from __future__ import division
import numpy as np
import time
import random
import math
np.random.seed(1234)
'''
定义了架构的四个基本CLASS,分别是:V2Vchannels,V2Ichannels,Vehicle,Environ。
其中Environ的方法(即函数)最多,Vehicle没有函数只有几个属性,其余两者各有两个方法(分别是计算路损和阴影衰落)。
'''
class V2Vchannels:
# Simulator of the V2V Channels
# 内部参数:这里将bs和ms的高度设置为1.5m,阴影的std为3,都是来自TR36 885-A.1.4-1(规范);载波频率为2,单位为GHz;
def __init__(self):
self.t = 0
self.h_bs = 1.5
self.h_ms = 1.5
self.fc = 2
self.decorrelation_distance = 10
self.shadow_std = 3
# 计算路损
def get_path_loss(self, position_A, position_B):
d1 = abs(position_A[0] - position_B[0])
d2 = abs(position_A[1] - position_B[1])
d = math.hypot(d1, d2) + 0.001 # 返回欧几里德范数
d_bp = 4 * (self.h_bs - 1) * (self.h_ms - 1) * self.fc * (10 ** 9) / (3 * 10 ** 8)
def PL_Los(d):
if d <= 3:
return 22.7 * np.log10(3) + 41 + 20 * np.log10(self.fc / 5)
else:
if d < d_bp:
return 22.7 * np.log10(d) + 41 + 20 * np.log10(self.fc / 5)
else:
return 40.0 * np.log10(d) + 9.45 - 17.3 * np.log10(self.h_bs) - 17.3 * np.log10(self.h_ms) + 2.7 * np.log10(self.fc / 5)
def PL_NLos(d_a, d_b):
n_j = max(2.8 - 0.0024 * d_b, 1.84)
return PL_Los(d_a) + 20 - 12.5 * n_j + 10 * n_j * np.log10(d_b) + 3 * np.log10(self.fc / 5)
if min(d1, d2) < 7:
PL = PL_Los(d)
else:
PL = min(PL_NLos(d1, d2), PL_NLos(d2, d1))
return PL # + self.shadow_std * np.random.normal()
# 更新阴影衰落 # 这个更新公式是出自文献[1]-A-1.4 Channel model表格后的部分
def get_shadowing(self, delta_distance, shadowing):
return np.exp(-1 * (delta_distance / self.decorrelation_distance)) * shadowing \
+ math.sqrt(1 - np.exp(-2 * (delta_distance / self.decorrelation_distance))) * np.random.normal(0, 3) # standard dev is 3 db
# 包含的两个方法和V2V相同,但是计算路损的时候不再区分Los了
# 两个方法均是文献[1]-Table A.1.4-2的内容和其后的说明
class V2Ichannels:
# Simulator of the V2I channels
def __init__(self):
self.h_bs = 25
self.h_ms = 1.5
self.Decorrelation_distance = 50
self.BS_position = [750 / 2, 1299 / 2] # center of the grids
self.shadow_std = 8
def get_path_loss(self, position_A):
d1 = abs(position_A[0] - self.BS_position[0])
d2 = abs(position_A[1] - self.BS_position[1])
distance = math.hypot(d1, d2)
return 128.1 + 37.6 * np.log10(math.sqrt(distance ** 2 + (self.h_bs - self.h_ms) ** 2) / 1000) # + self.shadow_std * np.random.normal()
def get_shadowing(self, delta_distance, shadowing):
nVeh = len(shadowing)
