// Code generated - DO NOT EDIT.
// This file is a generated binding and any manual changes will be lost.
package generateId
import (
"math/big"
"strings"
ethereum "github.com/ethereum/go-ethereum"
"github.com/ethereum/go-ethereum/accounts/abi"
"github.com/ethereum/go-ethereum/accounts/abi/bind"
"github.com/ethereum/go-ethereum/common"
"github.com/ethereum/go-ethereum/core/types"
"github.com/ethereum/go-ethereum/event"
)
// Reference imports to suppress errors if they are not otherwise used.
var (
_ = big.NewInt
_ = strings.NewReader
_ = ethereum.NotFound
_ = abi.U256
_ = bind.Bind
_ = common.Big1
_ = types.BloomLookup
_ = event.NewSubscription
)
// GenerateIdABI is the input ABI used to generate the binding from.
const GenerateIdABI = "[{\"constant\":false,\"inputs\":[{\"name\":\"_id\",\"type\":\"bytes32\"}],\"name\":\"setId\",\"outputs\":[],\"payable\":true,\"stateMutability\":\"payable\",\"type\":\"function\"},{\"constant\":true,\"inputs\":[],\"name\":\"id\",\"outputs\":[{\"name\":\"\",\"type\":\"bytes32\"}],\"payable\":false,\"stateMutability\":\"view\",\"type\":\"function\"},{\"inputs\":[],\"payable\":false,\"stateMutability\":\"nonpayable\",\"type\":\"constructor\"},{\"anonymous\":false,\"inputs\":[{\"indexed\":false,\"name\":\"_id\",\"type\":\"bytes32\"}],\"name\":\"LogId\",\"type\":\"event\"}]"
// GenerateIdBin is the compiled bytecode used for deploying new contracts.
const GenerateIdBin = `608060405234801561001057600080fd5b507f300000000000000000000000000000000000000000000000000000000000000060008190555060fc806100466000396000f3fe60806040526004361060265760003560e01c806349bfcf0214602b578063af640d0f146056575b600080fd5b605460048036036020811015603f57600080fd5b8101908080359060200190929190505050607e565b005b348015606157600080fd5b50606860c1565b6040518082815260200191505060405180910390f35b806000819055507f2ec078554f30cba9aad72c89ddc37203c0e04df1058fa6c1959f5be52ca282d46000546040518082815260200191505060405180910390a150565b6000548156fea265627a7a72305820829398546dbcf67bba9333d3db024a17d501d25356086c9d9996672df12012af64736f6c63430005090032`
// DeployGenerateId deploys a new Ethereum contract, binding an instance of GenerateId to it.
func DeployGenerateId(auth *bind.TransactOpts, backend bind.ContractBackend) (common.Address, *types.Transaction, *GenerateId, error) {
parsed, err := abi.JSON(strings.NewReader(GenerateIdABI))
if err != nil {
return common.Address{}, nil, nil, err
}
address, tx, contract, err := bind.DeployContract(auth, parsed, common.FromHex(GenerateIdBin), backend)
if err != nil {
return common.Address{}, nil, nil, err
}
return address, tx, &GenerateId{GenerateIdCaller: GenerateIdCaller{contract: contract}, GenerateIdTransactor: GenerateIdTransactor{contract: contract}, GenerateIdFilterer: GenerateIdFilterer{contract: contract}}, nil
}
// GenerateId is an auto generated Go binding around an Ethereum contract.
type GenerateId struct {
GenerateIdCaller // Read-only binding to the contract
GenerateIdTransactor // Write-only binding to the contract
GenerateIdFilterer // Log filterer for contract events
}
// GenerateIdCaller is an auto generated read-only Go binding around an Ethereum contract.
type GenerateIdCaller struct {
contract *bind.BoundContract // Generic contract wrapper for the low level calls
}
// GenerateIdTransactor is an auto generated write-only Go binding around an Ethereum contract.
type GenerateIdTransactor struct {
contract *bind.BoundContract // Generic contract wrapper for the low level calls
}
// GenerateIdFilterer is an auto generated log filtering Go binding around an Ethereum contract events.
type GenerateIdFilterer struct {
contract *bind.BoundContract // Generic contract wrapper for the low level calls
}
// GenerateIdSession is an auto generated Go binding around an Ethereum contract,
// with pre-set call and transact options.
type GenerateIdSession struct {
Contract *GenerateId // Generic contract binding to set the session for
CallOpts bind.CallOpts // Call options to use throughout this session
TransactOpts bind.TransactOpts // Transaction auth options to use throughout this session
}
// GenerateIdCallerSession is an auto generated read-only Go binding around an Ethereum contract,
// with pre-set call options.
type GenerateIdCallerSession struct {
Contract *GenerateIdCaller // Generic contract caller binding to set the session for
CallOpts bind.CallOpts // Call options to use throughout this session
}
// GenerateIdTransactorSession is an auto generated write-only Go binding around an Ethereum contract,
// with pre-set transact options.
type GenerateIdTransactorSession struct {
Contract *GenerateIdTransactor // Generic contract transactor binding to set the session for
TransactOpts bind.TransactOpts // Transaction auth options to use throughout this session
}
// GenerateIdRaw is an auto generated low-level Go binding around an Ethereum contract.
