博士僧小星
- 粉丝: 1947
- 资源: 5905
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

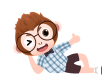
最新资源
- 网络爬虫软件研究与开发pdf
- Java项目-基于SSM+JSP的母婴用品网站的设计与实现(源码+数据库脚本+部署视频+代码讲解视频+全套软件)
- 基于微信小程序的购物商城app设计带Java后端+源代码+文档说明+数据库.zip
- 基于51单片机外设应用设计.DSN后缀PROTEUS仿真仿真源文件及C语言实例源码例程合集(300个).zip
- “Bunnies and Badgers”兔子和獾和是一个基于pygame库开发的射击游戏
- 华为打印机,华为打印机资料
- Java项目-基于SSM+JSP的医院门诊挂号系统的设计与实现(源码+数据库脚本+部署视频+代码讲解视频+全套软件)
- mac os button功能demo
- 如何在Ubuntu上安装软件?
- 华为HCIA-WLAN 3.0 课程视频(20 熟悉命令行.mp4)
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


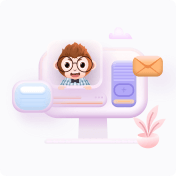
安全验证
文档复制为VIP权益,开通VIP直接复制