self.R = np.sqrt(0.5 * np.ones([nVeh, nVeh]) + 0.5 * np.identity(nVeh))
return np.multiply(np.exp(-1 * (delta_distance / self.Decorrelation_distance)), shadowing) \
+ np.sqrt(1 - np.exp(-2 * (delta_distance / self.Decorrelation_distance))) * np.random.normal(0, 8, nVeh)
class Vehicle:
# Vehicle simulator: include all the information for a vehicle
# 初始化时需要传入三个参数:起始位置、起始方向、速度。
# 函数内部将自己定义两个list:neighbors、destinations,分别存放邻居和V2V的通信端(这里两者在数值上相同,因为设定V2V的对象即为邻居)
def __init__(self, start_position, start_direction, velocity):
self.position = start_position
self.direction = start_direction
self.velocity = velocity
self.neighbors = []
self.destinations = []
class Environ:
# 初始化需要传入4个list(为上下左右路口的位置数据):down_lane, up_lane, left_lane, right_lane;地图的宽和高;
# 车辆数和邻居数。除以上所提外,内部含有好多参数,如下:
def __init__(self, n_veh, n_neighbor):
# ################## SETTINGS ######################
# 参数初始化:这部分直接写在代码中,没有函数,大概包括:地图属性(路口坐标,整体地图尺寸)、#车、#邻居、#RB、#episode,一些算法参数
# 对于地图参数 up_lanes / down_lanes / left_lanes / right_lanes 的含义,首先要了解本次所用的系统模型由3GPP TR 36.885的城市案例
# 给出,每条街有四个车道(正反方向各两个车道) ,车道宽3.5m,模型网格(road grid)的尺寸以黄线之间的距离确定,为433m*250m,
# 区域面积为1299m*750m。仿真中等比例缩小为原来的1/2(这点可以由 width 和 height 参数是 / 2 的看出来),
# 反映在车道的参数上就是在 lanes 中的 i / 2.0 。
'''
下面以 up_lanes 为例进行说明。在上图中我们可以看到,车道宽3.5m,所以将车视作质点的话,应该是在3.5m的车道中间移动的,
因此在 up_lanes 中 in 后面的 中括号里 3.5 需要 /2,第二项的3.5就是通向双车道的第二条车道的中间;
第三项 +250 就是越过建筑物的第一条同向车道,以此类推
'''
up_lanes = [i / 2.0 for i in
[3.5 / 2, 3.5 / 2 + 3.5, 250 + 3.5 / 2, 250 + 3.5 + 3.5 / 2, 500 + 3.5 / 2, 500 + 3.5 + 3.5 / 2]]
down_lanes = [i / 2.0 for i in
[250 - 3.5 - 3.5 / 2, 250 - 3.5 / 2, 500 - 3.5 - 3.5 / 2, 500 - 3.5 / 2, 750 - 3.5 - 3.5 / 2,
750 - 3.5 / 2]]
left_lanes = [i / 2.0 for i in
[3.5 / 2, 3.5 / 2 + 3.5, 433 + 3.5 / 2, 433 + 3.5 + 3.5 / 2, 866 + 3.5 / 2, 866 + 3.5 + 3.5 / 2]]
right_lanes = [i / 2.0 for i in
[433 - 3.5 - 3.5 / 2, 433 - 3.5 / 2, 866 - 3.5 - 3.5 / 2, 866 - 3.5 / 2, 1299 - 3.5 - 3.5 / 2,
1299 - 3.5 / 2]]
width = 750 / 2
height = 1298 / 2
self.down_lanes = down_lanes
self.up_lanes = up_lanes
self.left_lanes = left_lanes
self.right_lanes = right_lanes
self.width = width
self.height = height
self.V2Vchannels = V2Vchannels()
self.V2Ichannels = V2Ichannels()
self.vehicles = []
self.demand = []
self.V2V_Shadowing = []
self.V2I_Shadowing = []
self.delta_distance = []
self.V2V_channels_abs = []
self.V2I_channels_abs = []