type GenerateIdRaw struct {
Contract *GenerateId // Generic contract binding to access the raw methods on
}
// GenerateIdCallerRaw is an auto generated low-level read-only Go binding around an Ethereum contract.
type GenerateIdCallerRaw struct {
Contract *GenerateIdCaller // Generic read-only contract binding to access the raw methods on
}
// GenerateIdTransactorRaw is an auto generated low-level write-only Go binding around an Ethereum contract.
type GenerateIdTransactorRaw struct {
Contract *GenerateIdTransactor // Generic write-only contract binding to access the raw methods on
}
// NewGenerateId creates a new instance of GenerateId, bound to a specific deployed contract.
func NewGenerateId(address common.Address, backend bind.ContractBackend) (*GenerateId, error) {
contract, err := bindGenerateId(address, backend, backend, backend)
if err != nil {
return nil, err
}
return &GenerateId{GenerateIdCaller: GenerateIdCaller{contract: contract}, GenerateIdTransactor: GenerateIdTransactor{contract: contract}, GenerateIdFilterer: GenerateIdFilterer{contract: contract}}, nil
}
// NewGenerateIdCaller creates a new read-only instance of GenerateId, bound to a specific deployed contract.
func NewGenerateIdCaller(address common.Address, caller bind.ContractCaller) (*GenerateIdCaller, error) {
contract, err := bindGenerateId(address, caller, nil, nil)
if err != nil {
return nil, err
}
return &GenerateIdCaller{contract: contract}, nil
}
// NewGenerateIdTransactor creates a new write-only instance of GenerateId, bound to a specific deployed contract.
func NewGenerateIdTransactor(address common.Address, transactor bind.ContractTransactor) (*GenerateIdTransactor, error) {
contract, err := bindGenerateId(address, nil, transactor, nil)
if err != nil {
return nil, err
}
return &GenerateIdTransactor{contract: contract}, nil
}
// NewGenerateIdFilterer creates a new log filterer instance of GenerateId, bound to a specific deployed contract.
func NewGenerateIdFilterer(address common.Address, filterer bind.ContractFilterer) (*GenerateIdFilterer, error) {
contract, err := bindGenerateId(address, nil, nil, filterer)
if err != nil {
return nil, err
}
return &GenerateIdFilterer{contract: contract}, nil
}
// bindGenerateId binds a generic wrapper to an already deployed contract.
func bindGenerateId(address common.Address, caller bind.ContractCaller, transactor bind.ContractTransactor, filterer bind.ContractFilterer) (*bind.BoundContract, error) {
parsed, err := abi.JSON(strings.NewReader(GenerateIdABI))
if err != nil {
return nil, err
}
return bind.NewBoundContract(address, parsed, caller, transactor, filterer), nil
}
// Call invokes the (constant) contract method with params as input values and
// sets the output to result. The result type might be a single field for simple
// returns, a slice of interfaces for anonymous returns and a struct for named
// returns.
func (_GenerateId *GenerateIdRaw) Call(opts *bind.CallOpts, result interface{}, method string, params ...interf

博士僧小星
- 粉丝: 2402
- 资源: 5995
最新资源
- 不同颜色机器护垫检测27-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 西门子S7-1500博图程序 例程,大型生产线案例,程序涵盖有机器人块,汽缸块,电机块,伺服块,可调用,扫码块,可学习参考,快速提升技能 ,编程使用的语言有SCL,LD,STL,GRAPH 非常全
- java gis 开发中,点线面shp样例文件
- C++图书管理系统源代码(高分期末大作业项目)
- 安卓同步助手-1.apk
- GEATC 电脑 G5 型号官网驱动附件(win7/64位)
- LLC开关电源,60V5A半桥LLC串联谐振开关电源设计方案,提供原理图和PCB,BOM表,变压器制作说明书,配套半桥LLC电源软件 备注:原理图和PCB用AD软件打开
- Screenshot_20241229_173539_com.xunmeng.pinduoduo.jpg
- 2基于改进粒子群算法的微电网多目标优化调度 以微电网的运行成本、环境保护成本之和最小为目标,建立微电网环保与经济调度模型,并采用改进的PSO 算法对优化模型进行求解
- 基于ssm的超市进销存管理系统源码(java毕业设计完整源码+LW).zip
- 三菱PLC程序三菱Q系列案例三菱plc大型自动化程序生产线程序 规格如下: Q系列大型程序伺服12轴Q01U RS232通讯CCD 应用 实际使用中程序,详细中文注释 2个模块QD70P8,QD7
- 基于ssm的有机蔬菜商城源码(java毕业设计完整源码+LW).zip
- 不同颜色机器护垫检测47-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 基于ssm的游戏售卖网站源码(java毕业设计完整源码+LW).zip
- 基于ssm的企业仓储管理系统源码(java毕业设计完整源码+LW).zip
- 芝麻录屏 电脑高清录屏 会议录屏 直播录屏
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


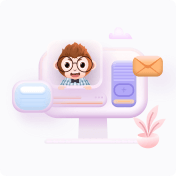