#!/usr/bin/env python
# -*- coding: utf-8 -*-
# @Date : 2019-04-08 11:58:13
# @Author : Residual Laugh (Residual.Laugh@gmail.com)
# @Link : https://blog.0xccc.cc
import os
import sys
reload(sys)
sys.setdefaultencoding('utf-8')
import optparse
import censys
import censys.certificates
import censys.ipv4
from IPy import IPSet, IP
UID = "your censys api UID"
SECRET = "your censys api SECRET"
def getv(d, key):
if key in d:
return d[key]
else:
return []
def censys_sub_domain(domain):
print u"[-] Start find subdomains.."
subdomains = []
try:
censys_certificates = censys.certificates.CensysCertificates(api_id=UID, api_secret=SECRET)
certificate_query = 'parsed.names: %s' % domain
certificates_search_results = censys_certificates.search(certificate_query, fields=['parsed.names'], max_records = 1000)
for search_result in certificates_search_results:
subdomains.extend(search_result['parsed.names'])
print u"[-] Stop find subdomains.."
return {'domain': domain,'subdomains':[ subdomain for subdomain in set(subdomains) if '*' not in subdomain and subdomain.endswith(domain) ]}
except censys.base.CensysUnauthorizedException:
print '[-] Your Censys credentials look invalid.\n'
except censys.base.CensysRateLimitExceededException:
print '[-] Looks like you exceeded your Censys account limits rate. Exiting\n'
except censys.base.CensysException as e:
# catch the Censys Base exception, example "only 1000 first results are available"
print '[-] Something bad happened, ' + repr(e)
finally:
return {'domain': domain,'subdomains': [ subdomain for subdomain in set(subdomains) if '*' not in subdomain and subdomain.endswith(domain) ]}
def censys_ipv4(domain):
print u"[-] Start find real ip.."
results = []
ip_list = []
IPV4_FIELDS = ['ip',
'ports',
'location.country',
'location.province',
'updated_at',
'80.http.get.title',
'80.http.get.headers.server',
'443.https.get.title',
'443.https.get.headers.server',
'443.https.tls.certificate.parsed.subject_dn',
'443.https.tls.certificate.parsed.names',
'443.https.tls.certificate.parsed.subject.common_name',
'443.https.tls.certificate.parsed.extensions.subject_alt_name.dns_names',
'25.smtp.starttls.tls.certificate.parsed.names',
'25.smtp.starttls.tls.certificate.parsed.subject_dn',
'110.pop3.starttls.tls.certificate.parsed.names',
'110.pop3.starttls.tls.certificate.parsed.subject_dn']
try:
c = censys.ipv4.CensysIPv4(api_id=UID, api_secret=SECRET)
data = list(c.search("(80.http.get.status_code: 200 or 443.https.get.status_code: 200) and 443.https.tls.certificate.parsed.names: %s or 25.smtp.starttls.tls.certificate.parsed.names: %s or 110.pop3.starttls.tls.certificate.parsed.names: %s" %(domain,domain,domain), IPV4_FIELDS, max_records = 1000))
if len(data)>999:
data = data[:999]
for i in data:
ip_list.append(i['ip'])
results.append({'ip': i['ip'],
'ports': i['ports'],
'location': getv(i,'location.country') or '' + ' ' + getv(i,'location.province') or '',
'updated_at': i['updated_at'],
'title': {'80': getv(i,'80.http.get.title'),'443': getv(i,'443.https.get.title')},
'server': {'80': getv(i,'80.http.get.headers.server'),'443': getv(i,'443.https.get.headers.server')},
'names': list(set(getv(i,'443.https.tls.certificate.parsed.names')+getv(i,'443.https.tls.certificate.parsed.extensions.subject_alt_name.dns_names')+getv(i,'25.smtp.starttls.tls.certificate.parsed.names')+getv(i,'110.pop3.starttls.tls.certificate.parsed.names')))
})
print u"[-] Stop find real ip.."
return results
except censys.base.CensysUnauthorizedException:
print '[-] Your Censys credentials look invalid.\n'
except censys.base.CensysRateLimitExceededException:
print '[-] Looks like you exceeded your Censys account limits rate. Exiting\n'