self.V2I_power_dB = 23 # 23dBm = 0.199W
# self.V2V_power_dB_List = [23, 15, 5, -100] # the power levels.23dBm=0.199W;15dBm=0.03W;5dBm=0.003W;-100dBm=0.1pW
#self.V2V_power_dB_List = [23, 20, 17, 14, 11, 8, 5, -100] # the power levels.23dBm=0.199W;15dBm=0.03W;5dBm=0.003W;-100dBm=0.1pW
self.sig2_dB = -114
self.bsAntGain = 8 # 基站单天线增益
self.bsNoiseFigure = 5 # 基站噪声系数
self.vehAntGain = 3 # 车辆单天线增益
self.vehNoiseFigure = 9
self.sig2 = 10 ** (self.sig2_dB / 10) # dB转化成mW单位
self.n_RB = n_veh # 正交频带数目
self.n_Veh = n_veh
self.n_neighbor = n_neighbor
self.time_fast = 0.001
self.time_slow = 0.1 # update slow fading/vehicle position every 100 ms
self.bandwidth = int(1e6) # bandwidth per RB, 1 MHz
self.demand_size = int((4 * 190 + 300) * 8 * 1) # V2V payload: 2 * 1060 Bytes every 100 ms
self.V2V_Interference_all = np.zeros((self.n_Veh, self.n_neighbor, self.n_RB)) + self.sig2 # 定义RB为频域上连续的12个子载波
# 添加车:有两个方法�

博士僧小星
- 粉丝: 2437
- 资源: 5998
最新资源
- 负载转矩观测 永磁同步电机FOC 1.采用一种简单新颖的负载转矩观测器,相比传统的龙伯格等方法观测器设计巧妙,参数调节容易,观测负载转矩快速准确;赠送龙伯格负载转矩观测器用于对比分析 2.将观测到的
- 三相电机容错控制,采用电流预测算法
- 光伏微网储能,实现电池充放电双向流动 双闭环控制策略,电压外环滑膜控制,可以替为pi控制,以及模糊pi控制策略
- FPGA设计的代码 对周期信号进行处理 两个版本的现成代码
- 基于西门子s7-200,基于西门子200的智能停车场监控系统,程序带有计时收费功能,仿真采用MCGS制作,基于plc智能停车场车位控制仿真 功能介绍: ①假设某停车场共有20个车位 ②在停车场入口处
- 欧姆龙PLC项目程序NJ系列模切机程序 1、12轴EtherCAT总线伺服运动控制,包含回零、点动、定位、速度控制 2、张力控制PID算法,收放卷径计算, 3、隔膜自动纠偏控制,模拟量数据平均
- 分布式电源优化配置 33节点 编程方法:采用matlab+yalmip编程,cplex或gurobi作为求解器 主要内容:以33节点为例,考虑配电网二阶锥模型,运行主体包括光伏、微燃机以及负荷,创新
- 适用于Matlab2019a和b版本 速度环模块儿分别用PI和MTPA控制策略 基于MRAS(模型参考自适应法)的无位置传感器控制系统设计
- Matlab simulink 风电调频,四机两区系统 突增负荷扰动,风电采用超速减载控制,惯性控制 下垂控制 仿真速度快,只需要20秒 比其他链接的仿真速度都要快 其他链接一般为离散模型
- BLDC转速控制仿真,BLDC双环控制,无刷直流电机速度控制仿真 外环转速PI控制,内环电流滞环控制或bang-bang控制,带霍尔传感器,采用六步相法驱动 只有仿真,没有代码
- 粒子群算法模版,有一维信号和2维信号的Matlab代码模板
- 基于空间矢量控制的永磁同步电机状态反馈控制转速系统设计及仿真,仿真平台基于MATLAB Simulink搭建 联系默认发仿真系统文件
- 元胞自动机模拟动态再结晶,CA法模拟动态再结晶程序,材料参数可调主 模型适用于金属材料,链接展示的是再结晶形核和晶粒长大,程序模型可改动,如位错模型,形核模型包括形核机制等 程序matlab编写
- 永磁同步风力发电机的matlab仿真模型 风力机控制采用最优叶尖速比控制 机侧为基于pi控制的双闭环控制(转速外环、电流内环) 网侧为基于pi控制的双闭环控制(电压外环、电流内环)
- 1.小波图像分解重构代码matlab 2.nlm算法图像去噪Matlab代码 3.中值滤波图像去噪Matlab代码 4.DNCNN图像去噪Matlab代码 5.BM3D图像去噪Matlab代码 6.均
- 双馈永磁风电机组并网仿真短路故障模型,kw级别永磁同步机PMSG并网仿真模型,机端由6台1.5MW双馈风机构成9MW风电场,风电场容量可调,出口电压690v,经升压变压器及线路阻抗连接至120kv交流
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


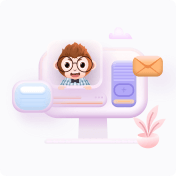
- 1
- 2
前往页