except censys.base.CensysException as e:
# catch the Censys Base exception, example "only 1000 first results are available"
print '[-] Something bad happened, ' + repr(e)
finally:
return results,ip_list
def output(domain,subdomains,results,ip_c):
y_domains = []
with open(os.path.join(domain+'.txt'),'w') as f:
for result in results:
y_domains += result['names']
print result['ip']+'\t'+','.join(result['names'])+'\t'+','.join('%s' %port for port in result['ports'])+'\t80:'+''.join(result['server']['80'])+' '+''.join(result['title']['80'])+'\t443:'+''.join(result['server']['443'])+' '+''.join(result['title']['443'])
f.writelines(str(result['ip']+'\t'+','.join(result['names'])+'\t'+','.join('%s' %port for port in result['ports'])+'\t80:'+''.join(result['server']['80'])+' '+''.join(result['title']['80'])+'\t443:'+''.join(result['server']['443'])+' '+''.join(result['title']['443']))+'\n')
print u"[-] No real IP was found in the following domain names"
print ' '.join(set(subdomains) - set(y_domains))
f.writelines('\n\nNo real IP was found in the following domain names\n')
for sdomain in list(set(subdomains) - set(y_domains)):
f.writelines(sdomain+'\n')
print u"[-] The above information has been output to %s" %os.path.join(domain+'.txt')
with open(os.path.join(domain+'_c.txt'),'w') as f:
print u"[-] You can focus on these C-segments"
for ip in ip_c:
print ip
f.writelines(str(ip)+'\n')
def ip_list2c(ip_list):
ips = []
for i in ip_list:
ips.append(IP(i+'/24', make_net=True))
for j in ips:
if ips.count(j) < 2:
ips.remove(j)
return IPSet(ips)
if __name__ == '__main__':
parser = optparse.OptionParser('usage: %prog target.com', version="%prog 1.0.1")
(options, args) = parser.parse_args()
if len(args) < 1:
parser.print_help()
sys.exit(0)
domain = args[0]
subdomains = censys_sub_domain(domain)
subdomains = subdomains['subdomains']
results, ip_list = censys_ipv4(domain)
output(domain, subdomains, results, ip_list2c(ip_list))
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
通过censys搜索引擎针对使用ssl证书的域名进行资产探测(真实ip)和子域名收集,这种方法可以发现常规资产探测不易发现的站点 用于日常渗透测试工作中 [+] 增加了自定义C段扫描,获取中间件和网站标题 Install 语言环境 For Python2.7 安装依赖 pip install censys 注册censys账号,替换脚本中的UID和SECRET
资源推荐
资源详情
资源评论
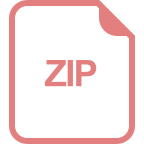
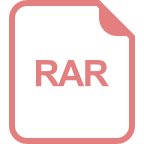
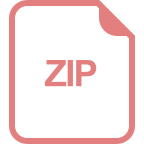
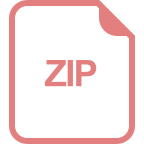
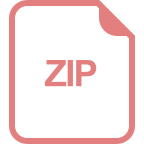
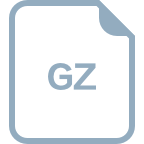
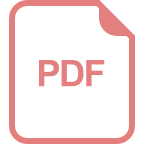
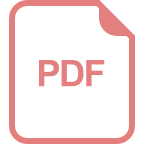
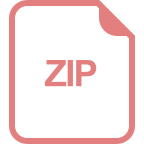
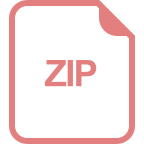
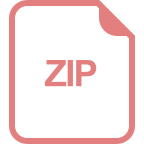
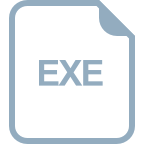
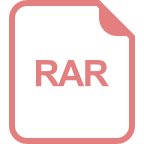
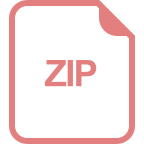
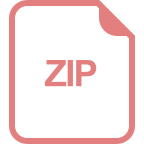
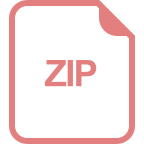
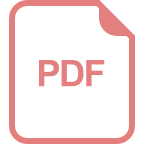
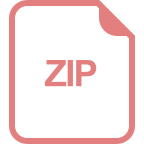
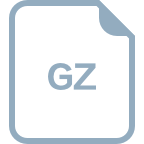
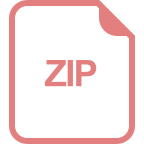
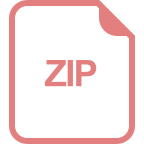
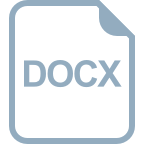
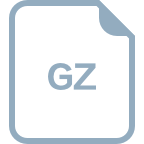
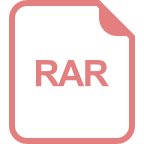
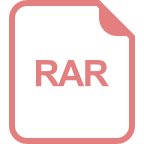
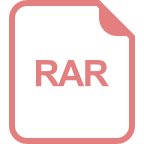
收起资源包目录


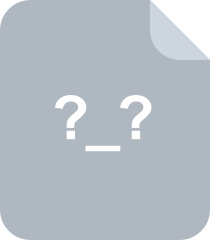
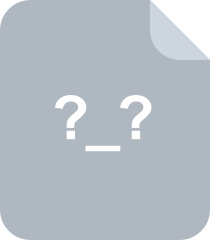
共 2 条
- 1
资源评论
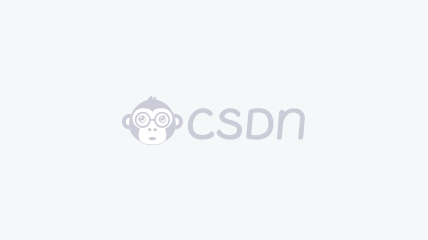
- 2301_764295132024-05-05超级好的资源,很值得参考学习,对我启发很大,支持!

博士僧小星
- 粉丝: 2247
- 资源: 5990
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

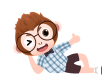
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


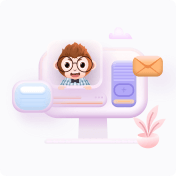
安全验证
文档复制为VIP权益,开通VIP直接复制
